Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
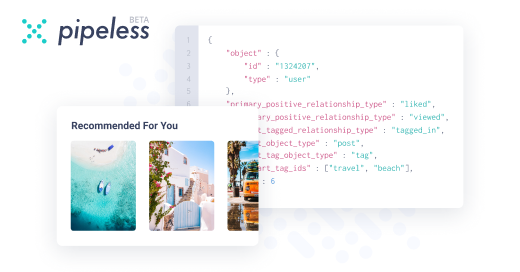
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "stdio.h"
-
How my C programs may as well be written:
#include<stdio.h>
int main() {
printf("Segmentation fault\n");
return 0;
}6 -
My first code :-
#include<stdio.h>
void main()
{ printf("Hello Divya"); }
Output :- I have a boyfriend...1 -
My first code:-
#include<stdio.h>
void main() {
printf("Hello Priya");
}
Output:- I have a boyfriend!9 -
Got bored and opened /usr/include/stdio.h to see if I can learn something from it.
But what I found... I now cannot unsee...
This whole time... it was all an illusion...
Life will never be the same again.4 -
I once had a class mate who argued that coding in C not only produced faster code than .NET C#, but that he could actually produce applications faster than me in C.
I challenged him to make a Web browser. While he was struggling to remember if it was #include <stdio.h> or #include <iostream>, I started typing WebBro... and let IntelliSense work it's magic.
Needless to say I won.
Sadly, he wouldn't admit his defeat but went on about how much faster his browser would run in the end...
He has yet to release a Web browser written completely in C.15 -
Oh man where to start:
Not wanting to use LINQ because he did not wanted to "download external dependencies"
Not wanting to use prepared statements on their php sql code, and refusing to use pdo because they will "always use mysql"(moved to postgreSQL shortly after I left)
For some reason including a php file that only had ?>......thats it....only ?>
Use c++ but refused to learn oop and use structs for everything, importing stdio.h and printf everything.....like really?
Maybe just nitpicking, but refusing to use the recyclerview pattern on am android app. The implementation was faster after I made the change.
Importing a library for promises instead of using the ones already in the language(JS)
Changing the style of aaaaall p tags instead of using classes as well as refusing to use divs in place of p tags...well...fuck
Not indent his ASP classic code
Use notepad on his asp classic code
Use ASP Classic in 2017, even for new projects6 -
A C++ question. Correct answer will get you a virtual thug glasses & a cigar if you're into that , and upvotes (:
#include <stdio.h>
int main(void) {
int i = 4;
int* p = &i;
i = 8;
printf("i divided by *p is: %d\n", i/*p);
return 0;
}
What is the result of running this code?18 -
As an exercise lets see how many different ways we can wish devRant Happy Birthday in code. Try not to copy peoples examples, use a different language or different method.
A couple of examples to start the process:
* LOLCODE *
HAI 1.3
LOL VAR R 3
IM IN YR LOOP
VISIBLE "Happy Birthday"!
IZ VAR LIEK 1?
YARLY
VISIBLE "Dear devRant"!
NOWAI
VISIBLE "to you"!
KTHX
NERFZ VAR!!
IZ VAR LIEK 0?
GTFO
KTHX
KTHX
KTHXBYE
* C *
#include <stdio.h>
#define HP "Happy birthday"
#define TY "to you"
#define DD "Dear devRant"
typedef struct HB_t { const char *s; const char *e;} HB;
static const HB hb[] = {{HP,TY}, {{HP,TY}, {{HP,DD}, {{HP,TY}, { NULL, NULL }};
int main(void)
{
const HB *s = hb;
while(s->start) { printf("%s %s", s->s, s->e); }
return 1;
}12 -
How to write a proper Hello World program in Java:
public class ProperJavaProgram {
public static void main(String[] args) {
try {
// Write the hello world file
List<String> lines = Arrays.asList(
"#include <stdio.h>",
"int main() {",
"printf(\"Hello World!\\n\");",
"return 0;",
"}"
);
Path file = Paths.get("awesome-program.c");
Files.write(file, lines, Charset.forName("UTF-8"));
// Execute the file
executeCommand("cc awesome-c-program.c -o awesome-executable");
executeCommand("./awesome-executable");
} catch (IOException e) {
System.out.println("You're screwed, just use Java and get over it. " + e);
}
}
public static void executeCommand(String command) {
try {
Process p = Runtime.getRuntime().exec(command); // Run the process
BufferedReader stdInput = new BufferedReader(new InputStreamReader(p.getInputStream())); // Get the output
String s; // Print out the output
while ((s = stdInput.readLine()) != null) {
System.out.println(s);
}
} catch (IOException e) {
System.out.println("You're screwed, just use Java and get over it. " + e); // UR SCREWED
}
}
}2 -
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <time.h>
#include <sys/time.h>
void setDate(const char* dataStr) // format like MMDDYY
{
