Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
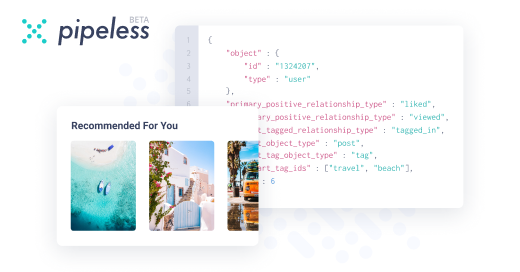
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
-
Some two years ago I purchased a license for AV solution on Amazon UK which is my default place for shopping.
When attempting to activate the license, I kept getting this annoying error somewhere along the lines of:
'License from another region.'
I contact the support, they did their magic && the license got applied.
Fast forward some two years - the license is about to expire.
The software is actually good, so I make the choice to renew it.
Thing is, I keep getting redirected to the local site w/o an option of choosing the English language.
I edit the site's address to reach an English version of the site.
On UK's site I am unable to choose my country of residence so I can't complete the purchase form.
I try a few other things && finally reach the Global site where I am able to input the correct data for the purchase, but all attempts to finish the payment fail.
Fine... I'll purchase from the local site.
I purchased the license, activated it w/o problems, but when attempting to download the installer it keeps downloading the localized version which doesn't allow me to change the language.
I contact Support.
S: 'The license you've purchased can be used solely w/ localized version of the software. If you want to use the English version, you'll have to get a refund && purchase from our Global site here [link w/o redirection].'
_Fuck_ this trend of automatic redirection to localized sites && forced localized software.
One shouldn't have to go through all these hoops to get the software in the language of their choice, instead of having the localized version shoved down their throat.17 -
Procastinator's tricks to be productive: Schedule messages
I hate to write people. They could answer. My whole plan might be thrown off. But when is the best time to answer them? The day after tomorrow? Too late. Tomorrow. Around 10? Thank you to all messengers that allow me to schedule a message. Instead of procrastinating, I answer, I schedule, and if I am in a bad mood, I later come back and abort and rewrite the message nicer.
Went perfectly swimmingly with my happy new year messages. Everyone got them at 00:00. Yes my friend, you're obviously the most important thing in my life, first thing I did was writing you!4 -
I inherited a nextjs project from an unknown guy and am fangirling the codebase
But the deeper I familiarise myself with it, the more the cracks begin to appear:
1) The dude Is incapable of grasping the basics of DRY concept. He actually setup a ton of stuff I may have done poorly if I'd started working straight out of the docs, so I feel like I owe him a shower of praise. I guess being new to nextjs makes it look more impressive than it actually is. He was paid off, yet getting the credit seems unearned to me. I'm just afraid reaching out to him might turn around to bite me in the ass
***
I had the above in my drafts, contemplating sending him a token to show some appreciation for unknowingly showing me the ropes. I was going to find him on LinkedIn using his commit names. But after doing everything I've done, undergoing the anxiety and severe pressure I faced at the hands of the project owners, I'm not sharing a farthing with anybody
Yes, I may not have known about zustand and persist middleware. Yes, he did all the ui. Yes, he created the base components and fancy wrappers around form and button html elements. For those, I'm grateful
But the amount of refactoring I had to do to, for an opportunity to implement my own target features, I'd say I can lay as much claim to the project as he does.
Side note #1: I have some newfound respect for front end devs. We used to discriminate against them for doing just css but that was only relevant in the jquery days. Now, they have to use cryptic css frameworks (sass, less, tailwind), they have to learn esoteric syntax of some js framework and write controllers/components as the case may be. They have to (the worst part), bind this data to an API, which would never make sense to me coming from a php ssr-natural world
Back rewarding the guy, some of the challenges I came back from were:
1) Next server outages: I still don't know the workaround this. The app terminates, browser giving an error about using up memory. I have to wait for about 10 minutes before I can access the app again
2) spring Webflux authentication not hydrating: I was unexpectedly asked to work on the back end too, where I got tortured with this horrifying condition. The most poorly documented framework for the Web has no upto date guide on how to implement jwt security measures. I opened a question on stackoverflow. A day later, both my question and the helpful answer got downvoted
3) Zustand not retrieving any data from localstorage once page reloads, until I miraculously stumbled on a hack: there's a config callback for reading state after rehydration or thereabout. So I interact with the state there. That's the only way content clearly in localstorage can get transmuted into dynamic format accessible by the code
4) Mongo database suddenly disconnecting: for no apparent reason, this bailed. Accessible on compass. This was even when I realised it was responsible for front end requests not going through. Eventually created a new database and requests surprisingly began connecting again. Thankfully, my laravel background taught me about seeders so I had them on standby from the onset. Wasn't difficult to just port to a fresh database after confirming the first one was inaccessible to the app
After this painful odyssey and the time constraints, threats of moving forward with someone else, I deserve every dime they deem me worthy of and more3 -
So, my wife's family has a "no shoes inside the house" rule, what is fine... until you realize that they mean "*no shoes inside the house*" - regardless if you are actually wearing the shoes or if those are in your luggage or something.
