Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
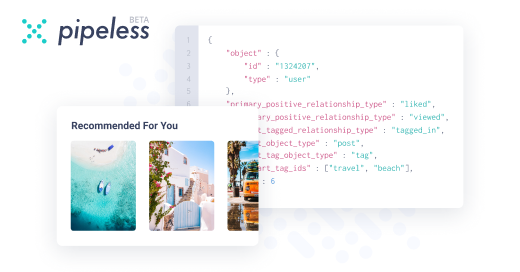
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "type casting"
-
The new mobile app codebase i'm working with, was clearly written by someone who just read a book on generics and encapsulation.
I need to pull out 2 screens into a separate library to have it shared around. The 1 networking request used is wrapped up in a 'WebServiceFactory' and `WebServiceObjectMapper`, used by a `NetworkingManager` which exposes a generic `request` method taking in a `TopLevelResponse` type (Which has imported every model) which uses a factory method to get the real response type.
This is needed by the `Router` which takes a generic `Action` which they've subclassed for each and every use case needing server communication.
Then the networking request function is part of a chain of 4 near identical functions spread across 4 different files, each one doing a tiny bit more than the last and casting everything to a new god damn protocol, because fuck concrete types.
Its not even used in that many places, theres like 6 networking calls. Why are people so god damn fucking stupid and insist on over engineering the shit out of their apps. I'm fed the fuck up with these useless skidmarks.3 -
Me: Return data from Firebase into unity project
Firebase: Returns data as object
*Type casting fails for 6hrs*
Finally find the reason Data return type is int64 and I was saving as int32
Now I sit and question my life while listening to epic music2 -
Have a function that takes parameters and then performs a switch statement to determine what function to call next with those same parameters. One of those parameters is a Union type.
During CR, my reviewer said they’d like if instead of returning the function per case, I instead assigned a handler to the value of the function per case and then returned that handler at the end of the switch. Simple change, right? Only snafu, I’m casting one of the parameters on a per-case basis.
Somehow, through no fucking change of my own, TypeScript in its wisdom has decided that the type of that value by the time I call the next function is a fucking Intersection.
WHY THE FUCK DO YOU THINK IT’S AN INTERSECTION?! I’m fucking casting it per case! I’m ensuring it’s the right type for the next function called on a per case basis!
…. And that, my friends, is how I wasted a day with a stupid refactor that was ultimately just scrapped because no one could figure out how to make it work.
Goddamn fucking TypeScript. I3 -
This is so far my worst Monday.
Ran to Emergency (A&E) because I was having breathing problems. Came back to work with Inhaler.
Work started with type casting and linker errors.
I hope the day ends well :( -
"And in a stunning turn of events, he got it to work!"
But seriously... I've literally been throwing shit at a wall and seeing what would stick.
Fucking DTOs and getting shit out of a database. I need better resources on how to do this properly!
Anyways, I found that just using 'object' and letting the compiler deal with the rest of the bullshit actually allowed my code to work and run. I'm still a little in shock.
I'm over here trying to keep things in a nice one-to-one because that's what my PM recommended... and instead I just get slammed by Type casting nonsense and more errors than I can begin to understand. And unfortunately, Stackoverflow is of no help because everyone's issues are very nuanced and unrelated to my problem... Maybe I'm the problem? 🤷
But here it is working without all that bullshit. I don't know man... This code base is not the rager I was expecting. I'm getting my ass kicked with code that doesn't fall in line with the book I'm learning from.
You know how they say, "forget everything you've read and learned"? I'm feeling that really hard right now.
Constantly fighting the urge to rip everything down and do it based on what my book is recommending, but then the logical natured side of me is like "you ain't got that kind of time to be unfucking someone's work, only to get caught in more trouble. Your ego is not worth it"
Anyways, it's fucking late here and I'm glad enough to not have to think about this issue anymore. Bye.3 -
Was recently asked if our team wanted to change from Java to Kotlin so I looked over the feature lists but don't see much that's impressive.
One argument was less boilerplate code but I think they're are libraries like Lomboq that can write that stuff for you.
The other was smart type casting and type inference and to that I'm like this sounds like giving monkeys a hammer.
Our JS codebase looks like shit... And our Java app just crashed in prod.
Getting a ton of text messages this morning and thankfully I'm on vacation still...
The error is not caused by NPE... but how some or logic spammed the db..
