Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
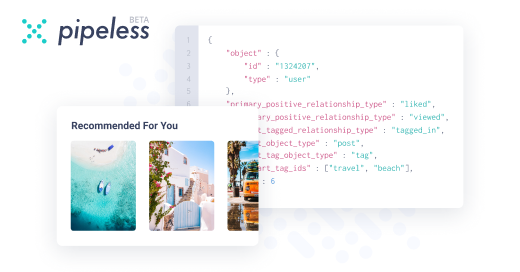
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "string is not number"
-
I had to open the desktop app to write this because I could never write a rant this long on the app.
This will be a well-informed rebuttal to the "arrays start at 1 in Lua" complaint. If you have ever said or thought that, I guarantee you will learn a lot from this rant and probably enjoy it quite a bit as well.
Just a tiny bit of background information on me: I have a very intimate understanding of Lua and its c API. I have used this language for years and love it dearly.
[START RANT]
"arrays start at 1 in Lua" is factually incorrect because Lua does not have arrays. From their documentation, section 11.1 ("Arrays"), "We implement arrays in Lua simply by indexing tables with integers."
From chapter 2 of the Lua docs, we know there are only 8 types of data in Lua: nil, boolean, number, string, userdata, function, thread, and table
The only unfamiliar thing here might be userdata. "A userdatum offers a raw memory area with no predefined operations in Lua" (section 26.1). Essentially, it's for the API to interact with Lua scripts. The point is, this isn't a fancy term for array.
The misinformation comes from the table type. Let's first explore, at a low level, what an array is. An array, in programming, is a collection of data items all in a line in memory (The OS may not actually put them in a line, but they act as if they are). In most syntaxes, you access an array element similar to:
array[index]
Let's look at c, so we have some solid reference. "array" would be the name of the array, but what it really does is keep track of the starting location in memory of the array. Memory in computers acts like a number. In a very basic sense, the first sector of your RAM is memory location (referred to as an address) 0. "array" would be, for example, address 543745. This is where your data starts. Arrays can only be made up of one type, this is so that each element in that array is EXACTLY the same size. So, this is how indexing an array works. If you know where your array starts, and you know how large each element is, you can find the 6th element by starting at the start of they array and adding 6 times the size of the data in that array.
Tables are incredibly different. The elements of a table are NOT in a line in memory; they're all over the place depending on when you created them (and a lot of other things). Therefore, an array-style index is useless, because you cannot apply the above formula. In the case of a table, you need to perform a lookup: search through all of the elements in the table to find the right one. In Lua, you can do:
a = {1, 5, 9};
a["hello_world"] = "whatever";
a is a table with the length of 4 (the 4th element is "hello_world" with value "whatever"), but a[4] is nil because even though there are 4 items in the table, it looks for something "named" 4, not the 4th element of the table.
This is the difference between indexing and lookups. But you may say,
"Algo! If I do this:
a = {"first", "second", "third"};
print(a[1]);
...then "first" appears in my console!"
Yes, that's correct, in terms of computer science. Lua, because it is a nice language, makes keys in tables optional by automatically giving them an integer value key. This starts at 1. Why? Lets look at that formula for arrays again:
Given array "arr", size of data type "sz", and index "i", find the desired element ("el"):
el = arr + (sz * i)
This NEEDS to start at 0 and not 1 because otherwise, "sz" would always be added to the start address of the array and the first element would ALWAYS be skipped. But in tables, this is not the case, because tables do not have a defined data type size, and this formula is never used. This is why actual arrays are incredibly performant no matter the size, and the larger a table gets, the slower it is.
That felt good to get off my chest. Yes, Lua could start the auto-key at 0, but that might confuse people into thinking tables are arrays... well, I guess there's no avoiding that either way.13 -
Coworker: I give up! Please help me!
Me: What's up?
C: Take a look at this. I have this function here that gets the tab index and I'm passing it to the Tabs component over there. I'm logging the index and as you can see it's 3, but the Tabs component isn't working. However if I replace the function call with a 3 it works!
Coworker 2: While you were explaining all that, shellbug already thought about at least 3 reasons why that isn't working.
Me: **sighs** Of what type is the value that function is returning?
C: **stares at me for a few seconds** It's a number.
Me: Are you sure?
C: Well, it's returning 3.
Me: Please do a typeof.
It was string.8 -
My Interview question was simple, just :
Create an algorithm using **JAVA** accepting row input which output corresponding diagram :
1.
*
**
***
****
2.
*
**
***
****
3.
54321
4321
321
21
1
I said, well this going to be easy. Turns out they give me one sheet of FUCKING PAPER. ARE YOU OUT OF YOUR MIND? THIS IS JAVA NOT A FUCKING PYTHON.
But in the end i complete the test except i don't write the :
public stupid static motherfucking main(String[] dick){}
in every single number. Got Zero in the test. Didn't get the job.
