Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
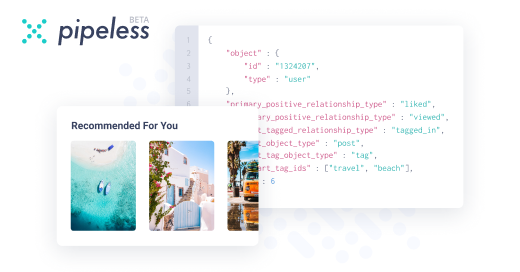
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "sqrt"
-
POSTMORTEM
"4096 bit ~ 96 hours is what he said.
IDK why, but when he took the challenge, he posted that it'd take 36 hours"
As @cbsa wrote, and nitwhiz wrote "but the statement was that op's i3 did it in 11 hours. So there must be a result already, which can be verified?"
I added time because I was in the middle of a port involving ArbFloat so I could get arbitrary precision. I had a crude desmos graph doing projections on what I'd already factored in order to get an idea of how long it'd take to do larger
bit lengths
@p100sch speculated on the walked back time, and overstating the rig capabilities. Instead I spent a lot of time trying to get it 'just-so'.
Worse, because I had to resort to "Decimal" in python (and am currently experimenting with the same in Julia), both of which are immutable types, the GC was taking > 25% of the cpu time.
Performancewise, the numbers I cited in the actual thread, as of this time:
largest product factored was 32bit, 1855526741 * 2163967087, took 1116.111s in python.
Julia build used a slightly different method, & managed to factor a 27 bit number, 103147223 * 88789957 in 20.9s,
but this wasn't typical.
What surprised me was the variability. One bit length could take 100s or a couple thousand seconds even, and a product that was 1-2 bits longer could return a result in under a minute, sometimes in seconds.
This started cropping up, ironically, right after I posted the thread, whats a man to do?
So I started trying a bunch of things, some of which worked. Shameless as I am, I accepted the challenge. Things weren't perfect but it was going well enough. At that point I hadn't slept in 30~ hours so when I thought I had it I let it run and went to bed. 5 AM comes, I check the program. Still calculating, and way overshot. Fuuuuuuccc...
So here we are now and it's say to safe the worlds not gonna burn if I explain it seeing as it doesn't work, or at least only some of the time.
Others people, much smarter than me, mentioned it may be a means of finding more secure pairs, and maybe so, I'm not familiar enough to know.
For everyone that followed, commented, those who contributed, even the doubters who kept a sanity check on this without whom this would have been an even bigger embarassement, and the people with their pins and tactical dots, thanks.
So here it is.
A few assumptions first.
Assuming p = the product,
a = some prime,
b = another prime,
and r = a/b (where a is smaller than b)
w = 1/sqrt(p)
(also experimented with w = 1/sqrt(p)*2 but I kept overshooting my a very small margin)
x = a/p
y = b/p
1. for every two numbers, there is a ratio (r) that you can search for among the decimals, starting at 1.0, counting down. You can use this to find the original factors e.x. p*r=n, p/n=m (assuming the product has only two factors), instead of having to do a sieve.
2. You don't need the first number you find to be the precise value of a factor (we're doing floating point math), a large subset of decimal values for the value of a or b will naturally 'fall' into the value of a (or b) + some fractional number, which is lost. Some of you will object, "But if thats wrong, your result will be wrong!" but hear me out.
3. You round for the first factor 'found', and from there, you take the result and do p/a to get b. If 'a' is actually a factor of p, then mod(b, 1) == 0, and then naturally, a*b SHOULD equal p.
If not, you throw out both numbers, rinse and repeat.
Now I knew this this could be faster. Realized the finer the representation, the less important the fractional digits further right in the number were, it was just a matter of how much precision I could AFFORD to lose and still get an accurate result for r*p=a.
