Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
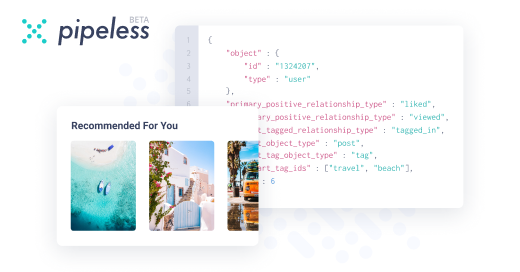
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "api nonsense"
-
I wrote a database migration to add a column to a table and populated that column upon record creation.
But the code is so freaking convoluted that it took me four days of clawing my eyes out to manage this.
BUT IT'S FINALLY DONE.
FREAKING YAY.
Why so long, you ask? Just how convoluted could this possibly be? Follow my lead ~
There's an API to create a gift. (Possibly more; I have no bloody clue.)
I needed the mobile dev contractor to tell me which APIs he uses because there are lots of unused ones, and no reasoning to their naming, nor comments telling me what they do.
This API takes the supplied gift params, cherry-picks a few bits of useful data out (by passing both hashes by reference to several methods), replaces a couple of them with lookups / class instances (more pass-by-reference nonsense). After all of this, it logs the resulting (and very different) mess, and happily declares it the original supplied params. Utterly useless for basically everything, and so very wrong.
It then uses this data to call GiftSale#create, which returns an instance of GiftSale (that's actually a Gift; more on that soon).
GiftSale inherits from Gift, and redefines three of its methods.
GiftSale#create performs a lot of validations / data massaging, some by reference, some not. It uses `super` to call Gift#create which actually maps to the constructor Gift#initialize.
Gift#initialize calls Gift#pre_init (passing the data by reference again), which does nothing and returns null. But remember: GiftSale inherits from Gift, meaning GiftSale#pre_init supersedes Gift#pre_init, so that one is called instead. GiftSale#pre_init returns a Stripe charge object upon success, or a Gift (and a log entry containing '500 Internal') upon failure. But this is irrelevant because the return value is never actually used. Pass by reference, remember? I didn't.
We're now back at Gift#initialize, Rails finally creates a Gift object using the args modified [mostly] in-place by all of the above.
Another step back and we're at GiftSale#create again. This method returns either the shiny new Gift object or an error string (???), and the API logic branches on its type. For further confusion: not all of the method's returns are explicit, and those implicit return values are nested three levels deep. (In Ruby, a method will return the last executed line's return value automatically, allowing e.g. `def add(a,b); a+b; end`)
So, to summarize: GiftSale#create jumps back and forth between Gift five times before finally creating a Gift instance, and each jump further modifies the supplied params in-place.
Also. There are no rescue/catch blocks, meaning any issue with any of the above results in a 500. (A real 500, not a fake 500 like last time. A real 500, with tragic consequences.)
If you're having trouble following the above... yep! That's why it took FOUR FREAKING DAYS! I had no tests, no documentation, no already-built way of testing the API, and no idea what data to send it. especially considering it requires data from Stripe. It also requires an active session token + user data, and I likewise had no login API tests, documentation, logging, no idea how to create a user ... fucking hell, it's a mess.)
Also, and quite confusingly:
There's a class for GiftSale, but there's no table for it.
Gift and GiftSale are completely interchangeable except for their #create methods.
So, why does GiftSale exist?
I have no bloody idea.
All it seems to do is make everything far more complicated than it needs to be.
Anyway. My total commit?
Six lines.
IN FOUR FUCKING DAYS!
AHSKJGHALSKHGLKAHDSGJKASGH.7 -
After two extensive talks with a potential employer (they lasted for hours), I decided to accept the offer, although the salary was ~25% lower than at my previous job. Everything else sounded fantastic and I needed that desperately since at the previous company everything was toxic for years.
These new guys wanted a senior php dev because they had none of them, except only wordpress and drupal people who were not skilled enough to take other types of projects (they called them "custom php"). I liked it and thought I'm gonna shine there and quickly earn a raise because the agency will start earning more by getting projects that they were unable to even bid for.
