Details
Joined devRant on 7/10/2016
Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
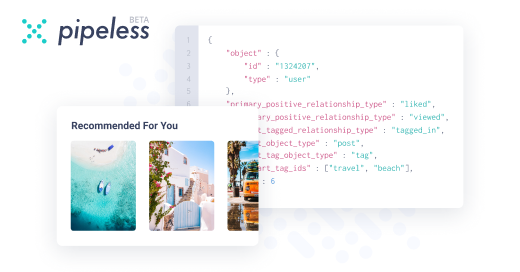
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
-
Heres some research into a new LLM architecture I recently built and have had actual success with.
The idea is simple, you do the standard thing of generating random vectors for your dictionary of tokens, we'll call these numbers your 'weights'. Then, for whatever sentence you want to use as input, you generate a context embedding by looking up those tokens, and putting them into a list.
Next, you do the same for the output you want to map to, lets call it the decoder embedding.
You then loop, and generate a 'noise embedding', for each vector or individual token in the context embedding, you then subtract that token's noise value from that token's embedding value or specific weight.
You find the weight index in the weight dictionary (one entry per word or token in your token dictionary) thats closest to this embedding. You use a version of cuckoo hashing where similar values are stored near each other, and the canonical weight values are actually the key of each key:value pair in your token dictionary. When doing this you align all random numbered keys in the dictionary (a uniform sample from 0 to 1), and look at hamming distance between the context embedding+noise embedding (called the encoder embedding) versus the canonical keys, with each digit from left to right being penalized by some factor f (because numbers further left are larger magnitudes), and then penalize or reward based on the numeric closeness of any given individual digit of the encoder embedding at the same index of any given weight i.
You then substitute the canonical weight in place of this encoder embedding, look up that weights index in my earliest version, and then use that index to lookup the word|token in the token dictionary and compare it to the word at the current index of the training output to match against.
Of course by switching to the hash version the lookup is significantly faster, but I digress.
That introduces a problem.
If each input token matches one output token how do we get variable length outputs, how do we do n-to-m mappings of input and output?
One of the things I explored was using pseudo-markovian processes, where theres one node, A, with two links to itself, B, and C.
B is a transition matrix, and A holds its own state. At any given timestep, A may use either the default transition matrix (training data encoder embeddings) with B, or it may generate new ones, using C and a context window of A's prior states.
C can be used to modify A, or it can be used to as a noise embedding to modify B.
A can take on the state of both A and C or A and B. In fact we do both, and measure which is closest to the correct output during training.
What this *doesn't* do is give us variable length encodings or decodings.
So I thought a while and said, if we're using noise embeddings, why can't we use multiple?
And if we're doing multiple, what if we used a middle layer, lets call it the 'key', and took its mean
over *many* training examples, and used it to map from the variance of an input (query) to the variance and mean of
a training or inference output (value).
But how does that tell us when to stop or continue generating tokens for the output?
Posted on pastebin if you want to read the whole thing (DR wouldn't post for some reason).
In any case I wasn't sure if I was dreaming or if I was off in left field, so I went and built the damn thing, the autoencoder part, wasn't even sure I could, but I did, and it just works. I'm still scratching my head.
https://pastebin.com/xAHRhmfH33 -
The end is near.
I threw together a devRant content saver just now. It's rough, but it works:
https://github.com/miloxeon/...
save your rants and pics until it's too late.12 -
So I promised a post after work last night, discussing the new factorization technique.
As before, I use a method called decon() that takes any number, like 697 for example, and first breaks it down into the respective digits and magnitudes.
697 becomes -> 600, 90, and 7.
It then factors *those* to give a decomposition matrix that looks something like the following when printed out:
offset: 3, exp: [[Decimal('2'), Decimal('3')], [Decimal('3'), Decimal('1')], [Decimal('5'), Decimal('2')]]
offset: 2, exp: [[Decimal('2'), Decimal('1')], [Decimal('3'), Decimal('2')], [Decimal('5'), Decimal('1')]]
offset: 1, exp: [[Decimal('7'), Decimal('1')]]
Each entry is a pair of numbers representing a prime base and an exponent.
Now the idea was that, in theory, at each magnitude of a product, we could actually search through the *range* of the product of these exponents.
So for offset three (600) here, we're looking at
2^3 * 3 ^ 1 * 5 ^ 2.
