Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
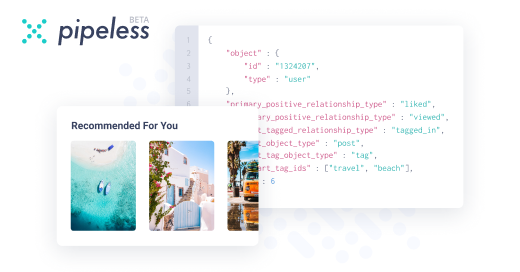
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "string processing"
-
/*
It's a pretty long rant. Hope you didn't get bored :P
*/
So I have this friend of mine who has learnt Python at good level (that's what he says) and is with me in all classes in college. I have worked with C, C++, C# and Java only and hated Python when it was taught (wk44).
So the following happened in the last 2 weeks:
Once he wrote a Python function in terminal just returning a hard coded string (lame right) and will show me how cool is it and that it is sooo much easier.
Whenever we do a mini project together he will force that we use Python. Even in Image processing when everyone is ready to work on Matlab, he insists that Python would be a better option.
We asked that this XYZ is very easy to implement on Matlab.
We then had to listen about the large and great community of Python and that it has Libraries for everything and that it is the greatest programming language ever.
One day he saw my C# project for DFA and NFA simulation which was the greatest project I have "completed" myself, and went like "Hmph, if I was you, I would use python and make a more "professional" code" (then went on arguing as always)
This happened today in Networking lab-
(Sockets was taught and we are expected to learn its programming aspects)
All students: Open linuxhowtos.org and start reading on socket programming
He : Opens some websites and downloads books on Networking with Python or someting
Now while I am reading the documentation of sockets and bind, he opens spider IDE, copy-paste the code in the book and start bugging ME that he is getting all these errors like literally showing me those errors and whining about all those problems.
Me: We are supposed to learn this in C. Here take a look at this link.
HE: No I'll use Python cuz it is better than your C. It has libraries for everything and is much easier.
Me: Alright whatever I am fed up, do whatever you want11 -
A story from around 2005:
Customer laying out specifications: “We expect this software to need to last 25 years or so, and it will need to keep historical file processing data by dates for at least that long, assume storage is no issue.”
Devs at the time: “look best I can do is support that start with 200 or 201, anything else is really too much to ask. Also understanding how to work with dates at all and not just string manipulation is waaaaayyy hard yo.”
Fuck you lazy motherfuckers. This is why people thought Y2K would be a problem. -
Wanna know about hacks? I'll tell you. There is a peace of software called SugarCRM. It has OAuth2 provider implementation. I was assigned to write OAuth2 consumer for it.
It turned out they just failed to make it right.
The list of hacks:
* Hack on standard Authentication header. They use custom.
* Hack on "scope". They send null which is standard violation. So it is replaced to empty string before response processing starts.
* This is my favorite. Refresh token simply doesn't work. So we need to store user's credentials in memory to be able to reauthenticate user transparently.2 -
I am trying to "invent" secure client-side authentication where all data are stored in browser encrypted and only accessible with the correct password. My question is, what is your opinion about my idea. If you think it is not secure or there is possible backdoor, let me know.
// INPUT:
- test string (hidden, random, random length)
- password
- password again
// THEN:
- hash test string with sha-512
- encrypt test string with password
- save hash of test string
// AUTH:
- decrypt test string
- hash decrypted string with sha-512
- compare hashes
- create password hash sha-512 (and delete password from memory, so you cannot get it somehow - possible hole here because hash is reversible with brute force)
// DATA PROCESSING
- encrypt/decrypt with password hash as secret (AES-256)
Thanks!
EDIT: Maybe some salt for test string would be nice8 -
StackOverflow locked my account. I'm hoping someone here might be kind enough to help me with a bash script I'm "bashing" my head with. Actually, it's zsh on MacOS if it makes any difference.