I win.13 -
Besides the fact that there would be an error handler, wouldn't it store a phone number as an object other than a computable number, like a string, cuz phone number is like a handler, a reference, and not something you'd ever perform arithmetic on?6
-
!rant
A rather long(it's 8 hrs long to be precise) story
So I just finished an amazing homework assignment. The goal was to open a new shell on Linux using a C program. We were asked to follow instructions from http://phrack.org/issues/49/14.html . However the instructions given were for 32 bit processors and we had to do same for 64 bit machines. In a nutshell we had to write a 64 bit shell code and use buffer-overflow technique to change the return address if the function to our shell code.
I was able to write my own shellcode within 1hr and was able to confirm that it's working by compiling with nasm and all. Also the "show-off-dev" inside me told me to execute "/bin/bash" instead of "/bin/sh"(which everyone else was going to do). After my assembly code was properly executing shellcode, I was excited to put it in my C code.
For that, I needed opcodes of assembly code in a string. Following again the "show-off-dev" inside me, I wrote a shell script which would extract the exact opcodes out of objdump output. After this I put it in my C code, call my friend and tell him that "hell yeah bro, I did it. Pretty sure sir is gonna give me full marks etc etc etc". I compiled the code and BOOM, IT SEGFAULTS RIGHT IN FRONT OF MY FRIEND. Worst, friend had copied a "/bin/sh" code from shellstorm and already had it working.
Really burned my ego, I sat continuously for 8 hrs in front of my laptop and didn't talk to anyone. I was continuously debugging the code for 8 hrs. Just a few minutes ago, I noticed that the shellcode which I'm actually putting in my C code is actually 2 bytes shorter than actual code length. WHAT THE F. I ran objdump manually and copied the opcodes one by one into the string (like a noob) and VOILA ! IT WORKED !!!
TURNS OUT I DIDN'T CUT THE LAST COLUMN OF OPCODES IN MY SHELL SCRIPT. I FIXED THAT AND IT WORKED !!
THE SINGLE SHITTY NUMBER MADE ME STRUGGLE 8 HRS OF MY LIFE !! SMH
Lessons learnt :
1)Never have such an ego that makes you think you're perfect, cuz you're retarded not perfect
2)Examine your scripts properly before using them
3)Never, I repeat NEVER!! brag about your code before compiling and testing it.
That's it!
If you've read this long story, you might as well press the "++" button.6 -
Regex.
I HATE YOU.
There. I've said it.
I spent the better part of the last hour trying to wrangle together, not one, but TWO regexes and getting them to work with Python's "re" module.
The worst part about these little shits? It's how well they work once you figure out how to get them to work. For example, pulling a phone number out of a paragraph is difficult with string methods, but cake with regex . . . if you can figure out the pattern.
But I think I'll always have hate in my heart for Regex just for how obtuse and frustrating they can be.
F**k you regex.19 -
While working on my one of the first project in java i ended up using deprecated Calendar API for the date. Since deadline was near i thought it would be a good idea to use the JCalendar API for as date picker (which is a third party API).BAD IDEA. It was the night before the submission round about 11pm when i realized that there is no way to convert JCal object into Calendar and it turned out it is not working as expected you have to subtract a particular number from the year to get date right.
To convert JCal into Calendar i used the toString function to get the date in string sliced it using substring into year,month,day then had to assign date to Calendar object via constructor.
Had to write 70 lines of code just to convert JCal into Calendar...
And then there were other complications related to this problem. Had tu pull an all nighter just to solve date related problems
LESSONS LEARNED :
NEVER USE A DEPRECATED API
NEVER USE THIRD PARTY APIs WITHOUT RESEARCH7 -
Hi every developer! My name is Allen. English is not my native language so forgive me if I say something that does not make any sense. Let me tell you my story how I become a programmer. (I am still learning) My first computer was a DELL OptiPlex GX 720 desktop. My father bought it for our self-employee job. Before he allow me to use the computer, I used to sit next to him and watching what he do, what he click and what he gets. When he allow me to use the computer, I was slow at typing. One or 2 WPM (word per minute) my father taught me how to use the computer. Very slowly, my typing speed improves. I understand how to use the computer. but one day, I do what make me regret. I was playing with some executables, when I double clicking it, it does not work I used to associate files with apps. I associate music files with every player I want. So, I did what I used to, I associate exe files with windows media center! The computer started to open hundreds of windows media center (WMC for short) whenever an app is clicked, it opens windows media center. Today, I realized that windows were trying to open every app and every process that regularly run. However, since I associate it with WMC, instead of the app itself, it opens WMC some days after the mistake, I wonder how apps work and how I can create my own. My father told me before that a program is simply a binary file that the computer can read. However, it was too advanced to me at the time.I begin my search with google. Everytime I search, it says "learn to code" or something like that. I see some C++ code but, it was disgusting. when I read just a few lines of a hello world code in java. it was too complex
What I seen
#$$#% $%&$%&*#!@
~
(&*%&$ (_(*^% #&&* (^^$(&^$%^( %^*$())
~
^$70^(`*#%`*#&%^)*!" Hello world "#@
~
~
The actual code:
class helloworld
{
public static void main(String args[])
{
System.out.println("Hello World!");
}
}
I look for an easy way but my attempts fail. then. I push
I to learn how to code.I try learning java. but it still
Very complex. i tried LibertyBASIC. from LibertyBASIC to
Java. after learning LibertyBASIC, it was easy!