First day at work and I got assigned to a new Drupal project, although it was supposed to be a simple restful API for a simple iOS app. It could be done in a week or less, with no rushing at all. But it had to be Drupal. And I happened to be around to hear that there is a queue of Drupal projects waiting. After 2 days leaving the office late and having my brain melted by nonsense I was looking at, I quit the job.
No offense to Drupal people, I really do admire you, but I just could not stand it after 8 years "doing custom php". It felt too much like being downgraded. But more than that I was pissed off by the fact that I have been shamelessly lied to and tricked to accept something I clearly said that I dont want.
This happened a year ago. I now earn 2.5x more money than those guys offered and work in a very healthy environment. In the meantime, I heard that the other guys shut their company down.2 -
I'm currently rewriting perfectly clean and functioning Scala code in Java (because "Enterprise", yay). The amount of unnecessary boilerplate I have to add is insane. I'm not even talking big complicated code but two liners or the lack of simple things like a range from 5 to 10.
Why do I have to write
List<Position> occupiedPositions = placedEntities.stream()
.flatMap((pe) -> pe.occupiedPositions().stream())
.collect(Collectors.toList());
instead of simply
val occupiedPositions = placedEntities.flatMap(_.occupiedPositions)
Why on earth does `occupiedPositions.distinct` suddenly become a monstrosity like `occupiedPositions.stream().distinct().collect(Collectors.toList())` where the majority of code is pure boilerplate? And this is supposed to be the new and better Java8 api which people use as evidence that Java is now suddenly "functional" (yeah no, just no).
Why do APIs that annotate parameters with @Nullable throw NullPointerExceptions when I pass a null? Why does the compiler not help prevent such stupidity? Why do we use static typing PLUS those annotations and it still crashes at runtime like every damn dynamic, interpreted language out there? That's not unfortunate, it's a complete waste of time.
Why is a simple idea like a range from x to 10 (in scala literally `x to 10`) not by default included in Java? There's Guava's version of Range which does not have a helper for integer ranges (even though they are the most used ones). Then there's apache.commons version which _has_ a helper for integers, but is strangely not iterable (wtf I don't even...).
Speaking of Iterable: How difficult could it be to convert an abstract Iterable<T> into a concrete List<T>? In scala it's surprisingly `someIterable.toList`. I found nothing like that so I took to stackoverflow where I found a thread in which people suggested everything from writing your own ListUtils helper class, using Guava (which is a huge dependency!) to using the new Java8 features inline (which is still about three lines long). I didn't know this was such a hard problem in computer science, TIL.
How anyone can be productive in this abomination of a language is beyond me now, even though I've used it for many years while learning to code (back then I didn't know there were much better ways to do things). The only good part is that I have to endure this nonsense for only about 3 days longer then I'm free again!12 -
OK heavy rant on 'modern' software development coming! --> don't take it to seriously though :-)
Electron... why does that shit exist? It is like stacking all the worst technologies available to mankind into an enormous pile of crap and polishing that turd to look like something wonderful. It is big, slow and overall AWFUL!
An example? ... Microsoft Teams :-( it burns your PC like fire and makes it squeal for mercy.
When a library/framework becomes the ultimate evolution of abstraction layer upon abstraction layer and it simply should stop to exist and a reset button needs to be pressed.
I would love to see some research on the real world environmental impact that all those shitty slow and bloated web technologies have.
Solution:
Software energy label!
C, C++ and Rust e.t.c. and all accompanying efficient UI libraries should be the only languages/implementations allowed to get a A, B and C label.
Python (without C libraries like Numpy), JavaScript and all those other slow interpreted scripting/Web API nonsense should get a D, E or F label by default.
Have fun!12 -
This might actually be my first real rant.
Whatever fucking cockgoblin decided that making dynamics GP so fucking confusing needs to suck a big bag of dicks. I'm so fucking tired of having to google every damned table name and column name because nothing makes any motherfucking sense.
