Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
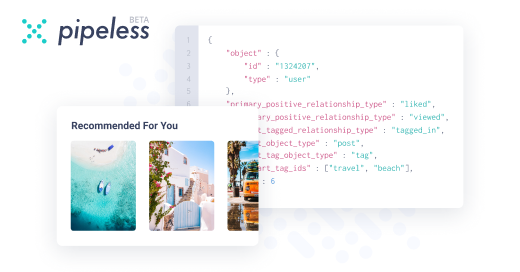
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "globals"
-
In my previous company, I used to work for a client company which had a terrible website. It was about financial data and people would have to wait too long before the page loaded because there was a freaking 1.2 megs of minified, compressed JS file that needed to load before you could do anything.
Everyone knew that was a pain in the ass and nobody wanted to touch spaghetti code and mess up something they didn't know.
I wanted to however take a shot at it. So an architect from client side and I discussed how we were gonna go about it and how we were gonna find the stuff that needed to load on page load and stuff that could be loaded later.
So we plan for it. We broke everything down from a globals polluting JS, found out the variables and functions that needed to run during first load by literally putting a console statement for each function and finally came up with two bundles.
The primary bundle was 120kb and would during first load and then every module would call it's own secondary bundle when the user interacted with it.
In the process, we removed half a meg of JS and the site became blazing fast.
I did it with a team of two members who, my manager thought were useless, learned a ton of stuff, setup proper process for the transition.
When the client didn't appreciate the amount of brain and effort we had put into it, these two members came forward to tell the client to acknowledge my effort and attributed the success of it to me.
I was totally moved. There was so much respect that I didnt care what anybody else thought. I was just so happy to work with those two humans.
When i left the company, i gifted them stuff they always talked about or wanted. :) Feels good.1 -
A sidebar.
Literally just a sidebar.
And yes, this was in Hell.
Its code was spread across at least 40 files, and it used a bunch of freaking global variables to unfurl accordion sections, hide other sections/items, highlight the active item, etc. These were set (and unset!) in controller actions, so if you didn’t unset one, it remained open and highlighted until another action unset it.
Some of the global variable checks (and permissions checks) were done in the individual views, some outside of the `render` statements that include them. Some of them inherited variables from the parent, some from the controller, some from globals. Getting a view to work was trial and error. Oh, and some had their own inline css, some used css classes.
Subsections were separate views, so were some individual items, both sometimes rendered using shared templates, and all of the views and templates had the exact. same. filename. (They were located in different directories, and thus located automagically via implicit relative paths.) So, it was a virtually endless parade of`render partial => “sidebar”`. Which file does that point to? Good luck figuring it out!
Also, comments in several places said adding a new section required a database migration. I never did figure out why.
Anyway, I discovered this because I had an innocuous-sounding ticket to rearrange the sidebar, group some sections/items under different permissions, move some items to another menu, and nest some others differently.
It took me two bloody weeks, and this was when I was extremely productive every day.
Afterward, I was so disgusted by it that I took a day and removed every trace of the sidebar I could find, and rewrote it. I defined the sidebar in a hash, and wrote a simple recursive builder to generate the markup. It supported optional icons, n-level nesting, automatic highlighting of the current item and all parent nodes, compound and inherited permissions, wrapping of long names, hover and unfurl animations, etc. Took me a couple hundred lines of Ruby at the most, plus about the same of css.
Felt so good to remove that blight.5 -
the official Android documentation says it stands for "What a Terrible Failure"...sure it does Google, sure it does....1
-
Okay, story time.
Back during 2016, I decided to do a little experiment to test the viability of multithreading in a JavaScript server stack, and I'm not talking about the Node.js way of queuing I/O on background threads, or about WebWorkers that box and convert your arguments to JSON and back during a simple call across two JS contexts.
I'm talking about JavaScript code running concurrently on all cores. I'm talking about replacing the god-awful single-threaded event loop of ECMAScript – the biggest bottleneck in software history – with an honest-to-god, lock-free thread-pool scheduler that executes JS code in parallel, on all cores.
