Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
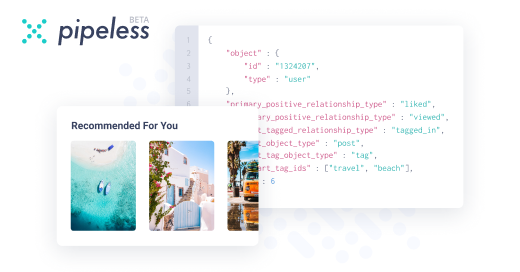
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "break-statement"
-
PineScript is absolute garbage.
It's TradingView's scripting language. It works, but it's worse than any language I have ever seen for shoddy parsing. Its naming conventions are pretty terrible, too:
transparency? no, "transp"
sum? no, cum. seriously. cum(array) is its "cumulative sum."
There are other terrible names, but the parser is what really pisses me off.
1) If you break up a long line for readability (e.g. a chained ternary), each fragment needs to be indented by more than its parent... but never by a multiple of 4 spaces because then it isn't a fragment anymore, but its own statement.
2) line fragments also cannot end in comments because comments are considered to be separate lines.
3) Lambdas can only be global. They're just fancy function declarations. Someone really liked the "blah(x,y,z) =>" syntax
4) blocks to `if`s must be on separate lines, meaning `if (x) y:=z` is illegal. And no, there are no curly braces, only whitespace.
There are plenty more, but the one that really got me furious is:
98) You cannot call `plot()`, `plotshape()`, etc. if they're indented! So if you're using non-trivial logic to optionally plot things like indicators, fuck you.
Whoever wrote this language and/or parser needs to commit seppuku.rant or python? pinescript or fucking euphoria? or ruby? why can't they just use lua? or javascript? tradingview16 -
Especially painful being a cybersecurity engineer;
Did something wrong with an if-statement.
Caused authentication to break completely; anyone could login as any user.
Was fixed veeeeeeery quickly 😅 (yes, was already live)8 -
POSTMORTEM
"4096 bit ~ 96 hours is what he said.
IDK why, but when he took the challenge, he posted that it'd take 36 hours"
As @cbsa wrote, and nitwhiz wrote "but the statement was that op's i3 did it in 11 hours. So there must be a result already, which can be verified?"
I added time because I was in the middle of a port involving ArbFloat so I could get arbitrary precision. I had a crude desmos graph doing projections on what I'd already factored in order to get an idea of how long it'd take to do larger
bit lengths
@p100sch speculated on the walked back time, and overstating the rig capabilities. Instead I spent a lot of time trying to get it 'just-so'.
Worse, because I had to resort to "Decimal" in python (and am currently experimenting with the same in Julia), both of which are immutable types, the GC was taking > 25% of the cpu time.
Performancewise, the numbers I cited in the actual thread, as of this time:
largest product factored was 32bit, 1855526741 * 2163967087, took 1116.111s in python.
Julia build used a slightly different method, & managed to factor a 27 bit number, 103147223 * 88789957 in 20.9s,
but this wasn't typical.
What surprised me was the variability. One bit length could take 100s or a couple thousand seconds even, and a product that was 1-2 bits longer could return a result in under a minute, sometimes in seconds.
This started cropping up, ironically, right after I posted the thread, whats a man to do?
So I started trying a bunch of things, some of which worked. Shameless as I am, I accepted the challenge. Things weren't perfect but it was going well enough. At that point I hadn't slept in 30~ hours so when I thought I had it I let it run and went to bed. 5 AM comes, I check the program. Still calculating, and way overshot. Fuuuuuuccc...
So here we are now and it's say to safe the worlds not gonna burn if I explain it seeing as it doesn't work, or at least only some of the time.
Others people, much smarter than me, mentioned it may be a means of finding more secure pairs, and maybe so, I'm not familiar enough to know.
For everyone that followed, commented, those who contributed, even the doubters who kept a sanity check on this without whom this would have been an even bigger embarassement, and the people with their pins and tactical dots, thanks.
So here it is.
A few assumptions first.
Assuming p = the product,
a = some prime,
b = another prime,
and r = a/b (where a is smaller than b)
w = 1/sqrt(p)
(also experimented with w = 1/sqrt(p)*2 but I kept overshooting my a very small margin)
x = a/p
y = b/p
1. for every two numbers, there is a ratio (r) that you can search for among the decimals, starting at 1.0, counting down. You can use this to find the original factors e.x. p*r=n, p/n=m (assuming the product has only two factors), instead of having to do a sieve.