Am I supposed to instinctively know what PM20201 does? What data it holds? I don't mind reading documentation. But it's hard to even know where to start when the shitbird API and database are more complicated than calculating orbital fucking decay.
I am done. Fuck you gp. Fuck you and your nonsense. I guess our sales people don't get to know when an invoice was paid.8 -
I am using VS Codium instead of VS Code to see what kind of things change when you scrape the Microsoft out of it. Apparently some tools for dotnet core like debugging are locked down and only allowed to run in Microsoft made IDEs.
I hate the sneaky Microsoft API lockdown nonsense and will be steering future projects away from any dotnet core development. I thought this was dead in VS Code but they managed to sneak it in.6 -
We use jira at my company. It's great for me, because no ticketing system's UI is worth a shit, but jira's API is excellent. But we're switching to a new system that is an absolute piece of garbage. Every page is 100% Javascript, so no source can ever be viewed, and the URL never changes to reflect what's onscreen. If you know a ticket number, no URL will ever get you straight to it. You have to navigate multiple slow-loading 25MB piles of Javascript to reach what you're seeking. And most damning of all: the new system has an API, but our highest management is withholding access to it, claiming it breeds laziness.
Is amazing the kind of shit you have to swallow when your management has regular meetings with really really super extremely good-looking sales people.10 -
In a meeting yesterday working through our WebAPI coding standards, starting from File -> New project..etc..etc.. and ironing out some of the left-or-right decisions so we can have a consistent coding style, working in a meeting room with an overhead projector and sharing keyboard around with one another.
Then we hit the routing 'rules' in the WebApiConfig, "api/{controller}/{id}"…
DevMgr: "Do we need the 'api' prefix? It seems redundant."
Ralph: "Yes it's needed. Prefixing the controllers with 'api' is industry best practice. Otherwise, how is anyone to know it's a web api"
Prancer: "Yea, it's part of the REST standard."
Me: "I don't think so. That is only part of the Asp.Net routing rule. We can put anything we want or take anything out."
DevMgr: "Yea, it looks silly. All the new services are going to be business process specific."
Ralph: "That's how everyone does it. It's kind of the point of why REST services are called WebApi"
Prancer: "What's the point of doing any of this work if we're not going to follow industry standards."
Me: "I understand if the service is part of larger web site, but we're developing standalone services. Prefixing routes with 'api' is redundant. I mean who are these 'everyone' you're talking about?"
<ralph rolls his eyes>
Ralph: "Lets see …uhhh… Netflix?. They're kinda a big deal."
Me: "Like I said, it's an integral part of their site and the services they provide. That's fine. I'm talking about the 12 other 3rd party services we integrate with. None of them have 'api' on any of their routes."
Prancer: "We're talking about serious web services."
Me: "Last time I checked, UPS is a big and serious service."
Ralph: "Their services are a fracking joke" – he didn't say fracking.
Me: "Our payroll system, our billing system, billion dollar companies, didn't have '/api' prefix anywhere. Heck, even that free faxing service we used for a while was a dead-simple routing path."
<I take the keyboard away from Ralph, remove the 'api' from the route.>
Me: "There. Done. Now, lets talk about error handling.."
Rest of the meeting Ralph and Prancer don't say much of anything, arms crossed…I swear Ralph looked like he was going to cry.
This morning I catch my boss…
Me: "What did you think of the meeting? I thought Ralph was going to take a swing at me when I took the keyboard away from him."
DevMgr: "Oh yes…I almost laughed out loud….blows my freaking mind how worked up people get about crap that doesn't matter. Api..or not…who the frack cares. Just make it consistent"
Me: "Exactly…I didn't care either way, but I enjoyed calling out that nonsense."
DevMgr: "Yes..waaay too much."
If I didn't call them on their BS and the 'standard' allowed to continue, I can bet my paycheck when the subject comes up in a few months (another mgr asks 'isn't this api prefix redundant?') Ralph and Prancer will be the first to say "Yea, its stupid. We fought really hard to remove it from the standard...its not our fault...its <insert scapegoat> fault." -
First of all sorry for the bad picture. Let's move on...
