Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
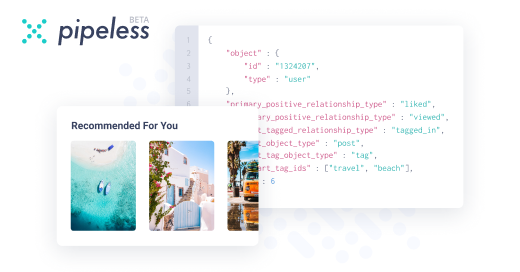
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "async function"
-
Manager: You can’t define an async function without using await.
Dev: Yes you can.
Manager: Well you shouldn’t, there’s no point!
Dev: Yes there is. It can turn blocking synchronous logic into work performed concurrently. In this case the perform—
Manager: It’s called async *await*. Async *AWAIT*! Did you hear the two parts to that? You shouldn’t ever have one without the other. THEY GO TOGETHER. Worrying about concurrency is for people who use callbacks which just goes to show how out of date your skills are. I’m reading a book on javascript and there are so many advanced techniques out there that I haven’t even seen you use ONCE!
Dev: …
*I looked at the book he’s reading, it’s from the < ES6 era… no wonder he doesn’t see me using any of those archaic patterns/hacks/workarounds…*13 -
When I write async code in ES7, I'm tempted to call my promises "chtulu", "messiah", "lastJudgement", etc.
async function doStuff() {
chtulu = someAsyncTask();
await chtulu;
}5 -
Found my most ridiculous function ever, back from when I was first learning.
async Task Delay(int time)
{
await Task.Delay(time)
}1 -
Oh man. Mine are the REASON why people dislike PHP.
Biggest Concern: Intranet application for 3 staff members that allows them to set the admin data for an application that our userbase utilizes. Everything was fucking horrible, 300+ php files of spaghetti that did not escape user input, did not handle proper redirects, bad algo big O shit and then some. My pain point? I was testing some functionality when upon clicking 3 random check boxes you would get an error message that reads something like this "hi <SENSITIVE USERNAME DATA> you are attempting to use <SERVER IP ADDRESS> using <PASSWORD> but something went wrong! Call <OLD DEVELOPER's PHONE NUMBER> to provide him this <ERROR CODE>"
I panicked, closed that shit and rewrote it in an afternoon, that fucking retard had a tendency to use over 400 files of php for the simplest of fucking things.
Another one, that still baffles me and the other dev (an employee that has been there since the dawn of time) we have this massive application that we just can't rewrite due to time constraints. there is one file with (shit you not) a php include function that when you reach the file it is including it is just......a php closing tag. Removing it breaks down the application. This one is over 6000 files (I know) and we cannot understand what in the love of Lerdorf and baby Torvalds is happening.
From a previous job we had this massive in-house Javascript "framework" for ajax shit that for whatever reason unknown to me had a bunch of function and object names prefixed with "hotDog<rest of the function name>", this was used by two applications. One still in classic ASP and the other in php version 4.something
Legacy apps written in Apache Velocity, which in itself is not that bad, but I, even as a PHP developer, do not EVER mix views with logic. I like my shit separated AF thank you very much.
A large mobile application that interfaced with fucking everything via webviews. Shit was absolutley fucking disgusting, and I felt we were cheating our users.
A rails app with 1000 controller methods.
An express app with 1000 router methods with callbacks instead of async await even though async await was already a thing.
ultraFuckingLarge Delphi project with really no consideration for best practices. I, to this day enjoy Object Pascal, but the way in which people do delphi can scare me.
ASP.NET Application in wich there seemed to be a large portion of bolted in self made ioc framework from the lead dev, absolute shitfest, homie refused to use an actual ioc framework for it, they did pay the price after I left.
My own projects when I have to maintain them.9 -
At my first big boy job at a start up with only 50 users, we noticed we had cloud cost spikes about 20x larger than we were expecting. I remember spending all night debugging it, checking the requests as they go through.
The culprit? Someone left an async on a UseEffect with a variable that was regularly updated as a parameter.
In other words: every time a request was fired, the variable would change… so the function would sense the variable changed and fire it again, and so on.
Felt like a total hero. -
What features would you want in a logger?
Here's what I'm planning so far:
- Tagged entries for easy scanning of log file
- Support for indenting to group similar sequential entries
- Multiple entry types (normal, info, event, warning, error, fatal, debug, verbose)
- Meta entries, so the logger logging about itself, e.g. disk i/o failures.
