Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
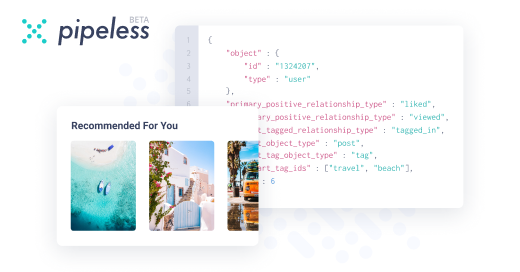
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "types in js"
-
Languages without a fully implemented type system.
Granted, it has been a fad for a quarter century, but everything points at one simple fact: Types matter in programming.
In dynamic languages, you tend to see that testing suites explode into thousands of tests, many of which wouldn't even be necessary if you had some type safety.
You see that languages like JS are forked into more typesafe dialects, like Typescript. Python got typehints since 3.6, and PHP added typehints for methods, then typehints for properties, and will soon even have compound types.
Maybe most languages will never reach the level of Haskell or Scala, and that's totally fine, but I think the direction languages are moving in is pretty much set in stone: No ambiguity, more safety. Code should fail before deploying, not after.32 -
I’m surrounded by idiots.
I’m continually reminded of that fact, but today I found something that really drives that point home.
Gather ‘round, everybody, it’s story time!
While working on a slow query ticket, I perused the code, finding several causes, and decided to run git blame on the files to see what dummy authored the mental diarrhea currently befouling my screen. As it turns out, the entire feature was written by mister legendary Apple golden boy “Finder’s Keeper” dev himself.
To give you the full scope of this mess, let me start at the frontend and work my way backward.
He wrote a javascript method that tracks whatever row was/is under the mouse in a table and dynamically removes/adds a “.row_selected” class on it. At least the js uses events (jQuery…) instead of a `setTimeout()` so it could be worse. But still, has he never heard of :hover? The function literally does nothing else, and the `selectedRow` var he stores the element reference in isn’t used elsewhere.
This function allows the user to better see the rows in the API Calls table, for which there is a also search feature — the very thing I’m tasked with fixing.
It’s worth noting that above the search feature are two inputs for a date range, with some helpful links like “last week” and “last month” … and “All”. It’s also worth noting that this table is for displaying search results of all the API requests and their responses for a given merchant… this table is enormous.
This search field for this table queries the backend on every character the user types. There’s no debouncing, no submit event, etc., so it triggers on every keystroke. The actual request runs through a layer of abstraction to parse out and log the user-entered date range, figure out where the request came from, and to map out some column names or add additional ones. It also does some hard to follow (and amazingly not injectable) orm condition building. It’s a mess of functional ugly.
The important columns in the table this query ultimately searches are not indexed, despite it only looking for “create_order” records — the largest of twenty-some types in the table. It also uses partial text matching (again: on. every. single. keystroke.) across two varchar(255)s that only ever hold <16 chars — and of which users only ever care about one at a time. After all of this, it filters the results based on some uncommented regexes, and worst of all: instead of fetching only one page’s worth of results like you’d expect, it fetches all of them at once and then discards what isn’t included by the paginator. So not only is this a guaranteed full table scan with partial text matching for every query (over millions to hundreds of millions of records), it’s that same full table scan for every single keystroke while the user types, and all but 25 records (user-selectable) get discarded — and then requeried when the user looks at the next page of results.
What the bloody fucking hell? I’d swear this idiot is an intern, but his code does (amazingly) actually work.
No wonder this search field nearly crashed one of the servers when someone actually tried using it.
Asdfajsdfk.rant fucking moron even when taking down the server hey bob pass me all the paperclips mysql murder terrible code slow query idiot can do no wrong but he’s the golden boy idiots repeatedly murdered mysql in the face21 -
WASM was a mistake. I just wanted to learn C++ and have fast code on the web. Everyone praised it. No one mentioned that it would double or quadruple my development time. That it would cause me to curse repeatedly at the screen until I wanted to harm myself.
The problem was never C++, which was a respectable if long-winded language. No no no. The problem was the lack of support for 'objects' or 'arrays' as parameters or return types. Anything of any complexity lives on one giant Float32Array which must surely bring a look of disgust from every programmer on this muddy rock. That is, one single array variable that you re-use for EVERYTHING.
Have a color? Throw it on the array. 10 floats in an object? Push it on the array - and split off the two bools via dependency injection (why do I have 3-4 line function parameter lists?!). Have an image with 1,000,000 floats? Drop it in the array. Want to return an array? Provide a malloc ptr into the code and write to it, then read from that location in JS after running the function, modifying the array as a side effect.
My- hahaha, my web worker has two images it's working with, calculations for all the planets, sun and moon in the solar system, and bunch of other calculations I wanted offloaded from the main thread... they all live in ONE GIANT ARRAY. LMFAO.If I want to find an element? I have to know exactly where to look or else, good luck finding it among the millions of numbers on that thing.
And of course, if you work with these, you put them in loops. Then you can have the joys of off-by-one errors that not only result in bad results in the returned array, but inexplicable errors in which code you haven't even touched suddenly has bad values. I've had entire functions suddenly explode with random errors because I accidentally overwrote the wrong section of that float array. Not like, the variable the function was using was wrong. No. WASM acted like the function didn't even exist and it didn't know why. Because, somehow, the function ALSO lived on that Float32Array.