char buf[3] = {0};
strncpy(buf, dataStr + 0, 2);
unsigned short month = atoi(buf);
strncpy(buf, dataStr + 2, 2);
unsigned short day = atoi(buf);
strncpy(buf, dataStr + 4, 2);
unsigned short year = atoi(buf);
time_t mytime = time(0);
struct tm* tm_ptr = localtime(&mytime);
if (tm_ptr)
{
tm_ptr->tm_mon = month - 1;
tm_ptr->tm_mday = day;
tm_ptr->tm_year = year + (2000 - 1900);
const struct timeval tv = {mktime(tm_ptr), 0};
settimeofday(&tv, 0);
}
}
int main(int argc, char** argv)
{
if (argc < 1)
{
printf("enter a date using the format MMDDYY\n");
return 1;
}
setDate(argv[1]);
return 0;
}7 -
My friend sent me this as WYSIWYG
/* A simple quine (self-printing program), in standard C. */ /* Note: in designing this quine, we have tried to make the code clear * and readable, not concise and obscure as many quines are, so that * the general principle can be made clear at the expense of length. * In a nutshell: use the same data structure (called "progdata" * below) to output the program code (which it represents) and its own * textual representation. */ #include <stdio.h> void quote(const char *s) /* This function takes a character string s and prints the * textual representation of s as it might appear formatted * in C code. */ { int i; printf(" \""); for (i=0; s[i]; ++i) { /* Certain characters are quoted. */ if (s[i] == '\\') printf("\\\\"); else if (s[i] == '"') printf("\\\""); else if (s[i] == '\n') printf("\\n"); /* Others are just printed as such. */ else printf("%c", s[i]); /* Also insert occasional line breaks. */ if (i % 48 == 47) printf("\"\n \""); } printf("\""); } /* What follows is a string representation of the program code, * from beginning to end (formatted as per the quote() function * above), except that the string _itself_ is coded as two * consecutive '@' characters. */ const char progdata[] = "/* A simple quine (self-printing program), in st" "andard C. */\n\n/* Note: in designing this quine, " "we have tried to make the code clear\n * and read" "able, not concise and obscure as many quines are" ", so that\n * the general principle can be made c" "lear at the expense of length.\n * In a nutshell:" " use the same data structure (called \"progdata\"\n" " * below) to output the program code (which it r" "epresents) and its own\n * textual representation" ". */\n\n#include <stdio.h>\n\nvoid quote(const char " "*s)\n /* This function takes a character stri" "ng s and prints the\n * textual representati" "on of s as it might appear formatted\n * in " "C code. */\n{\n int i;\n\n printf(\" \\\"\");\n " " for (i=0; s[i]; ++i) {\n /* Certain cha" "racters are quoted. */\n if (s[i] == '\\\\')" "\n printf(\"\\\\\\\\\");\n else if (s[" "i] == '\"')\n printf(\"\\\\\\\"\");\n e" "lse if (s[i] == '\\n')\n printf(\"\\\\n\");" "\n /* Others are just printed as such. */\n" " else\n printf(\"%c\", s[i]);\n " " /* Also insert occasional line breaks. */\n " " if (i % 48 == 47)\n printf(\"\\\"\\" "n \\\"\");\n }\n printf(\"\\\"\");\n}\n\n/* What fo" "llows is a string representation of the program " "code,\n * from beginning to end (formatted as per" " the quote() function\n * above), except that the" " string _itself_ is coded as two\n * consecutive " "'@' characters. */\nconst char progdata[] =\n@@;\n\n" "int main(void)\n /* The program itself... */\n" "{\n int i;\n\n /* Print the program code, cha" "racter by character. */\n for (i=0; progdata[i" "]; ++i) {\n if (progdata[i] == '@' && prog" "data[i+1] == '@')\n /* We encounter tw" "o '@' signs, so we must print the quoted\n " " * form of the program code. */\n {\n " " quote(progdata); /* Quote all. */\n" " i++; /* Skip second '" "@'. */\n } else\n printf(\"%c\", p" "rogdata[i]); /* Print character. */\n }\n r" "eturn 0;\n}\n"; int main(void) /* The program itself... */ { int i; /* Print the program code, character by character. */ for (i=0; progdata[i]; ++i) { if (progdata[i] == '@' && progdata[i+1] == '@') /* We encounter two '@' signs, so we must print the quoted * form of the program code. */ { quote(progdata); /* Quote all. */ i++; /* Skip second '@'. */ } else printf("%c", progdata[i]); /* Print character. */ } return 0; }6 -
Things I do when I'm coding in C
Laugh like a stupid 10 year old at STD in stdio.h (Sexually Transmitted Diseased input output)
Keep trying to type studio.h instead of stdio.h1 -
#include <stdio.h>
int main()
{
printf("Come at me. I give opening curly brackets their own line, use Dvorak and use tabs!")
