Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
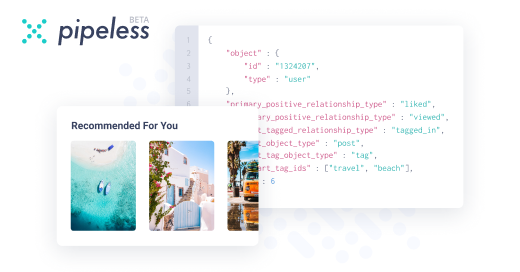
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "immutability"
-
"I'm almost done, I'll just need to add tests!"
Booom! You did it, that was a nuke going off in my head.
No, you shouldn't just need to add tests. The tests should have been written from the get go! You most likely won't cover all the cases. You won't know if adding the tests will break your feature, as you had none, as you refactor your untested mess in order to make your code testable.
When reading your mess of a test case and the painful mocking process you went through, I silently cry out into the void: "Why oh why!? All of this suffering could have been avoided!"
Since most of the time, your mocking pain boils down to not understanding what your "unit" in your "unit test" should be.
So let it be said:
- If you want to build a parser for an XML file, then just write a function / class whose *only* purpose is: parse the XML file, return a value object. That's it. Nothing more, nothing less.
- If you want to build a parser for an XML file, it MUST NOT: download a zip, extract that zip, merge all those files to one big file, parse that big file, talk to some other random APIs as a side-effect, and then return a value object.
Because then you suddenly have to mock away a http service and deal with zip files in your test cases.
The http util of your programming language will most likely work. Your unzip library will most likely work. So just assume it working. There are valid use cases where you want to make sure you acutally send a request and get a response, yet I am talking unit test here only.
In the scope of a class, keep the public methods to a reasonable minimum. As for each public method you shall at least create one test case. If you ever have the feeling "I want to test that private method" replace that statement in your head with: "I should extract that functionality to a new class where that method public. I then can create a unit test case a for that." That new service then becomes a dependency in your current service. Problem solved.
Also, mocking away dependencies should a simple process. If your mocking process fills half the screen, your test setup is overly complicated and your class is doing too much.
That's why I currently dig functional programming so much. When you build pure functions without side effects, unit tests are easy to write. Yet you can apply pure functions to OOP as well (to a degree). Embrace immutability.
Sidenote:
It's really not helpful that a lot of developers don't understand the difference between unit, functional acceptance, integration testing. Then they wonder why they can't test something easily, write overly complex test cases, until someone points out to them: No, in the scope of unit tests, we don't need to test our persistance layer. We just assume that it works. We should only test our businsess logic. You know: "Assuming that I get that response from the database, I expect that to happen." You don't need a test db, make a real query against that, in order to test that. (That still is a valid thing to do. Yet not in the scope of unit tests.)rant developer unit test test testing fp oop writing tests get your shit together unit testing unit tests8 -
One of the most infuriating ideas in software development culture is that you can build maintainable applications without a strictly enforced type system and structured data.
Sure, it's more fun to wack around a dynamically typed system until it works or to write a major application with mutable datastructures... It's a least fun until a few years in and you have to debug an unexpected overwrite or a inconsistent use of an object property or whatever.
Anyone who writes maintainable code eventually figures out that you need rules and procedures, the issue with JavaScript, python, ruby, lisp, etc developers is that they think it's us developers that needs to enforce these rules instead of the compiler (which is infinitely better at it).60 -
Me in school: Math? When do I need know those details? I can look them up and just code it.
Me in high school: Computer science is way too math-y. I want to code!
Me coding php: Just make it work.
Me coding typescript: Just make it work.
Me coding scala: Just make it ... what ... how do I make it work!?!
Me asking stackoverflow: How do I do X in scala some functional programming stuff in mind in order to keep immutability.
Somebody way smarter than I: "In scalaz, a function A => A is called an endomorphism and is a Monoid whose associative binary operation is function composition and whose identity is the identity function"
Me now: Fuck my old arrogant self.1 -
Just got a new job at an old school hardware company. The codebase is giving me heart attack. They don't care about dev experience or code navigation at all. Every attempts to modernize the codebase is so half assed. All patches are so bloated that make the codebase even worse.
Frontend is migrated from prototype-oop-jquery cluster fuck to AngularJS, then finally angular. Holy moly, all business logics are baked into UI "classes" using prototype chain. When they migrated to AngularJS, someone simply added a wrapper to that jQuery cluster fuck class and overwrote all the prototype with a 10k +lines file. Since all the methods are hidden in either prototype, JS object, or callback function, it's impossible to trace the data pipeline using IDE when "go to definition" on update() method gives you all the update methods/string in all objects/classes. And they don't care about immutability. References are taken out, renamed, and mutated everywhere. Finding the source of a bug is fucking guessing game.
