Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
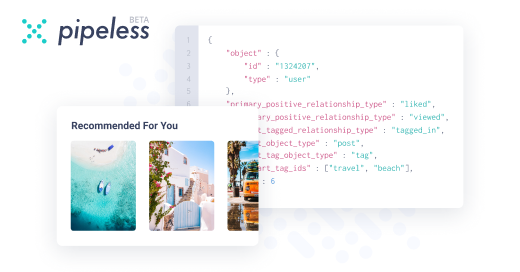
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "nested arrays"
-
I'M SO PROUD, I WROTE A FULLY-FUNCTIONAL JSON PARSER!
I used some data from the devRant API to test it :D
(There's a lot of useful tests in the devRant API like empty arrays, mixed arrays and objects, and nested objects)
Here's the devRant feed with one rant, parsed by Lua!
You can see the type of data (automatically parsed) before the name of the data, and you can see nested data represented by indentation.
The whole thing is about 200 lines of code, and as far as I can tell, is fully-featured.24 -
Why is it so important to some people to claim that "HTML and CSS are not programming languages"? I get it, you're a REAL programmer working with arrays, maybe tuples, objects and possibly direct memory management. Who the fuck has a right to call themselves a programmer for writing some brain dead markup or poorly designed selectors, right? Who fucking cares for semantic tags or nested selectors?
Just think for a few seconds about when you were taking your first baby steps to becoming the GOD ROCKING MEMORY HANDLER THAT WRITES _REAL_ CODE that you are today, and how good it felt to be able to create something that appeared on your screen. It felt pretty awesome, yeah?
Now imagine if someone much more experienced than you told you "You're not a real programmer, that is not real programming. You should see what I do, I do real programming".
I think you get it. Why spend your energy spreading bad vibes when you could spend it on something more productive. Like reading up on the new CSS4 specs ;)17 -
I know it's not done yet but OOOOOH boy I'm proud already.
Writing a JSON parser in Lua and MMMM it can parse arrays! It converts to valid Lua types, respects the different quotation marks, works with nested objects, and even is fault-tolerant to a degree (ignoring most invalid syntax)
Here's the JSON array I wrote to test, the call to my function, and another call to another function I wrote to pretty print the result. You can see the types are correctly parsed, and the indentation shows the nested structure! (You can see the auto-key re-start at 1)
Very proud. Just gotta make it work for key/value objects (curly bracket bois) and I'm golden! (Easier said than done. Also it's 3am so fuck, dude)15 -
Having to review an offshore C++ codebase made in Romania that the company I worked for they bought to control a wifi module on a complex RF mobile tech device that I can't legally give more details on.
If I could legally post this masterpiece, or should I say masterpiece-of-shit, all of you C++ dev would instantly get AIDS and all the existing types of cancer upon browsing it for 2 minutes.
It's laughably bad and unmaintainable. One of my colleague called it "the perfect example of human obfuscation" and it fits perfectly.
Think of a 100k LoC main function with nested loops and ifs with random sleep values, 1000 values of hardcoded 32 bits arrays declared globally in the first 10k lines for unknown reasons. Comments in Romanian mixed with english. Somehow, this shit works by some miracle.
The worst intern you can think of, while being piss drunk, could do better and it's no joke.5 -
!rant
!!pride
I tried finding a gem that would give me a nice, simple diff between two hashes, and also report any missing keys between them. (In an effort to reduce the ridiculous number of update api calls sent out at work.)
I found a few gems that give way too complicated diffs, and they're all several hundred lines long. One of them even writes the diff out in freaking html with colors and everything. it's crazy. Several of the simpler ones don't even support nesting, and another only diffs strings. I found a few possibly-okay choices, but their output is crazy long, and they are none too short, either.
Also, only a few of them support missing keys (since hashes in Ruby return `nil` by default for non-defined keys), which would lead to false negatives.
So... I wrote my own.
It supports diffing anything with anything else, and recurses into anything enumerable. It also supports missing keys/indexes, mixed n-level nesting, missing branches, nil vs "nil" with obvious output, comparing mixed types, empty objects, etc. Returns a simple [a,b] diff array for simple objects, or for nested objects: a flat hash with full paths (like "[key][subkey][12][sub-subkey]") as top-level keys and the diff arrays as values. Tiny output. Took 36 lines and a little over an hour.
I'm pretty happy with myself. 😁6 -
Saw a question on SO asking why foreach was slow with big data.
The code provided was 6 nested foreachs (basically a cartesian product between an array of arrays, and 4 other arrays).
Inside, a select query and an "update or create" operation.
"But why is foreach so slow?"4 -
Trying to work with an API that has no response object (it just returns empty arrays if something breaks) and it's JSON is not key-value.. just a bunch of dynamic nested values. just wow.3
-
This is so annoying, I had 9 diff. jobs the past 2 years and this is my 10th and if this doesn't change I might reconsider my options again.
