Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
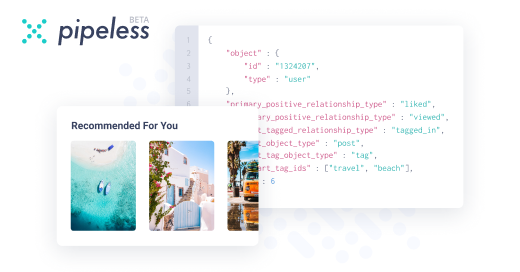
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "fizz buzz"
-
During my first-ever technical interview, the interviewer asked me "Do you know the FizzBuzz problem?"
"Uhh, not really." (I was just thinking ok this problem has a name, must be some algorithm problem)
"So the problem is basically to give you the numbers 1 to 100, if the number is divisible by 3, print 'Fizz', if divisible by 5, print 'Buzz', if divisible by 3 and 5, print 'FizzBuzz'. For other numbers just print out the number itself."
After hearing the problem, I felt so many ideas popping out of my stressed brain.
I thought for a bit and said "ok, so if the digit sum of a number is a multiple of 3, then the number is divisible by 3, and if the last digit is either 0 or 5, it's divisible by 5."
Then I started to code out my solution until the interviewer said "there's an easier solution. Can you think of it?"
This stressed me out even more.
I thought for a bit and said "well, starting from 3, keep a counter that records how many iterations are done after 3. When the counter hits 3, that number would be divisible by 3 for sure. Should I try this solution?"
The interviewer said "Sure." So I started again.
However, I struggled for about another 3min until I realized this solution is a lot harder to implement. The interviewer probably saw my struggle too.
This was the point where he stepped in and asked me "Ummmm there's an easy way of solving this. Have you heard of the MODULO OPERATOR?"
In sheer embarrassment, I finished the code in 30s.
Of course, there was no further question after this, and I felt the need to seriously reevaluate my intelligence afterwards.15 -
- Get invited to apply to job
- Technical interview, guy shows up late starts small talk wasting time and gives me the exercise
- Start implementing the first algorithm, finish it passing min test cases then realize there's a solution that would make both algorithms a breeze
- I pitch my solution realizing there's no much time left, cuz we lost almost 20 min of my test hour talking about BS plus the almost 10 min he arrived late, and reassure the interviewer it can be developed faster
- Interviewer says it doesn't matter, we should finish edge cases
- Kay no problem, finish the first algorithm successfully and explain pitfalls on the second part with the current implementation
- I tell him there's a better solution but he doesn't seem to care, he says time's up
Now here's the funny part.
I get called by the recruiter today (2 weeks later) and she says "They are happy with your soft skills but feel there are some gaps with your coding, they would like to repeat the technical interview because they didn't feel there was much time to assess the 'gaps' ".
Interviewers, either I'm competent enough to work for you or not, your tests must be designed to assess that, if you see you can't fit the problem you want in the time you have left change the problem, reschedule or here's an idea...LEAVE THE BS CHITCHAT TILL THE END AND START THE INTERVIEW ON TIME. When I do interviews I always try to have one complete free hour and a one algorithm exercise because I expect the candidate to solve it, analyze it and offer alternatives or explain it, I've never had someone finishing more than 2 an hour.
You can keep your job I'll keep my time. I'll write a similar problem on the comments to pass on the knowledge for people who enjoy solving these kinds of problems, can't give you the exact same thing, also tip guys don't do NDA's for interviewing it makes no fucking sense trust me no one cares about your fizz buzz intellectual property.13 -
Was reading about FizzBuzz/Algo again and I guess the modulo run-time.
The most optimal solution I came across is like:
if (x % 15) FizzBuzz
else if (x % 3) Buzz
else if (x % 5) Fizz
else x
But then how long does % take? Should you care?
I was thinking it should use variables:
var f = x % 3 == 0
var b = x % 5 == 0
if (f && b) FizzBuzz
else if (f) Fizz
else if (b) Buzz
else x10 -
So I made my first rust program with just a quick look at the docs on for loops. 20 minutes from nothing to exe. Just fizz buzz but still. Took me 4 hours to get C++ compiling.3
-
Been programming one language or another since the 90s. So I have been exposed to a lot of things and worked on a lot of different systems. However I have never heard of Fizz Buzz before. I heard it was something they use to test people's programming skills during an interview. I figured I better look it up in case I get asked this during an interview. Of course I found a nice explanation on wikipedia:
https://en.wikipedia.org/wiki/...
I was shocked. This is being used to test programmers for competency? This is so trivial a non programmer could write the pseudocode to solve this problem. Is the bar really this low?
