Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
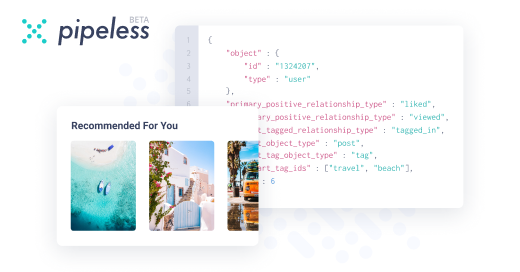
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "explicit code"
-
I wrote a database migration to add a column to a table and populated that column upon record creation.
But the code is so freaking convoluted that it took me four days of clawing my eyes out to manage this.
BUT IT'S FINALLY DONE.
FREAKING YAY.
Why so long, you ask? Just how convoluted could this possibly be? Follow my lead ~
There's an API to create a gift. (Possibly more; I have no bloody clue.)
I needed the mobile dev contractor to tell me which APIs he uses because there are lots of unused ones, and no reasoning to their naming, nor comments telling me what they do.
This API takes the supplied gift params, cherry-picks a few bits of useful data out (by passing both hashes by reference to several methods), replaces a couple of them with lookups / class instances (more pass-by-reference nonsense). After all of this, it logs the resulting (and very different) mess, and happily declares it the original supplied params. Utterly useless for basically everything, and so very wrong.
It then uses this data to call GiftSale#create, which returns an instance of GiftSale (that's actually a Gift; more on that soon).
GiftSale inherits from Gift, and redefines three of its methods.
GiftSale#create performs a lot of validations / data massaging, some by reference, some not. It uses `super` to call Gift#create which actually maps to the constructor Gift#initialize.
Gift#initialize calls Gift#pre_init (passing the data by reference again), which does nothing and returns null. But remember: GiftSale inherits from Gift, meaning GiftSale#pre_init supersedes Gift#pre_init, so that one is called instead. GiftSale#pre_init returns a Stripe charge object upon success, or a Gift (and a log entry containing '500 Internal') upon failure. But this is irrelevant because the return value is never actually used. Pass by reference, remember? I didn't.
We're now back at Gift#initialize, Rails finally creates a Gift object using the args modified [mostly] in-place by all of the above.
Another step back and we're at GiftSale#create again. This method returns either the shiny new Gift object or an error string (???), and the API logic branches on its type. For further confusion: not all of the method's returns are explicit, and those implicit return values are nested three levels deep. (In Ruby, a method will return the last executed line's return value automatically, allowing e.g. `def add(a,b); a+b; end`)
So, to summarize: GiftSale#create jumps back and forth between Gift five times before finally creating a Gift instance, and each jump further modifies the supplied params in-place.
Also. There are no rescue/catch blocks, meaning any issue with any of the above results in a 500. (A real 500, not a fake 500 like last time. A real 500, with tragic consequences.)
If you're having trouble following the above... yep! That's why it took FOUR FREAKING DAYS! I had no tests, no documentation, no already-built way of testing the API, and no idea what data to send it. especially considering it requires data from Stripe. It also requires an active session token + user data, and I likewise had no login API tests, documentation, logging, no idea how to create a user ... fucking hell, it's a mess.)
Also, and quite confusingly:
There's a class for GiftSale, but there's no table for it.
Gift and GiftSale are completely interchangeable except for their #create methods.
So, why does GiftSale exist?
I have no bloody idea.
All it seems to do is make everything far more complicated than it needs to be.
Anyway. My total commit?
Six lines.
IN FOUR FUCKING DAYS!
AHSKJGHALSKHGLKAHDSGJKASGH.7 -
The nightmare continues.
Currently dealing with a code review from a “principal” dev (one step above senior), who is unironically called a “legendary dev” by some coworkers. It’s painfully obvious he didn’t read the code, and just started complaining and nitpicking.
It’s full of requests to do things that make absolutely no sense, and would make the code an unmaintainable mess.
• Ex: moving the logic and data collection from the module’s many callers into the module instead of just passing in the data.
• Ex: hiding api endpoint declarations by placing them in the module itself, and using magic instance variables to pass data to it. Basically: using global functions and variables instead of explicit declarations and calls.
