Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
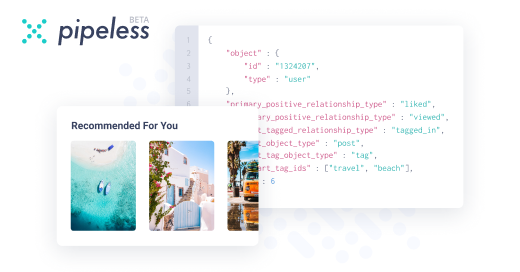
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "reference number"
-
I had to open the desktop app to write this because I could never write a rant this long on the app.
This will be a well-informed rebuttal to the "arrays start at 1 in Lua" complaint. If you have ever said or thought that, I guarantee you will learn a lot from this rant and probably enjoy it quite a bit as well.
Just a tiny bit of background information on me: I have a very intimate understanding of Lua and its c API. I have used this language for years and love it dearly.
[START RANT]
"arrays start at 1 in Lua" is factually incorrect because Lua does not have arrays. From their documentation, section 11.1 ("Arrays"), "We implement arrays in Lua simply by indexing tables with integers."
From chapter 2 of the Lua docs, we know there are only 8 types of data in Lua: nil, boolean, number, string, userdata, function, thread, and table
The only unfamiliar thing here might be userdata. "A userdatum offers a raw memory area with no predefined operations in Lua" (section 26.1). Essentially, it's for the API to interact with Lua scripts. The point is, this isn't a fancy term for array.
The misinformation comes from the table type. Let's first explore, at a low level, what an array is. An array, in programming, is a collection of data items all in a line in memory (The OS may not actually put them in a line, but they act as if they are). In most syntaxes, you access an array element similar to:
array[index]
Let's look at c, so we have some solid reference. "array" would be the name of the array, but what it really does is keep track of the starting location in memory of the array. Memory in computers acts like a number. In a very basic sense, the first sector of your RAM is memory location (referred to as an address) 0. "array" would be, for example, address 543745. This is where your data starts. Arrays can only be made up of one type, this is so that each element in that array is EXACTLY the same size. So, this is how indexing an array works. If you know where your array starts, and you know how large each element is, you can find the 6th element by starting at the start of they array and adding 6 times the size of the data in that array.
Tables are incredibly different. The elements of a table are NOT in a line in memory; they're all over the place depending on when you created them (and a lot of other things). Therefore, an array-style index is useless, because you cannot apply the above formula. In the case of a table, you need to perform a lookup: search through all of the elements in the table to find the right one. In Lua, you can do:
a = {1, 5, 9};
a["hello_world"] = "whatever";
a is a table with the length of 4 (the 4th element is "hello_world" with value "whatever"), but a[4] is nil because even though there are 4 items in the table, it looks for something "named" 4, not the 4th element of the table.
This is the difference between indexing and lookups. But you may say,
"Algo! If I do this:
a = {"first", "second", "third"};
print(a[1]);
...then "first" appears in my console!"
Yes, that's correct, in terms of computer science. Lua, because it is a nice language, makes keys in tables optional by automatically giving them an integer value key. This starts at 1. Why? Lets look at that formula for arrays again:
Given array "arr", size of data type "sz", and index "i", find the desired element ("el"):
el = arr + (sz * i)
This NEEDS to start at 0 and not 1 because otherwise, "sz" would always be added to the start address of the array and the first element would ALWAYS be skipped. But in tables, this is not the case, because tables do not have a defined data type size, and this formula is never used. This is why actual arrays are incredibly performant no matter the size, and the larger a table gets, the slower it is.
That felt good to get off my chest. Yes, Lua could start the auto-key at 0, but that might confuse people into thinking tables are arrays... well, I guess there's no avoiding that either way.13 -
Some days I feel like I work in a different universe.
Last night our alerting system sent out a dept. wide email regarding a high number of errors coming from the web site.
Email shows the number of errors and a summary of the error messages.
Ex. 60 errors
59 Object reference not set to an instance of an object
1 The remote server returned an unexpected response: (413) Request Entity Too Large
Web team responds to the email..
"Order processing team's service is returning a 413 error. I'll fill out a corrective action ticket in the morning to address that error in their service. "
Those tickets are taken pretty seriously by upper mgmt, so I thought someone on the order processing team would point out the 1 error vs. 59 (coming from the web team's code).
Two hours go by, nobody responds, so I decide to jump into something that was none of my business.
