Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
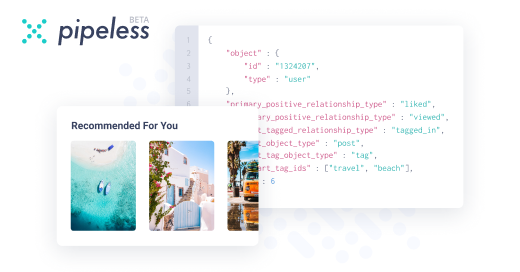
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "foreach"
-
Developer (master's degree, -bleeping- smart guy, no kidding) was bragging on how he made a piece of code 3x faster (with the usual pinch that the original dev was incompetent) and spent nearly 6 weeks working on it (wrote his own parallel-foreach library because Microsoft's parallel library was "too slow").
I was the original dev and he didn't know I had my own performance counters where I broke down each stack (database access, network I/O, and the code logic).
Average time was around 5ms (yes milliseconds) and worst case was around 10 seconds. His '3x improvement' was based on the worst test case, which improved by about a second. Showed our boss my graph (laughed out loud, said 'WTF', other curse words) and the dev hasn't spoken to me in weeks (I say 'hi' in the hall and he keeps walking)
Take that master's degree and high IQ and shove it.17 -
I work at a small company that uses very outdated coding approaches for their solutions.
About a year ago I went through our main application to improve performance and found quite a few areas that I could tackle such as using a dictionary data structure in place of (many) foreach loops that required to pull out a single object.
That specific change yielded a lot of improvement (you can only imagine) and the other developers wanted to learn the ways of dictionaries (because it was so revolutionary and new to them). I showed them many examples so that they could better understand this data structure.
Fast forward to a few months later, saw one of my coworker's code and noticed that they were using a dictionary... And iterating through each kvp similar to a foreach..... Wtf?!
P.S. that person's salary is much higher than mine :(
First time rant. Thanks for listening!10 -
We were learning to create a login system in mysql and php when one of my classmates showed me and the teacher his code.
He did a select all on the users table and looped through everything with a foreach.
We both tried to explain him why that wasn't the best method but he just refused to accept that.
He turned out a designer.
By the way, please never do it his way 😬18 -
Just replaced a 300 line switch case with a simple if statement inside a foreach loop.
Feels sooo good man9 -
Most satisfying bug I've fixed?
Fixed a n+1 issue with a web service retrieving price information. I initially wrote the service, but it was taken over by a couple of 'world class' monday-morning-quarterbacks.
The "Worst code I've ever seen" ... "I can't believe this crap compiles" types that never met anyone else's code that was any good.
After a few months (yes months) and heavy refactoring, the service still returned price information for a product. Pass the service a list of product numbers, service returns the price, availability, etc, that was it.
After a very proud and boisterous deployment, over the next couple of days the service seemed to get slower and slower. DBAs started to complain that the service was causing unusually high wait times, locks, and CPU spikes causing problems for other applications. The usual finger pointing began which ended up with "If PaperTrail had written the service 'correctly' the first time, we wouldn't be in this mess."
Only mattered that I initially wrote the service and no one seemed to care about the two geniuses that took months changing the code.
The dev manager was able to justify a complete re-write of the service using 'proper development methodologies' including budgeting devs, DBAs, server resources, etc..etc. with a projected year+ completion date.
My 'BS Meter' goes off, so I open up the code, maybe 5 minutes...tada...found it. The corresponding stored procedure accepts a list of product numbers and a price type (1=Retail, 2=Dealer, and so on). If you pass 0, the stored procedure returns all the prices.
Code basically looked like this..
public List<Prices> GetPrices(List<Product> products, int priceTypeId)
{
foreach (var item in products)
{
List<int> productIdsParameter = new List<int>();
productIdsParameter.Add(item.ProductID);
List<Price> prices = dataProvider.GetPrices(productIdsParameter, 0);
foreach (var price in prices)
{
if (price.PriceTypeID == priceTypeId)
{
prices = dataProvider.GetPrices(productIdsParameter, price.PriceTypeID);
return prices;
}
* Omitting the other 'WTF?' code to handle the zero price type
}
}
}
I removed the double stored procedure call, updated the method signature to only accept the list of product numbers (which it was before the 'major refactor'), deployed the service to dev (the issue was reproducible in our dev environment) and had the DBA monitor.
The two devs and the manager are grumbling and mocking the changes (they never looked, they assumed I wrote some threading monstrosity) then the DBA walks up..
DBA: "We're good. You hit the database pretty hard and the CPU never moved. Execution plans, locks, all good to go."