I came to work at a company that pays me like a Junior and treats me as an intern. My 20yo "boss" who acts as a project owner/lead dev doesn't want to learn anything new and sees any improvement as a waste of money. The problem is he thinks hes a great programmer but he doesn't know shit. Im mainly working on the Laravel installation because "I claimed I know Laravel". And its absolute garbage. They haven't used a single Laravel features besides routes and everything else is vanilla PHP. They write for loops that loop through $_REQUEST to remove a single character. Write 100 deep nested ifs and they abuse Elasticsearch to the point ES crashes because the program is using 1000 deep multidimensional arrays. Its only a webshop...
Everytime I try to make a suggestion like making the master branch protected, doing code reviews etc etc I get shut down because they are autistic and don't want anything to change.9 -
An anti-rant: I just made some code and out of nowhere it suddenly had an awesome feature that I didn't even program. No, not a euphemism for "bug", an actual feature.
Here's the story: A few months ago I made a shortcut for "System.out.println(…)" called "print(…)". Then I developed it further to also print arrays as "[1,2,3]", lists as "{1,2,3}", work with nested arrays and lists and accept multiple arguments.
Today I wanted to expand the list printing feature, which previously only worked for ArrayLists, to all types of List. That caused a few problems, but eventually I got it to work. Then I also wanted to expand it to all instances of Collection. As a first step, I replaced the two references to "List" with "Collection" and magically, no error message. So I tested it with this code:
HashMap<Integer, String> map = new HashMap<>();
map.put(1, "1");
map.put(2, "");
map.put(3, "a");
print(map);
And magic happened! The output was:
{1=1, 2=, 3=a}
That's awesome! I didn't even think yet about how I wanted to display key-value pairs, but Java already gave me the perfect solution. Now the next puzzle is where the space after the comma comes from, because I didn't program that in either.
I feel a bit like a character in "The subtle knife", who writes a barebones program to communicate with sentient elementary particles (believe me, it makes sense in context) and suddenly there's text alignment on the left and right, without that character having programmed any alignment.3 -
During my first semester of CS we were mostly using MatLab for basal scripting - assigning variables, learning about scope, that type of thing. I was excited to start learning programming but wanted to actually make something rather than reversing arrays and incrementing counters for weeks.
I discovered the image() function which takes a float[][] matrix and displays it as an image. I generated my arrays of random numbers and made a simple nested loop where I iterated over each element, averaging it with its neighbours, and - it worked on the first run! I made a freaking noise and blur filter!
That rush of planning it out, making it, and seeing it work I think is my main drive in coding. All the hours of undefined-but-they-are-tho import paths and mystery segfaults are worth it once there is that moment of "it lives!". -
Don't you ever try to translate code from one language to another. Instead grasp the problem and write the solution in the other language itself. You don't want to sit there for hours thinking if hashmaps are a good fit for phps nested arrays.1
-
Phps love of nested arrays is similar to that of structured programing and goto statements. You will do a lot of backtracking in both of them to see where things start. God bless PHP.3
-
!rant
Before I left my other company I was in the midst of finishing one project and I was ansious to finish everything to leave as a rockstar. Now, one of my js scripts brought a huge and long json response that had many nested items and arrays and whatnot. Instead of properly destructuring or finding a particular piece that went similarly to "status": "Verify input"(that was nested unser a shitload of items) i did the unspeakable......i stringified the whole object and just used indexOf.
I still feel guilty over it...but it works :P thing is, if it returns that it means that the user entered an invalid status into the app (it was an inventory application) but it works :P
Oh well. Mind you they thought it was going to take months and I finished in 1 week so yay. -
So I'm working on a snippet of JS to generate widgets for a custom data dashboard at the moment, in a project where I've been paired with a junior "developer" (he's more of a junior script monkey though), which is just plain painful...
Recently he wrote up a long message bitching about how my library API keeps changing, making it impossible for him to get any of his work done.. This particular message even made references to "writing his own widget library" and "stabbing me in the eye".
It's currently at version 0.1.0-ALPHA, just by the way. Major version 0 mother fucker.
Anyways, one of my colleagues stepped in the other day to try help him with the front-end stuff, which finally helped me get the feedback I was asking for. At which point we found out he's still currently working off a build I gave him 4 fucking weeks back.
Honestly though, I'd both love and hate to see him try make a library to do this: pull data from a non-standards company data API, parse said data from unnamed number arrays nested up to 4 levels deep, then morph that data into one of four different charts or one of five made up of custom markup.
All he has to do is create a UI to configure and present my widgets, but he can't even figure out how to integrate dependency management into his front-end project.
O.o
OMG. Can I stab him?? Pretty please?1 -
Aka... How NOT to design a build system.