"Am I missing something? Can everyone see the 59 null reference exceptions? The 413 exception only occurred once. It was the null reference exceptions that triggered the alert. Looking back at the logs, the site has been bleeding null reference exceptions for hours. Not enough for an alert, but there appears to be a bug that needs to be looked into."
After a dept. managers meeting this morning:
MyBoss: "Whoa..you kicked the hornets nest with your response last night."
Me: "Good. What happened?"
<Dan dept VP, Jake web dept mgr>
MyBoss: "Dan asked Jake if they were going to fix the null reference exceptions and Jake got pissed. Said the null reference errors were caused by the 413 error."
Me: "How does he know that? They don't log any stack traces. I don't think those two systems don't even talk to one another."
<boss laughs>
MyBoss:"That's what Dan asked!..oh..then Jake started in on the alert thresholds were too low, and we need to look into fixing your alerting code."
Me: "What!? Good Lord, tell me you chimed in."
MyBoss: "Didn't have to. Dan starting laughing and said there better be a ticket submitted on their service within the next hour. Then Jake walked out of the meeting. Oh boy, he was pissed."
Me: "I don't understand how they operate over there. It's a different universe.
MyBoss: "Since the alert was for their system, nobody looked at the details. I know I didn't. If you didn't respond pointing out the real problem, they would have passed the buck to the other team and wasted hours chasing a non-existent problem. Now they have to take resources away from their main project and answer to the VP for the delay. I'm sure they are prefixing your name right now with 'that asshole'"
Me: "Not the first, won't be the last."2 -
What is a pointer?
A descriptive and ELI5 answer found on Reddit:
You have a house.
When you’re outside, and you want to go home, you don’t make another house right where you are, because it’s too big for you to carry around or take apart.
So you carry a piece of paper or store on your phone the address of your house. Now you always know exactly how to find your house so that you can go home.
The piece of paper or your phone is a variable.
The variable contains an address (a reference) to your house.
You can make a copy of this piece of paper and hand it out to your friends when you invite them over, instead of building each friend a copy of your house.
You can have an address book filled with pages, where each page is an address (i.e., an array of pointers). Each page you turn (each index you increment) goes to the directly next address-containing variable.
Now if you cut the address book in half along its height, and then attach the lower half behind the upper half, then you have a book with smaller pages but more pages.
You can store phone numbers in this, and even if it’s the same total size, you have double the number of pages and double the number of phone numbers (if you store one number on each page).
Now, since the pointers to home-addresses are different from pointers to phone-numbers, turning the page in an address book (increment pointer by 1) moves forward by one address.
But turning the page in the phone book (incrementing pointer by 1) leads you to the next phone number, even if you technically turned only “half a page”.
That’s how pointer arithmetic works.
Source: https://reddit.com/r/...8 -
Valid Until Invalid date? Well, that kinda makes sense.undefined js government javascript node.js paid success appointment reference number application form govt3
-
Besides the fact that there would be an error handler, wouldn't it store a phone number as an object other than a computable number, like a string, cuz phone number is like a handler, a reference, and not something you'd ever perform arithmetic on?6
-
Not a rant about anything in particular. Just a summary of some feelings stored in the hateful part of my heart.
Developing for Android: Add this third-party library to your Gradle build. Use (this) built-in Android class to make the thing work.
*Clicks link
Deprecated since API version SUCKMYDICK-7. Use (this) instead
*Clicks link
Deprecated since API version LICKMYBALLS-32. Use...
Developing for Windows: Please use (this) API call. It was literally already available before Bill Gates was born. Carbon dating has placed this item to older than the universe itself and it is likely the entry point for the big bang. It is also still the best way to accomplish (task).
Developing for Linux: "Hmm, I wonder how to use this"
> > > Some shitty mailing list in small blue monospace font tells you to reference a man page that is three versions behind but the only version available.
What? Those three sentences didn't explain it enough? Well, maybe you aren't cut out for this type of thing.
JavaScript: you know how it is.
SQL: You expect a decent-quality answer from stack overflow but you always get an outdated and hacky response and it's using syntax from Microsoft SQL. You need MySQL.
C#: A surprising number of Microsoft forum results ranking high on Google. You click on one in hopes that it will be of any sort of quality. You quickly close the tab and wonder why you ever even had hope.
Literally any REST API: Is it "query" or "q"? "UserID" or "user_id"? Oh, fuck, where's the docs again?
You thought you escaped JavaScript, but it was a trick!: Some bullshit library you downloaded to make your other library work redefined one of the global variables in the project you inherited. Now you get 347 "<x> is not a function" errors in your console. Good luck, asshole.