But actually we're searching
2^3 * 3 ^ 1 * 5 ^ 2.
2^3 * 3 ^ 1 * 5 ^ 1
2^3 * 3 ^ 1 * 5 ^ 0
2^3 * 3 ^ 0 * 5 ^ 2.
2^3 * 3 ^ 1 * 5 ^ 1
etc..
On the basis that whatever it generates may be the digits of another magnitude in one of our target product's factors.
And the first optimization or filter we can apply is to notice that assuming our factors pq=n,
and where p <= q, it will always be more efficient to search for the digits of p (because its under n^0.5 or the square root), than the larger factor q.
So by implication we can filter out any product of this exponent search that is greater than the square root of n.
Writing this code was a bit of a headache because I had to deal with potentially very large lists of bases and exponents, so I couldn't just use loops within loops.
Instead I resorted to writing a three state state machine that 'counted down' across these exponents, and it just works.
And now, in practice this doesn't immediately give us anything useful. And I had hoped this would at least give us *upperbounds* to start our search from, for any particular digit of a product's factors at a given magnitude. So the 12 digit (or pick a magnitude out of a hat) of an example product might give us an upperbound on the 2's exponent for that same digit in our lowest factor q of n.
It didn't work out that way. Sometimes there would be 'inversions', where the exponent of a factor on a magnitude of n, would be *lower* than the exponent of that factor on the same digit of q.
But when I started tearing into examples and generating test data I started to see certain patterns emerge, and immediately I found a way to not just pin down these inversions, but get *tight* bounds on the 2's exponents in the corresponding digit for our product's factor itself. It was like the complications I initially saw actually became a means to *tighten* the bounds.
For example, for one particular semiprime n=pq, this was some of the data:
n - offset: 6, exp: [[Decimal('2'), Decimal('5')], [Decimal('5'), Decimal('5')]]
q - offset: 6, exp: [[Decimal('2'), Decimal('6')], [Decimal('3'), Decimal('1')], [Decimal('5'), Decimal('5')]]
It's almost like the base 3 exponent in [n:7] gives away the presence of 3^1 in [q:6], even
though theres no subsequent presence of 3^n in [n:6] itself.
And I found this rule held each time I tested it.
Other rules, not so much, and other rules still would fail in the presence of yet other rules, almost like a giant switchboard.
I immediately realized the implications: rules had precedence, acted predictable when in isolated instances, and changed in specific instances in combination with other rules.
This was ripe for a decision tree generated through random search.
Another product n=pq, with mroe data
q(4)
offset: 4, exp: [[Decimal('2'), Decimal('4')], [Decimal('5'), Decimal('3')]]
n(4)
offset: 4, exp: [[Decimal('2'), Decimal('3')], [Decimal('3'), Decimal('2')], [Decimal('5'), Decimal('3')]]
Suggesting that a nontrivial base 3 exponent (**2 rather than **1) suggests the exponent on the 2 in the relevant
digit of [n], is one less than the same base 2 digital exponent at the same digit on [q]
And so it was clear from the get go that this approach held promise.
From there I discovered a bunch more rules and made some observations.
The bulk of the patterns, regardless of how large the product grows, should be present in the smaller bases (some bound of primes, say the first dozen), because the bulk of exponents for the factorization of any magnitude of a number, overwhelming lean heavily in the lower prime bases.
It was if the entire vulnerability was hiding in plain sight for four+ years, and we'd been approaching factorization all wrong from the beginning, by trying to factor a number, and all its digits at all its magnitudes, all at once, when like addition or multiplication, factorization could be done piecemeal if we knew the patterns to look for.7 -
Why can no-one, not one single solitary fucker, on StackOverflow get it through their thick skull that when I call PHP's http_response_code() or try to get $_SERVER["REDIRECT_STATUS"], I want the response code from Nginx? No, not Apache. No, I don't want to pass a status code FROM PHP TO NGINX, I want the response code. FROM Nginx. TO PHP.
In what fucking universe does PHP know more about the response code than Nginx? It doesn't. Nginx knows the response code, because that's the fucker that redirected to the error page. I want the error. Passed to the page. From Nginx. To PHP.