<dba starts to walk away>
DevMgr: "No fucking way! Putting that code in a thread wouldn't have fix it"
Me: "Um, I didn't use threads"
Dev1: "You had to. There was no way you made that code run faster without threads"
Dev2: "It runs fine in dev, but there is no way that level of threading will work in production with thousands of requests. I've got unit tests that prove our design is perfect."
Me: "I looked at what the code was doing and removed what it shouldn't be doing. That's it."
DBA: "If the database is happy with the changes, I'm happy. Good job. Get that service deployed tomorrow and lets move on"
Me: "You'll remove the recommendation for a complete re-write of the service?"
DevMgr: "Hell no! The re-write moves forward. This, whatever you did, changes nothing."
DBA: "Hell yes it does!! I've got too much on my plate already to play babysitter with you assholes. I'm done and no one on my team will waste any more time on this. Am I clear?"
Seeing the dev manager face turn red and the other two devs look completely dumbfounded was the most satisfying bug I've fixed.5 -
Dear Microsoft,
Please kill Internet Explorer post haste. The last time I want to hear "IE" is from the screaming sounds of your hated browser dying in a fire.
Sincerely,
A web dev9 -
So this fucktard decided to write the most inefficient way to collect thousands of records.
The system I am working on allows users to book facilities. There is one feature where an admin can generate reports on the bookings made between any two dates. A report for bookings made between January and April generates 7878 records.
So this shithead, after making a call to the server and receiving 7878 records decides to put it through 4 fucking foreach loops (this takes around 44.94 seconds).
After doing that, he passes it to the controller to go through ANOTHER foreach loop to convert those records into a JSON string, using..string..manipulation. (this takes bloody 1 minute and 30 seconds).
Now, my dear, dear supervisor is asking me to fix this saying that there must be a typo somewhere. Typo my arse. This system has been up for more than a year. What have they been doing all this time??? Bloody hell. Fucking idiots everywhere. I now have to refactor
..fucking refactor.2 -
Ticket : "I have a blank page when I load this page"
I open the script (php), I see at least 7 nested foreach loop starting with a 2600+ long array. I spot the buggy loop. I mark the ticket "may need refactoring". I go drink a beer with colleagues. -
So a few years ago when I was getting started with programming, I had this idea to create "Steam but for mods". And just think about it - 13 and a half years old me which knew C# not even for a half of a year wanted to create a fairly sizable project. I wasn't even sure how while () or foreach () loops worked back in the day.
So I've made a post on a polish F1 Challenge '99-'02 game forum about this thing. The guy reached out to me and said: "Hey, I could help you out". This is where all started.
I've got in touch with him via Gadu-Gadu (a polish equivalent of ICQ). So I've sent him the source code... Packed in .ZIP file... By Zippyshare… And just think how BAD this code was. Like for instance, to save games data which you were adding they were stored in text files. The game name was stored in one .txt file. The directory in another. The .exe file name in yet another and so on. Back then I thought that was perfectly fine! I couldn't even make the game to start via this program, because I didn't know about Working Directory).
The guy didn't reply to me anymore.
Of course back then it wasn't embarrassing to me at all, but now when I think about it... -
When defining a range, let's say from 1 to 3, I expect:
[1, 2, 3]
Yet most range functions I come across, e.g. lodash, will do:
_.range(1, 3)
=> [1, 2]
And their definition will say: "Creates an array of numbers ... progressing from start up to, but not including, end."
Yet why the fuck not including end? What don't I understand about the concept of a frigging range that you won't include the end?
The only thing I can come up with that's this is related to the array's-indexes-start with-0-thing and someone did not want to substract `-1` when preparing a for loop over an 10 items array with range(0,10), even though they do not want a range of 0 to 10, they want a range from 0 to 9. (And they should not use a for loop here to begin with but a foreach construct anyway.)
So the length of your array does not match the final index of your array.
Bohhoo.
Yet now we can have ranges with very weird steps, and now you always have to consider your proper maximum, leading to code like:
var start = 10;
var max = 50;
var step = 10;
_.range(start, max + step, step)
=> [10, 20, 30, 40, 50]
and during code review this would scream "bug!" in my face.
And it's not only lodash doing that, but also python and dart.
Except php. Php's range is inclusive. Good job php.4 -
Exams comming up and I'm here wishing life to be codable:
If (atLeastTrying == true)
{
Foreach (char score in subjects)
{
score = 'A'
}
}5 -
I'm so fucking tired of OOP.
This bullshit never ends. Everyone treats OOP in their own, proper (of course) way. You read tons of those fashion books, like uncle bob and shit. and then comes a dumb asshole that starts reviewing your code, and tells you doing it wrong. FUCK. and you can't tell anything to your TL or PM cuz they are same dumb asholes. Because after you fix all the bullshit from the first asshole, those more responsible assholes come and tell you that you still doing it wrong.