And because you're using WASM to be fast, you're typically trying to overwrite things that do O(N) operations or more. NO ONE is going to use this return a + b. One off functions just aren't worth programming in WASM. Worst of all, debugging this is often a matter of writing print and console.log statements everywhere, to try and 'eat' the whole array at once to find out what portion got corrupted or is broke. Or comment out your code line by line to see what in forsaken 9 circles of coding hell caused your problem. It's like debugging blind in a strange and overgrown forest of code that you don't even recognize because most of it is there to satisfy the needs of WASM.
And because it takes so long to debug, it takes a massively long time to create things, and by the time you're done, the dependent package you're building for has 'moved on' and find you suddenly need to update a bunch of crap when you're not even finished. All of this, purely because of a horribly designed technology.
And do they have sympathy for you for forcing you to update all this stuff? No. They don't owe you sympathy, and god forbid they give you any. You are a developer and so it is your duty to suffer - for some kind of karma.
I wanted to love WASM, but screw that thing, it's horrible errors and most of all, the WASM heap32.7 -
The brief history of Facebook open source:
- FB releases React under an oppressive licence that tells "woopsie, can't sue FB if you use React"
- a lot of money goes into making React popular to gain leverage from mass adoption
- VMware bans React in their company
- FB releases Flux to bring state management. It flops. Replaced by what some Russian student wrote in several evenings (Redux)
- Preact is released. It's faster than React, and it has MIT licence. Vue beats React in GitHub stars.
- Under mass pressure, FB changes React's licence to MIT. Initial plan to gain leverage fails spectacularly.
- FB releases Flow Types. It flops. Replaced by TypeScript.
- FB releases their own app market for React Native. It flops.
- FB releases Relay. It flops. Replaced by Apollo.
- FB tries to push React.Suspense for the whole JS landscape to obey and comply to how it works. Community says "Fuck You".
- FB releases react-native-web. It flops.
- Web Components are out in all browsers, adopted as a standard. React doesn't support them.
- Google releases Lit, a virtual DOM framework on top of Web Components to fuck with React. It's a massive success.
- React 18 is out. Still no Web Components support.
- (you are here)16 -
Java:
Primitive streams. Their need to exist is a monument to legacy failure.
VB.net
OrElse and AndAlso short-circuiting operators. The language designers were too fucking lazy to process logic, so they give specific keywords for those cases.
PHP
Random Hebrew error messages
JS
Eval. It can be used responsibly, but most of the times you see it it's because someone fucked up.
C#
Lack of Tuple destructuring in argument specification. Tuples were added, and pattern matching was added, and it's been getting better. The gear grinding starts with how Tuple identity assignment in arguments is handled. Rather than destructuring into the current scope, it coalesces the identity specification into a dot property of whatever the argument name is. This seems like an afterthought given they have ootb support for ignore characters.
Typescript
This will probably be remedied in the next version or two, but Tuple identity forwarding between anonymous scopes normalizes to arrays of union types, because tuples compile to typeless arrays. It's irritating because you end up having to restate the type metadata in functional series even when there is no possibility for any other code branch to have occurred.12 -
I come from PHP and have been using jQuery as a crutch for years. I've dabbled in Node and obviously writing some vanilla JS is unavoidable, but now I'm starting to use ES6 with Babel and VueJS and the more I dig in, the more I hate javascript. Everything is an object (null, array), there seems to be a few gotchas with math (including the one pictured), nothing is strongly typed but types are very important (for instance, you can't loop an object and an array using the same code), and there are a lot of inconsistencies/functions missing. ucwords for instance. Why doesn't that exist? typeof only returns object, but Array.isArray and Object.isObject work fine. I'm going to give typescript a go soon and cross my fingers.20
-
young user @Mizukuro asked days ago for ways to improving his javascript skills.
I wasn't sure what to say at the moment, but then I thought of something.
Lodash is the most depended upon package in npm. 90k packages depend on it, more than double than the second most depended upon package (request with 40k).
Lodash was also created 6 years ago.
This means lodash has been heavily tested, and is production ready.
This means that reading and understanding its code will be very educational.
Also, every lodash function lives in its own file, and are usually very short.
This means it's also easy to understand the code.
You could start with one of the "is..." (eg isArray, isFunction).
The reason for such choice is that it's very easy to understand what these functions do from their name alone.
And you also get to see how a good coder deals with js types (which can be very impredictible sometines).
And to learn even more, read the test file for that function (located in tests/<original file name>.js. For the most part they are very readable and examples of very good testing code.
Here's the isFunction code
https://github.com/lodash/lodash/...
Here's the test for isFunction
https://github.com/lodash/lodash/...
The one thing you won't learn here is about es5, 6, or whatever.3 -
Angular is still a pile of steaming donkey shit in 2023 and whoever thinks the opposite is either a damn js hipster (you know, those types that put js in everything they do and that run like a fly on a lot of turds form one js framework to the next saying "hey you tried this cool framework, this will solve everything" everytime), or you don't understand anything about software developement.
I am a 14 year developer so don't even try to tell me you don't understand this so you complain.