- Ability to add custom entry types, including tag, log-level, etc.
- Customizable timestamp function
- Support for JS's async nature -- this equates to passing a unique key per 'thread'; the logger will re-write all the parent blocks for context, if necessary. if that sounds confusing, it's okay; just trust that it makes sense.
- Caching, retries, etc. in the event of disk i/o issues.
- Support for custom writers, allowing you to e.g. write logs to an API rather than console or disk.
How about these features?
- Multiple (named) logs with separate writers (console, disk, etc.)
- Ability to individually enable/disable writing of specific entry types. (want verbose but not info? sure thing, weirdo!)
- Multiple writers per log. Combined with the above, this would allow you to write specific entry types (e.g. error, warning, fatal) to stderr instead of stdout, or to different apis.
- Ability to write the same log entry to multiple logs simultaneously
What do you think of these features?
What other features would you want?
I'm open to suggestions!18 -
Wanna hear a story? The consultancy firm I work for has been hired to work on a WPF project for a big Fashion Industry giant.
We are talking of their most important project yet, the ones the "buyers" use to order them their products globally, for each of the retail stores this Fashion giant has around the world. Do you want to know what I found? Wel, come my sweet summer child.
DB: not even a single foreign key. Impossibile to understand without any priopr working experience on the application. Six "quantity" tables to keep aligned with values that will dictate the quantities to be sent to production (we are talking SKUs here: shoes, bags..)
BE: autogenerated controllers using T4 templates. Inputs directly serialized in headers. Async logging (i.e. await Logger.Error(ex)). Entities returned as response to the front end, no DTOs whatsoever.
WPF: riddled with code behind and third party components (dev express) and Business Logic that should belong to the Business Layer. No real api client, just a highly customized "Rest Helper". No error reporting or dealing with exceptions. Multiple endpoints call to get data that would be combined into one single model which happens to be the one needed by the UI. No save function: a timer checks the components for changes and autosaves them every x seconds. Saving for the most critical part occurring when switching cells or rows, often resulting in race conditions at DB level.
What do you think of this piece of shit?6 -
> turning the whole codebase into a muddy ball of dirt because the leader didn't like 1 (one) call to an async function on startup
Way to go buddy, you sure show them how it's done. -
As a pretty solid Angular dev getting thrown a react project over the fence by his PM I can say:
FUCK REACT!
It is nigh impossible to write well structured, readable, well modularized code with it and not twist your mind in recursion from "lift state up" and "rendercycle downwards only"
Try writing a modular modal as a modern function component with interchangeable children (passeable to the component as it should be) that uses portals and returns the result of the passed children components.
Closest I found to it is:
c o d e s a n d b o x.io/s/7w6mq72l2q
(and its a fucking nightmare logic wise and readability wise)
And also I still wouldn't know right of the bat how to get the result from the passed child components with all the oneway binding CLUSTERFUCK.
And even if you manage to there is no chance to do it async as it should be.
You HAVE to write a lot of "HTML" tags in the DOM that practically should not be anywhere but in async functions.
In Angular this is a breeze and works like a charm.
Its not even much gray matter to it...
I can´t comprehend how companies decide to write real big web apps with it.
They must be a MESS to maintain.
For a small "four components that show a counter and fetch user images" - OK.
But fo a big webapp with a big team etc. etc.?
Asking stuff about it on Stackoverflow I got edited unsolicited as fuck and downvoted as fuck in an instant.
Nobody explained anything or even cared to look at my Stackblitz.
Unsolicited edit, downvote, closevote and of they go - no help provided whatsoever.
Its completely fine if you don't have time to help strangers - but then at least do not stomp on beginners like that.
I immediately regretted asking a toxic community like this something that I genuinely seem to not understand. Wasn't SO about helping people?
I deleted my post there and won't be coming back and doing something productive there anytime soon.
Out of respect for my clients budget I'm now doing it the ugly react way and forget about my software architecture standards but as soon as I can I will advise switching to Angular.
If you made it here: WOW
Thank you for giving me a vent to let off some steam :)13 -
me vs marketing guy, again
me: yeah, the database server is not responding, so you cannot log in to post your blog, wait for it to get online.
MG: But, the website is online.
me: web host and database server are two distinct things, they are not the same, *share a screenshot of the error*
MG: Oh okay.
Literally 3 hours later this fucking idiot sends an email and I quote.
"Hi Dev,
@CTO FYI, Someone has removed this code So there is some tracking issue on it.
