Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
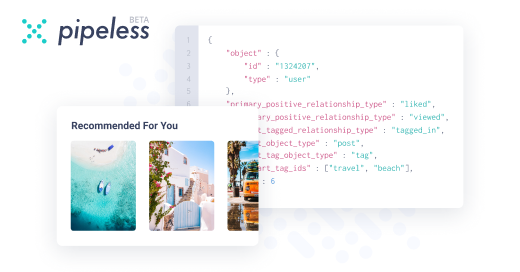
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "static types"
-
Recipe for a Great Programmer:
Ingredients:
-Books for a computer science curriculum from a top university
-Computer
-Headphones
-Internet
-Stress ball
-Pillow
-Lighter fluid
-Food
Directions:
1. Cover computer science books with lighter fluid
2. Light books on fire
3. Use flames to cook an energy-rich meal for the thousands of hours ahead
4. Pick an IDE
5. Choose a project beyond current capabilities. Good ways to push boundaries:
- Unfamiliar domain (e.g. large scale data processing, UI programming, high performance computing, games)
- Exotic programming language
- Larger in scope than any project before
6. Shut up about your IDE
7. Attempt to build
8. Stop procrastinating on Hacker News
9. Re-attempt to build
10. Squeeze stress ball and scream into pillow as necessary to keep sanity
When stuck:
- Paste stack traces into Google
- Find appropriate mailing list to get guidance
- Realize that real learning happens when you are stuck, uncomfortable, and/or frustrated
- Seek out books, classes, or other resources AFTER you have a good understanding of your deficiencies
11. Repeat #4 to #10 for at least 10 years
12. Results guaranteed! (to the same extent static types guarantee bug-free programs)
source: nathanmarz.com4 -
IF LIVES DEPEND ON A SYSTEM
1. Code review, collaboration, and knowledge sharing (each hour of code review saves 33 hours of maintenance)
2. TDD (40% — 80% reduction in production bug density)
3. Daily continuous integration (large code merges are a major source of bugs)
4. Minimize developer interruptions (an interrupted task takes twice as long and contains twice as many defects)
5. Linting (catches many typo and undefined variable bugs that static types could catch, as well as a host of stylistic issues that correlate with bug creation, such as accidentally assigning when you meant to compare)
6. Reduce complexity & improve modularity -- complex code is harder to understand, test, and maintain
-Eric Elliott12 -
Dynamically typed languages are barbaric to me.
It's pretty much universally understood that programmers program with types in mind (if you have a method that takes a name, it's a string. You don't want a name that's an integer).
Even it you don't like the verbosity of type annotations, that's fine. It adds maybe seconds of time to type, which is neglible in my opinion, but it's a discussion to be had.
If that's the case, use Crystal. It's statically typed, and no type annotations are required (it looks nearly identical to Ruby).
So many errors are fixed by static typing and compilers. I know a person who migrated most of the Python std library to Haskell and found typing errors in it. *In their standard library*. If the developers of Python can't be trusted to avoid simple typing errors with all their unit tests, how can anyone?
Plus, even if unit testing universally guarded against typing errors, why would you prefer that? It takes far less time to add a type annotation (and even less time to write nothing in Crystal), and you get the benefit of knowing types at compile time.
I've had some super weird type experiences in Ruby. You can mock out the return of the type check to be what you want. I've been unit testing in Ruby before, tried mocking a method on a type, didn't work as I expected. Checked the type, it lines up.
Turns out, nested away in some obscure place was a factory that was generating types and masking them as different types because we figured "since it responds to all the same methods, it's practically the same type right?", but not in the unit test. Took 45 minutes on my time when it could've taken ~0 seconds in a statically typed language.11 -
Extensible event system design in c++ - but also includes built-in static types for uniformity and performance.
Happy with it!13 -
Working on an Android app for a client who has a dev team that is developing a web app in with ember js / rails. These folks are "in charge" of the endpoints our app needs to function. Now as a native developer, I'm not a hater of a web apps way of doing things but with this particular app their dev teams seems to think that all programming languages can parse json as dynamically as javascript...