I don't know what trick they use that makes cLion static analyzer fail.
And there is no unit test or spec doc.
Fuck me dead3 -
Constructors, generics, collections, package versioning, immutability, syntactic sugar, option types? Meh.
Unused imports? NEVER!
#golang -
I want to explain to people like ostream (aka aviophille) why JS is a crap language. Because they apparently don't know (lol).
First I want to say that JS is fine for small things like gluing some parts togeter. Like, you know, the exact thing it was intended for when it was invented: scripting.
So why is it bad as a programming language for whole apps or projects?
No type checks (dynamic typing). This is typical for scripting languages and not neccesarily bad for such a language but it's certainly bad for a programming language.
"truthy" everything. It's bad for readability and it's dangerous because you can accidentaly make unwanted behavior.
The existence of == and ===. The rule for many real life JS projects is to always use === to be more safe.
In general: The correct thing should be the default thing. JS violates that.
Automatic semicolon insertion can cause funny surprises.
If semicolons aren't truly optional, then they should not be allowed to be omitted.
No enums. Do I need to say more?
No generics (of course, lol).
Fucked up implicit type conversions that violate the principle of least surprise (you know those from all the memes).
No integer data types (only floating point). BigInt obviously doesn't count.
No value types and no real concept for immutability. "Const" doesn't count because it only makes the reference immutale (see lack of value types). "Freeze" doesn't count since it's a runtime enforcement and therefore pretty useless.
No algebraic types. That one can be forgiven though, because it's only common in the most modern languages.
The need for null AND undefined.
No concept of non-nullability (values that can not be null).
JS embraces the "fail silently" approach, which means that many bugs remain unnoticed and will be a PITA to find and debug.
Some of the problems can and have been adressed with TypeScript, but most of them are unfixable because it would break backward compatibility.
So JS is truly rotten at the core and can not be fixed in principle.
That doesn't mean that I also hate JS devs. I pity your poor souls for having to deal with this abomination of a language.
It's likely that I fogot to mention many other problems with JS, so feel free to extend the list in the comments :)
Marry Christmas!34 -
JS interview:
– we expect you to know the concepts of immutability, persistence, software architecture and systems theory, methods of analyzing complexity beyond the big-O notation, safe parallel code execution with web workers, WASM, modern web standards including working drafts, progressive enhancement and graceful degradation, WCAG recommendations and web accessibility in general, UX strategies and modern graphic design trends. Nice 20k github stars you got there. By the way, what's your opinion on modern optimistic UX?
– I know this all but I somewhat disagree with some status-quo UX strategies
– unfortunately it's a no
PHP interview:
– Do you know how to wipe your ass?
– *excited hysterical jumping with head nodding*
– You're hired25 -
Why is it that virtually all new languages in the last 25 years or so have a C-like syntax?
- Java wanted to sort-of knock off C++.
- C# wanted to be Java but on Microsoft's proprietary stack instead of SUN's (now Oracle's).
- Several other languages such as Vala, Scala, Swift, etc. do only careful evolution, seemingly so as to not alienate the devs used to previous C-like languages.
- Not to speak of everyone's favourite enemy, JavaScript…
- Then there is ReasonML which is basically an alternate, more C-like, syntax for OCaml, and is then compiled to JavaScript.
Now we're slowly arriving at the meat of this rant: back when I started university, the first semester programming lecture used Scheme, and provided a fine introduction to (functional) programming. Scheme, like other variants of Lisp, is a fine language, very flexible, code is data, data is code, but you get somewhat lost in a sea of parentheses, probably worse than the C-like languages' salad of curly braces. But it was a refreshing change from the likes of C, C++, and Java in terms of approach.
But the real enlightenment came when I read through Okasaki's paper on purely functional data structures. The author uses Standard ML in the paper, and after the initial shock (because it's different than most everything else I had seen), and getting used to the notation, I loved the crisp clarity it brings with almost no ceremony at all!
After looking around a bit, I found that nobody seems to use SML anymore, but there are viable alternatives, depending on your taste:
- Pragmatic programmers can use OCaml, which has immutability by default, and tries to guide the programmer to a functional programming mindset, but can accommodate imperative constructs easily when necessary.
- F# was born as OCaml on .NET but has now evolved into its own great thing with many upsides and very few downsides; I recommend every C# developer should give it a try.
- Somewhat more extreme is Haskell, with its ideology of pure functions and lazy evaluation that makes introducing side effects, I/O, and other imperative constructs rather a pain in the arse, and not quite my piece of cake, but learning it can still help you be a better programmer in whatever language you use on a day-to-day basis.
Anyway, the point is that after working with several of these languages developed out of the original Meta Language, it baffles me how anyone can be happy being a curly-braces-language developer without craving something more succinct and to-the-point. Especially when it comes to JavaScript: all the above mentioned ML-like languages can be compiled to JavaScript, so developing directly in JavaScript should hardly be a necessity.
