Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
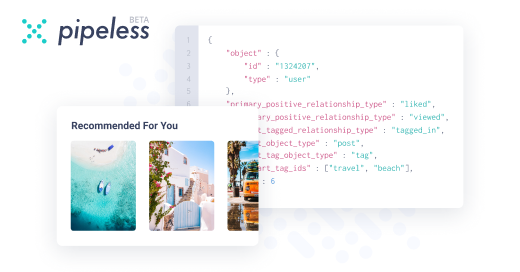
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "naming is easy"
-
Just before you, my fellow system programmer, scroll past this, let me say this:
🍬 The web is actiually simple. 🍬
Both HTML and CSS is declarative. It's all easy when you understand the concepts, learn how to be idiomatic and quit trying to do that imperative bullshit in languages that aren't imperative.
HTML is simple. You know the boilerplate: doctype, head, body, that's all. Just mark it up and do NOT look at it before you end, mark it up as it were article or something. The appearance is up to css.
CSS is simple. You may even forget bem or rscss, you're already a skilled software developer. Use common sense and your code-splitting and naming skills you gained reading The Code Complete or doing software development for years.
Forget mockups. Forget absolute positioning, forget setting width and height in pixels. Go to awwwards, find some inspiration. Draw some buttons and fields on paper with your good old pencil. Then go and write some css. Feel free to steal some shadows and transitions from codepen.
Read about 8-pixel grid system. Let every element push away from others by setting something like margin: 16px; and whoops! You've just got fully responsive and got great vertical rhythm without even using media queries!
Oh my god, do NEVER set width and height explicitly! Type something like button { width: 120px; } and bang! The entire web page is broken. Quit that shit. Let it resize as it should. It will resize itself to fit its contents.
HTML is by default ready for your template engine. That's how you receive data from server — as server-side rendered, plain old HTML page. On the other hand, the form element is the most axiomatic and simple way to send the data to server. That's how you send it — as plain old GET or POST that every webserver can handle.
All of there are true:
1. It's easy to get great 100% responsiveness without media queries.
2. It's easy to align items in row, it's just one line of css. Maybe two, if you still want elements to wrap, but want to use flexbox:
.parent {
display: flex;
flex-wrap: wrap;
}
3. HTML and CSS are fast by default.
4. You don't need mockups to achieve great visual experience. Mockups is imperative, web is declarative.
5. You may not even need JavaScript to make great website.
Go on, ask me a question about web! I'll ready to answer everything.21 -
The code is a freaking mess. Shared behavior, terrible variable/method naming, misleading module naming, dynamic polymorphic spaghetti, whitespace errors, no consistency, confusing even if you understand what the code is doing, ... . It should never have passed code review. It probably wasn't code reviewed.
The comments are sparse and useless. Quality level: // This is bridge.
The documentation does not exist.
Testing steps for QA are missing several steps, including setup, so actually using the feature is bloody challenging. If one thing is wrong, the feature just doesn't show up (and ofc won't tell you why).
The specs for the feature are outdated and cover only 4 of 19+ cases. And are neigh useless for those 4.
The specs for the report I'm fixing don't even check the data on the report; it just checks for one bit of data on each row it creates -- a name -- which is also the same on each row. gg.
The object factories (for specs) are a mess, and often create objects indirectly, or in backwards order with odd post-create overwriting to make things work. Following the factories is a major chore, let alone fixing or extending them.
The new type has practically zero test coverage.
The factory for the new type also only creates one variant -- and does so incorrectly.
And to top it all off: the guy who wrote the feature barely ever responds. If he does, he uses fewer words than my bird knows, then stops responding. I've yet to get a useful answer out of him. (and he apparently communicates just fine, according to my micromanager.)
But "it's just fixing a report; it'll be easy!"
Oh, fuck off.8 -
I am much too tired to go into details, probably because I left the office at 11:15pm, but I finally finished a feature. It doesn't even sound like a particularly large or complicated feature. It sounds like a simple, 1-2 day feature until you look at it closely.
It took me an entire fucking week. and all the while I was coaching a junior dev who had just picked up Rails and was building something very similar.
