Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
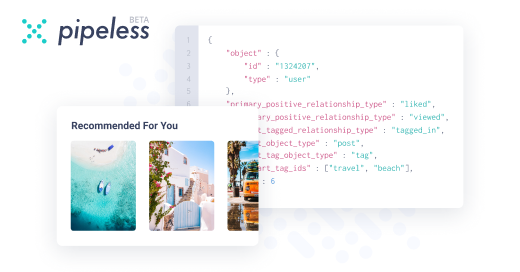
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "too many libraries"
-
!rant
This was over a year ago now, but my first PR at my current job was +6,249/-1,545,334 loc. Here is how that happened... When I joined the company and saw the code I was supposed to work on I kind of freaked out. The project was set up in the most ass-backward way with some sort of bootstrap boilerplate sample app thing with its own build process inside a subfolder of the main angular project. The angular app used all the CSS, fonts, icons, etc. from the boilerplate app and referenced the assets directly. If you needed to make changes to the CSS, fonts, icons, etc you would need to cd into the boilerplate app directory, make the changes, run a Gulp build that compiled things there, then cd back to the main directory and run Grunt build (thats right, both grunt and gulp) that then built the angular app and referenced the compiled assets inside the boilerplate directory. One simple CSS change would take 2 minutes to test at minimum.
I told them I needed at least a week to overhaul the app before I felt like I could do any real work. Here were the horrors I found along the way.
- All compiled (unminified) assets (both CSS and JS) were committed to git, including vendor code such as jQuery and Bootstrap.
- All bower components were committed to git (ALL their source code, documentation, etc, not just the one dist/minified JS file we referenced).
- The Grunt build was set up by someone who had no idea what they were doing. Every SINGLE file or dependency that needed to be copied to the build folder was listed one by one in a HUGE config.json file instead of using pattern matching like `assets/images/*`.
- All the example code from the boilerplate and multiple jQuery spaghetti sample apps from the boilerplate were committed to git, as well as ALL the documentation too. There was literally a `git clone` of the boilerplate repo inside a folder in the app.
- There were two separate copies of Bootstrap 3 being compiled from source. One inside the boilerplate folder and one at the angular app level. They were both included on the page, so literally every single CSS rule was overridden by the second copy of bootstrap. Oh, and because bootstrap source was included and commited and built from source, the actual bootstrap source files had been edited by developers to change styles (instead of overriding them) so there was no replacing it with an OOTB minified version.
- It is an angular app but there were multiple jQuery libraries included and relied upon and used for actual in-app functionality behavior. And, beyond that, even though angular includes many native ways to do XHR requests (using $resource or $http), there were numerous places in the app where there were `XMLHttpRequest`s intermixed with angular code.
- There was no live reloading for local development, meaning if I wanted to make one CSS change I had to stop my server, run a build, start again (about 2 minutes total). They seemed to think this was fine.
- All this monstrosity was handled by a single massive Gruntfile that was over 2000loc. When all my hacking and slashing was done, I reduced this to ~140loc.
- There were developer's (I use that term loosely) *PERSONAL AWS ACCESS KEYS* hardcoded into the source code (remember, this is a web end app, so this was in every user's browser) in order to do file uploads. Of course when I checked in AWS, those keys had full admin access to absolutely everything in AWS.
- The entire unminified AWS Javascript SDK was included on the page and not used or referenced (~1.5mb)
- There was no error handling or reporting. An API error would just result in nothing happening on the front end, so the user would usually just click and click again, re-triggering the same error. There was also no error reporting software installed (NewRelic, Rollbar, etc) so we had no idea when our users encountered errors on the front end. The previous developers would literally guide users who were experiencing issues through opening their console in dev tools and have them screenshot the error and send it to them.
- I could go on and on...
