Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
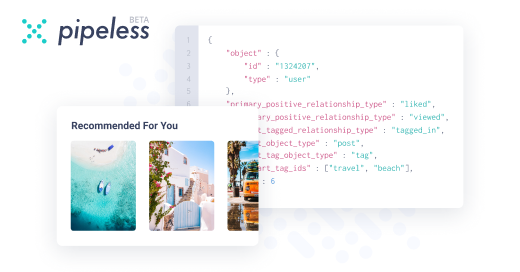
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "fast and secure"
-
Following a conversation with a fellow devRanter this came to my mind ago, happened a year or two ago I think.
Was searching for an online note taking app which also provided open source end to end encryption.
After searching for a while I found something that looked alright (do not remember the URL/site too badly). They used pretty good open source JS crypto libraries so it seemed very good!
Then I noticed that the site itself did NOT ran SSL (putting the https:// in front of the site name resulted in site not found or something similar).
Went to the Q/A section because that's really weird.
Saw the answer to that question:
"Since the notes are end to end encrypted client side anyways, we don't see the point in adding SSL. It's secure enough this way".
😵
I emailed them right away explaing that any party inbetween their server(s) and the browser could do anything with the request (includingt the cryptographic JS code) so they should start going onto SSL very very fast.
Too badly I never received a reply.
People, if you ever work with client side crypto, ALWAYS use SSL. Also with valid certs!
The NSA for example has this thing known as the 'Quantum Insert' attack which they can deploy worldwide which basically is an attack where they detect requests being made to servers and reply quickly with their own version of that code which is very probably backdoored.
This attack cannot be performed if you use SSL! (of course only if they don't have your private keys but lets assume that for now)
Luckily Fox-IT (formerly Dutch cyber security company) wrote a Snort (Intrustion Detection System) module for detecting this attack.
Anyways, Always use SSL if you do anything at all with crypto/sensitive data! Actually, always use it but at the very LEAST really do it when you process the mentioned above!31 -
POSTMORTEM
"4096 bit ~ 96 hours is what he said.
IDK why, but when he took the challenge, he posted that it'd take 36 hours"
As @cbsa wrote, and nitwhiz wrote "but the statement was that op's i3 did it in 11 hours. So there must be a result already, which can be verified?"
I added time because I was in the middle of a port involving ArbFloat so I could get arbitrary precision. I had a crude desmos graph doing projections on what I'd already factored in order to get an idea of how long it'd take to do larger
bit lengths
@p100sch speculated on the walked back time, and overstating the rig capabilities. Instead I spent a lot of time trying to get it 'just-so'.
Worse, because I had to resort to "Decimal" in python (and am currently experimenting with the same in Julia), both of which are immutable types, the GC was taking > 25% of the cpu time.
Performancewise, the numbers I cited in the actual thread, as of this time:
largest product factored was 32bit, 1855526741 * 2163967087, took 1116.111s in python.
Julia build used a slightly different method, & managed to factor a 27 bit number, 103147223 * 88789957 in 20.9s,
but this wasn't typical.
What surprised me was the variability. One bit length could take 100s or a couple thousand seconds even, and a product that was 1-2 bits longer could return a result in under a minute, sometimes in seconds.
This started cropping up, ironically, right after I posted the thread, whats a man to do?
So I started trying a bunch of things, some of which worked. Shameless as I am, I accepted the challenge. Things weren't perfect but it was going well enough. At that point I hadn't slept in 30~ hours so when I thought I had it I let it run and went to bed. 5 AM comes, I check the program. Still calculating, and way overshot. Fuuuuuuccc...
So here we are now and it's say to safe the worlds not gonna burn if I explain it seeing as it doesn't work, or at least only some of the time.
Others people, much smarter than me, mentioned it may be a means of finding more secure pairs, and maybe so, I'm not familiar enough to know.
For everyone that followed, commented, those who contributed, even the doubters who kept a sanity check on this without whom this would have been an even bigger embarassement, and the people with their pins and tactical dots, thanks.
