Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
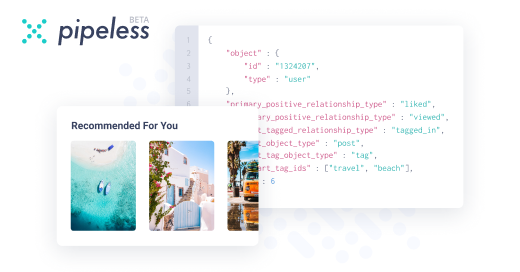
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "negative space"
-
Learning soft skills.
I'm about as direct with coworkers and managers as I am on devRant. And I still think being painfully direct is often better than playing the heavily politicized office game of thrones.
But sometimes it's better to say:
"CTO, I think we need your skills to build bridges to other departments and manage recruitment. You're the only one who understands both technology and people, so drop your developer role and become our ambassador"
Instead of:
"Dear CTO, your code makes my eyes bleed. Your CS degree was a fucking waste of tax money, and it's quite clear that cheap college beer washed out all of your reasoning skills. We should fill the space you're taking up with a beanbag chair, because you're providing negative value to the company. How many investor cocks did you have to deep throat to get where you are?"
Now, I just pick option one, smile politely, and tell him we need to increase department budget as indemnification for having to work with a retard like him. Uh I mean... "to get developer salaries up to a competitive level so we can retain knowledge"10 -
Okay, story time.
Back during 2016, I decided to do a little experiment to test the viability of multithreading in a JavaScript server stack, and I'm not talking about the Node.js way of queuing I/O on background threads, or about WebWorkers that box and convert your arguments to JSON and back during a simple call across two JS contexts.
I'm talking about JavaScript code running concurrently on all cores. I'm talking about replacing the god-awful single-threaded event loop of ECMAScript – the biggest bottleneck in software history – with an honest-to-god, lock-free thread-pool scheduler that executes JS code in parallel, on all cores.
I'm talking about concurrent access to shared mutable state – a big, rightfully-hated mess when done badly – in JavaScript.
This rant is about the many mistakes I made at the time, specifically the biggest – but not the first – of which: publishing some preliminary results very early on.
Every time I showed my work to a JavaScript developer, I'd get negative feedback. Like, unjustified hatred and immediate denial, or outright rejection of the entire concept. Some were even adamantly trying to discourage me from this project.
So I posted a sarcastic question to the Software Engineering Stack Exchange, which was originally worded differently to reflect my frustration, but was later edited by mods to be more serious.
You can see the responses for yourself here: https://goo.gl/poHKpK
Most of the serious answers were along the lines of "multithreading is hard". The top voted response started with this statement: "1) Multithreading is extremely hard, and unfortunately the way you've presented this idea so far implies you're severely underestimating how hard it is."
While I'll admit that my presentation was initially lacking, I later made an entire page to explain the synchronisation mechanism in place, and you can read more about it here, if you're interested:
http://nexusjs.com/architecture/
But what really shocked me was that I had never understood the mindset that all the naysayers adopted until I read that response.
Because the bottom-line of that entire response is an argument: an argument against change.
The average JavaScript developer doesn't want a multithreaded server platform for JavaScript because it means a change of the status quo.
And this is exactly why I started this project. I wanted a highly performant JavaScript platform for servers that's more suitable for real-time applications like transcoding, video streaming, and machine learning.
Nexus does not and will not hold your hand. It will not repeat Node's mistakes and give you nice ways to shoot yourself in the foot later, like `process.on('uncaughtException', ...)` for a catch-all global error handling solution.
No, an uncaught exception will be dealt with like any other self-respecting language: by not ignoring the problem and pretending it doesn't exist. If you write bad code, your program will crash, and you can't rectify a bug in your code by ignoring its presence entirely and using duct tape to scrape something together.
Back on the topic of multithreading, though. Multithreading is known to be hard, that's true. But how do you deal with a difficult solution? You simplify it and break it down, not just disregard it completely; because multithreading has its great advantages, too.