2. You don't need the first number you find to be the precise value of a factor (we're doing floating point math), a large subset of decimal values for the value of a or b will naturally 'fall' into the value of a (or b) + some fractional number, which is lost. Some of you will object, "But if thats wrong, your result will be wrong!" but hear me out.
3. You round for the first factor 'found', and from there, you take the result and do p/a to get b. If 'a' is actually a factor of p, then mod(b, 1) == 0, and then naturally, a*b SHOULD equal p.
If not, you throw out both numbers, rinse and repeat.
Now I knew this this could be faster. Realized the finer the representation, the less important the fractional digits further right in the number were, it was just a matter of how much precision I could AFFORD to lose and still get an accurate result for r*p=a.
Fast forward, lot of experimentation, was hitting a lot of worst case time complexities, where the most significant digits had a bunch of zeroes in front of them so starting at 1.0 was a no go in many situations. Started looking and realized
I didn't NEED the ratio of a/b, I just needed the ratio of a to p.
Intuitively it made sense, but starting at 1.0 was blowing up the calculation time, and this made it so much worse.
I realized if I could start at r=1/sqrt(p) instead, and that because of certain properties, the fractional result of this, r, would ALWAYS be 1. close to one of the factors fractional value of n/p, and 2. it looked like it was guaranteed that r=1/sqrt(p) would ALWAYS be less than at least one of the primes, putting a bound on worst case.
The final result in executable pseudo code (python lol) looks something like the above variables plus
while w >= 0.0:
if (p / round(w*p)) % 1 == 0:
x = round(w*p)
y = p / round(w*p)
if x*y == p:
print("factors found!")
print(x)
print(y)
break
w = w + i
Still working but if anyone sees obvious problems I'd LOVE to hear about it.36 -
Okay, story time.
Back during 2016, I decided to do a little experiment to test the viability of multithreading in a JavaScript server stack, and I'm not talking about the Node.js way of queuing I/O on background threads, or about WebWorkers that box and convert your arguments to JSON and back during a simple call across two JS contexts.
I'm talking about JavaScript code running concurrently on all cores. I'm talking about replacing the god-awful single-threaded event loop of ECMAScript – the biggest bottleneck in software history – with an honest-to-god, lock-free thread-pool scheduler that executes JS code in parallel, on all cores.
I'm talking about concurrent access to shared mutable state – a big, rightfully-hated mess when done badly – in JavaScript.
This rant is about the many mistakes I made at the time, specifically the biggest – but not the first – of which: publishing some preliminary results very early on.
Every time I showed my work to a JavaScript developer, I'd get negative feedback. Like, unjustified hatred and immediate denial, or outright rejection of the entire concept. Some were even adamantly trying to discourage me from this project.
So I posted a sarcastic question to the Software Engineering Stack Exchange, which was originally worded differently to reflect my frustration, but was later edited by mods to be more serious.
You can see the responses for yourself here: https://goo.gl/poHKpK
Most of the serious answers were along the lines of "multithreading is hard". The top voted response started with this statement: "1) Multithreading is extremely hard, and unfortunately the way you've presented this idea so far implies you're severely underestimating how hard it is."
While I'll admit that my presentation was initially lacking, I later made an entire page to explain the synchronisation mechanism in place, and you can read more about it here, if you're interested:
http://nexusjs.com/architecture/
But what really shocked me was that I had never understood the mindset that all the naysayers adopted until I read that response.
Because the bottom-line of that entire response is an argument: an argument against change.
The average JavaScript developer doesn't want a multithreaded server platform for JavaScript because it means a change of the status quo.
And this is exactly why I started this project. I wanted a highly performant JavaScript platform for servers that's more suitable for real-time applications like transcoding, video streaming, and machine learning.
Nexus does not and will not hold your hand. It will not repeat Node's mistakes and give you nice ways to shoot yourself in the foot later, like `process.on('uncaughtException', ...)` for a catch-all global error handling solution.
No, an uncaught exception will be dealt with like any other self-respecting language: by not ignoring the problem and pretending it doesn't exist. If you write bad code, your program will crash, and you can't rectify a bug in your code by ignoring its presence entirely and using duct tape to scrape something together.