So here it is.
A few assumptions first.
Assuming p = the product,
a = some prime,
b = another prime,
and r = a/b (where a is smaller than b)
w = 1/sqrt(p)
(also experimented with w = 1/sqrt(p)*2 but I kept overshooting my a very small margin)
x = a/p
y = b/p
1. for every two numbers, there is a ratio (r) that you can search for among the decimals, starting at 1.0, counting down. You can use this to find the original factors e.x. p*r=n, p/n=m (assuming the product has only two factors), instead of having to do a sieve.
2. You don't need the first number you find to be the precise value of a factor (we're doing floating point math), a large subset of decimal values for the value of a or b will naturally 'fall' into the value of a (or b) + some fractional number, which is lost. Some of you will object, "But if thats wrong, your result will be wrong!" but hear me out.
3. You round for the first factor 'found', and from there, you take the result and do p/a to get b. If 'a' is actually a factor of p, then mod(b, 1) == 0, and then naturally, a*b SHOULD equal p.
If not, you throw out both numbers, rinse and repeat.
Now I knew this this could be faster. Realized the finer the representation, the less important the fractional digits further right in the number were, it was just a matter of how much precision I could AFFORD to lose and still get an accurate result for r*p=a.
Fast forward, lot of experimentation, was hitting a lot of worst case time complexities, where the most significant digits had a bunch of zeroes in front of them so starting at 1.0 was a no go in many situations. Started looking and realized
I didn't NEED the ratio of a/b, I just needed the ratio of a to p.
Intuitively it made sense, but starting at 1.0 was blowing up the calculation time, and this made it so much worse.
I realized if I could start at r=1/sqrt(p) instead, and that because of certain properties, the fractional result of this, r, would ALWAYS be 1. close to one of the factors fractional value of n/p, and 2. it looked like it was guaranteed that r=1/sqrt(p) would ALWAYS be less than at least one of the primes, putting a bound on worst case.
The final result in executable pseudo code (python lol) looks something like the above variables plus
while w >= 0.0:
if (p / round(w*p)) % 1 == 0:
x = round(w*p)
y = p / round(w*p)
if x*y == p:
print("factors found!")
print(x)
print(y)
break
w = w + i
Still working but if anyone sees obvious problems I'd LOVE to hear about it.38 -
If programming languages had honest slogans, what would they be?
C: If you want a horse, make sure you feed it, clean it and secure it yourself. No warranties.
C++: If you want a horse, you need to buy a circus along with it.
Java: Before you buy a horse - buy a piece of land, build a house in that land, build a barn beside the house & if you are not bankrupt yet, buy the horse and then put the horse in the barn.
C#: You don’t want a horse, but Microsoft wants you to have a horse. Now it’s up to you if you want Microsoft or not.
Swift: Don’t buy an overpriced Unicorn if all you wanted was a horse.
JavaScript: If you want to buy a horse & confidently ride it, make sure you read a book named "You don't know horse".
PHP: After enough optimization, your horse can compete the top most horses in the world; but deep down, you'll always know it's an ass.
Hack: Let's face it, even if you take the ass from the ass lovers and give them back a horse in exchange, not many will ride it.
Ruby: If you want a horse, make sure you ride it on top of rail roads, even if the horse can't run fast on rails.
Python: Don't ride your horse and eat your sandwich on the same line, until you indent it on the next line.
Bash: Your horse may shit everywhere, but at least it gets the job done.
R: You are the horse. R will ride you.
Got this from Quora.
https://quora.com/If-programming-la...7 -
My first job was actually nontechnical - I was 18 years old and sold premium office furniture for a small store in Munich.
I did code in my free time though (PHP/JS mostly, had a litte browsergame back then - those were the days), so when my boss approached me and asked me whether I liked to take over a coding project, I agreed to the idea.