This is why you hire a real front-end engineer to build your web app instead of the cheapest contractors you can find from Ukraine.19 -
We're using a ticket system at work that a local company wrote specifically for IT-support companies. It's missing so many (to us) essential features that they flat out ignored the feature requests for. I started dissecting their front-end code to find ways to get the site to do what we want and find a lot of ugly code.
Stuff like if(!confirm("blablabla") == false) and whole JavaScript libraries just to perform one task in one page that are loaded on every page you visit, complaining in the js console that they are loaded in the wrong order. It also uses a websocket on a completely arbitrary port making it impossible to work with it if you are on a restricted wifi. They flat out lie about their customers not wanting an offline app even though their communications platform on which they got asked this question once again got swarmed with big customers disagreeing as the mobile perofrmance and design of the mobile webpage is just atrocious.
So i dig farther and farthee adding all the features we want into a userscript with a beat little 'custom namespace' i make pretty good progress until i find a site that does asynchronous loading of its subpages all of a sudden. They never do that anywhere else. Injecting code into the overcomolicated jQuery mess that they call code is impossible to me, so i track changes via a mutationObserver (awesome stuff for userscripts, never heard of it before) and get that running too.
The userscript got such a volume of functions in such a short time that my boss even used it to demonstrate to them what we want and asked them why they couldn't do it in a reasonable timeframe.
All in all I'm pretty proud if the script, but i hate that software companies that write such a mess of code in different coding styles all over the place even get a foot into the door.
And that's just the code part: They very veeeery often just break stuff in updates that then require multiple hotfixes throughout the day after we complain about it. These errors even go so far to break functionality completely or just throw 500s in our face. It really gives you the impression that they are not testing that thing at all.
And the worst: They actively encourage their trainees to write as much code as possible to get paid more than their contract says, so of course they just break stuff all the time to write as much as possible.
Where did i get that information you ask? They state it on ther fucking career page!
We also have reverse proxy in front of that page that manages the HTTPS encryption and Let's Encrypt renewal. Guess what: They internally check if the certificate on the machine is valid and the system refuses to work if it isn't. How do you upload a certificate to the system you asked? You don't! You have to mail it to them for them to SSH into the system and install it manually. When will that be possible you ask? SOON™.
At least after a while i got them to just disable the 'feature'.
While we are at 'features' (sorry for the bad structure): They have this genius 'smart redirect' feature that is supposed to throw you right back where you were once you're done editing something. Brilliant idea, how do they do it? Using a callback libk like everyone else? Noooo. A serverside database entry that only gets correctly updated half of the time. So while multitasking in multiple tabs because the performance of that thing almost forces you to makes it a whole lot worse you are not protected from it if you don't. Example: you did work on ticket A and save that. You get redirected to ticket B you worked on this morning even though its fucking 5 o' clock in the evening. So of course you get confused over wherever you selected the right ticket to begin with. So you have to check that almost everytime.
Alright, rant over.
Let's see if i beed to make another one after their big 'all feature requests on hold, UI redesign, everything will be fixed and much better'-update.5 -
writing library code is hard.
there are sooo many details that go into writing good libraries:
designing intuitive and powerful apis
deciding good api option defaults, disallowing or warning for illegal operations
knowing when to throw, knowing when to warn/log
handling edge cases
having good code coverage with tests that doesn't suck shit, while ensuring thry don't take a hundred years to run
making the code easy to read, to maintain, robust
and also not vulnerable, which is probably the most overlooked quality.
"too many classes, too little classes"
the functions do too much it's hard to follow them
or the functions are so well abstracted, that every function has 1 line of code, resulting in code that is even harder to understand or debug (have fun drowning in those immense stack traces)
don't forget to be disciplined about the documentation.
most of these things are
deeply affected by the ecosystem, the tools of the language you're writing this in:
like 5 years ago I hated coding in nodejs, because I didn't know about linters, and now we have tools like eslint or babel, so it's more passable now
but now dealing with webpack/babel configs and plugins can literally obliterate your asshole.