NO, http_response_code() DOES NOT MAGICALLY FUCKING WORK, IT RETURNS 200 BY DEFAUL- fuck it.7 -
When we subtract some number m from another number n, we are essentially creating a relationship between n and m such that whatever the difference is, can be treated as a 'local identity' (relative value of '1') for n, and the base then becomes '(base n/(n-m))%1' (the floating point component).
for example, take any number, say 512
697/(697-512)
3.7675675675675677
here, 697 is a partial multiple of our new value of '1' whose actual value is the difference (697-512) 185 in base 10. proper multiples on this example number line, based on natural numbers, would be
185*1,
185*2
185*3, etc
The translation factor between these number lines becomes
0.7675675675675677
multiplying any base 10 number by this, puts it on the 1:185 integer line.
Once on a number line other than 1:10, you must multiply by the multiplicative identity of the new number line (185 in the case of 1:185), to get integers on the 1:10 integer line back out.
185*0.7675675675675677 for example gives us
185*0.7675675675675677
142.000000000000
This value, pulled from our example, would be 'zero' on the line.
185 becomes the 'multiplicative' identity of the 1:185 line. And 142 becomes the additive identity.
Incidentally the proof of this is trivial to see just by example. if 185 is the multiplicative identity of 697-512, and and 142 is the additive identity of number line 1:185
then any number '1', or k=some integer, (185*(k+0.7675675675675677))%185
should equal 142.
because on the 1:10 number line, any number n%1 == 0
We can start to think of the difference of any two integers n, as the multiplicative identity of a new number line, and the floating point component of quotient of any number n to the difference of any number n-m, as the additive identity.
let n =697
let m = 185
n-m == '1' (for the 1:185 line)
(n-m) * ((n/(n-m))%1) == '0'
As we can see just like on the integer number line, n%1 == 0
or in the case of 1:185, it equals 142, our additive identity.
And now, the purpose of this long convoluted post: all so I could bait people into reading a rant on division by zero.30 -
A while back, I had a lot of telemarketers were calling me daily, and I mean A LOT of them.
I got so frustrated with he calls that I decided I had to figure out a better way to handle those calls.
At the time, I was working with a PBX software called Asterisk, which is used to handle hardware interfaces and network applications for phone calls.
I needed a suitable side project and there was a version of Asterisk designed for Raspberry Pi, so I made a fun little answering service for myself.
Whenever a telemarketer called, I asked them to call back later, but to "my personal number", and gave them the number to my phone robot. (which had a pre-paid SIM card in a GSM dongle mounted)
When it received a call, it would play a pre-recorded phrase, wait for 1000 ms of silence and then play the next phrase.
After all 16 phrases had been played, it would start from phrase 7 again and repeat until the caller gave up.
I had this set up running for a while, and then added another robot for english speaking callers.
The calls stopped after a few months.
MISSION ACCOMPLISHED!13 -
Today I'm ranting about Windows. No, it's not "WiNdOwS sUcKs!", it's more like "But why!?"
See, I'm an IT guy for the year, and in my office they use Windows. Now once upon a time, they had Active Directory and all that (well, actually, they still do) but then they got some new computers running Windows 10, and for some reason they just couldn't join them to the domain!
Why can't they, you ask? Well, Microsoft only allows Win 10 Pro and up to join a Domain, and since these computers came with Win 10 Home, that wasn't possible.
Long story short, I now have some 30 computers that need to be upgraded (possibly from 7) to Win 10 Pro, and joined to the Domain.
Thing is, I would like to do that all in one go, so I look into how to automatically setup Windows.
"Ah! Got it, provisioning packages!"
Lest you think they work let me spare you now: they don't. Just like real computers where everything is different, provisioning packages failed to work twice, and after wasting about a week trying to make it work, I gave up.
So now I realized that I need to try a different method, a custom windows image. Issue is, I've got no clue how to make one. See, microsoft decided to go all in on the provisioning packages thing (they do have advantages in certain use cases), and seemed to decide that making custom images was no longer necessary, so they documentation was nearly impossible to find.
But after a lot of searching, I figured out how to do it:
1. Install Windows in a VM.
2. Put it in audit mode.
3. Install your stuff.
4. Create an unattend.xml file with certain customizations.
5. Put the unattend in Windows\System32\Sysprep
6. Generalize the image.
7. Boot WinPE.
8. Open the console.
9. Capture the image.
10. Wait an hour or two.
11. Done!
I'm over simplifying, it was a huge PITA, and yet there were still issues.