I build every fucking thing imaginable. from firmware interfaces for high level languaces from C++, to RFID low level reading code, to full blown business level web apps (yes, unluckily even with js, and yes, even with Angular up to Angular15, Vue, React etc etc), barcode scanning and windows ce embedded systems, every flavour of sql and documental db, vectorial db code, tech assistance and help desk on every OS, every kind of .NET/C# flavour (Xamarin, CE, WPF, Net framework, net core, .NET 5-8 etc etc) and many more
Everytime, since I've put my hands on angularJs, up from angular 2, angular 8, and now angular 15 (the only 3 version I've touched) I'm always baffled on how bad and stupid that dumpster fire shit excuse of a framework is.
They added observables everywhere to look cool and it's not necessary.
They care about making it look "hey we use observables, we are coo, up to date and reactive!!11!!1!" and they can't even fix their shit with the change detection mechanism, a notorious shitty patchwork of bugs since earlier angular version.
They literally built a whole ecosystem of shitty hacks around it to make it work and it's 100x times complex than anything else comparable around. except maybe for vanilla js (fucking js).
I don't event want todig in in the shit pool that is their whole ecosystem of tooling (webpack, npm, ng-something, angular.json, package.json), they are just too ridiculous to even be mentioned.
Countless time I dwelled the humongous mazes of those unstable, unrealiable shitty files/tools that give more troubles than those that solve.
I am here again, building the nth business critical web portal in angular 16 (latest sack of purtrid shit they put out) and like Pink Floyd says "What we found, same old fears".
Nothing changed, it's the same unintelligible product of the mind of a total dumbass.
Fuck off js, I will not find peace until Brendan Eich dies of some agonizing illness or by my hands
I don't write many rants but this, I've been keeping it inside my chest for too long.
I fucking hate js and I want to open the head of js creator like the doom marine on berserk19 -
as a C# dev every time i have to code something in JS i'm just ranting because
- no types
- no fucking errors
i tried to move a Oval in an HTML5 canvas via Drag and Drop and after one hour I gave up...
such a fucking creepy broke language..
as a proof, if js wouldnt be that fucked up why is there typeScript, CoffeeScript, Brython, ... ?
Cant wait to finally use WebAssember...(really)9 -
Today I had a problem with a JS framework. The only person who was available who could help me was the one I avoid, because he always knows everything better.
Well, after I asked if he had time for me, he sits next to me and I started to explain.
After looking around, he started blaming my backend code.
(I belong to the kind of dev that tries to write small and simple code. But I also often use the more complex features of the languages.) He suddenly started accusing everyday things in the backend like inheriting a class or using objects and basic data types together as parameters of a method (WTF???) Hell, all I could say at that moment was that I had a problem with this JS framework and not with the backend that worked well. He probably tried for over an hour to find the bug in the backend and just wouldn't listen, after that he gave up. I wonder what this bitch has learned over the years. Can it really be that he forgot the basics of a programming language? Or has the fool never worked with an inheritance before? I think he's an incapable piece of shit, he hasn't even patched my reported vulnerability in his project in the last half year, which allows to inject own code onto the server.
Because of such fucking morons I get a headache when I think about it. How can it be that he's got a higher degree and earns about 50% more. I should leave this company!3 -
Why I hate typescript. Bored during quarantine so thought I rant a little more about this.
1. Compilation time, typescript increases project compilation time from 1 second to 3-4 seconds, which is basically triple or quadruple the time if you don't know math.
2. You write a minimum of 30% more code.
3. Many libraries are not written in TS by default, which means you end up having to manually install a fuckton of @types/(pckg name) manually which is incredibly shit.
4. Typescript is an absolute pain in the ass when using dynamic libraries. Plus when it works, it usually ends up finding maybe 1-2 errors in your code MAX, completely not worth it.
5.JSDoc is 100 times better. (Still don't use it though).
6. I actually enjoy loosely typed languages, having your compiler being smart enough to tell what the type of your input is is much better than it assuming you're a fucking retard so it forces you to manually type everything.
P.S if you hate loosely typed languages, kindly resort to Angular, C#, Java or whatever and leave JS alone, cunt.41 -
(long post is long)
This one is for the .net folks. After evaluating the technology top to bottom and even reimplementing several examples I commonly use for smoke testing new technology, I'm just going to call it:
Blazor is the next Silverlight.
It's just beyond the pale in terms of being architecturally flawed, and yet they're rushing it out as hard as possible to coincide with the .Net 5 rebranding silo extravaganza. We are officially entering round 3 of "sacrifice .Net on the altar of enterprise comfort." Get excited.
Since we've arrived here, I can only assume the Asp.net Ajax fiasco is far enough in the past that a new generation of devs doesn't recall its inherent catastrophic weaknesses. The architecture was this:
1. Create a component as a "WebUserControl"
2. Any time a bound DOM operation occurs from user interaction, send a payload back to the server
3. The server runs the code to process the event; it spits back more HTML
Some client-side js then dutifully updates the UI by unceremoniously stuffing the markup into an element's innerHTML property like so much sausage.