I have an input file. Four lines. No blank lines. Each of the four lines has two strings of text delimited by a tab. Each string on either side of the tab is either one word with no spaces or a bunch of words with spaces. Like this (using <tab> as a placeholder here on Devrant for where the tab actually is)
ABC<tab>DEF
GHI<tab>jkl mno pq
RST<tab>UV
wx<tab>Yz
I need to open and read the file, separate them into key-value pairs, and put them into an array for processing. I have this script to do that:
# Get input arguments
search_string_file="$1"
file_path="$2"
# Read search strings and corresponding names from the file and store in arrays
search_strings=()
search_names=()
# Read search strings and corresponding names from the file and store in arrays
while IFS= read -r line || [[ -n "$line" ]]; do
echo "Line: $line"
search_string=$(echo "$line" | awk -F'\t' '{print $1}')
name=$(echo "$line" | awk -F'\t' '{print $2}')
search_strings+=("$search_string")
search_names+=("$name")
done < "$search_string_file"
# Debug: Print the entire array of search strings
echo "Search strings array:"
for (( i=0; i<${#search_strings[@]}; i++ )); do
echo "[$i] ${search_strings[$i]} -- ${search_names[$i]}"
done
However, in the output, I get the following:
Line: ABC<tab>DEF
Line: GHI<tab>jkl mno pq
Line: RST<tab>UV
Line: wx<tab>Yz
Search strings array:
[0] --
[1] ABC -- DEF
[2] GHI -- jkl mno pq
[3] RST -- UV
That's it. I seem to be off by one because that last line...
Line: wx<tab>Yz
never gets added to the array. What I need it to be is:
[0] ABC -- DEF
[1] GHI -- jkl mno pq
[2] RST -- UV
[3] wx -- Yz
What am I doing wrong here?
Thanks.17 -
Today I made a php script to scrap a site.
And I needed to use str_replace in a string to cancel out some values.
Instead of doing simple str_replace I used explode func to separate them with spaces (without any comments on how or why the fuck I m using an explode instead of a str_replace).
Later, I used $p[1] for further processing. -
Oh god why!?
Somehow I decided that it would be nice to have a proper spell&grammar checker in VS Code for me and my friends to write our reports in Latex with.
Decided I wanted multiple language support, so I turned to language tool.
GOD is this thing slow as a very unmotivated snail!
3s to process 9 phrases!? And then add one second to pack the results in a JSON string!? An option that I'm still very grateful exists, but why the fuck would you disallow line-by-line processing, which you expressly recommend for IDEs, and which cuts processing time by 30%, when JSON output is asked!?
Well, serves me right for thinking for even a second that a Java program could have decent performance...
This whole thing is starting to look like it's not gonna be nearly as fun ss I anticipated it to be.2 -
I've been helping a friend of mine with his postgraduate project the last 3 months.
It was a Java based program made in Processing. Though I am not a Java developer and I never used processing before, it wasn't that hard to write the logic of the program.
I noticed that sometimes Java made me use loops for almost everything.
Also I had to communicate between server and client via JSON but I had to write it manually as string due to the lack of keys in Java.
The main trial though was with the logic of the project. It was supposed to be made as a framework to be extended from custom user classes. I had to change the core classes I made many times because the user class had methods that should run while the parent class didn't have them declared. That could be my fault for not knowing how to write desktop application framework but you can't expect a framework to be extended in a compiled state, or so I think. Processing on the other hand doesn't seem to like the idea of an external java library. At least it didn't workout for me, it should be able to work normally.
In the end the project was never as completed as we wanted. It could rum a basic sim but we hadn't the time to test other possibilities. -
taking hours for string/object conversion to handle cookie based favourites depending on number of clicks left me considering being dumb, finally seeing just 15 lines of code.
-
Anyone tried converting speech waveforms to some type of image and then using those as training data for a stable diffusion model?
Hypothetically it should generate "ultrarealistic" waveforms for phonemes, for any given style of voice. The training labels are naturally the words or phonemes themselves, in text format (well, embedding vectors fwiw)
After that it's a matter of testing text-to-image, which should generate the relevant phonemes as images of waveforms (or your given visual representation, however you choose to pack it)
I would have tried this myself but I only have 3gb vram.