Exhibit A:
- Sample Endpoint Documentation
* GetImportantInfo
* Params: $id // id of info to get details of
* Endpoint: get-info/$id
* Method: GET
* Entity Return {SampleInfoModel}
- Example API calls in desktop REST client
* get-info/1
- response
{
"a" : 0,
"b" : false,
"c" : null
}
* get-info/2
- response
{
"a" : [null, "random date stamp"],
"b" : 3.14,
"c" : {
"z" : false,
"y" : 0.5
}
}
* get-info/3
- response
{
"a" : "false" // yes as a string
"b" : "yellow"
"c" : 1.75
}
Look, I get that js and ruby have dynamic types and a string can become a float can become a Boolean can become a cat can become an anvil. But that mess is very difficult to parse and make sense of in a stack that relies on static types.
After writing a million switch statements with cases like "is Float" or "is String" from kotlin's Any type // alias for java.Object, I throw my hands in the air and tell my boss we need to get on the phone with these folks. He agrees and we schedules a day that their main developer can come to our shop to "show us the ropes".
So the day comes and this guy shows up with his mac book pro and skinny jeans. We begin showing him the different data types coming back and explain how its bad for performance and can lead to bugs in the future if the model structure changes between different call params. He matter of factually has an epiphany and exclaims "OHHHHHH! I got you covered dawg!" and begins click clacking on his laptop to make sense of it all. We decide not to disturb him any more so he can keep working.
3 hours goes by...
He burst out of our conference room shouting "I am the greatest coder in the world! There's no problem I can't solve! Test it now!"
Weary, we begin testing the endpoints in our REST clients....
His magic fix, every single response is a quoted string of json:
example:
- old response
{
"foo" : "bar"
}
- new "improved" response
"{ \"foo\" : \"bar\" }"
smh....8 -
I recently got into an argument with some people, and I want your opinion. I did a speed code in Java (just sped up clip of programming, because it looks cool lol), and someone commented:
"Way too much static abuse here. Jesus"
In which I replied:
"Actually, sir. There is near none at all, just because I use static methods does not make it static abuse. A static method belongs to the class, and is somewhat permanent. It is not a type (instance, cat, dog, animal, etc.) class, it is a Utility class, much like other dependencies you'd use are Utilities and not types."
To which they reply:
"Getting and setting is a Utility?"
Boi. If it is a static variable, yes. Like, what?5 -
What I'm doing now, writing a JS library for a simple kitchen timer (like, something that can be wound up, is ticking, can be paused, etc). Here's a list of neat stuff I've learned:
Polyfilling as a lib author (I decided against it).
Packaging the lib (using Rollup, ES6 modules are totes cool).
Using flow to add static typing in strategic places (started appreciating types in JS since reading up on functional programming).
Modelling state and transitions using an explicit state machine. (Fucking finally. There's usually an implicit state machine somewhere, only spread out all over the app...)
Using mostly side-effect free methods, being very explicit about when and why things are mutated).
Test-first/TDD (ish) using Jest and the awesome Wallabyjs.
Freeing up mental capacity by letting Prettier format my code for me (it was hard to let go but totally worth it).
Started using git.
Did all work on Ubuntu after pretty much a lifetime of Windows (initially to separate work from gaming) and finally swapped MS Visual Studio for Atom.
When it's finished I'm going to publish it on GitHub, which will also be a first for me. Might try out some CI platform while I'm at it.
tl;dr: wrote some js, felt good2 -
I started applying for jobs. As I have over 150 repos on GitHub and 10 years of relevant work experience, the company obviously had trouble validating if I had some basic coding skills. That's why they decided to send me a coding "homework" task to build an app in React Native.
Basically, the task was building an app with 2 screens and one bonus where they indicated "doesn't need a UI". I spent half a day spinning up their project, installing XCode, their specific versions of Ruby, and around half a day building the thing.
Obviously, I wanted to demonstrate my technical skills, so I added a few tests, proper typing, comments, and so on. The project was in a good state, and on the "bonus" screen I quickly added a few components. Since I have a lot of things going on, I capped the amount of time to one day of work. I felt it was good enough to demonstrate I can build something like this.
A few days later, I received a response from the recruiter telling me they wouldn't move forward. She in depth explained that this was because of a missing key property. I did indeed miss one key property on the "bonus" screen, in the part that was not even part of the core task. This was a list of very few static elements, and the entire list only got rerendered when changing routes. Basically in this case, there would not be any visible performance impact.
