Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
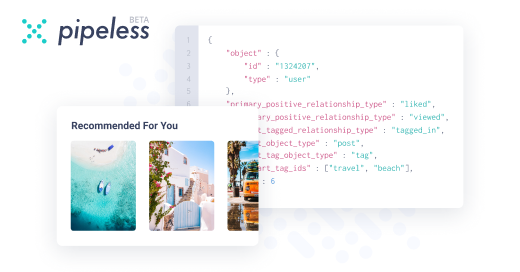
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "object key"
-
Let the student use their own laptops. Even buy them one instead of having computers on site that no one uses for coding but only for some multiple choice tests and to browse Facebook.
Teach them 10 finger typing. (Don't be too strict and allow for personal preferences.)
Teach them text navigation and editing shortcuts. They should be able to scroll per page, jump to the beginning or end of the line or jump word by word. (I am not talking vi bindings or emacs magic.) And no, key repeat is an antifeature.
Teach them VCS before their first group assignment. Let's be honest, VCS means git nowadays. Yet teach them git != GitHub.
Teach git through the command line. They are allowed to use a gui once they aren't afraid to resolve a merge conflict or to rebase their feature branch against master. Just committing and pushing is not enough.
Teach them test-driven development ASAP. You can even give them assignments with a codebase of failing tests and their job is to make them pass in the beginning. Later require them to write tests themselves.
Don't teach the language, teach concepts. (No, if else and for loops aren't concepts you god-damn amateur! That's just syntax!)
When teaching object oriented programming, I'd smack you if do inane examples with vehicles, cars, bikes and a Mercedes Benz. Or animal, cat and dog for that matter. (I came from a self-taught imperative background. Those examples obfuscate more than they help.) Also, inheritance is overrated in oop teachings.
Functional programming concepts should be taught earlier as its concepts of avoiding side effects and pure functions can benefit even oop code bases. (Also great way to introduce testing, as pure functions take certain inputs and produce one output.)
Focus on one language in the beginning, it need not be Java, but don't confuse students with Java, Python and Ruby in their first year. (Bonus point if the language supports both oop and functional programming.)
And for the love of gawd: let them have a strictly typed language. Why would you teach with JavaScript!?
Use industry standards. Notepad, atom and eclipse might be open source and free; yet JetBrains community editions still best them.
For grades, don't your dare demand for them to write code on paper. (Pseudocode is fine.)
Don't let your students play compiler in their heads. It's not their job to know exactly what exception will be thrown by your contrived example. That's the compilers job to complain about. Rather teach them how to find solutions to these errors.
Teach them advanced google searches.
Teach them how to write a issue for a library on GitHub and similar sites.
Teach them how to ask a good stackoverflow question :>6 -
Today I was wandering through the code of the project I work on. The project is on JS, turns out someone named an object key `undefined`. They had the wonderful idea since they didn't want to pass a key through on the side where the info stored in the object is used(or at least that's what I assume).
Look at the image. (not the actual implementation but you get it and it actually works if you want to try it)
👏🏼 I don't know who did it and I rather not git blame it, but pray to the 7 gods you never do something like that again while I'm around or you are going to be in need of prosthetic fingers to code again motherfucker.16 -
I hate access. I wish I could use literally any other program. But they're not authorized.
I'm the only one working on this, and everything is closed. How the fuck is the object in use somewhere else? And where the fuck did my primary key go???7 -
There's this junior I've been training. We gave him a bigger task than we usually do
"How do I link an object in table X with the corresponding object in table Y?"
"How are objects in two tables usually linked? How did you link Y with Z in the first place?"
"Em... Foreign Keys?"
"Yup"
"But there's not foreign key from X to Y."
"Well, create one. You've got full creative freedom over this task."
I sometimes feel like Juniors are either completely careless about past code or overly carefuly with not editing any past code. Frustrating but adorable2 -
PHP arrays.
The built-in array is also an hashmap. Actually, it's always a hashmap, but you can append to it without specifying indexes and PHP will use consecutive integers. Its performance characteristics? Who knows. Oh, and only strings, ints and null are valid keys.