Deleting this method like:
Who the hell did this nonsense?
I just took a picture of it so I could post it here and rant about it.
Sure felt stupid after deleting it for not thinking about a protected method in the parent class that is part of an API.
So... Yeah....
I was feeling too confident in my skills lately anyway6 -
I once had to write a feature, which should allow the user to login and edit an appointment, which was automatically set. All the data we got, came from an incredibly unreliable API. And with incredible unreliable I mean like heisenbug-level unreliable.
The API spoke perfectly unreadable xml and was a horror to work with.
After a few weeks of me being messed with by this shit piece of an API, I finally got something which did kind of work sometimes.
Proper error handling has been added later and just before I was done, fixing all the flaws of their data management and nonsense status codes (not http status codes) which rarely correlated in at least some way with their data, our client said "scrap this, we don't want it anymore"
Many hours and effort gone, this thing worked almost perfectly. -
Dude GoogleAuth is pure nonsense magic. On one line you get your auth-instance from gapi.auth2.init..
But then you render the auth-button with a static method aka gapi.signin2.render (which has some kind of success and error handlers, but don't worry, they fire randomly, they won't help you debug this api mess)
SOME-FUCKING-HOW this static signin2.rendershit knows of your auth2 instance and it works. But actually it makes no sense and is just a big mess of api-calls. Google, get your shit together, this ain't pretty.
Oh and forget your informative console.log.. this shit will get erased everytime you try something because of "Navigated to https://accounts.google.com/o/...". why ever the fuck this clears the console even tho it doesn't affect the top window. So preserve that fucking log and drown in a mass of bullshit.
In the end, as it is with everything, it somehow works. But FFS that's some weird api design Google has going on..4 -
I think the following is all in my head, or I am heading towards an office rivalry situation between my tech lead and me.
characters :
me : a no nonsense android guy who is sometimes very blunt when requested for unwarranted demands. i am also realising that i have been a bit too arrogant, as i come up with a lot of counter questions too fast (not related to story tho)
tech lead : an android guy who has been android dev for a total of 4 years (same as me), 3 of them in current company and somehow got promoted to TL
story: I find this guy to be too much political, delegating a lazy bum, and i kinda called him out in public , once during a discussion where other folks were also kinda calling him out and another time when we were having a small meeting of 3 people. he in turn has taken some actions (like giving me a lower kpi, not giving me appropriate data for doing some work and then asking about it in public, casually ignoring my leave requests) which looks he is taking out a revenge.
at first time i called him out in a discussion where everyone was getting against his havit of giving buttery responses to his boss (who occasionally joins our standups) . he says "we are on track" while we are already dependent on him to provide data/decisions.
he then says to us to do it faster , and when the work does not get completed ( because how it could be, without him doing his job), he blames it on devs.
i called him out on a similar but different topic of him making last moment task additions when we are already on brim with our planned tasks.
on second time i called him out on him not looking into the current task enough as he was expecting me to take decisions on my own.
the decision was about how a screens ui will be populated and there was no api payload available that would match the ui . i created 2 mock api jsons which would appropriately load that screen but was not sure if the 2 apis would be enough for the screen and wondered whete some missing data will come from?
this task is a long one, nd i did took a decision, but he should had validated them to make sure we are on track. the issue came when i took some questions to him and instead of answering them , he blamed on me not being mature enough to work without the data!
All things aside, I am on my weary ends with thins guy. He is my boss and holds incredible powers over me, but he is incredibly incompetent and his habits of delay, delegation and blaming is making my work life worse. I don't wanna leave this job too, because as much as i hate it, its currently one of the major names in industries and giving a solid power to my resume -
Disclamer: I don't want to give out what app I am talking about, so all names will be random to just represent the nonsense and my frustration.
So I was working with that app's API....
To begin with, some retrieved Objects have collection (iterable structure) of "Thing" objects, called "Things". But... there can be max one element in that particular collection!
