Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
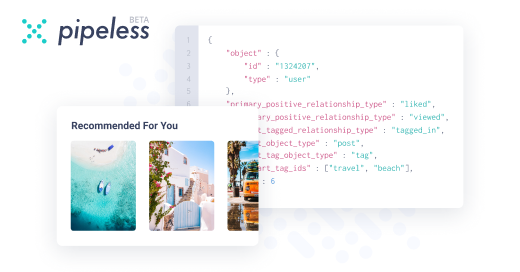
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "setter"
-
Last year, we had to do a big university project in randomly selected groups (5-6 students in every group).Three of the five guys were completely useless, I mean, both the other competent guy and me wrote around 20,000 lines of code each, the other ones wrote around 500 lines of code (combined).
After our first few meetings we quickly knew that we have to give them a small task which was so trivial that not even they can fuck it up. But we were wrong. Oh boy, so wrong.
They simply had to code the excel export of the data, which means they had to use two functions from a library and pass the correct data. But their solution was so bad, I lost faith in humanity and was fascinated by it at the same time.
For example, there was this simple class "Room", which had a few properties like size or number of seats and a few getter/setter etc. That was a core class and written by the other qualified guy. So how did the others fuck up the excel export? They somehow rewrote that class in German (although the other code was completely in English), implemented a function for each property that would write its value to a hardcoded cell in a hardcoded excel file.
And this was just the tip of the iceberg. Needlessly to say that I had to rewrite the whole export in the night before we had to present the project.5 -
I don't get it why there are so many people out, that think testing stupid model classes with getters and setter is needed.
Dude. There is no logic in this class ... it's pure data that gets not modified there (well except if you call the setter)
I've wasted way too much time writting useless unit test because some ppl want to masturbate on 100% code coverage 🤦🏻♂️12 -
So python does not have constants and the only way to create one is to create a class with a getter but no setter.
WHAT.THE.FUCK or am I just wrong?4 -
As I was refactoring a class in a TypeScript project, I changed calls from `this.config` to `this.getConfig()`.
Suddenly, the tests were failing as somehow the live credentials were used from within the test.
Digging deeper I discovered this.
interface Base {
public config;
public getConfig();
}
So far so good. Wondering why config needs to be public, though nothing too shabby, let's look further:
class MyImpl implements Base {
constructor() {
this.config = this.getConfig()
}
getConfig = () => someGlobalVar;
}
┻━┻︵ \(°□°)/ ︵ ┻━┻
Why would you do this? This breaks dependency injection completely.
In the tests, we were of course doing:
testMe = new MyImpl();
testMe.config = testConfig;
So even though you have a getter, you cannot call it safely as the global var would take precedence. It's rather used as a setter within the constructor. WTF.
Sad part is that this pattern is kept throughout the entire codebase. So yeah for consistency!?
(And yes, I found a quick workaround by doing
getConfig = () => this.config || someGlobalVar;
though still, who in their right mind would do something like this?)1 -
this is my legacy code, it's stupid, Change my mind.
a large number of lecturer and friends are against my thought. personally, i think this kind of code is told to be an OOP yet this is against the OOP concept. why ? first you assign the field to be private, but you implement Getter and Setter method later on, this is the same if you assign the field to be public in the first place.
another minor thing; yes this is old me, i use Bahasa Indonesia as a variable name.31 -
Girlfriend: I don't get why you love me...
Me: Maybe I should write a Setter method to make it clear.
She didn't understand that joke ._.3 -
If I find the genius who thought using getters and setters everywhere was a good idea, i'll gladly throw him into an active volcano.28
-
My dumbass removes a setter and then starts wondering why the API isn't accepting any query parameters anymore1
-
Imagine being the kind of officious prick who turns up to a bootcamp graduation evening and goes to each table in turn, condescending the graduate projects and telling them everything that they did wrong?
Imagine proceeding to try and 'break' one of the demonstration projects by 'injecting SQL into the url bar', and smashing the keyboard so fucking hard that the table collapses, beer spills over both demo laptops, and destroys them totally.
Imagine.1 -
other dev changed constructor from
public A(String val){
this.val = val;
}
to
public A(String val){
this.setVal(val);
}
this feels bad in many ways, but need some arguments to convince him that its wrong11 -
senior: we have no reason to change/mutate this property, we don't need a setter method, just set it once in the constructor
also senior: there's no reason to change/mutate this value, but i want you to write a setter method for it3 -
I'm staring at the setter portion of a legacy VB property I came across.
This is crazy... right?
Set(value As String)
_firstName = value.Substring(0, value.Length)
End Set4 -
"I don't think we should be playing with our privates {variables} like that" - framework designer
= context =
It was noticed that we have too many setter functions to change private variables just to do unit tests. So we had a small meeting to discuss what to do about this.