Please add below google analytics code on the website.
Note: Copy and paste this code as the first item into the <HEAD> of every web page that you want to track. If you already have a Global Site Tag on your page, simply add the config line from the snippet below to your existing Global Site Tag.
<!-- Global site tag (gtag.js) - Google Analytics -->
<script async src="https://googletagmanager.com/gtag/..."></script>
<script>
window.dataLayer = window.dataLayer || [];
function gtag(){dataLayer.push(arguments);}
gtag('js', new Date());
gtag('config', 'UA-xxxxxxxx-1');
</script>
"
The fucking issue was of him not being able to post his shitty blog, and he shares an email like this, FOR FUCK'S SAKE!2 -
I was just chatting with my dad. He used to be mostly a C# dev but changed jobs and is now doing mostly Java. He says he likes it better.... Because it doesn't have lambdas/anonymous functions.
Uh.... Java was the first and only language where you can define interface implementations in-line (aka a whole bunch of functions)...
And 1.8 supports lambdas for Interfaces that have a single function...
I bet he'll hate JS... Where functions are can be passed around like objects, ES6 now supports lambdas and await, async... and anonymous functions (apparently they're called arrow functions?)9 -
Dear other developers,
Don't throw exceptions in async callbacks goddammit. It doesn't abort the outer function where you think it does, and it crashes the app because you can't catch it.4 -
I wondering what could possibly slow down our server app , Just found out there's one mother fucker who use async in every cases and async everywhere. Hey not all function are async!3
-
Looking for platform specific language options for a new project at work and reading articles from well known sources.
If you start your article with something like, "when I think of a good programming language, I think of JavaScript", I'm going to punch out of your shitty opinion faster than an async function.
When you're trying to convey an unbiased message you generally don't start with, "I'm an absolute shill for {language}".
What the fuck happened to journalistic integrity?7 -
JavaScript is new the PHP.
Reading a stackoverflow question about async functions and await-usage, and lot of well-intended answers show no understanding of the concept. Some fail to understand that the scope is about promises. They don't know that, yes, *every* async function *always* returns a Promise and that within an async function it's synctically the same to do either `return x` or `Promise.resolve(x)` or `return new Promise((resolve) => {resolve(x)}), and they fail to realize what await does or doesn't do and are oblivious about how awaiting at certain stages can have huge performance impact when compared to either Promise.all or Bluebird.map.
Grasping promises is hard in the beginning, I get that. But please don't share your lack of understanding as fact. -
I don't want to ever hear that you're proficient in JavaScript if you put a callback function call inside of an async function right after using the await command.
All you manage to do in the end was make a simple function that gets data to populate a dropdown menu into something that is absolutely more awful to look at than the worst callback hell possible.
Refactoring this code base has really questioned my sanity and how much I'm willing to spend on alcohol.4 -
What the hell happened to the dotnet ecosystem in terms of stacktraces and async.
You used to be able to pin down your issues to the freaking exact line number using a stacktrace, but now im building a azure function and this is the crap im served when it blows up: How do you debug this?8 -
So if you’re awaiting a promise and the async function returning the promise calls another async function and doesn’t await it could this counterintuitively cause the whole thing to freeZe ?14
-
When I say "error in an async function" you say "no stack trace"!
"Error in an async function"!
"No stack trace"!
"No stack trace"!
"Error in an async function"!
"Unhandled promise rejection DEPRECATION WARNING Unhandled promise rejections will be shoved up your ass in node v7.coffee.9.10.666."3 -
It's a shame that people don't want to use F# but prise C# for how cool it became and continue becoming. At the same time, little do they know that many of the features were simply drawn from F#.
It's just rediculous how far this OO and C-Style syntax crap has progressed. They keep copying things from functional langugages, making the initial language to be a monstrocity like C++ is now, insted of just using languages like C#. I mean, it was right there before C#: async/task, immutablility, records, indexes, lambdas, non-null by default, who the hell knows what else.
Besides, many people (in my company at least) are just blindly overengineering with patterns and shit, where a simple function would be just enogh.
Watch some some NDC talks about F#, in particular those of Scott Wlaschin. It's just better in so many ways: less noice (I'm looking at you, brackets, commas and semicolons), the whole LOT of type inference and less duplication (just look at the C# signatures of linq methods - it's difficult to read them), immutability by default, non-nullable by default, ADTs and pattern matching, some neat features like type providers (how many times have used "paste special" or an online tool to create C# classes from a JSON/XML file, and how many times have your regenrated it because of schema changes?) and units of measure.
