Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
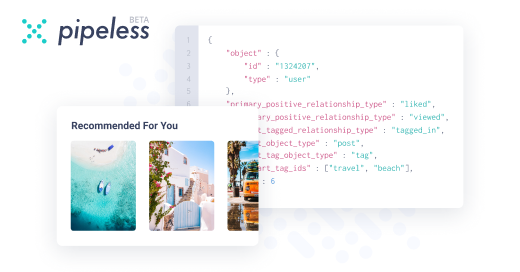
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "write before you type"
-
Every day.
I am a PHP developer.
Yeah, "another PHP is awful" rant... no, not really.
It's just unsuitable for some ambitious projects, just like Ruby and Python are.
First of all, DO NOT EVER use Laravel for large enterprise applications. The same goes for RoR, Django, and other ActiveRecord MVCs.
They are all neat frameworks for writing a todo app, as a better-than-wordpress flexible blogging solution, even as a custom webshop.
Beyond 50k daily users, Active Record becomes hell due to it's lazy fat querying habits. At more than a million users... *depressed sigh*.
PHP is also completely unsuitable for projects beyond 5M lines of code in my opinion. At more than 25M lines... *another depressed sigh*.
You can let your devs read Clean Code and books about architecture patterns, you can teach them about SOLID & DRY, you can write thousands of tests... it doesn't matter.
PHP is scaffolding, it's made of bamboo and rope. It's not brick or concrete. You can build quickly, but it only scales up to a certain point before it breaks in multiple places.
Eventually you run into patterns where even 100% test coverage still doesn't guarantee shit, because the real-life edge cases are just too complex and numerous.
When you're working on a multi-party invoicing system with adapters for various tax codes, or an availability/planning system working across timezones, or systems which implement geographical routefinding coupled to traffic, event & weather prediction...
PHP, Python, Ruby, etc are just missing types.
Every day I run into bugs which could have been prevented if you could use ADTs in a generic way in PHP. PHP7 has pretty good typehints, and they prevent a lot of messy behavior, but they aren't composable. There is no way to tell PHP "this method accepts a Collection of Users", or "this methods returns maybe either an Apple or a Pear, and I want to force the caller to handle both Apple/Pear and null".
Well, you could do that, but it requires a lot of custom classes and trickery, and you have to rewrite the same logic if you want to typehint a "Collection of Departments" instead of "Collection of Users" -- i.e., it's not composable.
Probably the biggest issue is that languages with a (mostly) structural type system (Haskell, Rust, even C#/JVM languages to some degree, etc) are much slower to develop in for the "startup" era of a project, so you grab a weak, quick prototyping language to get started.
Then, when you reach a more grown up phase, you wish you had a better type system at your disposal...28 -
Just before you, my fellow system programmer, scroll past this, let me say this:
🍬 The web is actiually simple. 🍬
Both HTML and CSS is declarative. It's all easy when you understand the concepts, learn how to be idiomatic and quit trying to do that imperative bullshit in languages that aren't imperative.
HTML is simple. You know the boilerplate: doctype, head, body, that's all. Just mark it up and do NOT look at it before you end, mark it up as it were article or something. The appearance is up to css.
CSS is simple. You may even forget bem or rscss, you're already a skilled software developer. Use common sense and your code-splitting and naming skills you gained reading The Code Complete or doing software development for years.
Forget mockups. Forget absolute positioning, forget setting width and height in pixels. Go to awwwards, find some inspiration. Draw some buttons and fields on paper with your good old pencil. Then go and write some css. Feel free to steal some shadows and transitions from codepen.
Read about 8-pixel grid system. Let every element push away from others by setting something like margin: 16px; and whoops! You've just got fully responsive and got great vertical rhythm without even using media queries!
Oh my god, do NEVER set width and height explicitly! Type something like button { width: 120px; } and bang! The entire web page is broken. Quit that shit. Let it resize as it should. It will resize itself to fit its contents.
HTML is by default ready for your template engine. That's how you receive data from server — as server-side rendered, plain old HTML page. On the other hand, the form element is the most axiomatic and simple way to send the data to server. That's how you send it — as plain old GET or POST that every webserver can handle.
All of there are true:
1. It's easy to get great 100% responsiveness without media queries.
2. It's easy to align items in row, it's just one line of css. Maybe two, if you still want elements to wrap, but want to use flexbox:
.parent {
display: flex;
flex-wrap: wrap;
}
3. HTML and CSS are fast by default.
4. You don't need mockups to achieve great visual experience. Mockups is imperative, web is declarative.
5. You may not even need JavaScript to make great website.
Go on, ask me a question about web! I'll ready to answer everything.21 -
Most satisfying bug I've fixed?
Fixed a n+1 issue with a web service retrieving price information. I initially wrote the service, but it was taken over by a couple of 'world class' monday-morning-quarterbacks.
The "Worst code I've ever seen" ... "I can't believe this crap compiles" types that never met anyone else's code that was any good.
After a few months (yes months) and heavy refactoring, the service still returned price information for a product. Pass the service a list of product numbers, service returns the price, availability, etc, that was it.
After a very proud and boisterous deployment, over the next couple of days the service seemed to get slower and slower. DBAs started to complain that the service was causing unusually high wait times, locks, and CPU spikes causing problems for other applications. The usual finger pointing began which ended up with "If PaperTrail had written the service 'correctly' the first time, we wouldn't be in this mess."
Only mattered that I initially wrote the service and no one seemed to care about the two geniuses that took months changing the code.
The dev manager was able to justify a complete re-write of the service using 'proper development methodologies' including budgeting devs, DBAs, server resources, etc..etc. with a projected year+ completion date.
My 'BS Meter' goes off, so I open up the code, maybe 5 minutes...tada...found it. The corresponding stored procedure accepts a list of product numbers and a price type (1=Retail, 2=Dealer, and so on). If you pass 0, the stored procedure returns all the prices.
Code basically looked like this..
public List<Prices> GetPrices(List<Product> products, int priceTypeId)
{
foreach (var item in products)
{
List<int> productIdsParameter = new List<int>();
productIdsParameter.Add(item.ProductID);
List<Price> prices = dataProvider.GetPrices(productIdsParameter, 0);
foreach (var price in prices)
{
if (price.PriceTypeID == priceTypeId)
{
prices = dataProvider.GetPrices(productIdsParameter, price.PriceTypeID);
return prices;
}
* Omitting the other 'WTF?' code to handle the zero price type
}
}
}
I removed the double stored procedure call, updated the method signature to only accept the list of product numbers (which it was before the 'major refactor'), deployed the service to dev (the issue was reproducible in our dev environment) and had the DBA monitor.
The two devs and the manager are grumbling and mocking the changes (they never looked, they assumed I wrote some threading monstrosity) then the DBA walks up..
DBA: "We're good. You hit the database pretty hard and the CPU never moved. Execution plans, locks, all good to go."
<dba starts to walk away>
DevMgr: "No fucking way! Putting that code in a thread wouldn't have fix it"
Me: "Um, I didn't use threads"
Dev1: "You had to. There was no way you made that code run faster without threads"
Dev2: "It runs fine in dev, but there is no way that level of threading will work in production with thousands of requests. I've got unit tests that prove our design is perfect."
Me: "I looked at what the code was doing and removed what it shouldn't be doing. That's it."