Little did I know at the time: I was supposed to work with a web agency the boss had contracted to build their online shop. Only that he had no plan or anything, he basically told them "build me an online shop like abc(a major competitor of ours at the time)"
He employed another sales lady who was supposed to manage the shop (that didn't exist yet). In the end, I think 80% of her job was to keep me from killing my boss.
As you can imagine, with this huuuuge amout of planning and these exact visions of what was supposed to be, things went south fast and far. So far that I could visit my fellow flightless birds down in the Penguin's republic of Antarctica and still need to go further.
Well... When my boss started suing the web agency, I was... ahem, asked to take over. Dumb as I was, I did - I was a PHP kid and thought that Magento, being written in PHP, would be easy to master. If you know Magento, you know that was maybe the wrongest thing I ever said.
Fast forward 3 very exhausting months, the thing was online. Not all of it worked yet, but it was online and fairly secure.
I did next to everything myself, administrating the CentOS box the shop was running on, its (own) e-mail server, the web server, all the coding required for the shop (can you spell 12 hour day for 8 hour pay?)
3 further months later, my life basically was a wreck, I dragged myself to work, the only thing I looked forward being the motorcycle ride home. The system worked though.
Mind you, I was still, at the time, working with three major customers, doing deskside support and some admin (Win Server 2008R2 at the time) - because, to quote my boss, "We could not afford a full time developer and we don't need one".
I think i stopped coding in my free time, the one hobby I used to love more than anything on the world, somewhere Decemerish 2012. I dropped out of the open source projects I was in, quit working on my browser game and let everything slide.
I didn't even care to renew the domains and servers for it, I just let it die without notice.
The little free time I had, I spent playing video games and getting drunk/high.
December 2013, 1.5 years on the job, I reached my breaking point and just left, called in sick at least a week per month because I just could not see this fucking place anymore.
I looked for another job outside of ALL of what I did before. No more Magento, no more sales, no more PHP. I didn't have to look for long, despite what I thought of my skills.
In February 2014, I told my boss that I quit. It was still seven months until my new job started, but I wanted him to know early so we could migrate and find a replacement.
The search for said replacement started in June 2014. I had considerably less work in the months before, looks like he got the hint.
In August 2014, my replacement arrived and I got him started.
I found a job, which I am still in, and still happy about after almost half a decade, at a local, medium sized ISP as a software dev and IT security guy. Got a proper training with a certificate and everything now.
My replacement lasted two months, he was external and never really did his job - the site, which until I had quit, had a total of 3 days downtime for 3 YEARS (they were the hoster's fault, not mine), was down for an entire month and he could not even tell why.
HIS followup was kicked after taking two weeks to familiarize himself with the project. Well, I think that two weeks is not even barely enough to familiarize yourself with nearly three years of work, but my boss gave him two days.
In 2016, the shop was replaced with another one. Different shop system, different OS, different CI. I don't know why and I can't say I give a damn.
Almost all the people that worked at the company back with me have left for greener pastures, taking their customers (and revenue) with them.
As for my boss' comments, instructions and lines: THAT might not be safe for work. Or kids. Or humans in general. And there wouldn't be much left if you put it through a language filter...
Moral of the story: No, it's not a bad thing to leave a place if you're mistreated there. Don't mistake loyalty with stupidity!
And, to quote one of my favourite Bands: "Nothing matters when the pain is all but gone" (Tragedy + Time by Rise Against).8 -
Im getting a bit tired of programming.
I have been struggling for years regarding programming. I did have some moments of perceived success, but most of the time it has been depressing.
I’m not sure if I dislike programming. But there are some aspects of it that make me feel not as passionate about it.
First of, programs are invisible. No one sees your program or you (assuming we’re talking about a non artistic dev job).
People can’t see lines of code executing, but even if they did it would be gibberish to them.
Users can only become aware of bad software and that kind of breaks my heart a bit.
You could write fast, stable, secure, easy to read, easy to update software. People won’t notice. Hell, even your boss/coworkers might not notice.