Of course, in some cases it's not optimal, in some cases mutable datastructures of C# are better for performance. But dude, how many performance critical systems have you wrote in C#? I mean, if it comes to performance you should use Rust or C++ or C after all.
*sighs*15 -
TL;DR: don’t use Array.forEach use
for … of … instead.
Array.forEach is synchronous, but pass it an async function and the bastard does not await it.
I was so sure that it was sync….
Wasted 2h14 -
Started learning python async with curio and trio just two days ago, and I must say I'm really having a blast.
I have a few pending ideas to combine it with websockets (also first time I'm learning how to use them).
Haven't had this much fun with python in a while!
Also, sidequestion: how can return a value from an async function with curio or trio? I can't find a proper answer or example about that, other than some hints about memory channels in trio3 -
It's nice that more and more languages are introducing async/await syntax, but by the example of Rust in particular I'm starting to wonder why we don't instead introduce this syntax for monads in general?
We could have a keyword (say, `bind`) which unwraps a value from any monad provided that the return value of the function is wrapped in the same monad. The ? operator does something a little similar, and I'll be intrigued whether it can actually be implemented for monads other than Result and Option once GATs are stabilized. In particular in the case of Rust, it would be possible to create a reference counting monad for heap-bound management of objects derived from references.9 -
Anyone ever seen the behavior with async methods and promises where at the end of what should finish the await in the calling code the function never progresses past the await even though it returned ?30
-
I expect somebody else has chosen this as well, but anyway:
Async.<any-function>, cause they brought me out of callback hell and taught me the undefined ways async...arghhhh fuck -
"You don't need to put a type annotation here, the type will be inferred by the compiler"
"We don't need to mark this function as async, since we are already returning a promise but not awaiting it yet within this method"
TypeScript codebase. Am I wrong to prefer that things be explicit rather than implicit? Sounds a bit tryhard to try and make things implicit, but maybe the async really changes behavior in the second case? I don't think so, and I prefer to mark an asynchronous operation as such, but I'm still doubtful; that's why I humbly ask you all what makes more sense here.7 -
tell me guys what would you prefer:
function a(){
..
b(..)
..
b(..)
..
}
function b(p1,p2,p3,p4,p5,p6){.
...
}
or
function a(){
..
b(..)
..
b(..)
..
}
function b(
p1,
p2,
p3,
p4,
p5,
p6
){
...
}
if you read this rant before expanding, you got a complete context on how what function a is, its calling b 2 times and how function b looks.
if instead of the first option, i had used 2nd block, you wouldn't even know the 2nd param of b function without expanding this rant.
my point?
i prefer to keeping unnecessary info on one line. and w lot of linters disagree by splitting up the code. and most importantly , my arrogant tl disagree by saying he prefers the splitted code "for readability" and becaue "he likes code this way, old-eng1 likes this and old-eng2 likes this" .
why tf does an ide have horizontal a scrolling option available when you are too stupid to use it?
ok, i know some smartass is going to point that i too can use vertical scrolling, but hear me out: i am optimising this!
case 1 : a function with 7 params is NOT split into 7 lines. lets calculate the effort to remember it
- since all params could have similar charactersticks ( they will be of some type, might have defaults, might be a suspendable/async function etc), each param will take similar memory-efforts points. say 5sp each.
- total memory-efforts= 5sp *7 = 35 sp.
- say a human has 100 sp of fast memory storage, he can use the remaining 65 sp for loading say 5 small lines above or below.
- but since 5 lines above are already read and still visible on screen, they won't be needed to be loaded again nd again, nd we can just check the lines below.
- thus we are able to store 65+35+65 = 165 sp or about 11 lines of code in out fast memory for just a 100sp brain storage
case 2 function with 7 params IS split into 7 lines.
- in this case all lines are somewhat similar. 5sp for param lines as they are still similar which implies same 35sp for storing current function and params
- remaining 65sp can only be used to store next 5 lines of 13sp as the previous code is no longer visible.
- plus if you wanna refresh the code above, you gotta scroll, which will result in removing bottom code from screen , and now your 65sp from bottom code is overwritten by 65sp of top code.