• Ex: moving the logic to determine which api endpoint to use, for all callers, into the view.
More comments about methods being “too complex” (barely holds water) right next to comments saying “why are these separate? merge them together!”
Incredulously asking how many times I’m checking permissions and how ridiculous it all is. (The answer? Twice.)
Conflating my “permissions” param and method names with a supposedly forthcoming permissions system overhaul, and saying I shouldn’t use permissions because my code will all have to get rewritten. Even if that were true, and it’s likely not, the ticket still needs to use the current permissions. I can’t just ignore them because they might be rewritten someday.
Requests to revert some code cleanup because the reviewer thought the previous heavily-nested and uncommented versions (with code duplication) were easier to read. Unsurprisingly, he wrote them.
On the same ticket, my boss wants me to remove all styling and clientside validation, debouncing, and error messages from a form. Says “success” and “connection failed” messages are good enough. The form in question sends SMS and email using arbitrary user input for addresses. He also says it shouldn’t be denounced on the server, and doesn’t want me to bother checking permissions. Hello, spam!
Related: the legendary dev reviewer says he can’t think of a reason why we would want to disable the feature for consumers, so I should remove the consumer feature flag.
You can’t make this stuff up.7 -
I'm working on a programming language with a "bytecode" interpreter and a compiler that translates source code to said bytecode and... it sort of actually works!
I want to recreate an Erlang-style environment, currently you can write functions, call C++ functions via wrappers, have immutable-only values, and it has no explicit control structure apart from statement sequencing and the if-expression because I want to make it as functional as possible. Next thing on the list is to add a green threads implementation and ability to spawn and send messages to processes.
Still a WIP and heck even design-in-progress.
Now for the rant:
I'm using CMake for building C++ (interpreter) and Stack for Haskell (compiler) and I've been trying to get them to talk to each other for hours because I want CMake to manage the Stack build too and shove all the executables into one place. CMake documentation is weird and Stack isn't too helpful either, so I guess I'll just spend another few hours trying to get Stack to fuckin reveal its build directory to CMake and/or build to a given directory. Ugh.8 -
On my former job we once bought a competing company that was failing.
Not for the code but for their customers.
But to make the transition easy we needed to understand their code and database to make a migration script.
And that was a real deep dive.
Their system was built on top of a home grown platform intended to let customers design their own business flows which meant it contained solutions for forms and workflow path design. But that never hit of so instead they used their own platform to design a new system for a more specific purpose.
This required some extra functionality and had it been for their customers to use that functionality would have been added to the platform.
But since they had given up on that they took an easy route and started adding direct references between the code and the configuration.
That is, in the configuration they added explicit class names and method names to be used as data store or for actions.
This was of cause never documented in any way.
And it also was a big contributing cause to their downfall as they hit a complexity they could not handle.
Even the slightest change required synchronizing between the config in the db and the compiled code, which meant you could not see mistakes in compilation but only by trying out every form and action that touched what you changed.
And without documentation or search tools that also meant that no one new could work the code, you had to know what used what to make any changes.
Luckily for us we mostly only needed to understand the storage in the database but even that took about a month to map out WITH the help of their developer ;)
It was not only the “inner platform” it was abusing and breaking the inner platform in more was I can count.
If you are going down the inner platform, at least make sure you go all the way and build it as if it was for the customers, then you at least keep it consistent and keep a clear border between platform and how it is used.12 -
TL.DR.: Emojis in commit messages + bad commit messages made by Microsoft™ employees.
Yes, I'm looking at you Microsoft. It would be helpful if I can, you know, understand your commit messages instead of trying to guess wtf _that_ emoji means. That is, if it is the same emoji on my machine. We didn't figure that one out yet. And no, "Some 💄 changes ✨" is not a good commit message, even if you interpret it correctly (which depends on your emoji icon set).
idk about you, but that shitty 💄 emoji tends to be (see image) and I happen to associate that with an XLR audio cable. I had to ask someone else to understand a commit message; a message supposed to be explicit—stating what you changed and optionally why you changed it (you can off-load that part to an issue tracker).
Furthermore, that "Some 💄 changes ✨" commit did none of that. "I made cosmetic changes somewhere for some reason without linking to an issue." If you didn't catch that little detail yet: "COSMETIC CHANGES" is vague as fuck. What is a cosmetic change?
