Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
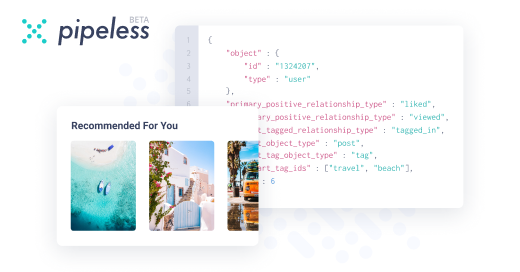
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "partial application"
-
I'm editing the sidebar on one of our websites, and shuffling some entries. It involves moving some entries in/out of a dropdown and contextual sidebars, in/out of submenus, etc. It sounds a little tedious but overall pretty trivial, right?
This is day three.
I learned React+Redux from scratch (and rebuilt the latter for fun) in twice that long.
In my defense, I've been working on other tasks (see: Alerts), but mostly because I'd rather gouge my freaking eyes out than continue on this one.
Everything that could be wrong about this is. Everything that could be over-engineered is. Everything that could be written worse... can't, actually; it's awful.
Major grievances:
1) The sidebars (yes, there are several) are spread across a ridiculous number of folders. I stopped counting at 20.
2) Instead of icon fonts, this uses multiple images for entry states.
3) The image filenames don't match the menu entry names. at all. ("sb_gifts.png" -> orders); active filenames are e.g. "sb_giftsactive.png"
4) The actions don't match the menu entry names.
5) Menu state is handled within the root application controller, and doesn't use bools, but strings. (and these state flags never seem to get reset anywhere...)
6) These strings are used to construct the image filenames within the sidebar views/partials.
7) Sometimes access restrictions (employee, manager, etc.) are around the individual menu entries, sometimes they're around a partial include, meaning it's extremely difficult to determine which menu entries/sections/subsections are permission-locked without digging through everything.
8) Within different conditionals there are duplicate blocks markup, with duplicate includes, that end up render different partials/markup due to different state.
9) There are parent tags outside of includes, such as `<ul>#{render 'horrific-eye-stabbing'}</ul>`
10) The markup differs per location: sometimes it's a huge blob of non-semantic filthiness, sometimes it's a simple div+span. Example filth: section->p->a->(img,span) ... per menu entry.
11) In some places, the markup is broken, e.g. `<li><u>...</li></u>`
12) In other places, markup is used for layout adjustments, such as an single nested within several divs adorned with lots of styles/classes.
13) Per-device layouts are handled, not within separate views, but by conditionally enabling/disabling swaths of markup, e.g. (if is_cordova_session?).
14) `is_cordova_session` in particular is stored within a cookie that does not expire, and within your user session. disabling it is annoying and very non-obvious. It can get set whether or not you're using cordova.
15) There are virtually no stylesheets; almost everything is inline (but of course not actually everything), which makes for fun layout debugging.
16) Some of the markup (with inline styling, no less) is generated within a goddamn controller.
17) The markup does use css classes, but it's predominately not for actual styling: they're used to pick out elements within unit tests. An example class name: "hide-for-medium-down"; and no, I can't figure out what it means, even when looking at the tests that use it. There are no styles attached to that particular class.
18) The tests have not been updated for three years, and that last update was an rspec version bump.
19) Mixed tabs and spaces, with mixed indentation level (given spaces, it's sometimes 2, 4, 4, 5, or 6, and sometimes one of those levels consistently, plus an extra space thereafter.)
20) Intentional assignment within conditionals (`if var=possibly_nil_return_value()`)
21) hardcoded (and occasionally incorrect) values/urls.
... and last but not least:
22) Adding a new "menu sections unit" (I still haven't determined what the crap that means) requires changing two constants and writing a goddamn database migration.
I'm not even including minor annoyances like non-enclosed ternaries, poor naming conventions, commented out code, highly inefficient code, a 512-character regex (at least it's even, right?), etc.
just.
what the _fuck_
Who knew a sidebar could be so utterly convoluted?6 -
I've optimised so many things in my time I can't remember most of them.
Most recently, something had to be the equivalent off `"literal" LIKE column` with a million rows to compare. It would take around a second average each literal to lookup for a service that needs to be high load and low latency. This isn't an easy case to optimise, many people would consider it impossible.
It took my a couple of hours to reverse engineer the data and implement a few hundred line implementation that would look it up in 1ms average with the worst possible case being very rare and not too distant from this.
In another case there was a lookup of arbitrary time spans that most people would not bother to cache because the input parameters are too short lived and variable to make a difference. I replaced the 50000+ line application acting as a middle man between the application and database with 500 lines of code that did the look up faster and was able to implement a reasonable caching strategy. This dropped resource consumption by a minimum of factor of ten at least. Misses were cheaper and it was able to cache most cases. It also involved modifying the client library in C to stop it unnecessarily wrapping primitives in objects to the high level language which was causing it to consume excessive amounts of memory when processing huge data streams.
Another system would download a huge data set for every point of sale constantly, then parse and apply it. It had to reflect changes quickly but would download the whole dataset each time containing hundreds of thousands of rows. I whipped up a system so that a single server (barring redundancy) would download it in a loop, parse it using C which was much faster than the traditional interpreted language, then use a custom data differential format, TCP data streaming protocol, binary serialisation and LZMA compression to pipe it down to points of sale. This protocol also used versioning for catchup and differential combination for additional reduction in size. It went from being 30 seconds to a few minutes behind to using able to keep up to with in a second of changes. It was also using so much bandwidth that it would reach the limit on ADSL connections then get throttled. I looked at the traffic stats after and it dropped from dozens of terabytes a month to around a gigabyte or so a month for several hundred machines. The drop in the graphs you'd think all the machines had been turned off as that's what it looked like. It could now happily run over GPRS or 56K.
