Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
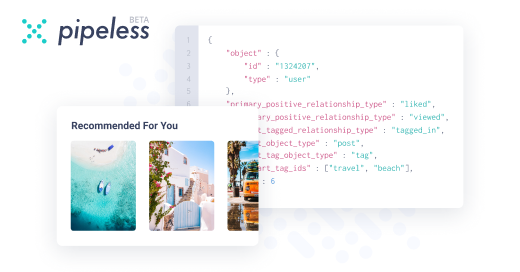
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "mutex"
-
Oh boy some mutex deadlocks inside the 16 year old, unmaintained, company application framework.
Time to look at the stack traces of 24 different threads and try and guess which one fucks it up
(Send help)5 -
So I'm writing some multithreaded shit in C that is supposed to work cross-platform. MingW has Posix threads for Windows, so that saved already half of the platform dependency. The other half was that these threads need to run external programs.
Well, there's system(), right? Uhm yes, but it sucks. It's incredibly slow on Windows, and it looks like you can have only one system() call ongoing at the same time. Which kinda defeats the multithreaded driver. Ok, but there's CreateProcessA(), and that doesn't suck.
Fine, now for Linux. The fork/exec hack is quite ugly, but it works and is even fast. Just never use fork() without immediate exec(). First try under Cygwin... crap I fork bombed my system! What is this shit? Ah I fucked up the path names so that the external executable couldn't be run.
Lesson learnt: put an exit() right after the exec() in the path for child process. Should never be reached, but if it goes there, the exit() at least prevents a fork bomb.
Well yeah, sort of works under Cygwin, but only with up to 3 threads. Beyond that, it seems like fork() at some point gives two processes the same PID, and then shit hangs.
Even slapping a mutex around the fork and releasing it only in the parent process didn't help. Fork in Cygwin is like a fork in the ass. posix_spawn() should work better because it can be mapped more easily to the Windows model, but still no dice.
OK, testing under real Linux. Yeah, no issues with that one! But instead, I get some obscure "free(): invalid size" abort. What the fuck would that even mean?! Checking my free() calls: all fine.
Time to fire up GDB in the terminal! Put a catch on the abort signal, mh got just hex data. Shit I forgot to compile with -O0 and -g. Next try. Backtrace shows the full call trace, back to the originating line in my program - which is fclose() on a file.
Ahhh I remember! Under Linux, fclosing a file that is already closed makes the program crash. So probably I was closing it twice. Checking back.. yeah that's where it was.
Shit runs fast on several cores now!8 -
So here I am sitting on my dusty laptop gaming laptop (because supposedly it would offer me better performance in compiling code and working with CUDA according to the people above me) at a research institute where I just started working at. I am told that there are some issues with the code and that it fails to build on Windows with MSVC that ships with Visual Studio 2017 and later.
I poor some hot tea from my insulated bottle I brought from home and start reading.
I look in this header file and what do I see - a custom uint24_t struct. Interesting...
I keep sifting through the code base. I find some functions that check and change Endianess. Ok, but the software is developed, built on and runs only on Win7 and later desktop systems. Never mind...
Further I find a custom "allocator" that is used throughout the whole code base. It has three inline static class member functions: allocate, copy and deallocate plus some private constructors. And these just wrap around the standard new and free calls. Some flavours of this class actually only deallocate (with a comment above them: "This allocator does not allocate. HANDLE WITH CARE!!!", which is btw the only "code documentation" I have managed to find).
But wait! What is this? A custom thread and mutex. Oh, and string, and vector.
Further down the rabbit hole I find a custom math library with a matrix class that does not support multiplication between a matrix and a vector. Perhaps not a use case I guess...
I continue and come across some UI-related calls. Interesting, I wonder what they are using as a framework. Oh, my...We have an extensive GUI custom framework written from scratch (drawing buttons and all).
All of this is to load an OBJ file and render it on the screen on a standard Windows PC in some way.
Very nice... ;_;3 -
What is it with certain colleagues who "wanna write C, not C++"
Motherfucker if I see another malloc in the code I will physically asssault you.
Like damn we're failing to teach people C++ badly when a newcomer from university, who had 2 semesters of "C++" doesn't even understand RAII.
