Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
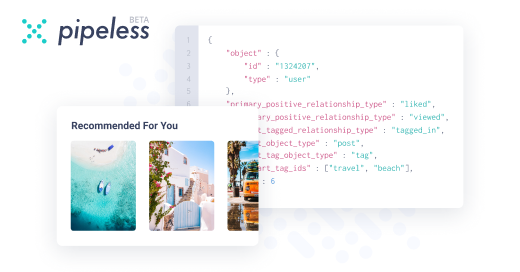
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "validations"
-
Recently I tried to apply for a job and the company sent me a task to complete. It was on Java, write an app to sort input file with ability to choose a method and dislpay it. GUI with Swing or JavaFX. They said normally it will took 8-10 hours to complete it and they wanted to see, what I can do in 4hrs. So after 4 hrs I've done~75% and sent it and after 2 more I've sent the whole app with monkey-proof protection (validations, prompts, etc). So total of 6hrs. I've followed MVC structure and implemented OOP principles.
They liked it and this Thursday I'm having an interview 😊
Wish me good luck :D6 -
I wrote a database migration to add a column to a table and populated that column upon record creation.
But the code is so freaking convoluted that it took me four days of clawing my eyes out to manage this.
BUT IT'S FINALLY DONE.
FREAKING YAY.
Why so long, you ask? Just how convoluted could this possibly be? Follow my lead ~
There's an API to create a gift. (Possibly more; I have no bloody clue.)
I needed the mobile dev contractor to tell me which APIs he uses because there are lots of unused ones, and no reasoning to their naming, nor comments telling me what they do.
This API takes the supplied gift params, cherry-picks a few bits of useful data out (by passing both hashes by reference to several methods), replaces a couple of them with lookups / class instances (more pass-by-reference nonsense). After all of this, it logs the resulting (and very different) mess, and happily declares it the original supplied params. Utterly useless for basically everything, and so very wrong.
It then uses this data to call GiftSale#create, which returns an instance of GiftSale (that's actually a Gift; more on that soon).
GiftSale inherits from Gift, and redefines three of its methods.
GiftSale#create performs a lot of validations / data massaging, some by reference, some not. It uses `super` to call Gift#create which actually maps to the constructor Gift#initialize.
Gift#initialize calls Gift#pre_init (passing the data by reference again), which does nothing and returns null. But remember: GiftSale inherits from Gift, meaning GiftSale#pre_init supersedes Gift#pre_init, so that one is called instead. GiftSale#pre_init returns a Stripe charge object upon success, or a Gift (and a log entry containing '500 Internal') upon failure. But this is irrelevant because the return value is never actually used. Pass by reference, remember? I didn't.
We're now back at Gift#initialize, Rails finally creates a Gift object using the args modified [mostly] in-place by all of the above.
Another step back and we're at GiftSale#create again. This method returns either the shiny new Gift object or an error string (???), and the API logic branches on its type. For further confusion: not all of the method's returns are explicit, and those implicit return values are nested three levels deep. (In Ruby, a method will return the last executed line's return value automatically, allowing e.g. `def add(a,b); a+b; end`)
So, to summarize: GiftSale#create jumps back and forth between Gift five times before finally creating a Gift instance, and each jump further modifies the supplied params in-place.
Also. There are no rescue/catch blocks, meaning any issue with any of the above results in a 500. (A real 500, not a fake 500 like last time. A real 500, with tragic consequences.)
If you're having trouble following the above... yep! That's why it took FOUR FREAKING DAYS! I had no tests, no documentation, no already-built way of testing the API, and no idea what data to send it. especially considering it requires data from Stripe. It also requires an active session token + user data, and I likewise had no login API tests, documentation, logging, no idea how to create a user ... fucking hell, it's a mess.)
Also, and quite confusingly:
There's a class for GiftSale, but there's no table for it.
Gift and GiftSale are completely interchangeable except for their #create methods.
So, why does GiftSale exist?
I have no bloody idea.
All it seems to do is make everything far more complicated than it needs to be.
Anyway. My total commit?
Six lines.
IN FOUR FUCKING DAYS!
AHSKJGHALSKHGLKAHDSGJKASGH.7 -
This happened via mail thread today.
Boss: we need this new brilliant feature I just made up and running asap! Top priority, it has to be done well, for my reputation is on the line!