A new language isn't going to fix this.... And a team that writes this sort of shit logic clearly shows they are incapable of learning a new one probably...
They are already script kiddies... Don't need them to become babies...6 -
Some compilers give an error message on forgotten type casting. From that it shows good typing style casting. So you also avoid clerical errors that can lead to the program crash in the worst case. With some types it is also necessary to perform type casting comma on others Types, however, do this automatically for the compiler.
In short:Type casting is used to prevent mistakes.
An example of such an error would be:
#include <stdio.h>
#include <stdlib.h>
int main ()
{
int * ptr = malloc (10*sizeof (int))+1;
free(ptr-1);
return 0;
}
By default, one tries to access the second element of the requested memory. However, this is not possible, since pointer calculation (+,-) does not work for a void pointer.
The improved example would be:
int * ptr = ((int *) malloc (10*sizeof (int)))+1;
Here, typecasting is done beforehand and this turns the void pointer into its int pointer and pointer calculation can be applied. Note: If instead of error "no output" is displayed on the sololearn C compiler try another compiler.1 -
Feel dirty writing in c. How do people even deal with unsafe pointer type casting/memory allocation/free? The codebase is plagued with memory leaks and there is no test.
I will just pretend I can't read c code and play dumb when shit happens13 -
The bug: Some string values for an identifier property in the data objects are being sent from our frontend prefixed with a '0'. Sometimes. When it happens, it usually gets stripped away again by the time it's passed to our backend. But not always.
This 0 is never explicitly set anywhere. I even searched for a few variants of " = 0" in both the frontend and backend projects without receiving any results. You might already be suspecting where this is going.
So it turns out.
The data object which holds this value is being initialized in the aspnet (don't ask) backend and passed to the frontend, which then hydrates it. This value is always an integer number, albeit incidentally so which is why string is used as the actual type. When this object is initialized, it's hardcoded with an anonymous type where this property is set as int because I guess someone figured "it's always an int though". Being a typed language, primitive scalars can't be null objects which means the property's value becomes the concrete int 0.
Okay weird. I can think of better ways of doing this but let's just set it to string as I can't start overhauling things right now. Let's just go find where this value is somehow concatenated into the incoming parameter.
You see, this happens because at the point where the frontend sets this value, it may be an int or string depending on where it came from, and I guess someone figured that in order to cast it to string you just go prop += arg seeing as the prop is empty string and all. Because explicitly casting it or - as much as I get a rash whenever I see it - going prop = "" + arg would be too verbose and unoriginal.
Bonus round: How come the 0 only sometimes made it all the way to our backend? The thing is that this bug has been fixed before. The fix is that because this string is "always" an int, you can parse it to int before passing it to the backend in case it has leading zeroes. This path is only taken in certain views because someone forgot to copypaste their fix into all the places this is repeated.
Sometimes you find a bug and you are just somehow more grumpy after fixing it.1 -
This *is* a question you silly wrong tagging mother fucker, how dare you doubt me?
Alright, no more disclaimer: I like dungeons and dragons, but it's too fucking much in terms of rules and systems and shit, as in just *making* a character can take a long ass while.
And if that's the highest level of all your ANAL preferences then OK, but I'm not you and things only come OUT of my ass, not inwards, I swear.
Anyhoo, I got fed up with it and wrote my own ruleset and setting as a last fuck you to everyone. It's very simple: if you want to be some kinky magical alien hermaphrodite royal prostitute half sewer dragon princess and three quarters bearded female incest child of demons and fairies then FINE, but you get no bonuses for that shit.
Get it? No complex racial level scaling bullshit, FUCK YOU, race and background is just for vibes, end of story.
You get no attribute or skills or shit to distribute on level one. All you get is a prompt: pick three actions, that's it. You wanna be sexy? Pick "seduce". You wanna set turds on fire? Pick "ignite". Are you an edge lord? Pick "summon". Would you be my wife? Pick "heal", "buff" and "smite".
The game is turn based, and each action you can take is effectively a spell. Everyone can cast a basic spell like walk, attack, talk, crouch, etcetera -- that costs no mana. Special crap like flying and firing fucking electricity costs mana, and you can only do those if you either picked the spell on level one or learnt it later from a book/tutor/demonic bargain/whatever.
Which spells are valid for taking at level one is up to the game master; I just tell people to pick three verbs or short sentences, and if they choose something that's too broken like "split the Red Sea" I'm like nah you're not Moses, try again.
