Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
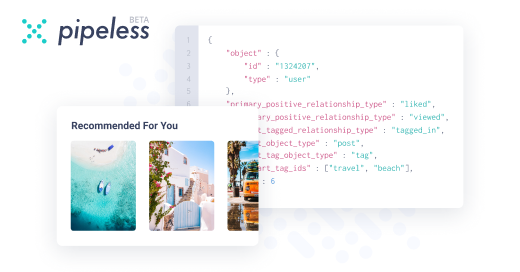
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "shoot me for bad code"
-
Okay, story time.
Back during 2016, I decided to do a little experiment to test the viability of multithreading in a JavaScript server stack, and I'm not talking about the Node.js way of queuing I/O on background threads, or about WebWorkers that box and convert your arguments to JSON and back during a simple call across two JS contexts.
I'm talking about JavaScript code running concurrently on all cores. I'm talking about replacing the god-awful single-threaded event loop of ECMAScript – the biggest bottleneck in software history – with an honest-to-god, lock-free thread-pool scheduler that executes JS code in parallel, on all cores.
I'm talking about concurrent access to shared mutable state – a big, rightfully-hated mess when done badly – in JavaScript.
This rant is about the many mistakes I made at the time, specifically the biggest – but not the first – of which: publishing some preliminary results very early on.
Every time I showed my work to a JavaScript developer, I'd get negative feedback. Like, unjustified hatred and immediate denial, or outright rejection of the entire concept. Some were even adamantly trying to discourage me from this project.
So I posted a sarcastic question to the Software Engineering Stack Exchange, which was originally worded differently to reflect my frustration, but was later edited by mods to be more serious.
You can see the responses for yourself here: https://goo.gl/poHKpK
Most of the serious answers were along the lines of "multithreading is hard". The top voted response started with this statement: "1) Multithreading is extremely hard, and unfortunately the way you've presented this idea so far implies you're severely underestimating how hard it is."
While I'll admit that my presentation was initially lacking, I later made an entire page to explain the synchronisation mechanism in place, and you can read more about it here, if you're interested:
http://nexusjs.com/architecture/
But what really shocked me was that I had never understood the mindset that all the naysayers adopted until I read that response.
Because the bottom-line of that entire response is an argument: an argument against change.
The average JavaScript developer doesn't want a multithreaded server platform for JavaScript because it means a change of the status quo.
And this is exactly why I started this project. I wanted a highly performant JavaScript platform for servers that's more suitable for real-time applications like transcoding, video streaming, and machine learning.
Nexus does not and will not hold your hand. It will not repeat Node's mistakes and give you nice ways to shoot yourself in the foot later, like `process.on('uncaughtException', ...)` for a catch-all global error handling solution.
No, an uncaught exception will be dealt with like any other self-respecting language: by not ignoring the problem and pretending it doesn't exist. If you write bad code, your program will crash, and you can't rectify a bug in your code by ignoring its presence entirely and using duct tape to scrape something together.
Back on the topic of multithreading, though. Multithreading is known to be hard, that's true. But how do you deal with a difficult solution? You simplify it and break it down, not just disregard it completely; because multithreading has its great advantages, too.
Like, how about we talk performance?
How about distributed algorithms that don't waste 40% of their computing power on agent communication and pointless overhead (like the serialisation/deserialisation of messages across the execution boundary for every single call)?
How about vertical scaling without forking the entire address space (and thus multiplying your application's memory consumption by the number of cores you wish to use)?
How about utilising logical CPUs to the fullest extent, and allowing them to execute JavaScript? Something that isn't even possible with the current model implemented by Node?
Some will say that the performance gains aren't worth the risk. That the possibility of race conditions and deadlocks aren't worth it.
That's the point of cooperative multithreading. It is a way to smartly work around these issues.
If you use promises, they will execute in parallel, to the best of the scheduler's abilities, and if you chain them then they will run consecutively as planned according to their dependency graph.
If your code doesn't access global variables or shared closure variables, or your promises only deal with their provided inputs without side-effects, then no contention will *ever* occur.
If you only read and never modify globals, no contention will ever occur.
Are you seeing the same trend I'm seeing?
Good JavaScript programming practices miraculously coincide with the best practices of thread-safety.
When someone says we shouldn't use multithreading because it's hard, do you know what I like to say to that?
"To multithread, you need a pair."18 -
Am i whiny or is resilience so glorified in this field?
I am a junior developer. I was assigned with two projects together with a friend and a senior. My friend and I finished our assigned tasks way before the deadline. Fast forward, my senior got reassigned to a different project since we are lacking with manpower. Naturally, his transactions were assigned to me and my friend. And my goodness, his existing codes are a piece of shit! It's all over the place. His variable naming is shit, his codes are all around the place, his codes doesn't even follow our company's coding standards, no try catch, a lot of unsafe practices. In short, cleaning his code is a pain in the ass and my friend and I got really busy with cleaning his mess. The testing of our system is really near but I just thought that maybe he's really busy with the other project that's why the quality of his codes deteriorated.