* Does a cosmetic change mean adjusting indentation?
* Does it mean deleting unnecessary abstraction to make the code more readable?
* Does it mean refactoring code to add that beauty factor?
* Does it mean all of the above? Or perhaps a specific combination of these?
Human communication is shit enough, don't make it worse than it already is.22 -
Staring at cursed blinking cursors.
Repairing work of worst thinking workers
Reverse merges or it'll murder the servers, it nurtures despair
Amateur managers, dimwitted savages interrupt all of us janitors
Cleaning up damages, spills and experiments using skills in embarrassment
Explicit foulness, in a minute it's straight to the bowels with weapons of limitless vowels
A bittersweet hateful machete, eviscerates stateful spaghetti
The slow disease flowing from keys knowing it's going to please
The growing unease, no one agrees, there's no guarantees with your useless degrees
Need more drugs, keyboard's crawling with bugs, falling as I chug
A bottle of cognac gotta love all the hacks, no poise for code that lacks
All the noise, gotta relax, before I destroy the syntax.
Excuse me for not making sense.
Too gloomy, aching and tense.10 -
someone pushes code and breaks ci.
me: you broke the build
her: (ignoring the explicit error message) it works on my machine, travis is broken
me: it doesn't even work on my machine!
her: I forgot to push one file, sorry.1 -
I just finished designing an entire asset management pipeline and christ on a fucking pogo stick, if it isn't convoluted.
Theres a lot of game engines out there, but all of them do it a little different. They all tackle a slightly different problem, without even realizing it.
1. asset management
2. asset change management
3. behavior change management
4. data management
5. combinatorial design management.
6. Combinatorial Behavior management
7. Feature completion
ASSET MANAGEMENT is exactly what it says on the tin.
ASSET CHANGE management can be thought of handling the import, export, formatting, platform specific packing, and versioning (including forking) of an asset.
BEHAVIORAL CHANGE management is a subset of asset management, because code is a subset of assets (depending on how you define 'assets'). The oldest known example of this is commenting and uncommenting code.
Or worse, printf debugging.
This can be file versioning, basic undo services, graph management of forks and mergers, toggles for features or modules, etc.
DATA management is about anything that doesn't fall into the other categories, everything from mission text to npc dialogues, quests, location names, item stats, the works. Anything you'd be tempted to put in a database, falls under this category. Haven't yet seen many engines offer this as an explicit built in tool as of yet, because the other problems are non-trivial as is, so this is a bit of low hanging fruit that gets handled by external tools, or loaded from formats as simple as json.
COMBINATORIAL DESIGN management is the idea of prefabbing, blueprints of broader object design using nested prototypes of existing game objects, to create more complex, reusable set pieces. Unity did this well. GM does this in part.
COMBINATORIAL BEHAVIOR management is entity-component systems, plus tooling to make it easy to add, remove, and configure components and their values on entity blueprints, also not uncommon. Both stencyl and unity do this. GM has a precursor to this in the form of configurable fields, but these fields are not based on component scripts attached to objects.
FEATURE COMPLETION is that set of gameplay mechanics or styles of design that an engine naturally makes easier to include or build in a game.
I don't think I'm aiming for all that, but I think at minimum a good engine has to do asset management, behavioral change management, prefabs, and entity-component systems with management tools for that. And ideally, asset change management.8 -
1. i'm drunk.
2. please do me a sanity check
3:
this video, at this timestamp, watch the following about 5 minutes or so:
https://youtu.be/oG-6Ltp1_yE?t=1129
4. tell me (and possibly him in comment) if i'm wrong in the (point) of the following comment i wrote under that video:
20:53 ARE YOU FUCKIN KIDDING ME YOU ABSOLUTE MORON?!
yes, US has an altitude software written in fuckin VBA with an explicit statement to ignore errors, and there's not about 10x more automated testing code for a critical piece of functionality, than there is of the code that handles the actual functionality, and it's not been tested off-line (in simulated environment) as well as on-line (IRL) for at least years in all conditions, before it was deployed, YOU ABSOLUTE FUCKING MORON.