I'm talking about concurrent access to shared mutable state – a big, rightfully-hated mess when done badly – in JavaScript.
This rant is about the many mistakes I made at the time, specifically the biggest – but not the first – of which: publishing some preliminary results very early on.
Every time I showed my work to a JavaScript developer, I'd get negative feedback. Like, unjustified hatred and immediate denial, or outright rejection of the entire concept. Some were even adamantly trying to discourage me from this project.
So I posted a sarcastic question to the Software Engineering Stack Exchange, which was originally worded differently to reflect my frustration, but was later edited by mods to be more serious.
You can see the responses for yourself here: https://goo.gl/poHKpK
Most of the serious answers were along the lines of "multithreading is hard". The top voted response started with this statement: "1) Multithreading is extremely hard, and unfortunately the way you've presented this idea so far implies you're severely underestimating how hard it is."
While I'll admit that my presentation was initially lacking, I later made an entire page to explain the synchronisation mechanism in place, and you can read more about it here, if you're interested:
http://nexusjs.com/architecture/
But what really shocked me was that I had never understood the mindset that all the naysayers adopted until I read that response.
Because the bottom-line of that entire response is an argument: an argument against change.
The average JavaScript developer doesn't want a multithreaded server platform for JavaScript because it means a change of the status quo.
And this is exactly why I started this project. I wanted a highly performant JavaScript platform for servers that's more suitable for real-time applications like transcoding, video streaming, and machine learning.
Nexus does not and will not hold your hand. It will not repeat Node's mistakes and give you nice ways to shoot yourself in the foot later, like `process.on('uncaughtException', ...)` for a catch-all global error handling solution.
No, an uncaught exception will be dealt with like any other self-respecting language: by not ignoring the problem and pretending it doesn't exist. If you write bad code, your program will crash, and you can't rectify a bug in your code by ignoring its presence entirely and using duct tape to scrape something together.
Back on the topic of multithreading, though. Multithreading is known to be hard, that's true. But how do you deal with a difficult solution? You simplify it and break it down, not just disregard it completely; because multithreading has its great advantages, too.
Like, how about we talk performance?
How about distributed algorithms that don't waste 40% of their computing power on agent communication and pointless overhead (like the serialisation/deserialisation of messages across the execution boundary for every single call)?
How about vertical scaling without forking the entire address space (and thus multiplying your application's memory consumption by the number of cores you wish to use)?
How about utilising logical CPUs to the fullest extent, and allowing them to execute JavaScript? Something that isn't even possible with the current model implemented by Node?
Some will say that the performance gains aren't worth the risk. That the possibility of race conditions and deadlocks aren't worth it.
That's the point of cooperative multithreading. It is a way to smartly work around these issues.
If you use promises, they will execute in parallel, to the best of the scheduler's abilities, and if you chain them then they will run consecutively as planned according to their dependency graph.
If your code doesn't access global variables or shared closure variables, or your promises only deal with their provided inputs without side-effects, then no contention will *ever* occur.
If you only read and never modify globals, no contention will ever occur.
Are you seeing the same trend I'm seeing?
Good JavaScript programming practices miraculously coincide with the best practices of thread-safety.
When someone says we shouldn't use multithreading because it's hard, do you know what I like to say to that?
"To multithread, you need a pair."18 -
My LinkedIn profile: Open to remote opportunities, or those in Hawaii. Need to stay local for family reasons.
Recruiter DMs: "Interested in an opportunity in:
Denver?
Nashville?
Connecticut?
Oklahoma?
Salt Lake?
...
-_-5 -
after an all nighter, I walked in at about 10:30am and unloaded a small bag of groceries on my desk I got to restock the community fridge. Boss walks up, "WTF?!? NO BEER?!?" Slams his credit card on my desk and walks away...1
-
New country, new company, new team, new projects.