FontAwesome/ Material fonts/ Any icon font pack: You search "Close" for a close button icon. No results. You search "Simplified railroad crossing sign without the railroad". You get a close icon.
I think that's all of my pent up rage. Each of them were too small for an individual rant so I had to do this essay.2 -
Ruby’s fanciness bit me in the butt today. It’s pretty rare, but often confusing AF when it happens.
array = [1, 2, 3, 4, 5, 6, 7]
array.count +1 +2
# => 1
What the fuck?
array.count +1 +2 +3
# => 1
What the fuck?
+1 +2 +3
# => 6
Okay.
(array.count +1 +2 +3)
# => 1
What the fuck?
(7 +1 +2 +3)
# => 13
Okay...
array.count + 1 + 2 + 3
# => 13
Alright, so spaces matter here...?
((array.count) +1 +2 +3)
# => 13
But not here!? ... Oh. I think I know what’s going on.
Array#count
Returns the number of elements. If an argument is given, counts the number of elements which equal it using ==
Well fuck me.
Ruby is seeing `array.count(+1+2+3)` instead of `array.count()+1+2+3` since `+1` is a value, not an operator followed by a value as is the case with `+ 1`.
Now, why was I using +1 +2 instead of adding some spaces like I normally would? So they would match what was in the comment next to them for easier reference. Heh.
Future dev, I did this for you! So this is all your fault. :|36 -
I did a job interview recently for a company and the test was something like this.
In ruby, write a web server that will serve a specific line number from a text file.
I thought up a simple solution and a more advanced solution, but I opted to go with the simple solution and submit my work quickly. I made a nice web server with tests and everything and it used the sed command to get the line number from the file.
Now, they had various instructions, like it had to perform. They asked how it'd perform with 10G, 100G files. I thought "Eh... it'll be alright."
The solution they were looking for was the "advanced" solution that I thought up, which involved storing a binary file of 32/64 bit integers that reference the byte-offset of the line they're looking for. Basically a binary index file.
This violates all of my sensibilities, because I would never build a database indexer like this using ruby, of all things.
I thought it was a stupid test, and how do these companies honestly expect me to spend hours coding and then tell me I didn't go far enough? It's unethical.
I actually followed-up with the "advanced" solution a couple hours after hearing I was out, just to show them that their process is flawed.2 -
A while back I took over responsibility for getting one of our developers up to speed, after the other guy basically gave up on him.
Management insisted that this new recruit was our guy. I was kind of going along, since I had been there during the recruits first meeting with us, and he seemed to know his stuff.
I was very wrong. He was suppose to have been working with kubernetes, but suddenly did not know what a container was. After explaining it to him, he said along the lines of “yeah, sure, I was only testing you, I know all about this”.
He did the same thing for a number of other technologies. Always said that he knew very well what it was, and that I did not need to teach him those things.
Yet, he always seemed to get stuck with basic stuff, like installing node, setting up env-vars, starting docker-containers locally and that sort of things.
I mean, it is perfectly fine to say that you don’t know. I even consider it a great answer; it shows honesty and makes me trust you more. But with this guy, it was just impossible to get him up and running, since he always “knew”, but yet always needed help.
We had to let him go. Since I had been the one who had spent most time with him, it was natural that I was to be the one to tell him. I was not looking forward to it, I’m not reallly a persons-guy. Still, I was calm and honest with him and basically told him that I had found it impossible to work with him, kind of harshly.
He then asked me if he could put me on as a reference for his future job-applications. I told him politely that I did not think that was a great idea. He asked why, I told him I would be unable to say anything that would benefit him. He then asked me to lie.
I didn’t know what to say, except for “no!”. Never saw him again after that.3 -
I am very frustrated today and I do not know where to "scream" so I will post this here since I believe you will know how I feel.
Here's the case...
I am developing an e-commerce web application where we sell industrial parts. So my boss told me on March that when we are going to show these parts, we should not show Part Number to visitors because they will steal our information.
Ok, this makes sense but there was a problem.
The Primary Key for these products in our internal system is a string which is the Part Number itself.
I told him on March that we have to come up with another unique number for all the products that we are selling, so this unique number will be the primary key, not the Part Number. This will be best because I will be dependent from the original Part Number itself. And in every meeting he said "That is not priority". So I kept developing the part using the original Part Number as primary key and hid is from the web app. (But the Part Number still shows on URL or on search because this is how my boss designed the app.)