I must say that the winning award in that category goes without any question to SBT.
SBT is like trying to use a claymore mine to put some nails in a wall. It most likely will work somehow, but the collateral damage is extensive.
If you ask what build tool would possibly do this... It was probably SBT. Rant applies in general, but my arch nemesis is definitely SBT.
Let's start with the simplest thing: The data format you use to store.
Well. Data format. So use sth that can represent data or settings. Do *not* use a programming language, as this can neither be parsed / modified without an foreign interface or using the programming language itself...
Which is painful as fuck for automatisation, scripting and thus CI/CD.
Most important regarding the data format - keep it simple and stupid, yet precise and clean. Do not try to e.g. implement complex types - pain without gain. Plain old objects / structs, arrays, primitive types, simple as that.
No (severely) nested types, no lazy evaluation, just keep it as simple as possible. Build tools are complex enough, no need to feed the nightmare.
Data formats *must* have btw a proper encoding, looking at you Mr. XML. It should be standardized, so no crazy mfucking shit eating dev gets the idea to use whatever encoding they like.
Workflows. You know, things like
- update dependency
- compile stuff
- test run
- ...
Keep. Them. Simple.
Especially regarding settings and multiprojects.
http://lihaoyi.com/post/...
If you want to know how to absolutely never ever do it.
Again - keep. it. simple.
Make stuff configurable, allow the CLI tool used for building to pass this configuration in / allow setting of env variables. As simple as that.
Allow project settings - e.g. like repositories - to be set globally vs project wide.
Not simple are those tools who have...
- more knobs than documentation
- more layers than a wedding cake
- inheritance / merging of settings :(
- CLI and ENV have different names.
- CLI and ENV use different quoting
...
Which brings me to the CLI.
If your build tool has no CLI, it sucks. It just sucks. No discussion. It sucks, hmkay?
If your build tool has a CLI, but...
- it uses undocumented exit codes
- requires absurd or non-quoting (e.g. cannot parse quoted string)
- has unconfigurable logging
- output doesn't allow parsing
- CLI cannot be used for automatisation
It sucks, too... Again, no discussion.
Last point: Plugins and versioning.
I love plugins. And versioning.
Plugins can be a good choice to extend stuff, to scratch some specific itches.
Plugins are NOT an excuse to say: hey, we don't integrate any features or offer plugins by ourselves, go implement your own plugins for that.
That's just absurd.
(precondition: feature makes sense, like e.g. listing dependencies, checking for updates, etc - stuff that most likely anyone wants)
Versioning. Well. Here goes number one award to Node with it's broken concept of just installing multiple versions for the fuck of it.
Another award goes to tools without a locking file.
Another award goes to tools who do not support version ranges.
Yet another award goes to tools who do not support private repositories / mirrors via global configuration - makes fun bombing public mirrors to check for new versions available and getting rate limited to death.
In case someone has read so far and wonders why this rant came to be...
I've implemented a sort of on premise bot for updating dependencies for multiple build tools.
Won't be open sourced, as it is company property - but let me tell ya... Pain and pain are two different things. That was beyond pain.
That was getting your skin peeled off while being set on fire pain.
-.-5 -
Working with nested arrays in MongoDb!! Who would have thought it would be such a pain to update data in nested arrays in a database! So frustrated!!!!
-
Y’all wouldn’t happen to have some handy mental model for remembering how to iterate through input without being an idiot about it, would you?
Referring to problems like having to get all possible substrings from a given string, etc.
Wishful thinking on my part, probably, but I figure it doesn’t hurt to ask. <39 -
Reading the source of a message queue system I'm planning on extending.
I don't see myself as a rockstar programmer or anything but the construction of arrays from hash tables, sorting those arrays and then a nested for loop to find matches really irks me. Luckily not on the critical message processing path but the stats collection thread. There are mutexes in play though that would probably delay processing a little bit when stats are collected. -
Ugh, retrieving specific data fields nested within several arrays and objects in Javascript/Json jacks me up every fucking time!!!
Anyone ever fuck with the MapQuest geolocation/geoqueries api??
I'm trying to retrieve the lat/lng values out of responses generated from submitted address strings, and it's nested about 8 json layers deep.
I feel like I'm overthinking this?
I can access the values in my web console, and can reach them after using the console to assign them to a temp var, but can't get to the values from my actual js code. Only when I run some business logic from the console.
Here's a shitty example of me explaining the tree:
[{...}]
0:
locations: Array(3)
0:
latLng:
lat: <data here>
lng: <data here)1 -
I’ve been doing a lot of solidity development lately in my professional life.
Now I get that nested arrays aren’t implemented yet. But it is still weird not being able to have an array of strings.
(Strings are arrays of characters and that would be a meted array)