Even rudimentary voice generation that produces recognizable words from text input, would be interesting to see implemented and maybe a first for SD.
In other news:
Implementing SQL for an identity explorer. Basically the system generates sets of values for given known identities, and stores the formulas as strings, along with the values.
For any given value test set we can then cross reference to look up equivalent identities. And then we can test if these same identities hold for other test sets of actual variable values. If not, the identity string cam be removed, or gophered elsewhere in the database for further exploration and experimentation.
I'm hoping by doing this, I can somewhat automate the process of finding identities, instead of relying on logs and using the OS built-in text search for test value (which I can then look up in the files that show up, and cross reference the logged equations that produced those values), which I use to find new identities.
I was even considering processing the logs of equations and identities as some form of training data perhaps for a ML system that generates plausible new identities but that's a little outside my reach I think.
Finally, now that I know the new modular function converts semiprimes into numbers with larger factor trees, I'm thinking of writing a visual browser that maps the connections from factor tree to factor tree, making them expandable and collapsible, andallowong adjusting the formula and regenerating trees on the fly.7 -
Am I in developer hell already? A shitty project is about to come to an end (hopefully), or should I rather say: It needs to come to an end. But I am still quite lost in how to deal with it, hence procrastinating on it - making the deadline come closer and with it the realization that I'll probably have to rewrite almost everything. I'm not sure how, but I do know that the current code is a dumpster fire.
Basically what I need to do is dealing with the APIs of different payment providers/gateways (like PayPal, AmazonPay). For most cases I'll get a payment ID from the shop and need to act on it later, e.g. capture the authorized money in the case of a credit card transaction or do refunds (without user interaction, unless there is an error). Now at first I put something together where I try to abstract the payment information into two tables:
orders{1}<->{0..n}payments
payments{1}<->{1..n}paymentDetails
Unfortunately trying to abstract the different payment methods and to squeeze them (and their different possible stati and functions) in these tables was not very successful, it's a total mess with magic numbers, half-broken behavior and without any consideration for partial payments/captures or unfinished requests (i.e. if there is an exception before the response is dealt with, there is no indication that anything has ever been sent). Also the current amount is calculated through the history of the paymentDetails table, which basically works differently for each payment type.
How to fix this mess in a way that I'll still have a job by next week?
I'm trying to improve the db schema first, as I think my biggest problems are lying there. Through some research I've come across a recommendation for making payment type specific subtables (with a magic number/string in the main table to prevent having to look up all subtables). That way I can record what I send and receive without having to abstract it too much, so I'll have an acceptable transaction log. The paymentDetails table can be removed (necessary fields go to the payments table). The payments table gets multiple fields for the amount (differentiating between open, authorized, captured, processing and refunded values) and always reflects the current status.
Tables:
payments
paymentRequestsPaypal
paymentRequestsAmazonpay
paymentRequestsXyz
I think I'm going in the right direction here. hm. Maybe there's some light at the end of this long, dark tunnel. Or a train. I'll have two days to find out.question kill me already send help thank you for being my rubber duck payment gateways deadline approaching rant/question burnout6 -
Today I started a project in which I must parse and extract some features from orders. Features can be product names, options, custom data and more and then do some validations/processing.
The (main) problem ? All I have is a String per order and of course most of the product/options have either change or been deleted.
I want to sudo rm -rf myself 😞 -
OK, so I've been working on processing a Japanese dictionary file and things are going smoothly for the most part. Out of ~185,000 entries, I've got 35 that are still causing problems.
The error I'm getting is "Incorrect string value '\xF0\xA4\xAD\xAF' for column...". I've checked all of my encoding and collation settings, and I'm pretty sure I've got it set to properly implement all of Unicode (as well as it does, anyway), as shown in the image attached. My suspicion is the problem characters are likely among the JIS X 0213 character set; in either case we're clearly dealing with a 4-byte character encoding issue here.
If needed I can attach a flag in the database and base64 encode these particular entries so the data isn't lost, but I'd like to just get it to handle the data properly in the first place if possible.
Anyone have any ideas on other items I can check to resolve the error?10