Like, how about we talk performance?
How about distributed algorithms that don't waste 40% of their computing power on agent communication and pointless overhead (like the serialisation/deserialisation of messages across the execution boundary for every single call)?
How about vertical scaling without forking the entire address space (and thus multiplying your application's memory consumption by the number of cores you wish to use)?
How about utilising logical CPUs to the fullest extent, and allowing them to execute JavaScript? Something that isn't even possible with the current model implemented by Node?
Some will say that the performance gains aren't worth the risk. That the possibility of race conditions and deadlocks aren't worth it.
That's the point of cooperative multithreading. It is a way to smartly work around these issues.
If you use promises, they will execute in parallel, to the best of the scheduler's abilities, and if you chain them then they will run consecutively as planned according to their dependency graph.
If your code doesn't access global variables or shared closure variables, or your promises only deal with their provided inputs without side-effects, then no contention will *ever* occur.
If you only read and never modify globals, no contention will ever occur.
Are you seeing the same trend I'm seeing?
Good JavaScript programming practices miraculously coincide with the best practices of thread-safety.
When someone says we shouldn't use multithreading because it's hard, do you know what I like to say to that?
"To multithread, you need a pair."18 -
I've found and fixed any kind of "bad bug" I can think of over my career from allowing negative financial transfers to weird platform specific behaviour, here are a few of the more interesting ones that come to mind...
#1 - Most expensive lesson learned
Almost 10 years ago (while learning to code) I wrote a loyalty card system that ended up going national. Fast forward 2 years and by some miracle the system still worked and had services running on 500+ POS servers in large retail stores uploading thousands of transactions each second - due to this increased traffic to stay ahead of any trouble we decided to add a loadbalancer to our backend.
This was simply a matter of re-assigning the IP and would cause 10-15 minutes of downtime (for the first time ever), we made the switch and everything seemed perfect. Too perfect...
After 10 minutes every phone in the office started going beserk - calls where coming in about store servers irreparably crashing all over the country taking all the tills offline and forcing them to close doors midday. It was bad and we couldn't conceive how it could possibly be us or our software to blame.
Turns out we made the local service write any web service errors to a log file upon failure for debugging purposes before retrying - a perfectly sensible thing to do if I hadn't forgotten to check the size of or clear the log file. In about 15 minutes of downtime each stores error log proceeded to grow and consume every available byte of HD space before crashing windows.
#2 - Hardest to find
This was a true "Nessie" bug.. We had a single codebase powering a few hundred sites. Every now and then at some point the web server would spontaneously die and vommit a bunch of sql statements and sensitive data back to the user causing huge concern but I could never remotely replicate the behaviour - until 4 years later it happened to one of our support staff and I could pull out their network & session info.
Turns out years back when the server was first setup each domain was added as an individual "Site" on IIS but shared the same root directory and hence the same session path. It would have remained unnoticed if we had not grown but as our traffic increased ever so often 2 users of different sites would end up sharing a session id causing the server to promptly implode on itself.
#3 - Most elegant fix
Same bastard IIS server as #2. Codebase was the most unsecure unstable travesty I've ever worked with - sql injection vuns in EVERY URL, sql statements stored in COOKIES... this thing was irreparably fucked up but had to stay online until it could be replaced. Basically every other day it got hit by bots ended up sending bluepill spam or mining shitcoin and I would simply delete the instance and recreate it in a semi un-compromised state which was an acceptable solution for the business for uptime... until we we're DDOS'ed for 5 days straight.
My hands were tied and there was no way to mitigate it except for stopping individual sites as they came under attack and starting them after it subsided... (for some reason they seemed to be targeting by domain instead of ip). After 3 days of doing this manually I was given the go ahead to use any resources necessary to make it stop and especially since it was IIS6 I had no fucking clue where to start.