DBA: "If the database is happy with the changes, I'm happy. Good job. Get that service deployed tomorrow and lets move on"
Me: "You'll remove the recommendation for a complete re-write of the service?"
DevMgr: "Hell no! The re-write moves forward. This, whatever you did, changes nothing."
DBA: "Hell yes it does!! I've got too much on my plate already to play babysitter with you assholes. I'm done and no one on my team will waste any more time on this. Am I clear?"
Seeing the dev manager face turn red and the other two devs look completely dumbfounded was the most satisfying bug I've fixed.5 -
Typical Tuesday morning. Got word that a client was having trouble viewing a mp4 video, thats being used as a background element on their website, on their iphone.
No biggie, I think to myself.
An hour in Im praying to the safari Gods and cursing the existence of iOs (or however the hell you spell it).
While debugging I realise the browser gives up on downloading the video 2 seconds in, the same way I gave up watching that Netflix Neath note abomination, two seconds in.
So i quickly write up an ajax script forcing the browser to download the file before displaying it...F.I.G.J.A.M
But hold up 'webkitURL' is deprecated. Please use 'URL' instead ..dafuq ?
Okay okay I got this just use a work around for that ..aaand done.
Should be working right? Wrong (-_-)
Half an hour later searching stackoverflow like its the gospel and judgement is upon me and I found the solution..I found the solution, simple stupid solution that would make you wanna facepalm so hard that your great grandkids would have marks on their face.
Declare the MIME type in the bloody source tag in the html ... shoot me now3 -
Long rant ahead.. so feel free to refill your cup of coffee and have a seat 🙂
It's completely useless. At least in the school I went to, the teachers were worse than useless. It's a bit of an old story that I've told quite a few times already, but I had a dispute with said teachers at some point after which I wasn't able nor willing to fully do the classes anymore.
So, just to set the stage.. le me, die-hard Linux user, and reasonably initiated in networking and security already, to the point that I really only needed half an ear to follow along with the classes, while most of the time I was just working on my own servers to pass the time instead. I noticed that the Moodle website that the school was using to do a big chunk of the course material with, wasn't TLS-secured. So whenever the class begins and everyone logs in to the Moodle website..? Yeah.. it wouldn't be hard for anyone in that class to steal everyone else's credentials, including the teacher's (as they were using the same network).
So I brought it up a few times in the first year, teacher was like "yeah yeah we'll do it at some point". Shortly before summer break I took the security teacher aside after class and mentioned it another time - please please take the opportunity to do it during summer break.
Coming back in September.. nothing happened. Maybe I needed to bring in more evidence that this is a serious issue, so I asked the security teacher: can I make a proper PoC using my machines in my home network to steal the credentials of my own Moodle account and mail a screencast to you as a private disclosure? She said "yeah sure, that's fine".
Pro tip: make the people involved sign a written contract for this!!! It'll cover your ass when they decide to be dicks.. which spoiler alert, these teachers decided they wanted to be.
So I made the PoC, mailed it to them, yada yada yada... Soon after, next class, and I noticed that my VPN server was blocked. Now I used my personal VPN server at the time mostly to access a file server at home to securely fetch documents I needed in class, without having to carry an external hard drive with me all the time. However it was also used for gateway redirection (i.e. the main purpose of commercial VPN's, le new IP for "le onenumity"). I mean for example, if some douche in that class would've decided to ARP poison the network and steal credentials, my VPN connection would've prevented that.. it was a decent workaround. But now it's for some reason causing Moodle to throw some type of 403.
Asked the teacher for routers and switches I had a class from at the time.. why is my VPN server blocked? He replied with the statement that "yeah we blocked it because you can bypass the firewall with that and watch porn in class".
Alright, fair enough. I can indeed bypass the firewall with that. But watch porn.. in class? I mean I'm a bit of an exhibitionist too, but in a fucking class!? And why right after that PoC, while I've been using that VPN connection for over a year?
Not too long after that, I prematurely left that class out of sheer frustration (I remember browsing devRant with the intent to write about it while the teacher was watching 😂), and left while looking that teacher dead in the eyes.. and never have I been that cold to someone while calling them a fucking idiot.
Shortly after I've also received an email from them in which they stated that they wanted compensation for "the disruption of good service". They actually thought that I had hacked into their servers. Security teachers, ostensibly technical people, if I may add. Never seen anyone more incompetent than those 3 motherfuckers that plotted against me to save their own asses for making such a shitty infrastructure. Regarding that mail, I not so friendly replied to them that they could settle it in court if they wanted to.. but that I already knew who would win that case. Haven't heard of them since.
So yeah. That's why I regard those expensive shitty pieces of paper as such. The only thing they prove is that someone somewhere with some unknown degree of competence confirms that you know something. I think there's far too many unknowns in there.
Nowadays I'm putting my bets on a certification from the Linux Professional Institute - a renowned and well-regarded certification body in sysadmin. Last February at FOSDEM I did half of the LPIC-1 certification exam, next year I'll do the other half. With the amount of reputation the LPI has behind it, I believe that's a far better route to go with than some random school somewhere.25 -
That's actually something that happened fairly recently.. just that I didn't have the energy left at the time to write it down. That, or I got my ass too drunk to properly write anything.. not sure actually.
So on paper I'm unemployed, but I do spend some time still on pretty much voluntary work for HackingVision, along with a handful of other people.
At the time, we were just doing the usual chit-chat in the admin channel, me still sick in my bed (actually that means that I wasn't drunk but really tired for once.. amazing!) and catching up to what happened, but unable to do any useful work in this sick state. So, tablet, typing on glass, right. I didn't have any keyboard attached at the time.
One of the staff members (a wanketeer from India) apparently had an assignment in a few hours for which he needed to write a server application in Java. Now, performance issues aside, I figured.. well I've got quite a bit of experience with servers, as well as some with client-server protocols. So I got thinking.. mail servers, way too overengineered. Web servers.. well that could work, I've done some basic netcat webservers that just sent an HTTP 200 OK and the file, those worked fine.. although super basic of course. And then there's IRC, which I've actually talked to an InspIRCd server through telnet before (which by the way is pretty much the only thing that telnet is still useful for, something that was never its purpose, lol) and realized that that protocol is actually quite easy to develop around. That's why I like it so much over modern chat protocols like XMPP, MQTT and whatnot. So I recommended that he'd write a little IRC server in Java. Or even just a chatbot like I attempted to at the time, considering that that's - with a stretch of course - a sort-of server too.
His fucking response however, so goddamn fucking infuriating. "If the protocol is so easy, then please write me down how to implement it in Java."
Essentially do his fucking work for him. I don't know Java, but as a fucking HackingVision admin, YOU SHOULD FUCKING KNOW THAT HACKERS CAN'T STAND LAZY CUNTS THAT CAN'T EVEN BE ASSED TO GOOGLE SHIT!!! If I wanted to deal with cunts like that, I'd have opened the page inbox with all its Fb h4xx0ring questions, not the fucking admin chat!
And type it on a goddamn fucking piece of glass, while fucking sick?! Get your ass fucked by a bobs and vegana horny fuck from the untouchable caste, because that's where you fucking belong for expecting THAT from me, you fucking bhenchod.
But at least I didn't get my ass enraged like that to say that to him in the admin chat. Although that probably wouldn't have been a bad thing, to get his feet right back on the ground again.1 -
Dynamically typed languages are barbaric to me.