He's not. One day, I saw his in discord that he's playing during work hours lol. And the worse part is that he is playing with our boss! YES. DURING WORK HOURS. I got mad but I couldn't say anything because he is really tight with the boss.
Later on that day, we had our meeting. I was surprised when my boss told me that she's expecting that the excel part of our system is already finished. A little background here, my boss asked me to study Excel VB. However, I didnt get to study that much because I was so busy fixing bugs and after that came the cleaning of our senior's shit codes.
So I tried to say these things to my boss but I was cut out by the same senior shouting "You can do it!" over and over again. No one listened to what I was trying to say! And to make it even worse, the boss had a very proud look on her face and she even had the audacity to tell me that I'm lucky I have such a good support system. I dont.
Now, the company is planning to put me in a very demanding project. I havent finished cleaning up my senior's codes, I havent started anything with the excel and the deadline is next week!
The boss told me that even if I enter the other project, that I will still be responsible for the Excel part of our system. So fucking shoot me in the face.They were telling me that I should have a good time management system, that I should be flexible, that I should adapt easily, yada yada yada. She just makes you feel bad about yourself if you're not as 'flexible' as her.
The thing is, even if I have the best time management techniques in the world, if you bombard me with a shitload of tasks, then I won't be able to do it properly! I don't even take breaks anymore! I work literally 8 hours a day, even more than that. And I dont understand, why the hell is she overworking me when her friend (the senior dev) is just playing during work hours?
Another funniest thing is that she told us that when we encounter technical problems, we should ask our senior dev. Oh boy, if only she knows how shitty his codes are.6 -
I learned very quickly that magic globals are baaaaad.
I mean, it's easier than using a queue for passing data between threads, right? -
Hi Devrant!
I've been doing mainly bugfixes for about 3 weeks now, and to keep myself sane, I have re-written Queens - Another one bites the dust, to fit my work. I hope you enjoy it too.
Let's go!
Dev walks warily down the street
With his brim pulled way down low
Ain't no sound but the sound of his keys
Keyboards ready to go
Are you ready, hey, are you ready for this?
Are you hanging on the edge of your seat?
Out of the hands the code rips
To the sound of the beat
Another bug bites the dust
Another bug bites the dust
And another bug gone, and another bug gone
Another bug bites the dust
Hey, I'm gonna get you too
Another bug bites the dust
How do you think I'm going to get along
Without you when you're gone?
You took me for everything that I had
And kicked me out on my own
Are you happy, are you satisfied?
How long can you stand the heat?
Out of the hands the code rips
To the sound of the beat, look out
Another bug bites the dust
Another bug bites the dust
And another bug gone, and another bug gone
Another bug bites the dust
Hey, I'm gonna get you too
Another bug bites the dust
Hey, another bug bites the dust
Another bug bites the dust
Another bug bites the dust
Another bug bites the dust
Shoot out
There are plenty of ways that you can hurt a man
And bring him to the ground
You can beat him, you can cheat him
You can treat him bad and leave him
When he's down
But I'm ready, yes, I'm ready for you
I'm standing on my own two feet
Out of the hands the code rips
Repeating to the sound of the beat
Another bug bites the dust
Another bug bites the dust
And another bug gone, and another bug gone
Another bug bites the dust
Hey, I'm gonna get you too
Another bug bites the dust
Shoot out4 -
I understand the reasoning behind switching to a new, maybe better, technology, but for fuck sake, it’s against typical Microsoft strategy to “kill and shoot to the dead corpse” something instead of maintaining backward compatibility. Why they’ve changed?
I still can develop VB6 software for Windows 11 that just works. But you removing newer tools for no reason.
In short: Xamarin is dead, and that’s alright, but they are even deciding to “remove” development tools from future updates of VS 2022. Why?? Keep it optional, allow me to write legacy code (just 4 years old actually) a bit longer. 🙃
And also, .NET MAUI doesn’t seem “great”, at least at the first sight.
Why you’re forcing me to switch to it if there are 0 benefit for my product?
It’s so bad the only way to bring developers is this one?!
What is incredible to me is that the “industry field”, which is HUGE is so often ignored because of the “customers field”. Keep them separated. If you don’t want to support old tools, just don’t, but leave them there.
They killed Windows Mobile 6.5 which was old but still alive and fine in the industries, you had the biggest market share in PDAs and decided to give it to fucking Google.
The manufacturers kept selling WM devices even in 2020… and they stopped just because you stopped selling licenses.
You acquired Xamarin, gave everyone for free the tools to keep writing .NET for Android and move the industry apps, and now you are saying “actually fuck you, do it again, even though nothing really changes, but convert your entire project to this bs we’ve created”. Why???
Microsoft response: it’s just a few clicks and everything works fine.
My response: No, it’s not… the entire UI is rendered in a different way, I have to rewrite the whole UI of my app and a lot of modules stopped working because of nuget packages I can’t install anymore…
I have to spend additional time to make it work THE SAME as before, not better. So what’s the fucking point?17