Fast forward, lot of experimentation, was hitting a lot of worst case time complexities, where the most significant digits had a bunch of zeroes in front of them so starting at 1.0 was a no go in many situations. Started looking and realized
I didn't NEED the ratio of a/b, I just needed the ratio of a to p.
Intuitively it made sense, but starting at 1.0 was blowing up the calculation time, and this made it so much worse.
I realized if I could start at r=1/sqrt(p) instead, and that because of certain properties, the fractional result of this, r, would ALWAYS be 1. close to one of the factors fractional value of n/p, and 2. it looked like it was guaranteed that r=1/sqrt(p) would ALWAYS be less than at least one of the primes, putting a bound on worst case.
The final result in executable pseudo code (python lol) looks something like the above variables plus
while w >= 0.0:
if (p / round(w*p)) % 1 == 0:
x = round(w*p)
y = p / round(w*p)
if x*y == p:
print("factors found!")
print(x)
print(y)
break
w = w + i
Still working but if anyone sees obvious problems I'd LOVE to hear about it.36 -
At a job interview.
Them: Can you please write a function that calculates fibonacci numbers on the whiteboard please.
Me:
fib=_=>($=>$.round(($.pow((1+$.sqrt(5))/2,_)-$.pow(-2/(1+$.sqrt(5)),_))/$.sqrt(5)))(Math)18 -
Remember the post about bruce's constant?(4.5099806905005)
Well apparently theres a convergent series for it found all the way back in 2015.
Apparently its an actual thing. Which connects e to the square root of this series.
And it converges on (bruce-1)**0.5.
I confirmed it myself.
The two people who found the series that converges are N. J. A. Sloane and Hiroaki Yamanouchi
Thank you Sloane and Hiroaki!
The actual formula is a series of embedded square roots with the repeating numbers 1,4,2,8,5,7
like so...
sqrt(1+sqrt(4+sqrt(2+sqrt(8+sqrt...
What this means is you can find e using this series.
All you do is run the series, raise by a power of 2, add 1, calculate J and K like so
J = log(2, 1.333333333333333) / log(2, 2)
K = log(2, 1.333333333333333) / log(2, 3)
then calculate (J+K)-(bruce-1)
and out pops our buddy e:
2.7182818284591317
I guess I bullshitted myself for so long, that I didn't believe people like scor when they said they legit witnessed by math skills grow.
Or maybe a blind squirrel occasionally DOES find a nut.
Pretty cool find either way.13 -
Behold the monstrosity regex my transpiler produces!
/val|var|[1-9]{1,32}|\+|\-|\*|s\/s|>>|=|;|(["'])(?:(?=(\\?))\2.)*?\1|print\(|log\(|sqrt\(|input\(|strToArray\(|httpGet\(|if\(|else|{|}|s==s|s>=s|s<=s|s>s|s<s|s&&s|\|\||!|;|\(|\)|\[|\]| |\w+/gi13 -
wk48 best question:
My go to question for dev interviews is how do you find all prime numbers from 2 to x because there's so much room for optimisation.
Start with the basics, loop over every number and check if it's divisible by any number less than it, then record the prime numbers and check only those, then move to something like the sieve of eratosthenes then reduce the problem space and only iterate through 2 to sqrt(x)5 -
More masturbation with numbers.
If you take some product p,
and do
√p**(1/p)
and it's factors
a**(1/p)
and
b**(1/p)
you might find something interesting.
Take for example
a=21977
b=43331
p=a*b=952285387
(√p)**(1/p) = 1.0000000108551363
a**(1/p) = 1.0000000104986928
b**(1/p) = 1.0000000112115799
More often then not, a, b, or both, will share one or two of the most significant digits in the mantissa, as the root of p.
It doesn't always work, but it seems to be true more often than you might expect.
This is probably obvious in hindsight but I still think it's cool.