some languages don't even have a stable line by line debugger (hard pass for me)
then there's also the several phases of the project:
you first conceive the idea, the api, and try to implement it, write some md's of usage examples.
as you do that, you iterate on the api, you notice that it could better, so you redesign it. once, twice, thrice.
so at that point you're spending days, weeks on this side project, and your boss is like "what the fuck are you doing right now?"
then, you reach fuckinnnnng 0.1.0, with a "frozen" api, put it on github with a shitton of badges like the badge whore you are.
then you drop it on forums, and slack communities and irc, and what do you get?
half of the community wants to ban you for doing self promotion
the other half thinks either
a) your library api is shitty
b) has no real need for it
c) "why reinvent the wheel bruh"
that's one scenario,
the other scenario is the project starts to get traction.
people start to star it and shit.
but now you have one peoblem you didn't have before: humans.
all sorts of shit:
people treating you like shit as if they were premium users.
people posting majestically written issues with titles like "people help, me no work, here" with bodies like "HAAAAAAAAAALP".
and if you have the blessing to work in the current js ecosystem, issues like "this doesn't work with esm, unpkg, cdnjs, babel, webpack, parcel, buble, A BROWSER".
with some occasional lunatic complaining about IE 4 having a very weird, obscure bug.
not the best prospect either.3 -
Hey guys it's not a rant, but i feel this place might help...
I am a 20 yr old, second year guy ...have got some experience in core Java and after that, i have been doing android for 8months... Yeah , i coded some basic apps got my hands dirty on firebase, sql libraries and some connectivity...
Even got landed in an internship.
Today i feel myself to be an intermediate android dev , nd i know their are many things that can be learnt in android that i don't know..
But what after that?development as a carrier interests me, but i fear for a job security ... I could learn more of Android,maybe learn ios after that but their are always articles coming out that react is future, webapps will replace android and stuff like that...
I Have also heard stuff like companies today want to squeeze more out of their techs, so they want less and complete developers having experience in both web and mobile app designing and other stuff like that
Are you freakin kidding me? Android and ios alone are like drinking Pacific and indian ocean and to add web developing, its like drinking out every drop of ocean in the world.
I guess their are guys which exist with knowledge of all three, maybe I can cover them all too(someday) but that would take my whole clg life of 4 years..(I guess)
And no ,I don't have problems with that too.. I actually like developing but again i hear big words like cloud computing, AR,VR AI, data sciences, automation, graphics designing, game dev, and many more...
Basically i hear too much and i fear too much 😅 and i don't think closing my ears would be a good choice...
So, which ocean of carrier should i aim to go for?nd are my fears real? Do companies really prefer some web guy designing Amazon like apps over android-only guys like me?is automation nd templates really gonna take all we, developers jobs?should i look into ai/data sciences?
Well , i am a simple guy, who got his first pc at 17 so naturally, i am fascinated even by the working of a calculator app and anything relates to tech so am open to pursue my interests in any fields23 -
I was reluctant to try out flutter earlier on because of claims online stating that hybrid frameworks aren't there yet. That's one hell of a crap!
I fell in love with flutter after completing my first flutter app. Shit was just too easy. So many helpful libraries which has eased my overall workload lately.
We built a Native Android app which took 2months+ to complete and I just finished porting it to flutter for iOS and Android in 3 weeks. Boss was happy, Client was happy, I am freaking joyous, everybody is happy!
From the mouth of a Native Android Dev with over 5yr of exp. This shit called flutter is worthy of all the hype. I fucking kid you not!
I don't know about the past... I assume it was shitty then cus I also blasted it based on git issues but now it seems even more faster to build production worthy apps than anything I've encountered.4 -
Too many to count, but this one useless meeting stands out the most.
I was working as an outside dev for software corporation. I was hired as an UI dev although my skill set was UI/engineer/devops at the time.
we wrote a big chunk of 'documentation' (read word files explaining features) before the project even started, I had 2 sprints of just meetings. Everybody does nothing, while I set up the project, tuned configs, added testing libraries, linters, environments, instances, CI/CD etc.