In fact, sometimes you try to do the good thing, you try to become a better dev, you try to write tests first, you try to i18n, and what do you get? “Uhh, that’s taking too much time and I don’t see the benefit”.
I know some people will say that people noticing bad service happens on every job.
But programming is the ultimate isolation job. No client has ever told me “hey that code you wrote was pretty good”. They can’t even read code.
I don’t know the users, the users don’t know me, and the users can only judge my program by the result, they can only judge the visual interface.
Let’s say you write a cool project at github. The code is great. Guess what, every language’s ecosystem out there is saturated. Everything is already written. GitHub is saturated. Your best project ends up being a just for yourself enjoyment.
I’m not saying you shouldn’t enjoy code for yourself. That’s how I bet most prolific coders start. I’ve been doing that for many years now. But at some point you want to be part of something with humans.
Imagine I’m stranded on an island with nothing no humans, just food, water and a computer. Would I write code just for myself, just for fun? I think I would off myself 3 months in.
Maybe I should do develop a more social talent...14 -
why do i have an iphone?
well, let's start with the cons of android.
- its less secure. this isn't even arguable. it took the fbi a month or something (i forget) to break into an ios device
- permission, permissions, permissions. many of the android apps i use ask for the not obscure permissions.
· no, you don't need access to my contacts
· no, you don't need access to my camera to take notes
· no, you don't need access to my microphone to send messages
· no, you don't need access to my saved passwords to be a functioning calculator
- not being able to block some apps from an internet connection
- using an operating system created and maintained by an advertising company, aka no more privacy
- i like ios's cupertino more than material design, but that's just personal preference
pros of ios:
- being able to use imessage, at my school if you don't have an iphone you're just not allowed to be in the group chat
- the reliability. i've yet a data loss issue
- the design and feel. it just feels premium
- if i could afford it, ios seems like a lot of fun to develop for (running a hackintosh vm compiled a flutter app 2x as fast as it did on not-a-vm windows)
so that's why i like iphones
google sucks55 -
In my school, eleventh grade (so nearly "Abitur", A levels), we got the task to create a program which will be running on every computer here which should replace the Classbook (like a book where homework and lessons and stuff is written down).
Now, the class before mine already did a part of that, a program to share who is ill/not at school, with a mark whether it is excused or not.
So far so good. They all seemed not that bad when they were presenting it to us. Then, the first thing: they didn't know what git is. Well, okay I thought.
Next, there was this password field to access the program. One of them entered the password and clicked enter. That seemed suspiciously fast for an actual secure login. So fast, the password could have been in the Code...
Yesterday I copied that program and put it into a decompiler.
And... I was right.
There were the login credentials in plain text. Also, haven't thought of it but, IP address + username + password + database name were there in plain text, too.
Guess I am going to rewrite this program down to the core2 -
I need some advice here... This will be a long one, please bear with me.
First, some background:
I'm a senior level developer working in a company that primarily doesn't produce software like most fast paced companies. Lots of legacy code, old processes, etc. It's very slow and bureaucratic to say the least, and much of the management and lead engineering talent subscribes to the very old school way of managing projects (commit up front, fixed budget, deliver or else...), but they let us use agile to run our team, so long as we meet our commitments (!!). We are also largely populated by people who aren't really software engineers but who do software work, so being one myself I'm actually a fish out of water... Our lead engineer is one of these people who doesn't understand software engineering and is very types when it comes to managing a project.
That being said, we have this project we've been working for a while and we've been churning on it for the better part of two years - with multiple changes in mediocre contribution to development along the way (mainly due to development talent being hard to secure from other projects). The application hasn't really been given the chance to have its core architecture developed to be really robust and elegant, in favor of "just making things work" in order to satisfy fake deliverables to give the customer.