It's pretty much universally understood that programmers program with types in mind (if you have a method that takes a name, it's a string. You don't want a name that's an integer).
Even it you don't like the verbosity of type annotations, that's fine. It adds maybe seconds of time to type, which is neglible in my opinion, but it's a discussion to be had.
If that's the case, use Crystal. It's statically typed, and no type annotations are required (it looks nearly identical to Ruby).
So many errors are fixed by static typing and compilers. I know a person who migrated most of the Python std library to Haskell and found typing errors in it. *In their standard library*. If the developers of Python can't be trusted to avoid simple typing errors with all their unit tests, how can anyone?
Plus, even if unit testing universally guarded against typing errors, why would you prefer that? It takes far less time to add a type annotation (and even less time to write nothing in Crystal), and you get the benefit of knowing types at compile time.
I've had some super weird type experiences in Ruby. You can mock out the return of the type check to be what you want. I've been unit testing in Ruby before, tried mocking a method on a type, didn't work as I expected. Checked the type, it lines up.
Turns out, nested away in some obscure place was a factory that was generating types and masking them as different types because we figured "since it responds to all the same methods, it's practically the same type right?", but not in the unit test. Took 45 minutes on my time when it could've taken ~0 seconds in a statically typed language.11 -
I was pressued to shift the blame.
We received an angry email from a customer that some of their data had disappeared. The boss assigns me to this task. This feature is relatively new and we've found some bugs in the past in here. I go through request logs, search the database, run some diagnostics, etc. for about 5 hours and I cannot find the problem. I focus on the bugs that we've had before but they don't seem to be the problem.
I tell the boss "sorry but I checked XYZ and I can't find the problem. I'm out of ideas." But the boss wanted answers by the end of the day. They did not want to admit to the client that we couldn't figure out what's wrong.
By now I was more pressured to find an answer, find something or someone to blame it on, not exactly to find the real solution. So I made up some BS:
"Sometimes, in HTML forms, the number inputs allow you to change the number by scrolling. We have some long forms where the user has to scroll. Perhaps the focus remained on the number input, so when they scrolled down they accidentally changed the number they meant to input."
The boss was happy with that. We explained this to the customer, and there's now a ticket to change type="number" to type="text" in our HTML forms and to validate it in th backend.
A week later another customer shows us a different error. This one is more clear because it had a stack trace, but I realise that this error is what caused our last error. It was pretty obscure, mind you, the unit tests didn't detect it.
I didn't tell the boss that they were connected tho.
With two angry clients in two weeks, I finally convinced the boss to give us more time to write more unit tests with full coverage. -
I don't know if I'm being pranked or not, but I work with my boss and he has the strangest way of doing things.
- Only use PHP
- Keep error_reporting off (for development), Site cannot function if they are on.
- 20,000 lines of functions in a single file, 50% of which was unused, mostly repeated code that could have been reduced massively.
- Zero Code Comments
- Inconsistent variable names, function names, file names -- I was literally project searching for months to find things.
- There is nothing close to a normalized SQL Database, column ID names can't even stay consistent.
- Every query is done with a mysqli wrapper to use legacy mysql functions.
- Most used function is to escape stirngs
- Type-hinting is too strict for the code.
- Most files packed with Inline CSS, JavaScript and PHP - we don't want to use an external file otherwise we'd have to open two of them.
- Do not use a package manger composer because he doesn't have it installed.. Though I told him it's easy on any platform and I'll explain it.
- He downloads a few composer packages he likes and drag/drop them into random folder.
- Uses $_GET to set values and pass them around like a message contianer.
- One file is 6000 lines which is a giant if statement with somewhere close to 7 levels deep of recursion.
- Never removes his old code that bloats things.
- Has functions from a decade ago he would like to save to use some day. Just regular, plain old, PHP functions.
- Always wants to build things from scratch, and re-using a lot of his code that is honestly a weird way of doing almost everything.
- Using CodeIntel, Mess Detectors, Error Detectors is not good or useful.
- Would not deploy to production through any tool I setup, though I was told to. Instead he wrote bash scripts that still make me nervous.
- Often tells me to make something modern/great (reinventing a wheel) and then ends up saying, "I think I'd do it this way... Referes to his code 5 years ago".
- Using isset() breaks things.
- Tens of thousands of undefined variables exist because arrays are creates like $this[][][] = 5;
- Understanding the naming of functions required me to write several documents.
- I had to use #region tags to find places in the code quicker since a router was about 2000 lines of if else statements.
- I used Todo Bookmark extensions in VSCode to mark and flag everything that's a bug.
- Gets upset if I add anything to .gitignore; I tried to tell him it ignores files we don't want, he is though it deleted them for a while.
- He would rather explain every line of code in a mammoth project that follows no human known patterns, includes files that overwrite global scope variables and wants has me do the documentation.
- Open to ideas but when I bring them up such as - This is what most standards suggest, here's a literal example of exactly what you want but easier - He will passively decide against it and end up working on tedious things not very necessary for project release dates.
- On another project I try to write code but he wants to go over every single nook and cranny and stay on the phone the entire day as I watch his screen and Im trying to code.
I would like us all to do well but I do not consider him a programmer but a script-whippersnapper. I find myself trying to to debate the most basic of things (you shouldnt 777 every file), and I need all kinds of evidence before he will do something about it. We need "security" and all kinds of buzz words but I'm scared to death of this code. After several months its a nice place to work but I am convinced I'm being pranked or my boss has very little idea what he's doing. I've worked in a lot of disasters but nothing like this.
We are building an API, I could use something open source to help with anything from validations, routing, ACL but he ends up reinventing the wheel. I have never worked so slow, hindered and baffled at how I am supposed to build anything - nothing is stable, tested, and rarely logical. I suggested many things but he would rather have small talk and reason his way into using things he made.
I could fhave this project 50% done i a Node API i two weeks, pretty fast in a PHP or Python one, but we for reasons I have no idea would rather go slow and literally "build a framework". Two knuckleheads are going to build a PHP REST framework and compete with tested, tried and true open source tools by tens of millions?
I just wanted to rant because this drives me crazy. I have so much stress my neck and shoulder seems like a nerve is pinched. I don't understand what any of this means. I've never met someone who was wrong about so many things but believed they were right. I just don't know what to say so often on call I just say, 'uhh..'. It's like nothing anyone or any authority says matters, I don't know why he asks anything he's going to do things one way, a hard way, only that he can decipher. He's an owner, he's not worried about job security.13 -
I started thinking and worrying about numbers much more than before
in the US, you write numbers like this:
1,000.00
in Germany usually like this:
1.000,00
and in programming languages like this:
1000.00
now i wonder how to type a number, whenever i have to use german software
should i use the US way, the german way or the dev way? the wrong one could possibly break it11 -
TL;DR: Stop using React for EVERYTHING. It's not the end-all solution to every application need.