LibertyBASIC -> Java -> Ruby -> NOW, C# and XAML
Today, I am learning C# and XAML.
My first OS : Windows 7
My first Computer : DELL OptiPlex GX 720
My first successful click : The Start menu
My first used App : Microsoft Encarta 2009
My first created App : Hi-Lo(number-guessing game. written in LibertyBASIC)
Thankyou for reading this Long story.
8 -
Crazy code.
You know when you come across some code, where you think: “I kinda see what you were going for, but it’s still hella dumb though...” right?
Currently doing some work on an F# backend, and the dev clearly knew enough of the language, but their code makes me question a lot.
The problem is simple: use a third party tool to generate images of each page in a pdf.
Said tool supports:
1. Querying the number of pages
2. Getting all pages as separate images in a single invocation
Can you guess how the dev solved it?
They’ve recursively incremented a page number, called the external tool to grab the image of that page.
“But how does it know when it’s run out of pages?” I hear you ask.
Simple. Catch the inevitable exception, and check against a hardcoded string literal to see whether it says “must be before the end of the document”.
I shit you not.
I nearly had an actual seizure when I was debugging some semi-related code and ended up in this wonderland of fuckery.
The recursion and pattern matching was flawless though, yet the tool’s website clearly states the supported functionality.
The whole thing feels like they tried to do it the right way, but couldn’t be bothered / couldn’t get it right, so they ended up creating this insane bit of madness.5 -
"It works on our end", the sentence that made me lose my shit.
I've been working on a project were we're supposed to integrate an API into our system.
When trying to get some user id's (UUID) from said API, we got a type-error in the response (???), so I called their integration support and asked what the fuck they were doing (not really, i was kinda calm at this point).
The answer I got was following:
Integration guy: "Uh, bro, like, I don't even know, it's probably on your end"
Me: "We literally used this endpoint with the same parameters yesterday, and got a result we expected. I noticed you updated your API this morning, did you make any major changes?"
Integration guy: "Yeah we changed the type of user id from string to number"
Me: "So, you changed the type of a UUID (uuid4) from string to number? How did you not think that would be an issue? I can see in your forums that everyone else is having the same issue."
Integration guy: "Nah, it's probably a bug in your code, it works on our end"
Me in my mind: *IT WORKS ON YOUR END?!? IT DOESN'T FUCKING MATTER IF IT WORKS ON YOUR END, FUCKTARD.*
What I actually said: "Uhm, I'm not sure if works on your end either, I'm not even sure how this change made it to production. But hey, thanks I guess, bye."
WHY AM I NOT ABLE TO YELL AT PEOPLE WHEN THEY ARE BEING RETARDED???
But really though, when you're maintaining an API, you shouldn't fucking care if things work on your end in your dev environment. What matters is how it works in production, for the end user/users.
And I know that 99% of cases it's the users fault by entering the wrong parameters or trying to request with wrongly setup auth and what not, but still.
Don't ASSUME nothing's wrong on your end. It's your fucking job to fix the issues.
And guess what? The problem was on their side.
I'm going fucking bald.2 -
I am very frustrated today and I do not know where to "scream" so I will post this here since I believe you will know how I feel.
Here's the case...
I am developing an e-commerce web application where we sell industrial parts. So my boss told me on March that when we are going to show these parts, we should not show Part Number to visitors because they will steal our information.
Ok, this makes sense but there was a problem.
The Primary Key for these products in our internal system is a string which is the Part Number itself.
I told him on March that we have to come up with another unique number for all the products that we are selling, so this unique number will be the primary key, not the Part Number. This will be best because I will be dependent from the original Part Number itself. And in every meeting he said "That is not priority". So I kept developing the part using the original Part Number as primary key and hid is from the web app. (But the Part Number still shows on URL or on search because this is how my boss designed the app.)
I built the app and is on a test server. Until one of out employees asked my boss: "There is no unique number or Part Number. How are the clients going to reference these parts? If a client buys 20 products and one of those has a problem, how is he going to tell us which products has a problem?"
My boss did not know what to say, and later said to me that I was right and primary key was priority.
I really hate when a guy that knows shit from developing does not listen to suggestions given by developers.
FUCK MY LIFE!
I'm sorry if you did not understand anything.5 -
Writing a brainfuck interpreter is a lot fun: Mine does recursion within loops. I also added functions: strings, you can cheat. Stackdump(!), Exit script(*) , go to first cell (^), go to last cell(?), nulling cell (0) It parses this for example: "retoor" ^[.>]. It will dump string retoor. Explanation: the string moves ptr to sixth place. ^ will reset pointer to first. [] is a loop that executes as long there's data in current cell. The "." prints char of current cell (Number if not alpha etc). ">" moves a cell to right. [.>] will thus print until it moved to an empty cell. To move to first, I could've also used my repeater function by adding times to repeat after command: <6 moves six places to left. .>.>.>.>.>.> is also a way to print six chars. +[,.] works as the Linux program "cat". , is one char keyboard input.