The recruiter explained in the email that I was missing the eye for detail they need, and that I should "educate" myself more about lists in React. I made one tiny silly mistake in a one-day project, that a linter would've taken out (if this project had one). I've contributed to React Native myself and worked with React for almost 7 years now? Yeah, it's a stupid thing, but what is the point of these types of tasks? I thought this was to demonstrate my skillset, not to be called out on.
Either way, my question here is this: at which point does it become appropriate to send an invoice for the time I wasted on this?6 -
Today in Rust I defined a function that takes Any and returns Any specifically so that parametric types turned into trait objects of this trait can still receive commands without having to know their concrete type.
Bridging static and dynamic typing is one hell of an exercise.8 -
I need guidance about my current situation.
I am perfectionist believing in OOP, preventing memory leak in advance, following clean code, best practices, constantly learning about new libraries to reduce custom implementation & improve efficiency.
So even a single bad variable name can trigger my nerves.
I am currently working in a half billion $ IT service company on a maintenance project of 8 year old Android app of security domain product of 1 of the top enterprise company of the world, which sold it to the many leading companies in the world in Govt service, banking, insurance sectors.
It's code quality is such a bad that I get panic attacks & nightmares daily.
Issues are like
- No apk obfuscation, source's everything is openbook, anybody can just unzip apk & open it in Android Studio to see the source.
- logs everywhere about method name invoked,
- static IV & salt for encryption.
- thousands of line code in God classes.
- Irrelevant method names compared to it's functionality.
- Even single item having list takes 2-3 seconds to load
- Lag in navigation between different features' screens.
- For even single thing like different dimension values for different density whole 100+ lines separate layout files for 6 types of densities are written.
- No modularized packages, every class is in single package & there are around 100+ classes.
Owner of the code, my team lead, is too terrified to change even single thing as he don't have coding maturity & no understanding of memory leak, clean code, OOP, in short typical IT 'service' company mentality.
Client is ill-informed or cost-cutting centric so no code review done by them in 8 years.
Feeling much frustrated as I can see it's like a bomb is waiting to blast anytime when some blackhat cracker will take advantage of this.
Need suggestions about this to tackle the situation.10 -
I really hate PHP frameworks.
I also often write my own frameworks but propriety. I have two decades experience doing without frameworks, writing frameworks and using frameworks.
Virtually every PHP framework I've ever used has causes more headaches than if I had simply written the code.
Let me give you an example. I want a tinyint in my database.
> Unknown column type "tinyint" requested.
Oh, doctrine doesn't support it and wont fix. Doctrine is a library that takes a perfectly good feature rich powerful enough database system and nerfs it to the capabilities of mysql 1.0.0 for portability and because the devs don't actually have the time to create a full ORM library. Sadly it's also the defacto for certain filthy disgusting frameworks whose name I shan't speak.
So I add my own type class. Annoying but what can you do.
I have to try to use it and to do so I have to register it in two places like this (pseudo)...
Types::add(Tinyint::class);
Doctrine::add(Tinyint::class);
Seems simply enough so I run it and see...
> Type tinyint already exists.
So I assume it's doing some magic loading it based on the directory and commend out the Type::add line to see.
> Type to be overwritten tinyint does not exist.
Are you fucking kidding me?
At this point I figure out it must be running twice. It's booting twice. Do I get a stack trace by default from a CLI command? Of course not because who would ever need that?
I take a quick look at parent::boot(). HttpKernel is the standard for Cli Commands?
I notice it has state, uses a protected booted property but I'm curious why it tries to boot so many times. I assume it's user error.
After some fiddling around I get a stack trace but only one boot. How is it possible?
It's not user error, the program flow of the framework is just sub par and it just calls boot all over the place.
I use the state variable and I have to do it in a weird way...
> $booted = $this->booted;parent::boot();if (!$booted) {doStuffOnceThatDependsOnParentBootage();}
A bit awkward but not life and death. I could probably just return but believe or not the parent is doing some crap if already booted. A common ugly practice but one that works is to usually call doSomething and have something only work around the state.
The thing is, doctrine does use TINYINT for bool and it gets all super confused now running commands like updates. It keeps trying to push changes when nothing changed. I'm building my own schema differential system for another project and it doesn't have these problems out of the box. It's not clever enough to handle ambiguous reverse mappings when single types are defined and it should be possible to match the right one or heck both are fine in this case. I'd expect ambiguity to be a problem with reverse engineer, not compare schema to an exact schema.