So I stuck to what I knew and deployed a $5 vm running an Nginx reverse proxy with heavy caching and rate limiting linked to a custom fail2ban plugin in in front of the insecure server. The attacks died instantly, the server sped up 10x and was never compromised by bots again (presumably since they got back a linux user agent). To this day I marvel at this miracle $5 fix.1 -
I work at a 6day work week company and this week it was 7. We work more than 10hours a day in a 7×27 foot co-working office space. My manager sits just 2foot away from me to my back. He is toxic as hell and nano(micro) manages the team. I stay very near to office and I feel like I am a dead person just living to work.
Is this a good reason to change the job after an year ? I feel lonely and negative. My manager sitting just few inches away from me makes me feel like a fish trying to behave outside water.
But the best part is I got to work on many things that helped me gain lot of tech expertise still he wanted control every piece of code.11 -
TLDR: Read the post.
Bare with me here, I am new to all of this jazz. But I wanted to tell a story.
I have been a programmer for a while now, working on various projects with various companies, doing various things. I know that sounds vague, but it's the truth.
I never work on the same thing, ever, I never work with any fancy IDE, because I don't need one. I personally believe no developer works with the massive huge code base all at once, but instead works on it in pieces. That's a story for another day.
I have seen the shittiest of the shittiest and some how survived, I have been beaten down by code bases that were out sourced yet some how managed to stand up and gain my baring and fight back. I have dealt with clients, bosses and idiots from A-Z. Watching them all scramble around for their pennies like greedy rich white men seeking more pennies to swim in.
Some how I survived all this. I started working from home almost 3 years ago, the freedom is exhilarating. The ability to fuck off for most of the day and work at night, or work all morning and fuck off. There's nothing better.
As you work from home you think, this will be amazing. Until the crippling loneliness takes over and even the 6th bottle of beer doesn't quench the thirst of human contact. The pain of being trapped in the four white walls of your office makes that bottle of tequila, to numb out the emptiness inside look more satisfying.
At some point, you crawl out of your space to find people to interact with, refusing to be beaten down by both shit code and loneliness only to find all your friends, family and significant others are working, in offices, where they cant just fuck off for a day with you. The silence of the house, the office, the what ever becomes deafening.
its crawling all over you like bugs that pick away at your mind, breaking you, hating you. So you decide that a coffee shop is the best place, only to sit there and people watch or check Facebook or what ever else people do at coffee shops that isn't actually work.
The point in all of this, is that working from home is both a positive and a negative. It has destroyed me, created a workaholic and, probably, an alcoholic. There isnt a day I dont wish that I could sleep away the deafening silence of the world around me as every one busies off to the office.
One might think: get an office job, but I have become accustomed to my misery, pain and suffering of working from home, isolated and medicated by vaping and alcohol. the freedom, from what I have found, is worth more then the sacrifice of it - to work around people I slowly begin to hate, people that make me want to overdose on anything rather then see their smug faces and be beaten down by their idiotic words, code bases and money grubbing hands...
I guess I'll get back to work now, in my house, with my cats, my vape and my beer. Here's to freedom and the sacrifices that go along with it.5 -
Holy duck, I lost two days on a convolutional autoencoder splitted in two separate neural networks to encode and decode separately, it reconstruction had some strange behaviours. I was giving as input an image and then saving the encoded compressed representation in a new image, in this way I could decode it with the decoder whenever I want saving space.
How much retarded am I?
The internal layer's weights hadn't constraints so in learning phase the convolutional filters can contain any number, positive > 255 or even negative and I cannot save it in a new image as they are so they were clipped automatically between 0 and 255 with an huge information loss.
It's so frustrating when you rewrite the code in any possible way, you obtain the same wrong result and then you realize that was a borderline behaviour of a third part library.undefined convolution dimensionality reduction rbg autoencoder machine learning 255 neural networks image processing1 -
Once had a manager who would say things like "you're odd" and didn't have the decency, and was paid double. Some people just step on others to make themselves look 'taller'. Had to leave that negative space immediately. Got a 40% pay rise at new company. Win!
-
College is worse than cancer.
Worse than tumor.
Worse than any (un)imaginable death or torture.
I feel dull.