Me: *looks at the specifics* 'kay, looks easy enough, this evening max and it will be ready. I just nees some extra info about what kind of data validations (I speak no accountant) are needed, and some other details (a total of 3 questiona).
B: Sure! Remember, it needs to be perfect, as my reputation is at stake. Call me on the phone and I'll give you the details!
M: Can't you answer via mail? Thua way both me and the other devs will have clewr guidelines
B: Just call me! Why do you need it to be written down? It's faster this way!
...Fine. I'll keep asking until you're ready to give me a written answer to my questions. No way I'll take security details via phone for something you want in production this evening. No chance in Hell I'll take responsibility for "misunderstanding" what you said on the phone. Why does it always has to be like that?8 -
CTO: Research, problem analysis, customer need validations, and data based prioritisation is stupid.
Me: So, then why should we solve this problem?
CTO: Because my team invests a lot of time in here (read "because we build a shitty system in past without thinking and we are doing it again").
Me: I don't see this as a good idea.
CTO: I become emotional when I request product to align and they don't. We must solve this problem and not what customers want.
Me: I am not participating here.
CTO: And I want you to work on weekends to support my team.
Me: *disconnects*3 -
Sometimes, being the only fullstack dev with access to a lot of systems gives you the ability to introduce functionality that:
A) prevents future errors
B) introduces new validations to users to make sure A) is prevented.
C) apply these changes to different projects
But most importantly...
D) without a single person in management getting involved or having to sign it off.
It's like running a company you own, but without owning it.
Granted with such power, comes the trust and responsibility of deploying changes with the adequate testing being done prior and handling change management, but fuck, sometimes I wonder if "god mode" for lack of a better term, is too much, or just enough to get the job done without the politics.7 -
I don't know if I'm being pranked or not, but I work with my boss and he has the strangest way of doing things.
- Only use PHP
- Keep error_reporting off (for development), Site cannot function if they are on.
- 20,000 lines of functions in a single file, 50% of which was unused, mostly repeated code that could have been reduced massively.
- Zero Code Comments
- Inconsistent variable names, function names, file names -- I was literally project searching for months to find things.
- There is nothing close to a normalized SQL Database, column ID names can't even stay consistent.
- Every query is done with a mysqli wrapper to use legacy mysql functions.
- Most used function is to escape stirngs
- Type-hinting is too strict for the code.
- Most files packed with Inline CSS, JavaScript and PHP - we don't want to use an external file otherwise we'd have to open two of them.
- Do not use a package manger composer because he doesn't have it installed.. Though I told him it's easy on any platform and I'll explain it.
- He downloads a few composer packages he likes and drag/drop them into random folder.
- Uses $_GET to set values and pass them around like a message contianer.
- One file is 6000 lines which is a giant if statement with somewhere close to 7 levels deep of recursion.
- Never removes his old code that bloats things.
- Has functions from a decade ago he would like to save to use some day. Just regular, plain old, PHP functions.
- Always wants to build things from scratch, and re-using a lot of his code that is honestly a weird way of doing almost everything.
- Using CodeIntel, Mess Detectors, Error Detectors is not good or useful.
- Would not deploy to production through any tool I setup, though I was told to. Instead he wrote bash scripts that still make me nervous.
- Often tells me to make something modern/great (reinventing a wheel) and then ends up saying, "I think I'd do it this way... Referes to his code 5 years ago".
- Using isset() breaks things.
- Tens of thousands of undefined variables exist because arrays are creates like $this[][][] = 5;
- Understanding the naming of functions required me to write several documents.
- I had to use #region tags to find places in the code quicker since a router was about 2000 lines of if else statements.
- I used Todo Bookmark extensions in VSCode to mark and flag everything that's a bug.
- Gets upset if I add anything to .gitignore; I tried to tell him it ignores files we don't want, he is though it deleted them for a while.
- He would rather explain every line of code in a mammoth project that follows no human known patterns, includes files that overwrite global scope variables and wants has me do the documentation.
- Open to ideas but when I bring them up such as - This is what most standards suggest, here's a literal example of exactly what you want but easier - He will passively decide against it and end up working on tedious things not very necessary for project release dates.
- On another project I try to write code but he wants to go over every single nook and cranny and stay on the phone the entire day as I watch his screen and Im trying to code.