CAN YOU JUST PLEASE FOR THE LOVE OF ALL THAT'S HOLY STICK TO WHAT YOU ACTUALLY PROPERLY UNDERSTAND?!
HOLY FUCK THE LEVEL OF ARROGANCE IN YOU IN ASSUMING THAT JUST BECAUSE YOU KNOW VBA YOU KNOW HOW PROPER SOFTWARE DEVELOPMENT IS DONE, HOLY FUCKING SHIT.
I've worked in companies of 1k employees and less, on absolutely non-critical stuff, that has DevOps and QA processes and infrastructure that would make your script kiddie head spin for WEEKS, LET ALONE FUCKIN MILITARY SW DRIVING MILITARY EQUIPMENT YOU ARROGANT KNOWITALL FUCK.
Please, just please, FOCUS ON FUCKING DOING VIDEOS ABOUT STUFF YOU FUCKING UNDERSTAND, instead of stuff your ego overinflated from years of debunking dunning-krugers tells you that you're an expert in despite never actually having worked even near those fields. PLEASE. You are amazing when doing those, but this bullshit is just fucking rage-inducing. Don't ever talk about software again, because that's obviously YOUR dunning-kruger area, you fuckin bigheaded script kiddie.12 -
What I'm doing now, writing a JS library for a simple kitchen timer (like, something that can be wound up, is ticking, can be paused, etc). Here's a list of neat stuff I've learned:
Polyfilling as a lib author (I decided against it).
Packaging the lib (using Rollup, ES6 modules are totes cool).
Using flow to add static typing in strategic places (started appreciating types in JS since reading up on functional programming).
Modelling state and transitions using an explicit state machine. (Fucking finally. There's usually an implicit state machine somewhere, only spread out all over the app...)
Using mostly side-effect free methods, being very explicit about when and why things are mutated).
Test-first/TDD (ish) using Jest and the awesome Wallabyjs.
Freeing up mental capacity by letting Prettier format my code for me (it was hard to let go but totally worth it).
Started using git.
Did all work on Ubuntu after pretty much a lifetime of Windows (initially to separate work from gaming) and finally swapped MS Visual Studio for Atom.
When it's finished I'm going to publish it on GitHub, which will also be a first for me. Might try out some CI platform while I'm at it.
tl;dr: wrote some js, felt good2 -
So I do not get why people use ReactJS. I hate it. for 3 years passionately. And I have to work with it every day.
- one-way data binding
this makes you write twice as much code, which will have twice as much bugs, you need to read through twice as much code from other devs.
- mixing html and JS
after all I like to pour my coffee on my omlette so I can eat and drink at the same time in the morning. This kills productivity and ugly AF
- not unified
Every dev uses their own special snowflake framework with React there is no unified way of doing things and you cannot use your familiar tools. Every project you need to start over from zero.
- Bugs bugs bugs
infinite loops, max update depth reached, key not present on list element. Let me ask you something dear ReactJS. If you know that there should be a unique key on that element. Why cannot you just put it there and shut the f up?
- works reeaally slow when compiled with TS
ReactJS was never designed to work with TS and now the tools for it are really slow. And why TS? Explicit contract is always better than an implicit contract. TS helps you in coding time, but for some reason React devs decided to worth 3 seconds to wait for compile and then realize you mad an error. ReactJS is bad and inefficient so stop making projects with it please.9 -
My Gripe With Implicit Returns
In my experience I've found that wherever possible code should be WYSIWYG in terms of the effects per statement. Intent and the effects thereof should always be explicit per statement, not implicit, otherwise effects not intended will eventually slip in, and be missed.
It's hard to catch, and fix the effects of a statement intent where the statement in question is *implicit* because the effect is a *byproduct* of another statement.
Worse still, this sort of design encourages 'pyramid coding recursion hell', where some users will first decompose their program into respective scopes, and then return and compose them..atomically as possible, meaning execution flow becomes distorted, run time state becomes dependent not on obvious plain-at-sight code, but on the run time state itself. This I've found is a symptom of people who have spent too much time with LISP or other eye-stabbingly fucky abominations. Finally implicit returns encourage a form of thinking where programmers attempt to write code that 'just works' without thinking about how it *looks* or reads. The problem with opaque-programming is that while it may or may not be effortless, much more time is spent in reading, debugging, understanding, and maintaining code than is spent writing it--which is obviously problematic if we have a bunch of invisible returns everywhere, which requires new developers reading it to stop each and every time to decide whether to mentally 'insert' a return statement.