Thanks for listening to my tedtalk8 -
I can not fuckin stress how goddamn annoying it is to work with strings in C++. I'm not talking about std::string, those are bearable. But fucking char foo[number], char* foo, and const char* foo. AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA17
-
my fist job... i get to edit a c++ code written by a (mind you) programming company that they teamed with for the past(mind you again) 3 years ...
now just for starters, this code was edited by self taught coders that are really good engineers(they are really good), that didnt really know how the code worked before yet they still changed it, and it worked, how ever they wanted some changes.
i get the project files, and there is not one single comment describing what is happening... only code commented out... and no documentation what so ever were done....
so below are some of my comments that i wrote after i finished adding what i had to add, and fixing what i had to fix:
/*first rule of C anything coding, no actual functions in the header, well let me introduce you to a fully functioning thread running program all in the header, enjoy*/
//used to control the thread
// i honestly dont know why, but it worked soooooo yea...
// TG uncommented // for absolutely no reason what so ever...
//used to communicate with the port
//the message to be sent to the inverter, which has a code that will handle it
//hmmmmmm...
//again not usefull since we are using radioButtons
// same ...
// same ...
// same ...
// they said they dont even use this mode, but none the less, same ...
// calculate the checksum for the message
// ....
// one of the things that work, and god forbids i touch
// used for the status displayed on screen
// used for the (censored :P) status in the message
// used for the (censored :P) status in the message
// not used at all, but the message structure contains it and i refuse to edit that abomination
// used for the (censored :P) status in the message
// used for the (censored :P) status in the message
// just dont ask and roll with it, i didnt want to touch this
// saaaaame ...
// if before true this saaaaaame ...
// value of the (censored :P)
// it pains me to say it again, but this is no use
// (censored :P) input
// (censored :P) input
// only place seen , like for real it was just defined,sooooo yea :D
// well you know how it is
// message string
// check sum string
/****below from feed back****/
// (censored :P) coming in
// (censored :P) coming in
// (censored :P) coming in
// (censored :P)
/****below is the output to the receiver ****/
//(censored :P)
// (censored :P)
// (censored :P)
// (censored :P)
//you thought we were done.... nope, no idea. it comes in the feedback
// not used, literally commented out the one time it was used
// same ...
// XD, man this is a blast, same ...
// nope ...
// used to store the port chosen for the communication
// is a static for the number of data we have recorded so far, and as a row indicator for the recording method
// used to indicate the page we are on in the excel file, as well as the point in physical point in the test
// same ... oh look at this a positive same :D
// same ...
// same ...6 -
Lord. Please deliver us from the cycle of unfinished programming languages and code benches that are designed to create more work for us. We beseech thee in thy mercy to transmute all this asynchronous lead that is found in javascript into a purer form of threading that is sensible and can be willfully blocked or not so in a way that works and does not divide us through our ugly code. May also we be given the ability to purge from our midst all child molesters and string them up by barbed wire off a line of telephone poles across the entire continental usa and may there be a sudden increase in the number of ravens and buzzards to feed on them, being nice birdies that I miss seeing so much. May half their positively identified population be kept alive and delivered unto us that we might remove their scrotum with a hook-ed barb and something resembling a serrated metallic spork, amen.
and please fix fucking node js. i agree that its asynchronous methods suck ass for literally everything as there is no use for it that seems to work given its a shitty emulated single thread.2 -
Tldr: I failed a test and was sad about jt
So a while ago I had a python exam for my study, nothing special like a certification or anything, just the basics. We are not allowed to use internet because they want to prevent people from communicating from one another. Usually im fine with this rule, but this time it screwed me over so much.
The exam is setup in 3 main assignments each of which has 5 subassignments. Hence, if you cant do subassignment 1, you fail the entire main assignment and lose 33% of your grade. I completely blacked out during the exam and couldnt remember how to simply get a number from a string interpreted as an int and forgot how to work with json. Because we weren't allowed to use internet I wasn't able to figure this out and have now failed the test.
I'm so sad and mad at myself for not acing such an easy test and for a day I felt unworthy of being a programmer. Thank God I got over that and have a resit somewhere next week.2 -
So first rant, here goes weirdness, and also lengthy rant
So in my company we have the hr and accounting managed by the same person which also deals with all things employee related and she had a need for a way to extract a birthday from, what is in our country the personal identification number, things go great i get a formula that performs parts of the magic up to the point where the first digit of the number dictates the gender and century to be used when forming the full year, mind you only the last two digits of the year are in plain within the id number so i thy a number of ideas. After bashing around google sheets for a while ( i've got open office installed and formulas don't export well to the excel that person uses but google sheets does so i built it there).