What's the iteration order for arrays if you use them as hashmaps (string keys)? Well, they have their internal order. So it's actually an ordered hashmap that's being called an array. And you can produce an array which has only integer keys starting with 0, but with non-sequential internal (iteration) order.
This array weirdness has some non-trivial implications. `json_encode` (serializes argument to JSON) assumes an array corresponds to a JSON array if its keys are consecutive integers in increasing order starting with 0, otherwise the array becomes a JSON object. `array_filter` (filters arrays/hashmaps using callback predicate) preserves keys, so it will punch holes in the int key sequence if non-last items are removed, thus turning arrays into hashmaps and changing your JSON structure if you forget to discard keys before serialization.
You may wonder how JSON deserialization works, then? There's a special class for deserialized JSON objects, `stdClass`. It's basically a hashmap too, but it's an object, not an array, and all functions that would normally accept arrays won't work with it. So basically its only use is JSON (de)serialization. You can even cast arrays to objects, producing `stdClass`.
Bonus PHP trivia:
Many functions return nonsensical values. `preg_match`, the regex matching function, returns 1 for success, 0 for no matches and false for malformed regular expression. PHP supports exceptions, so it could just throw one on errors. It would even make more sense to return true, false and null for these three cases. But no, 1, 0 and false. And actual matches are returned by output arg.
`array_walk_recursive`, a function supposed to recursively apply callback to each element of an array. That's what docs say. It actually applies it to leafs only. It will also silently accept object instead of array and "walk" it, but without recursing into deeper objects.
Runtime type enforcing is supported for function arguments and returned values. You can use scalar types, classes, array, null and a few special keywords. There's also a `mixed` keyword, which is used in docs and means "anything". It's syntactically valid, the parser will accept it, but it matches no values in runtime. Calling such function will always cause a runtime error.
Strings can be indexed with negative integers. Arrays can't.
ReflectionClass::newInstanceWithoutConstructor: "Creates a new class instance without invoking the constructor". This one needs no commentary.
`array_map` is pretty self-explanatory if you call it with a callback and an array. Or if you provide more arrays of equal length via varargs, callback will be called with more arguments, one from each array. Makes sense so far. Now, you can also call `array_map` with null instead of callback. In that case it treats provided arrays as rows of a matrix and returns that matrix, transposed.5 -
So I need to "fix" a false-positive security warning (mass-assignment of a foreign key). Do I "fix" it by...
A) Setting it manually and double-saving the object?
B) Rewriting the mass-assignment so the linter doesn't realize what I'm doing?
Both options suck.
But security is going to complain if I don't do it.
Guess what?
I'm not doing it.
SMD you ducks.10 -
I just read a rant about Java by a guy who moved from JS/python.
FFS, until now you were using some aberration of OOP by creating "objects" and making them however you want instead of sticking to concept of blueprinting and managing your classes in a way shit happens properly.
Yes, I do hate js/python-like langs if I would have to do something big in them, because I need to constraint my program so it works predictably every time. I don't feel like I'm doing that by putting some arbitrary key-value pairs into some random object :v
Java and her sexier sister Kotlin ftw and nothing gonna change my mind <315 -
A typical bouba coder:
- thinks a kilobyte contains 1024 bytes
- thinks Object.assign clones an object
- codes in react.js, thinks he knows reactive programming
- “amd is better for games, intel is better for work”
- thinks that the main advantage of ssh is that you don’t need to enter your password manually
- watches porn in incognito mode
- “crapple”
- “uhm, is it immutable?”
- thinks “persistent” means saved to local storage
- thinks designer is an inferior job because “they only draw shapes”
- thinks good accessibility is when the tab key works
- “All non-mechanical keyboards are trash”
- “C is outdated and nobody uses it anymore”
- “Zuck quit uni and now he’s a billionaire, everybody should quit”
- thinks “pointer” is a shape of the cursor53 -
What is starting out progaming without explaining to your parents why you making an object move when you press a key, is not only impressive but also took you 15 hours to learn?4
-
"What is your project status? I need an update!"
So spoke the team leader. I was mildly hesitant about just rudely leaving work - which I was about to do, I was literally halfway out the building - without answering and delaying to Monday.
My project leader - the one that is supposed to look after me and my project every now and then - is on vacation. He's also the one, who is supposed to update the team leader.