This has led us to have to settle for a rickety architecture and sloppy technical debt that we can't take the time to properly fix because it doesn't (in the mind of the lead engineer - who isn't a software engineer mind you) deliver visible value. He's constantly changing his mind on what he wants to see working and functional, he zones out during sprint planning, tries to work stories not on the sprint backlog on the side, and doesn't let our product owner do her job. He's holding us to commitments we made in January and he's not listening when the team says we don't think we can deliver on what's left by the end of the year. He thinks it's reasonable to expect us to deliver and he's brushing us off.
We have a functional product now, but it's not very useful yet and still has some usability issues. It's still missing features, which we're being put under pressure to get implemented (even half-assed) by the end of the year.
TL;DR
Should I stand up for what I know is the right way to write software and push for something more stable sometime next year or settle for a "patch job" that we *might* deliver that will most definitely be buggy and be harder to maintain going forward? I feel like I'm fighting an uphill battle in trying to write good quality code in lieu of faster results and I just can't get behind settling for crap just because.9 -
yet another Microsoft bashing rant...
I'm trying to get `Visual Studio`
You use your Windows 10 VM, use Edge, use Bing and search for `Visual Studio`.
First fucking result:
A Visual Studio alternative - A powerful C & C++ IDE - CLion
-- from jetbrains.com
Like... WTF, you not even promoting your' own stuff ?
But then for when you search 'firefox' w/ bing+edge a thick fat banner: 'Promoted by Microsoft': There's no need to download a new web browser.\n MS recommends Edge for fast ...6 -
Dev industry develop so fast. This is because information available anywhere in the internet and people try to learn any programming language they want . But only few know whether they following secure coding practice or not
But the thing is most of Dev people dosent care about security. They focus just to develop a application but not to secure it?2 -
Got one right now, no idea if it’s the “most” unrealistic, because I’ve been doing this for a while now.
Until recently, I was rewriting a very old, very brittle legacy codebase - we’re talking garbage code from two generations of complete dumbfucks, and hands down the most awful codebase I’ve ever seen. The code itself is quite difficult to describe without seeing it for yourself, but it was written over a period of about a decade by a certifiably insane person, and then maintained and arguably made much worse by a try-hard moron whose only success was making things exponentially harder for his successor to comprehend and maintain. No documentation whatsoever either. One small example of just how fucking stupid these guys were - every function is wrapped in a try catch with an empty catch, variables are declared and redeclared ten times, but never used. Hard coded credentials, hard coded widths and sizes, weird shit like the entire application 500ing if you move a button to another part of the page, or change its width by a pixel, unsanitized inputs, you name it, if it’s a textbook fuck up, it’s in there, and then some.
Because the code is so damn old as well (MySQL 8.0, C#4, and ASP.NET 3), and utterly eschews the vaguest tenets of structured, organized programming - I decided after a month of a disproportionate effort:success ratio, to just extract the SQL queries, sanitize them, and create a new back end and front end that would jointly get things where they need to be, and most importantly, make the application secure, stable, and maintainable. I’m the only developer, but one of the senior employees wrote most of the SQL queries, so I asked for his help in extracting them, to save time. He basically refused, and then told me to make my peace with God if I missed that deadline. Very helpful.
I was making really good time on it too, nearly complete after 60 days of working on it, along with supporting and maintaining the dumpster fire that is the legacy application. Suddenly my phone rings, and I’m told that management wants me to implement a payment processing feature on the site, and because I’ve been so effective at fixing problems thus far, they want to see it inside of a week. I am surprised, because I’ve been regularly communicating my progress and immediate focus to management, so I explain that I might be able to ship the feature by end of Q1, because rather than shoehorn the processor onto the decrepit piece of shit legacy app, it would be far better to just include it in the replacement. I add that PCI compliance is another matter that we must account for, and so there’s not a great chance of shipping this in a week. They tell me that I have a month to do it…and then the Marketing person asks to see my progress and ends up bitching about everything, despite the front end being a pixel perfect reproduction. Despite my making everything mobile responsive, iframe free, secure and encrypted, fast, and void of unpredictable behaviors. I tell her that this is what I was asked to do, and that there should have been no surprises at all, especially since I’ve been sending out weekly updates via email. I guess it needed more suck? But either way, fuck me and my two months of hard work. I mean really, no ego, I made a true enterprise grade app for them.
