Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
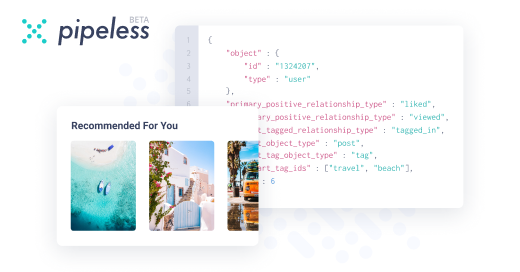
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "matrices"
-
Started being a Teaching Assistant for Intro to Programming at the uni I study at a while ago and, although it's not entirely my piece of cake, here are some "highlights":
* students were asked to use functions, so someone was ingenious (laughed my ass off for this one):
def all_lines(input):
all_lines =input
return all_lines
* "you need to use functions" part 2
*moves the whole code from main to a function*
* for Math-related coding assignments, someone was always reading the input as a string and parsing it, instead of reading it as numbers, and was incredibly surprised that he can do the latter "I always thought you can't read numbers! Technology has gone so far!"
* for an assignment requiring a class with 3 private variables, someone actually declared each variable needed as a vector and was handling all these 3 vectors as 3D matrices
* because the lecturer specified that the length of the program does not matter, as long as it does its job and is well-written, someone wrote a 100-lines program on one single line
* someone was spamming me with emails to tell me that the grade I gave them was unfair (on the reason that it was directly crashing when run), because it was running on their machine (they included pictures), but was not running on mine, because "my Python version was expired". They sent at least 20 emails in less than 2h
* "But if it works, why do I still have to make it look better and more understandable?"
* "can't we assume the input is always going to be correct? Who'd want to type in garbage?"
* *writes 10 if-statements that could be basically replaced by one for-loop*
"okay, here, you can use a for-loop"
*writes the for loop, includes all the if-statements from before, one for each of the 10 values the for-loop variable gets*
* this picture
N.B.: depending on how many others I remember, I may include them in the comments afterwards19 -
My favorite client just brought in a new team member who thinks he's god's gift to web development and design. Every week he gives me a long list of things he thinks are wrong with the website.
Now he's cloning pages of the site and adding hideously distorted images and excel screenshots of information matrices formatted the way he wants them. And he wants them published as he has made them because his ideas are obviously the best ones! (guess who he voted for)
He also claims that nobody can figure out how to purchase anything on the site, including him! Even after I've made it so you'd have to be frickin' Helen Keller not to be able to stumble over a BIG FAT BUY NOW BUTTON literally everywhere you look because this site is for geriatric senile MORONS who can't click their way out of a paper bag!!!5 -
And once again:
18:00: *writing a Mandelbrot algo in glsl for the GPU*
19:00: "This should be working now..."
22:00: "why isn't it working??!"
22:30: "Oh my uniform vectors become zero when they arrive on the GPU"
01:00: "Oh. I uploaded them as matrices..."
I wasted about 4 fucking hours because I suck dick.5 -
I could bitch about XSLT again, as that was certainly painful, but that’s less about learning a skill and more about understanding someone else’s mental diarrhea, so let me pick something else.
My most painful learning experience was probably pointers, but not pointers in the usual sense of `char *ptr` in C and how they’re totally confusing at first. I mean, it was that too, but in addition it was how I had absolutely none of the background needed to understand them, not having any learning material (nor guidance), nor even a typical compiler to tell me what i was doing wrong — and on top of all of that, only being able to run code on a device that would crash/halt/freak out whenever i made a mistake. It was an absolute nightmare.
Here’s the story:
Someone gave me the game RACE for my TI-83 calculator, but it turned out to be an unlocked version, which means I could edit it and see the code. I discovered this later on by accident while trying to play it during class, and when I looked at it, all I saw was incomprehensible garbage. I closed it, and the game no longer worked. Looking back I must have changed something, but then I thought it was just magic. It took me a long time to get curious enough to look at it again.
But in the meantime, I ended up played with these “programs” a little, and made some really simple ones, and later some somewhat complex ones. So the next time I opened RACE again I kind of understood what it was doing.