Back on the topic of multithreading, though. Multithreading is known to be hard, that's true. But how do you deal with a difficult solution? You simplify it and break it down, not just disregard it completely; because multithreading has its great advantages, too.
Like, how about we talk performance?
How about distributed algorithms that don't waste 40% of their computing power on agent communication and pointless overhead (like the serialisation/deserialisation of messages across the execution boundary for every single call)?
How about vertical scaling without forking the entire address space (and thus multiplying your application's memory consumption by the number of cores you wish to use)?
How about utilising logical CPUs to the fullest extent, and allowing them to execute JavaScript? Something that isn't even possible with the current model implemented by Node?
Some will say that the performance gains aren't worth the risk. That the possibility of race conditions and deadlocks aren't worth it.
That's the point of cooperative multithreading. It is a way to smartly work around these issues.
If you use promises, they will execute in parallel, to the best of the scheduler's abilities, and if you chain them then they will run consecutively as planned according to their dependency graph.
If your code doesn't access global variables or shared closure variables, or your promises only deal with their provided inputs without side-effects, then no contention will *ever* occur.
If you only read and never modify globals, no contention will ever occur.
Are you seeing the same trend I'm seeing?
Good JavaScript programming practices miraculously coincide with the best practices of thread-safety.
When someone says we shouldn't use multithreading because it's hard, do you know what I like to say to that?
"To multithread, you need a pair."18 -
Long rant ahead.. so feel free to refill your cup of coffee and have a seat 🙂
It's completely useless. At least in the school I went to, the teachers were worse than useless. It's a bit of an old story that I've told quite a few times already, but I had a dispute with said teachers at some point after which I wasn't able nor willing to fully do the classes anymore.
So, just to set the stage.. le me, die-hard Linux user, and reasonably initiated in networking and security already, to the point that I really only needed half an ear to follow along with the classes, while most of the time I was just working on my own servers to pass the time instead. I noticed that the Moodle website that the school was using to do a big chunk of the course material with, wasn't TLS-secured. So whenever the class begins and everyone logs in to the Moodle website..? Yeah.. it wouldn't be hard for anyone in that class to steal everyone else's credentials, including the teacher's (as they were using the same network).
So I brought it up a few times in the first year, teacher was like "yeah yeah we'll do it at some point". Shortly before summer break I took the security teacher aside after class and mentioned it another time - please please take the opportunity to do it during summer break.
Coming back in September.. nothing happened. Maybe I needed to bring in more evidence that this is a serious issue, so I asked the security teacher: can I make a proper PoC using my machines in my home network to steal the credentials of my own Moodle account and mail a screencast to you as a private disclosure? She said "yeah sure, that's fine".
Pro tip: make the people involved sign a written contract for this!!! It'll cover your ass when they decide to be dicks.. which spoiler alert, these teachers decided they wanted to be.
So I made the PoC, mailed it to them, yada yada yada... Soon after, next class, and I noticed that my VPN server was blocked. Now I used my personal VPN server at the time mostly to access a file server at home to securely fetch documents I needed in class, without having to carry an external hard drive with me all the time. However it was also used for gateway redirection (i.e. the main purpose of commercial VPN's, le new IP for "le onenumity"). I mean for example, if some douche in that class would've decided to ARP poison the network and steal credentials, my VPN connection would've prevented that.. it was a decent workaround. But now it's for some reason causing Moodle to throw some type of 403.
Asked the teacher for routers and switches I had a class from at the time.. why is my VPN server blocked? He replied with the statement that "yeah we blocked it because you can bypass the firewall with that and watch porn in class".
Alright, fair enough. I can indeed bypass the firewall with that. But watch porn.. in class? I mean I'm a bit of an exhibitionist too, but in a fucking class!? And why right after that PoC, while I've been using that VPN connection for over a year?
Not too long after that, I prematurely left that class out of sheer frustration (I remember browsing devRant with the intent to write about it while the teacher was watching 😂), and left while looking that teacher dead in the eyes.. and never have I been that cold to someone while calling them a fucking idiot.
Shortly after I've also received an email from them in which they stated that they wanted compensation for "the disruption of good service". They actually thought that I had hacked into their servers. Security teachers, ostensibly technical people, if I may add. Never seen anyone more incompetent than those 3 motherfuckers that plotted against me to save their own asses for making such a shitty infrastructure. Regarding that mail, I not so friendly replied to them that they could settle it in court if they wanted to.. but that I already knew who would win that case. Haven't heard of them since.