My team is staffed about 50/50 with tenured devs, and junior devs who have never written a full application and don't understand the specific benefits of different libraries/framworks. As a result, most of these junior devs have jumped on the React train, and they're under the impression that React is the end-all answer to any possible application need. Doesn't matter what type of app is, what kind of data is going to be flowing through the app, data scale, etc. In their eyes, React is always the answer. Now, while I'm not a big fan of React myself, I will say that it does its job when its tasked with a data-heavy application that needs to be refreshed/re-rendered dynamically and frequently (like Facebook.) However, my main gripe is that some people insist on using it for EVERYTHING. They refuse to acknowledge that there can be better library/framework choices (Angular, Vue, or even straight jQuery,) and they refuse to learn any other frameworks. You can hit them with countless technical reasons as to why React isn't a good choice for a particular application, and they'll just spout off the same tidbits from the "ReactJS Makes My Nips Hard 101" handbook: "React is the future," "Component-based web architecture is the future," (I'm not arguing with that last one) "But...JSX bro.," "Facebook and Netflix use it, so that's how you know it's amazing." They'll use React for a simple app, and make it overly-complex, and take months to write something that should have taken them a week. For example, we have one dev who has never used any other frameworks/libraries apart from React, and he used React (via create-react-app) to write what is effectively a single form and a content widget inside of a bootstrap template. It took him 4 MONTHS to write this, and it still isn't fully functioning. The search functionality doesn't really work (in fact, it's just array filtering,) and wont return any results if you search for the first word in an entry. His repo is a mess, filled with a bunch of useless files that were bootstrap'd in via create-react-app. We've built apps like this in a week in the past using different libraries/frameworks, and he could have done the same if he didn't overly-complicate the project by insisting on using React. If your app is essentially a dynamic form, you don’t need a freaking virtual DOM.
This happens every time a big new framework hits the scene. New young developers get sucked into it, because it's the cool hip new framework (or in React's case, library.) and they use it for everything, even when it's not the best choice. It happened with Angular, Rails, and now it's happening with React.
React has its benefits, but please please please consider which library/framework is the best choice from a technical standpoint before immediately jumping on the React train because "Facebook uses it bro."2 -
love-hate relationship with Python semi-rant
The year is 2020.
I have already grown accustomed to the idea that in order to do ML without worrying too much about having to completely jump through hoops with the tech stack I have chosen that I would have to settle with Python, which I like.....for small scripts that don't do much other than piping data around or doing simple admin tasks, that is generally our use of Python at work.
For anything bigger I would prefer something else. Not because I find anything inherently horrible in Python, I find it to be a nice language overall, that has made it possible for many to find a passion inside of the world of development and possibly an interesting in overall engineering and computer science principles. Much respect Python, good game Guido VR, what you did changed the world.
But it is that damn whitespace that gets me, the need to use it as a way to properly write blocks, I just can't make myself like syntactical whitespace no matter what I do. I can do without static typing, shit I did it for the longest time with JS way tf before Node and Typescript were a thing, and I have done it before PHP's attempt at having type hints, which still leave much to be desired. Ruby(imho) the most elegant language around doesn't have it and that is fine really, it does not bother me as much, if mypy gets powerful and widely adopted enough it will then be a non-issue.
But another thing that the 4 languages i mentioned before have is non-existent syntactical whitespace......I just can't stand it.
So, why am I saying all of this nonsense? Today I wanted to recreate a conda environment and landed on the use of YAML............which has syntactic whitespace and I lost my shit.
I seldom bitch about languages and technologies, shit, I used VBScript before, not only did I get paid handsomely for it, but I fucking enjoyed it(probably cuz I am a masochist).
But two things I cannot abide: VBA and syntactic whitespace.
Once I get enough knowledge for it I will push for the same level of tooling in Python to be ported to Scala.
Thank you for coming to my whiny post about something as small as bitching about syntactic whitespace.8 -
Look, I get that it's really tricky to assess whether someone is or isn't skilled going solely by their profile.
That's alright.
What isn't center of the cosmic rectum alright with the fucking buttsauce infested state of interviews is that you give me the most far fetched and convoluted nonsense to solve and then put me on a fucking timer.
And since there isn't a human being on the other side, I can't even ask for clarification nor walk them through my reasoning. No, eat shit you cunt juice swallowing mother fucker, anal annhilation on your whole family with a black cock stretching from Zimbabwe to Singapore, we don't care about this "reasoning" you speak of. Fuck that shit! We just hang out here, handing out tricks in the back alley and smoking opium with vietnamese prostitutes, up your fucking ass with reason.
Let me tell you something mister, I'm gonna shove a LITERAL TON of putrid gorilla SHIT down your whore mouth then cum all over your face and tits, let's see how you like THAT.
Cherry on top: by the time I began figuring out where my initial approach was wrong, it was too late. Get that? L'esprit d'escalier, bitch. I began to understand the problem AFTER the timer was up. I could solve it now, except it wouldn't do me any fucking good.
The problem? Locate the topmost 2x2 block inside a matrix whose values fall within a particular range. It's easy! But if you don't explain it properly, I have to sit down re-reading the description and think about what the actual fuck is this cancerous liquid queef that just got forcefully injected into my eyes.
But since I can't spend too much time trying to comperfukenhend this two dollar handjob of a task, which I'd rather swap for teabagging a hairy ass herpes testicle sack, there's rushing in to try and make sense of this shit as I type.
So I'm about 10 minutes down or so already, 35 to go. I finally decipher that I should get the XY coords of each element within the specified range, then we'll walk an array of those coordinates and check for adjacency. Easy! Done, and done.
Another 10 minutes down, all checks in place. TEST. Wait, wat? Where's the output? WHERE. THE FUCK. IS. THE OUTPUT?! BITCH GIMME AN ANSWER. I COUT'D THE RETURN AND CAN SEE THE TERMINAL BUT ITS NOT SHOWING ME ANYTHINGGG?! UUUGHHH FUCKKFKFKFKFKFKFKFUFUFUFFKFK (...)
Alright, we have about 20 minutes left to finish this motorsaw colonoscopy, and I can't see what my code is outputting so I'm walking through the code myself trying to figure out if this will work. Oh, look at that I have to MANUALLY click this fucking misaligned text that says "clear" in order for any new output to register. Lovely, 10/10 web design, I will violate your armpits with an octopus soaked in rabid bear piss.
Mmmh, looks like I got this wrong. Figures. I'm building the array of coordinates sequentially, as a one dimentional list, which is very inconvenient for finding adjacent elements. No problem, let's try and fix that aaaaaand... SHIT IM ALMOST OUT OF TIME.
QUICK LYEB, QUICK!! REMEMBER WHAT FISCELLA TAUGHT YOU, IN BETWEEN MOLESTING YOUR SOUL WITH 16-BIT I/O CONSOLE PROBLEMS, LIKE THAT BITCH SNOWFALL THING YOU HAD TO SOLVE FOR A FRIEND USING TURBO C ON A FUCKING TOASTER IN COMPUTER LAB! RUN MOTHERFUCKER RUN!!!
I'm SWEATING. HEAVILY. I'm STEAMING, NON-EROTICALLY. Less than 10 minutes left. I'm trying to correct the code I have, but I start making MORE dumbfuck mistakes because I'm in a hurry!
5 minutes left. As I hit this point of no return, I realize exactly where my initial reasoning went wrong, and how I could fix it, but I can't because I don't have enough time. Sadface.
So I hastily put together skeleton of the correct implementation, and as the clock is nearly up, I write a comment explaining the bits I can't get to write. Page up, top of file, type "the editor was shit LMAO" and comment it out. SUBMIT.
This violent tale of brain damaged badmouth schizoid baby versus badly worded code challenges was brought to you by ButtholeSuffers. Tired of taking low-quality viagra before engaging in unprotected anal sex? Then try ButtholeSuffers, the new way to strengthen your everday erections! You'll be as fucking HARD as a WALL!