It's not that we don't talk regularly, apart from his questions every two months or so, he is informed about my project on a bi-to-tri-weekly basis whether he wants it or not.
The team leader on the other hand seemed quite uninformed about my project.
Wouldn't it be nice if people would fucking talk to each other?!
Other than that, I'm learning C# for roughly six months now and today was the day it all started making sense. OO is a concept that is hard for me to grasp - I understand it, but I continuously failed to implement it. (That I have no regular code review right now isn't helping.)
Today, it just clicked.
I feel kind of stupid and in awe at the same time right now. :D2 -
Fuck Unity.
Every single time I try to use Unity to develop my well-along-in-development video game, it finds some way of fucking itself up.
Be it from somehow failing to compile a DLL - which is something completely out of my control, the inspector failing to update itself when I select a new object every five minutes, to the engine managing to fail to load its UI layout because it somehow managed to lose a file responsible for containing the layout, the Inspector forgetting to include a scrollbar and as such trying to cram a bunch of components into one area, crashing in a certain area because I tried using reflections, crashing because I tried running the game in a place that always works, all the way to the whole thing closing instantaneously when I try selecting a new layout.
My experience with using this god-forsaken configuration of code and imagery has been one of endless torment; I've spent hours lamenting about the pain this piece of utter horseshit has caused me to those who'd listen.
I don't know what I did to this thing to deserve to be shown the absolute worst of this engine for the year I've been working on my game for. I can't even take a look at its source code to see if I can piece together things I'll pick up from alien code to fix obnoxious bugs myself because you cunts have it under lock-and-key for some dumbass reason.
Even updating my install of this engine is a gamble; I remember clear-as-day updating my project from 2019.3.14 to whichever one was most recent at the time, and everything breaking. This time, I got lucky and managed to update to 2020.1.4 with no issue on the surface, except I inadvertently let in a host of other issues that somehow made the editor worse than the older one.
There's little point in even bothering to report a bug because this shit happens so randomly that I could be just working on auto-pilot and the next thing I know Unity's stupid "crash handler" rears its ugly head yet again, or you people are probably too busy adding support for platforms no sane person uses like fucking Chromebooks.
There've been times where it's crashed upwards of three times in the span of 40 minutes of light use.
How is one expected to cough up hundreds of dollars a year to use a "pro" version of this horrid editor when every session of use yields a 50/50 chance that it'll either work like it's supposed to, or break in one way or another?
It's a miracle I even managed to type all of this out in one go, I expected the website to just stop responding entirely once I got past four lines.
Do what you will with my post, I don't care.6 -
Trying to work with an API that has no response object (it just returns empty arrays if something breaks) and it's JSON is not key-value.. just a bunch of dynamic nested values. just wow.3
-
Reverse engineering an applications internal object model and creating an database model for it...
The reason: Several versions of application exist, each deliver flat data by rest. The data is a complete potpourri of several different entities. *yaaaay*
Eg. an example fictional call (real call and data would get me in trouble I think....)
get_fiscal_report returning the fiscal data for _several_ companies, the companies _subsidiaries_ and the respective _segments_ for a _year_ with a key value enumeration.
So it's an happy fuck up of N:N associative data that usually would be a hierarchical relationship...
Year - Company
Each Company has subsidiaries
Each Company subsidiary has segments
Each segment has a fixed enumeration of keys
Each key has then the monetary value (e.g. 'operating_income' - 155_000 US-$)
Example is made up, but my data contains exactly such a lovely nested hierarchical data flattened and misnamed to a point where it's close to garbage.
Yaaaay.
I had now 6 days of untucking this mess to a usable database representation...
Sprinkling Unique Keys everywhere...
Running persist script...
Getting exceptions...
Changing associations...
Running persist script...
Screaming.
Changing associations...
Violently cursing.
Running persist script.
Starting sacrificing interns...
6 days.
I need a new brain and a format of my soul.
-.-
Reverse engineering proprietary software is really an morbid adventure.1 -
Recently, I had to make a minor modification to some Node.js code a coworker wrote a year ago which buffers stringified JSON into Kinesis. I just needed to add a new key to the input object, it took minutes to make the change, but hours to make sense out the absolute trash spaghetti code this guy wrote. After spending half a day trying to make his code readable, I just got so pissed off. I replaced his 15 files/+1,500 lines of uncommented code, filled with classes, factory functions, poorly named functions and vars, and so, so many spelling mistakes.
