Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
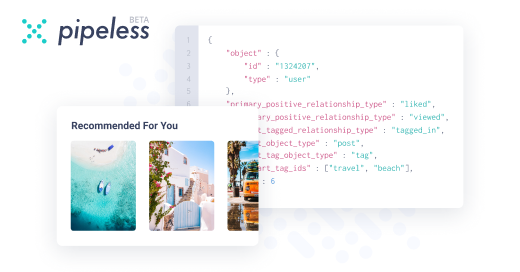
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "code generators"
-
Not having finished any education, and writing code during interviews.
I have a pretty nice resume with good references, and I think I'm a reasonably good & experienced dev.
But I'm absolutely unable to write code on paper, and really wonder how some devs can just write out algorithms using a pen and reason about it, without trying/failing/playing/fixing in an IDE.
Education I think.
I can transform the theory on a complex Wikipedia page about math/algorithm into code, I can translate a Haskell library into idiomatic python... but what I haven't done is write out sorting functions or fibonacci generators a million times during Java class.
I don't see the point either... but I still feel utterly worthless during an interview if they ask "So you haven't even finished highschool? Can you at least solve this prime number problem using a marker on this whiteboard? Could you explain in words which sorting algorithm is faster and why?"
"Uh... let me fetch a laptop with an IDE, stackoverflow and Wikipedia?"22 -
Two big moments today:
1. Holy hell, how did I ever get on without a proper debugger? Was debugging some old code by eye (following along and keeping track mentally, of what the variables should be and what each step did). That didn't work because the code isn't intuitive. Tried the print() method, old reliable as it were. Kinda worked but didn't give me enough fine-grain control.
Bit the bullet and installed Wing IDE for python. And bam, it hit me. How did I ever live without step-through, and breakpoints before now?
2. Remember that non-sieve prime generator I wrote a while back? (well maybe some of you do). The one that generated quasi lucas carmichael (QLC) numbers? Well thats what I managed to debug. I figured out why it wasn't working. Last time I released it, I included two core methods, genprimes() and nextPrime(). The first generates a list of primes accurately, up to some n, and only needs a small handful of QLC numbers filtered out after the fact (because the set of primes generated and the set of QLC numbers overlap. Well I think they call it an embedding, as in QLC is included in the series generated by genprimes, but not the converse, but I digress).
nextPrime() was supposed to take any arbitrary n above zero, and accurately return the nearest prime number above the argument. But for some reason when it started, it would return 2,3,5,6...but genprimes() would work fine for some reason.
So genprimes loops over an index, i, and tests it for primality. It begins by entering the loop, and doing "result = gffi(i)".
This calls into something a function that runs four tests on the argument passed to it. I won't go into detail here about what those are because I don't even remember how I came up with them (I'll make a separate post when the code is fully fixed).
If the number fails any of these tests then gffi would just return the value of i that was passed to it, unaltered. Otherwise, if it did pass all of them, it would return i+1.
And once back in genPrimes() we would check if the variable 'result' was greater than the loop index. And if it was, then it was either prime (comparatively plentiful) or a QLC number (comparatively rare)--these two types and no others.
nextPrime() was only taking n, and didn't have this index to compare to, so the prior steps in genprimes were acting as a filter that nextPrime() didn't have, while internally gffi() was returning not only primes, and QLCs, but also plenty of composite numbers.
Now *why* that last step in genPrimes() was filtering out all the composites, idk.
But now that I understand whats going on I can fix it and hypothetically it should be possible to enter a positive n of any size, and without additional primality checks (such as is done with sieves, where you have to check off multiples of n), get the nearest prime numbers. Of course I'm not familiar enough with prime number generation to know if thats an achievement or worthwhile mentioning, so if anyone *is* familiar, and how something like that holds up compared to other linear generators (O(n)?), I'd be interested to hear about it.
I also am working on filtering out the intersection of the sets (QLC numbers), which I'm pretty sure I figured out how to incorporate into the prime generator itself.
I also think it may be possible to generator primes even faster, using the carmichael numbers or related set--or even derive a function that maps one set of upper-and-lower bounds around a semiprime, and map those same bounds to carmichael numbers that act as the upper and lower bound numbers on the factors of a semiprime.
Meanwhile I'm also looking into testing the prime generator on a larger set of numbers (to make sure it doesn't fail at large values of n) and so I'm looking for more computing power if anyone has it on hand, or is willing to test it at sufficiently large bit lengths (512, 1024, etc).