Options:
- don't do the test
- ignore till another time (ie: keep the functions till its a problem)
- put the variables into a provider
- use reflection (the above quote was a reaction to this option)6 -
Usually, when I programme for myself or in a German-only team and they agree, I/we do it in German because it makes naming things much simpler (no naming conflicts, never, and a strict visible separation between your code and libraries).
This time, I thought: "Nooo, let's do it in English, because, you know, reasons and it fits into the situation"...
Booom, stack overflow!
"How in the hell did that happen?" I never had a stack overflow before outside of recursive programming.
And what was it? I had made a class to encapsulate an API, added a property with getter and setter, naming in English and similar to the said API... very similar... in fact, the property had the same name as the API function, resulting in a getter calling itself over and over again.
This was a harmless mistake, and found very quickly, but it's interesting so see how a habit (or working method) probably prevented similar or worse sources of error in the past.3 -
Setter and getter are anti-patterns. Eradicate all of them from your code with no mercy and you'll see your code magically transform for the better.5
-
I wrote my first proper promise today
I'm building a State-driven, ajax fed Order/Invoice creation UI which Sales Reps use to place purchases for customers over the phone. The backend is a mutated PHP OSCommerce catalog which I've been making strides in refactoring towards OOP/eliminating spahgetti code and the need for a massive bootstrapper file which includes a ton of nonsense (I started by isolating the session and several crucial classes dealing with currency, language and the cart)
I'm using raw JS and jquery with copious reorganization.
I like state driven design, so I write all my data objects as classes using a base class with a simple attribute setter, and then extend the class and define it's attributes as an array which is passed to the parent setter in the construct.
I have also populateFromJson method in the parent class which allows me to match the attribute names to database fields in the backend which returns via ajax.
I achieve the state tracking by placing these objects into an array which underscore.js Observe watches, and that triggers methods to update the DOM or other objects.
Sure, I could do this in react but
1) It's in an admin area where the sales reps using it have to use edge/chrome/Firefox
2) I'm still climbing the react learning curve, so I can rapid prototype in jquery faster instead of getting hung up on something I don't understand
3) said admin area already uses jquery anyway
4) I like a challenge
Implementing promises is quickly turning messy jquery ajax calls into neat organized promise based operations that fit into my state tracking paradigm, so all jquery is responsible for is user interaction events.
The big flaw I want to address is that I'm still making html elements as JS strings to generate inputs/fields into the pseudo-forms.
Can anyone point me in the direction of a library or practice that allows me to generate Dom elements in a template-style manner.4 -
Question.js
How do I create a simple store for state management. I don't want to use redux. I achieved most of things I wanted I'm kinda stuck at something. Here say the store
obj = { attr1 : { some : { nested : ["json"] } } }
I used setter in es6 to avoid writing to attr1 after attr1 is set.
My dilemma here is how do I freeze the nested object being changed? I tried various ways arround Object.freeze. it didn't work. I'm looking for some way I can do it? Or someone can help me understand redux or vuex without going through all of their code. -
Fucking Quarkus. Fucking Panache. Fucking ORM.
I wanted to do a fucking simple projection. First this piece of fuck, the Panache, won't let me do a Projection because of a fucking bug, that haven't implemented it properly until 2.12 (fuck and you call this v2?). Ok, upgraded, to the latest 2.16, cuz why the fuck, i'm upgrading already. But now the whole fucking quarkus app won't start! Noice! Ok, fuck it, let's go down exactly to 2.12. Quarkus started, perfect. But now, this pice of fuck Hibernate says 'collection was evicted' whenever i tried to read a collection in the setter (Access.PROPERTY), which worked just fucking fine before. But okay, fuck you. I'll write a @PostLoad method, fine, just fuck off.
But that's not the end! Now it says I cannot write `select parent.someColl is not null and parent.collection is empty as canProcess` because "is empty" only supported in where clauses. What fucking wonderful system! Well, fuck you. I'll write a union query. But guess what! JPA standard does not support union queries, nor HQL (Eclipse Link does, btw). Ok, fuck this shit, let's write a native query. But hey, fucking Panache does not support that. There is no fucking place in their fucking docs stating anything about how to use native queries.
So, fuck you quarkus, fuck you panache, fuck you hibernate, fuck you overcomplicated limiting bullshit called full-fledged ORMs. I'm moving to a fucking mybatis and fuck it. It's simple as fuck, does not fucking restrict me in writing whatever shit query I want to write and let's me map the shit just fine.1 -
Funny to find out that IntelliJ has a Shortcut for getter and Setter after making a own Programm that writes those methods trough String building...
-
Learning C# coming from Java...
What's the fuss about properties? As i see it, theyre only usefull for binding, as else they just work as syntetic sugar instead of getter/setter methods.
But properties are also limited to give response back, like a successfull set, unless you start throwing exceptions..
And if a set property has if(age>5){this.age=age} then if i pass the property a 4, you will never know as a user that it failed (again, unless you start throwing exceptions)
Im kinda feeling like i want to use get/set methods until i need to bind, then of course use property ?? Am i all off here?25