Still with me? Good. You get eight points of health, four points of mana, and one point of stamina. They're all energy, and you can use it to power your magery, but spending all your health means you fucking die.
Stamina recharges fully every turn, and is used for the aforementioned basic actions. All of these cost one point of stamina each. If you run out of stamina, you can use mana. Or your BLOOD.
Level one spells cost one mana, level two cost two and so on. You get back one point of mana each turn, and you can fire all the spells you want during it, long as you have mana. Or BLOOD.
That's good and all, but if you spend anywhere over eleven combined points of energy in one go, you spontaneously combust and die, erasing all signs of life in a twenty-meter radius. This is called incineration, and it *will* leave behind a blackened crater from which the dark servants of the Horror Immemorial may or may not crawl out of.
In case you didn't guess by now, your blood doesn't fucking come back unless you eat, sleep or see a healer.
But anyway, the more points you spend into casting a spell -- and remember, basic attack counts as a spell -- the more powerful it is, so the bigger your diceroll can get. My rule is I add one dice for every fourth point of energy spent, so (1d4), (1d4 + 1d6), (1d4 + 1d6 + 1d8), incineration.
Additionally, for every three points of energy spent, your spell can hit one more target. That's right, you like AoE? Then spend more mana, bitch. Oh, and if you're using shit like poison it lasts one more turn for every two points of energy spent.
How do we calculate damage? Diceroll over two and fuck your mother. Armor class? Resistances? Out of my face with that shit. Damage reduction is called "tyranny" and is for dungeon bosses only.
If you live long enough to get to level two, you *do* get attributes. Pick:
- Grit: +2 health, +1 to fighter shit type rolls.
- Cunning: +2 mana, +1 to rogue shit type rolls.
- Allure: +1 stamina, +2 to wizard shit type rolls.
- Spirit: +1 to elemental shit type spells.
- Faith: +1 to benefactor paragon asshole shit type spells.
- Hatred: +1 to demonic murder hobo destructive shit type spells.
On second level, you can pick one of the spells you know to get +1 to it, specifically. Eh, "+1" just means you get a bonus to some diceroll, no time to explain I'm running out of characters what the fuck.
On level three, the cycle repeats. Pick attr, pick spell. DONE.
Oh right, and weapons. Mostly just vibes, pick your fancy and fuck off. Normally, you can hit things one tile away; if you have a BIG melee weapon you can hit from *two* tiles away, and if you have a ranged weapon you can shoot anyone in sight, but you need to spend one point of energy to reload.
And there, all bases covered in less that 5000 characters with some flair to spare, now suck my fucking cock Hasbro.
What was the question? Oh yeah right, I'm gonna GPL this shit and put it in browsers. I think I'm going to write it in Kotlin but I'm open to suggestions. Would you guys like to play it/contribute to it's development for shits and giggles?8 -
Those of you working with .NET, do you prefer C# casting syntax:
(Type)variable;
Or VB casting syntax:
variable as Type;3 -
Algolia says:
"So our price widget doesn't allow decimals, you'll have to create a custom widget"
I do it.
"Hey, It's not working and I verified it's applying the filter correctly. I noticed my price is a string in your index, maybe that's incorrect and causing it to not work?"
They say: "Yep, you'll need to run an update to fix that and change all to floats" (charges an arm and a leg for the thousands of index operations needed to update the data type)
I clear the index and send a single one as a test, verifying it's a float by casting it using (float) then var_dumping. It shows "double(3.99)", but when it gets to Algolia, it's 0.
So I contact support.
"Hi, I'm sending across floats like you say but it's receiving it as 0, am I doing something wrong? Here's my code and the result of the var_dump"
They respond: "Looks like you're doing it right, but our log shows us receiving 3.999399593939, maybe check your PHP.ini for "serialize_precision" and make sure it's set to -1"
I check and it's fine, then I realize that var_dump is probably rounding to 2 decimal points so I change my cast to (float) number_format($row['Price'], 2) and wallah...it works.
Now I've wasted days of paying for their service, a ton of charges for indexing operations, and it was such a simple fix.
if they had thrown an error for the infinite decimal, that would have helped, but instead I had to reach out to find out that was the issue.
#Frustrated. -
No matter how hard you try to stick magnet with a wood it just won’t work out in any way.
i hope its possible to change the wood into metal just like as easy as type-casting an integer into string, but it just won’t happen anyway~~2