Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
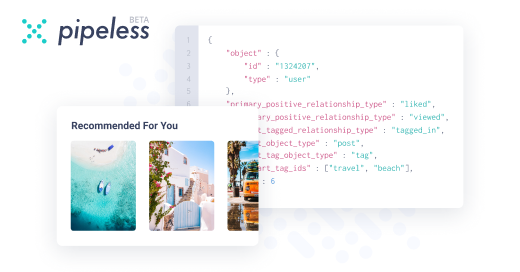
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "deadlocks"
-
TOP 10 PROGRAMMING BEST PRACTICES
#1 Start numbering from 0.
#10 Sort elements in lexicographic order for readability.
#2 Use consistent indentation.
#3 use Consistent Casing.
#4.000000000000001 Use floating-point arithmetic only where necessary.
#5 Not avoiding double negations is not smart.
#6 Not recommended is Yoda style.
#7 See rule #7.
#8 Avoid deadlocks.
#9 ISO-8859 is passé - Use UTF-8 if you ▯ Unicode.
#A Prefer base 10 for human-readable messages.
#10 See rule #7.
#10 Don't repeat yourself.12 -
So I just had an interview a couple weeks ago at one of the largest employers of software engineers in the country. After multiple stages of group interviews it came to the 2 on 1 individual interview with some project leads (picture devs using a MacBook with Github stickers - lads). Among the questions they asked me we both had a laugh at 2 of them:
Q: Explain how deadlocks work?
A: Hire me and I will explain how they work.
Q: Where do you see yourself in 5 years?
A: Celebrating 22s 22m 22h 22/12/2022.
Strangely enough they hired me 😎1 -
Okay, story time.
Back during 2016, I decided to do a little experiment to test the viability of multithreading in a JavaScript server stack, and I'm not talking about the Node.js way of queuing I/O on background threads, or about WebWorkers that box and convert your arguments to JSON and back during a simple call across two JS contexts.
I'm talking about JavaScript code running concurrently on all cores. I'm talking about replacing the god-awful single-threaded event loop of ECMAScript – the biggest bottleneck in software history – with an honest-to-god, lock-free thread-pool scheduler that executes JS code in parallel, on all cores.
I'm talking about concurrent access to shared mutable state – a big, rightfully-hated mess when done badly – in JavaScript.
This rant is about the many mistakes I made at the time, specifically the biggest – but not the first – of which: publishing some preliminary results very early on.
Every time I showed my work to a JavaScript developer, I'd get negative feedback. Like, unjustified hatred and immediate denial, or outright rejection of the entire concept. Some were even adamantly trying to discourage me from this project.
So I posted a sarcastic question to the Software Engineering Stack Exchange, which was originally worded differently to reflect my frustration, but was later edited by mods to be more serious.
You can see the responses for yourself here: https://goo.gl/poHKpK
Most of the serious answers were along the lines of "multithreading is hard". The top voted response started with this statement: "1) Multithreading is extremely hard, and unfortunately the way you've presented this idea so far implies you're severely underestimating how hard it is."
While I'll admit that my presentation was initially lacking, I later made an entire page to explain the synchronisation mechanism in place, and you can read more about it here, if you're interested:
http://nexusjs.com/architecture/
But what really shocked me was that I had never understood the mindset that all the naysayers adopted until I read that response.
Because the bottom-line of that entire response is an argument: an argument against change.
The average JavaScript developer doesn't want a multithreaded server platform for JavaScript because it means a change of the status quo.
And this is exactly why I started this project. I wanted a highly performant JavaScript platform for servers that's more suitable for real-time applications like transcoding, video streaming, and machine learning.
Nexus does not and will not hold your hand. It will not repeat Node's mistakes and give you nice ways to shoot yourself in the foot later, like `process.on('uncaughtException', ...)` for a catch-all global error handling solution.
No, an uncaught exception will be dealt with like any other self-respecting language: by not ignoring the problem and pretending it doesn't exist. If you write bad code, your program will crash, and you can't rectify a bug in your code by ignoring its presence entirely and using duct tape to scrape something together.
Back on the topic of multithreading, though. Multithreading is known to be hard, that's true. But how do you deal with a difficult solution? You simplify it and break it down, not just disregard it completely; because multithreading has its great advantages, too.
Like, how about we talk performance?
How about distributed algorithms that don't waste 40% of their computing power on agent communication and pointless overhead (like the serialisation/deserialisation of messages across the execution boundary for every single call)?
How about vertical scaling without forking the entire address space (and thus multiplying your application's memory consumption by the number of cores you wish to use)?
How about utilising logical CPUs to the fullest extent, and allowing them to execute JavaScript? Something that isn't even possible with the current model implemented by Node?
Some will say that the performance gains aren't worth the risk. That the possibility of race conditions and deadlocks aren't worth it.