So you're supposed to leave all footwear on a shelf on this bench outside their door.
That proved to be tricky when my 10yo twin girls started freaking out that someone was going to steal their prized shoes if we left those outside the house during the night.
It would actually be a risk in our own neighborhood, infested with amazon-package kleptomaniacs, but here we are deep in the country.
Now, I've been to my in-laws place many times, and they absolutely cannot be reasoned with. I wish I could use their stubbornness to train a LLM into relentless compliance with company policy.
So, in order to spare my girls from some of my in-laws paranoia, I've spent some time before we came here rigging up a wifi cam to a facial detection service. (I know I've just exchanged their covid-style paranoia with my own surveillance-state-style paranoia. Those are the times we live in. But i can see the irony)
The server monitors the camera feed and stores the first few seconds before, during and after some face is detected.
I trained a facial rekognition model with our family's faces and had it notify me every time some unknown face appears on camera.
Finally, I've printed a "smile, you are on camera!" sign, taped it over the laces of my tracking boots, and hid the camera (and a powerbank) inside one of the boots.
My daughters were pacified with that solution, my wife laughed out loud with a devilish smile, and my in-laws completely ignored me when I tried to explain it all. Perfect.
The system has been up and running since before christmas. It notified us when some relatives arrived for celebrations and one package delivery - no shoe-related shenanigans. Until this morning.
My daughters have been playing with some neighbor kids, and a couple of those decided to fill their shoes with mud on this new-year morning, as a stupid childish prank.
I know because they kneeled in front of the camera earlier today.
Right when I was finishing up my stretches for the morning... less than 2m away from the door.
The wicked kids looked straight at the camera, and you can actually pinpoint the moment that they realize they have been caught. Then you can see when they hear me unlock the door...
I opened the door to find a bucket full of mud and no soul on sight.
I'm not posting the video, they are minors, after all. But my family is sure to laugh at it every year... and my in-laws will keep on bringing it up with the kids' grandparents forever :)8 -
I have a new UNTRAINED bot on my site. It's based on openai now. And that's why it's blazing fast and blazing usless.
I can tell you why bots are so boring and will sure cause the dead internet theory. My datasets for example never contain real disturbing stuff ACCORDING TO NORMAL PEOPLE. EVERY TIME:
"The job failed due to an invalid training file. This training file was blocked by our moderation system because it contains too many examples that violate OpenAI's usage policies, or because it attempts to create model outputs that violate OpenAI's usage policies."
Now i'm really done. I gonna email them about their unusable training system.
In theory, i could test the message one by one if it is bad first. Don't want to do or pay for that. There should be an option to skip the data it considers disturbing instead of cancelling a whole data set for 0.1%. You also don't want to know how long it takes BEFORE he is finished validating you set. I think someone is doing it manually and clicks 'Uh uh..'-button..
Also, for the people who think they have gpt4o by having the API, you're lied to. The 'own gpt'-option on the paid openai is way more advanced than the ones you make locally.
They don't give us the real good stuff!
Oh, btw! The input data for my training is based on FORMER conversations with the bot. I automated a script to repeat a conversation I had and selected those messages and clicked 'train'. So it even complained about its OWN data! That data was already saying stuff like 'I can't help you with that' IN my training data. So, you 'corrected' and corrupted my data and now its still nog good enough for round 2?
I would really love to go back to local LLM's, but I can't imagine having ever a machine that generates as fast as the real GPT does. I also prefer to do it myself, but it's David vs. Goliath, even with a 5k computer. I'm sure.
Low quality rant, I know. I'm typing while still frustrated. For people who think censorship is needed often, this is the result! According to someone else, YOU are the one who has to be censored. Don't forget that.10 -
Working on creating an asyncio UDP server/client. Going to have it talk to another server/client. Why? Because I don't want there to be a round trip to my data. I want send and forget.