If you understand that, you've adequately understood how Blazor works. There's some optimization like signalR WebSockets for update streaming (the first and only time most blazor devs will ever use WebSockets, I even see developers claiming that they're "using SignalR, Idserver4, gRPC, etc." because the template seeds it for them. The hubris.), but that's the gist. The astute viewer will have noticed a few things here, including the disconnect between repaints, inability to blend update operations and transitions, and the potential for absolutely obliterative, connection-volatile, abusive transactional logic flying back and forth to the server. It's the bring out your dead approach to seeing how much of your IT budget is dedicated to paying for bandwidth and CPU time.
Blazor goes a step further in the server-side render scenario and sends every DOM event it binds to the server for processing. These include millisecond-scale events like scroll, which, at least according to GitHub issues, devs are quickly realizing requires debouncing, though they aren't quite sure how to accomplish that. Since this immediately becomes an issue with tickets saying things like, "scroll event crater server, Ugg need help! You said Blazorclub good. Ugg believe, Ugg wants reparations!" the team chooses a great answer to many problems for the wrong reasons:
gRPC
For those who aren't familiar, gRPC has a substantial amount of compression primarily courtesy of a rather excellent binary format developed by Google. Who needs the Quickie Mart, or indeed a sound markup delivery and view strategy when you can compress the shit out of the payload and ignore the problem. (Shhh, I hear you back there, no spoilers. What will happen when even that compression ceases to cut it, indeed). One might look at all this inductive-reasoning-as-development and ask themselves, "butwai?!" The reason is that the server-side story is just a way to buy time to flesh out the even more fundamentally broken browser-side story. To explain that, we need a little perspective.
The relationship between Microsoft and it's enterprise customers is your typical mutually abusive co-dependent relationship. Microsoft goes through phases of tacit disinterest, where it virtually ignores them. And rightly so, the enterprise customers tend to be weaksauce, mono-platform, mono-language types who come to work, collect a paycheck, and go home. They want to suckle on the teat of the vendor that enables them to get a plug and play experience for delivering their internal systems.
And that's fine. But it's also dull; it's the spouse that lets themselves go, it's the girlfriend in the distracted boyfriend meme. Those aren't the people who keep your platform relevant and competitive. For Microsoft, that crowd has always been the exploratory end of the developer community: alt.net, and more recently, the dotnet core community (StackOverflow 2020's most loved platform, for the haters). Alt.net seeded every competitive advantage the dotnet ecosystem has, and dotnet core capitalized on. Like DI? You're welcome. Are you enjoying MVC? Your gratitude is understood. Cool serializers, gRPC/protobuff, 1st class APIs, metadata-driven clients, code generation, micro ORMs, etc., etc., et al. Dear enterpriseur, you are fucking welcome.
Anyways, b2blazor. So, the front end (Blazor WebAssembly) story begins with the average enterprise FOMO. When enterprises get FOMO, they start to Karen/Kevin super hard, slinging around money, privilege, premiere support tickets, etc. until Microsoft, the distracted boyfriend, eventually turns back and says, "sorry babe, wut was that?" You know, shit like managers unironically looking at cloud reps and demanding to know if "you can handle our load!" Meanwhile, any actual engineer hides under the table facepalming and trying not to die from embarrassment.36 -
I want to explain to people like ostream (aka aviophille) why JS is a crap language. Because they apparently don't know (lol).
First I want to say that JS is fine for small things like gluing some parts togeter. Like, you know, the exact thing it was intended for when it was invented: scripting.
So why is it bad as a programming language for whole apps or projects?
No type checks (dynamic typing). This is typical for scripting languages and not neccesarily bad for such a language but it's certainly bad for a programming language.
"truthy" everything. It's bad for readability and it's dangerous because you can accidentaly make unwanted behavior.
The existence of == and ===. The rule for many real life JS projects is to always use === to be more safe.
In general: The correct thing should be the default thing. JS violates that.
Automatic semicolon insertion can cause funny surprises.
If semicolons aren't truly optional, then they should not be allowed to be omitted.
No enums. Do I need to say more?
No generics (of course, lol).
Fucked up implicit type conversions that violate the principle of least surprise (you know those from all the memes).
No integer data types (only floating point). BigInt obviously doesn't count.
No value types and no real concept for immutability. "Const" doesn't count because it only makes the reference immutale (see lack of value types). "Freeze" doesn't count since it's a runtime enforcement and therefore pretty useless.
No algebraic types. That one can be forgiven though, because it's only common in the most modern languages.
The need for null AND undefined.
No concept of non-nullability (values that can not be null).
JS embraces the "fail silently" approach, which means that many bugs remain unnoticed and will be a PITA to find and debug.
Some of the problems can and have been adressed with TypeScript, but most of them are unfixable because it would break backward compatibility.
So JS is truly rotten at the core and can not be fixed in principle.
That doesn't mean that I also hate JS devs. I pity your poor souls for having to deal with this abomination of a language.
It's likely that I fogot to mention many other problems with JS, so feel free to extend the list in the comments :)
Marry Christmas!34 -
So I've created this account specifically for this rant. I usually just browse anonymously.