I'm supposed to be the TL of a team working on a React project.
A guy in his late 40s celebrates himself as "the senior", he basically just finished watching a youtube thing, React 101 crash course or similar. The other two juniors who did only Wordpress so far venerate him like a god.
The code, of course, is one on the finest pieces of crap I ever had the pleasure to deal with in my life: naturally a bunch of JQuery plugins for everything, no tests, no state management, side effects everywhere, shared state and globals like hell, everything written in ES3/ES5 style, no types, no docs, build and deploy totally manual, deep props drilling at every level... and not to mention the console.log() shipped in prod.
First day, already headache.
Full rewrite start tomorrow.
Hiring real devs as well.4 -
I fucking hate chained methods. Ok, not all of them. Query things like array.where.first... that stuff is ok.
Specially if it's part of the std lib of a lang, which would be probably written by a very competent coder and under scrutiny.
But if you're not that person, chances are you'll produce VASTLY inferior code.
I'm talking about things like:
expect(n).to.be(x).and.not(y)
And the reason I don't like it is because it's all fine and dandy at first.
But once you get to the corner cases, jesus christ, prepare to read some docpages.
You end up reading their entire fucking docs (which are suboptimal sometimes) trying to figure if this fucking dsl can do what you need.
Then you give up and ask in a github issue. And the dev first condescends you and then tells you that the beautiful eden of code he created doesn't let you do what you want.
The corner cases usually involve nesting or some very specific condition, albeit reasonable.
This kind of design is usually present in testing or validation js libraries. And I hate all of those for it.
If you want a modern js testing lib that doesn't suck ass, check avajs. It's as simple as testing should be.
No magic globals, no chaining, zero config. Fuck globals forced by libs.
But my favorite thing about it that is I can put a breakpoint wherever the fuck I want and the debugger stops right fucking there.
Code is basically lines of statements, that's it, and by overusing chaining, by encouraging the grouping of dozens of statements into one, you are preventing me from controlling these statements on MY code.
As an end dev, I only expect complexity increases to come from the problems themselves rather than from needlessly "beautified" apis.
When people create their own shitty dsl, an image comes to my mind of an incoherent rambling man that likes poetry a lot and creates his own martial art, which looks pretty but will get your ass kicked against the most basic styles of fighting.
I fucking hate esoteric code.
Even if I had to execute a list of functions, I'd rather send them in an array instead of being able to chain them because:
a) tree shaking would spare from all the functions i didn't import
b) that's what fucking arrays are for, to contain several things.
This bad style of coding is a result of how low the barrier to code in higher level langs are.
As a language or library gets easier to use you might think that's a positive thing. But at the same time it breeds laziness.
Js has such a low learning curve that it attacts the wrong kind of devs, the lazy, the uninspired, the medium.com reader, the "i just care about my paycheck" ones.
Someone might think that by bashing bad js devs I'm trying to elevate myself.
That'd be extremely stupid. That's like beating a retarded blind man in a game and then saying "look, I'm way better than this retarded blind man".
I'm not on a risky point of view, just take a stroll down npmjs.com. That place is a landfill. Not really npm's fault, in fact their search algorithm is good.
It's just the community.
Every lang has a ratio of competence. Of competent to incompetent devs.
You have the lang devs and most intelligent lib devs at the top. At the bottom you have the bottom.
Well js has a horrible ratio. I wouldn't be shocked to find out that most js devs still consider using import or await the future.
You could say that js improved a lot, that it was way worse beforr. But I hate chaining now, and i hated back then!
On top of this, you have these blog web companies, sucking the "js tutorial" business tit dry, pumping out the most obscenely unprofessional and bar lowering tutorials you can imagine, further capping the average intelligence of most js devs.