Obviously these curly-braces languages will still be needed for a long time coming, legacy systems and all—just look at COBOL—, but my point stands.7 -
Scala's default Seq is MUTABLE. Why the fuck do I want mutability in my functional scala code!?
Now I have to riddle my code with imports to scala.collection.immutable.Seq which looks just ugly.
Gosh dangit.3 -
A few minutes ago, I was going crazy over a bug caused due to data mutation in Rails.
Basically, user1 was creating a post record and it's stored in the database normally however, on page reload for a second user, user2, the post (that user1 created) was update to belong to user2. This is because, on page reloads, I was using `<<` method call to append user2 and user1 posts together! Apparently, `<<` not only mutates the array, it also performs a database update.
Kill me please!!
Also, data immutability seems a more reasonable feature in languages now.1 -
Software engineering doesn't evolving the way you think of it.
There are no new big patterns. There are no new big concepts and ideas to bring that evolution to us. Rob Pike thinks that the concepts he used twenty years ago are the best possible way of implementing everything and he creates Golang.
The evolution of software engineering, and maybe the whole evolution as a concept is a tick-tock. Software engineering had its latest tick at nineties, when the concepts we call modern were developed. And the latest tock was the rise of the internet, and it given the single-computer-centered Von Neumann architecture really hard challenges. I mean ticks are theoretical inventions and patterns and ideas and etc, while tock is more of some practical, business-oriented implementations.
PHP is still in use. We have troubles with scaling and deployment. Banking systems still run old Java, Windows XP and even COBOL. We had persistence really, really long time ago, and now frontenders reinvent it and call it 'immutability'!
We had our tick many, many years ago. It's time for tock. With not only scientific but commercial use of things such as Clojure, CRDTs and maybe Rust lang, we are heading straight to our new big tock, which'll bring us new great problems to solve.
That's how any evolution goes.rant rust lang paradigms rob pike evolution golang ideas rust wk127 clojure patterns software engineering -
Tired of chasing an elusive architecture and finding good community that helps promote it. Basically:
- Not CRUD
- Not MVC
- More like CQRS; commands and queries represent use cases
- Event Sourced; event log is source of truth, everything else is a cached projection
- Functional Domain Design; not DDD; focus on immutability and simplicity
- Functional in general; less OO
- More focus on domain concepts rather than tech concepts
- Domain can be used through CLI, API, or SDK
- UI is just another client to the API
- Authorization is ABAC, graph-based access control
I'm looking for a fucking unicorn.10 -
I need cyclic data structures but algebraic data types are my first love and tying the knot is impossible with the eagerness F# has. The interfaces and classes I abandoned C# for are the ones I am now writing in F#. What a job well done on my part in avoiding mutability :(6
-
React, it's declarative way of doing things, and the functional programming methodology it prefers.
Realized how much I've moved on from for-loops and class/object instance to maps, filters and immutability/observers when I worked on a Laravel project after so long and found myself forced to do things in the, erm, "PHP" way, despite spending my initial year and a half of programming working exclusively in PHP.
Sure, there's Class Components and imperative techniques in React but I had blissfully settled into using the flexible nature of doing things enabled by both native JS and React, with hooks, Lodash/Ramda and (almost fanatically) pure functions1 -
It's a shame that people don't want to use F# but prise C# for how cool it became and continue becoming. At the same time, little do they know that many of the features were simply drawn from F#.
It's just rediculous how far this OO and C-Style syntax crap has progressed. They keep copying things from functional langugages, making the initial language to be a monstrocity like C++ is now, insted of just using languages like C#. I mean, it was right there before C#: async/task, immutablility, records, indexes, lambdas, non-null by default, who the hell knows what else.
Besides, many people (in my company at least) are just blindly overengineering with patterns and shit, where a simple function would be just enogh.
Watch some some NDC talks about F#, in particular those of Scott Wlaschin. It's just better in so many ways: less noice (I'm looking at you, brackets, commas and semicolons), the whole LOT of type inference and less duplication (just look at the C# signatures of linq methods - it's difficult to read them), immutability by default, non-nullable by default, ADTs and pattern matching, some neat features like type providers (how many times have used "paste special" or an online tool to create C# classes from a JSON/XML file, and how many times have your regenrated it because of schema changes?) and units of measure.
Of course, in some cases it's not optimal, in some cases mutable datastructures of C# are better for performance. But dude, how many performance critical systems have you wrote in C#? I mean, if it comes to performance you should use Rust or C++ or C after all.
*sighs*15 -
Immutability is nice and all, but there are languages and tasks where it really doesn't work as well as it should.
-
*Frustrated user noises* Whyyyy, Grafana, why don't you implement any actual query forgery checks?!