First idea : make a few conditionals to check for the value so we have 1 and 2 for 19th century, 3 and 4 for 18th century , 5 and 6 for 20th so i go ahead and write my conditions and they fail, all evaluates to false, it cascades through the else variants up to the last one so i'm wondering if the "if" itself doesn't support the or operator, seems it does, next i think it's the bloody condition written wrong so i reevaluate my logic in php in a test script, it works as intended, then i think ok not the right function called, let's see the docs, docs confirm i'm doing it right but what was wrong was the way i was getting that first number, using left seems to produce a string although the base thing is a number, now i start searching how i can cast it, like you would normaly do when the data type is fried, value function appears to be the solution but it isn't working....now i'm thinking "ok so i have a value and different things to print out so let's look for a switch, maybe it can understand that" switch function found under the form of choice, i get it sorted but am stuck wondering why the heck was the if and value combination not working.
Simple answer to that : value doesn't work well with function results, a known bug listed by someone in a comment, a comment i have failed to read for about 45 minutes of trying to understand.
All in all it worked well for the person asking for it so it's nice. -
While attempting to quit smoking and after spending a full day trying to understand why the previous devs took this approach to encrypting a string and my lack of nicotine addled brain not allowing me to see that this was a “Secure”String and so uses a machine specific key (that’s why the code that worked locally wouldn’t run on production 😑) this is my rant on comments added to the helper I had to write
/// <summary>
/// If you are using this class and it's not for backward compatibility - then you probably shouldn't be using it
/// Nothing good comes from "Secure" strings
/// Further to this Secure strings are only "useful" for single user crypto as the encryption uses the login creds, transferring
/// this data to another client will result in them never being able to decrypt it
///
/// Windows uses the user's login password to generate a master key.
/// This master key is protected using the user's password and then stored along with the user's profile.
/// This master key then gets used to derive a number of other keys and it's these other keys that are used to protect the data.
///
/// This is also a broken crypto method via injection (see Hawkeye http://hawkeye.codeplex.com/) plus the string is stored in plain
/// text in memory, along with numerous other reasons not to use it.
/// </summary>
public class SecureStringHelper
{3 -
I have to go through roughly 700 data entries and adjust the system's data accordingly ... by hand.😑
We don't have the tools to automate this reliably, the only available tool, tells you that an error occured and what happened but not where. That would've been kinda helpful...
The problem is that the respective data object of the data entry often contains a phone number, which has to be in a standardized format ... which it is not. Every number is formatted in like 10 variations!? A dozen different separators like spaces, commas, slashes and hyphens. And it must be edited manually 😖
My solution: built a goddamn chrome extension to format the string on click. Done. Saves a few seconds each time and a lot of headache in future. Of course given the correctness of the extension.4 -
There were many issues that came about during my entire employment, but I woke up today with some, honestly, quite bizarre questions from my manager that made me open an account here. This is just the latest in many frustrations I have had.
For context, my manager is more of a "tech lead" who maintains a few projects, the number can probably be counted in one hand. So he does have the knowledge to make changes when needed.
A few weeks ago, I was asked to develop a utility tool to retrieve users from Active Directory and insert them into a MSSQL Database, pretty straight forward and there were no other requirements.
I developed it, tested it, pushed it to our repository, then deployed the latest build to the server that had Active Directory, told my manager that I had done so and left it at that.
A few weeks later,
Manager: "Can you update the tool to now support inserting to both MSSQL and MySQL?"
Me: "Sure." (Would've been nice to know that beforehand since I'm already working on something else but I understand that maybe it wasn't in the original scope)
I do that and redeploy it, even wrote documentation explaining what it did and how it worked. And as per his request, a technical documentation as well that explains more in depth how it works. The documents were uploaded as well.
A few days after I have done so,
Manager: "Can you send me the built program with the documentation directly?"
I said nothing and just did as he asked even though I know he could've just retrieved it himself considering I've uploaded and deployed them all.
This morning,
Manager: "When I click on this thing, I receive this error."
Me: "Where are you running the tool?"
Manager: "My own laptop."
Me: "Does your laptop have Active Directory?"
Manager: "Nope, but I am connected to the server with Active Directory."
Me: "Well the tool can only retrieve Active Directory information on a PC with it."
Manager: "Oh you mean it has to run on the PC with Active Directory?"
Me: "Yeah?"
Manager: "Alright. Also, what is the valid value for this configuration? You mentioned it is the Database connection string."
After that I just gave up and stopped responding. Not long after, he sent me a screenshot of the configuration file where he finally figured out what to put in.
A few minutes later,
Manager: "Got this error." And sends a screenshot that tells you what the error is.
Me: "The connection string you set is pointing to the wrong database schema."
Manager: "Oh whoops. Now it works. Anyway, what are these attribute values you retrieve from Active Directory? Also, what is the method you used to connect/query/retrieve the users? I need to document it down for the higher ups."
Me: "The values are the username, name and email? And as mentioned in the technical documentation, it's retrieving using this method."
The 2+ years I have been working with this company has been some of the most frustrating in my entire life. But thankfully, this is the final month I will be working with them.21 -
PL/SQL is one of my primaries at work.
Does not have an official "multidimensional array" per-say. Instead you define anonymous tables types and point it at a different "record" type to define the structure. Then you can make a table of that table indexed by string/number/record/etc. It's all tables. Tables of tables of tables.