We now have a single, well commented, 300 line file that does the same thing.
Get that shit code out of here. -
For hours I spent my time debugging my code, trying different approach to the same code function. Looking for one simple invisible mistake, that is when I want to make a delete request to the IndexedDB.
The request are fine without running a single error, the success event fires perfectly. But one thing which is unexpected, the object inside IndexedDB did not vanish at all. The data stay the same without any flaws (but how can that be, when the 'delete success' event fired? IT SHOULD BE GONE BY NOW!). No kidding, for hours I debug my code, yet found nothing's wrong!
Until one moment I found out the datatype of key I gave the request are different from the object I wanted to delete, the object has a key of 4 and I gave the request "4". I'm so pissed at this moment making me googled 'developer rant' and found this site.
Really! God Bless 1 !== '1'.5 -
So about two months ago in my consulting firm I was asked to replace a colleague on a project (node and Angular). The project is only a few months old but it’s already a total clusterfuck. DB is very poorly designed. It’s supposed to be a relational database but there’s not a trace of a foreign key or any key for that matter and I’ve seen joins like tableA.name = tableB.description (seriously, that’s your relation??). The code is a mess with entire blocks of code copied from another project and many parts of the code aren’t even used. He didn’t even bother renaming variables so they would make sense in the context they were shamelessly thrown into. The code is at best poorly typed if not typed at all.
During our dailies I sometimes express my frustration with my other colleagues as I very politely allude to my predecessor’s code as being hard to work with. (They are all “good friends" with him). I always get the same response from my colleagues: "yeah but you’ve gotta understand Billybob was under a lot of pressure. The user stories were not well defined. He didn’t have time to do a proper job". That type of response just makes me boil inside.
Because you think I have time to deal with this shit? You don’t think I’m working with the same client and his user stories that are barely intelligible? How long does it take to write type definitions for parameters going into a function? That’s right, 30 seconds at most? Maybe a minute if it’s a more elaborate object? How much time do you think you’ll save yourself with a properly typed function or better yet an interface? Hard to tell but certainly A LOT MORE than those 30 seconds you lost (no, the 30 seconds you INVESTED) in writing that interface!!!
FUCK people with their excuses! Never tell me you don’t have time to do a proper job! You’ve wasted HOURS of my time just because you were too fucking lazy to type your functions, too lazy to put just a little more thought into designing your tables, too lazy to rename a variable so that it’s name actually makes sense where it’s being used. It’s not because you were short on time. You’re just lazy!
FUCK!!!!!!3 -
Aren't you, software engineer, ashamed of being employed by Apple? How can you work for a company that lives and shit on the heads of millions of fellow developers like a giant tech leech?
Assuming you can find a sounding excuse for yourself, pretending its market's fault and not your shitty greed that lets you work for a company with incredibly malicious product, sales, marketing and support policies, how can you not feel your coders-pride being melted under BILLIONS of complains for whatever shitty product you have delivered for them?
Be it a web service that runs on 1980 servers with still the same stack (cough cough itunesconnect, membercenter, bug tracker, etc etc etc etc) incompatible with vast majority of modern browsers around (google at least sticks a "beta" close to it for a few years, it could work for a few decades for you);
be it your historical incapacity to build web UI;
be it the complete lack of any resemblance of valid documentation and lets not even mention manuals (oh you say that the "status" variable is "the status of the object"? no shit sherlock, thank you and no, a wwdc video is not a manual, i don't wanna hear 3 hours of bullshit to know that stupid workaround to a stupid uikit api you designed) for any API you have developed;
be it the predatory tactics on smaller companies (yeah its capitalism baby, whatever) and bending 90 degrees with giants like Amazon;
be it the closeness (christ, even your bugtracker is closed and we had to come up with openradar to share problems that you would anyway ignore for decades);
be it a desktop ui api that is so old and unmaintained and so shitty, but so shitty, that you made that cancer of electron a de facto standard for mainstream software on macos;
be it a IDE that i am disgusted to even name, xcrap, that has literally millions of complains for the same millions of issues you dont even care to answer to or even less try to justify;
be it that you dont disclose your long term plans and then pretend us to production-test and workaround-fix your shitty non-production ready useless new OS features;
be it that a nervous breakdown on a stupid little guy on the other side of the planet that happens to have paid to you dozens of thousands of euros (in mandatory licences and hardware) to actually let you take an indecent cut out of his revenues cos there is no other choice in a monopoly regime, matter zero to you;
Assuming all of these and much more:
How can you sleep at night with all the screams of the devs you are exploiting whispering in you mind? Are all the money your earn worth?