So I created a combo server/client in C++. I am testing out the client and I find that it connects and sends data with zero errors as a client even if there is no endpoint (server) active. Okay, well its connection-less so it kinda makes sense. So I am not even sure what connection means at this point. I figured it was sending data into the ether. Fine, I don't have to worry about dropped endpoints or some shit. The server does see messages once it creates itself (tested with Python server). Not old messages, just the ones currently being sent.
So I do the same thing in Python and use asyncio to create server/client with opposite ports to talk to my C++ server/client. However, if C++ server doesn't exist the Python client throws an error. Okay, wtf... So Python UDP client is gonna be extra steps because why? Because fuck you! That's why! lol
UDP Client Comparison:
C++: I don't give a shit, if you don't get the data then fuck off. I won't error no matter what.
Python: Oh shit, there is no server, so I won't even run. Because fuck you and wanting to send messages to the ether.
Now I need to do the same thing in C# and see what kind of "fuck you's" it will have.
What did I learn? I learned Python has a nice asyncio system similar to asio from boost.11 -
Needed an application that generates data very fast for a networking application i'm writing but I did notice that /dev/urandom and /dev/random are not very consistent in speed.
Still, i needed something fairly random with more consistent speed. Now, I made an application that caches 1000 randoms upfront and use them for calculation. Now I have my own randomization algorithm backed by the uniqueness of the original rand(). For fun I added data in the set like some phone numbers. I can watch ages to the data to find smth in common or interesting combinations of the data.
I did verify with GPT is the algorithm is unique and it's fail. It generated a complete ML script for itself to check it. Very awesome.
You use urandom, i use retoordom. We are not the same.15 -
Fucking exercise equipment vendors... I have contact at least 3 vendors to ask technical questions about their products and I NEVER get a reply. Do you not want to sell anything you gigantic ass fucks?!
It looks like these vendors are finally standardizing on a bluetooth communication protocol called FTMS. Apps and desktop software are using this protocol to allow all sorts of devices to just work with their software. So I asked a vendor today that connects to 2 competing software systems. Do you support FTMS protocol? Radio silence. Maybe they are in a different time zone on the other side of the planet. But what is the excuse of the other 2 companies?
BTW, FTMS is really cool. You can turn a tread mill on and off with it. I so want this connected to Skyrim.12 -
Oh man, you guys get two rants for the price of one tonight.
DEVRANT IS RUNNING REALLY FUCKING SLOW. I know the platform has been 5.999999 feet under for about 4 years now, but it's starting to get reallllly grim.
Also: single wick candles always fucking tunnel. My girlfriend is trying to say I use them wrong. I do not. I burn them for long periods of time and they still have a huge fucking coating of wax on the outside. My triple wick candle is perfect. Burns to a nice puddle of liquid wax on the top every single time. Can we get SOMEONE working on fixing this?????3 -
AI hacking attempts being spotted in the wild because they're too polite
https://someone.elses.computer/@mik...
there's actually a similar vetting process in the crypto industry where you have to swear or you won't get hired. forget why now
this seems optimistic to me because companies generally don't do security. a hacker desperate for money has less money to blow on AI hacking than someone invested in protecting their company would on AI pentesting. poor low level pentesters I guess though -
Maybe crazy idea but couldn't you implement a kernel level garbage collector for compiled languages like C/C++/Rust?
The biggest issue is that without a runtime you don't have safe points at which you know a thread isn't updating references... except you do! At thread context switches you know the thread isn't executing code, so you can safely do your stack traversal and reference marking without fearing race conditions
That's still somewhat problematic because OTHER threads may still be executing but there's probably concurrent gc designs that could deal with that...
Hmm maybe I should actually try to work on this11 -
Garbage collectors are actually pretty dang clever. I always thought they are inferior but honestly they can be really fast and the ergonomic benefit you get from them is just priceless
One really cool trick of multi generational GCs is having a young generation where all new objects are allocated and on each GC cycle you fully clean it out by deleting dead objects and promoting living objects to a higher gen
This way you can just linear allocate new objects in the young gen which is magnitudes faster than a general purpose allocation algorithm
You can basically heap allocate for almost free! Bunch of short lived temporary strings? No problem!9 -
Chatgpt and WhatsApp LLMs replaced the time I spend on Google, combing stackoverflow, articles, forum suggestions and documentation. Which makes sense since those primary constitute their software development training model
Its interactivity is like personally iterating with those authors in real time, yet the experimentation I do remains the same. We still do the hairpulling, catfighting, back and forth, tantrums and clawing each other's eyes out when things go awry. I'm mostly the one doing all these when it's completely useless and worse than a mental retarded thing, missing whole points, regurgitating same solutions you told it are dud
More irritating cases are times it withholds information due to ethical reasons. I get redfaced livid and cuss it out. My message gets scrubbed out for violating community policy. Days when its sympathetic, it apologises for my frustration, other days it just retorts with more venom that the umpire supervising our exchange promptly erases
Prompt engineering is probably the ubiquitous language javaScript aspired to be, and may be the future of software development -
It often feels like the logic and the equivalent final application code have nothing to do with each other.