I've recently been hired in a big company that is one of the biggest Microsoft users in the world and my essentially revolves on making it easier for our collaborators to work with SharePoint (and other ms software)
Never in my life have I hit that much of a roadblock. So for the past week I've been trying to integrate what Ms calls webparts. And to modify the default webparts Ms provides you need to their properties (or Metadata). Except here's the big problem these are NOT documented anywhere (unless I failed to find it, if you do know where it is documented please HMU), so I've found myself trying to reverse engineer the js scripts that are served with SharePoint to figure out what the webpart properties are called and what type of data they are! I've been going through endless github repos using the CSOM nuget package (it's the library everyone uses to interact with SharePoint) and I finally found out about this other library called PnP which is a wrapper around CSOM that makes it easier to use. That wrapper has a way for me to load existing page and look at the properties of existing webparts. So here I thought it was the end of my suffering and I could finally get an idea of what it should be. Turns out this method doesn't work because one of the dependencies it has has had breaking changes and they still updated it even though it breaks their code! So for the past two days I've been trying random combinations of key values with different data types and json serialization methods.
Oh and yeah I've also looked at all the http calls via the chrome network tab, the metadata is not served as an individual file but is computed by Ms servers when they're serving you their html files.
So uh yeah run from CSOM if you can..3 -
While taking the basic JS interview:
Me: what are the different data types in JavaScript
Candidate: We have a 'var' keyword
Me: :|2 -
Working on an Android app for a client who has a dev team that is developing a web app in with ember js / rails. These folks are "in charge" of the endpoints our app needs to function. Now as a native developer, I'm not a hater of a web apps way of doing things but with this particular app their dev teams seems to think that all programming languages can parse json as dynamically as javascript...
Exhibit A:
- Sample Endpoint Documentation
* GetImportantInfo
* Params: $id // id of info to get details of
* Endpoint: get-info/$id
* Method: GET
* Entity Return {SampleInfoModel}
- Example API calls in desktop REST client
* get-info/1
- response
{
"a" : 0,
"b" : false,
"c" : null
}
* get-info/2
- response
{
"a" : [null, "random date stamp"],
"b" : 3.14,
"c" : {
"z" : false,
"y" : 0.5
}
}
* get-info/3
- response
{
"a" : "false" // yes as a string
"b" : "yellow"
"c" : 1.75
}
Look, I get that js and ruby have dynamic types and a string can become a float can become a Boolean can become a cat can become an anvil. But that mess is very difficult to parse and make sense of in a stack that relies on static types.
After writing a million switch statements with cases like "is Float" or "is String" from kotlin's Any type // alias for java.Object, I throw my hands in the air and tell my boss we need to get on the phone with these folks. He agrees and we schedules a day that their main developer can come to our shop to "show us the ropes".
So the day comes and this guy shows up with his mac book pro and skinny jeans. We begin showing him the different data types coming back and explain how its bad for performance and can lead to bugs in the future if the model structure changes between different call params. He matter of factually has an epiphany and exclaims "OHHHHHH! I got you covered dawg!" and begins click clacking on his laptop to make sense of it all. We decide not to disturb him any more so he can keep working.
3 hours goes by...
He burst out of our conference room shouting "I am the greatest coder in the world! There's no problem I can't solve! Test it now!"
Weary, we begin testing the endpoints in our REST clients....
His magic fix, every single response is a quoted string of json:
example:
- old response
{
"foo" : "bar"
}
- new "improved" response
"{ \"foo\" : \"bar\" }"
smh....8 -
Being a front end developer and working in a team of motivated "full stack" developers sucks big time.
So, recently joined this new company with a very small project which just started, basically a cloud version of a really old desktop app. Few people from the team completely from the asp dotnet background decided the architecture few months before I joined in.
So, they did it something like this -
- mono repo dotnet project with VueJs app served within it (because that would be maintainable 😑)
- vue app served by pointing the built files through dotnet index file (simply because they didn't care about the gift to the front end world which is webpack or even had any knowledge about it 😑)
- added typescript because, u know it's cool 😑, without even knowing that they don't possess that team which know how to write the types (f***ers write classes for every payload object coz they don't know what interfaces are)
- no loader to load typescript, they are running tsc in watch mode and we have .js and .js.map for every .ts file in our project which some teammates are even pushing to repo
Recently, I added eslint with git hooks to the project so that everyone will at least stick to the coding standards. Now, to avoid the errors they are bypassing the git hooks by uninstalling the library and then installing it after the commit😂😂
Then we have a girl who uses document.getElementById to programmatically change styles in a Vue project😑😑😑😂
Then we have dotnet people using dotnet coding conventions all over the front end app.
People, how do I deal with these so called "full stack" people?12 -
Many "purists" love to piss on JavaScript and web development. And to an extent I can understand ostream’s frustration with these people.
It’s easy to criticize because yes: many web projects are indeed shit.
But I’d like to argue that the reason why so many of these projects are crappy is because of bad management:
- unrealistic deadlines
- no clear testing strategy
- or no testing at all because of deadlines
- no time allotted to catch up on technical debt
- etc.
This type of management is far more commonplace in web projects because things need to get delivered quickly and if they’re delivered with bugs, it’s no big deal as lives aren’t at stake.
I doubt this type of management is tolerated in projects where you’re working on software for welding machines (for example), where the stakes are that "you’re expected not to kill anyone" (to quote demolishun)
So in these types of projects, management can’t tolerate anything much below perfection and thus has to adapt by setting realistic deadlines that take into account the need for quality processes and thorough testing.
