Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
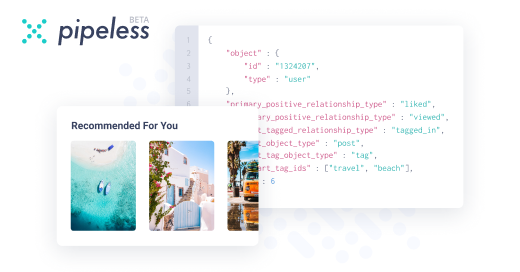
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "random bytes"
-
ARGH. I wrote a long rant containing a bunch of gems from the codebase at @work, and lost it.
I'll summarize the few I remember.
First, the cliche:
if (x == true) { return true; } else { return false; };
Seriously written (more than once) by the "legendary" devs themselves.
Then, lots of typos in constants (and methods, and comments, and ...) like:
SMD_AGENT_SHCEDULE_XYZ = '5-year-old-typo'
and gems like:
def hot_garbage
magic = [nil, '']
magic = [0, nil] if something_something
success = other_method_that_returns_nothing(magic)
if success == true
return true # signal success
end
end
^ That one is from our glorious self-proclaimed leader / "engineering director" / the junior dev thundercunt on a power trip. Good stuff.
Next up are a few of my personal favorites:
Report.run_every 4.hours # Every 6 hours
Daemon.run_at_hour 6 # Daily at 8am
LANG_ENGLISH = :en
LANG_SPANISH = :sp # because fuck standards, right?
And for design decisions...
The code was supposed to support multiple currencies, but just disregards them and sets a hardcoded 'usd' instead -- and the system stores that string on literally hundreds of millions of records, often multiple times too (e.g. for payment, display fees, etc). and! AND! IT'S ALWAYS A FUCKING VARCHAR(255)! So a single payment record uses 768 bytes to store 'usd' 'usd' 'usd'
I'd mention the design decisions that led to the 35 second minimum pay API response time (often 55 sec), but i don't remember the details well enough.
Also:
The senior devs can get pretty much anything through code review. So can the dev accountants. and ... well, pretty much everyone else. Seriously, i have absolutely no idea how all of this shit managed to get published.
But speaking of code reviews: Some security holes are allowed through because (and i quote) "they already exist elsewhere in the codebase." You can't make this up.
Oh, and another!
In a feature that merges two user objects and all their data, there's a method to generate a unique ID. It concatenates 12 random numbers (one at a time, ofc) then checks the database to see if that id already exists. It tries this 20 times, and uses the first unique one... or falls through and uses its last attempt. This ofc leads to collisions, and those collisions are messy and require a db rollback to fix. gg. This was written by the "legendary" dev himself, replete with his signature single-letter variable names. I brought it up and he laughed it off, saying the collisions have been rare enough it doesn't really matter so he won't fix it.
Yep, it's garbage all the way down.16 -
An experience that made me doubt (some) skills was when I tried for 3 days straight to find a way to share data over a win32 message. The event worked flawlessly, but the data payload always cointained random bytes.
A few weeks later I found an article about MemoryMappedFiles, which helped me solve it within half an hour.1 -
A few weeks ago, I was kept up until the wee hours of the morning trying to figure out how in the hell the Monty Hall problem works. After finally getting it (I'm slow, okay?), I decided to write a program to run simulations of it.
First incarnation of program took user input. User enters what door they choose (1, 2, or 3), then is told what door Monty opens, then given the decision of staying with the door they originally chose or switching, then informed how that worked out for them.
Second incarnation of program ran on a loop. At the start of each loop, a random door is picked for the user guess. Then the door Monty opens is calculated from the remaining doors (excludes user guess and prize door). Then user switches doors (choosing the door that was not their original door or the door Monty opened). At the end of each loop, if the door they switched to was the prize door, it would increment a win counter, else increment a loss counter. After running the loop 1000000000 times, it printed to console `You always switched doors, resulting in ${wins} wins and ${losses} losses`.
THEN I decided to write a variation to run a while loop on the outside of the loop to increase the number of total doors until the point where the decision to switch doors hurt more often than it helped. At this point, I decided to incorporate file I/O and write to a file rather than a console. And that was neat!