This really isn't a rant, as much as an old bitter gripe from the guy that got stuck with the job of debugging. And admittedly I've admired lisp from afar, but I didn't want to catch the "everything is functional, DOWN WITH THE STATE" fever, I'm no radical.
Just god damn, think of the future programmer who may have to read your code eventually.2 -
What I learned about C# this weekend:
1. I am shit at C#
2. There is a nice selection of libraries both from Microsoft and third party.
3. I like a lot of the design decisions to not make foot guns. Like you can't use an int as a bool. You must be explicit so you don't have hidden type conversions.
4. WPF isn't terrible, but it took me a bit to figure out how to make sure the front end can see changes by triggering events when a property changes. I knew this was a thing, but it took forever to figure out how. The new terminology for things is tripping me up.
5. I am shit at C#.
So what do I get out of 1.5 days of banging my head against the wall? An https server that simulates a hardware device and exchanges json messages back and forth. It does what my python code is doing now.
I found a nice library for https:
https://github.com/chronoxor/...
I learned quite a bit reading the code from this library.8 -
Python ecosystem drives me nuts!
Not the language tho, i kinda like it, and some features are damn straight awesome.
But ecosystem... man!
The way ppl write code in it, the lack of documentation (or in quality of it)...
I recently wanted to check how library does one thing (debug purposes), and not only i had to track some method up 3 classes, the other method i hunted only by signature and still i have no idea how it ends up being accessible where it should...
"Explicit is better than implicit" my ass...
Also dev managed to make the code very unreadable. In Python. Language with such strong opinions about code formatting. HOW ?!!
And the worst part is, it wasn't that big of a library and didn't really need the full freaking Enterprise OOP treatment with layers over layers of generally named classes and fucked up architecture.
FUCK THAT LIB, FUCK THAT DEV, FUCK IT ALL !!!
PS.
Project seems to be abandoned for a year or two, so there is hardly an option to fix things with the author sadly :(3 -
"Reflective" programming...
In almost every other language:
1. obj.GetType().GetProperties()
or
for k, v in pairs(obj) do something end
or
fieldnames(typeof(obj))
or
Object.entries(obj)
2. Enjoy.
In C++: 💀
1. Use the extern keyword to trick compilers into believing some fake objects of your chosen type actually exist.
2. Use the famous C++ type loophole or structured binding to extract fields from your fake objects.
3. Figure out a way to suppress those annoying compiler warnings that were generated because of your how much of a bad practice your code is.
4. Extract type and field names from strings generated by compiler magic (__PRETTY_FUNCTION__, __FUNCSIG__) or from the extremely new feature std::source_location (people hate you because their Windows XP compilers can't handle your code)
5. Realize your code still does not work for classes that have private or protected fields.
6. Decide it's time to become a language lawyer and make OOPers angry by breaking encapsulation and stealing private fields from their classes using explicit template instantiation
7. Realize your code will never work outside of MSVC, GCC or CLANG and will always be reliant on undefined behaviors.
8. Live forever in doubt and fear that new changes to the compiler magic you abused will one day break your code.
9. SUFFER IN HELL as you start getting 5000 lines worth of template errors after switching to a new compiler.13 -
I did not think that making a serverless Discord bot would be such a learning experience. The code itself was easy. The hard part was the infrastructure, because I decided to automate it all with Terraform and deploy it on AWS.
Before this project, I had no idea how API Gateways worked. Now I still have very little idea how they work but I managed to build one anyway. Eventually. And then I had to figure out how to automate the deployment of a lambda layer and function that would both still be managed in the Terraform state, with any code changes triggering a rebuild and update for the resource.
And then I had to untangle a dependency mess because API Gateways have some weird issues where two resources that have no explicit dependencies on each other will throw an error if they don't deploy in the right order.
And then I went the wrong way with Github actions trying to conditionally chain multiple workflows together before I realized I could just put multiple jobs with conditions in a single workflow.