Ok... I get it... I might exaggerate a bit... fine, let it be.
I had to mention it for the further part, and also got to mention that "Thing" objects are globally available and predefined, and Objects can only choose one, unused "Thing".
To the point.
Someone thought it would be good to separate representation of one structure into two classes.
We have collection of "A" objects ("As"), which have "Name", "Things" and other, mostly GUI/config related attributes.
Collection "Bs", of "B" objects, they have "Name" and rather lower-level attrs.
The "As" and their attributes can be set in the GUI, but the list where you do it is named "List of Bs" and vice versa.
Interesting, huh?
I had to use both "A" and "B" definition for given name, so I tried to map it... and things gone South.
Collecions have "Get" method with name as an argument.
But it turns out that while the "A" use its GUI name all the time, "B" uses either name that can be found in "As" or, if not all "Thing" objects are used, the "Thing" names.
Example:
global "Things" = "t0", "t1"
"As" = "a0"("t0"), "a1"("t1") -> "Bs" == "a0", "a1"
"As" = "a0"("t0"), "a1"() -> "Bs" == "a0", "t1"
"As" = "a0"(), "a1"() -> "Bs" == "t0", "t1"
That means if at least one of "A" objects have empty "Things", then the mapping will fail.
Only solution is that the app works only partially when any of "A"'s "Things" is empty, so I might raise error too, but I have to provide solution that will work even in the cases when the app don't care... so... not gonna happen.1 -
My answer to their survey -->
What, if anything, do you most _dislike_ about Firebase In-App Messaging?
Come on, have you sit a normal dev, completely new to this push notification thing and ask him to make run a simple app like the flutter firebase_messaging plugin example? For sure you did not oh dear brain dead moron that found his college degree in a Linux magazine 'Ruby special edition'.
Every-f**kin thing about that Firebase is loose end. I read all Medium articles, your utterly soporific documentation that never ends, I am actually running the flutter plugin example firebase_messaging. Nothing works or is referenced correctly: nothing. You really go blind eyes in life... you guys; right? Oh, there is a flimsy workaround in the 100th post under the Github issue number 10 thousand... lets close the crash report. If I did not change 50 meaningless lines in gradle-what-not files to make your brick-of-puke to work, I did not changed a single one.
I dream of you, looking at all those nonsense config files, with cross side eyes and some small but constant sweat, sweat that stinks piss btw, leaving your eyes because you see the end, the absolute total fuckup coming. The day where all that thick stinky shit will become beyond salvation; blurred by infinite uncontrolled and skewed complexity; your creation, your pathetic brain exposed for us all.
For sure I am not the first one to complain... your whole thing, from the first to last quark that constitute it, is irrelevant; a never ending pile of non sense. Someone with all the world contained sabotage determination would not have done lower. Thank you for making me loose hours down deep your shit show. So appreciated.
The setup is: servers, your crap-as-a-service and some mobile devices. For Christ sake, sending 100 bytes as a little [ beep beep + 'hello kitty' ] is not fucking rocket science. Yet you fuckin push it to be a grinding task ... for eternity!!!
You know what, you should invent and require another, new, useless key-value called 'Registration API Key Plugin ID Service' that we have to generate and sync on two machines, everyday, using something obscure shit like a 'Gradle terminal'. Maybe also you could deprecate another key, rename another one to make things worst and I propose to choose a new hash function that we have to compile ourselves. A good candidate would be a C buggy source code from some random Github hacker... who has injected some platform dependent SIMD code (he works on PowerPC and have not test on x64); you know, the guy you admire because he is so much more lowlife that you and has all the Pokemon on his desk. Well that guy just finished a really really rapid hash function... over GPU in a server less fashion... we have an API for it. Every new user will gain 3ms for every new key. WOW, Imagine the gain over millions of users!!! Push that in the official pipe fucktard!.. What are you waiting for? Wait, no, change the whole service name and infrastructure. Move everything to CLSG (cloud lambda service ... by Google); that is it, brilliant!