So yeah. That's why I regard those expensive shitty pieces of paper as such. The only thing they prove is that someone somewhere with some unknown degree of competence confirms that you know something. I think there's far too many unknowns in there.
Nowadays I'm putting my bets on a certification from the Linux Professional Institute - a renowned and well-regarded certification body in sysadmin. Last February at FOSDEM I did half of the LPIC-1 certification exam, next year I'll do the other half. With the amount of reputation the LPI has behind it, I believe that's a far better route to go with than some random school somewhere.25 -
"I'm almost done, I'll just need to add tests!"
Booom! You did it, that was a nuke going off in my head.
No, you shouldn't just need to add tests. The tests should have been written from the get go! You most likely won't cover all the cases. You won't know if adding the tests will break your feature, as you had none, as you refactor your untested mess in order to make your code testable.
When reading your mess of a test case and the painful mocking process you went through, I silently cry out into the void: "Why oh why!? All of this suffering could have been avoided!"
Since most of the time, your mocking pain boils down to not understanding what your "unit" in your "unit test" should be.
So let it be said:
- If you want to build a parser for an XML file, then just write a function / class whose *only* purpose is: parse the XML file, return a value object. That's it. Nothing more, nothing less.
- If you want to build a parser for an XML file, it MUST NOT: download a zip, extract that zip, merge all those files to one big file, parse that big file, talk to some other random APIs as a side-effect, and then return a value object.
Because then you suddenly have to mock away a http service and deal with zip files in your test cases.
The http util of your programming language will most likely work. Your unzip library will most likely work. So just assume it working. There are valid use cases where you want to make sure you acutally send a request and get a response, yet I am talking unit test here only.
In the scope of a class, keep the public methods to a reasonable minimum. As for each public method you shall at least create one test case. If you ever have the feeling "I want to test that private method" replace that statement in your head with: "I should extract that functionality to a new class where that method public. I then can create a unit test case a for that." That new service then becomes a dependency in your current service. Problem solved.
Also, mocking away dependencies should a simple process. If your mocking process fills half the screen, your test setup is overly complicated and your class is doing too much.
That's why I currently dig functional programming so much. When you build pure functions without side effects, unit tests are easy to write. Yet you can apply pure functions to OOP as well (to a degree). Embrace immutability.
Sidenote:
It's really not helpful that a lot of developers don't understand the difference between unit, functional acceptance, integration testing. Then they wonder why they can't test something easily, write overly complex test cases, until someone points out to them: No, in the scope of unit tests, we don't need to test our persistance layer. We just assume that it works. We should only test our businsess logic. You know: "Assuming that I get that response from the database, I expect that to happen." You don't need a test db, make a real query against that, in order to test that. (That still is a valid thing to do. Yet not in the scope of unit tests.)rant developer unit test test testing fp oop writing tests get your shit together unit testing unit tests8 -
Today on "How the Fuck is Python a Real Language?": Lambda functions and other dumb Python syntax.
Lambda functions are generally passed as callbacks, e.g. "myFunc(a, b, lambda c, d: c + d)". Note that the comma between c and d is somehow on a completely different level than the comma between a and b, even though they're both within the same brackets, because instead of using something like, say, universally agreed-upon grouping symbols to visually group the lambda function arguments together, Python groups them using a reserved keyword on one end, and two little dots on the other end. Like yeah, that's easy to notice among 10 other variable and argument names. But Python couldn't really do any better, because "myFunc(a, b, (c, d): c + d)" would be even less readable and prone to typos given how fucked up Python's use of brackets already is.
And while I'm on the topic of dumb Python syntax, let's look at the switch, um, match statements. For a long time, people behind Python argued that a bunch of elif statements with the same fucking conditions (e.g. x == 1, x == 2, x == 3, ...) are more readable than a standard switch statement, but then in Python 3.10 (released only 1 year ago), they finally came to their senses and added match and case keywords to implement pattern matching. Except they managed to fuck up yet again; instead of a normal "default:" statement, the default statement is denoted by "case _:". Because somehow, everywhere else in the code _ behaves as a normal variable name, but in match statement it instead means "ignore the value in this place". For example, "match myVar:" and "case [first, *rest]:" will behave exactly like "[first, *rest] = myVar" as long as myVar is a list with one or more elements, but "case [_, *rest]:" won't assign the first element from the list to anything, even though "[_, *rest] = myVar" will assign it to _. Because fuck consistency, that's why.