When we started actual project we had at least 2 meetings that were 2-3 hours long on a daily basis, then I said : look guys, you are paying me just to sit here and listen to you, I would rather be working as we are behind the schedule and long meetings don't help us at all.
ok, but there is that one more meeting i have to be on.
So some senior architect(just a senior backend engineer as I found out later) who is really some kind of manager and didn't wrote code for like 10 years starts to roast devs from the team about documentation and architectural decisions. I was like second one that he attacked.
I explained why I think his opinion doesn't matter to me as he is explaining server side related issues and I'm on the client-side and if he wants to argue we can argue on actual client-side decisions I made.
He tried to discuss thinking that he is far superior to some noob UI developer (Which I wasn't, but he didn't know that).
I started asking some questions and soon he felt lost and offended. We ended that discussion with conclusion that I made my own decisions on the client-side. That lasted less than 10 minutes.
So I just sit there and eat popcorn for next 4 and half hours listening to their unnecessary discussions where some angry manager that did programing decades ago wanted to show that we are all noobs and stupid.
what a sad human being.
what a waste of time, but hey I got payed for this 5 hour meeting.1 -
I don't understand Laravel...
I'm just a software undergrad in my final year. Coming from JS side of things (Express, NextJS), I find Laravel so complex, and maybe unnessecarily complex?
Like, when I wanna learn Laravel, I understand the MVC structure. However, going deeper into it, there are libraries/names like
1. Vagrant
2. Facade
3. Artisan
4. Guard
5. Gate
6. Policies
ALL OF THESE
WHICH I DON'T UNDERSTAND HOW IT TIES TO THE FUCKING MVC STRUCTURE
I'm seriously giving up... My courses forces us to learn this framework, and I feel more and more inadequate because I have so many things to learn, including things for my FYP, which involves the use of NextJS. And can I mention HOW EASY AND MINIMALISTIC JS FRAMEWORKS ARE?
LIKE, I JUST WANNA MAKE A STUPID FUCKING APP MAN, WHY MUST I KNOW SHIT LIKE ARTISAN MAKE, WHAT THE FUCK VAGRANT IS, HOW GATES ARE RELATED TO POLICIES, HOW POLICIES RELATE TO VIEWS, WHY THE FUCK DOES FACADE EXIST, and other fucking stupid questions I need to ask in order to utilize Laravel correctly?
Don't even get me started on JETSTREAM, FORTIFY, LARAVEL/UI, BREEZE. Like, WHY THE FUCK CAN'T YOU JUST HAVE ONE SINGLE PATTERN, AND THEN HAVE GOOD TUTORIALS RELATED TO THAT ONE SINGLE THING?
I don't know, am I just stupid? Looking at Laravel, I feel like my braincells die more and more looking at the words used, the unusual terms, and the pain that comes with trying to learn it, because I don't have time. I'm going to fucking fail this subject because I have too much other stuff in my life to learn about.
I'm fucking tired man...35 -
Python devs and data analysts....
Do you recommend using pycharm for working with jupyter notebooks? I surely had a bad time with it.
I have been using many jetbrains softwares , and am a fan of their docs search and autocompletion. But I don't think there is a full support for jupyter jn it, because sometimes my graphs made using matploit or seaborn just brakes.
And some libraries have a lot of functions taking parameters as " *args, **kwargs " , I don't know what that means but those function take a lot of "value" parameters i guess?(like this: plt.figure(figsize=[13,6], axis=False) )
Pycharm also don't seem to have access to list of those arguments...
Are you having such problems too? Have you found some better ide with autocompletions and support for jupyter? Do tell.
(Ps: i know jupyter can be run directly on a browser, but as i said "auto completions and documentations" )5 -
I need guidance about my current situation.
I am perfectionist believing in OOP, preventing memory leak in advance, following clean code, best practices, constantly learning about new libraries to reduce custom implementation & improve efficiency.