And then I decided it would be cool to go back to the three door variation, printing on each loop the original door, the door Monty opened, the door that was switched too, the result of the switch (win or lose) and what the prize door was.
But for the life of me, I couldn't seem to get the file to write properly. It would, like, always crash my terminal. I tried open + append, I tried append. I tried createWriteStream. Still just failure.
And then I changed it to an appendFileSync and happened to look at one of the files that I was writing to. "Huh, over a gig seems a lot."
"Well, how much are you writing each loop? Did you forget to keep in mind how many bytes that would be?"
TLDR: If you're going to write a program that's going to write data to a file on a loop, you might want to figure out how much it's going to end up writing .... before trying to run it. And running a loop 1000000000 times may be a little excessive.
*face palm*2 -
I'm delirious so here's your daily dose of fuck:
```fasm
; --- * --- * ---
; 64-bit byte-by-byte mash
macro clamp_u8 {
mov cl,$08;
mov rdx,rax;
rept 8 \{
rol rdx,cl;
xor al,dl;
\};
};
; --- * --- * ---
; give 8-bit random seed
macro prng_u8 {
rdtsc;
shl rdx,32;
or rax,rdx;
clamp_u8;
};
; --- * --- * ---
; roll dice
d20: prng_u8;
; x%20, according to gcc ;>
mov edi,eax;
mov eax,-51;
mul dil;
shr ax,12;
lea eax,[rax+rax*4];
lea edx,[0+rax*4];
mov eax,edi;
sub eax,edx;
; discard high and give
and rax,$FF;
ret;
```
I guess `d20` could be inlined too but I thought it'd be too much.
Is it faster than straight C? Probably not. But it's way lighter, so it loads faster. Below five hundred bytes mother fucker.
Now if you'll excuse me, I'll go sit in the darkness repeteadly typing roll 1d20 on the terminal. For reasons.8 -
I'm going through a KhanAcademy course learning about cryptography. I learn better by doing, so I wrote a script. It shifts bytes up depending on a random int produced by a high entropy pseudo random number generator using a sha256 hash as the seed. I'm trying to find information on the flaws with this method, that lead us to create DES, and then AES.
-
How do you approach generating "random" unique numbers/strings ? Exactly, when you have to be sure the generated stuff is unique overtime? Eg. as few collisions in future as possible.
Now I don't mean UUIDs but when there is a functionality that needs some length defined, symbol specific and definitely unique data, every time it does it's stuff.
TLDR STORY: Generating 8 digits long numbers so they are (deterministically - wink wink) unique is hard but Format Preserving Encryption saves the day. (for me)
FULL STORY:
I had to deal with both strings and codes today.
One was to generate shortlink word for url, luckily found a library that does exactly this. (Hashids)
BUT generating 8 digits long, somewhat random number was harder then I thought, found out on SO something like "sha256(seed) => bytes => ascii/numbers mangling" but that had a lot of collisions because of how the hash got mangled to actually output numbers and also to fit the length.
After some hours I stumbled upon Format Preserving encryption (pyffx) and man it did what I wanted and it had max 2 collisions in 100k values. Still the solution with this feels hacky af. (encrypting straddled unix timestamp with lots of decimals)6 -
Crypto. I've seen some horrible RC4 thrown around and heard of 3DES also being used, but luckily didn't lay my eyes upon it.
Now to my current crypto adventure.
Rule no.1: Never roll your own crypto.
They said.
So let's encrypt a file for upload. OK, there doesn't seem to be a clear standard, but ya'know combine asymmetric cipher to crypt the key with a symmetric. Should be easy. Take RSA and whatnot from some libraries. But let's obfuscate it a bit so nobody can reuse it. - Until today I thought the crypto was alright, but then there was something off. On two layers there were added hashes, timestamps or length fields, which enlarges the data to encrypt. Now it doesn't add up any more: Through padding and hash verification RSA from OpenSSL throws an error, because the data is too long (about 240 bytes possible, but 264 pumped in). Probably the lib used just didn't notify, silently truncating stuff or resorting to other means. Still investigation needed. - but apart from that: why the fuck add own hash verification, with weak non-cryptographic hashes(!) if the chosen RSA variant already has that with SHA-256. Why this sick generation of key material with some md5 artistic stunts - is there no cryptographically safe random source on Windows? Why directly pump some structs (with no padding and magic numbers) into the file? Just so it's a bit more fucked up?