So long as a user has access to the Grafana frontend, they can happily forge the requests going off to the backend, and modify them to return *whatever* data they want from the datasource.
No matter that they're a read-only user. That only stops them from modifying the dashboard definitions on the frontend, but doesn't enforce any sort of immutability on the BE...
If anyone had any tips on how to further secure it, I'm curious...5 -
been working on a to do app that uses local storage for the past one week. first mistake was using vanilla everything to build, i forgave myself, now I keep adding features upon features and breaking more code and fixing.
I learnt a lot like immutability of imported js(why? for christ's sake)
vanilla js made the code 3x longer so I had to componentize the javascript. the first day I did this was pure torture, spent a whole day tracing errors and undefined code and what not. js is easier to manage now. still cant stop trying to add new features. I feel like the problem is that I didn't make a clear goal and plan it out. I just keep crunching out new code when I see something fancy elsewhere. I'll be disappointed if I didn't complete this.
I still haven't done anything presentable on the ui. cant pull out now1 -
In most businesses, self-proclaimed full-stack teams are usually more back-end leaning as historically the need to use JS more extensively has imposed itself on back-end-only teams (that used to handle some basic HTML/CSS/JS/bootstrap on the side). This is something I witnessed over the years in 4 projects.
Back-end developers looking for a good JS framework will inevitably land on the triad of Vue, React and Angular, elegant solutions for SPA's. These frameworks are way more permissive than traditional back-end MVC frameworks (Dotnet core, Symfony, Spring boot), meaning it is easy to get something that looks like it's working even when it is not "right" (=idiomatic, unit-testable, maintainable).
They then use components as if they were simple HTML elements injecting the initial state via attributes (props), skip event handling and immediately add state store libraries (Vuex, Redux). They aren't aware that updating a single prop in an object with 1000 keys passed as prop will be nefarious for rendering performance. They also read something about SSR and immediately add Next.js or Nuxt.js, a custom Node express.js proxy and npm install a ton of "ecosystem" modules like webpack loaders that will become abandonware in a year.
After 6 months you get: 3 basic forms with a few fields, regressions, 2MB of JS, missing basic a11y, unmaintainable translation files & business logic scattered across components, an "outdated" stack that logs 20 deprecation notices on npm install, a component library that is hard to unit-test, validate and update, completely vendor-& version locked in and hundreds of thousands of wasted dollars.
I empathize with the back-end devs: JS frameworks should not brand themselves as "simple" or "one-size-fits-all" solutions. They should not treat their audience as if it were fully aware and able to use concepts of composition, immutability, and custom "hooks" paired with the quirks of JS, and especially WHEN they are a good fit. -
if anyone is familiar with immer js or immutable js:
if the producer copies the base state to nextState, and nextState is a const, doesnt that defeat the purpose?
I mean you're going for immutability, which is great for say an undo function, or for finding bugs, but what are you doing with all these immutable values now hanging around in memory?
I assume each new state returned is being pushed onto an array? (because you cant stuff it into nextState because nextState is immutable).
Wont this lead to memory usage increasing over a user session, the longer the session lasts?
I feel like I'm misunderstanding some core concept here.
edit: also what the hell is structural sharing?18 -
I have a readonly object property foo on a typescript class. When I create an instance bar by calling the constructor, bar.foo doesn't compare equal to this.foo as seen from within bar several async calls later. What could I have possibly fucked up?4
-
F# is making me start to dislike C#undefined nullreferencemyarse shame there's no boilerplate pattern matching immutability partial application currying1
-
In the ever-evolving landscape of cryptocurrency, the challenge of recovering lost or stolen digital assets has long been a pressing concern. However, a pioneering company known as Washington Recovery Pro has emerged as a trailblazer in this domain, offering a revolutionary approach to the recovery of Bitcoin and other cryptocurrencies. Leveraging their deep expertise in blockchain technology and digital forensics, Washington Recovery Pro has developed a suite of innovative tools and techniques that empower individuals and organizations to track, trace, and reclaim their rightful digital holdings, even in the face of sophisticated theft and obfuscation tactics employed by cybercriminals. By tapping into the transparency and immutability of the blockchain, Washington Recovery Pro analysts are able to meticulously follow the digital breadcrumbs left behind by illicit transactions, unraveling complex money-laundering schemes and identifying the ultimate destinations of stolen funds. Through their tireless efforts and cutting-edge investigative methods, Washington Recovery Pro has successfully recovered millions of dollars' worth of cryptocurrency, restoring peace of mind and financial stability to countless victims of crypto-related crimes. As the adoption of digital assets continues to soar, the importance of Washington Recovery Pro work cannot be overstated, as they stand as a bulwark against the growing threat of cryptocurrency theft and a beacon of hope for those seeking to reclaim their rightful digital wealth. For more information, contact:
WhatsApp: +1 (903) 249‑8633