And abusing SEO while they're at it, littering the entire web with copy paste content.2 -
Not sure if this technically counts as all nighter, but when Udacity released their basics Android nanodegree they had a contest where the first 100 worldwide to finish got a scholarship for the advanced Android nanodegree. I followed the uberman sleepy schedule, 20 min nap every 4 hours, around the clock for 6 days. Finished #17. They didn't even have the videos or lessons for the last 3 sections, just a description and a project for each, so they set up a slack channel to let us talk with staff and collaborate with others trying to get it done.
-
¯\_(ツ)_/¯
probably the best app ever, I'm going to stop trying now...
https://play.google.com/store/apps/...8 -
Me (out loud in a meeting): "we currently have a SQL Express license, but the traffic with the additional servers is causing crashes when the cache fills up. We need to upgrade to the standard version.”
Follow up email from mngr planning to retire in 6 months currently making roughly 3x my salary: "See if we can get a proposal to purchase better Sequel software and install."
Me:
(-_-;) ... ಠ︵ಠ ...
┻┻︵ヽ(`Д´)ノ︵┻┻1 -
Okay it's FUCKing rant time... FUCK you prestashop!
FUCK your utterly bizarre "coding standard"
Also a big FUCK your config files, since when did config files start to include application logic, multiple includes/requires and modification of super-globals. When did I miss that memo?
This file is full of so much FUCKing horseshit, my FUCKing testicles hurt.
FUCK your "module overrides", yes let's duplicate 20-30MB senseless horror code into another folder, just so we can modify one line, without having future updates breaking our stuff.
And your attempt to migrate to a symphony stucture is FUCKing pathetic, do it properly, or don't do it at all.. FUCKtards..
I know wordpress can be bad, but this...
Prestashop takes FUCKing lousy, headache/cancer- giving, piece of crapware to the next FUCKing level.
I wouldn't even wish this FUCKing upon my worst enemy.2 -
300 global variables.. THREE HUNDRED FUCKING GLOBAL VARIABLES?
Are you for real?
Now let me check the line numbers again..
hmm.. line 97 to .. yep line 410, just a few new lines to seperate some of them or.. group? Idk, I've given up on trying to understand those.
Now you may ask "But ThatPerlDeb, where did you see this and what was the intention?"
Low and behold, take a chair and I may explain this to you.
First of all: Fuck the dev that wrote this!
Second: Fuck all the devs that kept up with this practice or whatever you want to fucking call this!
Now, the application is our POS system that our customers can use for a monthly fee (That this piece of garbage even requires payment is disgusting) but anyway..
The global variables sometimes are declared for labels, sometimes for some frames, sometimes just for random values to be there.
We're using Perl for the POS system and Perl ain't the best at OOP, so in the dev's defense I can understand why you'd use a few global variables, but not fucking 300!! FUCK OFF WITH THIS BULLSHIT!!
So now I'm going through this torture slowly but surely deleting globals and putting them into some sort of scope and always MANUALLY test if something broke. Again, this company sucks ass and there's nothing that could even be considered a "unit test" or something like that, so fuck that, too.
After two hours I've brought down the count of global variables to about 260, so there's progress being made..
But then, there comes more!
"But how???" you may ask, and you're right, I've asked that myself.
Now to resolve the global stuff in each file some of the initial globals are used, we got about 20-30 files which do different stuff, all fair and square, at least there was an attempt at seperating functions but god this mess is so fucking fucked up. So in order to "safely" delete a global variable I have to check if any of the variables are used in another file, and if so, in which scope and how they are used.
Spaghetti would be a compliment for this fucking disgusting piece of utter bullshit.
Let alone the code quality of this "code"
Indendation? Dafuq is dat?
Scope? Nah, we got everything global anyway
Function size? Well, some are 5 lines, some are 900 lines, who cares anyways, right?
I'm so fucking glad once I leave this shithole, for real.6 -
In PHP, constants can only be of simple data types like strings or floats.
You can't make a database connection a constant because it's not a simple data type.
That makes the only way of accessing complex "constants" within functions using the keyword global... which is not encouraged and forces you to make the database connection global (that may not be convenient in some software patterns).