Reading it can be wild.1 -
Am I in developer hell already? A shitty project is about to come to an end (hopefully), or should I rather say: It needs to come to an end. But I am still quite lost in how to deal with it, hence procrastinating on it - making the deadline come closer and with it the realization that I'll probably have to rewrite almost everything. I'm not sure how, but I do know that the current code is a dumpster fire.
Basically what I need to do is dealing with the APIs of different payment providers/gateways (like PayPal, AmazonPay). For most cases I'll get a payment ID from the shop and need to act on it later, e.g. capture the authorized money in the case of a credit card transaction or do refunds (without user interaction, unless there is an error). Now at first I put something together where I try to abstract the payment information into two tables:
orders{1}<->{0..n}payments
payments{1}<->{1..n}paymentDetails
Unfortunately trying to abstract the different payment methods and to squeeze them (and their different possible stati and functions) in these tables was not very successful, it's a total mess with magic numbers, half-broken behavior and without any consideration for partial payments/captures or unfinished requests (i.e. if there is an exception before the response is dealt with, there is no indication that anything has ever been sent). Also the current amount is calculated through the history of the paymentDetails table, which basically works differently for each payment type.
How to fix this mess in a way that I'll still have a job by next week?
I'm trying to improve the db schema first, as I think my biggest problems are lying there. Through some research I've come across a recommendation for making payment type specific subtables (with a magic number/string in the main table to prevent having to look up all subtables). That way I can record what I send and receive without having to abstract it too much, so I'll have an acceptable transaction log. The paymentDetails table can be removed (necessary fields go to the payments table). The payments table gets multiple fields for the amount (differentiating between open, authorized, captured, processing and refunded values) and always reflects the current status.
Tables:
payments
paymentRequestsPaypal
paymentRequestsAmazonpay
paymentRequestsXyz
I think I'm going in the right direction here. hm. Maybe there's some light at the end of this long, dark tunnel. Or a train. I'll have two days to find out.question kill me already send help thank you for being my rubber duck payment gateways deadline approaching rant/question burnout6 -
Aka... How NOT to design a build system.
I must say that the winning award in that category goes without any question to SBT.
SBT is like trying to use a claymore mine to put some nails in a wall. It most likely will work somehow, but the collateral damage is extensive.
If you ask what build tool would possibly do this... It was probably SBT. Rant applies in general, but my arch nemesis is definitely SBT.
Let's start with the simplest thing: The data format you use to store.
Well. Data format. So use sth that can represent data or settings. Do *not* use a programming language, as this can neither be parsed / modified without an foreign interface or using the programming language itself...
Which is painful as fuck for automatisation, scripting and thus CI/CD.
Most important regarding the data format - keep it simple and stupid, yet precise and clean. Do not try to e.g. implement complex types - pain without gain. Plain old objects / structs, arrays, primitive types, simple as that.
No (severely) nested types, no lazy evaluation, just keep it as simple as possible. Build tools are complex enough, no need to feed the nightmare.
Data formats *must* have btw a proper encoding, looking at you Mr. XML. It should be standardized, so no crazy mfucking shit eating dev gets the idea to use whatever encoding they like.
Workflows. You know, things like
- update dependency
- compile stuff
- test run
- ...
Keep. Them. Simple.
Especially regarding settings and multiprojects.
http://lihaoyi.com/post/...
If you want to know how to absolutely never ever do it.
Again - keep. it. simple.
Make stuff configurable, allow the CLI tool used for building to pass this configuration in / allow setting of env variables. As simple as that.
Allow project settings - e.g. like repositories - to be set globally vs project wide.
Not simple are those tools who have...
- more knobs than documentation
- more layers than a wedding cake
- inheritance / merging of settings :(
- CLI and ENV have different names.
- CLI and ENV use different quoting
...
Which brings me to the CLI.
If your build tool has no CLI, it sucks. It just sucks. No discussion. It sucks, hmkay?
If your build tool has a CLI, but...
- it uses undocumented exit codes
- requires absurd or non-quoting (e.g. cannot parse quoted string)
- has unconfigurable logging
- output doesn't allow parsing
- CLI cannot be used for automatisation
It sucks, too... Again, no discussion.
Last point: Plugins and versioning.
I love plugins. And versioning.
Plugins can be a good choice to extend stuff, to scratch some specific itches.
Plugins are NOT an excuse to say: hey, we don't integrate any features or offer plugins by ourselves, go implement your own plugins for that.
That's just absurd.
(precondition: feature makes sense, like e.g. listing dependencies, checking for updates, etc - stuff that most likely anyone wants)
Versioning. Well. Here goes number one award to Node with it's broken concept of just installing multiple versions for the fuck of it.
Another award goes to tools without a locking file.
Another award goes to tools who do not support version ranges.
Yet another award goes to tools who do not support private repositories / mirrors via global configuration - makes fun bombing public mirrors to check for new versions available and getting rate limited to death.