Visit triple doble minus you dot triple doble YOU dot doble-u doble www dotbit lyshAdy wwwwww academy smashlikeachamp ai/professional/$$%$X$/0FD0EFF~ \*¨-`++ ifyouclickurstupid for for a FREE coupon to get MINUS NaN OFF on a close-encounter with an inter-continental dick, and use my promo code HOPONBITCH if you'd like it *RAMMED* --FAR-- and D E E P L Y.
(lel ad break should continue I'm cutting it shortt) [CENSORED] grants *physical* access to your pants! Big ups to Annihilate for sponsoring this mental breakdown.
Also hi ;>5 -
Nope, definitely not going to work for that customer anymore. Fuck this shit. At least for this week.
My background: mid-30 years old, some kind of business & IT consultant / lead dev working for a mid sized CRM consulting company, with approx 15 years of experience in development and software architecture, most of the time "thinking" in C#, still learning new languages, being a cloud evangelist and team lead. We usually have customers with customers (B2B/B2C).
Personality type "campaigner" (ENFP-A).
Today the project lead of my client (a big corporation in the energy industry) told me that he still didn't order all the necessary resources for the cloud project. Just to be clear: He's on the client side. We (the architects, one internal and me) told him one month ago what we need for the beginning. Just a few things - an Azure subscription, a license for the CRM platform, and our dev tools.
And now let's guess when the project is planned to begin? Yeah, right: 1st of April. NO APRIL'S FOOL. And guess what? Next Tuesday we'll do the onboarding for the new (external) devs, and NOTHING will be ready. Yeah, just let us build stuff in our minds, and on the whiteboards, because it's an AGILE project, right? We don't need any systems and tools...
And now he sent me the questionnaires which need to be answered before any cloud service can be ordered by the corporate IT. And yes, he didn't answer a single thing, and just meant "Those are architecture questions" (they are not) and (of course) "please provide the answers until Monday morning, so we can FINALLY order the services."
Yeah, you fucktard. Of course it's MY FAULT now. Maybe I should write an email to your boss asking how we can speed things up a little bit...3 -
Everyone and their dog is making a game, so why can't I?
1. open world (check)
2. taking inspiration from metro and fallout (check)
3. on a map roughly the size of the u.s. (check)
So I thought what I'd do is pretend to be one of those deaf mutes. While also pretending to be a programmer. Sometimes you make believe
so hard that it comes true apparently.
For the main map I thought I'd automate laying down the base map before hand tweaking it. It's been a bit of a slog. Roughly 1 pixel per mile. (okay, 1973 by 1067). The u.s. is 3.1 million miles, this would work out to 2.1 million miles instead. Eh.
Wrote the script to filter out all the ocean pixels, based on the elevation map, and output the difference. Still had to edit around the shoreline but it sped things up a lot. Just attached the elevation map, because the actual one is an ugly cluster of death magenta to represent the ocean.
Consequence of filtering is, the shoreline is messy and not entirely representative of the u.s.
The preprocessing step also added a lot of in-land 'lakes' that don't exist in some areas, like death valley. Already expected that.
But the plus side is I now have map layers for both elevation and ecology biomes. Aligning them close enough so that the heightmap wasn't displaced, and didn't cut off the shoreline in the ecology layer (at export), was a royal pain, and as super finicky. But thankfully thats done.
Next step is to go through the ecology map, copy each key color, and write down the biome id, courtesy of the 2017 ecoregions project.
From there, I write down the primary landscape features (water, plants, trees, terrain roughness, etc), anything easy to convey.
Main thing I'm interested in is tree types, because those, as tiles, convey a lot more information about the hex terrain than anything else.
Once the biomes are marked, and the tree types are written, the next step is to assign a tile to each tree type, and each density level of mountains (flat, hills, mountains, snowcapped peaks, etc).
The reference ids, colors, and numbers on the map will simplify the process.
After that, I'll write an exporter with python, and dump to csv or another format.
Next steps are laying out the instances in the level editor, that'll act as the tiles in question.
Theres a few naive approaches:
Spawn all the relevant instances at startup, and load the corresponding tiles.
Or setup chunks of instances, enough to cover the camera, and a buffer surrounding the camera. As the camera moves, reconfigure the instances to match the streamed in tile data.
Instances here make sense, because if theres any simulation going on (and I'd like there to be), they can detect in event code, when they are in the invisible buffer around the camera but not yet visible, and be activated by the camera, or deactive themselves after leaving the camera and buffer's area.
The alternative is to let a global controller stream the data in, as a series of tile IDs, corresponding to the various tile sprites, and code global interaction like tile picking into a single event, which seems unwieldy and not at all manageable. I can see it turning into a giant switch case already.
So instances it is.
Actually, if I do 16^2 pixel chunks, it only works out to 124x68 chunks in all. A few thousand, mostly inactive chunks is pretty trivial, and simplifies spawning and serializing/deserializing.
All of this doesn't account for
* putting lakes back in that aren't present
* lots of islands and parts of shores that would typically have bays and parts that jut out, need reworked.
* great lakes need refinement and corrections
* elevation key map too blocky. Need a higher resolution one while reducing color count
This can be solved by introducing some noise into the elevations, varying say, within one standard div.
* mountains will still require refinement to individual state geography. Thats for later on
* shoreline is too smooth, and needs to be less straight-line and less blocky. less corners.
* rivers need added, not just large ones but smaller ones too
* available tree assets need to be matched, as best and fully as possible, to types of trees represented in biome data, so that even if I don't have an exact match, I can still place *something* thats native or looks close enough to what you would expect in a given biome.
Ponderosa pines vs white pines for example.
This also doesn't account for 1. major and minor roads, 2. artificial and natural attractions, 3. other major features people in any given state are familiar with. 4. named places, 5. infrastructure, 6. cities and buildings and towns.
Also I'm pretty sure I cut off part of florida.
Woops, sorry everglades.
Guess I'll just make it a death-zone from nuclear fallout.
Take that gators!5 -
This weekly is getting really popular with so many rants coming in.
Shows the extent to which real coders/programmers/developers are tormented by the incompetent education system.
Here is my two cents:
Students should be taught to build their logic on paper before jumping on to their keyboards.2 -
I hate the new android update. Cause my phone is even more Google, than it was before. Since I like some services they offer I wouldn't complain. But they are going way to far... For instance I now have a Google keyboard and it is actually pretty good in suggesting words, but sometimes it just autocorrects my text and swaps words I wrote.
Like:
'Hey, you don't know as good what you wanted to write as I do. Let me correct that for you!'
Just let me type my own thoughts, you smart bitch of a software. And also fuck you.5 -
I don't scream because my teams are in a different country and we communicate by IM and email.
I do write long ranting/passive-aggressive emails or type really quick replies when I'm pissed though. .
An example of the latter:
Boss: I need you to make a "quick" fix...
Me: hmm ok sounds like we should implement it like ...
Boss: I was thinking something like this... since it's a temp fix
Need: (typing faster) why is it a temp fix... why not builds it properly so it can be reused
Boss: but that takes time, this is quicker
Me: it's bad design because ... (Typing so fast I'm making typos)... Anyway I can do it. This is better...
