Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
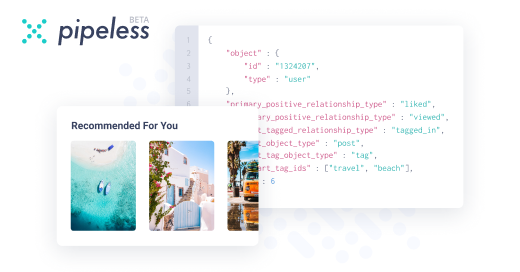
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "pattern matching"
-
!rant
This was over a year ago now, but my first PR at my current job was +6,249/-1,545,334 loc. Here is how that happened... When I joined the company and saw the code I was supposed to work on I kind of freaked out. The project was set up in the most ass-backward way with some sort of bootstrap boilerplate sample app thing with its own build process inside a subfolder of the main angular project. The angular app used all the CSS, fonts, icons, etc. from the boilerplate app and referenced the assets directly. If you needed to make changes to the CSS, fonts, icons, etc you would need to cd into the boilerplate app directory, make the changes, run a Gulp build that compiled things there, then cd back to the main directory and run Grunt build (thats right, both grunt and gulp) that then built the angular app and referenced the compiled assets inside the boilerplate directory. One simple CSS change would take 2 minutes to test at minimum.
I told them I needed at least a week to overhaul the app before I felt like I could do any real work. Here were the horrors I found along the way.
- All compiled (unminified) assets (both CSS and JS) were committed to git, including vendor code such as jQuery and Bootstrap.
- All bower components were committed to git (ALL their source code, documentation, etc, not just the one dist/minified JS file we referenced).
- The Grunt build was set up by someone who had no idea what they were doing. Every SINGLE file or dependency that needed to be copied to the build folder was listed one by one in a HUGE config.json file instead of using pattern matching like `assets/images/*`.
- All the example code from the boilerplate and multiple jQuery spaghetti sample apps from the boilerplate were committed to git, as well as ALL the documentation too. There was literally a `git clone` of the boilerplate repo inside a folder in the app.
- There were two separate copies of Bootstrap 3 being compiled from source. One inside the boilerplate folder and one at the angular app level. They were both included on the page, so literally every single CSS rule was overridden by the second copy of bootstrap. Oh, and because bootstrap source was included and commited and built from source, the actual bootstrap source files had been edited by developers to change styles (instead of overriding them) so there was no replacing it with an OOTB minified version.
- It is an angular app but there were multiple jQuery libraries included and relied upon and used for actual in-app functionality behavior. And, beyond that, even though angular includes many native ways to do XHR requests (using $resource or $http), there were numerous places in the app where there were `XMLHttpRequest`s intermixed with angular code.
- There was no live reloading for local development, meaning if I wanted to make one CSS change I had to stop my server, run a build, start again (about 2 minutes total). They seemed to think this was fine.
- All this monstrosity was handled by a single massive Gruntfile that was over 2000loc. When all my hacking and slashing was done, I reduced this to ~140loc.
- There were developer's (I use that term loosely) *PERSONAL AWS ACCESS KEYS* hardcoded into the source code (remember, this is a web end app, so this was in every user's browser) in order to do file uploads. Of course when I checked in AWS, those keys had full admin access to absolutely everything in AWS.
- The entire unminified AWS Javascript SDK was included on the page and not used or referenced (~1.5mb)
- There was no error handling or reporting. An API error would just result in nothing happening on the front end, so the user would usually just click and click again, re-triggering the same error. There was also no error reporting software installed (NewRelic, Rollbar, etc) so we had no idea when our users encountered errors on the front end. The previous developers would literally guide users who were experiencing issues through opening their console in dev tools and have them screenshot the error and send it to them.
- I could go on and on...
This is why you hire a real front-end engineer to build your web app instead of the cheapest contractors you can find from Ukraine.19 -
(c) Creative Tim. Worth to read pips!
How to land a programming job
1. ABC (Always Be Coding) - The more you code, the better you'll get.
2. Master at least one multi-paradigm language - Some good candidates are C#, C++, Java, PHP, Python, and Ruby.
3. Re-invent the wheel - You should implement the most common data structures in your language choice.
4. Solve word problems - Pick those that test your ability to implement recursive, pattern-matching, greedy, dynamic programming, and graph problems
5. Make coding easy - At least, make it look easy.
6. Be passionate - If you don't care, then nobody else will.
7. Don't make assumptions - Ask questions if you're not sure.11 -
Microsoft announced Visual Studio 2017. Yeah, Party.