In some instances if you then do, say
sqrt(log(p, 1.000000010)), it comes pretty close to the original factors, but thats really hit or miss.8 -
Everyone argues about the perfect date, so I searched and found it using complex machine learning, a lot of trial and error, and too much alcohol:
'#76ab%Y%Y@98:%M%D%h@()%m&%m%Y%D%Y€¥$¢%M%h+%s-%s%%'
Where:
- %Y stands for one number of the last year
- %M stands for one number of the following month
- %D stands for one number (09 are two numbers for example) of SQRT((CURRENT_DAY^7)/3)
- %h stands for one number of the hour next evening(12h system)
- %% stands for either 7 or 3, 7 means that the hour(%h) is a.m., 3 means that the hour is p.m.
- %m stands for the minute the next solar eclipse will happen
- %s stands for one number of the second you will hate yourself to have this system implemented.
How to use it im 3 simple steps:
1. Implement it using ???
2. ?????
3. Profit? -
The first fruits of almost five years of labor:
7.8% of semiprimes give the magnitude of their lowest prime factor via the following equation:
((p/(((((p/(10**(Mag(p)-1))).sqrt())-x) + x)*w))/10)
I've also learned, given exponents of some variables, to relate other variables to them on a curve to better sense make of the larger algebraic structure. This has mostly been stumbling in the dark but after a while it has become easier to translate these into methods that allow plugging in one known variable to derive an unknown in a series of products.
For example I have a series of variables d4a, d4u, d4z, d4omega, etc, and these are translateable now, through insights that become various methods, into other types of (non-d4) series. What these variables actually represent is less relevant, only that it is possible to translate between them.
I've been doing some initial learning about neural nets (implementation, rather than theoretics as I normally read about). I'm thinking what I might do is build a GPT style sequence generator, and train it on the 'unknowns' from semiprime products with known factors.
The whole point of the project is that a bunch of internal variables can easily be derived, (d4a, c/d4, u*v) from a product, its root, and its mantissa, that relate to *unknown* variables--unknown variables such as u, v, c, and d4, that if known directly give a constant time answer to the factors of the original product.
I think theres sufficient data at this point to train such a machine, I just don't think I'm up to it yet because I'm lacking in the calculus department.
2000+ variables that are derivable from a product, without knowing its factors, which are themselves products of unknown variables derived from the internal algebraic relations of a product--this ought to be enough of an attack surface to do something with.
I'm willing to collaborate with someone familiar with recurrent neural nets and get them up to speed through telegram/element/discord if they're willing to do the setup and training for a neural net of this sort, one that can tease out hidden relationships and map known variables to the unknown set for a given product.17 -
Tomorrow i have school starting.
Which inspires me to rant about how school fails. Ill omit the "arguments" - feel free to append arguments for my words in the comments. Lol
Dont get be wrong. I LOVE acquiring knowledge. And this is where my first point starts : PACE. My class is basically an assortiment of dumbfucks who dont understand anything without "learning by heart over the course of several weeks"
Ill give you a concrete example.
Our maths teacher wanted to make us think scientifically. So he invented a new type of numbers "root 6 numbers" that are formed like so:
a + b * sqrt(6)
Now he wants us to find out wether the sum of two root 6 numbers is also a root six number. this is all dandy, BUT CLASSMATES STILL DIDNT GET WHAT ROOT 6 NUMBERS ARE, EVEN AFTER SEVERAL EXPRANATIONS. Worse: they went to the main teacher to blacken the math teacher.
Another example would be the time our class needed to understand functions(x) : 4 weeks. Ik, as a programmer i have some ease, but four weeks is a bit too much.
Because of this slow pace, i am irreversibly bored of and in school.
And this leads to another problem: homework. Since i know most of the stuff (the few things i dont get at school, i research at home) the homework are useless to me and since the others dont get much, the homeworks are often more than abundant {in a negative way}.
So i dont do them - but that makes teachers disregard me. Which im sickened of.
Worse: often i dont get overly good grades (i honestly have no clue why. I know everything and go over most of the stuff with my menthor),which empowers teacher of the argument of "you are not good enuff, so you cant read in class".