I would like us all to do well but I do not consider him a programmer but a script-whippersnapper. I find myself trying to to debate the most basic of things (you shouldnt 777 every file), and I need all kinds of evidence before he will do something about it. We need "security" and all kinds of buzz words but I'm scared to death of this code. After several months its a nice place to work but I am convinced I'm being pranked or my boss has very little idea what he's doing. I've worked in a lot of disasters but nothing like this.
We are building an API, I could use something open source to help with anything from validations, routing, ACL but he ends up reinventing the wheel. I have never worked so slow, hindered and baffled at how I am supposed to build anything - nothing is stable, tested, and rarely logical. I suggested many things but he would rather have small talk and reason his way into using things he made.
I could fhave this project 50% done i a Node API i two weeks, pretty fast in a PHP or Python one, but we for reasons I have no idea would rather go slow and literally "build a framework". Two knuckleheads are going to build a PHP REST framework and compete with tested, tried and true open source tools by tens of millions?
I just wanted to rant because this drives me crazy. I have so much stress my neck and shoulder seems like a nerve is pinched. I don't understand what any of this means. I've never met someone who was wrong about so many things but believed they were right. I just don't know what to say so often on call I just say, 'uhh..'. It's like nothing anyone or any authority says matters, I don't know why he asks anything he's going to do things one way, a hard way, only that he can decipher. He's an owner, he's not worried about job security.13 -
The frustration that comes to each developer when he tries to write the code structured by thinking through each situations, and your team lead comes and tells you why r you doing so much validations just hard code them we need to release it today client is waiting. After 3-4 months the same team lead goes through the code and shouts at you telling why the he'll you hard coded all these, can't you write the code correctly by thinking through.3
-
We had 1 Android app to be developed for charity org for data collection for ground water level increase competition among villages.
Initial scope was very small & feasible. Around 10 forms with 3-4 fields in each to be developed in 2 months (1 for dev, 1 for testing). There was a prod version which had similar forms with no validations etc.
We had received prod source, which was total junk. No KT was given.
In existing source, spelling mistakes were there in the era of spell/grammar checking tools.
There were rural names of classes, variables in regional language in English letters & that regional language is somewhat known to some developers but even they don't know those rural names' meanings. This costed us at great length in visualizing data flow between entities. Even Google translate wasn't reliable for this language due to low Internet penetration in that language region.
OOP wasn't followed, so at 10 places exact same code exists. If error or bug needed to be fixed it had to be fixed at all those 10 places.
No foreign key relationships was there in database while actually there were logical relations among different entites.
No created, updated timestamps in records at app side to have audit trail.
Small part of that existing source was quite good with Fragments, MVP etc. while other part was ancient Activities with business logic.
We have to support Android 4.0 to 9.0 of many screen sizes & resolutions without any target devices issued to us by the client.
Then Corona lockdown happened & during that suddenly client side professionals became over efficient.
Client started adding requirements like very complex validation which has inter-entity dependencies. Then they started filing bugs from prod version on us.
Let's come to the developers' expertise,
2 developers with 8+ years of experience & they're not knowing how to resolve conflicts in git merge which were created by them only due to not following git best practice for coding like only appending new implementation in existing classes for easy auto merge etc.
They are thinking like handling click events is called development.
They don't want to think about OOP, well structured code. They don't want to re-use code mostly & when they copy paste, they think it's called re-use.
They wanted to follow old school Java development in memory scarce Android app life cycle in end user phone. They don't understand memory leaks, even though it's pin pointed by memory leak detection tools (Leak canary etc.).
Now 3.5 months are over, that competition was called off for this year due to Corona & development is still ongoing.
We are nowhere close to completion even for initial internal QA round.
On top of this, nothing is billable so it's like financial suicide.
Remember whatever said here is only 10% of what is faced.
- An Engineering lead in a half billion dollar company.4 -
New office saga continues... SE1E05
I transitioned from a B2B to B2C role. Now the company and the product is entirely consumer facing.
Many or rather all are actively engineering the product to be more and more dystopian in nature.
Using concepts like FoMo, social validations, and other techniques to get users to spend more into consumerism in the name of building better experience.
It's the darkest shit I have seen so far. And this company is ethically a great one. I can only imagine how pathetic Meta and others would be.