Moving on, I spent a year learning TI-Basic, and eventually reached the limit of what it could do. Along the way, I learned that all of the really amazing games/utilities that were incredibly fast, had greyscale graphics, lowercase text, no runtime indicator, etc. were written in “Assembly,” so naturally I wanted to use that, too.
I had no idea what it was, but it was the obvious next step for me, so I started teaching myself. It was z80 Assembly, and there was practically no documents, resources, nothing helpful online.
I found the specs, and a few terrible docs and other sources, but with only one year of programming experience, I didn’t really understand what they were telling me. This was before stackoverflow, etc., too, so what little help I found was mostly from forum posts, IRC (mostly got ignored or made fun of), and reading other people’s source when I could find it. And usually that was less than clear.
And here’s where we dive into the specifics. Starting with so little experience, and in TI-Basic of all things, meant I had zero understanding of pointers, memory and addresses, the stack, heap, data structures, interrupts, clocks, etc. I had mastered everything TI-Basic offered, which astoundingly included arrays and matrices (six of each), but it hid everything else except basic logic and flow control. (No, there weren’t even functions; it has labels and goto.) It has 27 numeric variables (A-Z and theta, can store either float or complex numbers), 8 Lists (numeric arrays), 6 matricies (2d numeric arrays), 10 strings, and a few other things like “equations” and literal bitmap pictures.
Soo… I went from knowing only that to learning pointers. And pointer math. And data structures. And pointers to pointers, and the stack, and function calls, and all that goodness. And remember, I was learning and writing all of this in plain Assembly, in notepad (or on paper at school), not in C or C++ with a teacher, a textbook, SO, and an intelligent compiler with its incredibly helpful type checking and warnings. Just raw trial and error. I learned what I could from whatever cryptic sources I could find (and understand) online, and applied it.
But actually using what I learned? If a pointer was wrong, it resulted in unexpected behavior, memory corruption, freezes, etc. I didn’t have a debugger, an emulator, etc. I had notepad, the barebones compiler, and my calculator.
Also, iterating meant changing my code, recompiling, factory resetting my calculator (removing the battery for 30+ sec) because bugs usually froze it or corrupted something, then transferring the new program over, and finally running it. It was soo slowwwww. But I made steady progress.
Painful learning experience? Check.
Pointer hell? Absolutely.4 -
math be like:
"Addition (often signified by the plus symbol "+") is one of the four basic operations of arithmetic; the others are subtraction, multiplication and division. The addition of two whole numbers is the total amount of those values combined. For example, in the adjacent picture, there is a combination of three apples and two apples together, making a total of five apples. This observation is equivalent to the mathematical expression "3 + 2 = 5" i.e., "3 add 2 is equal to 5".
Besides counting items, addition can also be defined on other types of numbers, such as integers, real numbers and complex numbers. This is part of arithmetic, a branch of mathematics. In algebra, another area of mathematics, addition can be performed on abstract objects such as vectors and matrices.
Addition has several important properties. It is commutative, meaning that order does not matter, and it is associative, meaning that when one adds more than two numbers, the order in which addition is performed does not matter (see Summation). Repeated addition of 1 is the same as counting; addition of 0 does not change a number. Addition also obeys predictable rules concerning related operations such as subtraction and multiplication.
Performing addition is one of the simplest numerical tasks. Addition of very small numbers is accessible to toddlers; the most basic task, 1 + 1, can be performed by infants as young as five months and even some members of other animal species. In primary education, students are taught to add numbers in the decimal system, starting with single digits and progressively tackling more difficult problems. Mechanical aids range from the ancient abacus to the modern computer, where research on the most efficient implementations of addition continues to this day."
And you think like .. easy, but then you turn the page:17 -
Work for 2 days trying to solve a Level 2 foobar problem and then realize I need to know linear algebra to solves matrices programmatically... And the results have to be in Fractions10
-
#machinelearning #ml #datascience #tensorflow #pytorch #matrices #dsjoke/meme tensor flow and ml/ai is new helloworld deep learning pytorch machinelearning tensorflow lite tensorflow6
-
Shit Developers say:
Fuck you Jasmine and your camelCase
I’ve been wrestling cucumbers all day
Oh no all the cucumbers are broken
In a fit of refactoring madness I have gone and changed a lot
Did you seriously just give ME nil?... No!