Boss: ... ok fine... if you can finish it before deadline6 -
Over the summer I was recruited to be a supplement instructor for a data structures course. As a result of that I was asked (separately by the professor) to be a grader for the course. Because of pay limitations I've mostly been grading homework project assignments. In any case, it's a great job to get my foot into the department and get recognized.
Over the course of the semester I've had this one person, OSX, named after their operating system of choice, who has been giving me awkward submissions. On the first assignment they asked the professor for extra time for some reason or the other, and that's perfectly fine.
So I finally receive OSX's submission, and it's a .py file as per course of the course. So I pop up a terminal in the working directory and type "python OSX_hw1.py". Get some error spit out about the file not being the right encoding. I know that I can tell python to read it in a different encoding, so I open it up in a text editor. To my surprise it's totally not a text file, but rather a .zip file!
I've seen weirder things done before, so no big deal. I rename the file extension, and open it up to extract the files when I see that there's no python files. "Okay, what's goin on here OSX..." I think to myself.
Poking around in the files it appears to be some sort of meta-data. To what, I had no clue, but what I did find was picture files containing what appeared to be some auto-generated screenshots of incomplete code. Since I'm one to give people the benefit of doubt even when they've long exhausted other peoples', I thought that it must be some fluke, and emailed OSX along with the professor detailing my issue.
I got back a rather standard reply, one of which was so un-notable I could not remember it if my life depended on it. However, that also meant I didn't have to worry about that anymore. Which when you're juggling 50 bazillion things is quite a relief. Tragically, this relief was short lived with the introduction of assignment 2.
Assignment 2 comes around, and I get the same type of submission from OSX. At this time I also notice that all their submissions are *very* close to the due time of 11:59pm (which I don't care about as long as it's in before people start waking up the next morning). I email OSX and the professor again, and receive a similar response. I also get an email from OSX worried about points being deducted. I reply, "No issue. You know what's wrong. Go and submit the right file on $CentralGradingCenter. Just submit over your old assignment".
To my frustration OSX claimed to not know how to do this. I write up a quick response explaining the process, and email it. In response OSX then asks if I can show them if they comes to my supplemental lesson. I tell OSX that if they are the only person, sure, otherwise no because it would not be a fair use of time to the other students.
OSX ends up showing up before anyone else, so I guide them through the process. It's pretty easy, so I'm surprised that they were having issues. Another person then shows up, so I go through relevant material and ask them if they have any questions about recent material in class. That said, afterwards OSX was being somewhat awkward and pushy trying to shake my hand a lot to the point of making me uncomfortable and telling them that there's no reason to be so formal.
Despite that chat, I still did not see a resubmission of either of those two assignments, and assignment 3 began to show it's head. Obviously, this time, as one might expect after all those conversations, I get another broken submission in the same format. Finally pissed off, I document exactly how everything looks on my end, how the file fails to run, how it's actually a zip file, etc, all with screenshots. That then gets emailed to the professor and OSX.
In response, I get an email from OSX panicking asking me how to submit it right, etc, etc. However, they also removed the professor from the CC field. In response I state that I do not know how to use whatever editor they are using, and that they should refer to the documentation in order to get a proper runnable file. I also re-CC the professor, making sure OSX's email to me is included in my reply.
OSX then shows up for one of my lessons, and since no one had shown up yet, I reiterate through what I had sent in the email. OSX's response was astonished that they could ever screw up that bad, but also admits that they had yet to install python(!!!). Obviously, the next thing that comes from my mouth is asking OSX how they write their code. Their response was that they use a website that lets them run python code.
At this point I'm honestly baffled and explain that a lot of websites like those can have limitations which might make code run differently then it should (maybe it's a simple interpreter written on JavaScript, or maybe it is real python, but how are you supposed to do file I/O?) .
After that I finally get a submission for assignment 1! -
I've been inspired by cpg grey (I think that's the name), mainly his 10 tips on how to be miserable, to write a blog or some kind of post explaining the 8 things you need to do if you hate your coworkers and want your codebase to go to shit.
So it'll be like a anti-SOLID, anti-pattern type of blog promoting every code smell imaginable
I feel like 8 tips to fuck up your codebase (php perhaps) might he more memorable than actual helpful advise. I'm sure that this has been done before, but I was wondering:
Do you think this would be effective? Would it help people understand why not to do the 8 tips? Does this reverse psychology work?3 -
I decided to upgrade my intellij ultimate from 2019.3 to 2020.2 and I saw there is update button.
I clicked on it.
As I expected it didn’t work and it was 30 minutes waiting looking at progress bar going back and forth couple of times before I decided just to download latest version and drag and drop it to applications folder ( took me 5 minutes) - I use mac so it replaces all crap ( I think ).
I cleared the old cache that growed to 2 gigabytes leaving some configuration files.
Next as always crash on startup cause of incompatible plugins with long java stacktrace - at least I could click the close button or popup closed itself I can’t remember ( one version I remember this button couldn’t be clicked cause it was off the screen and you need to do some cheating to launch ide )
The font has changed and I see that it at least work a little faster - that is nice. Indexing is finally fixed after all those years - probably thanks to visual studio code intellisense pushing those lazy bastards to deal with this.
But the preloader on first logo disappears so I think they decided to remove it cause it’s so fast - no it loads the same time or maybe little longer when I launch it on my old macbook.
After that as always I looked at plugins to see if there’s something interesting, so to find ability to scroll over whole plugins I needed to click couple of times. I think they assume I remember all the nice plugins in their marketplace and I only type search.
Maybe I should be type of user who reads best 2020 plugins for your best ide crap articles filled with advertising or even waste more time to watch all of this great videos about ide ( are there any kind of this stuff ? )
After a few operations I unfortunately clicked apply instead of restart ide and it hanged up on uninstalling some plugin I’m no longer interested in for 5 minutes so I decided to use always working ‘kill -9’ from command line.
Launched again and this time success.
Fortunately indexing finished for this workspace and I can work.
I’m intellij ultimate subscriber for 7+ years and I see those craps are not changing from like forever.
What’s the point of automate something that you can’t regression test ?
I started thinking that now when most people are facebook wall scrolling zombies companies assume that when new software comes out everyone is installing it right away and if not they’re probably not our customers cause they’re dead.
What a surprise they have when I pay for another year I can only imagine ( to be fair probably they even don’t know who I am ).
Yeah for sure I am subscribed to newsletters and I have jetbrains as a start page cause I shit myself with money and have nothing better to do then be grupie ( is there corporate grupies already a big community? )
Well I am a guy who likes to spend some time when installing anything and especially software that is responsible for my main source of income and productivity speed up.
Anyway I decided to upgrade cause editing es7 and typescript got to be pain in the ass and I see it’s working fine now. I don’t know if I like the font but at least the editor it’s working the same or maybe faster then the original that is huge improvement as developers lose most of their time between keyboard and screen communication protocol.
I don’t write it to discourage intellij as it’s great independent ide that I love and support for such a long time but they should focus on code editor and developers efficiency not on things that doesn’t make sense.
Congratulations if you reached this point of this meaningless post.
Now I started thinking that maybe it’s working faster cause I removed 2 gigs of crap from it.
Well we’ll see. -
I really hate PHP frameworks.
I also often write my own frameworks but propriety. I have two decades experience doing without frameworks, writing frameworks and using frameworks.
Virtually every PHP framework I've ever used has causes more headaches than if I had simply written the code.
Let me give you an example. I want a tinyint in my database.
> Unknown column type "tinyint" requested.