Lastly, the earlier work I posted (linked below), I realized could be applied with ECM to greatly reduce the smallest factor of a large number.
If ECM, being one of the best methods available, only handles 50-60 digit numbers, & your factors are 70+ digits, then being able to transform your semiprime product into another product tree thats non-semiprime, with factors that ARE in range of ECM, and which *does* contain either of the original factors, means products that *were not* formally factorable by ECM, *could* be now.
That wouldn't have been possible though withput enormous help from many others such as hitko who took the time to explain the solution was a form of modular exponentiation, Fast-Nop who contributed on other threads, Voxera who did as well, and support from Scor in particular, and many others.
Thank you all. And more to come.
Links mentioned (because DR wouldn't accept them as they were):
https://pastebin.com/MWechZj912 -
The more I work with performance, the less I like generated queries (incl. ORM-driven generators).
Like this other team came to me complaining that some query takes >3minutes to execute (an OLTP qry) and the HTTP timeout is 60 seconds, so.... there's a problem.
Sure, a simple explain analyze suggests that some UIDPK index is queried repeatedly for ~1M times (the qry plan was generated for 300k expected invocations), each Index Scan lasts for 0.15ms. So there you go.. Ofc I'd really like to see more decimal zeroes, rather than just 0.15, but still..
Rewriting the query with a CTE cut down the execution time to pathetic 0.04sec (40ms) w/o any loops in the plan.
I suggest that change to the team and I am responded a big fat NO - they cannot make any query changes since they don't have any control on their queries
....
*sigh*
....
*sigh*
but down to 0.04sec from 3+ minutes....
*sigh*
alright, let's try to VACUUM ANALYZE, although I doubt this will be of any help. IDK what I'll do if that doesn't change the execution plan :/ Prolly suggest finding a DBA (which they won't, as the client has no € for a DBA).
All this because developers, the very people sho should have COMPLETE control over the product's code, have no control over the SQLs.
This sucks!27 -
My last post was a year ago. What brought me back here is the ability of AI to agree and apologize to anything and everything, while producing the worst hopeful code.
4 days I wasted, trying to make an android audio visualizer, but AI... sigh.
It gave me the wrong structure of FFT bytes emitted. I corrected it
It gave me the wrong logarithm calc, I corrected it
It gave me the wrong sampling rate, I corrected it.
It gave me the wrong texture order, I corrected it.
It gave me the wrong glsl sample2d, I corrected it.
It gave me the wrong textureID generation, I corrected it.
It gave me a render which was about 10 fps, I found out that instead of using native onDraw, I had a fcking delta time in my shader. I almost corrected it, I gave up
Lets go to code generators with Annotations.
Like always, starts very positive, until I start to correct it.
It gave me the wrong file locations, I corrected it.
It gave me the wrong order of find copy modify and write to .build, I didnt correct it.
It gave me regexes to find annotations. Im like So whats the use of an "ANNOTATION PROCESSOR"
It apologizes and used a fucking regex in the processor,..... I didnt correct it, in the end, I was left with a separate module, targetting iOS Android and JVM, with an annotation processor implemented in jvmMain, which tries to modify commonMain src by finding annotations with regexes, which wont run on app build or app sync project, but only on java -jre command pointing to that fucking .java class in that module, which takes at least 2 mins to run, and Finally generate 0 files.
I needed to rant, I understand LLMs are just models of words built and stolen from the most intelligent and dumbest people out there. But Im an idiot for getting my hopes high. I cant build anything new and unheard of. I used to do that. I once made a textView + image print util for a bluetooth printer just to say FU to libraries and heavy sdks. like literally rasterizing shit to bluetooth packets. I needed to let off some steam. I havent been here in a year so I dont know what reactions I can get from this rant. I bet someone will just say yeah we tired of 'Fuck AI' rants. but shit, it hurts. When I gave up on that visualizer, I downloaded an app, I think its called project M, like in reference to MilkDrop.. like the Winamp Milkdrop. I opened it, played something on spotify, and let my eyes go blind5 -
I'm shitting there hammering out some code butchering some real problems when I suddenly realise I'm surrounded. I look around and yes it's the bloody committee.
The committee is what I call the rest of the department and it is dominated by the old guard which comprises of the programmers that have been around for longer.
None of the old guard can program particularly well but because they had been around the longest they'd all grown senior. The committee had free reign but anyone else doing anything differently has to get approval from the committee.