In case someone has read so far and wonders why this rant came to be...
I've implemented a sort of on premise bot for updating dependencies for multiple build tools.
Won't be open sourced, as it is company property - but let me tell ya... Pain and pain are two different things. That was beyond pain.
That was getting your skin peeled off while being set on fire pain.
-.-5 -
Heres the initial upgraded number fingerprinter I talked about in the past and some results and an explanation below.
Note that these are wide black images on ibb, so they appear as a tall thin strip near the top of ibb as if they're part of the website. They practically blend in. Right click the blackstrip and hit 'view image' and then zoom in.
https://ibb.co/26JmZXB
https://ibb.co/LpJpggq
https://ibb.co/Jt2Hsgt
https://ibb.co/hcxrFfV
https://ibb.co/BKZNzng
https://ibb.co/L6BtXZ4
https://ibb.co/yVHZNq4
https://ibb.co/tQXS8Hr
https://paste.ofcode.org/an4LcpkaKr...
Hastebin wouldn't save for some reason so paste.ofcode.org it is.
Not much to look at, but I was thinking I'd maybe mark the columns where gaps occur and do some statistical tests like finding the stds of the gaps, density, etc. The type test I wrote categorizes products into 11 different types, based on the value of a subset of variables taken from a vector of a couple hundred variables but I didn't want to include all that mess of code. And I was thinking of maybe running this fingerprinter on a per type basis, set to repeat, and looking for matching indexs (pixels) to see what products have in common per type.
Or maybe using them to train a classifier of some sort.
Each fingerprint of a product shares something like 16-20% of indexes with it's factors, so I'm thinking thats an avenue to explore.
What the fingerprinter does is better explained by the subfunction findAb.
The code contains a comment explaining this, but basically the function destructures a number into a series of division and subtractions, and makes a note of how many divisions in a 'run'.
Typically this is for numbers divisible by 2.
So a number like 35 might look like this, when done
p = 35
((((p-1)/2)-1)/2/2/2/2)-1
And we'd represent that as
ab(w, x, y, z)
Where w is the starting value 35 in this case,
x is the number to divide by at each step, y is the adjustment (how much to subtract by when we encounter a number not divisible by x), and z is a string or vector of our results
which looks something like
ab(35, 2, 1, [1, 4])
Why [1,4]
because we were only able to divide by 2 once, before having to subtract 1, and repeat the process. And then we had a run of 4 divisions.
And for the fingerprinter, we do this for each prime under our number p, the list returned becoming another row in our fingerprint. And then that gets converted into an image.
And again, what I find interesting is that
unknown factors of products appear to share many of these same indexes.
What I might do is for, each individual run of Ab, I might have some sort of indicator for when *another* factor is present in the current factor list for each index. So I might ask, at the given step, is the current result (derived from p), divisible by 2 *and* say, 3? If so, mark it.
And then when I run this through the fingerprinter itself, all those pixels might get marked by a different color, say, make them blue, or vary their intensity based on the number of factors present, I don't know. Whatever helps the untrained eye to pick up on leads, clues, and patterns.
If it doesn't make sense, take another look at the example:
((((p-1)/2)-1)/2/2/2/2)-1
This is semi-unique to each product. After the fact, you can remove the variable itself, and keep just the structure in question, replacing the first variable with some other number, and you get to see what pops out the otherside.
If it helps, you can think of the structure surrounding our variable p as the 'electron shell', the '-1's as bandgaps, and the runs of '2's as orbitals, with the variable at the center acting as the 'nucleus', with the factors of that nucleus acting as the protons and neutrons, or nougaty center lol.
Anyway I just wanted to share todays flavor of insanity on the off chance someone might enjoy reading it.1 -
Reverse number(logic)
------
First Approach :=
void reverseMethod(int n)
{
String str="";
int temp=0;
while(n>0)
{
temp=n%10;
n=n/10;
str=str+""+temp;
}
System.out.println(str);
}
-----
Second Approach :=
void reverseMethod(int n)
{
int temp=0;
int rev=0;
while(n>0)
{
temp=n%10;
n=n/10;
rev=rev*10+temp;
}
System.out.println(rev);
}
-----
why the fuck second one is recommended??
In first, at least we do not required to remember that formula.9 -
So we now do continuous deployment to a development environment. Once a PR gets merged it gets deployed there. We then have to manually deploy to staging every so often.
We did this because QA wined that the Dev was constantly breaking Staging, when we contentiously deployed to that.
So now we have a staging instance that is always behind. Which isn't big deal, because its supposed to be stable right?
Well now the stupid fucking QA team is always making mountains of tickets and noise for stuff that is already fixed on the development instance.
Fucking shit that they message me about, or have to call me about. "Hey let me tell me about this thing I found." And then I'm like I already fixed that thing last week.
So it seems to be wasting everyone time to not just CDCI into staging. I have to wait weeks to retest my bugs on staging. To make sure that some other stupid fuckeshir on my team didn't undo or break my fucking fix. Shit keeps getting kicked out of QA Review. Fuck. lol.