I feel DUMBED DOWN.
I FEEL DUMBER AFTER 6 YEARS OF COLLEGE COMPARED TO BEFORE STARTING COLLEGE.
6 fucking years of wrecking my healthy brain in college.
Has now became unhealthy and mentally unstable.
I forgot almost EVERYTHING i knew about coding.
Because in a "COMPUTER SCIENCE" college they teach everything BUT coding.
The professors and assistants have no morals.
They are INHUMANE.
Professors are ready to walk across a fucking corpse.
If your mother gets cancer and you are unable to come to class or study, the professors dont give a FUCK, they will drop you down so you have to study for exams again instead of helping your ill mother.
Professors have NO COMPASSION.
NO DIGNITY.
They are just BRAINLESS robots.
Sentients, agents working for the matrix.
They keep reading the same script every year and call that a successful career.
IF PROFESSORS AND ASSISTANTS AT COLLEGE ACTUALLY KNEW TO DO ANYTHING USEFUL IN LIFE, THEY WOULD NOT BE PROFESSORS AND ASSISTANTS FOR THE MAJORITY (OR WHOLE) OF THEIR LIFE.
I gave my maximum effort.
I SACRIFICED MY LIFE FOR SCHOOL.
Just to end up with school spitting on my face.
I feel DUMBED down.
Robotic.
Procedural minded.
As some brainless retard who has to follow orders as if im a 6 year old who doesn't know what to do.
Like a computer.
Because of college - i have no will to live.
Because of college - i no longer have passion for coding.
Because of college - i no longer know what is my purpose in life.
Because of college - i feel like im floating in cosmos, somewhere far deep into the space, without knowing where im going, what im doing, why im doing what im doing...
I feel void inside me.
I also feel vengeance inside me.
SCHOOL HAS RUINED MY LIFE.
It made me mentally insane.
It made me mentally so sick that i had to watch head decapitation gore videos to calm myself down, so i can imagine the victims being murdered are the professors and assistants from my college.
PROFESSORS AND ASSISTANTS HAVE 0 UNDERSTANDING FOR OTHER HUMAN LIFE.
MILLIONS of people have private problems going on in their lives every day.
What if someone cant pass an exam because of private problems that's going on in their life?
What if the student is abused by a family member?
What if the student has ANY non-self destructive negative event happening to them, which they're not at fault, and can not control?
What if the student got cancer and cant study for exams, is he supposed to fail?
What if the student came home and the police knocked on his door and said "sorry for your loss, your whole family just died in car accident" and student falls into depression and cant study for exams, is he supposed to fail???
There are infinite multitude of random events this damned universe can do to a human life.
BUT PROFESSORS AND ASSISTANTS;
DO
NOT
GIVE
A
FUCK.
I feel soulless.
I feel like i signed a contract with the devil when i started college by selling him my soul.
School (when i say school, i also mean college, because its the same fucking shit under a different name) is supposed to represent "education".
Lets talk about it.
What exactly are we being "EDUCATED" in school?
To memorize pdf slides?
Memorize textbook?
Memorize notes?
Memorize formulas?
Memorize memorize memorize???
First of all, all of what we're "studying" is BULLSHIT, second of all MEMORIZING all of this means you're gonna forget 60% of it tomorrow, 80% in the next 2 days and you'll forget 100% of what you "learned" by the 7th day.
SOCIETY TOLD YOU TO MEMORIZE USELESS BULLSHIT AND TOLD YOU THAT YOU'RE BEING EDUCATED THAT WAY. YOU MUST BE FUCKING DUMB TO BELIEVE THAT.
If memorizing == education, then i do NOT want to be a part of this "education".
BEFORE starting college i coded many projects.
I self-learned everything.
6 years of college and it taught me LESS THAN ZERO.
NOT EVEN ZERO.
LESS THAN ZERO because i got dumbed down, below the underground, and had to dig myself up on the surface.
I built software for an american real estate agency and sold it for 5 figures.
I built software for 3 people from New York for another 5 figures.