I hate ny role. I hate how I have to do this for a living. Knowingly or unknowingly, I got myself here and absolutely hate where we are headed as a human race.
I don't like it anymore and I am only doing it as a job. No longer proud or excited of my job profile.
Fuck the impact, technology will be a catalyst for human extinction.
And with that, I found a good solution to my Mac 😏
Do check: https://reddit.com/r/Unexpected/...6 -
Stupid stupid stupid API that returns a 204 on failed validations.
Informative docs? Hell no! Here's a few hundred long-ass field names that you need to pass as a JSON.
Doesn't work huh? Yeah, you're structure's all wrong. Some of these are grouped in vaguely named keys like "Wholesale".
Oh you need those as well? Yeah, you can see the whole structure if you try to GET an object.
Oh you need an ID to GET an object? Yeah you can just go ahead and create as many as you want. This is just a sandbox API, it's cool.
Oh that's not the point? Ahh you need the structure to be able to create one! *haha* Right, I'll get back to you on that.
* Email correspondence over 2 weeks time. I have still yet to be able to make a an actual successful request. The fucking 204 doesn't count if it doesn't actually create the resource.
Fucking fucky fuckity fuck fuck fuck.
I swear to god if I ever meet this guy in person, I will probably buy him coffee or beer and have a long talk about how to build proper REST APIs.
Because I'm nice like that.8 -
Company had problematic client projects that each client has a bucket load of change requests. Company doesn't know how to say "No" to them. Company can't afford to pay the subvendors for the changes and the subvendors aren't willing to do them for free.
I went in, reverse engineer the shit out of each application, database, system, documented my own findings, changed according to each client request. This involves editing tables in MSSQL, rerouting PHP files, adding field and validations in C#, passing parameters in VB to Crystal Report, and managed every change request into my own personalize ticket system (that the company does not have).
Saved the company, everyone was grateful. A couple of months later, the company hasn't paid my salary on time, I left like a boss.
They're in shit again and need my help. Haha! -
I made a web app where I can build any kind of form, with sections, validations, multiple type of inputs (even coordinates or polygons, photos, etc.) while allowing to see a preview of how that would look in a mobile app. That preview is because when you're done building the form and saved it to the DB, then in a mobile app (which I made as well) you can choose that form and use it.
Everything is beautiful, but after 3 sections with 25 questions each, everything becomes really slow because of the insane amount of actions that redux-form fires on every character type 😢
Today I made a public demonstration, and despite my code is very clean and well made, I think, that slowness made me look like a shitty developer, even thought I know what I made is like a 1000 times better than what I was asked to develop.8 -
I hope I did not make the wrong decision here:
Been working on a side project using React Js for a year now. After getting to know more about Vue, I just started rewriting it and moving it to Vue, to speed things up I'm using core JS classes for network stuff and validations ...etc just rewriting Redux to Vuex and React Components to Vue Templates
If I made the wrong decision I'd appreciate if anyone tell me about it before I go deeper in the rewrite process lol
It is not that I found speed difference both perform the same from what I've seen for my scenarios. But the output code of Vue is soooo much cleaner than what I found in React, either I failed to write a clean react code no matter how hard I try to optimize it, or Vue really takes the short way and keeps things clean.19 -
Project with partner company, during the meeting I asked them how can we secure the communication between two services. I suggested api keys, tokens. They were like nope, no need. But I asked them for their IPs to do whitelisting on our side in Nginx.
But their side, nah not even whitelisting, no tokens, no validations. If one has address, can send anything from anywhere.
How hard would it be to do at least, AT LEAST simple token validation. And they are using the very old IIS server. I think for them as long as data flows in as expected, it is fine.3 -
Working for a large client converting paper forms to the web. Stated goals, simplify data entry for clients, improve data quality, reduce resourcing in backend human processing.
We met to review prototype and discuss workflow questions. Crazy deadlines, with the usual changing scope creep.
We start to point out the need for data validation, to shorten # of questions based on answers.
Business says no. All forms should be submittable regardless of what user enters, don’t put validations in because all that warning messaging confuses them and takes up more time.
Web form should behave like the paper copy....