Maybe another time I'll talk about those.22 -
Hey everyone,
We have a few pieces of news we're very excited to share with everyone today. Apologies for the long post, but there's a lot to cover!
First, as some of you might have already seen, we just launched the "subscribed" tab in the devRant app on iOS and Android. This feature shows you a feed of the most recent rant posts, likes, and comments from all of the people you subscribe to. This activity feed is updated in real-time (although you have to manually refresh it right now), so you can quickly see the latest activity. Additionally, the feed also shows recommended users (based on your tastes) that you might want to subscribe to. We think both of these aspects of the feed will greatly improve the devRant content discovery experience.
This new feature leads directly into this next announcement. Tim (@trogus) and I just launched a public SaaS API service that powers the features above (and can power many more use-cases across recommendations and activity feeds, with more to come). The service is called Pipeless (https://pipeless.io) and it is currently live (beta), and we encourage everyone to check it out. All feedback is greatly appreciated. It is called Pipeless because it removes the need to create complicated pipelines to power features/algorithms, by instead utilizing the flexibility of graph databases.
Pipeless was born out of the years of experience Tim and I have had working on devRant and from the desire we've seen from the community to have more insight into our technology. One of my favorite (and earliest) devRant memories is from around when we launched, and we instantly had many questions from the community about what tech stack we were using. That interest is what encouraged us to create the "about" page in the app that gives an overview of what technologies we use for devRant.
Since launch, the biggest technology powering devRant has always been our graph database. It's been fun discussing that technology with many of you. Now, we're excited to bring this technology to everyone in the form of a very simple REST API that you can use to quickly build projects that include real-time recommendations and activity feeds. Tim and I are really looking forward to hopefully seeing members of the community make really cool and unique things with the API.
Pipeless has a free plan where you get 75,000 API calls/month and 75,000 items stored. We think this is a solid amount of calls/storage to test out and even build cool projects/features with the API. Additionally, as a thanks for continued support, for devRant++ subscribers who were subscribed before this announcement was posted, we will give some bonus calls/data storage. If you'd like that special bonus, you can just let me know in the comments (as long as your devRant email is the same as Pipeless account email) or feel free to email me (david@hexicallabs.com).
Lastly, and also related, we think Pipeless is going to help us fulfill one of the biggest pieces of feedback we’ve heard from the community. Now, it is going to be our goal to open source the various components of devRant. Although there’s been a few reasons stated in the past for why we haven’t done that, one of the biggest reasons was always the highly proprietary and complicated nature of our backend storage systems. But now, with Pipeless, it will allow us to start moving data there, and then everyone has access to the same system/technology that is powering the devRant backend. The first step for this transition was building the new “subscribed” feed completely on top of Pipeless. We will be following up with more details about this open sourcing effort soon, and we’re very excited for it and we think the community will be too.
Anyway, thank you for reading this and we are really looking forward to everyone’s feedback and seeing what members of the community create with the service. If you’re looking for a very simple way to get started, we have a full sample dataset (1 click to import!) with a tutorial that Tim put together (https://docs.pipeless.io/docs/...) and a full dev portal/documentation (https://docs.pipeless.io).
Let us know if you have any questions and thanks everyone!
- David & Tim (@dfox & @trogus)53 -
Waaaay too many but let's go with this one for now.
At my previous job there was a web application which was generating about 1gb of log data a second. Server was full and the 'fullstack engineers' we called had zero clue about backend stuff and couldn't fix it.
Me and another engineer worked our asses off to figure this out but eventually the logging stopped and it went back to normal.
Great, right?
For that moment. I was the on-call server engineer and at like 3am I got called awake because this shit was happening again.
Sleep drunk with my phone I ssh'd into the server, not sure about what to do at first but then suddenly: let's chattr the goddamn log file...
$ chattr +i /var/log/logfile
Bam, worked, done, back to sleep.
(this comment + param marks the file in a way that it can only be read until the mark is removed, so you can't write to it or move it or remove it or whatever)13 -
I absolutely love the email protocols.
IMAP:
x1 LOGIN user@domain password
x2 LIST "" "*"
x3 SELECT Inbox
x4 LOGOUT
Because a state machine is clearly too hard to implement in server software, clients must instead do the state machine thing and therefore it must be in the IMAP protocol.