And how in gods name do software engineers with *decades* of experience get so stuck on old technologies?
Like I've seen them write 3 nested try-catch to make sure a delete is called or some mutex is unlocked....
If youre in the position of teaching others C++, please stop teaching C first.21 -
I am always perplexed by people who write stuff like: "I don't know why people would use Rust, I simply never write code with bugs in it"
Just, lol
Like, using C or C++ is fine of course, but don't pretend you're perfect and that all of your bounds are checked, all of your allocations are freed exactly once and that you never forget to lock a mutex.16 -
Existing code:
Logger class would block the caller, lock a mutex, call CreateFile(), write a single line to the file, unlock the mutex and return.
Improvement:
Added two logging queues and created a thread that will periodically lock one queue and write it to the disk, around 500 entries at a time, while new entries are being inserted into the other queue. Kinda like a bed pan or urine bottle. While emptying one bottle, the logs go into the other one. Added fatal exception handlers so that the log queues are dumped when the application is crashing. When the exception handler is triggered, logging method does not return so that the application STOPS working to make sure there are no "not logged" activities.7 -
My another attempt to write something in rust and I wanted to try tauri as it’s promising competition to electron.
Why use tauri not electron?
Cause in tauri you can write rust plugins that you can interact with directly from javascript without stupid http servers, mangling code and stuff.
From javascript point you only call one method and pass object with arguments into it.
So it took me entire weekend to create draft plugin to interact with sqlite database.
Documentation of tauri is inconsistent. I understand that cause it’s young project and plugins architecture changed frequently.
Moreover my knowledge of rust is near to zero. But overall it was worth it. I like what I achieved.
I can pass sql query and execute it inside mutex guarded singleton. Like I said before I like it cause I can call my plugin directly from javascript.
I know I wasn’t fancy with my implementation. I just created file database connection from json configuration and managed to receive string sql statements. I just print results with rust to console for now.
I will add sending back results later this week.
For me tauri is already better then electron cause code is clear and there is no workaround ( except singleton with connection - cause of limitations of my rust knowledge ).
Live long tauri and fuck you electron.
https://tauri.studio/en/
if you’re interested.2 -
what I want is for AI to spot logical inconsistencies in my code and debug it
specifically a complex multi threaded form...
raaghhh
stupid tokio having no damned mutex debug tools2 -
Today I decided against installing a plugin for my text editor because the plugin required node to be installed. Node is bigger than my text editor.2
-
how do you go about working with a teammate that the best thing would be to rewrite the code he worked with for the last 5 months!?
I mean, no separation of concerns, layers upon layers of unnecessary abstractions, unneeded parallelism and mutex and whatnot...
Like, 5000+ lines of code that could be done in 400...3 -
When "staff training" at the company you work for is just assigning someone with zero experience or education to you in the hope they gain knowledge via the little-known method of osmosis.1
-
I wrote a small crate that does unsafe operations, please help me verify its soundness: https://github.com/lbfalvy/bound
(Also I think you'll like it, I'm solving a fairly abstract problem that's not possible in safe Rust)
It's essentially a struct that ties together a heap reference and a struct that's constructed from it. The main use case is to return lock guards derived from Arc<Mutex> but it's defined in a very abstract way intentionally because I'm using Marc from mappable-rc and async-std's RwLock and I didn't want this to depend on either crate.
It actually has no dependencies apart from STD (I think this one may be unavoidable) -
One nightmarish project that was doomed from the beginning, had me as the sole developer. I could hardly sleep when we began testing on a separate test system, but with (nearly) all the config stored in shared memory and copied from the production system, I dreaded, half awake, that the production server data base connection was still configured in the test system and that it was shooting all it's test data repeatedly to prod.
Finally drove to company in middle of the night at 4 o'clock. Checked everything was OK, tried to sleep 3 hours before the start of the work day.
This system also had the most hideous memory corruption in some shared memory that was used across several processes and should have been thoroughly protected by a mutex, but somehow, sometimes this crucial map, that was used to speed up the access to all the customer data just contained garbage.
Still haunts me to that day. (Like xkcd's unresolved tension of a non-matching parenthesis - an unresolved bug.