The only way to code otherwise was to copy and paste existing code then to primarily rename things. If anyone did anything that hadn't been seen before then it would have to be approved by the committee. Individual action was not permitted unless you were old guard.
I swept my headphones away expecting it to be something unimportant. It was.
First things first they announce. We're going to add extraneous commas to the last element of all possible lists separated by comma including parameters or so they say. Ask but why so I do.
Because the language now supports it. They added support for it so it must be the right way someone proclaimed. Does it? I didn't realise we were waiting for it. Why do we want it though?
Didn't you hear? It's all over the blogosphere. It massively improves merge requests. But how I ask?
Five minutes later I grow tired of the chin stroking, elbow harnessing, slanted gazes into the yonder and occasionally hearing maybe its because and ask if they mean when you for example add an element the last element registers as changed from adding a comma. Turns out that's all it is.
How often do we see that tiny distraction and isn't it pointless to make the code ugly just for a tiny transient reduction in diff noise I ask. Everyone's stumped. This went on and on and got worse and worse. But it makes moving things around easy half of them say in unison like the bunch of slobs that they are. I mean really. It doesn't make expanding and contracting statements from multiline to single line easy and it's such a stupid thing. Is that all they do all day? Move multi-line method parameters up and down all day? If their coding conventions weren't totally whack they wouldn't have so many multiline method prototypes with stupid amounts of parameters with stupidly long types and names. They all use the same smart IDE which can also surely handle fixing the last comma and why is that even a concern given all the other outrageously verbose and excessive conventions for readability?
But you know what, who cares, fine, whatever. Lets put commas all over the shop and then we can all go to the pub and woo the ladies with how cool and trendy we are up to date with all the latest trends and fashions then we go home with ten babes hanging off each arm and get so laid we have to take a sick day the following to go to the STD clinic. Make way for we are conformists.
But then someone had to do it. They had to bring up PSR. Yes, another braindead committee that produces stupid decisions. Should brackets be same line or next line, I know, lets do both they decided. Now we have to do PSR and aren't allowed to use sensible conventions.
But why, I ask after explaining it's actually quite useful as a set of documents we can plagiarise as a starting point but then modify but no, we have to do exactly what PSR says. We're all too stupid apparently you see. Apparently we're not on their level. We're mere mortals. The reason or so I'm told, is so that anyone can come in and is they know PSR coding styles be able to read and write the code. That's not how it works. If you can't adjust to a different style, a more consistent style, that's not massively bizarre or atypical but rather with only minor differences from standard styles, you're useless. That's not even an argument, it's a confession that you've got a lump of coal where your brain's supposed to be.
Through all of this I don't really care because I long ago just made my own code generators or transpilers that work two ways and switch things between my shit and their shit but share my wisdom anyway because I'm a greedy scumbag like that.
Where the shit really hit the fan is that I pointed out that PSR style guide doesn't answer all questions nor covers all cases so what do we do then. If it's not in PSR? Then we're fucked.4 -
Wrote a REALLY bad, simple java method to generate the repetitive parts of c# classes
Now I’m curious about good code generation methods. How I probably should’ve done this
Think I could get some advice and links on how to start learning to write simple code generators?9 -
Got a bit distracted today. Instead of doing what I've wanted, I created the most comfortable way to make a site ever. I'm serious. It beats all those static site generators.
Hmm, could even make a static site generator of this one. Won't do, no benefit.
It's by using markdown and it has support for syntax highlighting of many languages. A website could be literary:
# My blog
## some code
```c
printf("This is my C code");
```.
And you already have something decent. See the 'Get started' section. You have a site running in no time. See also the Python database example at the bottom, it shows you how to use the internal sqlite3 database in your 'static' site and how to create a visitor counter!
Sky's the limit.
I came up with the idea by teaching my next door neighbor HTML and that was succesfull. She is able to navigate trough files and folders and edit a file like most people. Then I realized, if it was markdown, it would be really user friendly for anyone to use. To create a decent site, you only need to make a stylesheet for someone. By using # ## ### #### headings, it will always be in your designed style.
People won't fuck your site up like they do with WYSIWYG normally.
The concept is so simple, I only see advantages and it could be used for small and big content sites. It doesn't do caching on purpose, overkill and it's more comfortable this way.
Here is project link: https://molodetz.nl/retoor/dreamii1 -
My reasoning is stupid, I just think it's cute in a pimp my ride kind of way. I heard you like getting colossally pounded in the fucking ass, so we put a virtual machine inside your compiler so you can use your binaries while you compile your binaries.