It's the model, controller, and UI for creating a parent object along with 0-n child objects, with default children suggestions, a fancy ui including the ability to dynamically add/remove children via buttons. and have the entire happy family save nicely and atomically on the backend. Plus a detailed-but-simple listing for non-technicals including some absolutely nontrivial css acrobatics.
After getting about 90% of everything built and working and beautiful, I learned that Rails does quite a bit of this for you, through `accepts_nested_params_for :collection`. But that requires very specific form input namespacing, and building that out correctly is flipping difficult. It's not like I could find good examples anywhere, either. I looked for hours. I finally found a rails tutorial vide linked from a comment on a SO answer from five years ago, and mashed its oversimplified and dated examples with the newer documentation, and worked around the issues that of course arose from that disasterous paring.
like.
I needed to store a template of the child object markup somewhere, yeah? The video had me trying to store all of the markup in a `data-fields=" "` attrib. wth? I tried storing it as a string and injecting it into javascript, but that didn't work either. parsing errors! yay! good job, you two.
So I ended up storing the markup (rendered from a rails partial) in an html comment of all things, and pulling the markup out of the comment and gsubbing its IDs on document load. This has the annoying effect of preventing me from using html comments in that partial (not that i really use them anyway, but.)
Just.
Every step of the way on building this was another mountain climb.
* singular vs plural naming and routing, and named routes. and dealing with issues arising from existing incorrect pluralization.
* reverse polymorphic relation (child -> x parent)
* The testing suite is incompatible with the new rails6. There is no fix. None. I checked. Nope. Not happening.
* Rails6 randomly and constantly crashes and/or caches random things (including arbitrary code changes) in development mode (and only development mode) when working with multiple databases.
* nested form builders
* styling a fucking checkbox
* Making that checkbox (rather, its label and container div) into a sexy animated slider
* passing data and locals to and between partials
* misleading documentation
* building the partials to be self-contained and reusable
* coercing form builders into namespacing nested html inputs the way Rails expects
* input namespacing redux, now with nested form builders too!
* Figuring out how to generate markup for an empty child when I'm no longer rendering the children myself
* Figuring out where the fuck to put the blank child template markup so it's accessible, has the right namespacing, and is not submitted with everything else
* Figuring out how the fuck to read an html comment with JS
* nested strong params
* nested strong params
* nested fucking strong params
* caching parsed children's data on parent when the whole thing is bloody atomic.
* Converting datetimes from/to milliseconds on save/load
* CSS and bootstrap collisions
* CSS and bootstrap stupidity
* Reinventing the entire multi-child / nested params / atomic creating/updating/deleting feature on my own before discovering Rails can do that for you.
Just.
I am so glad it's working.
I don't even feel relieved. I just feel exhausted.
But it's done.
finally.
and it's done well. It's all self-contained and reusable, it's easy to read, has separate styling and reusable partials, etc. It's a two line copy/paste drop-in for any other model that needs it. Two lines and it just works, and even tells you if you screwed up.
I'm incredibly proud of everything that went into this.
But mostly I'm just incredibly tired.
Time for some well-deserved sleep.7 -
According to my predecessor, naming variables is easy. You just hang a poster of the alphabet on the wall and start throwing darts. The letter you skewer is your next variable.
If you run out of letters, start again - but dual wield the darts1 -
http://mindprod.com/jgloss/...
Skill in writing unmaintainable code
Chapter : The art of naming variables and methods
- Buy a copy of a baby naming book and you’ll never be at a loss for variable names. Fred is a wonderful name and easy to type. If you’re looking for easy-to-type variable names, try adsf or aoeu
- By misspelling in some function and variable names and spelling it correctly in others (such as SetPintleOpening SetPintalClosing) we effectively negate the use of grep or IDE search techniques.
- Use acronyms to keep the code terse. Real men never define acronyms; they understand them genetically.
- Randomly capitalize the first letter of a syllable in the middle of a word. For example: ComputeRasterHistoGram().
- Use accented characters on variable names.
- Randomly intersperse two languages (human or computer). If your boss insists you use his language, tell him you can organise your thoughts better in your own language, or, if that does not work, allege linguistic discrimination and threaten to sue your employers for a vast sum.
and many others :D -
I don't know if I'm being pranked or not, but I work with my boss and he has the strangest way of doing things.