Welcome to 1975!!! This is why 2018 won’t be like 2018...1 -
fuck the guy that writed the api that I consume at my company
he's not the worst guy ever, and he might be going through some stuff in life, or maybe he's just happy. There's no way to know actually.
but fuck him. fuck this fucking guy. fuck him with a thousnd dicks.
this guy defends his postures on the api like this thing was fucking sacred and masterly designed ok?
if I ask him to change one url's method from get to post so that I can send more longer data for the request, he comments "i cant believe they still haven't figured out a get request with a body". I appreciate him caring abkut the correctness.
but this is the same piece of shit that makes NOOO fucking validations on whatever I send to it. I get 500 for fucking EVERYTHING.
And if he does 400, the actual response messages are garbage, the same fucking text with no explanation.
FUCK YOU!!!!!!
I hate the way he structures the names of the url and the parameters, sometimes I have to send arrays of strings, other times arrays of objects, the naming is garbage and INCOSISTENT.
And when we asked him to do the API dotnet core, he was like "nah" FUCK YOU FOR USING SOON TO OBSOLETE TECHNOLOGIES!!!
THIS PIECE OF SHIT IS SLOW, because a coworker did another spi in core and the response times are hugely better.
I wouldnt mind if he was 100% of the time careless, but he actually makes a stand for his ideas, as if he actually gave two shits.
he's actually an ok guy though but... fuck hiim!!!! ive been holding onto this for a while... and I'm sure I have some flaws too.7 -
Tweet: Angular is slow.
Response:
vdom is worse than angular.
Then why not fix the stupid change detection strategy, broken form type/validations, late subscription bugs.
"Angular is for enterprise app".
This sentence means nothing.
Wtf angular community is so toxic
https://twitter.com/mgechev/status/...14 -
Why Apple has to do every configuration so f**ckin difficult? After a thousand logins, validations, and 2FA just to change my f**ckin region I find that I need to contact local support by chat or call even if my account is clean (no payment method added, no purchases made, etc.). Yeah right, great products, but crappy website UX.1
-
When people say they wish a language was more strict. I'm a fan of the loose lol.
Tried Python. Script failed due to indent not at right amount. Bye
C#/.NET... Typecasting out the a$$. Goodbye lol
PHP. Anything goes. Hello, this is my kind of world. Never had an issue with types but I write my code to handle values properly.
And I know people will have opposing views. However I will say that you can still achieve the strictness in PHP by putting in your own checks. Create a few custom functions to do your validations and you are good to go :)3 -
I had a colleague, who built a bunch of smaller systems for the company I'm working in. He didn't want to waste his time building a "perfect" system (which I generally agree with, the question is just where to draw the line).
But because it took him so long to build the prototype, usually it went into production without being hardened (like basic input validations were missing. It wouldn't allow anything malicious, but instead of a validatiom error it'd just 500).
When he left, literally less then a week later, one of his systems, which was a prototype and nobody except him could maintain, because it was done in a fancy new technology, which wasn't even v1 at that time and their documentation said, it's production ready when we release v1. Anyway, that one system started crashing just few days after him leaving. Another Dev and me tried to fix it, but every time we touched it, it just got worse.
At some point, we gave up and just configured a cron job to reboot it every 12h. He could have probably fixed it, but to us it was just black magic.
Anyhow, this rent isn't about him, AFAIK all the systems still working, as long as you provide the correct input. Nor is it about the management decisions, which lead to this Frankenstein service on live support, which we had to increase, to be restarted every 8 hours, 6h, 4h, 3h, .....
It's about the service itself, which I'm looking forward to every day, when the rewrite will be done and I can nuke the whole git repository.
I was even thinking about moving all the related files onto a USB stick and putting that on 🔥, once we're done rewriting it....
Maybe next month or in 2. Hopefully before we'll have to configure the cron job to restart the service every couple minutes.... -
Is Amplify of AWS really no backend Development? Anyone of you has an app in production with this? I want to know, for example, when you need to add SMS validations or 3rd part payments or webhooks, are those features supported?2
-
Today I started a project in which I must parse and extract some features from orders. Features can be product names, options, custom data and more and then do some validations/processing.
The (main) problem ? All I have is a String per order and of course most of the product/options have either change or been deleted.
I want to sudo rm -rf myself 😞 -
Save method returns true. No validations errors. Everything looks good, except that a boolean field that controls the record has been set to false from true...even though it wasn't in the objects changeset!