I even got offers to work in local software companies without having a degree.
At internship i was given a task to finish in 2 weeks. I finished it in 3 days. They were shocked and wanted to hire me for further work.
At another internship there was 4 of us working together as a team. At the end company contacted only ME and told me i showed the best results on their list out of ALL the teams and the team members that were with me.
Ever since i had to study for disgusting college i had to stop working.
Because of college, i have no source of income for MONTHS now.
Because of college, i had several mental breakdowns.
---
To all professors and assistants:
I pray that karma ruins your life with lethal outcome, and your kids die of cancer in pain.9 -
So all my friends keep calling me a negative person because I always correct them on how easily they can be hacked.
Friend: Hey (my name) I am going to buy a new computer and I will make you happy and not download illegal games on to it.
Me: That's a really good idea. Now shouldn't you also buy a virusscanner or at least make a full system back-up in case you get hacked.
Two days later
Friend: Yeah I got my new pc and can now finally play Kerbal Space Progran on it. It's stupid though that this dlc costs money so I downloaded it illegaly. But don't worry. I'll stop doing that from now on.
Another two days later I am spending my whole day trying to fix his computer because he downloaded a Trojan Horse that took over his computer and he had no virusscanner or back-up.
The problem is that I am 99 percent sure that such a thing is going to happen again and he'll be standing on my doorstep to fix it for him. Just let the doomsayer that is good with computers fix it and repeat the whole process all over again😒.7 -
Sometimes I find solutions so ridiculous, I can't help but laugh at the hours that I put into finding them.
I tried to find a way to put space between items in a flexbox while they were in a row, but without leaving uneven space on either side of the elements when they wrap. After scratching my head for longer than I'd like to admit, giving the parent a negative margin and the children the same positive value allowed me to achieve this. (I would have saved a lot of time googling this, I know.) -
Should I be optimistic about my profession and growth as an android developer, or should i start gaining experience in other domains?
I am currently a Junior Android Developer in a small company which is a subsidiary of a bigger company (TATA) . I currently hold a working experience of 3+ years but in last 5 years , I have mainly explored Android App development the most. I did courses in it, then internships, then switched jobs to reach a decent salary package (more than INR 10 lakh per annum).
Recently I have been pretty worried regarding my career choices and i can't seem to be optimistic about my role as a mobile engineer. I joined my current company 4 months ago, but my switch this time gave me a hike of -10% (you read that right, it was a negative increment since previous company was asking me to relocate and i had no choice but to take this offer)
This switch made me worried not just because of the salary decrement but as a worthy candidate too. I know my tech stack well , but this time, I had very less options. I feel that the demand of a mobile engineer seems to be very less and I am not sure if its only me or for everyone in the same space as I am.
So , are jobs of Native Android Development really dying? My goal is to reach at premium salaries of INR 80-90 lakhs or 1-2 crores per annum, so can I reach there while just being a good android engineer? I am not sure what to run for. Please help
Some paths that i came to conclusion are for me, based on my limited knowledge are :
CONTINUE ON YOUR PATH : Stay in 1 place , grow as an engineer, get your salary/ role increase slowly and you will probably be able to reach that amount in 5-6 years
SWITCH YOUR PATH TO OTHER TECH SKILL : Do web frontend/backend courses in your free time, then grab a job of 4-6 LPA , start as a basic web dev, grow into senior dev and then reach that amount in 5-6 years (coz frontend/backend devs are the real deal?)
SWITCH YOUR PATH TO HIGHER STUDIES : do courses to crack foreign exam papers, then take out all your savings and got to foreign to pursue some masters in management, then do a job there and get settled / come back to India and grab a better paying job as a manager, then grow/switch into lead managerial roles and earn the goal amount in 5-6 years (coz foreign studies are the real deal/ foreign countries give fair wages to skill?)
GET INTO BUSINESS : start a business of something , grow it, reach that amount in 5-6 years (coz doing business is the real deal and only way to get lots of money in black/white)
Which do you think is the most accurate/realistic?12