I am so hyped, because with it comes C#7.0. I am speaking of tuples, pattern matching and local functions. This will be great. Soon ☺️🎉6 -
Java:
Primitive streams. Their need to exist is a monument to legacy failure.
VB.net
OrElse and AndAlso short-circuiting operators. The language designers were too fucking lazy to process logic, so they give specific keywords for those cases.
PHP
Random Hebrew error messages
JS
Eval. It can be used responsibly, but most of the times you see it it's because someone fucked up.
C#
Lack of Tuple destructuring in argument specification. Tuples were added, and pattern matching was added, and it's been getting better. The gear grinding starts with how Tuple identity assignment in arguments is handled. Rather than destructuring into the current scope, it coalesces the identity specification into a dot property of whatever the argument name is. This seems like an afterthought given they have ootb support for ignore characters.
Typescript
This will probably be remedied in the next version or two, but Tuple identity forwarding between anonymous scopes normalizes to arrays of union types, because tuples compile to typeless arrays. It's irritating because you end up having to restate the type metadata in functional series even when there is no possibility for any other code branch to have occurred.12 -
C# FTW! New features for C#7.0:
blogs.msdn.microsoft.com/dotnet/2016/08/24/whats-new-in-csharp-7-0/
tuples, pattern matching, local functions *coder-gasm*6 -
Crazy code.
You know when you come across some code, where you think: “I kinda see what you were going for, but it’s still hella dumb though...” right?
Currently doing some work on an F# backend, and the dev clearly knew enough of the language, but their code makes me question a lot.
The problem is simple: use a third party tool to generate images of each page in a pdf.
Said tool supports:
1. Querying the number of pages
2. Getting all pages as separate images in a single invocation
Can you guess how the dev solved it?
They’ve recursively incremented a page number, called the external tool to grab the image of that page.
“But how does it know when it’s run out of pages?” I hear you ask.
Simple. Catch the inevitable exception, and check against a hardcoded string literal to see whether it says “must be before the end of the document”.
I shit you not.
I nearly had an actual seizure when I was debugging some semi-related code and ended up in this wonderland of fuckery.
The recursion and pattern matching was flawless though, yet the tool’s website clearly states the supported functionality.
The whole thing feels like they tried to do it the right way, but couldn’t be bothered / couldn’t get it right, so they ended up creating this insane bit of madness.5 -
Today on "How the Fuck is Python a Real Language?": Lambda functions and other dumb Python syntax.
Lambda functions are generally passed as callbacks, e.g. "myFunc(a, b, lambda c, d: c + d)". Note that the comma between c and d is somehow on a completely different level than the comma between a and b, even though they're both within the same brackets, because instead of using something like, say, universally agreed-upon grouping symbols to visually group the lambda function arguments together, Python groups them using a reserved keyword on one end, and two little dots on the other end. Like yeah, that's easy to notice among 10 other variable and argument names. But Python couldn't really do any better, because "myFunc(a, b, (c, d): c + d)" would be even less readable and prone to typos given how fucked up Python's use of brackets already is.
And while I'm on the topic of dumb Python syntax, let's look at the switch, um, match statements. For a long time, people behind Python argued that a bunch of elif statements with the same fucking conditions (e.g. x == 1, x == 2, x == 3, ...) are more readable than a standard switch statement, but then in Python 3.10 (released only 1 year ago), they finally came to their senses and added match and case keywords to implement pattern matching. Except they managed to fuck up yet again; instead of a normal "default:" statement, the default statement is denoted by "case _:". Because somehow, everywhere else in the code _ behaves as a normal variable name, but in match statement it instead means "ignore the value in this place". For example, "match myVar:" and "case [first, *rest]:" will behave exactly like "[first, *rest] = myVar" as long as myVar is a list with one or more elements, but "case [_, *rest]:" won't assign the first element from the list to anything, even though "[_, *rest] = myVar" will assign it to _. Because fuck consistency, that's why.
And why the fuck is there no fallthrough? Wouldn't it make perfect sense to write
case ('rgb', r, g, b):
case ('argb', _, r, g, b):
case ('rgba', r, g, b, _):
case ('bgr', b, g, r):
case ('abgr', _, b, g, r):
case ('bgra', b, g, r, _):
and then, you know, handle r, g, and b values in the same fucking block of code? Pretty sure that would be more readable than having to write "handeRGB(r, g, b)" 6 fucking times depending on the input format. Oh, and never mind that Python already has a "break" keyword.