** As someone already told you elsewhere, HAVE SOME FUCKING PRIDE, shitty people AND WRITE THE FUCKING DOCS AND FIX THE FUCKING BUGS you lazy motherfuckers, your are paid more than 99.99% of people on earth, move your fucking greasy little fingers on that fucking keyboard. **
PT2: why the fuck did you remove the ESC key from your shitty keyboards you fuckshits? is it cos autocomplete is slower than me searching the correct name of a function on stackoverflow and hence ESC key is useless? at least your hardware colleagues had the decency of admitting their error and rolling back some of the uncountable "questionable "hardware design choices (cough cough ...magic mouse... cough golden charging cables not compatible with your own devices.. cough )?12 -
What retarded way of writing a json feed is this? what the fuck!
Each product in the feed is translatable, but instead of creating a new object in the feed with the translated content, there's an additional field pointing to a language code (this applies to all translateable fields).
Then each translatable field is appended the number which matches the number appended to the language field containing the desired language code.
In addition to that, the keys doesn't have any sensible meaning, but appearently they abide by som obscure "GS1 / GDSN" standard.
So for each "distinct" field I have to look up the definition of that key
If just the language code and the actual value keys was grouped together.. but no, the feed is ordered by the number after the 'D'.
Yeah for being stuck with something that looks like infinite scrolling, because one product object is fucking gigantic.
FUCKING INSANE MANIAC PIECE OF TRASH.
@#%!#€&7 -
I never liked YAML. But lately, I'm starting to dislike it more and more.
I mean, wtf is that?
- digest YAML input -- a valid YAML
- digest JSON input -- a valid YAML
A language that embeds another language.
Can it be any more confusing..?
Sure it can. the
```
script:
- echo "John said: hello there"
```
will fail YAML linter, because, even though I used quotes, yaml sees `echo "John said` as an object key
I think I'm yet to find more nonsense with YAML. And eventually, I'll grow to hate it.8 -
The joy when tools do not have machine parseable output.
I'm looking at you SBT. My favorite pile of poo.
Remove the logging level from each line, then trim the line, then stab around inside the line with regexes, fishing for a possible match which hopefully is right...
Then stripping scala information like the object type, cause yeah...
A line can be for example "[info] Vector(File(...),File(...))" where info is the log level, Vector the wrapping sequence type, File(...) the wrapping element type and the string inside File(...) what yours truly needs.
As this is lot of shitty shabby string stabby stabby, we need to add a fuckton of boiler plate validation cause who knows what we just murdered.
To make it even more fucked up, a multi project project can produce different output for the same key.
:-)
Yeah. So we need to fix that too.
By the way, one can set log output to unbuffered in SBT.
Then the output is in random order :-)
Isn't that fun? Come on, you wanna poke that pile of shit, too.
The SBT plugin way is by the way no alternative, as I need a full Java environment for execution.
Which brings me to the last point:
For fucks sake, writing CLI applications in Java is so much bloody boilerplate code.
There's ugly and then there's the "please kill me" kind of level.
50 lines just to write a basic validation of argc / argv with commons cli.
That's 6 lines in python. Not kidding. :(
I currently hate everything.
Moments where the job sucks: When you have to hotwire two electric cables with high currency by giving both cables the blowjob of your life.3 -
Some Java code I looked through to figure out how to accurately rework a mapping of value intervals to status colors:
• 16 levels of indentation
• Calls an instance method one line before a null-check
• Assigns that same value to a new variable and null-checks it again
• Insistently loops over existing HashMaps' entry sets to find a value by key
• Stringifies a Gson object, parses back the string and then null-checks the result.