- uh.. bruh, why don't you make interface for everything? that' S.O.L.I.D, you know.. it just right thing.
- bruh, why don't you use enum and switch case. we need a factory.
- bruh, we don't use abstract classes, use interface
- could you rewrite your linq/stream thing into a class and a method. it's just simpler for us. foreach loop is something everyone knows.
well,then go and LEARN the tool you're dealing with, coderfucker.
FUUUUCK.13 -
I need bleach...
Lot of bleach.
When you think that not using a JS framework is bad...
Ever saw a Frankenstein of a HTML, PHP and JQuery? Full rewrite of an old project with more than enough time allowed....
Just... That was not awful enough.
*sobs* so the dev added bootstrap onto this pile of garbage... Instead of rewrite....
Think I missed CSS or included it in HTML? Lol. No.
No CSS. Inline. HTML 4 Tags.
?>
<table width=40 class="table table-striped">
<?php
foreach( $table as $row ) {
....
<input onkeyup="..." onkeydown="..." class="form-control"
...
To give you a basic example of how worse it is...
But the best. The lead developer does not understand why I was speechless.
i need more beer. And bleach. Filth and disgust must get out of my system2 -
Part of the commit message I wrote yesterday after discovering that I used break instead of continue to skip a foreach iteration.
-
Got a mini project assignment in college. We decided to make a game using Unity that recgonizes sign language gestures from Leap Motion.
I asked my colleague to make the function to compare the hand gesture, and I'll do the rest of the game. One more friend does the documentations and reports.
Three weeks passed, asked for the code but he said he hasn't finished it yet. I told him to ask me if he has any problems with it.
He sent me this 24 hrs before the deadline.
Me and my other friend died on the spot.
I screamed at him the whole night on the phone call whilsth trying to do his part in just 3 hours...
Needless to say, we didn't finish the project on time.rant compscistudent unity why are we still here just to suffer wk125 student foreach leap motion forloop assignments why do i even try -
Saw a question on SO asking why foreach was slow with big data.
The code provided was 6 nested foreachs (basically a cartesian product between an array of arrays, and 4 other arrays).
Inside, a select query and an "update or create" operation.
"But why is foreach so slow?"4 -
Earth keeps rotating
Seasons repeats
Life goes and come back
Machines in factories doing repetitive job
You sleep, you wakeup, you eat daily
... and y'all think loops are bad?2 -
I swear I NEVER hated PHP before, but after seeing this shit, I just can't love it anymore, I feel betrayed
I mean, WTF? Just bc I'm using the variable as a reference in the NEXT loop the LAST element changes? What even is the fucking reason?
Yeah, I read the answer on StackOverflow, but seriously...
SO ref: https://stackoverflow.com/questions...12 -
Found this on prod
Don't know who did this but wwhhhyyyy do you need to add foreach loop😅
Just add directly9 -
You wrote a little simple and clean mvc framework to work faster on some new projects. It can "compile" tags as {% var %} or {% array.key %} in the html code with support of {% for arrayOfHash in hash %} foreach construct and nice features, it can call api's callback in a smart way as ghost methods of a class, he can make routes with the route provider. You tested it and made a little example, after you went in the bathroom you read the index code and you started staring at the beauty and elegance of it. You go to bed happy and sleep. The day after you wake up and realize that it's unuseful because there's a lot of mvc framework that surely are better and ready to use, so you lost useful time. Have you ever feel this way? MVC: Me Versus Creativity.5
-
A long long time ago ( 2007 I think ) I worked for a company that made landing sites, so basically an email campaign would go out, users would be sent to a 1 page website with a form to capture their data, ready to be spammed even more. You know how it was back then.
So I worked with a guy who we had just hired, I didn't do the hiring but his CV checked out, so I gave him one of my tasks. Now most pages were made with js and html, with a PHP backend ( called with Ajax). Now this guy didn't know PHP so I was like all good, ASP works too at the end of the day we don't judge, we do like 2 or 3 of these a day and never look at them again. So he goes of and does is thing.
3 weeks later, the customer calls up to me they still haven't received their landing page. Ok so he probably forgot to email the customer np, I tell him to double check he has emailed the customer. Another week goes by end the customer calls back, same problem. At this point I'm getting worried, because we're days away from the deadline and it was originally my task.
So I go back to the guy and I tell him I want that landing page so I can send it myself, half thinking to myself that we had a freeloader, that guy that comes in to companies for 3 weeks, doesn't work, but still cashes his pay. But no, this was much worse.
So he tells me he has finished yet. I ask him why, what's the blocker ? You had 4 weeks to tell me you were blocked and couldn't progress. And his answer was simply, because I wasn't blocked I have been working on it this whole time. So I tell him to zip his project up and email it to me. We didn't do SVN or git back then, simply wasn't worth it. So he comes back to me and says the email server is telling him attachments can't be bigger then 50mb. At this point I'm thinking he didn't properly sized the art or something, so I give him a flash drive to put it on.