This is numpty country. Changing TINYINT UNSIGNED to TINYINT UNSIGNED. IT can't even compare two before and after strings.
There's a few other boots I could use but who cares. The internet seems to want to use that boot function. There's also init stages missing. Believe it or not there's a shutdown and reboot for the kernel. It might not be obvious but the Type::add line wants to go not in the boot method but in the top level scope along with the class definition. The top level scope is run only once.
I think people using OOP frameworks forget that there's a scope outside of the object in PHP. It's not ideal but does the trick given the functionality is confined to static only. The register command appears to have it's own check and noop or simply overwrite if the command is issued twice making things more confusing as it was working with register type before to merely alias a type to an existing type so that it could detect it from SQL when reverse engineering.
I start to wonder if I should just use columnDefinition.
It's this. Constantly on a daily basis using these pretentious stuck up frameworks and libraries.
It's not just the palava which in this case is relatively mild compared to some of the headaches that arise. It's that if you use a framework you expect basic things out of the box like oh I don't know support for the byte/char/tinyint/int8 type and a differential command that's able to compare two strings to see if they're different.
Some people might say you're using it wrong. There is such a thing as a learning curve and this one goes down, learning all the things it can't do. It's cripplesauce.12 -
Working with a SOAP endpoint. I know it is some .NET server due to the style of stacktrace on exceptions. Nice, a framework where I can expect some type safety granted by static types. I build some xsl to transform the SOAP wsdl files into classes and structs to interact with the endpoint. Works out perfectly.
Plottwist!
Elements which are defined in the xsd/wsdl with maxOccur=unbounded and minOccur=0 should represent a simple collection of this type. Therefore does my implementation expect a collection of this type. But no. The shipped SOAP client in my stack ignores the definition and simply deserializes the SOAP response into T and not a collection of T.
Where the duck are the types when they are defined all over the place?2 -
Any other people here that find Python to be actually a harder language than Java? With Java it's much easier to keep track of your code and to track what variables refer to certain object types.
It feels like Python has much more quirks and feels therefore much more inconsistent as a language. Object oriented programming is more verbose with static methods and decorators being vague for example. This makes it harder to grasp concepts like design patterns and SOLID principles in Python imo.7 -
Every once in a while the flexibility of dynamic types comes back and bites you in the ars:
So I created method that returns the date significance (day, month, year) or null when no date is set.
I chose a class constant DAY with the value 0.
This is not a problem in if statements as I always use === but in this case a switch made more sense. And as you can guess no date set (NULL) was handled in the self::DAY (0) case... 😐😑😶 Silently resulting in wrong results when no date is given! #£#@$& (and other comic swearing symbols)
Even though php7 finally has decent type hinting resulting in much clearer defined API's we can still go very wrong.
More love to Go for less verbose static typing! To bad we can rarely use it at the office 😥2 -
ok found the object orientated guide but for rust which is functional spaghetti: https://howtocodeit.com/articles/...
it has moved into architecture
... and actually makes a good case for interfaces / traits. generally in languages I just used generics to get around limitations of having to type a lot / duplicate code, and I'd remove interfaces because they're annoying to have to deal with, but I can see this be useful for once now.
like you can start a prototype app with files as a database then move to a small database type then later a more monolithic big data one and all that would be through one trait the whole time. so you could anticipate natural progressions of an app, instead of having to build the last version you can put jank behind interfaces and then switch things in and out to test new technologies which does actually give me a lot of relief for my newfound anxiety of me rewriting my rust codebases because I get some small things wrong. I've been coding in circles due to it and I have several saved files that are out of date now but I don't want to delete and they make the compiler mad cuz I had no interface boundaries as such and now stuff has changed somewhere else in the app and by God pls argh
this also means you can code "top-down". in carl Jung typology that's Te and most programmers are Ti-types so they do the little details and then sort of glue everything together (?) but not everybody thinks this way. I naturally think more top-down, which works for more dynamic languages and is annoying in static languages because then you're just fighting semantics and your earlier work the whole time (actually this is a surprisingly good write-up on the different thinking types: https://bothsidesofthetable.com/the...)
wheeeee -
Okay, my initial revulsion for ABI has receded. All things considered, my options aren't that bad. I just had to change my perspective from "huge downgrade from static linkage" to "huge upgrade from a message channel".
Just like a web API, I have to draw a continuous line through the program that separates specific concerns of interest that must fall on one side or another, and which can only cross through things with specific properties.