If the shit sticks, then we put nice paint on it
Fucking red dot motherfucker (Ben and his failing specs)
You know what we don’t do often..kill each others builds. Kill them and reschedule for later. Mwahaha ha ha.
This build is going to be so rad...(5mins later)...Ok this is not going to pass..I can feel it in my waters!
Can i do that in a digital way or do i have to move my meaty body downstairs to find him?
All the donkeys have be out the gate by sundown
God, imagine if you could patent mathematical solutions
actually, I wouldn't be surprised if you can in the states "no, you can't use a laplace transform, you haven't got the rights, you have to use a less accurate transform on your matrices"
ooooo a boolean that's phrased in the negative, my favourite for code review destruction!
Fuck the police i'll call the object here
Web RTC - its super easy, all you have to do is..probably some hard stuff
I want to go to that conference so I can start arguments with dickheads about semicolons. Just for fun.
This this is not the same as that this.
Can’t come to work I can’t find any clothes. It’s best for everyone if I just don’t come in. ...2 hours later... Yeah my clothes were just in the other room and i couldn’t be fucked moving
(OH about bad bug reports) - you know when they are all like oh joogly joogly doesn’t doodle doodle and it should wobbly doodle you know? and im all like fuck i don’t know any of that shit you are talking about.
Him: "I don’t like it, it’s against REST convention its so 2006 that my eyes are bleeding. As a privileged white male i feel entitled to complain about this." Me: "you. were. eleven in 2006
Source: Kellective Github2 -
I wrote a Blender plugin that uses vector math, matrices, calculus, trigonometry, and likely other types of math. There's recursion, filesystem access, image processing, interface logic, and on and on.
And worst of all - other people are expected to use it, so there's added pressure to do a good job.
Oh, the hours I spent trying to figure out why the imported geometry looked like an exploded mess. Fumbling around with mathematics I didn't fully understand was exhausting. Finding help was impossible at times because I didn't have the vocabulary to even describe the problems I was having. And getting it to complete an import before the heat death of the universe was not easy.
Every time I made progress and thought I was done, I would discover a bug that other importers didn't have, leaving me to sift through languages that definitely aren't Python to see if I could reverse engineer the logic they used.
I almost gave up a few times, but didn't.
Now I have something that, while not used by many people, works very well, is very efficient, and doubles as a palette cleanser when I need to do something for fun or for a challenge. Plus I learned a lot along the way.4 -
Got a mathematics library I develop and maintain. Someone filed a feature request ticket for matrices of matrices. As in, each value of the primary matrix is another matrix. Not understanding why anyone would need such a convoluted concept, I asked for clarification.
Response: "This piece of shit library isn't feature complete without it, now stop being a lazy fag in your mother's basement and actually do something"
Lololololol. Sure thing. Let me go waste two or more weeks of my life developing something i've never seen used in math, without any justification beyond "feature".4 -
Still on the primenumbers bender.
Had this idea that if there were subtle correlations between a sufficiently large set of identities and the digits of a prime number, the best way to find it would be to automate the search.
And thats just what I did.
I started with trace matrices.
I actually didn't expect much of it. I was hoping I'd at least get lucky with a few chance coincidences.
My first tests failed miserably. Eight percent here, 10% there. "I might as well just pick a number out of a hat!" I thought.
I scaled it way back and asked if it was possible to predict *just* the first digit of either of the prime factors.
That also failed. Prediction rates were low still. Like 0.08-0.15.
So I automated *that*.
After a couple days of on-and-off again semi-automated searching I stumbled on it.
[1144, 827, 326, 1184, -1, -1, -1, -1]
That little sequence is a series of identities representing different values derived from a randomly generated product.
Each slots into a trace matrice. The results of which predict the first digit of one of our factors, with a 83.2% accuracy even after 10k runs, and rising higher with the number of trials.
It's not much, but I was kind of proud of it.
I'm pushing for finding 90%+ now.
Some improvements include using a different sort of operation to generate results. Or logging all results and finding the digit within each result thats *most* likely to predict our targets, across all results. (right now I just take the digit in the ones column, which works but is an arbitrary decision on my part).
Theres also the fact that it's trivial to correctly guess the digit 25% of the time, simply by guessing 1, 3, 7, or 9, because all primes, except for 2, end in one of these four.