Oh, doctrine doesn't support it and wont fix. Doctrine is a library that takes a perfectly good feature rich powerful enough database system and nerfs it to the capabilities of mysql 1.0.0 for portability and because the devs don't actually have the time to create a full ORM library. Sadly it's also the defacto for certain filthy disgusting frameworks whose name I shan't speak.
So I add my own type class. Annoying but what can you do.
I have to try to use it and to do so I have to register it in two places like this (pseudo)...
Types::add(Tinyint::class);
Doctrine::add(Tinyint::class);
Seems simply enough so I run it and see...
> Type tinyint already exists.
So I assume it's doing some magic loading it based on the directory and commend out the Type::add line to see.
> Type to be overwritten tinyint does not exist.
Are you fucking kidding me?
At this point I figure out it must be running twice. It's booting twice. Do I get a stack trace by default from a CLI command? Of course not because who would ever need that?
I take a quick look at parent::boot(). HttpKernel is the standard for Cli Commands?
I notice it has state, uses a protected booted property but I'm curious why it tries to boot so many times. I assume it's user error.
After some fiddling around I get a stack trace but only one boot. How is it possible?
It's not user error, the program flow of the framework is just sub par and it just calls boot all over the place.
I use the state variable and I have to do it in a weird way...
> $booted = $this->booted;parent::boot();if (!$booted) {doStuffOnceThatDependsOnParentBootage();}
A bit awkward but not life and death. I could probably just return but believe or not the parent is doing some crap if already booted. A common ugly practice but one that works is to usually call doSomething and have something only work around the state.
The thing is, doctrine does use TINYINT for bool and it gets all super confused now running commands like updates. It keeps trying to push changes when nothing changed. I'm building my own schema differential system for another project and it doesn't have these problems out of the box. It's not clever enough to handle ambiguous reverse mappings when single types are defined and it should be possible to match the right one or heck both are fine in this case. I'd expect ambiguity to be a problem with reverse engineer, not compare schema to an exact schema.
This is numpty country. Changing TINYINT UNSIGNED to TINYINT UNSIGNED. IT can't even compare two before and after strings.
There's a few other boots I could use but who cares. The internet seems to want to use that boot function. There's also init stages missing. Believe it or not there's a shutdown and reboot for the kernel. It might not be obvious but the Type::add line wants to go not in the boot method but in the top level scope along with the class definition. The top level scope is run only once.
I think people using OOP frameworks forget that there's a scope outside of the object in PHP. It's not ideal but does the trick given the functionality is confined to static only. The register command appears to have it's own check and noop or simply overwrite if the command is issued twice making things more confusing as it was working with register type before to merely alias a type to an existing type so that it could detect it from SQL when reverse engineering.
I start to wonder if I should just use columnDefinition.
It's this. Constantly on a daily basis using these pretentious stuck up frameworks and libraries.
It's not just the palava which in this case is relatively mild compared to some of the headaches that arise. It's that if you use a framework you expect basic things out of the box like oh I don't know support for the byte/char/tinyint/int8 type and a differential command that's able to compare two strings to see if they're different.
Some people might say you're using it wrong. There is such a thing as a learning curve and this one goes down, learning all the things it can't do. It's cripplesauce.12 -
The Zen Of Ripping Off Airtable:
(patterned after The Zen Of Python. For all those shamelessly copying airtables basic functionality)
*Columns can be *reordered* for visual priority and ease of use.
* Rows are purely presentational, and mostly for grouping and formatting.
* Data cells are objects in their own right, so they can control their own rendering, and formatting.
* Columns (as objects) are where linkages and other column specific data are stored.
* Rows (as objects) are where row specific data (full-row formatting) are stored.
* Rows are views or references *into* columns which hold references to the actual data cells
* Tables are meant for managing and structuring *small* amounts of data (less than 10k rows) per table.
* Just as you might do "=A1:A5" to reference a cell range in google or excel, you might do "opt(table1:columnN)" in a column header to create a 'type' for the cells in that column.
* An enumeration is a table with a single column, useful for doing the equivalent of airtables options and tags. You will never be able to decide if it should be stored on a specific column, on a specific table for ease of reuse, or separately where it and its brothers will visually clutter your list of tables. Take a shot if you are here.
* Typing or linking a column should be accomplishable first through a command-driven type language, held in column headers and cells as text.
* Take a shot if you somehow ended up creating any of the following: an FSM, a custom regex parser, a new programming language.
* A good structuring system gives us options or tags (multiple select), selections (single select), and many other datatypes and should be first, programmatically available through a simple command-driven language like how commands are done in datacells in excel or google sheets.
* Columns are a means to organize data cells, and set constraints and formatting on an entire range.
* Row height, can be overridden by the settings of a cell. If a cell overrides the row and column render/graphics settings, then it must be drawn last--drawing over the default grid.
* The header of a column is itself a datacell.
* Columns have no order among themselves. Order is purely presentational, and stored on the table itself.
* The last statement is because this allows us to pluck individual columns out of tables for specialized views.
*Very* fast scrolling on large datasets, with row and cell height variability is complicated. Thinking about it makes me want to drink. You should drink too before you embark on implementing it.
* Wherever possible, don't use a database.
If you're thinking about using a database, see the previous koan.
* If you use a database, expect to pick and choose among column-oriented stores, and json, while factoring for platform support, api support, whether you want your front-end users to be forced to install and setup a full database,
and if not, what file-based .so or .dll database engine is out there that also supports video, audio, images, and custom types.
* For each time you ignore one of these nuggets of wisdom, take a shot, question your sanity, quit halfway, and then write another koan about what you learned.
* If you do not have liquor on hand, for each time you would take a shot, spank yourself on the ass. For those who think this is a reward, for each time you would spank yourself on the ass, instead *don't* spank yourself on the ass.
* Take a sip if you *definitely* wildly misused terms from OOP, MVP, and spreadsheets.5 -
Help. I work with a guy who really wants to learn programming (he’s sales/support rn) and is even taking some courses on it. He seems eager enough to learn, the problem is he is just so fucking stupid I don’t know whether to encourage him or level with him.
He somehow managed to pass a course on Java (which I still don’t believe since I had to help him put his lines of code in the right order ffs), but now he’s signed up for C++ and data structures and I honestly don’t know how he’s going to do it.
This is the type of guy who loves “coding” but thinks debugging is a waste of time.
Normally I encourage anyone who wants to learn programming do so, but let’s be honest it does take a modicum of intelligence and this guy has zero common sense at all. We’re talking about a guy who sent me a *screenshot* of an Excel file that I needed to copy some activation codes from. And then had absolutely no idea what was wrong when I replied “are you fucking with me right now?”
*sigh*
And that’s not even scratching the surface. I sent him a zip file containing some updated code and walked him through how to update them on Slack (really basic, copy/replace files stuff). Then the VERY next day when I sent him a second update he asks “is there something you want me to do with this?”
The instructions were literally the last thing we talked about in the chat log.
I actually fear the stuff this guy would unleash upon the world if someone were actually able to teach him how to write a whole program.
What should I do? Right now my plan is to be vaguely supportive but secretly hope he will realize he’s in over his head and drop out before any damage is done. But my worry is he may just be SO dumb that he actually thinks he can do it. At that point I guess I just have to put my faith in his school and pray that they aren’t just giving degrees away to whoever can afford them. Because fear the day this guy ever gets a degree in programming.9 -
I am the responsible for the atlassian Suite at work, as I maintain the systems, set them up, and stuff.