And why the fuck is there no fallthrough? Wouldn't it make perfect sense to write
case ('rgb', r, g, b):
case ('argb', _, r, g, b):
case ('rgba', r, g, b, _):
case ('bgr', b, g, r):
case ('abgr', _, b, g, r):
case ('bgra', b, g, r, _):
and then, you know, handle r, g, and b values in the same fucking block of code? Pretty sure that would be more readable than having to write "handeRGB(r, g, b)" 6 fucking times depending on the input format. Oh, and never mind that Python already has a "break" keyword.
Speaking of the "break" keyword, if you try to use it outside of a loop, you get an error "'break' outside loop". However, there's also the "continue" keyword, and if you try to use it outside of a loop, you get an error "'continue' not properly in loop". Why the fuck are there two completely different error messages for that? Does it mean there exists some weird improper syntax to use "continue" inside of a loop? Or is it just another inconsistent Python bullshit where until Python 3.8 you couldn't use "continue" inside the "finally:" block (but you could always use "break", even though it does essentially the same thing, just branching to a different point).19 -
Update: https://devrant.com/rants/5445368/...
My previous bosses were real awesome people. However, the current one is an intentional asshole.
He wants to review every piece of work. He thinks I am a retard who knows shit. He has no sense of feedback vs. humiliating criticism.
Fucker questions every single word.
For example, consider the following statement, "They are taking the Hobbits to Isengard."
He'd critical question every word like,
What do you mean by 'they'?
Why have you mentioned it?
Why does 'They' exists in English vocabulary?
Why cannot you try 'Your'?
What data points you have?
And after endless questioning, he'd repeat the same with next word. Making sure to break my spirit of working for him.
And let me add that his communication is saturated with heavy jargons which are difficult to understand. At times, I slow down to understand and absorb and he has a problem with that as well.
My past experience says that I learned a lot from strict managers.
But this fucker intentional criticises every aspect with zero to negative appreciation. All in the name of feedback.
I have gotten tons of compliments and good ratings in the past based on my communication and thought process. However, this fucker feels that my thought process is shit and I don't know how to communicate. Furthermore, he feels that I lack sense of ownership.
I really don't know what he saw in my resume or me to even hire me in the first place.
Given how he treats me and others, no wonder people are leaving. And if he fires me, good luck to him finding a sensible replacement who matches his expectations or puts up with his crap.3 -
So my dear programming teacher really hates break statements... I mean really really really. He thinks it's better for readability if you don't break from any kinds of loops (not even ifs) well then we came across a switch statement in class. He says "breaks only exist because it's needed in switches" well how about returning from a fcking swith? or goto? then you need no break...
Is there anyone who could explain why I should NEVER use breaks and why it's bad in any piece of code? Why is it better to just use whiles because fors are apparently evil again? Srsly I just wanna ask him to show me some big code bases without breaks...6 -
Soooo it's Monday........ 🤯
@C0D4 started the day fixing current projects defects (4 tickets smashed before coffee 💪)
Then after coffee, run a test coverage report and see a significant decline over the past few months, so spends a couple hours adding more tests to get some areas filled in - meh, nothing like 50+ lines per test... to test a if() statement but whatever - complex scenarios will be complex to get too, but no my tests break and I'm missing data I didn't know about🤦♂️
So let's comment all that out, and go to lunch ... mmmm lunch.
Get back, start working on those again, and then get handed a new issue, so comment that all back out again, ( ok I know what you're thinking, but I'm working in an environment that does not use git for deployments - don't ask, real pain in the ass I haven't had time to invest into yet - but as code versioning only) anywho, starts to workout this new issue but don't figure it out, enter a 30 minute meeting.................. yea that was 2 hours later but was a very practical whiteboard session only to work out I have something like 16-20 weeks of work over 4-5 projects to get out in like 6 weeks... hahahahahahaha fml..... oh and that's excluding another project which had a 6 weeks of work in the pipeline to get to somehow.... I'm not seeing this one happening, and probably conflicting projects needed on top of that down the track... but we'll leave those out for now!