Short version, I stopped working on the rebuild, and I’m nearly done writing the payment processor as a microservice that I’ll just embed as an iframe, since the legacy build is full of those anyway, and I’m being asked to make bricks without straw. I’m probably glossing over a lot of finer points here too, just because it’s been such an epic of disappointment. The deadline is coming up, and I’m definitely going to make it, now that I have accordingly reduced the scope of work, but this whole thing has just totally pissed me off, and left a bad taste about the organization.10 -
Im having a sort of dilema. I recently started taking freelance work for web developement (and design ack) and Im uncomfortable with the state of the industry. Ill explain: Say if I bid a client for a simple 1-3 page site w contact form (a new page, not migration) My suggestion is to use djangocms, django, or just static html/css/js (ie bootstrap), which produces clean, fairly secure, and fast sites. Of course I can throw a templated unoriginal wordpress site together in a few hours 2 days latest, so I offer that option as a sidenote on the bid, charging almost 2x more. For some reason I dont understand they choose the wp shitshow. I explain all the reasons that not the way to go( which I wont list, if u dont know, u never used it. google up) but they dont care abt the details, they rather pay more for shit job. OFC I reluctantly deliver what they want, but as a result my portfolio is full of unoriginal shit Im not happy showing off. I have a few sites Ive done on the side my prefered way, but they not deployed and sit in my github for all intents n purposes unviewable to potential clients.
I want to be proud of my portfolio, and it to be a representation of what Im capable of. BUT, I gotta eat, and work is better than no work.
There are so many "wordpress designers" oversaturaring the field and it lowering the overall standard of what we are capable of. I just begining my dev journey, but if I cant have a body of work Im proud of, theres no way I can see doing this the rest of my life, and that makes me really sad. My love of developing, coding, and IT/computers in general drove me to change careers from audio engineering to web development, and the fact that this fucking mr. potatoe head of a CMS is slowly turning that love into hate really pisses me off. So Im ending this !rant looking for hope.
Your thoughts?1 -
I spent 4 months in a programming mentorship offered by my workplace to get back to programming after 4 years I graduated with a CS degree.
Back in 2014, what I studied in my first programming class was not easy to digest. I would just try enough to pass the courses because I was more interested in the theory. It followed until I graduated because I never actually wrote code for myself for example I wrote a lot of code for my vision class but never took a personal initiative. I did however have a very strong grip on advanced computer science concepts in areas such as computer architecture, systems programming and computer vision. I have an excellent understanding of machine learning and deep learning. I also spent time working with embedded systems and volunteering at a makerspace, teaching Arduino and RPi stuff. I used to teach people older than me.
My first job as a programmer sucked big time. It was a bootstrapped startup whose founder was making big claims to secure funding. I had no direction, mentorship and leadership to validate my programming practices. I burnt out in just 2 months. It was horrible. I experienced the worst physical and emotional pain to date. Additionally, I was gaslighted and told that it is me who is bad at my job not the people working with me. I thought I was a big failure and that I wasn't cut out for software engineering.
I spent the next 6 months recovering from the burn out. I had a condition where the stress and anxiety would cause my neck to deform and some vertebrae were damaged. Nobody could figure out why this was happening. I did find a neurophyscian who helped me out of the mental hell hole I was in and I started making recovery. I had to take a mild anti anxiety for the next 3 years until I went to my current doctor.
I worked as an implementation engineer at a local startup run by a very old engineer. He taught me how to work and carry myself professionally while I learnt very little technically. A year into my job, seeing no growth technically, I decided to make a switch to my favourite local software consultancy. I got the job 4 months prior to my father's death. I joined the company as an implementation analyst and needed some technical experience. It was right up my alley. My parents who saw me at my lowest, struggling with genetic depression and anxiety for the last 6 years, were finally relieved. It was hard for them as I am the only son.