When I then open the flash drive, the archive is 300mb, thinking to myself, the images weren't even that big to begin with.
So I open it up, and I don't even find any images, just a single asp page. About 500mb. When I opened that up and it finally loaded, I saw the most horrendous things ever.
The first 500 lines was just initializing empty vars. Then there was some code that created an empty form with an onChange event that submits the form. After that.. it was just non stop nested if's. No loops, no while, for, foreach, NO elseif's, just nested if's, for every possible combination of the state the form could be in. Abou 5000 of them, in a single file. To make matters worse, all the form ( and page ) layout was hardcoded in the if's. Includes inline css, base64 encoded images, nothing but as dynamic, based on the length of the form he changes the layout, added more background etc. He cut the images up for every possible size of the page and included them in the code.
I showed it to my boss, he fired the guy on the spot. I redid the work from scratch, in under 4 hours. Send it to the client. they had no ammends to make, happy as Larry. Whish I kept the code somewhere.
Morale of the story, allways do a coding test on interviews, even if small things just to sanity check.3 -
Not sure if it's the worst code review but it's a recent one.
We don't really do code reviews where I work unfortunately but my coworker used my framework for the first time (build some nice composer libraries for cmdline projects) and asked if I could make them do autoloading.
He never used namespaces before so I was glad to help him out.
What I saw was a dreadful mess. His project was called "scripts" so good luck picking a namespace...
Than it was all lose functions in the executable file. All those functions are however called by a class in another file (if they where not calling eachother as a cascading mess). That class was extending an abstract class from my library as instructed. However I never imagined my lib being raped like that.
The functions themselves are a horrible mess. Nothing uniform completely different style (our documentation states PSR's should be used).
Parameters counts higher than 5.
Variable names like Object and Dobject (in calling function Dobject is Object but it needs a fresh one.
If statements on parameters that need basically split it in two (should simply be to functions)
If else statement with return of same variable as a single line (sane people use ternary for that)
Note that I said functions. All of it should have been OO and methods. Would have saved at least some of the parameter hell.
I could go on and on. Do I think the programmer is bad yes (does not even grasp interfaces, dep injection, foreach loops). Is this his best work no. He said that for a one of script like this it just has to work. Not going to be used elsewhere. I disagree as it is a few thousand lines of code that others have to read too.2 -
Why I try to ALWAYS use semicolons in JS:
In short, weird shit happens sometimes
An example:
So I'm doing a small project for freeCodeCamp, working with the Twitch API. I decided to make an array on the fly to append a few elements to a documentFrag in order after setting all my props. Forgot a semicolon. Apparently, Babel transpiles this:
info.innerHTML = (``)
[span, caret, info].forEach(elm => frag.appendChild(elm));
to this if you omit the semicolon:
info.innerHTML = ' '[(span, caret, info)]
this is why you should avoid relying on ASI, you're going to have to remember them in other languages out there, so for your own sanity, might as well get used to them. Just thought I'd share--who knows, might help a JS newb out there somewhere.5 -
Nothing but love for my coworker;
but my coworker (who's a fellow programmer for at least 3 years) thought the `continue` statement in a `foreach` literally meant to keep going with the code.
He was confused his code after the continue didn't run. xD11 -
$test = TRUE;
$baby = TRUE;
$egg = 1
$sperm = 1;
if ($test != 'TRUE') {
print "No Baby.";
} else {
foreach($eggs as $egg)
{
$baby = count($egg + $sperm);
return $baby;
printf("Congratulations");
}
}10 -
Adobe's ExtendScript toolkit is abyssmal. I find posts from 2008 referring to issues that have not changed even in CC2017. Do you think they are small issues I'm bitching about? I'll list 2. First, the toolkit only colours "var, return, for, foreach" and a bit more keywords and the strings, of course you can set up color schemes but those are limited and not colouring stuff. The second issue is auto-complete, it rarely kicks in and suggestions have 0 connection to what are you doing and are always the same. It doesn't recognize anything of what are you doing.
Probably in 2008 you had to program with the manual near you like writing assembler, now there's an improvement in 2017, they got a window named object browser or something like that that actually is a summarised portable manual that could've been easily transformed in auto-complete suggestions.
Adobe writes about this and I quote: "a complete integrated development environment". Although I will not write much scripts in it, I need to write a big one and thought about extracting that object data and putting it in a more capable javascript editor. LO and Behold what I discovered, the ExtendScript Toolkit that's supposed to edit Extended javascript and save it as jsx or jsxbin is almost completely (it has some dlls too) built using around 100 jsx files. It's the equivalent of building a js IDE to edit js.