SMTP:
I should be careful with this one since there's already more than enough spam on the interwebs, and it's a good thing that the "developers" of these email bombers don't know jack shit about the protocol. But suffice it to say that much like on a real letter, you have an envelope and a letter inside. You know these envelopes with a transparent window so you can print the address information on the letter? Or the "regular" envelopes where you write it on the envelope itself?
Yeah not with SMTP. Both your envelope and your letter have them, and they can be different. That's why you can have an email in your inbox that seemingly came from yourself. The mail server only checks for the envelope headers, and as long as everything checks out domain-wise and such, it will be accepted. Then the mail client checks the headers in the letter itself, the data field as far as the mail server is concerned (and it doesn't look at it). Can be something else, can be nothing at all. Emails can even be sent in the future or the past.
Postfix' main.cf:
You have this property "mynetworks" in /etc/postfix/main.cf where you'd imagine you put your own networks in, right? I dunno, to let Postfix discover what your networks are.. like it says on the tin? Haha, nope. This is a property that defines which networks are allowed no authentication at all to the mail server, and that is exactly what makes an open relay an open relay. If any one of the addresses in your networks (such as a gateway, every network has one) is also where your SMTP traffic flows into the mail server from, congrats the whole internet can now send through your mail server without authentication. And all because it was part of "your networks".
Yeah when it comes to naming things, the protocol designers sure have room for improvement... And fuck email.
Oh, bonus one - STARTTLS:
So SMTP has this thing called STARTTLS where you can.. unlike mynetworks, actually starts a TLS connection like it says on the tin. The problem is that almost every mail server uses self-signed certificates so they're basically meaningless. You don't have a chain of trust. Also not everyone supports it *cough* government *cough*, so if you want to send email to those servers, your TLS policy must be opportunistic, not enforced. And as an icing on the cake, if anything is wrong with the TLS connection (such as an MITM attack), the protocol will actively downgrade to plain. I dunno.. isn't that exactly what the MITM attacker wants? Yeah, great design right there. Are the designers of the email protocols fucking retarded?9 -
Last night me and my friend were on a WhatsApp call discussing how we should practice algorithms and data structures more. We texted the logistics after and he sent me a calendar invite on google. I swear to god this morning I had adds talking about data algorithms all over YouTube but I have never googled this issue in the near past 🤔 this can’t be a coincidence...9
-
People having sleep deprivation.
If your health is at stake, you may want to aim for maximum healing potential.
Humen always should prefer more natural substances and techniques.
As chemicals mostly alter the status quo but tackle the ultimate reason why.
Military has developed glasses that simulate the sunrise to wake you up / keep you awake.
That technique is like 20 years old. And who is gonna get those glasses for me?
Nowadays, studies published find humen feel more at ease by rising and sleeping with the sun and moon.
Having two weeks of camping once in a while is recommended. At least once a year.
Alternately you can try to regulate your days rhythm.
Start your day with a cup of hot speedwell tea.
Like every freaking day.
Its augmenting your activity thus easing your sleep at night.
Give every technique at least two weeks time to take effect.
And always remember :
Sleep is a thing that can be influenced but never will be controlled.
Good night ;P9 -
Now that I have time to approach my ultimate dream ( being the pro penrester ) , asked a hacker for a road map and he gave me (U'll rarely see such open hackers that share knowledge :) )
Surprisingly I've been familiar with all the topics but being the most pro , requires u to be pro in every single topic .
Guess what ? I'm starting from basic linux commands all over again 😂
echo 'hello world :/'25 -
Why is every company so BAD at working with spaces in passwords? Just trying to setup Hulu on my PS4, apparently I forgot my password? No, my password had a space in it. So maybe Hulu's just one of those companies that doesn't allow spaces in passwords? Wait no, I can log in with no problems on my Switch or PC with the space. It's just SPECIFICALLY the PS4 app that doesn't allow spaces. Cool cool cool.
Like, am I missing something? Is there some reason it's harder to hash than other characters? It's just an ASCII character, it's not like I'm copy/pasting in some fringe unicode shit. Some companies straight up ban it. Some like Amazon don't recognize it as a special character, while demanding I use a special character. Why is this so terrible?4 -
It was around 2013, I was working on a project that had a great business idea, a really really bright feature (to this day I state the same) and all I was getting was around 400e/month of salary. (still was a junior dev)
So, I've been going on vacation to Spain for almost 1.5 month, everything was settled, there were no more pending jobs for me as I've finished everything that I could until more things would be done on the application and design that were needed.