If this type of management was more common in web development, I can guarantee that web applications would be much more reliable and of better quality.
I can also guarantee that poorly managed non-web projects as outlined above would be just shitty as many web products.
My point being that’s it’s really DUMB to criticize fellow devs that work with web technologies on the basis that the state of websites/web apps is a mess. It just so happens that JS is the language of the web and that the web is where things are expected to be delivered quickly (and dirty … but we can fix it later mentality)
Stop acting like you’re the elite. I have no doubt you’re super smart and great at what you do. So be smart all the way and stop criticizing us poor webdevs that have to live with the sad state of affairs. ❤️38 -
Hi everyone, I’m new here and this is also my first rant.
I’m in the job hunting boat once again and I’ve been looking at Junior front-end positions. I thought I’d rant about something that always annoys me when looking through the requirements.
Wait, so in order to land a Junior front-end job, I have to be a freshly graduated person with a Master’s degree in CS, with a minimum of 3 years working experience and all that just to come code in HTML, CSS and JS?
For the love of god, I’m one person damn it. It’s not like I’m a self-taught developer that taught myself those things and more in a shorter period of time after quitting college.
On a more serious note, I’m not by any means claiming that I know everything, but having a CS Master’s degree for these types of positions is clearly ridiculous in my opinion.
Sometimes I wonder if the people writing these things are making it up as they go or whether they’re actually serious.8 -
What I'm doing now, writing a JS library for a simple kitchen timer (like, something that can be wound up, is ticking, can be paused, etc). Here's a list of neat stuff I've learned:
Polyfilling as a lib author (I decided against it).
Packaging the lib (using Rollup, ES6 modules are totes cool).
Using flow to add static typing in strategic places (started appreciating types in JS since reading up on functional programming).
Modelling state and transitions using an explicit state machine. (Fucking finally. There's usually an implicit state machine somewhere, only spread out all over the app...)
Using mostly side-effect free methods, being very explicit about when and why things are mutated).
Test-first/TDD (ish) using Jest and the awesome Wallabyjs.
Freeing up mental capacity by letting Prettier format my code for me (it was hard to let go but totally worth it).
Started using git.
Did all work on Ubuntu after pretty much a lifetime of Windows (initially to separate work from gaming) and finally swapped MS Visual Studio for Atom.
When it's finished I'm going to publish it on GitHub, which will also be a first for me. Might try out some CI platform while I'm at it.
tl;dr: wrote some js, felt good2 -
Apparently my learning style is more rote memorization than learn-by-doing and I've been trying to learn by doing for years as a hobbyist.
It took a fucking *national quarantine* to get me to try something different and I'm blown away.
What would have taken me many months to learn I've all but grasped in detail in a matter of 20 hours of study over the course of a week.
Fuck you javascript. I WIN THIS ROUND. No more looking at the documentation for stupid shit like how to write a regex, or why everything is wrapped in fucking parenthesis (IIFE), or why
I keep getting a uncaught reference exception.
The important thing to realize about learning is NEVER be obstinate about it. Try many things, and don't get stuck in one way of learning unless you know thats what works for you.
This is why having study partners and mentors are important.
I think experience/practice and rote learning work in tandem. Rote learning lets you skip the much longer step of grasping the fundamentals, bootstrapping the process of learning the abstractions that are composed of those fundamentals.
I'm still adding cards to my anki flash card deck, but if anyone wants it I'm willing to share. It's mostly just 1. practice questions, 2. detail questions (what are the types? What does this regex do?, etc), 3. implication questions (heres this bit of code. It's XYZ, why did it fail? Correct it.), combining core details to memorize, and the application of the facts learned.
It helped me to learn and I'm apparently retarded, so if you're new to programming and want to learn JS, it can probably help you too. Unless you're more of a tard than me lol.1 -
I'm currently between jobs and have a few rants about my previous job (naturally). In retrospect, it's somewhat therapeutic to range about the sheer brainfuckery that has taken place. Enjoy!
First, let me set the scene: legacy B2B web app made with LEMP stack and sencha ext.js 3 + 4 (don't ask) and a lot of madness. Let's call that app "Alpha".
Alpha is a self made CMS build for typical ERP stuff. Yes, a self made CMS: entities are containers, containers have types and fields and values. Like so many legacy PHP apps, it does not have a dedicated FE: the HTML is rendered on the server and then spewed out to the browser.
Easy right? Coding like it's 1999! But there was a twist: Because everything is basically a container, the HTML-templates are saved in the DB. Along with the nessary JS and the CSS. And the translation variables. Why? Because fuck you! That's why. Who needs a git history anyways.
For some reason, Alpha was kinda slow.
There was also an editor, that allowed you to modify templates (web, mail, pdf) on the fly in prod. Because templates contain repeating data (header/footer), one template could contain additional templates. Much confusion. You could change templates via migration (slow, boring) or just ctrl-c/ctrl-v that sucker (fast, much excitement).
Did I mention Alpha was slow?