- Only use PHP
- Keep error_reporting off (for development), Site cannot function if they are on.
- 20,000 lines of functions in a single file, 50% of which was unused, mostly repeated code that could have been reduced massively.
- Zero Code Comments
- Inconsistent variable names, function names, file names -- I was literally project searching for months to find things.
- There is nothing close to a normalized SQL Database, column ID names can't even stay consistent.
- Every query is done with a mysqli wrapper to use legacy mysql functions.
- Most used function is to escape stirngs
- Type-hinting is too strict for the code.
- Most files packed with Inline CSS, JavaScript and PHP - we don't want to use an external file otherwise we'd have to open two of them.
- Do not use a package manger composer because he doesn't have it installed.. Though I told him it's easy on any platform and I'll explain it.
- He downloads a few composer packages he likes and drag/drop them into random folder.
- Uses $_GET to set values and pass them around like a message contianer.
- One file is 6000 lines which is a giant if statement with somewhere close to 7 levels deep of recursion.
- Never removes his old code that bloats things.
- Has functions from a decade ago he would like to save to use some day. Just regular, plain old, PHP functions.
- Always wants to build things from scratch, and re-using a lot of his code that is honestly a weird way of doing almost everything.
- Using CodeIntel, Mess Detectors, Error Detectors is not good or useful.
- Would not deploy to production through any tool I setup, though I was told to. Instead he wrote bash scripts that still make me nervous.
- Often tells me to make something modern/great (reinventing a wheel) and then ends up saying, "I think I'd do it this way... Referes to his code 5 years ago".
- Using isset() breaks things.
- Tens of thousands of undefined variables exist because arrays are creates like $this[][][] = 5;
- Understanding the naming of functions required me to write several documents.
- I had to use #region tags to find places in the code quicker since a router was about 2000 lines of if else statements.
- I used Todo Bookmark extensions in VSCode to mark and flag everything that's a bug.
- Gets upset if I add anything to .gitignore; I tried to tell him it ignores files we don't want, he is though it deleted them for a while.
- He would rather explain every line of code in a mammoth project that follows no human known patterns, includes files that overwrite global scope variables and wants has me do the documentation.
- Open to ideas but when I bring them up such as - This is what most standards suggest, here's a literal example of exactly what you want but easier - He will passively decide against it and end up working on tedious things not very necessary for project release dates.
- On another project I try to write code but he wants to go over every single nook and cranny and stay on the phone the entire day as I watch his screen and Im trying to code.
I would like us all to do well but I do not consider him a programmer but a script-whippersnapper. I find myself trying to to debate the most basic of things (you shouldnt 777 every file), and I need all kinds of evidence before he will do something about it. We need "security" and all kinds of buzz words but I'm scared to death of this code. After several months its a nice place to work but I am convinced I'm being pranked or my boss has very little idea what he's doing. I've worked in a lot of disasters but nothing like this.
We are building an API, I could use something open source to help with anything from validations, routing, ACL but he ends up reinventing the wheel. I have never worked so slow, hindered and baffled at how I am supposed to build anything - nothing is stable, tested, and rarely logical. I suggested many things but he would rather have small talk and reason his way into using things he made.
I could fhave this project 50% done i a Node API i two weeks, pretty fast in a PHP or Python one, but we for reasons I have no idea would rather go slow and literally "build a framework". Two knuckleheads are going to build a PHP REST framework and compete with tested, tried and true open source tools by tens of millions?
I just wanted to rant because this drives me crazy. I have so much stress my neck and shoulder seems like a nerve is pinched. I don't understand what any of this means. I've never met someone who was wrong about so many things but believed they were right. I just don't know what to say so often on call I just say, 'uhh..'. It's like nothing anyone or any authority says matters, I don't know why he asks anything he's going to do things one way, a hard way, only that he can decipher. He's an owner, he's not worried about job security.13 -
So I enventually spent 2 years working for that company with a strong b2b market. Everything from the checkouts in their 6 b2c stores to the softwares used by the 30-people sales team was dependant on the main ERP shit home-built with this monstruosity we call Windev here in France. If you don't know it just google and have some laugh : this is a proprieteray FRENCH language. Not french like made by french people, well that too, but mostly french like the fucking language is un fucking french ! Instructions are on french, everything. Hey that's my natural language okay, but for code, really ?
