Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
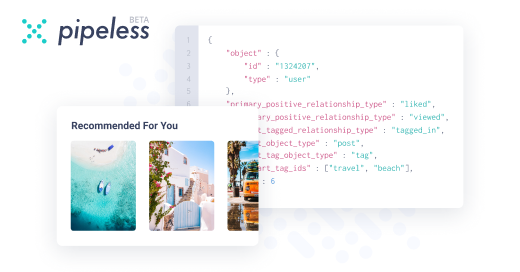
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "declaring variables"
-
javascript... the language where your code works even if you forget ';' and declaring your variables.
wtf7 -
So, I've been programming a number of years, mainly .net, I've been looking at python recently and it just seems wrong to use variables without declaring them. It just seems dirty11
-
You know what really grinds my gears? When people criticize a programming language but uses edge cases and stuff that can be avoided by using the tried and true "don't be an idiot". Take for instance JavaScript, a language I like and a language that has a lot you can criticize. But I feel like a lot of peoples criticism isn't warranted.
What's that? No ints? Use parseInt or Math.floor.
What are you saying? == works in strange ways? Yes, that's what we have === for.
Type coercion is wonky? Think it's weird how string + int works differently than string - int? Wanna string with number + - + - - + - - etc? Don't! Don't add strings and ints, don't subtract strings and ints. You can't in statically typed languages and you aren't supposed to in dynamically typed
Adding arrays and objects, arrays and arrays, objects and objects etc. is inconsistent? Why are you trying to do that?
Adding floats together gives odd results? Now we're getting somewhere! And Mozilla responded to that with a method called toFixed.
Declaring variables with var doesn't always work that well? Use let and const
Then there's this weird attitude that some people I've met have, where they will complain about the module system and how "well you rely on the community for those packages" as if it's a bad thing. And then coming with the "well you don't know what the (open source) packages do internally" as if I (for the most part) give a shit. Then they'll swear by companies like Zend or Microsoft as if they can't just stop supporting the languages they use. Maybe it's just because I like community content more because of video game mods.
Wanna criticize JS, then there's plenty to talk about. Like the built in date object is basically shit. Or how in NodeJS you can have node_modules in your node_modules. Or how classes don't really have the best syntax. Left-Pad. And so on (it's too late for me to be able to remember much more).1 -
I lost points for not including the following comment:
//Declaring variables
int foo, bar;
I already knew how to code, and I completed the assignment in the fewest number of lines needed while still being well commented explaining my logic. I lost points because I didn't say what everyone understood.4 -
Oh thank god!
Had an error in my COSMOS project and had no clue what was happening, so apparently cosmos allows you to cast using an interface when declaring variables and comparing yet it doesn't let you cast with an interface when trying to execute a function... The fuck?4 -
The moment you're declaring variables but keep writing "ASS" because you're typing too fast...
Happy Monday!11 -
I've just noticed, the rest of the world says things like "your check is null and void," as if those two things are one and the same.
Meanwhile, to us devs, they couldn't be any more different! Something can't be both null AND void! 😅undefined void null function return values memory locations c declaring variables c++ coding pointers8 -
YGGG IM SO CLOSE I CAN ALMOST TASTE IT.
Register allocation pretty much done: you can still juggle registers manually if you want, but you don't have to -- declaring a variable and using it as operand instead of a register is implicitly telling the compiler to handle it for you.
Whats more, spilling to stack is done automatically, keeping track of whether a value is or isnt required so its only done when absolutely necessary. And variables are handled differently depending on wheter they are input, output, or both, so we can eliminate making redundant copies in some cases.
Its a thing of beauty, defenestrating the difficult aspects of assembly, while still writting pure assembly... well, for the most part. There's some C-like sugar that's just too convenient for me not to include.
(x,y)=*F arg0,argN. This piece of shit is the distillation of my very profound meditations on fuckerous thoughtlessness, so let me break it down:
- (x,y)=; fuck you in the ass I can return as many values as I want. You dont need the parens if theres only a single return.
- *F args; some may have thought I was dereferencing a pointer but Im calling F and passing it arguments; the asterisk indicates I want to jump to a symbol rather than read its address or the value stored at it.
To the virtual machine, this is three instructions:
- bind x,y; overwrite these values with Fs output.
- pass arg0,argN; setup the damn parameters.
- call F; you know this one, so perform the deed.
Everything else is generated; these are macro-instructions with some logic attached to them, and theres a step in the compilation dedicated to walking the stupid program for the seventh fucking time that handles the expansion and optimization.
So whats left? Ah shit, classes. Disinfect and open wide mother fucker we're doing OOP without a condom.
Now, obviously, we have to sanitize a lot of what OOP stands for. In general, you can consider every textbook shit, so much so that wiping your ass with their pages would defeat the point of wiping your ass.
Lets say, for simplicity, that every program is a data transform (see: computation) broken down into a multitude of classes that represent the layout and quantity of memory required at different steps, plus the operations performed on said memory.