And now after all that work over the course of 2 days, I have a bot that does this:2 -
God damn the last few days:
JUST give me some modular-ish code that is a bit more explicit and doesn't measure every fucking thing over and over.
Like I get how taking a couple .lenght and a bunch of other variables adds up to "Make a meat lovers pizza".
But fuck man when the code goes all over the place just give me a block of code that measures all that shit in ONE PLACE a god damn pizzType variable that I can use elsewhere and just fucking know what is going on.
Every damn corner becomes this maze of measurements that you cant be sure is exactly the same unless you fucking watch every damn variable, I get how that happens but god damn.2 -
Consider,
```
namespace A;
macro m00 a,b;
··display a,b;
end macro;
macro m01 a;
··m00 a,$0A;
end macro;
m01 $24;
end namespace;
```
OUT: $0A24 ("$\n").
Ah, but you just HAD to fuck me in the ass, didncha fasm2? Initializing anal bleeding:
```
namespace B;
A.m01 $24;
end namespace;
```
OUT: illegal instruction 'm00'.
Explanation: macros carry no context, and so 'm00' is unreachable from 'B' namespace.
Could I maybe write a code-capture macro to generate a wrapper that resets the namespace? Yes, and in fact, I did; brilliant exercise in mystical mindfuckery.
But why try so hard? Just pass the fucking ref, like a MAN.
EXPLICIT CTX, BITCH.
```
define @CAS $non; current addressing space
define %ICE @CAS;
macro self:PA&; recursive macro, uuuh spooky-spooky
··match ice , %ICE;
····; self->proc(args)
····match =-=> proc (args) , PA;
······path.proc ice,args; pass ref and args
····; ^self->proc()
····else match =-=> proc () , PA;
······path.proc ice; pass ref only
····; ^sneaky sneaky. set current context when self ctx;
····else match cas , @CAS;
······%ICE equ cas.PA;
····end match;
··end match;
end macro;
```
ALL TOGETHER NOW
```
namespace A;
macro m00 ice,a,b;
··display a,b;
end macro;
macro m01 ice,a;
··self->m00(a,$0A); could also be 'ice.m00 ice,a,$0A' lmao
end macro;
end namespace;
namespace B;
self A; set ice
self->m01($24);
restore %ICE; back to previous
end namespace;
```
OUT: $0A24 ("$\n").
A-há, now **I** got you from behind motherfucker!
Final note: this is 100% perl DNA seeping through the cracks of my sanity and into a fresh fasm2 framework. Why? Dingle-dongle-jungle-berries, that's why. Also crack.
Have a nice wallop.45 -
Not loving the implicit return statement within Scala. I like to avoid else statements to keep the level of nesting low and do early return yet Scala doesn't allow that.
(I am aware that I should flatMap that shit though in some cases I just want a simple if not foo then throw exception line. And continue with the next block until I return something.)
So you either have to create if-else-nesting beats or use pattern matching. The latter seems overly complicated for this use case (though it has its moments).
I know that I can make the return explicit yet the linter warns against that. It feels so verbose and I currently do not see any benefits and would argue that the code becomes both harder to read and maintain.3 -
Nothing like trying to understand a single 1500-line source file that implements the API usage in the frontend. Without a single comment.
No, wait. There are comments! But it's only commented-out code. Or explicit shit (like "gets the version" before a getAssetVersion function).
Functions with unused parameters? ✅
Weird var names (like "tmpX")? ✅
`console.log(var)` everywhere? ✅
Long-ass lines with 150+ chars? ✅
Duplicate code? ✅✅
Not a single interface was used so everything is var: any? ✅
Random unreadable RegEx? ✅
If-chains of 6+ more levels? ✅
Many `else if` towers instead of a switch? ✅
And did I mention it was written by a fucker who can't speak proper English so shit like visiable, cataloge and isExist is everywhere? Yeah.
Fun day at the office reading spaghetti code 🙃 -
Browser automation is a PITA. I’m going on my fourth side mission with this crap and I honestly still look like a newbie. I’ve tried Java Selenium with Chrome, Excel VBA with IE9, Vanilla JS in the browser console, and tonight I’m thinking to concoct some kind of hybrid CDP & Selenium approach in Chrome. Never used CDP before, not even sure where to start but I heard it sucks like anything else unless you get some extra libraries and plugins and stuff.