- thus at a time, you are storing only 6 lines worth of code info. this makes you slow.
this is some imaginary math, but i believe it works10 -
Typescript is my new favorite and my grudge is the stupid scoping of type assertions. I have an async function that checks whether a variable is set and awaits a change event if it's undefined. This function is working javascript but invalid according to typescript, because it relies on the exact type changing while the function is running. I had to convert it to a mess of promises to bypass this because (and this is the best) the callback-based syntax of identical meaning will reset all type assertions, even locals that are never written after the callback's creation.8
-
I can't google jargon, what do you call it when you "flip" a function call, such that the call becomes an event in some dispatch system and return becomes a call on the event? I had to implement five such APIs this week with surface level differences and I'm starting to feel like it has to have a name if it's this popular.
For the pedantic, I mean async calls in JS in particular, I know you can't just invert a synchronous function call that uses a stack without peek.5 -
I know I sound stupid but I need help, I create a repo on GitHub using gh-api ```js
export async function createARepo({name,description,token}) {
const headers = {
"Authorization": `token ${token}`,
"Accept": "application/vnd.github.v3+json",
}
const {data} = await axios(
{
method: "POST",
url: "https://api.github.com/user/repos",
data: {name,description,auto_init: true},
headers
}
)
return data
// console.log(res)
}```
when I run this code it only creates an empty project with a readme but I also want to create a file with a .html extension of the project can anybody help me with how I do this?7 -
Heroku logs be like
2018-07-16T00:05:06.883023+00:00 app[bot.1]: Ready!
2018-07-16T00:05:11.940150+00:00 app[bot.1]: (node:4) UnhandledPromiseRejectionWarning: Error: 410 Gone
2018-07-16T00:05:11.940171+00:00 app[bot.1]: at _response.transport.request.then (/app/node_modules/snekfetch/src/index.js:193:21)
2018-07-16T00:05:11.940173+00:00 app[bot.1]: at <anonymous>
2018-07-16T00:05:11.940175+00:00 app[bot.1]: at process._tickCallback (internal/process/next_tick.js:188:7)
2018-07-16T00:05:11.940248+00:00 app[bot.1]: (node:4) UnhandledPromiseRejectionWarning: Unhandled promise rejection. This error originated
either by throwing inside of an async function without a catch block, or by rejecting a promise which was not handled with .catch(). (rejection id: 2)
2018-07-16T00:05:11.940340+00:00 app[bot.1]: (node:4) [DEP0018] DeprecationWarning: Unhandled promise rejections are deprecated. In the future, promise rejections that are not handled will terminate the Node.js process with a non-zero exit code. -
Hello chat, its been a long week with no progress, i have four problems right now.
1 node js repl cant recognize <> in js, and i cant find a fix for that anywhere on the internet, is there a way to convert html tags in js to smooth brackets code?
2 node js repl dosnt recognize codes like import react from react, and i have to do an async function load, and i dont know y or how to fix it.
3 i dont know how to write import exg.csv into an async function load, its usually something like “import react from react” to “async function load(){let react=await import (‘react’);}”, and i dont know how to do this.
4 i tried to install materials.ui using npm into a folder called materialsui in modules in node, it deleted all the other modules in module folder, installed, and then left the materialsui folder empty. I complained and i dont know how to get it to not do that.
5 how do i fix out of memory errors?8 -
Being new to NodeJS, I wanted to use the framework for a small script that involved connecting to a MySQL database and updating 1500+ records.
With NodeJS's preference towards functional programming over sequential, I wanted to do things the NodeJS way with callback functions instead how I'm used to doing it, using loops (and all the MySQL functions were async).
I couldn't update all the rows at once, so I wrote a callback function that calls back itself after the SQL statement is executed. A recursive callback function... am I doing this right?7 -
You know
When I first saw etherum talking about am distributed state machine i thought wow. Not very practical but NEAT. I envisioned being able to make a byte code that could be stored in transactions and run by individual clients in an async function and each step of the resulting execution and the values of managed ram would be stored at intervals so other clients could take over and execute a few more statements and compare what should always be expected results that are identical
A grand incredibly inefficient system however really neato from the theoretical computer nerd standpoint !
Boy was I disappointed lol all it is a basic contracts language but yet they state it could be like a word computer ! How ? I thought maybe if you had enough nodes participating maybe you could store registers and the like in transaction values ? Wouldn’t that be the way ?
Seems like as a word computer they’re stuck somewhere between very simplistic js and something prior to amptron in usability yet they advertised as a world computer
Am i missing something ? I mean you could create something that would translate higher level code into smal numeric statements and then send it additions values but what would it be useful for and how would you actually. Store anything ?