On with the rant: e-mails! How do they work? Noone knows. How to send mails asynchronous in PHP? Witchcraft is the only possible answer to that riddle. Here is your enterprise™ solution:
1. create mail
2. insert mail into DB
3. WAIT UP TO 59 SECONDS FOR A FUCKING CRON TO SEND MAIL
Why? "Because that way, we can resend mails in case the network is down :)"
Same procedure for the SOAP-API (db-queue + cron). You read that right: all requests to various other systems are processed once a minute.
Alpha slow.
Alpha was only one of several systems. Imagine a bunch of monolithic php apps, interconnected via SOAP, REST and GraphQL like a godamn intergalactic orgy. Image having to debug that cluster fuck.
Let's say there is a bad request. These things happen. No biggie. Remember the db-queue? Let's try to send the bad request a second time! And a third time! Still no luck? How odd. Let's create a specific file in a specific directory: a LOCK-file. Now, "the db-queue is on hold and no request gets processed :)"
Golly gee thanks Alpha.
Anyhow, did you know that MySQL has a join limit of 61 tables?3 -
I have always been interested in computers. when I was in second grade, I decided I was no good at electronic circuits, and decided I wanted to program instead. My dad told be to check out free basic, and I immediately downloaded FBIDE, and followed tutorial videos on YouTube. once I finished the videos, I started to write mad libs programs. I made various types of calculators, etc. and loved it, so later I learned a bit of VB. I messed with that a bit, but didn't like it too much, and started web developing. The moment I saw some JS code, it was like an instinctive second language to me. I learned js and started making some ugly, but cool interactive web pages. When computercraft came out for minecraft, I learned lua and got a deeper understanding of programming. Now, I am using node to build a personal-use IoT server and currently making a drone flight program using a raspberry pi3
-
To me this is one of the most interesting topics. I always dream about creating the perfect programming class (not aimed at absolute beginners though, in the end there should be some usable software artifact), because I had to teach myself at least half of the skills I need everyday.
The goal of the class, which has at least to be a semester long, is to be able to create industry-ready software projects with a distributed architecture (i.e. client-server).
The important thing is to have a central theme over the whole class. Which means you should go through the software lifecycle at least once.
Let's say the class consists of 10 Units à ~3 hours (with breaks ofc) and takes place once a week, because that is the absolute minimum time to enable the students to do their homework.
1. Project setup, explanation of the whole toolchain. Init repositories, create SSH keys for github/bitbucket, git crash course (provide a cheat sheet).
Create a hello world web app with $framework. Run the web server, let the students poke around with it. Let them push their projects to their repositories.
The remainder of the lesson is for Q&A, technical problems and so on.
Homework: Read the docs of $framework. Do some commits, just alter the HTML & CSS a bit, give them your personal touch.
For the homework, provide a $chat channel/forum/mailing list or whatever for questions where not only the the teacher should help, but also the students help each other.
2. Setup of CI/Build automation. This is one of the hardest parts for the teacher/uni because the university must provide the necessary hardware for it, which costs money. But the students faces when they see that a push to master automatically triggers a build and deploys it to the right place where they can reach it from the web is priceless.
This is one recurring point over the whole course, as there will be more software artifacts beside the web app, which need to be added to the build process. I do not want to go deeper here, whether you use Jenkins, or Travis or whatev and Ansible or Puppet or whatev for automation. You probably have some docker container set up for this, because this is a very tedious task for initial setup, probably way out of proportion. But in the end there needs to be a running web service for every student which they can reach over a personal URL. Depending on the students interest on the topic it may be also better to setup this already before the first class starts and only introduce them to all the concepts in a theory block and do some more coding in the second half.
Homework: Use $framework to extend your web app. Make it a bit more user interactive with buttons, forms or the like. As we still have no backend here, you can output to alert or something.
3. Create a minimal backend with $backendFramework. Only to have something which speaks with the frontend so you can create API calls going back and forth. Also create a DB, relational or not. Discuss DB schema/model and answer student questions.
Homework: Create a form which gets transformed into JSON and sent to the backend, backend stores the user information in the DB and should also provide a query to view the entry.
4. Introduce mobile apps. As it would probably too much to introduce them both to iOS and Android, something like React Native (or whatever the most popular platform-agnostic framework is then) may come in handy. Do the same as with the minimal web app and add the build artifacts to CI. Also talk about getting software to the app/play store (a common question) and signing apps.
Homework: Use the view API call from the backend to show the data on the mobile. Play around with the mobile project to display it in a nice way.
5. Introduction to refactoring (yes, really), if we are really talking about JS here, mention things like typescript, flow, elm, reason and everything with types which compiles to JS. Types make it so much easier to refactor growing codebases and imho everybody should use it.
Flowtype would make it probably easier to get gradually introduced in the already existing codebase (and it plays nice with react native) but I want to be abstract here, so that is just a suggestion (and 100% typed languages such as ELM or Reason have so much nicer errors).
Also discuss other helpful tools like linters, formatters.
Homework: Introduce types to all your API calls and some important functions.
6. Introduction to (unit) tests. Similar as above.
Homework: Write a unit test for your form.
(TBC)4 -
I to myself:
"I do not really need to add type annotations here, it is just the small coding part of my thesis!"
(about 500 LOC)
Later...
"Hmm, lets just activate flow and get the typedefs of some packages from flowtyped..."
Found 5 Errors!