So even a single bad variable name can trigger my nerves.
I am currently working in a half billion $ IT service company on a maintenance project of 8 year old Android app of security domain product of 1 of the top enterprise company of the world, which sold it to the many leading companies in the world in Govt service, banking, insurance sectors.
It's code quality is such a bad that I get panic attacks & nightmares daily.
Issues are like
- No apk obfuscation, source's everything is openbook, anybody can just unzip apk & open it in Android Studio to see the source.
- logs everywhere about method name invoked,
- static IV & salt for encryption.
- thousands of line code in God classes.
- Irrelevant method names compared to it's functionality.
- Even single item having list takes 2-3 seconds to load
- Lag in navigation between different features' screens.
- For even single thing like different dimension values for different density whole 100+ lines separate layout files for 6 types of densities are written.
- No modularized packages, every class is in single package & there are around 100+ classes.
Owner of the code, my team lead, is too terrified to change even single thing as he don't have coding maturity & no understanding of memory leak, clean code, OOP, in short typical IT 'service' company mentality.
Client is ill-informed or cost-cutting centric so no code review done by them in 8 years.
Feeling much frustrated as I can see it's like a bomb is waiting to blast anytime when some blackhat cracker will take advantage of this.
Need suggestions about this to tackle the situation.10 -
!rant
For all of youse that ever wanted to try out Common Lisp and do not know where to start (but are interested in getting some knowledge of Common Lisp) I recommend two things:
As an introductory tutorial:
https://lisperati.com/casting.html/
And as your dev environment:
https://portacle.github.io/
Notice that the dev environment in question is Emacs, regardless of how you might feel about it as a text editor, i can recommend just going through the portacle help that gives you some basic starting points regarding editing. Learn about splitting buffers, evaluating the code you are typing in order for it to appear in the Common Lisp REPL (this one comes with an environment known as SLIME which is very popular in the Lisp world) as well as saving and editing your files.
Portacle is self contained inside of one single directory, so if you by any chance already have an Emacs environment then do not worry, Portacle will not touch any of that. I will admit that as far as I am concerned, Emacs will probably be the biggest hurdle for most people not used to it.
Can I use VS Code? Yes, yes you can, but I am not familiar with setting up a VSCode dev environment for Emacs, or any other environment hat comes close to the live environment that emacs provides for this?
Why the fuck should I try Common Lisp or any Lisp for that matter? You do not have to, I happen to like it a lot and have built applications at work with a different dialect of Lisp known as Clojure which runs in the JVM, do I recommend it? Yeah I do, I love functional programming, Clojure is pretty pure on that (not haskell level imo though, but I am not using Haskell for anything other than academic purposes) and with clojure you get the entire repertoire of Java libraries at your disposal. Moving to Clojure was cake coming from Common Lisp.
Why Common Lisp then if you used Clojure in prod? Mostly historical reasons, I want to just let people know that ANSI Common Lisp has a lot of good things going for it, I selected Clojure since I already knew what I needed from the JVM, and parallelism and concurrency are baked into Clojure, which was a priority. While I could have done the same thing in Common Lisp, I wanted to turn in a deliverable as quickly as possible rather than building the entire thing by myself which would have taken longer (had one week)
Am I getting something out of learning Common Lisp? Depends on you, I am not bringing about the whole "it opens your mind" deal with Lisp dialects as most other people do inside of the community, although I did experience new perspectives as to what programming and a programming language could do, and had fun doing it, maybe you will as well.
Does Lisp stands for Lots of Irritating Superfluous Parentheses or Los in stupid parentheses? Yes, also for Lost of Insidious Silly Parentheses and Lisp is Perfect, use paredit (comes with Portacle) also, Lisp stands for Lisp Is Perfect. None of that List Processing bs, any other definition will do.