Sorry for formatting, I'm on mobile, I tried. -
lecturer teaching us ASP.NET in the final year of degree: Are you guys familiar with a foreach loop?
(we've been studying C# for the past 3 years and are advanced students)
me: -facepalm and leaves the class-2 -
My C# class loves to come up with weird/unrealistic scenarios to teach a specific language feature... I feel like the more effective way to teach would be to mention a real life scenario where it makes more sense to use the feature and give it some context rather than coming up with some arbitrary series of classes to represent departments and employees and then say "write extension methods for them to write them out"
If you tell me that I'm going to go, ok this works, but is there a specific reason I should do this instead of using a for or foreach to do the exact same thing? Don't get me wrong I see the appeal of extension methods as well as LINQ but this class never gives any sort of context as to why we're doing stuff. This class could be good, I've had classes that focus on language specific features taught in ways that make sense... My Java prof did a great job...
Also all the slides are terribly written...
Like I attached an example of the description for extension methods... The slides then go on to explain how the syntax for them works and gives an example...
Like ok I guess technically you told me what they are and how to use them, but gave zero context...
On the opposite end of the spectrum, I go to MSDN for their definition of extension methods, and it is much more clearly written and gives context to where/why they're used... and this is supposed to be a 5th semester course...2 -
foreach(string language in LANGUAGES){
System.Console.writeLine(language.GetPhrase("Greeting"));
}2 -
if(obj == value) {
stuff;
}
Is for boys,
Arrays.asList(obj).filter(o -> o == value).forEach(o -> stuff);
Is for men1 -
I went down a rabbit hole of code changes to try and delete a stupid for loop with a break in it.
It was super stupid and I gave up and submitted to the fact that some battles are not worth the time and stress.
OK... But seriously, It was returning multiple entities from the database, but we only always want the first one. My logic is that we should just go in there and fix the LINQ so we are explicitly getting one entity out.
But fuck that logic. No I'll have to change fucking everything that's tied to that method and expects a list from it. Every fucking thing. That includes error handling, parsing, for loops..... Nevermind...
You can have your foreach and your break. I'm taking mine, now.rant break my back on this stupid code what do you want on the frontend last minute changes did this to me they couldn't decide1 -
Happend a few months ago...
We encountered a performance issue somewhere in our Code, written by a guy who already left.
It was kind of this:
foreach(var id in idList)
{
CallServiceWithDataBaseAccess(new List<string>()
{
id
}
}
Well. It was obvious as in this example... -
ARRAY LIKE OBJECTS
Long story short, i am fiddling a bit around with javascripts, a json object a php script created and encountered "array-like" objects. I tried to use .forEach and discovered it doesnt work on those.
Easy easy, there is always Array.from()..just..it doesnt work, well it does work for one subset called ['data'] which contains the actual rows i generate a table from, but for the ['meta'] part of the json object it just returns a length 0 object..me no understanderino
at least something cheered me up when researching, it was an article with the quote: "Finally, the spread operator. It’s a fantastic way to convert Array-like objects into honest-to-God arrays."
I like honest-to-God arrays..or in my case honest to Fortuna..doesnt solve my problem though2 -
My whole workflow for HTML is frustrating. I rather write pug, because it has mixins, includes and isn't that fucking repetitive. But to write pug within Laravel blade views, I have to preprocess it too blade. Then sometimes the syntax gets crazy because of escaping blade loops like an insane guy ('| @foreach($foo as $bar)'), but you get used to it after time.3
-
Devs who use the array map method for purposes other than generating a new array, and who use an empty return statement to satisfy the linter, should receive a slap in the face. A gentle one, but a slap nonetheless3
-
CLEARLY! ...
I'm drunk.
//motherfucking populate the mailings table yo
Route::get('fuckman', function () {
$statuses = array('queued', 'sent', 'failure');
$statusKey = rand(0,2);
$mailings=App\Models\Mailing::all();
foreach($mailings as $mailing){
$mailing->status=$statuses[$statusKey];
$mailing->save();
}
return 'fuckyou';
}); -
### Functions ###
range = $(if $(filter $1,$(lastword $3)),$3,$(call range,$1,$2,$3 $(words $3)))
make_range = $(foreach i,$(call range,$1),$(call range,$2))
equal = $(if $(filter-out $1,$2),,$1)
### Variables ###
limit := 101
numbers := $(wordlist 2,$(limit),$(call range,$(limit)))
threes := $(wordlist 2,$(limit),$(call make_range,$(limit),2))
fives := $(wordlist 2,$(limit),$(call make_range,$(limit),4))
fizzbuzz := $(foreach v,$(numbers),\
$(if $(and $(call equal,0,$(word $(v),$(threes))),$(call equal,0,$(word $(v),$(fives)))),FizzBuzz,\
$(if $(call equal,0,$(word $(v),$(threes))),Fizz,\
$(if $(call equal,0,$(word $(v),$(fives))),Buzz,$(v)))))
### Target ###
.PHONY: all
all: ; $(info $(fizzbuzz)) -
JS' array function forEach. Why. Why does it exist? How is a function with a callback better than a freaking simple language feature like for of? If I recall correctly, forEach is older than for of, but people still use it nowadays, and too frequently...