• Mixes up the 'leq' and 'geq' comparison operators twice, which is why I went to check the implementation in the first place.
And this wasn't even legacy code. It's from last year.1 -
"What do you mean I can't use a regex object as the key in a hashmap??
...ok, you know, now that I actually said it out loud"3 -
Flutter help please.
When creating a new website, code has the following:
MyHomePage({Key key, this.title}) : super(key: key);
What does {} content mean? Is it like Javascript, that there is an object passed and we take only those two params?
Because this is how they use it:
MyHomePage(title: 'Flutter Demo Home Page'),
I'm not sure what is the "key" thing7 -
It started when i was about 10 old.
My uncle showed me how to display something in dos-prompt using the echo command in a custom batch-file.
A few commands later, i was able to "program" a flip-book of an ascii ski-driver. Each ascii picture was separated by pressing any key and cls ^^
Aaaaah. Sweet childhood memories!
Later on i used a programming-language for beginners in windows.
This language gave you control of a triangle called "turtle".
My first high-level programming language was Delphi.
Since i had no idea of databases, i created a pseudo database of magic the gathering play-cards. Each card had it's very own windows formular filled up completely with an uncompressed image object displaying the chosen card modally. *sigh*
I scanned each card by using a feed scanner.
Finally, my application consisted of 200 cardimages and forced my PC to swap the required memory from my harddisk.
Boy o boy. I was such a noob! ^^
Over the years i discovered and felt in love with a lot of languages (jsp, java (script), c#, php, ...) and concepts (mvvm, mvc, clean-architecture, tdd, ...)! ;) -
Some interesting keyboard shortcuts that are lesser-known but can be quite useful:
1.Windows Key + . (Period): In Windows 10 and later versions, this shortcut opens the emoji panel, allowing you to quickly insert emojis into your text.
2.Ctrl + Shift + T: This shortcut reopens the last closed tab in most web browsers (Chrome, Firefox, Edge). It's handy if you accidentally close a tab and want to retrieve it quickly.
3.Ctrl + Backtick (`): In some text editors and IDEs (like Visual Studio Code), this shortcut toggles the integrated terminal window, allowing you to quickly switch between editing and running commands.
4.Ctrl + Shift + Esc: This directly opens the Task Manager in Windows, skipping the intermediary step of opening Ctrl + Alt + Delete and selecting Task Manager.
5.Alt + Drag: In many graphics and design applications (like Photoshop), holding down the Alt key while dragging an object duplicates it. This can save time compared to copying and pasting.
6.Ctrl + Alt + D: This shortcut shows the desktop on Windows, minimizing all open windows to quickly access icons and shortcuts on your desktop.
7.Ctrl + Shift + N: In most web browsers, this shortcut opens a new incognito or private browsing window, useful for browsing without saving history or cookies.
8.Alt + Enter: In Excel, this shortcut opens the Format Cells dialog box for the selected cell or range, allowing quick formatting changes without navigating through menus.
9.Shift + F10: This shortcut performs a right-click action on the selected item or text, useful when you can't or don't want to use the mouse.
10.Ctrl + Shift + V: In many applications, including Google Chrome and Microsoft Word, this shortcut pastes text without formatting (paste as plain text). It's useful when copying text from websites or other documents.
++ if you like this6 -
Random thoughts on more out of the box tools/environments.
Subject: Pharo
Some time ago I had shown one of my coworkers about Pharo and he quickly got the main idea behind it but mentioned how he didn't like the idea of leaving behind his text editor to deal with source code.
Some time last week I showed the dude some cool 3d animations you can do with Pharo while simultaneously manipulating the code to change them in real time. Now that caught his attention particularly and he decided he wanted to know more about the language but in particular the benefits of fucking around with an image based environment rather than a file based.
Both of us reached the conclusion that image based makes file based dev enviroments seem quaint in comparison, but estimated that it was nothing more than a sentiment rather than a fact.