And Oh, yeah, to secure the whole void, bury the doc for the new hash under 3000 words, lost between v2, v1 and some other deprecated doc that also have 3000 and are still first result on Google. Finally I think about it, let go the doc, fuck it... a tutorial, for 'weak ass' right.
One last thing, rewrite all your tech in the latest new in house language, split everything in 'femto services' => ( one assembly operation by OS process ) and finally cramp all those in containers... Agile, for sure it has to be Agile. Users will really appreciate the improvements of your mandatory service. -
TL;DR I just recently started my apprenticeship, it's horrible so far, I want to quit, but don't know what to do next...
Okay, first of all, hey there! My name is Cave and I haven't been on here for a while, so I hope the majority of you is doing rather okay. I'm programming for 6 years now, have some work experience already, since I used to volunteer for a company for half a year, in which I discovered my love for integrations and stuff. These background information will probably be necessary to understand my agony in full extend.
So, okay, this is about my apprenticeship. Generally speaking, I was expecting to work, and to learn something, gaining experience. So far, it only involved me, reading through horrible code, fixing and replacing stuff for them, I didn't learn a thing yet, and we are already a month in.
When I said the code is horrible, well, it is the worst I have ever seen since I started programming. Little documentation - if any -, everywhere you look there is deprecated code, which may or may not been commented out, often loops or simply methods seem to be foreign for them, as the code is cluttered with copy paste code everywhere and on top of that all, the code is slow as heck, like wtf.
I spent my past month with reading their code, trying to understand what most of this nonsense is for, and then just deleting and rewriting it entirely. My code suddenly is only 5% or their size and about 1000 times faster. Did I mention I am new to this programming language yet? That I have absolutely no experience in that programming language? Because well I am new and don't have any experience, yet, I have little to no struggle doing it better.
Okay, so, imagine, you started programming like 20 years ago, you were able to found your own business, you are getting paid a decent amount of money, sounds alright, right? Here comes the twist: you have been neglecting every advancement made in developing software for the past 20 years, yup, that's what it feels like to work here.
At this point I don't even know, like is this normal? Did git, VSCode and co. spoil me? Am I supposed to use ancient software with ancient programming languages to make my life hell? Is programming supposed to be like this? I have no clue, you tell me, I always thought I was doing stuff right.
Well, this company is not using git, infact, they have every of their project in a single folder and deleting it by accident is not that hard, I almost did once, that was scary. I started out working locally, just copying files, so shit like that won't happen, they told me to work directly in the source. They said it's fine, that's why you can see 20 copies of the folder, in the same folder... Yes, right, whatever.
I work using a remote desktop, the server I work on is Windows server 2008, you want to make icons using gimp? Too bad, Gimp doesn't support windows server 2008, I don't think anything does anymore, at least I haven't found anything, lol.
They asked me to integrate Google Maps into their projects, I thought it is gonna be fun, well, turns out their software uses internet explorer 9.. and Google maps api does not support internet explorer 9... I ended up somehow installing CEF3 on that shit and wrote an API for it in JS. Writing the API was actually kind of fun, but integrating it in their software sucked and they told me I will never integrate stuff ever again, since they usually don't do that. I mean, they don't have a Backend as far as I can tell, it looks like stuff directly connects with their database, so I believe them, but you know... I love integrating stuff..
So at this point you might be thinking, then why don't you just quit? Well I would, definitely. I'm lucky that till December I can quit without prior notice, just need a resignation as far as I can tell, but when I quit, what do I do next? Like, I volunteered for a company for half a year and I'd argue I did a good job, but with this apprenticeship it only adds up to about 7 months of actual work experience. Would anybody hire somebody with this much actual work experience? I also consider doing freelancing, making a living out of just integrating stuff, but would people pay for that? And then again, would they hire somebody with this much experience? I don't want to quit without a plan on what to do next, but I have no clue.
Am I just spoiled, is programming really just like that, using ancient tools and stuff? Let me know. Advice is welcomed as well, because I'm at a loss. Thanks for reading.10