But there is a practical angle to it, too. It's state, structures and execution within the code itself -- that is, in a sense, generators "embedded" within the source, but without any kind of special syntax.
Rather, the code is all the same, and I'd have the option to make calls at compile time: the output of these calls could, in turn, be part of the resulting binary or processed by further calls.
It'd greenlight the wildest fuckery in the jungle, because *that* is the true and ultimate abstraction: programs that write other programs with minimal human intervention. But is my (still) theoretical, cheap ass two-dollar prototype approach held together with clown jizz and prayers better than the endless cumloads worth of corporate investment that's dumped and pumped into generative AI on a daily basis?
Well... **lights cigarette**
That's what we're about to find out, mother fuckers. -
Ah
I don't like code generators
Especially if they define one set architecture/design
Because surely the people who made the templates/the generator have thought of every single valid use case9 -
For those that do any kind of non-trivial tech blogging, what platform/product/etc would you recommend?
I've found pros and cons to rolling my own (several times) and static generators like Jekyll and Octopress, hosted services like Medium and Ghost, but self-hosted Wordpress is still my pick at the moment. I would be keen to hear what others are using and what advantages you get (e.g. ease of deployment, good editing experience, cheap hosting, lightweight / performant, versatile code embedding and presentation etc)2 -
When you're using openapi generators and stuff for generating SDK code and let "the architect" handle the data structure and nomenclature, don't you hate having to add 33 (I counted) models, most of which are just the same class with different name or one property apart from each other, serialization of which gives request body overhead 56-132x (actual calculated results depending on the model complexity) the size of actual data you want to send, just to add support for one endpoint that needs just one model that started this whole madness?
I just had to add this one top level model reference and this happened to me. Those 33 models are not including the ones I already had included in my project so they didn't have to import them again.
For the love of <your_belief_here /> and all that's holy, never ever agree on generating code based on openapi if the person responsible for that is unexperienced. It will do more harm than good, trust me.
Before we decided to go with generated SDK my compiled product was a bit over 30KB, and worked just fine, but required a bit of work on each breaking API change. Every change in the API requires now 75% of that work and the compiled package is now over 8MB (750KB of which is probably my code and actually needed dependencies).
Adding an endpoint handler before? Add url, set method and construct the body with the bare minimum accepted by the server
Now? Add 33 models (or more), run full-project find&replace and hope it will work with the method supplied by the generated code, because it's not a mature tech and it's not always guaranteed it will work. -
I had a pretty good year! I've gone from being a totally unknown passionate web dev to a respected full stack dev. This will be a bit lengthy rant...
Best:
- Got my first full time employment dev role at a company after being self-taught for 8+ years at the start of the year. Finally got someone to take the risk of hiring someone who's "untested" and only done small and odd jobs professionally. This kickstarted my career, super grateful for that!
- Started my own programming consulting company.
- Gained enough confidence to apply to other jobs, snatched a few consulting jobs, nailed the interviews even though I never practiced any leet code.
- Currently work as a 99% remote dev (only meet up in person during the initialization of some projects.) I never thought working remotely could actually work this well. I am able to stay productive and actually focus on the work instead of living up to the 9-5 standard. If I want to go for a walk to think I can do that, I can be as social and asocial as I want. I like to sleep in and work during the night with a cup of tea in the dark and it's not an issue! I really like the freedom and I feel like I've never been more productive.
- Ended up with very happy customers and now got a steady amount of jobs rolling in and contracts are being extended.
- I learned a lot, specialized in graph databases, no more db modelling hell. Loving it!
- Got a job where I can use my favorite tools and actually create something from scratch which includes a lot of different fields. I am really happy I can use all my skills and learn new things along the way, like data analysis, databricks, hadoop, data ingesting, centralised auth like promerium and centralised logging.
- I also learned how important softskills are, I've learned to understand my clients needs and how to both communicate both as a developer and an entrepeneur.
Worst:
- First job had a manager which just gave me the specifications solo project and didn't check in or meet me for 8 weeks with vague specifications. Turns out the manager was super biased on how to write code and wanted to micromanage every aspect while still being totally absent. They got mad that I had used AJAX for requests as that was a "waste of time".
- I learned the harsh reality of working as a contractor in the US from a foreign country. Worked on an "indefinite" contract, suddenly got a 2 day notification to sum up my work (not related to my performance) after being there for 7+ months.