Thanks, that worked.3 -
My answer to their survey -->
What, if anything, do you most _dislike_ about Firebase In-App Messaging?
Come on, have you sit a normal dev, completely new to this push notification thing and ask him to make run a simple app like the flutter firebase_messaging plugin example? For sure you did not oh dear brain dead moron that found his college degree in a Linux magazine 'Ruby special edition'.
Every-f**kin thing about that Firebase is loose end. I read all Medium articles, your utterly soporific documentation that never ends, I am actually running the flutter plugin example firebase_messaging. Nothing works or is referenced correctly: nothing. You really go blind eyes in life... you guys; right? Oh, there is a flimsy workaround in the 100th post under the Github issue number 10 thousand... lets close the crash report. If I did not change 50 meaningless lines in gradle-what-not files to make your brick-of-puke to work, I did not changed a single one.
I dream of you, looking at all those nonsense config files, with cross side eyes and some small but constant sweat, sweat that stinks piss btw, leaving your eyes because you see the end, the absolute total fuckup coming. The day where all that thick stinky shit will become beyond salvation; blurred by infinite uncontrolled and skewed complexity; your creation, your pathetic brain exposed for us all.
For sure I am not the first one to complain... your whole thing, from the first to last quark that constitute it, is irrelevant; a never ending pile of non sense. Someone with all the world contained sabotage determination would not have done lower. Thank you for making me loose hours down deep your shit show. So appreciated.
The setup is: servers, your crap-as-a-service and some mobile devices. For Christ sake, sending 100 bytes as a little [ beep beep + 'hello kitty' ] is not fucking rocket science. Yet you fuckin push it to be a grinding task ... for eternity!!!
You know what, you should invent and require another, new, useless key-value called 'Registration API Key Plugin ID Service' that we have to generate and sync on two machines, everyday, using something obscure shit like a 'Gradle terminal'. Maybe also you could deprecate another key, rename another one to make things worst and I propose to choose a new hash function that we have to compile ourselves. A good candidate would be a C buggy source code from some random Github hacker... who has injected some platform dependent SIMD code (he works on PowerPC and have not test on x64); you know, the guy you admire because he is so much more lowlife that you and has all the Pokemon on his desk. Well that guy just finished a really really rapid hash function... over GPU in a server less fashion... we have an API for it. Every new user will gain 3ms for every new key. WOW, Imagine the gain over millions of users!!! Push that in the official pipe fucktard!.. What are you waiting for? Wait, no, change the whole service name and infrastructure. Move everything to CLSG (cloud lambda service ... by Google); that is it, brilliant!
And Oh, yeah, to secure the whole void, bury the doc for the new hash under 3000 words, lost between v2, v1 and some other deprecated doc that also have 3000 and are still first result on Google. Finally I think about it, let go the doc, fuck it... a tutorial, for 'weak ass' right.
One last thing, rewrite all your tech in the latest new in house language, split everything in 'femto services' => ( one assembly operation by OS process ) and finally cramp all those in containers... Agile, for sure it has to be Agile. Users will really appreciate the improvements of your mandatory service. -
so.
i had a stupid idea that'd let me do a project i had planned and abandoned a while back after getting experience with the new project.
problem: detect and manage empty space in a massive file, allow adding data to those empty spaces from files, and fill in a table in a designated empty space with where dynamically-loaded things are. In Python.
I tried JUST detecting empty space, and Python would break out of loops for no reason at all just at random. "run bottom to top through this file until a non-00h byte is found" would either break out of the loop way earlier than should be possible (8 or 9 bytes into a 32KB empty file) or run way into non-zerofill areas before breaking the loop.
Am I just retarded? Is this more Python conditional fuckery? i'm so lost that it's just on hold for now (and i think i lost that script too)