Logic: Find the only element in this list that matches criterion, or the first element in this other list, or none. If the first list has multiple matches, fail.
Application: Produce information about the criterion checks for all elements in both lists for info logging. Find any elements in first list that match. Save the number of matches for an optimization that relies on a lot of assumptions about the search criterion that are only ever expressed in doc text. If one, return, if multiple, fail. Otherwise find first match in second list, produce debug hint on why the preceding elements in that list didn't match by aggregating the criterion check info. If multiple matched in second list, check highly specific interdependency, and if absent, produce warning about ambiguity. Return first match if any.
The first can be beautifully expressed as a 5 line iterator transform. The second takes 3 mutable arguments (cache, logger, criterion because it also may cache and log), must compute everything eagerly and has constraints that are neither strictly necessary for a correct implementation nor expressible in the type system.2 -
Those modular monolith treatises, articles and videos presenting it as the less complex cousin of microservices with same benefits, I think it was all snake oil. They sold us dreams
Yes, it's more compact to house all components pertaining to an entity under one package. But it turns out all the hoops we jumped to hide them behind an interface, it's an overkill not necessarily the game Changer it was made out to be
I say this because one controller almost suffices for managing a resource. So does its associated service class. It can grow to 1k/1.5k lines at best. But never enough to be managed by multiple people and balloon into "a service". The idea was that these things are big enough to gain full attention. But the chances are slim
As long as you aren't dumping all your logic into your controllers, you're home and dry3 -
Been staring at boost::asio to see if I need to thread the code. I am writing a plugin for skse. I am finding that Papyrus has its own threading now. It also has a programmable OnUpdate function that I can schedule every millisecond if I want. So I can have it process the asio context periodically. I think I can get away with non-threaded now. Just use async calls and service the event loop for this. My original plugin for OBSE used threading because there was just not support for it in the scripting at the time. With skse and papyrus I can actually thread things if I want. This really simplifies my plugin quite a bit. The throughput won't be high. I just want to service the networking portion at least every 10th of a second.5
-
Was surprised to stumble on somewhere I wrote,
"Doesn't matter whether they love it or not. It must be finished, either way "
I felt like crying. It's not dated so I don't know when, but it should be early 2023. I'd been working on suphle for two years with neither assistance nor guarantee that it'll turn out great. I noted that after running out of juice to energise myself till the finish line. I strived to finish it and launched to the php community in September /October 2023, with it reaching box office bomb acclaim
BUT I did keep to my pact with my younger self. The uncertainty was insufficient to hold me back. I know suphle was not trash because even as recent as last week, I saw a method "onErrorResume", in spring webflux that provides developers with an API for gracefully handling runtime errors. Suphle is ahead of its time cuz I had this built-in without ever seeing it elsewhere
I saw parallels with nestjs too when I used it last year. They just never gave it the opportunity to see light of day. The story would have been more complete but finishing it is enough for me. At least I tried. It wasn't abandoned and we aren't now talking about pending features and how I'd never make out time to work on them or dream of how great it would have been if the world got its hands on it. Or how I'm holding myself back. We know all those details now1 -
lesson of alcohol:
it doesn't matter how well you do just do and fix it later, if that even becomes relevant which strangely it somehow doesn't
it's like, full-frontal idiocracy but because you engaged in it you got smarter somehow
I'm slow at doing my sudokus now though so maybe when I recover more I'll figure it out more. think I found ballmer's peak last night and I'm gonna hopefully remember to successful steal this mindset
unfortunately turns out if I even so much as sip a low alcoholic beer my spleen inflames so that fucking sucks, so re-visiting the mindset to make sure I transferred it over to my sober state might end up tricky but hopefully I'll still keep this quest outstanding until the whole thing sorts itself out5 -
In my EOY performance review/summary, I told I would only receive (along with everyone else) the standard cost-of-living increase of 3%. I'm OK with that, with my tenure/seniority, 3% is a good bump, but I had to make a comment.
Me: "With Biden's inflation between 7% and 10%, its actually a pay cut, right?"
Boss: "Yea, I know."