Whoot is fucking home time!!!
🤷♂️I'm starting to think I'm like a team of 5-10 devs right now, maybe I should start asking for 5-10x more 😏
#letsBringOnTuesday!!!!4 -
Applied to a company as an electronics technician for work starting in the summer of 2019. This application was back in October 2018. Got a quick reply that they already have a candidate and are willing to look into mine if he isnt the right guy. Two months later I hear back from them. They will look into my application now and I will receive their feedback after the christmas break. K. Finally a response. Ended up taking them a month longer with following reply. It appears we have forgotten about you we will have some more info by next week. This was on a monday. Thursday I receive a call. Errr. I accidentally sent you the wrong message. It should have been an interview invitation. Are you able to come by tomorrow morning?
Meanwhile I was in military service during this time till april 2019. This was written in the application with a statement, that anytime I have to ask for a day off, I need to apply for it atleast three weeks in advance. Ended up saying I have no more interest in their offer as I had signed my current work contract the week before they called me.
BTW: During the call some girls were constantly giggling childishly in the background. Which gives them even less credibility for being a serious company!1 -
Just spent an entire night eaning up my codebase...
I optimized some of the functions got rid of unnecessary global variables and changed up the whole file hirearchy so it would be easier to read. After spending all night doing this I went to run the program and for once it seemed everything worked right the first time! However a portion of my application that is supposed to happen at a certain date and time never would run. After spending all night comparing each and every line for what I changed versus my last commit I couldn't find the fallacy in my logic. Everything should still work like it did before. After spending more time looking for bugs I finally realized I didn't break anything when I switched over to this new structure it was the old code that was broken. I went through the old code and after some debugging eventually found the culprit an extra continue statement that prevented my loop from fully executing. Lesson learned sometimes the biggest bugs can spawn from one line of code.4 -
One of my TL said to me during code review that place a break statement after return statement in switch case.
Being with a bar leader can certainly degrade your code quality.10 -
How do you debate the "it's more complex in my opinion" statement?
So, some months ago I was looking at some code which has stuff as 300 lines of code function(s) and I could feel the bad smell irl...
I analyze it a bit and there is a lot of stuff which is misplaced, repeated or unsafe.
I first re-arrange it and remove redundancy, then break it down in about five functions (plus a caller), all is now readable and assignIcon k(made-up name) only assigns an icon, it doesn't also send a rocket in space.
But then I put the code in review and the previous author of the code says that it's now unreadable, because s/he has to look as multiple functions. I counter by showing how s/he does not need to read 300 lines of code to find a bug, but approximately 60, and I point at how misleading having an `assignIcon` function which also sends rockets in space is.
The counter? "But it looks confusing to have smaller functions, revert it."
How would you debate that? I am shy and hate myself a lot, so I have issues debating good points, but I am really really sure a lot of bugs I encountered were due to stuff like this so I would like to be able to explain my point in a more efficient way, for future teams.12 -
I run update without where on mysql console on production database Today.
CLASSIC
Just because I needed to fix database after bug fix on the backend of the application.
I thought I wrote good sql statement after executing it on my local machine and then everything got bad.
Luckily it was only one column with some cached statistics data and I checked that it was not important data before I actually started fixing stuff but still ...
Almost got hard attack afterwards.
Made a script to fix this column and it took me only 15 minutes but still...
Bug was caused in part I got no unit tests and application grow after 3 years of development from simple one for one customer and volumes of documents around 50k to over 40 customers and volumes over 2mil per month, don’t know how many pages each, just in one year after we completed all needed features.
I have daily backups and logs of every api operation but still.
I think this got to far for one backend developer.
I got scared that I will loose money cause I am contractor and the only backend developer working on it.
I am so tired of this right now I think I need a break from work.
Responsibility is killing me so hard right now.
It will take a week to get back to normal.2 -
The fact that four to eight dollars a week could break me and cause me to lose my job before I've even started is a statement on how bad the american economy is, and what kind of future people have in america: none.