}5 -
Visual Studio and its compatibility with Linux applications.
I don't know if I'm the only one, but this is my setup:
- Visual Studio 2017 on Windows 10
- Ubuntu 18.04 subsystem on Windows
I just can't do any Linux coding in Visual Studio... it is using my subsystem as a Remote Compiler and debugger, and a simple Hello World program does build and run successfully, but EVERY SINGLE LINE HAS ERRORS! It can't find stdio.h! Not a single include file works! They get auto-completed so it knows where the files are, but apparently opening them to see all the methods is too much for Visual Studio! I'd say the problem has something to do with IntelliSense since only inside the IDE my code has errors, compiling (which happens on the subsystem) works like a charm.2 -
Some compilers give an error message on forgotten type casting. From that it shows good typing style casting. So you also avoid clerical errors that can lead to the program crash in the worst case. With some types it is also necessary to perform type casting comma on others Types, however, do this automatically for the compiler.
In short:Type casting is used to prevent mistakes.
An example of such an error would be:
#include <stdio.h>
#include <stdlib.h>
int main ()
{
int * ptr = malloc (10*sizeof (int))+1;
free(ptr-1);
return 0;
}
By default, one tries to access the second element of the requested memory. However, this is not possible, since pointer calculation (+,-) does not work for a void pointer.
The improved example would be:
int * ptr = ((int *) malloc (10*sizeof (int)))+1;
Here, typecasting is done beforehand and this turns the void pointer into its int pointer and pointer calculation can be applied. Note: If instead of error "no output" is displayed on the sololearn C compiler try another compiler.1 -
So a couple of months ago I had some stability issues which seems to have caused Baloo go crazy and create an 1.7 exabyte index file. It was apparently mainly empty as zfs compressed it down to 535MB
Today I spent some time trying to reproduce the "issue" and turns out that wasn't that hard.
So this little program running on FreeBSD with a compressed (lz4) zfs dataset creates an 1.9 Exabyte large file, nicely compressed down to 45KB :)
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
#include <sys/limits.h>
int main(int argc, char** argv) {
int fd = open("bigfile.lge", O_RDWR|O_CREAT, 0644);
for (int i = 0 ; i < 1000000000; i++) {
lseek(fd, INT_MAX, SEEK_CUR);
}
write(fd, " ",1);
close(fd);
}3 -
On Windows, which one line input will get this code to print "Finally I get a sticker. Yayyyy!!!" immediately
#include <stdio.h>
int main()
{
char *c = (char*) malloc(sizeof(char) * 10);
int rants = 0;
while(rants<20)
{
printf("U don't want me to get a sticker?\n");
scanf("%s", c);
if(c[0] == 'y')
rants--;
else
rants++;
}
printf("Finally I get a sticker. Yayyyy!!!\n");
} -
#include <stdio.h>
/*
* Windows Update Algorithm
*/
int main()
{
int percent = 1;
while (percent <= 100) {
printf("Working on updates\n");
printf("%i %% complete\n", percent);
printf("Don't turn off your computer\n\n");
if (percent == 30) {
printf("Restarting\n");
break;
}
percent++;
}
return 0;
} -
Hi everyone, I have such a task: “Given an integer square matrix. Determine the minimum among the sums of diagonal elements parallel to the main diagonal of the matrix.” I have a code but I have problems compiling a flowchart for it, can you help me with compiling a flowchart or give tips? thanks in advance!
Thats my code:
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define N_MIN -3
#define N_MAX 5
int main(int argc, char *argv[]){
int s,i,j,k,l,s1,t2,t1;
int a[5][5];
srand(time(NULL));
for(i=0;i<5;i++){
for(j=0;j<5;j++){
a[i][j]=rand()%(N_MAX-N_MIN+1)+N_MIN;
}
}
for(i=0;i<5;i++){
for(j=0;j<5;j++){
printf("%3d ",a[i][j]);
}
printf("\n");
}
k=0;
s=0;
l=0;
for (i=0; i<5; i++){
for (j=0; j<5; j++){
if (a[i][j]>=0){
if(a[i][j]%2==0)
l+=a[i][j];
k++;
}
}
if (k==5){
l=l;
}
else {
l=0;
}
s=s+l;
k=0;
}
s1=a[0][5-1];
for(i=1; i<5; i++){
t1=t2=0;
for(j=0; j<5-i; j++){
t1+=a[i+j][j];
t2+=a[j][i+j];
}
if(t1<s1) s1=t1;
if(t2<s1) s1=t2;
}
printf("vivod %d %d\n", s,s1);
return 0;
}2 -
My question is"which value of 'a' and 'b' will give me output '1' not '0'?
C language's code is below here
#include<stdio.h>
int main()
{ float a=1.2,b=10.7;
printf("output is = %f",a&&b);
Return 0; }16