Hate it, definitely.
Also, talking about enumeration in JS, Object's "static" method entries. I can't see how it can't be an instance method. Same for keys and values, but I usually don't care about them.12 -
5 fucking days of Google search after Google search. Error after fucking error. Deadline getting closer by the fucking minute. teammates interrupting me every 10 minutes over discord asking for help on their fucking part of the project
and it turns out the solution was just one damn line
One fucking line in a forEach to iterate over the model data sending the necessary aspects to the Javascript function to create map pins for the database locations
5 fucking horrible days all amounts to 1 line
Really shows how much I still have to learn. And the yelling at my screen reveals my need to take an anger management class1 -
Best Explanation I found of every(), filter(), map(), some() and foreach() methods in Javascript
(at least for me as a non-js dev)
could help someone there
https://coderwall.com/p/_ggh2w/...2 -
I am very close to being a violent psychopath from "Always code as if the person who ends up maintaining your code is a violent psychopath who knows where you live."
Foreach within a foreach with counters and a check if the counters match in the inner foreach and in that case do something.... And that's not even the worst part of the code I have to modify ever so slightly... Fml3 -
I am happy today cause I manage to write a query in which two table have inner join and with third left .. haha...
I mean I was thinking of handling that situation with foreach.. But managed to do it in query by myself :)
Just hoping that query won;t break for different scenarios. But let just be me happy while it last .. I mean my client make some test -
C#: The fact that T[,] (multi dimensional arrays) implement IEnumerable, but not IEnumerable<T>.
But one dimensional arrays do.
But you can still foreach over them.
What in the actual hell?
Now I have to write something ugly like
var bruh = values.Cast<float>();23 -
I love C# but it almost seems to reward foreach abuse and object orientation overkill. (And that VS designer.cs files are auto generated and blow out any changes you make in them)3
-
when did you follow PHP developers last used PHP without a framework or at least used PHP itself for the most part?
a foreach and if don't count .. obviously 😁7 -
The forEach in JavaScript makes no sense. It looks like a map /filter/reduce but doesn’t actually return the array. Can you please loop like a normal language?8
-
At the other they I was writing a foreach but at the middle of line decided to change to a simple for.
Spent the next 10 minutes reflecting at my life thinking that I couldn't do a simple for given the compiler errors just to realize that I forgot to delete the each part of the for -
Back in time i was monitoring an asterisk server on a friday night. Usually it's monitoring cli is a calm terminal with infos and periodic notifications. On a random check i saw about a KM length red shit / blue shit. As it turned out my boss was using the password 2500 with the same username on a fucking SIP server and while watching football (heard from the voice logs) some romanian script kiddie's brute force script fucked it up. The journey wasn't stopped here. Next step was to them to foreach some calls with high rates to their own special phone number on about 30-50 lines. The first step was to stop the service but because it is a nice app it wont stop till you have an active call, took about 5 mins to realise it . Had to kill it a few times until it gave up. That was the moment when the 'now they are gonna fire me' feel kicked in. Do not use weakass passwords kids!
-
Continuation of my first comment regarding the poor use of dictionaries...
Co-worker's code:
Foreach kvp in dict1{
ForEach kvp2 in dict2{
...
ForEach x in list{
ForEach kvp3 in dict3{
...
}}}
At least 2 of those kvp iterations can be changed to a standard dictionary use
What the hell am I looking at...... :(3 -
I’m fucked off tonight.
I’m having to pull a very complex data set out of dB and loop through results in a table.
Easy, done, but one of the Columns I’m pulling out needs further broken down. It’s a comma delimited string.
I can’t get the data, and inside the same loop explode that string so that the contents can be handled independently.
Raging! I have foreach loops inside foreach loops and arrays inside objects that are inside arrays.