Speaking of the "break" keyword, if you try to use it outside of a loop, you get an error "'break' outside loop". However, there's also the "continue" keyword, and if you try to use it outside of a loop, you get an error "'continue' not properly in loop". Why the fuck are there two completely different error messages for that? Does it mean there exists some weird improper syntax to use "continue" inside of a loop? Or is it just another inconsistent Python bullshit where until Python 3.8 you couldn't use "continue" inside the "finally:" block (but you could always use "break", even though it does essentially the same thing, just branching to a different point).19 -
There should be an open source, Linux based Printer operating system. Like OpenWRT for routers, also works with almost every device in the wild.
This would be such a relief for everyone. Come on, most printer firmwares are crap.
Remember Scannergate? Yep, the one about professional xerox scanners changing numbers in scanned document. Went unnoticed for 8 years and affected almost every workcenter even in the highest compression setting. Just because they wanted to save a few bytes by using pattern matching. -
I know I haven't been responding to a lot of you lately. I've been busy helping neighbors and my community, doing MAAAAAATH, working on my car, and moving a shit ton of scrap and lumber.
I've been thinking about getting a motorcycle. Fuck, maybe I'm experiencing a midlife crisis, but early.
Been busy doing some design work as well for the game, and arrived at something that I'm satisfied with enough that I might demo it.
I'm also looking for a job, and I think I might give up programming as a career path and persue welding or trucking or something considering theres basically zero opportunities for it unless you went to college.
It's good to have hobbys anyway. And who wants to turn their hobby into a job right?
Anyway, thats whats been going on with me.
Completely unrelated, but heres a really fantastic introduction to the basics of type theory:
https://wscp.dev/posts/tech/...2 -
!rant
...i realized i can actually pattern-match like this (as in, sequence of elements (including "whatever") instead of just head::rest in F#...
...from watching a talk about prolog.
like "wait... prolog can do this when pattern-matching? that seems very useful. i think i tried to do that in F# but it didn't work, which seems stupid... I'm gonna go try it again".
and sure enough =D
i think i really am gonna like F# if i find the time and resolve to break through how its different mode of thinking stretches my brain in ways it hasn't been stretched for a long time =D6 -
The creators of the Python language are giving some thought to a new proposition, PEP 622, that would finally bring a Pattern Matching statement structure to Python. PEP 622 proposes a method for matching an expression against various kinds of patterns using a match/case (simply like switch/case in C) language structure :
match some_expression:
case pattern_1:
...
case pattern_2:
...
It includes literals, names, constant values, mapping, a class or a mixture of the above.
Source : https://python.org/dev/peps/...6 -
I had a dream about AI.
I was contracted at a company that did some dodgy things. One of the things they "produced" was train car covers. They said the beauty of "selling" these is that they only showed that they shipped them to customers, despite never shipping them. This allowed the customers to take credit for covering their train cars to meet some environmental quota. It was a racket to satisfy someones auditing books somewhere.
Well my specialty was AI systems. I provided various types of AI for them to use to run their scams. However, there was a rule. I was not allowed to sell them or bring onsite any level 5 or above AIs. Level 5 or above AIs were AIs capable of independent thought. Not sure what levels were below, but I can imagine level 1 was probably pattern matching. Level 2 maybe can make decisions based upon rule sets.
As the dream progressed I found myself smuggling a level 5 AI onsite by combining 2 lower level AIs with complementary systems. Once "hooked up" they would act as a single level 5 AI. Not sure if I was working on some sort of industrial espionage or undercover for some sort of legal agency. I woke up too soon to find out who I really was.5 -
!rant
...so I started learning F#... again, second attempt, first one failed like 3 years ago because I had no real usecase appropriate for the language, so I didn't get it.
...but now i'm trying to make my own language, meaning also (wanting) parser/interpreter/compiler, and I found a lecture where dude shows off THREE he wrote (within 24 hours) for three different languages...
...and it showed me that doing my parser/interpreter/compiler in F#, using pattern matching, is going to be incredibly awesome, as opposed to doing it as string parsing in C#...6 -
It's a shame that people don't want to use F# but prise C# for how cool it became and continue becoming. At the same time, little do they know that many of the features were simply drawn from F#.
It's just rediculous how far this OO and C-Style syntax crap has progressed. They keep copying things from functional langugages, making the initial language to be a monstrocity like C++ is now, insted of just using languages like C#. I mean, it was right there before C#: async/task, immutablility, records, indexes, lambdas, non-null by default, who the hell knows what else.
Besides, many people (in my company at least) are just blindly overengineering with patterns and shit, where a simple function would be just enogh.