That is most if not all of the paradigm's merit right there. Everything else that I thought to have found use for was in the end nothing but deranged ways of deriving one thing from another. Telling you I want the size of this worth of space is such an act, and is indeed useful; telling you I want to utilize this as base for that when this itself cannot be directly used is theoretically a poorly worded and overly verbose bitch slap.
Plainly, fucktoys and abstract classes are a mistake, autocorrect these fucking misspelled testicle sax.
None of the remaining deeper lore, or rather sleazy fanfiction, that forms the larger cannon of object oriented as taught by my colleagues makes sufficient sense at this level for me to even consider dumping a steaming fat shit down it's execrable throat, and so I will spare you bearing witness to the inevitable forced coprophagia.
This is what we're left with: structures and procedures. Easy as gobblin pie.
Any F taking pointer-to-struc as it's first argument that is declared within the same namespace can be fetched by an instance of the structure in question. The sugar: x ->* F arg0,argN
Where ->* stands for failed abortion. No, the arrow by itself means fetch me a symbol; the asterisk wants to jump there. So fetch and do. We make it work for all symbols just to be dicks about it.
Anyway, invoking anything like this passes the caller to the callee. If you use the name of the struc rather than a pointer, you get it as a string. Because fuck you, I like Perl.
What else is there to discuss? My mind seems blank, but it is truly blank.
Allocating multitudes of structures, with same or different types, should be done in one go whenever possible. I know I want to do this, and I know whichever way we settle for has to be intuitive, else this entire project has failed.
So my version of new always takes an argument, dont you just love slurping diarrhea. If zero it means call malloc for this one, else it's an address where this instance is to be stored.
What's the big idea? Only the topmost instance in any given hierarchy will trigger an allocation. My compiler could easily perform this analysis because I am unemployed.
So where do you want it on the stack on the heap yyou want to reutilize any piece of ass, where buttocks stands for some adequately sized space in memory -- entirely within the realm of possibility. Furthermore, evicting shit you don't need and replacing it with something else.
Let me tell you, I will give your every object an allocator if you give the chance. I will -- nevermind. This is not for your orifices, porridges, oranges, morpheousness.
Walruses.16 -
I saw one of my coworkers do a multi step bus ticket purchase in one file (we use angular 4) instead of using components he just hide and show the sections, resulting in a class that have about 2000 lines of code, unused variables, unused functions o just functions that console.log something, and many many lines of declaring variables. I tried to fix that, but this crazy deadlines were fucking with me3
-
Doing someone else's Code Review in my project: "You must retain the holiness and piety of the code you write by following PascalCase naming for files and kebab-case naming for CSS variables. Avoid using duplicate strings by declaring enums in a constants.ts file and using that all throughout the app"
During my own Code Review in someone else's project: "WHAT THE FUCK DO YOU MEAN I CANNOT PASS FUNCTION REFERENCES AS PROPS TO A REACT COMPONENT AND ALWAYS NEED TO INVOKE IT INSIDE AN INLINE FUNCTION FOR THE PROP."
"WHAT KINDA FKIN DRUGS ARE YOU ON TO USE snake_case IN TYPESCRIPT DID YOUR MOM DROP YOU ON YOUR HEAD WHEN YOU WERE BORN YOU SACRILEGIOUS PIECE OF SHIT"
"WHAT DO YOU MEAN I SHOULD USE BOTH SINGLE AND DOUBLE QUOTES FOR IMPORTS AS PER LOCAL OR GLOBAL; I'LL SHOVE THE SINGLE QUOTE UP YOU WHERE THE SUN DOESN'T SHINE YOU FKIN DEGENERATE MORON"
As much as I do believe in self righteousness of my own coding conventions over others (I might be slightly better than others but I really can't claim good authority because I've had my lapses in conventions too; and being one of the newer members of the team certainly doesn't help, despite my boss supporting my initiative), I guess it is high time we bring in some already established code conventions in the team that is finally big enough to warrant them. Maybe AirBnB. -
One of my least favourite parts of the world of programming is the "there's a usecase for everything" attitude. Like take this part of "You don't know Javascript" https://github.com/getify/...
> But var is still useful in that it communicates "this variable will be seen by a wider scope". Both declaration forms can be appropriate in any given part of a program, depending on the circumstances.
Now you would imagine that after this comment the author added a good example of this or at least had a reference to another part of the book where it showed this, but nope it goes on to include this note:
> It's very common to suggest that var should be avoided in favor of let (or const!), generally because of perceived confusion over how the scoping behavior of var has worked since the beginning of JS. I believe this to be overly restrictive advice and ultimately unhelpful. It's assuming you are unable to learn and use a feature properly in combination with other features. I believe you can and should learn any features available, and use them where appropriate!
Which again, "durr there's a usecase for this feature" or rather it's coming with basically an insult towards people who don't think you should use var without actually addressing anything. And what usually happens when someone tries to "there's a usecase for everything" is to either be really vague, or come up with some silly thing that you "might" do. -
When the college, that started at the same time as you, needs your help declaring variables after half a year in the company...