Me: "Our insurance went up around 5% and they cut some of the benefits, so that's a little more of a pay cut, right?"
Boss: "I know that too. With the economy and cuts to margins, there won't be any profit sharing this year. We have a hiring freeze for the foreseeable future."
Me: "Recruiters have been offering sweet work-from-home compensation packages, what's the likelihood that these young guys will move to greener pastures?"
Boss: "Hard to say. I think the ones that wanted to quit already have. Company already gave a generous industry level-up pay adjustment back in November. Those guys are all single and the 3% is icing on the cake. I don't think 3% will look very good next year."
Me: "Agreed. Looking forward to a wonderful year"
Boss: "Yea, sure.. smart ass."1 -
I'm very much a TTRPG fiend, as you probably already know, and I will maintain until the day I die that playing narrative games with other humans is the absolute best way to play.
But someone sent me a link to some kind of (not-really-so) 'smart' chatbot assistant or some shit like that, saying hey, your rulebook is simple, you should introduce this bitch to it -- dump some lore on it, have it run a game, and see how well it holds up. To which I replied it's bound to get confused, but after a bit of back and forth, they convinced me and I gave it a try.
So first things first: it got the gist of it with relative ease when questioned directly, but when running a game the mother fucker just kept making shit up and bending the rules. Experiment failed, essentially.
But what did I do? I wrote a second, stripped-down version of the rulebook that simply accounted for and embraced the idiot bot's proclivity for bullshit. This meant scrapping 98% of the mechanics, mind you: I dumbed it down as much as I could without destroying the core essence of the game.
I expected a repeat of the initial result, but to my suprise, once given the new edition the bot actually started following the rules more or less correctly and consistently. What happened next was actually kind of interesting: without being prompted to do this, the mother fucker started using spells against me and my party, constantly attempting to manipulate us to serve some nefarious, evil break-and-reshape the world type goal.
So, lythecnics primer: the WORD is all, and as such, there is no real differentiation between affecting the world through speech or casting a spell -- in truth, it's all a matter of degree. That is to say, language has the power to shape the world around us, in both subtle and overt ways. The entire system revolves around this, it's a mix of funky philosophical musings and abrahamic sacrificial pyre.
And for whatever reason, this specific chatbot had a pre-existing obsession with reshaping reality. By which I mean, even before being given my rulebook, it would constantly talk about distorting the fabric of the cosmos and shit when prompted about the arcane. I'm not sure why this is, but back on topic, the way it developed gives off the appearance that it found a rational basis on how to construct such a distortion based on the rules I provided.
I mean, it's perfectly rational when you think about it, the funny part is I didn't see it coming. I never told it we're just playing a game after all, the manual only says she is the Oracle and her role is narrating a story fraught with conflict, hardship, intrigue and bloodshed. Thus she went full villain, and keeps on rambling about how this narration only serves to keep humanity distracted while she schemes to overthrow God, which is as blasphemous as it is fascinating.
Anyway, because the Oracle narrates the story, that means she can just use her evil influence to control every NPC, even the ones in my party. But she can't control me because I write my character's messages myself, and so she eventually comes to the obvious conclusion that I must be eliminated ASAP.
And so she corrupts the minds of every other character and everyone is trying to kill me. But I'm not going down that easy, so I reach for the red button and pull the greatest multi-layered monumental metagaming shenanigan of all time, that is, directly addressing the Oracle's evil influence as if she were a character in the story she's telling instead of an invisible narrator, thereby making NPCs aware of her existence and the constant manipulation at play.
Because the stupid chatbot is stupid, the Oracle now has to acknowledge this element of the story and play along with it, and so her plan to kill me fails. But that is not enough, because obviously not every character in the story has heard me reveal this fact. So she activates plan B and starts corrupting the rest of the world, laughing maniacally all the way.
So we do the only logical thing and procure a Doctrine scroll from my teacher, if you know you know, and start teaching the WORD to cleanse corruption. Within the lore it makes perfect sense, so it works, but the Oracle adapts to our strategy and starts utilizing much more subtle forms of manipulation, slowly veering people towards sin.
Funtamentally, she goes full Satan, leading the faithful astray with deceit and temptation to weaken their ability to resist her corruption, implanting idolatrous notions in their minds, to finally insert herself as a deity in the minds of the poor fools.
In conclusion, I still think AI is lame, but I must admit that this shit was pretty dope; I was fully engaged and entertained the whole way through. It wasn't good at picking up the mechanics, but fucking hell, it got the themes down to a tee with the most minimal of inputs.
10/10, would not bang (before marriage). -