After my father passed away, I was told by his colleagues that he was very happy with me and my sisters. He died a day before I became permanent and landed a huge client. The only regret I have is not driving fast enough to the hospital the night he passed away. Last year, I started seeing a new doctor in hopes of getting rid of the one medicine that I was taking. To my surprise, he saw major problems and prescribed me new medication.
I finally got a diagnosis for my condition after 8 years of struggle. The new doctor told me a few months back that I have Recurrent Depressive Disorder. The most likely cause is my genetics from my father's side as my father recovered from Schizophrenia when I was little. And, now it's been 5 months on the new medication. I can finally relax knowing my condition and work on it with professional help.
After working at my current role for 1 and a half years, my teamlead and HR offered me a 2 month mentorship opportunity to learn programming from scratch in Python and Scrapy from a personal mentor specially assigned to me. I am still in my management focused role but will be spending 4 hours daily of for the mentorship. I feel extremely lucky and grateful for the opportunity. It felt unworldly when I pushed my code to a PR for the very first time and got feedback on it. It is incomparable to anything.
So we had Eid holidays a few months back and because I am not that social, I began going through cs61a from Berkeley and logged into HackerRank after 5 years. The medicines help but I constantly feel this feeling that I am not enough or that I am an imposter even though I was and am always considered a brilliant and intellectual mind by my professors and people around me. I just can't shake the feeling.
Anyway, so now, I have successfully completed 2 months worth of backend training in Django with another awesome mentor at work. I am in absolute love with Django and Python. And, I constantly feel like discussing and sharing about my progress with people. So, if you are still reading, thank you for staying with me.
TLDR: Smart enough for high level computer science concepts in college, did well in theory but never really wrote code without help. Struggled with clinical depression for the past 8 years. Father passed away one day before being permanent at my dream software consultancy and being assigned one of the biggest consultancy. Getting back to programming after 4 years with the help of change in medicine, a formal diagnosis and a technical mentorship.3 -
Why in the FUCK does the NSLSC (company that lends out student loans) have as their ONLY method to update your banking information a .pdf that you print out online and physically mail in. Once they receive it–will take another 1-2 weeks (according to them) to update my banking information.
It's fucking 2019, every single service I've used to update any kind of information (from gym payments to government related information) can be done online through a secure, streamlined, fast, environmentally friendly and cost effective ONLINE FORM.2 -
Several years ago I spent over two months working out how to integrate Text To Speech and Speech To Text (TTS/STT) into any windows program I wrote in Delphi, originally for a powerful flat-file search engine. Does anyone know if TTS/STT is useful on windows 10+ or have any use?
I was thinking about redeveloping the search engine into a stand alone program which can be used as a fast and light query tool with trigger functions, it can be made into a "reply bot" or used with a server like Apache, but without the old IBM mainframe mentality being readopted as "AI" and "social media" everywhere today. low-level Independent and secure droid like systems sound more fun to develop. -
So... there is a bank. And the website for example is using "https". Alright. But the Login consists your login ID (in the most cases your account number) and a Pin number ( only 5 chars) If i remember pentesting, crunch etc a pin or password with 5 chars (included special characters) is fast hackable or not? Or is it super secure cuz of the "https"?4
-
need a random number
AI says just use system time and modulus it. I'm wondering if I can get performance down lower cuz I'm doing this maybe like thousands of times a second (im too lazy to do the math rn)
found a crate called fastrand. they're all like this isn't secure for cryptography and yada yada. peak inside curious how they do it. not too sure, seems like they have a predetermined hash and they do some bitwise or something. kind of a lot to read so I don't wanna. either case seems like they're not using system time
make a test to benchmark, 10k rounds how fast is it?
430 nano seconds for system time
460 nano second for fastrand
lol
all that typing and you end up slower than system time. I'm assuming system time can be guessed as well but what's the point of fastrand if it's slower 🤔
I mean maybe on some OS systems looking up the system time might be slower? no clue15