It doesn’t help that I can’t get just anything I want from our IT Department. It would be another PITA to ask for puppeteer. If puppeteer is totally legit please let me know.
Selenium sucks. The buttons don’t click, the waits don’t wait. Its unusable. Iframes are annoying as all hell but I can deal with that. HTML Tables suck too. It doesn’t help I have to restart my whole java program and whole Chrome every time an element doesn’t get picked correctly. Scripting one single element can take all fucking night.
Chrome dev tools what the fuck. Why the fuck is the DOM explorer in the same window as the web page I’m working on?? I can’t undock it. Am I supposed to use a fucking TV screen to work with this bastard?? If I use the remote chrome tools on port 9225 or whatever - It Still Renders The Whole Fucking Page Alongside The Console. Get Out Of My Way!!! The nested HTML CODE IS ONE CHARACTER WIDE ALL THE TIME. I can’t for the life of me figure out what the fuck I’m looking at. Haven’t you people ever heard of A HORIZONTAL SCROLL BAR at least.
Fuck I tried using getElementById, and the Xpath thing and its not all that great seeing I have seemingly 1000s of nested Divs all over the god damned place oftentimes containing a single element. I’m finally on chrome now should I learn Jquery now? I mean seriously wtf.
I use this one no code tool for dev it has web automation built in. As you can imagine its just as broken as anything else!! I have 10 screens to navigate it gets stuck on the second screen all the damn time. Fuck I love clicking the buttons when my script misses and playing catch up with it.
So as a work around to Selenium not waiting even 1 millisecond when I use explicit wait or implicit wait or fluent wait, I’m guessing maybe I can attach both Chrome Dev Tools Protocol (CDP as ive called it earlier) and selenium to the same browser and maybe I can use CDP to perform a Wait with any degree of success. Selenium will do nothing more than execute vanilla javascript Element.click(); This is the only way I know to even ACTUALLY use selenium beyond the simplest html documents possible. Hell I guess CDP can execute js idk.
I can’t get the new selenium that has CDP but I do have some buggy ass selenium from a few years back. Yeah, I remember reading there was a pretty impactful regression defect in the version I have. Maybe I’m being gaslighted by some shit copy of selenium?
The worst part is that I do seem to be having issues that the rest of the internet’s devs do not seem to be having. People act like browser automation is totally viable and pretty OK. How in the fuck hell is my Selenium Test Suite going to be more reliable my application under test?!!?? I’ll have more fucking bugs in my test suite than in my application. Today, I have less than half a test script and, I. already. fucking. do.
I am still SUPER PISSED at the months of 12 hour days (always 8 hours spent on normal sprint work btw only 4 to automation) I spent trying to automate our regression tests. I got NOWHERE.
I did learn a lot about HTML and JS though like I’m not that mad…but I’m just trying to emphasize my achievement on my task was zero.
The buttons don’t click. There are so many divs and I swear you sometimes need to select a div somewhere in the middle sometimes to get it working. The waits don’t wait. XHR requests are invisible. Java crashes 100 times before I find an xpath and thread.sleep() combo that works. I have no failure modes to use — Sometimes I click the same element 20x in a script because I have no way to know if it clicked the first time! Sometimes you gotta scroll the page to make the click work. So many click methods all broken. So many wait methods all broken. Its not just the elements don’t click! There are so many ways to click that almost work but surely they all fail the same in the end. ok at this point I’m just repeating myself…
there yet even more issues that I can’t remember…and will soon remember as I journey into this project yet again…
thanks for reading I hope I entertained and would love to hear your experience!5 -
Top 12 C# Programming Tips & Tricks
Programming can be described as the process which leads a computing problem from its original formulation, to an executable computer program. This process involves activities such as developing understanding, analysis, generating algorithms, verification of essentials of algorithms - including their accuracy and resources utilization - and coding of algorithms in the proposed programming language. The source code can be written in one or more programming languages. The purpose of programming is to find a series of instructions that can automate solving of specific problems, or performing a particular task. Programming needs competence in various subjects including formal logic, understanding the application, and specialized algorithms.