I’m going to bed furious.2 -
<?php
// This is the demo code of a PHP gotcha which took me some hours to figure out
$hr = "\n<hr>\n";
$JSON = '{"2":"Element Foo","3":"Element Bar","Test":"Works"}';
$array = (array)json_decode($JSON);
echo "Version: " . phpversion() . $hr;
// Tested on: 5.5.35 and 7.0.15
var_dump($array);
// Prints: array(3) { '2' => string(11) "Element Foo" '3' => string(11) "Element Bar" 'Test' => string(5) "Works" }
echo $hr;
var_dump($array['Test']);
// Prints: string(5) "Works"
echo $hr;
var_dump($array[2]);
var_dump($array['2']);
var_dump($array["2"]);
var_dump($array[3]);
var_dump($array['3']);
var_dump($array["3"]);
// Prints: NULL + Notice: Undefined offset ... in ...
echo $hr;
$newArray = array();
foreach ($array as $key => $value) $newArray[$key] = $value;
var_dump($newArray[2]);
var_dump($newArray['2']);
var_dump($newArray["2"]);
// Prints three times: string(11) "Element Foo"
var_dump($newArray[3]);
var_dump($newArray['3']);
var_dump($newArray["3"]);
// Prints three times: string(11) "Element Bar"1 -
Foreach (DevrantUser user in devRantUsers)
{
Bool hundredPlusPlus = false;
While (user.hundredPlusPlus == false)
{
Rant myRant = new Rant(awesomeness);
user.postRant(myRant);
If(myRant.plusPlus >= 100)
hundredPlusPlus = true;
}
}3 -
The crazy shenanigans you can do with C++ standard libs are fascinating.
Like implementig multithreading with just a foreach, and bindings which can make member function pointers to simple function pointers, and placeholders in bindings. Also lambda functions are cool.
Something between the lines:
my_crazy_class *tmp = new my_crazy_class(...);
std::vector<type> my_array = .....;
std::for_each(std::execution::par,my_array.begin(),my_array.end(),
[&](type in){
auto fn = std::bind( &my_crazy_class::my_crazy_fnc,*tmp,_1,random_static_value);
return fn(in);
});
ps:
It's pretty much pseudocode, and please don't do things like this, it's bad for your mental health.
pps:
I need to learn how to use this tools wisely. -
DailyCodingProblem: #1
Given an array of integers, return a new array such that each element at index i of the new array is the product of all the numbers in the original array except the one at i.
For example, if our input was [1, 2, 3, 4, 5], the expected output would be [120, 60, 40, 30, 24]. If our input was [3, 2, 1], the expected output would be [2, 3, 6].
this is my quickly solution in php:
$input_array = [1, 2, 3, 4, 5];
echo('INPUT ARRAY:');
print_r($input_array);
echo("<br/>");
foreach($input_array as $key => $value){
$works_input_array = $input_array;
unset($works_input_array[$key]);
$result[] = array_product($works_input_array);
}
echo('OUTPUT ARRAY:');
print_r($result);
outpout:
INPUT ARRAY:Array ( [0] => 3 [1] => 2 [2] => 1 )
OUTPUT ARRAY:Array ( [0] => 2 [1] => 3 [2] => 6 )5 -
Me : Here, a list with multiple strings, do your job, Foreach block
Code : *do only one iteration*
that code is lazier than me1 -
A wild random shitcode my coworker wrote 2 years ago appeared
var thingsToCheck = new List<String>();
foreach (var thing in thingsToCheck)
{
// 10 lines of logic
}
Random shit code used confusion. It's super effective.
But honestly, these were the only few lines in his checkin. We still try to figure out what he thought when writing this. -
I see a lot of React devs (ab)using the array.map function in cases where a forEach would be more suitable (e.g. without assigning the result, and without return statements). What are your thoughts on this?5
-
using System;
using System.Text;
using System.Text.Encodings;
//Bitwise XOR operator is represented by ^. It performs bitwise XOR operation on the corresponding bits of two operands. If the corresponding bits are same, the result is 0. If the corresponding bits are different, the result is 1.
//If the operands are of type bool, the bitwise XOR operation is equivalent to logical XOR operation between them.
using System.Text.Unicode;
using System.Windows;
using System.IO;
namespace Encryption2plzWOrk
{
class Program
{
static void Main(string[] args)
{
//random is basically a second sepret key for RSA exhanges I know there probaley is a better way to do this please tell me in github comments.//
Random r = new Random();
int random = r.Next(2000000,500000000);
int privatekey = 0;
int publickey = 0;
string privateKeyString = Console.ReadLine();
byte[] bytes3 = Encoding.ASCII.GetBytes(privateKeyString);
foreach(byte b in bytes3)
{
privatekey = b + privatekey;
}
int permutations = random/ 10000;
if(privatekey < 256)
{
while(permutations > 0)
{
foreach (byte b in bytes3)
{
privatekey = privatekey + (privatekey ^ permutations)*20;
}
}
}
publickey = privatekey*random;
Console.WriteLine("your public key is {0}",publickey);
}
}
}
would this be considerd ok HOBBYIST encryption and if not how would I do a slow improvment I used bitwise to edit bits so thats a check :D12 -
PrestaShop irony:
* Theirs modules have >3500 lines per class (eg. blocklayered.php)
* Theirs controllers have > 5000 lines and contains a LOT of html code inside
AND when I tried to add own module to theirs addons store they declined it because:
* I had unused $key var in foreach and this is "bad practice" as I was told
* In one hook I was returning 1 line of html code (i had to add global Js var) and they told me that I should put it into separate template file
-.-'2 -
How should you override Equals in Java?