I was working on a project with a lot of data and noticed these huge tables and horrible queries. The tables were all the results of queries. Someone wrote terrible SQL then to optimise it ran it in the background with all possible variable values then store the results of joins and aggregates into new tables. On top of those tables they wrote more SQL. I wrote some new queries and query generation that wiped out thousands of lines of code immediately and operated on the original tables taking things down from 30GB and rapidly climbing to a couple GB.
Another time a piece of mathematics had to generate all possible permutations and the existing solution was factorial. I worked out how to optimise it to run n*n which believe it or not made the world of difference. Went from hardly handling anything to handling anything thrown at it. It was nice trying to get people to "freeze the system now".
I build my own frontend systems (admittedly rushed) that do what angular/react/vue aim for but with higher (maximum) performance including an in memory data base to back the UI that had layered event driven indexes and could handle referential integrity (overlay on the database only revealing items with valid integrity) or reordering and reposition events very rapidly using a custom AVL tree. You could layer indexes over it (data inheritance) that could be partial and dynamic.
So many times have I optimised things on automatic just cleaning up code normally. Hundreds, thousands of optimisations. It's what makes my clock tick.4 -
So I wrote an application that loads data from a 3rd party API. It allows the user to enter a record locator number and pull it up. By design, the value can be a partial match and it will pull up the record still.
The first API call I make only took 2-3 seconds, so I didn't see an issue as it's loading most of the data the app needs. I keep the filters/fields as they are and move on.
Fast forward 6 months. The user is complaining that the records are taking 30-45 seconds to load. Sure enough, load times are terrible. I've made lots of changes to what fields I'm loading through the API, and I'm calling several additional APIs, so I start pulling pieces of code out to see if anything improves. They all barely make any difference--still 30+ second load times. I end up removing everything except the first API call I developed that was taking 2-3 seconds before. Still taking 30+ seconds.
The 3rd party API allows you to filter using "starts with" or "contains". I used "contains" initially and had no issue, but I decided to try "starts with" since it should fit most use cases.
Load time is less than one second. I add back everything else. Load time is just over a second.
It seems that the 3rd party updated the API and multiplied load times by 10 when using that particular filter. I spent almost an hour on this since the platform doesn't support performance or debugging tools very well, and it all came down to a one line fix.4 -
!dev
Whoever the fuck wit coded the entire system for the university/college application information portal over here in my country needs to be hung, shot, hung again and shot.
It's **ABSOLUTE FUCKING GARBAGE** on the design. First we have the search box. It literally takes a good 20 seconds to query 1000 entries at low traffic and 3 MINUTES at high traffic. Bad enough? Because it would also take that long to give you a table of search result which is, I shit you not, identical to the drop-down results you get while typing except rendered inside a <table></table> with some overlay!
Oh, did I mention it didn't have partial match? Yea, IT DIDN'T. For example, "John Hurr Doe City" would not match "John Hurr Doe city" just to piss you off. And then we have the fuckers that do this:
- University A John Hurr Doe city
- University B JHD City
- University C JHD city
That and no partial match. Yea. It's BS.
Also. if you wanna search again after view a school, you have to press "Back", the physical "Back" of the browser. Fair, it's good, but if you press anything other than that button, welll, you're fucked although lightly.
The cherry on top of the rant cone? The whole thing is made by the studentfucking Ministry of Education and Training, the mother of overlord of students. Yea. The fucking Ministry itself. Really. You wanna go "catch up with the world and master the 4.0 Industrial Revolution" and yet you can't fucking code the site properly. Fuck you, fuck you, fuck your horse you're riding and probably fuck you as well.
Sorry for getting slightly political at the end, the damn page is getting on my nerve. -
Back home from vacations tomorrow.
It wasn't the best time I had but the thought of returning to daily life is already giving me a stomach ache.
Gotta take care of my little pug too, my anxiety about his partial eye keratosis isn't doing great too. Since the caretakers don't apply eye medication regularly.
There's this fear of my productivity before uni begins, I really don't want my vacation to end with me returning without completing my application.
I've still got a lot to do, anyone want to partner up with me ? I've still got load balancing and failover mechanisms which I have no real-time experience with (excluding api related stuff). I've got a general idea to use nginx. -
3 hours in to work and I've already wished Java had two more features: native tuples and partial application in method references.5
-
Sometimes I think that TypeScript is like a poor developer's Haskell. I thought "Isn't this supposed to support functional programming?" and searched for a way to do currying or partial application, and only found hackish solutions :/ Then again, maybe I don't know Haskell well enough to make a proper judgment.1
-
F# is making me start to dislike C#undefined nullreferencemyarse shame there's no boilerplate pattern matching immutability partial application currying1
-
I've been sitting here staring at extension types and I wonder, what if I had a partial file with partial data ?
In general one could say that in every case where say a header is missing that is ALWAYS going to have some identifying characteristics even given a characteristic statistically frequent pattern of data, that there is always a null value that appears as total chaos.
But I wonder, is there a way beyond simply trying every goddamn possible combination of things until meaningful data is extracted to identify a file by its content when part of that content that is usually used for such a purpose, is missing ?
What kind of application or technology would be required for this ? Certainly not neural networks, but obviously some kind of ai right ?10