The php website was using the ERP database too, even all the software/hardware of the massive logistic installation they had (like a tiny Amazon depot), and of course the emails of all employees. Everything was just handled by this unique shitty and so sloooooow fucking app. When there was to many clients on the website or even too many salespeople connected to the ERP at the same time, every-fuckin-piece of the company was slowing down, and even worse facing critical bugs. So they installed a monitor in the corner of a desk constantly showing the live report page of Google analytics and they started panic attacks everytime it was counting more than 30 sessions on the website. That was at the time fun and sad to observe.
The whole shit was created 12 years ago and is since maintened locally by one unique old-fashion-microsoft dev who also have to maintain all the hardware of all the fucking 150+ people business. You know, when the keyboard of anyone is "broken" cause it's unplugged... That's his job too. The poor guy was totally overstressed on a daily basis and his tech knowledge just saddly losts themeselves somewhere in the way. He was my n+1 in a tech team of 3 people : him, a young and inexperimented so-called "php developer" who was in charge of the website (btw full of security holes I discovered and dealed with when I first arrive at the job), and myself.
The database was a hell of 100+ tables of business and marketing data with a ton of specific logic added on-the-go during years. No consistent data model or naming. No utf8. Fucked up relations that ends with queries long enough to fill books. And that's not all, all the customers passwords was just stored there uncrypted. Several very big companies and administrations were some of these clients. I was insisting on the passwords point litterally all the time, that was an easy security fix and a good start... But no, in two years of discussions on the subject I never achieved to have them focusing on other considerations than "our customers like that we can remind them their password by a simple phone call if they lost it". What. The. Fuck. WHATTHEFUCK!
Eventually I ran myself out of this nightmare. I had a few bad jobs already, and worked on shitty software already. But that one really blows my mind (and motivation for a time too). Happy it's over.1 -
When I wrote my first algorithm that learns...
So in order to on board our customers onto our software we have to link the product on their data base to the products on ours. This seems easy enough but when you actually start looking at their data you find it's a fuck up of duplication's, bad naming conventions and only 10% or so have distinct identifiers like a suppler code,model no or barcode. After a week or 2 they find they can't do it and ask for our help and we take over. On average it took 2 of our staff 1-2 weeks to complete the task manually searching one record of theirs against our db at a time. This was a big problem since we only had enough resources to on board 2-4 customers a month meaning slow growth.
I realized when looking at different customers databases that although the data was badly captured - it was consistently badly captured similar to how crap file names will usually contain the letters 'asd' because its typed with the left hand.
I then wrote an algorithm that fuzzy matched against our data and the past matches of other customers data creating a ranking algorithm similar to google page search. After auto matching the majority of results the top 10 ranked search results for each product on their db is shown to a human 1 at a time and they either click the the correct result or select "no match" and repeat until it is done at which point the algo will include the captured data in ranking future results.
It now takes a single staff member 1-2 hours to fully on board a customer with 10-15k products and will continue to get faster and adapt to changes in language and naming conventions. Making it learn wasn't really my intention at the time and more a side effect of what I was trying to achieve. Completely blew my mind. -
in personal projects, it is really easy to tell when I'm horny. you will find variable names like
- hotVar
- sluttyVar
- FUCK_ME_VAR
15 minutes after that commit naming becomes
- var1 -
I really really hope that no one post this,a friend texted it to me and I wanted to share it because made my day.
Idk where it comes, so feel free if know where this came from to post it:
//FUN PART HERE
# Do not refactor, it is a bad practice. YOLO
# Not understanding why or how something works is always good. YOLO
# Do not ever test your code yourself, just ask. YOLO
# No one is going to read your code, at any point don’t comment. YOLO
# Why do it the easy way when you can reinvent the wheel? Future-proofing is for pussies. YOLO
# Do not read the documentation. YOLO
# Do not waste time with gists. YOLO
# Do not write specs. YOLO also matches to YDD (YOLO DRIVEN DEVELOPMENT)
# Do not use naming conventions. YOLO
# Paying for online tutorials is always better than just searching and reading. YOLO
# You always use production as an environment. YOLO
# Don’t describe what you’re trying to do, just ask random questions on how to do it. YOLO
# Don’t indent. YOLO
# Version control systems are for wussies. YOLO
# Developing on a system similar to the deployment system is for wussies! YOLO
# I don’t always test my code, but when I do, I do it in production. YOLO
# Real men deploy with ftp. YOLO
So YOLO Driven Development isn’t your style? Okay, here are a few more hilarious IT methodologies to get on board with.