It would be JUST FINE if the only problem were teachers - but my peers are horrible too.
I know our brains are growing, but thats no reason for being stupid.
I literally get told that i need to stop wearing shorts because they look horrible.
Yep. Also, most people think they are empowered of teaching me and talking about my defaillance - because they do their homework. Even though they know i know stuff better than them.
Now to one of the worst issues: a group work where we had to de a Radio report. The guy (the one who thinks he is intelligent BECAUSE he has good grades) invited himself and his gf to me, he wanted me to translate 22 pages from german to english (because he was too lazy to write in german), wanted me to do audiorecording, audioediting and writing of a report. When i left the group because i was called "weakest link" he spread the word that i he had done everythinh and that because i left his group had failed (noticed the flow in logic?)
NOW everybody thinks of me as stupid weirdo. And honestly - i think i will stop listening to them. Ive always hated people, i dont need a significant other.
Even though this will come with the secondary effect of me being gossiped at.
But honestly its fine.
You might have noticed my elojquent way of expressing myseld. I did that in order to show that i am, despite my grades, overly proficient in english
Ok. So now comes the conclusion. What should i do? Do you Think that i am like that because im pubescent myself? How can i stop having nightmares of every possible social situotion that could occur?
Does this have to do with me being a dev?
Well. ありがとう for reading.18 -
heres something interesting:
The golden ratio is 1.618...
If you're not familiar with it, doing 1/goldenratio
the result is 0.618...
It gives you back the float component exactly.
Discovered that it is actually part of a series.
First of all:
2-(((5-sqrt(5))/2)-1) =
1.618033988749895 -> thats our golden ratio
In other words:
(2%gold) =
0.381966011250106
While:
((5-sqrt(5))/2) =
1.381966011250105
Ok, now we're getting somewhere. We can turn these into variables
First of all, lets see if we can get the golden ratio back out:
2-(((5-sqrt(5))/2)-1) = 1.618033988749895
Okay good.
The formula looks something like
j-(((i-sqrt(i))/2)-1)
Where j = (i*2)+1
That means we can easily figure out what j we need from our i value. (i-1)/2 = j
We run it back far enough we get
1-(((3-sqrt(3))/2)-1) =
1.3660254037844386
Thats the golden ratios little brother. Doesn't look anything like it, but it is part of the series.
And I found a boat load of research documents scattered *all* over the net, where this number and others in the series inexplicably crop up in power series, in chemistry, and elsewhere. Just looks like random floats if you don't know better.
We can actually go lower in the series:
0.5-(((2-sqrt(2))/2)-1)
1.2071067811865475
At the lowest positive value for j, we get
0-(((1-sqrt(1))/2)-1) = 1
It's kinda elegant.
I even wrote a little script to do the conversions:
def gr(k):
....i = k
....j = (i-1)/2
....return j-(((i-sqrt(abs(i)))/2)-1)
The dots are so devrant doesn't break pythons formatting.3 -
This morning, I tried to abstract myself from my computer while trying to calculate sqrt(1.81).
I came up with what I thought to be a genius method. I tried to find B such as (1+B)^2=1.81. Then I ended up calculating the discriminant of 1+2B+B^2 and had 4*1.81. Sounded funny at first, but upon calculating the positive solution amongst the 2 possible ones, I ended up with (-2+2sqrt(1.81))/2 = ... sqrt(1.81)-1. Upon replacing in the initial equation, one gets (1+sqrt(1.81)-1)^2 = (sqrt(1.81))^2 = 1.81.
I'm sorry for having let you down, dear pasokon. Please forgive me.2 -
When white space isn't that significant.
disc=b*b-4*a*c;if(disc<0){
num_sol=0;}else{t0=-b/a;if(
disc==0){num_sol=1;sol0=t0/2
;}else{num_sol=2;t1=sqrt(disc/a;
sol0=(t0+t1)/2;sol1=(t0-t1)/2;}}2