We have model classes with lots of fields and the we override the details equals to compare all the fields. I guess like doing a deep comparison.
And in all these classes SONAR is complaining about lots of ifs, complexity, etc.
And it's killing the analysis time... Old issue never fixed because whoever setup sonar was too incompetent to bother asking, researching, or fixing...
Is there a better way to override the equals to get the same result but without triggering SONAR issues?
Pretty sure this is a solved problem. And well if the top of my head, is just create a Util method that uses reflection like
Boolean equals( Object a, b, Class class)
foreach (String f: class, getFields()) {if !compare(class.getField(f,a),class.getField(f,b)) return false; }
return true5 -
HI all.
I'm a novice in Web Development and I've made simple Web App I've just publish on Github. I just wanted to play a little to learn API manipulation and JavaScript's Fetch. So I decided to build an app based on Discogs' API for my personal use.
ShuffleBum is an app, which after providing a username in the dedicated field, retrieves all items of user's collection then randomize one of them before displaying it. That's help me to find my next listening records (I have 400+ vinyls in my collection and I always listenning too many time the same records ^^).
Because of Discogs' API construction, I must perform two fetch to retrieve all the items (Items are stacked at 50 per page). So, for my collection, there is 10 pages with 50 items. So, I used a foreach and a fetch to scan items and store them into an array.
But, this is really slow (800ms for each request), so for 10 pages, that's a lot. But I can't see how to improve those request. I can't do otherwise. Well, I guess.
Hope I will have so recommandation, hints, tricks.
Thank you.13 -
i have this js object containing an array with about 7000 values. firefox, chrome and even ie11 need about 1.5 to 2 seconds to process it.
but edge takes 10 times longer! does anybody know about this behaviour? i could not find anything.
so, serious question. i just find results for ie being slow but THIS time it does its work properly. console shows no errors. is there information about edge-specific issues with javascript?9 -
//not a rant
Ok so weird bug. Fellow C# people, help me out.
//already made it work so no I don't need to post it on SO
I write a Switch Case block based on the user's combo-box selection id.
if id 0, add everything to the mainpage grid
if 1, a foreach loop filtering out the ones with a certain attribute of the object as false and adding em to the grid on the mainpage
if 2, similar scenario as 1.
Countless times I had a null exception with the "count" variable being the number of items in the post which, wasn't null. there was no other variable that was being initialized from within the block, so I had no idea what was causing it.
Moving to an if-else statement doing the same thing, same issue.
In the end I created 2 empty lists before the switch case and filled them up and then another loop filling the mainpage grid with the now-filled list.
In the end im doing the same thing, with no issues, but I don't understand why adding it directly caused an error, what was null?
I wanna understand the working that might be causing this.. if anyone else came across this, would be glad to hear from you8 -
“Just a quick fix” Classic start to a reported ticket. Ticket states that a form field was not doing anything.
Think to myself ok this sounds like a nice easy one for the morning. A few hours later I find something like the following written by a senior member of the dev team.
SearchClass {
//...list of getter and setters
Private $snakeCaseName;
SearchFunction() {
Foreach($this as $property => $value){
//... if property keys = string for each object property then do code
If($property == “snakecaseProperty”){
//...do stuff
}
}
}
}
Why does this loop exist!!!!! All it does is remove any error checking if a getter method is misspelt...
To make matters worse the entire search method was over 300 lines building a MySQL query string.... even though there was an ORM and entity classes available!!!2 -
DevRant.Pet PetMaker(Account myAcc){
List<Account> accs = new List<Account>();
For(int i=0; i<100){
myAcc.Post(new Rant());
If(i%10==0)
accs.Add(new Account());
}
Foreach(Rant r in myAcc.rants){
Foreach(Account a in accs){
a.PlusPlus(r);
}
}
return myAcc.Buy(new Pet(cat));
} -
I don't really like the way how JavaScript does a foreach loop. Either you have to use the "for in" loop, or you have to use the "Array.prototype.forEach" function which opens a new scope.2
-
foreach(var strTable in strTableNames)
{
DjingisKhanWantsMyDataEverywhere()
}
...I am not fond of legacy code -
Why does every programming language have to have so many different ways of doing the same thing? I mean, come on, do we really need both for and foreach loops? And why do we have to choose between switch and if-else? Can't we all just get along and use the same damn structure? #FirstWorldProblems30