Then there like I can update the thing on the database through the front end tool. Well tough shit buddy, your going to have to wait a week unti next staging deployment to see if that tool is fixed. This is your fault for fucking up our pure CDCI with your ideas. Now everything takes longer for everyody.
To sum things up. Some dumb bug makes it into the manual staging deployment and gets fixed an hour later. Doesn't get deployed until next fucking week. QA makes a bunch of noise about it. A thing that is fixed and in the pipe-line.
Also a dumb fucking bug will make it into staging, lets say a critical front-end back office tool that needs to send numbers to the backend, they send a fucking string instead of a number and break it. Now we have to redeploy the tool and backend to staging because there related. Then if we deploy backend we have to deploy the client facing site too. since it also depends on backend.
Its a fucking hassle.
Now if the fucking DevOps guy could do his job, and make a god-damn deploy button for all the staging servers that would be great.1 -
The bug: Some string values for an identifier property in the data objects are being sent from our frontend prefixed with a '0'. Sometimes. When it happens, it usually gets stripped away again by the time it's passed to our backend. But not always.
This 0 is never explicitly set anywhere. I even searched for a few variants of " = 0" in both the frontend and backend projects without receiving any results. You might already be suspecting where this is going.
So it turns out.
The data object which holds this value is being initialized in the aspnet (don't ask) backend and passed to the frontend, which then hydrates it. This value is always an integer number, albeit incidentally so which is why string is used as the actual type. When this object is initialized, it's hardcoded with an anonymous type where this property is set as int because I guess someone figured "it's always an int though". Being a typed language, primitive scalars can't be null objects which means the property's value becomes the concrete int 0.
Okay weird. I can think of better ways of doing this but let's just set it to string as I can't start overhauling things right now. Let's just go find where this value is somehow concatenated into the incoming parameter.
You see, this happens because at the point where the frontend sets this value, it may be an int or string depending on where it came from, and I guess someone figured that in order to cast it to string you just go prop += arg seeing as the prop is empty string and all. Because explicitly casting it or - as much as I get a rash whenever I see it - going prop = "" + arg would be too verbose and unoriginal.
Bonus round: How come the 0 only sometimes made it all the way to our backend? The thing is that this bug has been fixed before. The fix is that because this string is "always" an int, you can parse it to int before passing it to the backend in case it has leading zeroes. This path is only taken in certain views because someone forgot to copypaste their fix into all the places this is repeated.
Sometimes you find a bug and you are just somehow more grumpy after fixing it.1 -
So I was playing around with JS inside of QML (Qt). I wanted to turn a number into a string and then modify individual chars based on certain criteria. Then I would convert back to a number. Should be simple right? Well JS would not let me write to the indexed element of the string. What the actual fuck? I need to drop to C++ to do actual string manipulation? I ended up looping the string and turning it into an array and then a join back to a string. There is probably a better way to do this in a more modern version of JS, but I am now starting to see why JS is a pariah on here. It has some quirks that make you question your devlife choices.
What was I working on? Some stupid crop circle thingy. I was trying to use the QML Canvas to draw some "simple" crop circles. So I started with this crop circle:
https://plus.maths.org/content/...
The result ended up being kind of a swirling crop circle thing. The static image was too boring:
https://imgur.com/gallery/900hogf
And yes, I was too lazy/cheap to license the screen capture program.7 -
How to write programs on Android 10 that work with files/directories? Have used a number of JVM-based languages like Groovy, Clojure and Kotlin.
My last try was with Groovy. I ran it under Dcoder which has to be cloud-, based as it supports numerous languages. I gave it permission to access storage but got a file not found error from Java. Copied this excerpt for the file path.
import java.io.File
class Example {
static void main(String[] args) {
new File("/storage/emulated/0/read_file.grvy").eachLine {
line -> println "line : $line";
}
}
}
Do I need root? Do I need to change file permissions using Termux? Why can't I find a way to write simple software on a Motorola Super, 3 GB RAM and 8 cores? I hate using a phone for a computer but a seizure has me in a nursing home with only one usable hand.
Any help is greatly appreciated.5 -
Lost about 4 days debugging bug about date conversion between frontend to backend as an api request.
This shit is mad fucking annoying
The date format was always wrong.
So i gotta ask. Is it better to always have date fields as a Long which contains just a huge number that represents a timestamp, and that way whenever i want to see what date it is i would have to convert it every time on both frontend and backend from timestamp into LocalDateTime, or is it better to keep it as Date/LocalDateTime and not string/long, and that way risk fucking up the date format?
How is it done in real world projects? Whats the right way to do it and why?3 -
Brain fart.
In Java and many other languages there are basic types, like char and String. So why does Java have char and String, but not a digit type?
A number is basically a series of digits. For modular arithmetic it is very useful to be able to extract the 3 in the number 1234, it's just the 3rd digit in a number.
Base 2, base 10, base anything could be supported easily too. E.g. a base 2 digit would be:
digit d = 0b2; // or 1b2, but 2b2 would be a compilation error
A number would then be some kind of string of digits.
Any thoughts on this?9