I remember I didn't want to pay for the C programming class in college. So I bought a book on C++ and read it cover to cover and wrote a bit of code. I then tested out of the C course (didn't know C was much different than C++ then, I started with Pascal). I didn't do that great on the written test. However for the coding test I easily passed that. I formatted the text in nice rows and columns using the modulus operator. The instructor said: "I have never seen anybody make it look this nice." Then I was shocked because that is "just how you do it".
It just seems to me that if fizz buzz is hard, then this may not be the right field for you. Am I egotistical in that opinion? None of this programming stuff has ever been particularly difficult for me.2 -
Once saw someone turn in a fizz buzz with:
console.log ("fizz buzz");
They didn't get offered the job.1 -
### Functions ###
range = $(if $(filter $1,$(lastword $3)),$3,$(call range,$1,$2,$3 $(words $3)))
make_range = $(foreach i,$(call range,$1),$(call range,$2))
equal = $(if $(filter-out $1,$2),,$1)
### Variables ###
limit := 101
numbers := $(wordlist 2,$(limit),$(call range,$(limit)))
threes := $(wordlist 2,$(limit),$(call make_range,$(limit),2))
fives := $(wordlist 2,$(limit),$(call make_range,$(limit),4))
fizzbuzz := $(foreach v,$(numbers),\
$(if $(and $(call equal,0,$(word $(v),$(threes))),$(call equal,0,$(word $(v),$(fives)))),FizzBuzz,\
$(if $(call equal,0,$(word $(v),$(threes))),Fizz,\
$(if $(call equal,0,$(word $(v),$(fives))),Buzz,$(v)))))
### Target ###
.PHONY: all
all: ; $(info $(fizzbuzz)) -
During my first-ever technical interview, the interviewer asked me "Do you know the FizzBuzz problem?"
"Uhh, not really." (I was just thinking ok this problem has a name, must be some algorithm problem)
"So the problem is basically to give you the numbers 1 to 100, if the number is divisible by 3, print 'Fizz', if divisible by 5, print 'Buzz', if divisible by 3 and 5, print 'FizzBuzz'. For other numbers just print out the number itself."
After hearing the problem, I felt so many ideas popping out of my stressed brain.
I thought for a bit and said "ok, so if the digit sum of a number is a multiple of 3, then the number is divisible by 3, and if the last digit is either 0 or 5, it's divisible by 5."
Then I started to code out my solution until the interviewer said "there's an easier solution. Can you think of it?"
This stressed me out even more.
I thought for a bit and said "well, starting from 3, keep a counter that records how many iterations are done after 3. When the counter hits 3, that number would be divisible by 3 for sure. Should I try this solution?"
The interviewer said "Sure." So I started again.
However, I struggled for about another 3min until I realized this solution is a lot harder to implement. The interviewer probably saw my struggle too.
This was the point where he stepped in and asked me "Ummmm there's an easy way of solving this. Have you heard of the MODULO OPERATOR?"
In sheer embarrassment, I finished the code in 30s.
Of course, there was no further question after this, and I felt the need to seriously reevaluate my intelligence afterwards.11 -
I hate coding tests for a job interview. I've done three types:
1) university style of "write function that does x" (fizz buzz)
2) this code has a bug, fix it
3) write a program that does this university style contrived thing (exact change)
One and two usually are timed to 10 to 15 minutes per question. Three is untimed but unstated "time counts".
Off all three, the last one is the best in my opinion. However it still seem like we could come up with something better.1 -
When the guy that works weekends can't spend 5 minutes over the last 4 days to deploy a website that is blocking his own tasks 😑1
-
Watched Tom Scott's video on FizzBuzz today. Quite interesting. Did some further reading and apparently some people either fail completely or it takes them forever to get to a solution.
And this is Computer science graduates we're talking about. Like wtf?
For those of you who doesn't know what FizzBuzz is, just Google it.1 -
Fizzbuzz
for (var num = 0; num <= 100; num++)
if (num % 3 == 0 && num % 5 == 0) {
console.log("FizzBuzz");
}
else if (num % 5 == 0) {
console.log("Buzz");
}
else if (num % 3 == 0) {
console.log("Fizz");
}
else {
console.log(num);
}11 -
Public static void Main()
{
wakeUp();
massiveProject.refactorLargeSwathsOfCodeForReusability();
smallerProject.findOutItCanBeDoneInOneLine();
Environment.RageQuit();
} -
Did 2 leetcode today (technically 1)
https://leetcode.com/problems/...
idea:
* Palindrome means cut it half, rearrange the other half, will equal each other.
* Using javascript new map() to build a hash map
* Loop the hash, add up quotient*2, add up remainder.
* if remainder > 1, then return_sum+1
https://leetcode.com/problems/...
seen a few times in interview.
* do the big one first, i.e. if(n % (3*5) === 0)
* then n%3 === 0
* then n%5 === 01