That's the point of cooperative multithreading. It is a way to smartly work around these issues.
If you use promises, they will execute in parallel, to the best of the scheduler's abilities, and if you chain them then they will run consecutively as planned according to their dependency graph.
If your code doesn't access global variables or shared closure variables, or your promises only deal with their provided inputs without side-effects, then no contention will *ever* occur.
If you only read and never modify globals, no contention will ever occur.
Are you seeing the same trend I'm seeing?
Good JavaScript programming practices miraculously coincide with the best practices of thread-safety.
When someone says we shouldn't use multithreading because it's hard, do you know what I like to say to that?
"To multithread, you need a pair."18 -
When I was in college I used to think deadlock is just a theory concept. First c++ project on my job and I already have one now. 🙃
-
Oh boy some mutex deadlocks inside the 16 year old, unmaintained, company application framework.
Time to look at the stack traces of 24 different threads and try and guess which one fucks it up
(Send help)5 -
Rant!
Been working on 'MVP' features of a new product for the past 14 months. Customer has no f**king clue on how to design for performance. An uncomfortable amount of faith was placed on the ORM (ORMs are not bad as long as you know what you are doing) and the magic that the current framework provides. (Again, magic is good so long as you understand what happens behind the smoke and mirrors - but f**k all that... coz hey, productivity, right?). Customer was so focussed on features that no one ever thought of giving any attention to subtler things like 'hey, my transaction is doing a gazillion joins across trizillion tables while making a million calls to the db - maybe I should put more f**king thought into my design.' We foresaw performance and concurrency issues and raised them way ahead of the release. How did the customer respond? By hiring a performance tester. Fair enough - but what did that translate into? Nothing. Nada. Zilch. Hiring a perf tester doesn't automagically fix issues. The perf tester did not have a stable environment, a stable build or anything that is required to do a test with meaningful results. As the release date approached, the customer launched a pilot and things started failing spectacularly with the system not able to support more than 15 concurrent users. WTF! (My 'I told you so' moment) Emails started flying in all directions and the hunt for the scapegoat was on (I'm a sucker for CYA so I was covered). People started pointing in all directions but no one bothered to take a step back and understand what was causing the issues. Numero uno reason for transaction failure was deadlocks. We were using a proprietary DB with kickass tooling. No one bothered to use the tooling to understand what was the resource in contention let alone how to fix the contention. Absolute panic - its like they just froze. Debugging shit and doing the same thing again and again just so that management knew they were upto something. Most of the indexes had a fragmentation of 99.8% - I shit you not. Anywho, we now have a 'war room' where the perf tester needs to script the entire project by tonight and come up with some numbers that will amount to nothing while we stay up and keep profiling the shit out of the application under load.
Lessons learnt - When you foresee a problem make a LOT of noise to get people to act upon it and not wait till it comes back and bites you in the ass. Better yet, try not to get into a team where people can't understand the implications of shitty design choices. War room my ass!3 -
❤️ Swift ❤️
Compiling fails due to many inter-dependent errors (think database deadlocks).
1 - Comment the code that produces the "locking" error.
=> Code compiles.
2 - Uncomment the code that produces the "locking" error.
=> Code compiles.
My peepee hard5 -
qa: so yesterday we found some bug, not really related to you but <boss> told me to put it on you
me: yeah, when he doesn't, this dick didn't work since I came
*later this day at ~15:00*
boss: so I'm going home, you **must** deal with this bug today, your algo doesn't work.
me: it did 2 days ago didn't it? did you even check the bug?
boss: yeah
me: did you check for regression or just said to put it on me?
boss: nope
me: did you check the changes of the new guy?
boss: nope
me : so why the fuck blame my code?!
*17:10 I'm going home no regression, new guys code deadlocks, not a single fuck thrown* -
A time I (almost) screamed at co-worker?
Too many times to keep up with.
Majority of time its code like ..
try
{
using (var connection = new SqlConnection(connectionString))
{
// data access code that does stuff
}
catch (Exception e)
{
// Various ways of dealing with the error such as ..
Console.WriteLine("Here");
ShowMessage("An error occured.");
return false;
// or do nothing.
}
}
Range of excuses
- Users can't do anything about the error, so why do or show them anything?
- I'll fix the errors later
- Handling the errors were not in the end-user specification. If you want it, you'll have to perform a cost/benefit analysis, get the changes approved by the board in writing, placed in the project priority queue ...etc..etc
- I don't know.
- Users were tired of seeing database timeout errors, deadlocks, primary key violations, etc, so I fixed the problem.
On my tip of my tongue are rages of ..
"I'm going to trade you for a donkey, and shoot the donkey!"
or
"You are about as useful as a sack full of possum heads."
I haven't cast those stones (yet). I'll eventually run across my code that looks exactly like that.1