*The Pigeon Methodology*
Boss flies in, shits all over everything, then flies away.
*ADD (Asshole Driven Development)*
An old favourite, which outlines any team where the biggest jerk makes all the big decisions. Wisdom, process and logic are not the factory default.
*NDAD (No Developers Allowed in Decisions)*
Methodology Developers of all kinds are strictly forbidden when it comes to decisions regarding entire projects, from back end design to deadlines, because middle and top management know exactly what they want, how it should be done, and how long it will take.
*FDD (Fear Driven Development)*
The analysis paralysis that can slow an entire project down, with developments afraid to make mistakes, break the build, or cause bugs. The source of a developer’s anxiety could be attributed to a failure in sharing information, or by implicating that team members are replaceable.
*CYAE (Cover Your Ass Engineering)*
As Scott Berkun so eloquently put it, the driving force behind most individual efforts is making sure that when the shit hits the fan, you are not to blame.2 -
My biggest pet peeve is that too many developers don't realize that "regex != regular expressions", probably because of bad naming and bad documentation. It's easy to assume that they're the same, but most regex syntaxes today are actually at least context-free grammars, since they support backreferences.7
-
So typescript 4.5 beta is out .... holy moly what did those guys smoke? 🧐🤨🤪
They keep adding stuff on top, that nobody needs. But they don't fix the stuff that is broken (like emitting broken prefix-paths ...🤦)
Imho, they should focus on the overall development experience, make it easy an consistent to setup a proper multi-module project with linter, auto-formatter, folder structure, file naming.
And please fix this ugly #private fields - just ignore this mess of a spec and emit TS private fields as #private fields. That's the only logical way. Everything else is BS.8 -
OK here's lang, very easy.
set lines, (split "\n", orc fname). ; ORC -- open, write, close
map lines, strip.
grep lines, filter blanks.
map lines, # lineproc:
- on line match, qr~^# \s* (?<name> [\a]+) \((?<type> [\a+])\) \s*:\s*~x.
· · * have type or default type.
· · * set dst, name or new type name.
- on line match, qr~^\- (?<name> .*)~x.
· · * dst->cur eq dst->[name] eq new list name.
- on line match qr~^\* (?<item> .*)~x.
· · * push dst->cur, item.
- else
· · * cat dst->cur, line or throw fuk.
I'm skipping over a couple edge cases (no dst/cur, I be throw fuk for brevity) but you get the gist of it maybe.
Anyway what's this for? Lists, like so:
```rol
# dst (type):
- attr:
· · * hi im item one in list
· · · still item one lmao.
· · * hi im item two in list.
```
That gives `dst { attr: [item_1, item_2] }`. There's another bit I'm omitting to make this recursive so as to allow for nested dicts, but nevermind that it's a tree you get it right.
So what it's lame. Yes. Let's smoke some crack now I can add preprocessor in subclass:
```
# dst (cracktype):
$:%fn args;>
(text)
$:/fn;>
```
That will call `fn text,args` to process text __before__ lineproc, `fn` is just callback from callback table in Nebraska maybe.
$:peso;> syntax is just so text can contain funstuff OK.
I like <fn args /> better, and $:this;> is just stuff no one ever writes so it's safe to use.
Want to reference object in text too, what? `{$obj [fn args]}` anywhere in text to make call, now can do database lookup so naming be important. Have import mechanism to fetch collections, can't bother showing.
Anyway what's the point I dunno, just copying and pasting from local library to pack entire app in single html file. Why? Can't remember; doesn't matter.
Also can convert to json but I prefer my own version of it.
Called jargon.
Same thing but no quotes just because so `obj {attr:[(value), (value)]}`.
Now eat baguettes.
Have a nice wallop.1