It was 2nd week there, I didn't have a laptop with me as it was full vacation mode, no internet connection as it was almost 100e/month at that time, house I've lived in had no internet either. Then, one morning I receive a call that I must be on a skype meeting in any case - it was live or die situation. Me being me - went to a local internet cafe that was around 3km away from the house (on foot) - logged in to the call and proceeded. (I knew something is going to be fishy).
And there it was - I was needed to go back to my laptop and code a huge ass functionality so that we could present it to our testing clients. It was estimated to take around 3 weeks of full working days. No future payment, no compensation was offered but as stupid as I was - I went on with that and worked half of my vacation on full-day schedule... The functionality was delivered... Only after 4 months since the delivery date - the functionality was tested and after total of 9 months - was presented to the testers... I was pissed and asked for compensation as it was my vacation but all I heard was - NO, you took too long of a vacation and therefore it's your own fault. Soon after that I've started to receive every bit of blame if I was even 1 hour off the set deadline that was set by the manager that didn't have a single clue how programming works or even how to use the internet properly....
All in all, I'm still hurt of the 3 weeks that I've missed but since I've left the job 4 years ago (my salary had increased but I've quadrupled it since then) - I tend to see that it's a common practice to require things NOW and only deal with them MONTHS later...
Morale of the story:
Avoid working on your vacation at any means. If that will mean a lost job - then be it, you'll find a new one, presumably a better job.12 -
Paranoid Developers - It's a long one
Backstory: I was a freelance web developer when I managed to land a place on a cyber security program with who I consider to be the world leaders in the field (details deliberately withheld; who's paranoid now?). Other than the basic security practices of web dev, my experience with Cyber was limited to the OU introduction course, so I was wholly unprepared for the level of, occasionally hysterical, paranoia that my fellow cohort seemed to perpetually live in. The following is a collection of stories from several of these people, because if I only wrote about one they would accuse me of providing too much data allowing an attacker to aggregate and steal their identity. They do use devrant so if you're reading this, know that I love you and that something is wrong with you.
That time when...
He wrote a social media network with end-to-end encryption before it was cool.
He wrote custom 64kb encryption for his academic HDD.
He removed the 3 HDD from his desktop and stored them in a safe, whenever he left the house.
He set up a pfsense virtualbox with a firewall policy to block the port the student monitoring software used (effectively rendering it useless and definitely in breach of the IT policy).
He used only hashes of passwords as passwords (which isn't actually good).
He kept a drill on the desk ready to destroy his HDD at a moments notice.
He started developing a device to drill through his HDD when he pushed a button. May or may not have finished it.
He set up a new email account for each individual online service.
He hosted a website from his own home server so he didn't have to host the files elsewhere (which is just awful for home network security).
He unplugged the home router and began scanning his devices and manually searching through the process list when his music stopped playing on the laptop several times (turns out he had a wobbly spacebar and the shaking washing machine provided enough jittering for a button press).
He brought his own privacy screen to work (remember, this is a security place, with like background checks and all sorts).
He gave his C programming coursework (a simple messaging program) 2048 bit encryption, which was not required.
He wrote a custom encryption for his other C programming coursework as well as writing out the enigma encryption because there was no library, again not required.
He bought a burner phone to visit the capital city.
He bought a burner phone whenever he left his hometown come to think of it.
He bought a smartphone online, wiped it and installed new firmware (it was Chinese; I'm not saying anything about the Chinese, you're the one thinking it).
He bought a smartphone and installed Kali Linux NetHunter so he could test WiFi networks he connected to before using them on his personal device.
(You might be noticing it's all he's. Maybe it is, maybe it isn't).
He ate a sim card.
He brought a balaclava to pentesting training (it was pretty meme).
He printed out his source code as a manual read-only method.
He made a rule on his academic email to block incoming mail from the academic body (to be fair this is a good spam policy).
He withdraws money from a different cashpoint everytime to avoid patterns in his behaviour (the irony).
He reported someone for hacking the centre's network when they built their own website for practice using XAMMP.