Dang! -
I wrote a type checking utility that also considers all types (JS without TypeScript, so this meant arrays etc.). The desired type had to be declared in a config file and the data didn’t even come from the config.
What would I not do to prevent all possible attack vectors... -
Looking for some advice from anyone who has used and transitioned away from Material-ui (React).
https://material-ui.com/
Tasked with removing the Material-ui used in our react project. Old team took shortcuts like using these types of frameworks at every step and the software is brittle.
Any advice from anyone who has ripped Material-ui out of a project.
We are doing this to move away from CSS-in-JS and this is conflicting with every fucking element in the fucking project.
<TextField> with it's special internal props
<Buttons> with its fucking "classes" prop that one take fucking CSS-in-JS
Any advice or just say some random shit or post a GIF for the lulz.
Peace.2 -
NEED AN URGENT HELP HERE!!!
As much as I try to stay away from the satanic language that is javascript, I have to read up on it if I need to pass this semester.
Guide me towards the different types of objects in javascript, anyone?
Here's what I know so far- js treats everything as an object, but what I don't know is that are there categories? [the "everything" referring to the primitive data types]14 -
I have become very fond of React, but the pushback I've experienced when suggesting typescript is crazy.
I think this is the reason why so many enterprise apps are written in Angular. Type safety isn’t a crutch, it's a tool. Plus, interfaces are a dev time construct, and will not bloat the codebase since it doesn’t transpile into js.
But Ts also gives us a lot of other goodies that allow for cleaner design patterns and a better adoption of oop principals.
Also, generics/constructor types, whatever you wanna call it, are your friends. An Array<SomeEntity> or SomeEntity[] will give you peace of mind I’m so many scenarios.
Anyway, I have bitched enough.
Rant over.
Have a wonderful Christmas, everyone.
Ps. This isn’t aimed at anyone in particular, but a the react community as a collective. :)3 -
I think I may have just had a bit of an epiphany...
Algorithms and Data Structures are sort of like libraries and frameworks.
JS is probably the easiest example since npm has a lot of libraries.
For most cases, underscore.each and lodash.each $.each achieve pretty much the same thing except one maybe better in certain cases.
And data structures are sort of like Angular, React, Electron, ReactNative. Each has its own purpose or style and are good for solving certain types of problems?
So day-to-day you don't really care what Sort() algo you use.. unless you really need to perfoarmance tune it for a specific case.
And data structures, depending on the type of problem you have, one is easier to work/think about the problem in although not examply... Usually have to use a few of them at once.
How is this going to help me in interviews... I'm not sure... other than reconfirming that knowing specific implementations of Sort and Trees is usually BS... most of the time just need to know you should Sort or use a Tree... -
A year ago I built my first todo, not from a tutorial, but using basic libraries and nw.js, and doing basic dom manipulations.
It had drag n drop, icons, and basic saving and loading. And I was satisfied.
Since then I've been working odd jobs.
And today I've decided to stretch out a bit, and build a basic airtable clone, because I think I can.
And also because I hate anything without an offline option.
First thing I realized was I wasn't about to duplicate all the features of a spreadsheet from scratch. I'd need a base to work from.
I spent about an hour looking.
Core features needed would be trivial serialization or saving/loading.
Proper event support for when a cell, row, or column changed, or was selected. Necessary for triggering validation and serialization/saving.
Custom column types.
Embedding html in cells.
Reorderable columns
Optional but nice to have:
Changeable column width and row height.
Drag and drop on rows and columns.
Right click menu support out of the box.
After that hour I had a few I wanted to test.
And started looking at frameworks to support the SPA aspects.
Both mithril and riot have minimal router support. But theres also a ton of other leightweight frameworks and libraries worthy of prototyping in, solid, marko, svelte, etc.
I didn't want to futz with lots of overhead, babeling/gulping/grunting/webpacking or any complex configuration-over-convention.
Didn't care for dom vs shadow dom. Its a prototype not a startup.
And I didn't care to do it the "right way". Learning curve here was antithesis to experimenting. I was trying to get away from plugin, configuration-over-convention, astronaut architecture, monolithic frameworks, the works.
Could I import the library without five dozen dependancies and learning four different tools before getting to hello world?
"But if you know IJK then its quick to get started!", except I don't, so it won't. I didn't want that.
Could I get cheap component-oriented designs?
Was I managing complex state embedded in a monolith that took over the entire layout and conventions of my code, like the world balanced on the back of a turtle?
Did it obscure the dom and state, and the standard way of doing things or *compliment* those?
As for validation, theres a number of vanilla libraries, one of which treats validation similar to unit testing, which seems kinda novel.
For presentation and backend I could do NW.JS, which would remove some of the complications, by putting everything in one script. Or if I wanted to make it a web backend, and avoid writing it in something that ran like a potato strapped to a nuclear rocket (visual studio), I could skip TS and go with python and quart, an async variation of flask.
This has the advantage that using something thats *not* JS, namely python, for interacting with a proper database, and would allow self-hosting or putting it online so people can share data and access in real time with others.
And because I'm horrible, and do things the wrong way for convenience, I could use tailwind.
Because it pisses people off.
How easy (or hard) would it be to recreate a basic functional clone of the core of airtable?
I don't know, but I have feeling I'm going to find out!1