Are there any other books? Yes, the famous online text Practical Common Lisp can be easily read online for free, I would recommend the Lisperati tutorial first to get a feel for it since PCL demands more tedious study. There is also Common Lisp a gentle introduction. If you want to go the Clojure route try Clojure for the brave and true.
What about Scheme and the Structure and Interpretation of Computer Programs? Too academic for my taste, and if in Common Lisp you have to do a lot of things on your own, Scheme is a whole other beast. Simple and beautiful really, but I go for practical in terms of Lisp, thus I prefer Common Lisp.
how did you start with Lisp?
I was stupid and thought I should start with it after a failed attempt at learning C++, then Java, and then Javascript when I started programming years ago. I was overwhelmed, but I continued. Then I moved to other things. But always kept Common Lisp close to heart. I am also heavy into A.I, Lisp has a history there and it is used in a lot of new and sort of unknown projects dealing with Knowledge Reasoning and representation. It is also Alien tech that contains many things that just seem super interesting to me such as treating code as data and data as code (back-quoting, macros etc)
I need some inspiration man......show me something? Sure, look for a game called Kandria in youtube, the creator, Shimera (Nicolas Hafner) is an absolute genius in the world of Lisp and a true inspiration. He coded the game in Common Lisp, he is also the person behind portacle. If that were not enough, he might very well also be Shirakumo, another prominent member of the Common Lisp Community.
Ok, you got me, what is the first thing in common lisp that I should try after I install the portacle environment? go to the repl and evaluate this:
(+ 0.1 0.2)
Watch in awe at what you get.
In the truest and original sense of the phrase (MIT based) "happy hacking!"9 -
Finish my biggest project :)
In 2017 the project was counting 60k lines of code (which doesn't look as too many) but I was able to remove some libraries and make my custom system, now the project is counting 10k lines of code :)
My dream is to open in these months -
A Joke/Meme/Story. Sit down and enjoy
In my job we develop WebApps for any company that uses accounting stuff (like you must be wondering, all types of companies).
Some web developers may understand the problem with Internet Explorer and Bootstrap and some libraries 😂 and yes, we had a situaion where we had to put a message at the login to say that you must use Chrome or Firefox in order to use our system properly instead of Internet Explorer (unfortunately, too many factories in my city only use Internet Explorer)
The last week I had too much deadtime and I found this video (watch it from minute 0:55)
https://youtu.be/dfuMvkaDNfg
I laughed so hard 😂 it represents our situation with those Internet Explorer lovers 😂👊🏻
P.D. The video is in spanish, but don‘t worry. If you don‘t speak spanish, in few words, this video is about two roomies (alternative Bert and Ernie) and Bert is mad because Ernie installed Internet Explorer on Bert‘s laptop, so he ask him to uninstall it. Ernie uninstalled it, but he also erased disk C 😂joke/meme internet explorer compatibility bootstrap bert and ernie internet explorer sucks web development sesame street6 -
8 years old, first computer. 12 tears old first laptop. Around the time of bebo, I started messing with Photoshop making skins, then I made a website to put these skins on, after that I became involved with the SMF message board software, offering support, creating mods and themes. Eventually started working with individuals and businesses designing and building there websites, went to college got a taste of Java & vB, continued onto a degree and now I can program in Java, vB, C#, C, Javascript/Coffeescript, Node, PHP, Python and Bash with experience with too many libraries and frameworks to count, at 24 years of age going into the last year of my degree. I never really realised I wanted to become a dev. I just kind of naturally progressed into it.3
-
How the fuck does a community write an open source library/package that works wonders, but fail to write a proper documentation.
So many times I have to look into the core of frameworks, libraries and packages so form my own documentation in my head.
I guess I should be contributing too.
We are all to blame.1 -
Browser automation is a PITA. I’m going on my fourth side mission with this crap and I honestly still look like a newbie. I’ve tried Java Selenium with Chrome, Excel VBA with IE9, Vanilla JS in the browser console, and tonight I’m thinking to concoct some kind of hybrid CDP & Selenium approach in Chrome. Never used CDP before, not even sure where to start but I heard it sucks like anything else unless you get some extra libraries and plugins and stuff.