I have also yet to find a trace with a specific bias for predicting either the smaller of two unique factors *or* the larger. But I haven't really looked for one either.
I still need to write a generate that takes specific traces, and lets me mutate some of the values, to push them towards certain 'fitness' levels.
This would be useful not just for very high predictions, but to find traces with very *low* predictions.
Why? Because it would actually allow for the *elimination* of possible digits, much like sudoku, from a given place value in a predicted factor.
I don't know if any of this will even end up working past the first digit. But splitting the odds, between the two unique factors of a prime product, and getting 40+% chance of guessing correctly, isn't too bad I think for a total amateur.
Far cry from a couple years ago claiming I broke prime factorization. People still haven't forgiven me for that, lol.6 -
So a little bit explanation to my last fuck rant
I was trying to make a cuda code faster, specifically eigen value decomposition for 12 by 12 matrices. For a week a made a fast and accurate version and a faster but less accurate version, both are faster than cuda. Then I was thinking about how to make the faster version more accurate.
Then we had this idea of using power iterations. And honestly I hoped it won’t work. But then, fuck me it worked, which means I had more work to do.
But hey, at least now I’m way faster than cuda on this18 -
I just cant Wrap my head around matrices and linear Algebra.
I am currently doing a uni course about this and need to implement some eigenvalue solvers. I somehow manage to implement the stuff with the help of pseudocode and the internet but I have nö idea conceptually what all these things like norms, eigenvalues, conditioning etc are supposed to tell me and why.
How do people handle this so naturally?8 -
Whenever I'm looking at automata, matrices, sets, anything confusing and maths based, I always remind myself how I used to be in awe of the year 6's (5th grades) getting to learn about negative numbers...
Negative number seem so much easier than sets and strings... -
Taking an online test to practise programming. They give me code that takes two 3x3 matrices as inputs and outputs a third. I have to multiply them together and ouput results.
Within minutes I've worked my head around it, got four lines of code to do it all. Output fails.
Twenty minutes later, nearly failing the time limit I find out that they couldn't output the array proplerly in C++
Are3[I, j];
;( What a hair puller.1 -
Fuck you MATLAB and your shitty inefficient for loops. Now I have to rewrite most of my code to use matrices instead of structures cause you take so long. Fuck you and your stupid ability to scale my neural network.....who needed sleep anyway6
-
Why is vectorization library faster than hand-written for loops ? I mean, somewhere down the line, the matrices/vectors must be multiplied (or any other operation) and thus be one-by-one (for loop??) calculated and stored.
Why is it then faster to use these libraries than just manually writing for loops all over the place ?
I guess some low level magic (OpenBLAS ?) goes on there but I just don't see it..
P.S. [Would have posted it on stack overflow but I'd be ripped apart so I'm pioneering new ways]8 -
First and foremost, students should be carefully taught the logic and mentality behind programming. Most of the time I see that the introductory programming courses waste so much energy in teaching the language itself. So students kinda just get fucked cause many people end up ending the course without having actually gained the "programming perspective".
Stop teaching pointers and lambdas and even leave the object oriented stiff till later. If a student doesn't know why we use a For loop then how can they learn anything else.
I believe once that thing in your brain clicks about programming, everything goes smooth from there... kinda :P
Second of all, and this pertains mainly to the engineering and science disciplines.
We need a fundamental and strong mathematical foundation. And no I don't mean taking fucking double integrals. Teach us Linear Algebra, Graph theory, the properties of matrices, and Probability theory.
One of the things I suffered from most and regret in university is having a weak foundation in math and having to spend more time catching myself up to speed.
It's so annoying reading a paper on a new algorithm or method and feeling like an idiot because I can't understand what magic these people did.
Numerical Methods...
Ok this is more deeper, maybe a 2nd year course.
But this is something we take for granted.
Computers don't magically add and subtract and multiply.
They fuck up.
And it'll bite you in the ass if you're not even aware that the computer we all love so much isn't as perfect as we think
Some hardware knowledge.