The last option is passing the database connection as a parameter (either to the function or to the constructor of the instance whose methods will use the connection)... which would be good if I didn't want to go full OO. Because it's a pain to do so.
So all in all, constants are not well supported by PHP.
Come on, constants...12 -
Global variables destroyed my day
Of course you can call me a bad programmer and all. When you have the idea, sometimes globals make it seem easy, accessible and "saving resources". They are devils.
The app was connected to a suite of applications. So I ended up silently destroying it neighbors. I committed and pushed the shit. Just when the testers made their weed high smoke tests, a server stopped working.
I got an email that boss wanted the latest version, I reverted the wrong branch, which had code unrelated to its name, pushed shit again and voila.
I went to the bathroom and laughed. I had to take a smoke. I'm still laughing while typing this. The damage is too much and I can't help it. I'll go home, leave this pc on and work remotely through the night. It might be the hysterics speaking now, but I messed up, and I need magic by friday morning.6 -
Got this in my LinkedIn inbox today
"I see you are open to new job opportunity!
I am looking for Sr. Android Developer for a start-up company in San Francisco. They are rebuilding their Android SDK and looking for an Android Engineer like you.
Let me know if you are interested. I would be happy to have a quick chat with you and share more details."
Apparently they're developing a new way of Android development 🤔5 -
There was a big hairy ball of SW mud from another project that a poor coworker had to "reuse". Only that it was impossible because there was no documentation, shit was partly auto-generated with mysterious Excel tables, and the actual code was just as bad. No APIs and nothing, just hacking shit into globals, several nested state machines that were overriding each other's states, and with global side effects. WTF.
Two devs took a look at it - minimum 8 weeks. Schedule was some days, and PM insisted that it was "already working". But the worst thing was that the dev in charge had been looking for another job anyway and quit, so the whole clusterfuck suddenly was on my desk.
The code was so awful that I could only bear it with both eyes closed, so I instead read the spec of this project closely. Turned out that it didn't actually demand this feature, only a small subset of what the ball of mud was supposed to achieve - which I was able to implement from scratch within a day, plus another one for documentation. Phew. -
I learned very quickly that magic globals are baaaaad.
I mean, it's easier than using a queue for passing data between threads, right? -
for the last 6 consecutive Wednesdays, I've gotten some form of an email stating "sorry, venue super swamped, we definite want to find a way to work with you. we're still is talks with other developers, but we really like your knowledge of the project and want to work with you in some capacity". The only stated reason for not going with me in the first place was I told them the project would take 8-12 weeks, but they needed it turned around in 10 weeks max.... you do the math...1
-
I couldn't find a devRant IRC channel so I created one.
Feel free to join: irc.oftc.net #devrant
It should be only me for now but I hope that will change soon :D
P.S. : FUCK ANYONE USING GLOBALS WITH THREE FUCKING LETTERS AAAAAAAAAARGH13 -
So now my whole frigging weekend is shot, gotta rewrite all my projects...
https://github.com/lhartikk/... -
Any Symfony expert here? I've got quite a tricky question, that I'd love to disqus with another dev.
It's about twig within symfony. I'd like to add a custom node parser, but not for an own tag, but to set some value on each template if it's not set (which can't be done with globals in this case!). I've thought about using a visitor, but from my understanding it gets executed to late, as it requires (probably) to modify the twig AST at compile/parse time.4 -
As my first dev job, I took over role of solo programmer maintaining all kinds of custom-made software used by local ISP. It was about 10 years ago.
My first question was where can I find test environment and repo. Apparently there was none and I should learn and develop on production.
My sin was to quickly give up on setting up both test and repo.
My second sin was to continue using the same copy&paste PHTML with register_globals enabled, building over it without attempting to refactor it with templates. I did not use globals in any new code at least.
And I suppose my third sin was that I was playing games when I was done with my tasks. I could have used that time to refactor a bit.