There are several crates shipping a number of different binary-compatible types, even generic types. Not everything can cross, sure, but maybe not everything should cross either. Maybe a DLL should receive an opaque handle for certain things, such as interpreter internal code representations. Maybe having these separated is important enough to justify having a translation layer.
I'm sure there's much woe ahead, but I'm learning to stop worrying and love the ABI. -
in JavaScript I would just call something what it is and then keep changing the data type as I get more data to add to it because you can
in rust because it's not dynamic types but static and everything is a static struct I need to find like 9 different names for all the different intermediary data types and holy shit I don't understand what to name everything and this is annoying me
I never understood why people complained about naming problems. I found it fun. now I hate it.
stats object. cool. well it converts an address to stats. an address has swaps. each swap was done on a mint. so I guess I make a MintStats object? wrong. because that's confusing.
swaps -> swaps divided by the mint they belong in -> stats for each mint swap set -> then you can add all the mint swap set stats to the address stats object
now what the fuck do you call all these
there's also something I called a MintAttitude and it's an enum. these types just keep growing out of trees. fuk. I don't like long names either. I should probably just call it Attitude but call it via mint::Attitude and get the same clarity result with far less redundancy (which I hate, another annoying thing)
swaps -> ??? mint history? -> MintStats -> then I have a "MintData" that has the history and stats wrapped in it -> MintsData that has many mints and their MintData -> then I can convert MintsData into AddressStats but what and I hate this and also I have a Mint object that does something totally different elsewhere. I hate this. data isn't even descriptive but to call something history when it also has stats seems imprecise.
brain spaghetti. classification part of my brain is shit. no historical training / experience either. I just see everything like vague blobs. bah. naming required clear delineations which is hard enough on its own to get used to5 -
What's the point of using TypeScript if you type all of your code with `any` or worst part is you don't provide any types!?
P.S
If you're anti-typescript or any static linting tool, I know this will not prevent any runtime errors, but this is part of the testing/code quality. My teammates do not care for quality, and I'm tired of it.2 -
nothing new, just another rant about php...
php, PHP, Php, whatever is written, wherever is piled, I hate this thing, in every stack.
stuff that works only according how php itself is compiled, globals superglobals and turbo-globals everywhere, == is not transitive, comparisons are non-deterministic, ?: is freaking left associative, utility functions that returns sometimes -1, sometimes null, sometimes are void, each with different style of usage and naming, lowercase/under_score/camelCase/PascalCase, numbers are 32bit on 32bit cpus and 64bit on 64bit cpus, a ton of silent failing stuff that doesn't warn you, references are actually aliases, nothing has a determined type except references, abuse of mega-global static vars and funcs, you can cast to int in a language where int doesn't even exists, 25236 ways to import/require/include for every different subcase, @ operator, :: parsed to T_PAAMAYIM_NEKUDOTAYIM for no reason in stack traces, you don't know who can throw stuff, fatal errors are sometimes catchable according to nobody knows, closed-over vars are passed as functions unless you use &, functions calls that don't match args signature don't fail, classes are not object and you can refer them only by string name, builtin underlying types cannot be wrapped, subclasses can't override parents' private methods, no overload for equality or ordering, -1 is a valid index for array and doesn't fail, funcs are not data nor objects when clojures instead are objects, there's no way to distinguish between a random string and a function 'reference', php.ini, documentation with comments and flame wars on the side, becomes case sensitive/insensitive according to the filesystem when line break instead is determined according to php.ini, it's freaking sloooooow...
enough. i'm tired of this crap.
it's almost weekend! 🍻1 -
I just animating third type of button. Now i have.
.link.type-button - standard button with default, primary and destruct color, small, default and large size, animation on it's back, selectable icon.
.link.type-roll - wierd type of button, with only icon visible and text on hover with some wierd shit animation, selectable icon ofcourse. Various sizes and colors as well.
.link.type-more - More or less static link with right arrow and ofcourse animation on hover.
So one site has three types of buttons with various properties.
And thinking about how overengeneered JS world is.
Fuck you JS world.6 -
Write C++ like it's Python, write Python like it's C++.
C++ everyone else has to use types so you can get away with auto and the compiler will tell you when you've done something wrong. Python, nobody else has to use types so type everything and let the static checker tell them when they've done something wrong so they don't pester you.