We then considered what could be the advantage/disadvantages of such environments but I couldn't come up with anything other than the system not having something like Vim or VS Code or whatever which people love, but that it makes up for it with some of the craziest IDE tools I had ever seen. Plugins in this case act like source code repos that you can download and activate into your workflow in what feels something similar to VS Code being extended via plugins written in JS, and since the GUI is maleable as it is(because everything is basically just subsets of morp h windows) then extending functionality becomes so intuitive that its funny
Whereas with Emacs(for example) you have to really grind your gears with Elisp or Vimscript in Vim etc etc, with Pharo your plugin system is basicall you just adding classes that will convert your OS looking IDE into something else.
Because of how light the vm machine is, portability is a non issue, and passing pharo programs arround is not like installing Java in which you need the JVM.
Source code versioning, very important, already integrated into every live environment and can be extended to do pushes through simple key bindings with no hassle.
I dunno, I just feel that the tool is too good to be true. I keep trying to push limits into it but thus far I have found: data visualization and image modeling to work fine, web development with Teapot to be a cakewalk and work fine, therr are even packages for Arduino development.
I think its biggest con would be the image based system, but would really need to look into how this is bad by any reason other than "aww man I want vim!" since apparently some psychos already made Emacs and VS code packages for interfacing with Pharo source trees.
Embedded is certainly out of the question for any real project since its garbage collected and not the most performant cookie in the jar.
For Data science I can see some future, seems just as intuitive and interesting as a Jupyter Notebook actually, but the process can't and will not be the same since I still don't know of a way to save playground snippets unless you literally create classes for it, in which case every model you build gets saved inside of an object, sounds possible but, strange since it is not a the most common workflow in jupyter.
Some of the environment is sometimes glitchy, but it does have continuos development and have not found many hassles.
There is a biased factor from my side: I seem to be wired to understand the syntax and simple object model better than in other languages. To me this feels natural as if I was just writing ideas rather than code, mostly because I feel that there really ain't much in terms of syntax, the language gets out of my way and the IDE feels like the most intuitive environment in the world to me. I can see why some people would find it REALLY weird of counterintuitive tho.
Guess I really am a simple dude. -
JS has
dynamic object literal keys
String object literal keys
Why aren't template literal keys allowed, and _why_ isn't there a proper error message for them?7 -
// Pouring over idiot API developer's crappy documentation.
Example:
Goal Detail
* From Docs "table_breakdown key will return an array but will always only contain one json object.
json -> "table_breakdown" [
{
"field" : "value",
"etc" : "etc"
}
]
WHYYYY!!!!1 -
I just like bulding silly things, my ideal devjob would be one where I could just make random junk that makes me smile all day...
Like recently I made an NoSQL database using azure AD. They give you 50000 AD objects free, but I found you could encode all sorts of data in the AD objects variables. So basically I setup a framework that uses Security groups as Collections, AD objects as Documents, and object variables as key pairs.
It's really slow, like roughly 50 queries a minute, but hey. It was fun proving it could be done...
Yeah, that would be my ideal devjob :P that kind of stuff all day2 -
I'm going insane.
So let's say you have an object in database, with 20-30 related objects (Or lists of objects) (All related objects have a foregn key to the "main" object).
Now, as long as you just edit/create thinga everything is fine.
But the deletion... Oh MY GOD
"Just put on delete cascade", right ? RIGHT ?
WRONG ! presence of some objects should block delete, while others can be deleted and some are "situational" depending on the first object state.
So delete operation with all the checks takes .... 1 - 2 seconds.
Seems fine ? WRONG ! When you have 40 or more objects to delete, even 1 second is too long.
Should I say "fuck it" and just write a stored proc which will crash if object cannot be deleted for any reason ? because with Entity Framework, I don't see how I can do it effitenly.
But I HATE stored proc, after couple of month/years noone remembers how they work...
RHAAAAA.
Ok I feel better.8 -
Today, after 1.8 years since the start of the project, the PM decided that the primary key of the object shouldn't be using the 'IMPL' prefix and must be also changed in historical data... Why? 'I don't like it' was all that he said in the email.
I like my job :)1 -
Me: GUI team needs help from backend team to add UIDs to a (shitty) object in (their in-house shitty) db.
Backend:
Backend can display these (shitty) objects properly, why can't GUI use the entire object as composite primary key? Backend can't just add some random UID to fix GUI display bug.1