I'm going to stop there. I could tell you so many more stories about these guys, some about them being paranoid and some about the stupid antics Cyber Security and Information Assurance students get up to. Well done for making it this far. Hope you enjoyed it.26 -
As a person from low-paying country, how do I reconcile with the fact that for the same work, and the same 8 hours, I get 1/3 of what a person in Germany does? In my previous team (same company), one of my teammates was from Germany. The same team, the same work, but he happened to earn a lot more.
This bothers me a lot sometimes. I have seen people requesting to be transferred to another country, and being denied, presumably because of the salary difference. Then, the person leaves, and someone in Australia gets hired. So, rather than moving a veteran person of whom you know fits your company culture to a higher-paying country, you let him go and hire a newbie in an equally-expensive country? What the fuckity fuck?
And to my friends from high-paying countries, especially managers: you don't have to feel bad, but have some common decency. If you come to my country, do not say "oh gosh, everything here is so cheap," or "the dinner for the whole team costs less than buying my family of four a dinner back home." That's offensive as fuck. If that's the case, fucking give me a raise you cheap fuck!30 -
Why haven't I learned about js fetch before now. I've even used socket.io!
Fetch is amazing and I just built a function for using fetch with WordPress AJAX hooks. Yes I know it's WordPress and there's jQuery but all of my custom plugins use vanilla js as a little fuck you.
Oh yeah but ie doesn't support it at all2 -
This week I reached a major milestone in a Machine Learning/Music Analysis project that I've been working on for a long time!!
I'm really proud to launch 'The Harmonic Algorithm' as an open source project! It represents the evolution of something that's grown with me through two thesis' (initially in music analysis and later in creative computation) and has been a vessel for my passion in both Music and Computation/Machine Learning for a number of years.
For more info, detailed usage examples (with video clips) and installation instructions for anyone inclined to try it out, have a look at the GitHub repo for the project:
https://github.com/OscarSouth/...
"The Harmonic Algorithm, written in Haskell and R, generates musical domain specific data inside user defined constraints then filters it down and deterministically ranks it using a tailored Markov Chain model trained on ingested musical data. This presents a unique tool in the hands of the composer or performer which can be used as a writing aid, analysis device, for instrumental study or even in live performance."1 -
To the freelancers.
Do you offer web-hosting? What are your experiences? Where do you rent servers? What do you charge?
Thanks in advance5 -
To all Linux-, Unix-, MacOS-Users here.
Stop whatever your doing,
Install Elvish-Shell,
Forget what what you wanted to do because you get distracted by this awesome piece of software,
Eventually continue what you were doing in a few hours.5 -
TIL: American’s are having a super freak out over the singular use of “they” rather than “he” or “she”.
I’m British, and this is utterly baffling to me.
Singular they is super common here, and has actually been in considerable use for hundreds of years, and used by people like Shakespeare.
I had absolutely no idea that the American derivation of English had such crazy little grammar quirks like this.
What’s everyone’s take on this? Why have such a problem over a word that rather elegantly avoids any conflict over gendered usage of pronouns?
It avoids mislabelling when you’re not sure, it allows you to make statements that are inclusive, and it’s just cleaner.
(And no, I’m not at a ‘Zir’ level of madness)24 -
Completed Flexbox Froggy.
Finally Learned FlexBox , would recommend it 100%.
From: flexboxfroggy.com12 -
How does GDPR affect you as a freelancer in mobile/web development?
I'm asking because I eventually plan on freelancing but I have no clue how to deal with these stuff.8 -
M: Me
FAC : Fucking annoying colleague
1.
FAC: Hey how did you set up your microservices?
M: I used docke...
FAC: But docker is hard to setup, i want an easier option
2.
FAC: Which services do you have?
M: I have one service for the api, one with redi..
FAC: Redis is not a service
3.
FAC: Do you use AWS API gateway?
M: No, in set up my ow..
FAC: why would you set up your own? I just use the one from AWS.
4.
FAC: How many instances are you have running
M: I have 5 replic...
FAC: 5 replicas? That's why i hate microservices,they are costly
5.
FAC: How did you divide up your app?
M: Since I am starting, its better to run the monolithic and then break it up lat...
FAC: I knew it,you don't actually use microservices
6.
M:(thinking)* Fucker, if you know it well why are you fucking disturbing me?? *2