There is none.9 -
So the other day, I was working on some Python project when there was this bug that kept transforming. Like seriously, I would turn from "bool not defined" to "function does not exist" to literally "file does not exist"... within the FILE. And when I fixed them, new bugs kept popping up, and I couldn't find anything that was a problem. Nothing. There was this one function in there that, if I changed even the comments in there, would break. And so.... I turned off Atom and turned it on again. ( ha ) Didn't work. I restarted my computer. I copy pasted the file into another file. I used another IDE. I restarted GHCI. I restarted Jupyter Notebook... and after 6 hours... I found that it was because an if statement has a comparison between a bool and a bool, with a = in the middle. (not ==). I swear I almost threw the computer on the floor.1
-
If ever there was something like dark ages, then we are living it: which programmer still knows what his statement does through the whole software stack down to the CPU (and could also account for what a modern CPU does with all its cores, caches, pipelines and -1, -2, -3 rings). Piled higher and deeper. I know nothing. So it's like being a cargo cult sorcerer, conjuring copy&pasted spells from SO to invoke Bjarne's, Linus' or whomevers forlorn spirit, so this shit won't break.2
-
So, a while ago i thought i was the inventor of the while-if. If a while statement fails, it would execute the else behind it. I had that idea for the C language:
It looks like this:
while(false){
// will not be executed since while condition is false
}else{
// will be executed since while condition is false
}
I've contacted the C work group if it is something to build in C since it prolly won't break any existing code bases.
I was enthousiast. Imagine if you could invent a new feature to such a classing language.
I got response back: is it like the python while-else?
Me, been while have been python developer for a while, finds out NOW that python has it already! Damn, such a great language.
while False:
# won't be executed
else:
# will be executed
DAMMIT! Still, they said that it doesn't mean it won't become a standard and got requested more examples. Did that ofc. Let's hope19 -
Just spent several hours trying to figure out why a binary file wasn’t being written correctly.
I missed a break statement.
Also it’s been a while since I’ve been here, maybe I’ll actually come back. -
I don't know how it's out there, but where I'm from, we don't get a lot of practical classes. The curriculum has tried to include practical alongside theory but its just not working. All we do is theory and more theory. Maybe include a major portion of marks for practicals rather than theory. And yes, please no coding in paper.
Another major thing we lack is teaching logical thinking. I have met final year under grads who find using a (!foo) to invert the value of foo mind blowing. They would rather use a full blown if-then statement to do the same. I think we need to incorporate chapters that motivates students into logical thinking to make better programmers.
Another essential part CS education around here lacks is in relevant examples and chances for internship. If you're studying something, I believe you would understand it much better if you see and experience it. Curriculum should include a real world project that you would use in a daily basis. Maybe break down and analyse a successful application and its component. -
Is inserting a break statement anywhere but in a switch statement a horrible idea and results almost always in bad code? Why? My lecture notes say so. lol.
e.g.:
loop(someJsonDataSet){
if(company.getName().equals("xyz")){
break; // don't process records of this
company
}
}14 -
The moment when u forget to break from a switch statement from its one of its cases and try to figure wtf is wrong with ur code.1
-
So I'm writing my compiler and I decide to test error handling, see if I'm catching unexpected tokens and whatnot. I try duplicating a semi-colon at the end of a line, for sure it'll give me an error since that's an unexpected token, isn't it? So I run the compiler and... No errors? I start debugging for a few minutes, snoop around, everything seems ok... "Huh, that's weird" and then it dawns on me, a semi-colon only marks the end of a statement. So, technically, it's not an unexpected token if you have an empty statement (which wouldn't break any rules about statements). I decide to try out my theory. I put ;;;;;;;; at the end of a random line in my rust code, hit compile and... it compiles! So that means it is not a bug anymore! I mean, if the big guys that actually know a tad about language design, compilers and all that cool stuff allow it in their languages, why shouldn't it? So I did it, I turned a bug into a feature and now I can go to sleep in peace and stop dreaming about fucking abstract syntax trees (don't mind my kinks >:) ).
Yeah anyways thanks for reading, till next time! Bye!1 -
I've been playing around with Nim lately, and I'd have a question. Already asked on StackOverflow, but my question disappeared without a trace (if you allow others to delete stuff, at least notify me it got deleted!).
Question is: if I have "defer: doSomething()" in an iterator, does doSomething get called when I break iteration early (before consuming everything) using a break statement?1 -
The build broke right before code review with the Lead. It didn't just break in one place, oh no, that would be too simple. It broke everywhere, right down to the core mechanic. I spent the next 3 hours trying to find out why it broke, checking everything involved in this part of the system. It was a freaking Initialization call placed inside a conditional statement instead of outside.