It doesn’t help that I can’t get just anything I want from our IT Department. It would be another PITA to ask for puppeteer. If puppeteer is totally legit please let me know.
Selenium sucks. The buttons don’t click, the waits don’t wait. Its unusable. Iframes are annoying as all hell but I can deal with that. HTML Tables suck too. It doesn’t help I have to restart my whole java program and whole Chrome every time an element doesn’t get picked correctly. Scripting one single element can take all fucking night.
Chrome dev tools what the fuck. Why the fuck is the DOM explorer in the same window as the web page I’m working on?? I can’t undock it. Am I supposed to use a fucking TV screen to work with this bastard?? If I use the remote chrome tools on port 9225 or whatever - It Still Renders The Whole Fucking Page Alongside The Console. Get Out Of My Way!!! The nested HTML CODE IS ONE CHARACTER WIDE ALL THE TIME. I can’t for the life of me figure out what the fuck I’m looking at. Haven’t you people ever heard of A HORIZONTAL SCROLL BAR at least.
Fuck I tried using getElementById, and the Xpath thing and its not all that great seeing I have seemingly 1000s of nested Divs all over the god damned place oftentimes containing a single element. I’m finally on chrome now should I learn Jquery now? I mean seriously wtf.
I use this one no code tool for dev it has web automation built in. As you can imagine its just as broken as anything else!! I have 10 screens to navigate it gets stuck on the second screen all the damn time. Fuck I love clicking the buttons when my script misses and playing catch up with it.
So as a work around to Selenium not waiting even 1 millisecond when I use explicit wait or implicit wait or fluent wait, I’m guessing maybe I can attach both Chrome Dev Tools Protocol (CDP as ive called it earlier) and selenium to the same browser and maybe I can use CDP to perform a Wait with any degree of success. Selenium will do nothing more than execute vanilla javascript Element.click(); This is the only way I know to even ACTUALLY use selenium beyond the simplest html documents possible. Hell I guess CDP can execute js idk.
I can’t get the new selenium that has CDP but I do have some buggy ass selenium from a few years back. Yeah, I remember reading there was a pretty impactful regression defect in the version I have. Maybe I’m being gaslighted by some shit copy of selenium?
The worst part is that I do seem to be having issues that the rest of the internet’s devs do not seem to be having. People act like browser automation is totally viable and pretty OK. How in the fuck hell is my Selenium Test Suite going to be more reliable my application under test?!!?? I’ll have more fucking bugs in my test suite than in my application. Today, I have less than half a test script and, I. already. fucking. do.
I am still SUPER PISSED at the months of 12 hour days (always 8 hours spent on normal sprint work btw only 4 to automation) I spent trying to automate our regression tests. I got NOWHERE.
I did learn a lot about HTML and JS though like I’m not that mad…but I’m just trying to emphasize my achievement on my task was zero.
The buttons don’t click. There are so many divs and I swear you sometimes need to select a div somewhere in the middle sometimes to get it working. The waits don’t wait. XHR requests are invisible. Java crashes 100 times before I find an xpath and thread.sleep() combo that works. I have no failure modes to use — Sometimes I click the same element 20x in a script because I have no way to know if it clicked the first time! Sometimes you gotta scroll the page to make the click work. So many click methods all broken. So many wait methods all broken. Its not just the elements don’t click! There are so many ways to click that almost work but surely they all fail the same in the end. ok at this point I’m just repeating myself…
there yet even more issues that I can’t remember…and will soon remember as I journey into this project yet again…
thanks for reading I hope I entertained and would love to hear your experience!5 -
There are definitely too many Javascript libraries, if only because every name that I want on npm is already taken 😔4
-
JS is such open language with too many frameworks and too many libraries. Takes lot of time choosing good code to use.