Probably a basic embedded systems course with arduinos
just so you can get a feel for how our beautiful software actually makes those electrons go weeeeeeeee
And finally
Practice practice
Projects projects
like honestly
just give me the internet and some projects
Ill learn everything else
Projects are the best motivation
I hate this purely theoretical approach
where we memorize or read code and write these stupid exams
Test what we are capable off
make us do projects that take sleepless nights and litres of coffee
And judge our methods, documentation, team work, and output
Team work skills and tools (VCS, communicating, project management, etc.)
Documentation and Reporting
Properly
:)
maybe even with LaTeX :D
Yeah that's the gist of whats on my mind at the moment regarding an ideal computer science education
At least the foundations
The rest I leave it to the next dude. -
Exploring dedekinds and kronecker products (script at - https://pastebin.com/dDuT3dTp)
and the thing I immediately notice, if you output the matrix is that it is a lower triangular matrix. I don't know a lot about the kronecker or matrices in general, but if dedekinds can be generated in this manner, shouldn't some standard approaches like back substitution or forward substitution be applicable here or am I off in left field on this?6 -
Y’all wouldn’t happen to have some handy mental model for remembering how to iterate through input without being an idiot about it, would you?
Referring to problems like having to get all possible substrings from a given string, etc.
Wishful thinking on my part, probably, but I figure it doesn’t hurt to ask. <39 -
How useful is my degree? I'm not sure to be honest. I did get to dive into a lot of subject matter which I find interesting and challenging. I also had to learn stuff I hate (solving matrices of differential equations). Strangely though, even though I doubt I will ever use this I am proud of myself for having slugged though it.
The teachers were helpful and supportive, I got to study in groups and had access to resources such as the university's GPU cluster.
In my day2day? So far, I cannot see anything I use directly. However, the university forced me to learn to pick up different technologies quickly, read the documentation, ask for help when your don't understand something. So, in that regard I think I profited from university.
I wasn't the best student by a long shot. My class mates helped me a lot. I struggled A LOT. Having been in the recieving end of a helping hand, o return the favour where ever I can. -
I need to tell you the story of my MOAB (Mother of all bugs).
I need to write some stuff in C (which i am fairly used to) and have a function that allocates memory for a Matrix on the heap. The matrix has a rows and columns property and an associated data array, so it looks like this
struct Matrix{
uint8_t rows;
uint8_t columns;
uint8_t data[];
}
I allocate rows*columns + 2 bytes of memory for it.
I also have a function to zero it out which does something like this
for(int i=0; i < rows*columns;i++){
data[i]=0;}
Let‘s come to the problem:
On my Mac the whole stuff works and passes all tests. We tried the code on a Linux machine and suddenly the code crashed in various places, sometimes a realloc got an invalid pointer, sometimes free got an invalid pointer and basically the code crashed at arbitrary points randomly.
I was confused af because did i really make THAT many errors?
I found out that all errors occured when testing my matrices so i looked more into it and observed it through the debugger.
Eventually i came to the function that zeroes out my matrix and it went unusually high and wondered if my matrix really was that big.
Then i saw it
The matrix wasn‘t initialised yet
It had arbitrary data that was previously in the heap.
It zeroed out a huge chunk of the heap space.
It literally wrote a zero to a shitload of addresses which invalidated many pointer.
You can imagine my facepalm2 -
Ah the joys of debugging a finite element mesh using Backwards Euler...
Accidentally added one variable twice which produced a change of 0.001 in some of my matrices compared to everyone else. Strangely enough produces a rather larger error when summed over 100,000 iterations....
God damn you transient problems.... -
I am trying to decompose a 3D matrix using python library scikit-tensor. I managed to decompose my Tensor (with dimensions 100x50x5) into three matrices. My question is how can I compose the initial matrix again using the decomposed matrix produced with Tensor factorization? I want to check if the decomposition has any meaning. My code is the following:
import logging
from scipy.io.matlab import loadmat
from sktensor import dtensor, cp_als
import numpy as np
//Set logging to DEBUG to see CP-ALS information
logging.basicConfig(level=logging.DEBUG)
T = np.ones((400, 50))
T = dtensor(T)
P, fit, itr, exectimes = cp_als(T, 10, init='random')
// how can I re-compose the Matrix T? TA = np.dot(P.U[0], P.U[1].T)
I am using the canonical decomposition as provided from the scikit-tensor library function cp_als. Also what is the expected dimensionality of the decomposed matrices.1