1. Write Unit Test for Non-Public Methods
Many developers do not write unit test methods for non-public assemblies. This is because they are invisible to the test project. C# enables one to enhance visibility between the assembly internals and other assemblies. The trick is to include //Make the internals visible to the test assembly [assembly: InternalsVisibleTo("MyTestAssembly")] in the AssemblyInfo.cs file.
2. Tuples
Many developers build a POCO class in order to return multiple values from a method. Tuples are initiated in .NET Framework 4.0.
3. Do not bother with Temporary Collections, Use Yield instead
A temporary list that holds salvaged and returned items may be created when developers want to pick items from a collection.
In order to prevent the temporary collection from being used, developers can use yield. Yield gives out results according to the result set enumeration.
Developers also have the option of using LINQ.
4. Making a retirement announcement
Developers who own re-distributable components and probably want to detract a method in the near future, can embellish it with the outdated feature to connect it with the clients
[Obsolete("This method will be deprecated soon. You could use XYZ alternatively.")]
Upon compilation, a client gets a warning upon with the message. To fail a client build that is using the detracted method, pass the additional Boolean parameter as True.
[Obsolete("This method is deprecated. You could use XYZ alternatively.", true)]
5. Deferred Execution While Writing LINQ Queries
When a LINQ query is written in .NET, it can only perform the query when the LINQ result is approached. The occurrence of LINQ is known as deferred execution. Developers should understand that in every result set approach, the query gets executed over and over. In order to prevent a repetition of the execution, change the LINQ result to List after execution. Below is an example
public void MyComponentLegacyMethod(List<int> masterCollection)
6. Explicit keyword conversions for business entities
Utilize the explicit keyword to describe the alteration of one business entity to another. The alteration method is conjured once the alteration is applied in code
7. Absorbing the Exact Stack Trace
In the catch block of a C# program, if an exception is thrown as shown below and probably a fault has occurred in the method ConnectDatabase, the thrown exception stack trace only indicates the fault has happened in the method RunDataOperation
8. Enum Flags Attribute
Using flags attribute to decorate the enum in C# enables it as bit fields. This enables developers to collect the enum values. One can use the following C# code.
he output for this code will be “BlackMamba, CottonMouth, Wiper”. When the flags attribute is removed, the output will remain 14.
9. Implementing the Base Type for a Generic Type
When developers want to enforce the generic type provided in a generic class such that it will be able to inherit from a particular interface
10. Using Property as IEnumerable doesn’t make it Read-only
When an IEnumerable property gets exposed in a created class
This code modifies the list and gives it a new name. In order to avoid this, add AsReadOnly as opposed to AsEnumerable.
11. Data Type Conversion
More often than not, developers have to alter data types for different reasons. For example, converting a set value decimal variable to an int or Integer
Source: https://freelancer.com/community/...2 -
Rant and geniune question:
How can a group of 'devs' make 2 functional(enough unfortunately), many active users, phone apps, not just basic af, over a few years... but apparently not know how to make a working referral link of any kind???
Am i missing something like referral code link logic being extremely hard for typical devs to understand? Or some odd or trending reason for for forcing manual, explicit, referral code binding instead of a simple (imo) link that attributes the new user account to the referring user's? If you dont believe me u can check either/both of their actively running, decent/moderate size user base apps, claw eden (on play store) and/or claw party (possibly only via finding an apk... at least not on google play).
They have legit rewards, relatively very fair/honest policy... etc... i get 60+ items won/delivered a month (i have paranormal crane game skills #AutisticSuperpower) which i donate the vast majority of to charity (1 of 3 reasons the IRS reeeeally dislikes me).
Im just baffled by this apparent inability.2 -
Hot take: Rust doesn't go far enough with explicit clone; dropping any type that isn't trivially copiable should also be explicit. I don't mean that it should be leaked if you don't delete it, I mean that the compiler should force you to explicitly say
"I am done with this object, anything that had to know about it has already been notified and either there isn't a single last point of use or it isn't a clone, therefore dropping is justified."
This is the whole meaning of dropping a complex object. I think that this is far too strong a statement to imply in bulk for every value in scope at the end of the function.4