One day, our crowd (the authentication and authorization application) just went crazy. At like lunch time it could not connect to the AD anymore. No reasons. Throwing XSRF errors (cross site scripting), because http would connect to https. "won't do it, fuck you" it told me. Out of the blue. Noone changed anything. And yea, seriously. Noone did.
It just refused to connect (as connecting to AD is connecting yourself with you own api. And refusing yourself talking to yourself). It runs behind a proxy. Therefore http/https. Well, this worked for years. But out of sudden not anymore.
Yea. Fuck you.
It was reported some hours later, at like 3pm, as people could not login to the applications using crowd as authentication and authorization server.
Tried to debug the system, where nothing was did, to make it work. At best time to fail.
First workaround: if you are logged into one of the other applications of atlassian, just refresh the site, so your SSO token gets a refresh and you are signed on again.
Then I searched more and more. And more.
But nothing worked, nothing helped.
So I addressed an emergency maintenance, take down the whole Suite, restart crowd, to apply some changes to it's settings, not knowing what happening then, because all connections of SSO will then be released. Sent out the mail like 30 minutes beforehands.
While waiting for the window, I just typed my credentials... And redid, and redid, so to type and being bored.
Three minutes before the window...
It just worked again.
Well. Wtf. Serioudl
Just came back.
No Intrusion, no changes at all. Just came back, as nothing has happened.
Kind of best part of this story... A headhunter messaged me on my way home to offer me a job as an Atlassian Suite SysAdmin for a company, at kinda the double of my salary.
At first I was thinking to go there, and when someone then asked me sth about Atlassian just start to laugh and then leave still laughing...
But then I very nicely respond that I dont want to cry at work. And wished him best luck.
I am doing some bad upgrades now on our Suite. Very painful.
And I looked into the start scripts. Some Look like the untalented intern tells another one to write scripts. Seriously wtf.
Today I followed the guide to Update a confluence and change database to Postgres. Didnt work, Postgres error.
Try it again, jquery won't load. Next try, tomcat not starting anymore. Did same thing. Every fucking time.
Yea. Maintenance window to get a nice new export soon. Will only take an hour.
To switch database in confluence, you need to set it up very fresh. And then Import your export.
Export takes an hour at our system.
Importing maybe the same time. Hope it will work (hint: Nope).
Oh, can be nice also. Just tell the Bitbucket to migrate databases, there is a fucking setting for it. Enter new database, ready, go, finished.
At least they don't raise costs very much every kinda year.
Oh sorry, yes, they do.4 -
I'm very much a TTRPG fiend, as you probably already know, and I will maintain until the day I die that playing narrative games with other humans is the absolute best way to play.
But someone sent me a link to some kind of (not-really-so) 'smart' chatbot assistant or some shit like that, saying hey, your rulebook is simple, you should introduce this bitch to it -- dump some lore on it, have it run a game, and see how well it holds up. To which I replied it's bound to get confused, but after a bit of back and forth, they convinced me and I gave it a try.
So first things first: it got the gist of it with relative ease when questioned directly, but when running a game the mother fucker just kept making shit up and bending the rules. Experiment failed, essentially.
But what did I do? I wrote a second, stripped-down version of the rulebook that simply accounted for and embraced the idiot bot's proclivity for bullshit. This meant scrapping 98% of the mechanics, mind you: I dumbed it down as much as I could without destroying the core essence of the game.
I expected a repeat of the initial result, but to my suprise, once given the new edition the bot actually started following the rules more or less correctly and consistently. What happened next was actually kind of interesting: without being prompted to do this, the mother fucker started using spells against me and my party, constantly attempting to manipulate us to serve some nefarious, evil break-and-reshape the world type goal.
So, lythecnics primer: the WORD is all, and as such, there is no real differentiation between affecting the world through speech or casting a spell -- in truth, it's all a matter of degree. That is to say, language has the power to shape the world around us, in both subtle and overt ways. The entire system revolves around this, it's a mix of funky philosophical musings and abrahamic sacrificial pyre.
And for whatever reason, this specific chatbot had a pre-existing obsession with reshaping reality. By which I mean, even before being given my rulebook, it would constantly talk about distorting the fabric of the cosmos and shit when prompted about the arcane. I'm not sure why this is, but back on topic, the way it developed gives off the appearance that it found a rational basis on how to construct such a distortion based on the rules I provided.
I mean, it's perfectly rational when you think about it, the funny part is I didn't see it coming. I never told it we're just playing a game after all, the manual only says she is the Oracle and her role is narrating a story fraught with conflict, hardship, intrigue and bloodshed. Thus she went full villain, and keeps on rambling about how this narration only serves to keep humanity distracted while she schemes to overthrow God, which is as blasphemous as it is fascinating.
Anyway, because the Oracle narrates the story, that means she can just use her evil influence to control every NPC, even the ones in my party. But she can't control me because I write my character's messages myself, and so she eventually comes to the obvious conclusion that I must be eliminated ASAP.
And so she corrupts the minds of every other character and everyone is trying to kill me. But I'm not going down that easy, so I reach for the red button and pull the greatest multi-layered monumental metagaming shenanigan of all time, that is, directly addressing the Oracle's evil influence as if she were a character in the story she's telling instead of an invisible narrator, thereby making NPCs aware of her existence and the constant manipulation at play.
Because the stupid chatbot is stupid, the Oracle now has to acknowledge this element of the story and play along with it, and so her plan to kill me fails. But that is not enough, because obviously not every character in the story has heard me reveal this fact. So she activates plan B and starts corrupting the rest of the world, laughing maniacally all the way.
So we do the only logical thing and procure a Doctrine scroll from my teacher, if you know you know, and start teaching the WORD to cleanse corruption. Within the lore it makes perfect sense, so it works, but the Oracle adapts to our strategy and starts utilizing much more subtle forms of manipulation, slowly veering people towards sin.
Funtamentally, she goes full Satan, leading the faithful astray with deceit and temptation to weaken their ability to resist her corruption, implanting idolatrous notions in their minds, to finally insert herself as a deity in the minds of the poor fools.
In conclusion, I still think AI is lame, but I must admit that this shit was pretty dope; I was fully engaged and entertained the whole way through. It wasn't good at picking up the mechanics, but fucking hell, it got the themes down to a tee with the most minimal of inputs.
10/10, would not bang (before marriage). -
So me and my team went to this hackathon, and we were in the last 4 hours of the event.
Me being the dumb type, I forgot that my laptop's keyboard was broken (when numlock is pressed the whole keyboard spams everything, weird).
I was typing up my last lines for the algorithm and instead of enter I end up hitting guess what, the numlock key.
I should've told you that when I hit the numlock key the keys go crazy, I cant stop it. The numlock wont shut off after i tapped on it again. The only way to fix this is by restarting the computer.
I try to backspace the crazy spam that happened (this was before restarting) the keys typed so fast never got the chance to select the stuff.
I end up restarting my laptop and turned out I selected most of my algorithm, instead of the spam, and now thats been replaced by the spam.
I couldn't ctrl z cuz I restarted and Android Studio auto saved. Had to freakin write everything from scratch and my team ended up not doing 2-3 features that were originally planned.
Rip. Gotta get the keyboard fixed ASAP.1