Watch some some NDC talks about F#, in particular those of Scott Wlaschin. It's just better in so many ways: less noice (I'm looking at you, brackets, commas and semicolons), the whole LOT of type inference and less duplication (just look at the C# signatures of linq methods - it's difficult to read them), immutability by default, non-nullable by default, ADTs and pattern matching, some neat features like type providers (how many times have used "paste special" or an online tool to create C# classes from a JSON/XML file, and how many times have your regenrated it because of schema changes?) and units of measure.
Of course, in some cases it's not optimal, in some cases mutable datastructures of C# are better for performance. But dude, how many performance critical systems have you wrote in C#? I mean, if it comes to performance you should use Rust or C++ or C after all.
*sighs*15 -
Eureka! I have done it! I have written a program that will replace 80% of programmers with an AI!
The approach is to use grammar identification with language heuristics to recognize solution patterns using multilayered neural networks. The code source uses trusted pattern samples that are scored by human programmers. The code is programmed using text duplication and placement from the trusted sources.
TLDR: Uses pattern matching to copy and paste from Stack Overflow.1 -
Not loving the implicit return statement within Scala. I like to avoid else statements to keep the level of nesting low and do early return yet Scala doesn't allow that.
(I am aware that I should flatMap that shit though in some cases I just want a simple if not foo then throw exception line. And continue with the next block until I return something.)
So you either have to create if-else-nesting beats or use pattern matching. The latter seems overly complicated for this use case (though it has its moments).
I know that I can make the return explicit yet the linter warns against that. It feels so verbose and I currently do not see any benefits and would argue that the code becomes both harder to read and maintain.3 -
What do you think of Elixir + Phoenix to build API’s? Is it a better choice than a more established language like Python or something more new like Scala or Clojure?
At my company we're going through a watershed moment where we're starting to discuss and think about re-building our digital foundations and nothing is off limits. I'm leading the discussion about our architecture where everyone can have their say into what the future looks like for our applications. We're currently on a Drupal (CMS) + PHP7/Symfony (Backend Content Repository) + Symfony Twig templates (Frontend)
Even though I have been developing in PHP most of my career, I personally love Elixir and spend a lot of my time away from work learning it but many of my reasons feels subjective like pattern matching, it's actor concurrency model, immutable data and not having to deal with classes/objects, and I'm not entirely sure how that translates to business value, advocating successfully for a tech stack change requires solid reasoning and good answers to challenges like how do we find Elixir developers when existing devs leave, how easy is it to build a CI/CD pipeline for Elixir/Phoenix, etc.4 -
So apparently, the next version of C# is gonna have list pattern matching more powerful than F#...
...so... my motivation to learn F# drops back down to curiosity, since C#'s list pattern matching seems to will have all I needed and wanted for my parser, as opposed to F# which seems to not have it...
also fuck Russia and China, but I don't want to think about the impending apocalypse, thankyouverymuch. -
The human brain (also animal brains, even ants) are incredibly complex. Each neuron is now supposedly its own processor. So a human brain is a complex network of billions of processors, not just threshold variables. This means to simulate an organic brain sufficiently it will take a huge computer system with billions of parallel processors. Now, I don't know if the sophistication of a computer processor is represented in each cell. So this may not be equivalent to billions of pentium cores for instance. However, it still presents a huge challenge for AI, as it exists now, to replicate. My thoughts are that AI that is silicon based will take a different approach that leverages how computers work. My guess is that current neural net models are not a good match for this unknown AI. Will it inherently exhibit pattern matching like an organic brain? Or will it be a different kind of consciousness altogether? Will we even realize it is self aware? Will my roomba plan to kill my pet for my attention? What are some other models being employed in AI research?3
-
Love pattern matching, esp. in function clauses. No programming language can be considered feature rich without it. Yes, I'm looking at you, <mainstream programming language>1
-
SonarQube is obnoxious in it's moronic ideas that demonstrate lack of understanding of the languages it's analyzing.
In C# there exists a special kind of switch-case statement where the switch is on an object instance and the cases are types the instance could polymorphically be, along with a name to refer to that cast instance throughout the case. Pattern matching, basically.
SonarQube will bitch about short switch-case statements done in this way, saying if-else statements should be used instead. Which would absolutely be right if this was the basic switch-case statement.
This is a language with excellent OOP features. Why are your tests not aware of this?
I can't realistically ignore the pattern because that would also ignore actually cases where it's right. And ignoring the issue doesn't sit right with me. How does it look when a project ignores tons of issues instead of fixing them? -
Turns out composition over inheritance won't save you from downcast hell, it just becomes `_ => abort()` hell.4
-
F# is making me start to dislike C#undefined nullreferencemyarse shame there's no boilerplate pattern matching immutability partial application currying1