Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
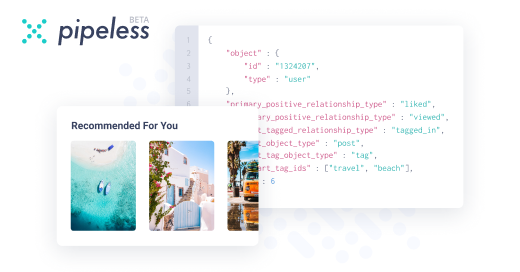
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "string literals"
-
What the cinnamon toast fuck is this?!
This dude combined template literals and the good ol' fashion string concatenate method. But whhyyy8 -
Sharing a first look at a prototype Web Components library I am working on for "fun"
TL;DR left side is pivot (grouped) table, right side is declarative code for it (Everything except the custom formatting is done declaratively, but has the option to be imperative as well).
====
TL;DR (Too long, did read):
I'm challenging myself to be creative with the cool new things that browsers offer us. Lani so far has a focus on extreme extensibility, abstraction from dependencies, and optional declarative style.
It's also going to be a micro CSS framework, but that's taking the back-seat.
I wanted to highlight my design here with this table, and the code that is written to produce this result.
First, you can see that the <lani-table> element is reading template, data, and layout information from its child elements. Besides the custom highlighting code (Yellow background in the "Tags" column, and green gradient in the "Score" column), everything can be done without opening even a single script tag.
The <lani-data-source> element is rather special. It's an abstraction of any data source, and you, as a developer can add custom data sources and hook up the handlers to your whim (the element itself uses the "type" attribute to choose a handler. In this case, the handler is "download" which simply sends a fetch request to the server once and downloads the result to memory).
Templates are stored in an html file, not string literals (Which I think really fucks the code) and loaded async, then cached into an object (so that the network tab doesn't get crowded, even if we can count on the HTTP cache). This also has the benefit of allowing me to parse the HTML templates once and then caching the parsed result in memory, so templates are never re-parsed from string no matter how many custom elements are created.
Everything is "compiled" into a single, minified .js file that you include on your page.
I know it's nothing extraordinary, but for something that doesn't need to be compiled, transpiled, packaged, shipped, and kissed goodnight, I think it's a really nice design and I hope to continue work on it and improve it over time1 -
So, in C#, are there any tips or guidelines as to how to write "clean" multiline strings? I mean, imo it doesn't look as neat when the code looks like:
static string kindOfLongVariableName = @"First line of string.
Second line of string...";
With the first line sort of hovering on the side. What I'm used to is with Python where you can just:
variable_name = """'\
First line.
Second line.
"""
And use the '\' to escape the newline, but that obviously doesn't work in C#. Can anyone point me in a direction to start looking? The docs are a bit confusing and not very beginner friendly. :/20 -
Have some downtime today, so since I lucked out and found some old backups (from before I used Git) of a project I was planning on revisiting, I decided to fire it up and see what I can do to get that going again.
And discovering just how much my coding style has changed since then...
[Code is in PHP, for reference]
* Virtually no documentation (whereas my current style is near-obsessive with PHPdoc blocks)
* Where comments exist, they only use // and are a full tab after the end of the line
* All assignment operators are dutifully aligned on tabs
* Have to update the entire codebase because it relies on deprecated `mysql_*` calls
* Have to flip all of the quotes throughout the codebase because I used double-quotes as my primary at the time instead of single quotes.
* Also relied on magic quotes for injecting variable content into strings
* Associative array practices varied; sometimes the names are encased in double quotes, but I just hit a block where it's all leaving it to the compiler to interpret unquoted string literals
And perhaps the most egregious so far...
* Any time we get database results back the process for cycling through them is to do `$count = mysql_num_rows($result);` (or $count2, etc.), then do a `for ($i = 0; $i < $count; $i++)` (again, or $j, $k, etc.), instead of just a simple `while ($data = $result->fetch_assoc())`2 -
Where do I start...
I have seen a QA load local code to a machine, run it and then say it was ready to deploy. Little did we know she wasn’t following the deployment process at all and didn’t even realize she had to. We were a week trying to figure out why the deploys wouldn’t work until she spoke up.
I knew a dev/founder that said to me “source control is only for large projects”, I tried to convince him and his cofounder to use github or bitbucket. Nope, they weren’t into it (fresh out of school listening to professors who hadn’t worked a development day in 20 years) One cofounder got disgruntled, thought he was doing most of the work and decided to quit, he also decided to wipe the code off his co-founders machine. I literally saw a grown man come out of a meeting crying knowing he would never gain back the respect of those mentors and advisors.
I once saw a developer create a printed ticket receipt for a web app. Instead of making a page and styling it to fit a smaller width, he decided to do everything in string literals. More precisely, he made one big long fucking strong literal and then broke it up using custom regex to add styling to different sections. We had a meeting and he was totally convinced this was the only way. In the end we scrapped the entire code and the dude didn’t last very long after that.
Worst of all! I once saw a developer find a IBM Model M keyboard and said “I’m gonna throw out this junky keyboard”. I told him to shut his stupid fucking mouth and give the the keyboard.
He did -
I use the ICU format often for translation because it's simple enough and supported on many platforms. It's something of a standard so I can use the same translation string format and similar library functions everywhere.
ICU is like a really simple templating language, somewhere between printf and something like smarty or twig simplified and specifically intended for internationalisation.
I updated a library providing ICU compatible parsing and formatting for one of the platforms I'm using and find tests break. I assume that only thing to change is the API. ICU very rarely changes and if it did it would be unexpected for it to break the syntax in a major way without big news of a new syntax.
The main contributor of the library has changed since some time last year. Someone else picked up the project from previous contributors.
Though the library is heavily advertised as using ICU it has now switched to using a custom extended format that's not fully compatible and that is being driven by use case demand rather than standardisation.
Seems like a nice chap but has also decided for a major paradigm shift for the library.
The ICU format only parses ICU templates for string substitution and formatting. The new format tries to parse anything that looks XML like as well but with much more strict rules only supporting a tiny subset of XML and failing to preserve what would otherwise be string literals.
Has anyone else seen this happen after the handover of an opensource library where the paradigm shifts?3 -
Instead of adopting the ` character to represent multi-line string literals like JavaScript, Swift opts for three consecutive double-quotes. """ smh4