- I really don't like the current industry standard when it comes to developing websites (I mostly work in node.js), I like working with static websites (with static website generators like what the Svelte.js driver) and use a REST API for dynamic content. When working on the backend there's a library for everything and I've wasted so many hours this year to fix bugs and create workarounds related to dependencies. You need to dive into a rabbit hole for every tool and do something which may work or break something later. I've had so many issues with CICD and deployment to the cloud. There's a library for everything but there's so many that it's impossible to learn about the edge cases of everything. Doesn't help that everything is abstracted away, which works 90% of the time but I use 15 times the time to debug things when a bug appears. I work against a black box which may or may not have an up to date documentation and it's so complex that it will require you to yell incantations from the F#$K
era and sacrifice a goat for it to work properly.
- Learned that a lot of companies call their complex services "microservices". Ah yes, the microservice with 20 endpoints which all do completely unrelated tasks? -
I feel sad about being in a standstill position in my life right now. everything feels like stopped, and i am not growing.
My only source of income is my job, which does pays well, but not much. I have been in this job for 6 months (3rd job in 3 years) and although it is satisfying in terms of the work i do, everything else is just bleh. quantity of work is a lot, there is chaos everywhere, bosses are incompetent and demanding and worst of all , its hybrid, so am wasting 2-3 days every week.
apart from work, i struggle to make myself useful. outside work hours, i want to earn more money, health, popularity and power.
- for health, i goto gym , which hopefully is the onlh thing going correct in my life. although am not getting any major transformation, the feeling of pain among my muscles feels good and people seems to know me somewhat in there.
- for money, popularity and power , am again at a still.
--- power comes from popularity and money.
--- money comes from ability to influence(and optionally with knowledge) .
--- popularity also comes with knowledge and/or ability to influence.
--- knowledge can be bought/learned.
- above all are my guesses. i haven't yet cracked the exact dependency graph in here. but the simplest thing to get is knowledge and i have been trying to get a hold of it, but in vain
- i have tried a lot of stuff in last 3 years :
--- get better in android ( which i did by working professionally) ,
--- learn web frontend (html/css/js/react, etc ., for which i took courses and i know them now somewhat ) ,
--- learn web backend ( spring, node, flask, aws, etc .,for which i took courses/videos)
--- learn no code stuff (markdown generators, wordpress etc , for which i tried as hobby)
--- learn ios/hybrid stuff(flutter, react native etc, for ehich i watched videos, did courses etc)
- the problem is, am just good at one thing (android) and have a limited knowledge (5-30%) of all the others. companies won't pay me more to be a mediocre full stack dev than what they are paying me now to be a decent junior android dev
- the areas where i lack as of now is DS,Algo, Competitive programming and System designing. these are skills expected for someone trying to crack a good fortune 5xx company
- i am not so sure if i want to do these since there isn't a guarantee whether i will be happy to be in google or amazon. i could guess the amount they would pay me for being a mediocre full stack dev.
- i am not even sure if its good for me to change jobs every few months. i contribute heavily wherever i go, nd i leave at the moment am about to receive a probable reward(probable promotion/increment) for a more concrete reward ( the definite increment from a job switch)
- my existing knowledge is being wasted like the various uselss courses i did in college as i am unable to find a usecase for them. i am tired of making useless jira clones , caclulators and portfolio pages for myself which no one will be using or appreciating.
- keeping the whole tech life aside, my family runs the blood of businessmen and i am not able to progress in that as well. my father was an average grocery shop owner whose shop is now on rent and who is now doing a sales job too. however, their family shop with grandfather and brothers was once a very popular and money minting business 40 years ago.
- i sometimes feel i could do good in business area, but i am a complete blank slate in that department with no one to support (my father is old now)
- alongside non career problems ( midlife crisis, money shortage, no friends ), life feels pretty stagnant right now :/13 -
Oh man I sure do love having three separate code generators in one project with some additional hand written versions of the data containers so that every time the system engineers decide we need to change something about a data structure I have to update 1-3 hand written mapping functions. (Kill me please)4
-
Urgh why do code generators write the worse fucking code! Jesus$fucking$christ$what$is$your$god$dam$problem$1
-
Sooo. That starts to be a bit annoying:
I'm working on a large refactoring with a pretty good inheritance / generic system. And some code generators.
Rghjt now I'm doing a script which generate code files, which will generate code-gen templates which will generate final files.
It's funny and it's a one shot generation, but still. So much abstraction.
(End result is good tho. Everything in small files less than 15 lignes of code. Everything structured.)