But I think in the end I was absolved from them since I was the only one suffering from this. I stayed with company until it got sold and helped migrate data over (along with myself). -
I backed this Kickstarter project for a programmable E-ink display called "Displio". The documentation is about 40 lines and is basically just a list of globals without any explanation followed by a link to MomentJS docs. worst of all you have to modify the page with an inspector to see it all.
-
what's your preference: QWERTY ,DVORAK, etc...
Personally I love DVORAK, especially on my stock Mac QWERTY keyboard. It's like an extra layer of security. haha -
headphone preferences and why? I'm currently using the AIAIAI TMA-2 (young guru) but I'm always on the lookout for good gear8
-
nothing new, just another rant about php...
php, PHP, Php, whatever is written, wherever is piled, I hate this thing, in every stack.
stuff that works only according how php itself is compiled, globals superglobals and turbo-globals everywhere, == is not transitive, comparisons are non-deterministic, ?: is freaking left associative, utility functions that returns sometimes -1, sometimes null, sometimes are void, each with different style of usage and naming, lowercase/under_score/camelCase/PascalCase, numbers are 32bit on 32bit cpus and 64bit on 64bit cpus, a ton of silent failing stuff that doesn't warn you, references are actually aliases, nothing has a determined type except references, abuse of mega-global static vars and funcs, you can cast to int in a language where int doesn't even exists, 25236 ways to import/require/include for every different subcase, @ operator, :: parsed to T_PAAMAYIM_NEKUDOTAYIM for no reason in stack traces, you don't know who can throw stuff, fatal errors are sometimes catchable according to nobody knows, closed-over vars are passed as functions unless you use &, functions calls that don't match args signature don't fail, classes are not object and you can refer them only by string name, builtin underlying types cannot be wrapped, subclasses can't override parents' private methods, no overload for equality or ordering, -1 is a valid index for array and doesn't fail, funcs are not data nor objects when clojures instead are objects, there's no way to distinguish between a random string and a function 'reference', php.ini, documentation with comments and flame wars on the side, becomes case sensitive/insensitive according to the filesystem when line break instead is determined according to php.ini, it's freaking sloooooow...
enough. i'm tired of this crap.
it's almost weekend! 🍻1 -
Anyone had experience running IDEs on the new pixel books? I'm specifically thinking of using it with Android Studio and JetBrains' Products
I'm specifically thinking of getting the i7/16gb ram/512gb model.1 -
Noob here. I'm an Android guy, looking to create a web app. Looking for pointers on where to get started learning. It's going to be enterprise level. Basically a hub of operations for small companies ~10-30 employees. Handle a lot of private info, see need to be secure.3
-
"Globals? What? I don't do no globals, maaaan"
aham, ok, what's that local dressedup as global there?
"but that's still a local, dude!"
gsus xrist! I'd cry if it wasn't endemic 🤦🏻1 -
I'm no iOS developer, so sorry if this is a stupid question. I was told a particular app I'm looking to make an Android version for was built in objective-C and uses Ruby and AWS for the backend. so presumably I'd need to plug into AWS for my app,im just not sure how Ruby fits in...2
-
Infineon infineon infineon...
Your aurix tricore is amazing for all safet systems... On paper.
Your support is abysmal. Tried forums, support line to verify a demo that only seems to work sometimes.
I just wanted to get ethernet communication using the demo. But hey one week gone and no success....
And the code seems to behave differently for each run :| the debugger works only on global variables and no printf statements. But hey just make a lot of globals right? So little footprint available so not possible :-\
Hoped that some forum could confirm the demo so I knew I was just making a fuck up, but cannot get that verified...
Embedded programming not for me... :/ -
So I have a huge family, and a core group of close friends, but I'm the only semi-tech-literate individual I know. The closest I have is a relative that uses a drag and drop interface to control some "internet of things" style systems in his 9 to 5. DevRant is great, and reddit can be, any place/platform/bar/dogpark you all use to meet other devs/engineers/algorithm junkies? For context, I'm a remote dev for a small team, purty much solo...