I built the app and is on a test server. Until one of out employees asked my boss: "There is no unique number or Part Number. How are the clients going to reference these parts? If a client buys 20 products and one of those has a problem, how is he going to tell us which products has a problem?"
My boss did not know what to say, and later said to me that I was right and primary key was priority.
I really hate when a guy that knows shit from developing does not listen to suggestions given by developers.
FUCK MY LIFE!
I'm sorry if you did not understand anything.5 -
Client from hell, next chapter.
As money comes in, project won't be canceled.
Only madness comes for free.
Newest insanity.... We had to explain that in a warehouse system a unique article number as an identifier is a must.... This discussion took 20 minutes.
The reason? Saving 2 secs as they don't have to enter the supplier item number....
Their plan?
Article Number can be...
Integer, automatically assigned
String, Supplier Name and Supplier Item Number seperated by a delimiter....
The argument that stopped this insanity... Costs.
We argued that if we are forced to implement something which we believe will be a high costs, no value, nonsense feature we won't do the necessary migration and programming after reversal as in 'find someone else who unbreaks this insanity'...
Did they understand that a warehouse system without article number for reference is dumb? Nope.1 -
went on contract for an insurance company to build their new platform. looking at the current system, found that they used lookup tables but they were putting the "description" column of the lookup into the main tables. I said that they should be putting a foreign key reference into these tables and the senior developers response... "then it would come up with a number rather than the description when I query the table"3
-
I used to think that I had matured. That I should stop letting my emotions get the better of me. Turns out there's only so much one can bottle up before it snaps.
Allow me to introduce you folks to this wonderful piece of software: PaddleOCR (https://github.com/PaddlePaddle/...). At this time I'll gladly take any free OCR library that isn't Tesseract. I saw the thing, thought: "Heh. 3 lines quick start. Cool.", and the accuracy is decent. I thought it was a treasure trove that I could shill to other people. That was before I found out how shit of a package it is.
First test, I found out that logging is enabled by default. Sure, logging is good. But I was already rocking my own logger, and I wanted it to shut the fuck up about its log because it was noise to the stuffs I actually wanted to log. Could not intercept its logging events, and somehow just importing it set the global logging level from INFO to DEBUG. Maybe it's Python's quirk, who knows. Check the source code, ah, the constructors gaves `show_log` arg to control logging. The fuck? Why? Why not let the user opt into your logs? Why is the logging on by default?
But sure, it's just logging. Surely, no big deal. SURELY, it's got decent documentation that is easily searchable. Oh, oh sweet summer child, there ain't. Docs are just some loosely bundled together Markdowns chucked into /doc. Hey, docs at least. Surely, surely there's something somewhere about all the args to the OCRer constructor somewhere. NOPE! Turns out, all the args, you gotta reference its `--help` switch on the command line. And like all "good" software from academia, unless you're part of academia, it's obtuse as fuck. Fine, fuck it, back to /doc, and it took me 10 minutes of rummaging to find the correct Markdown file that describes the params. And good-fucking-luck to you trying to translate all them command line args into Python constructor params.
"But PTH, you're overreacting!". No, fuck you, I'm not. Guess whose code broke today because of a 4th number version bump. Yes, you are reading correctly: My code broke, because of a 4th number version bump, from 2.6.0.1, to 2.6.0.2, introducing a breaking change. Why? Because apparently, upstream decided to nest the OCR result in another layer. Fuck knows why. They did change the doc. Guess what they didn't do. PROVIDING, A DAMN, RELEASE NOTE. Checked their repo, checked their tags, nothing marking any releases from the 3rd number. All releases goes straight to PyPI, quietly, silently, like a moron. And bless you if you tell me "Well you should have reviewed the docs". If you do that for your project, for all of your dependencies, my condolences.
Could I just fix it? Yes. Without ranting? Yes. But for fuck sake if you're writing software for a wide audience you're kinda expected to be even more sane in your software's structure and release conventions. Not this. And note: The people writing this, aren't random people without coding expertise. But man they feel like they are.5 -
Why do people who cannot write specs still write specs? There are guys who just cannot produce anything human readable.
- Don't list 50 things in the same sentence separated with semicolon. Don't you have list bullets in your Word?? Or table, anyone??
- Now that you managed to add a table, don't write a novel into the cells. Especially now that you have decided to use 30pt font size and 3cm wide columns.
- If it's not an equation, don't use parenthesis. Why? Since they (and this is just my opinion (someone else might think otherwise)) are a little bit (or a lot, depending on the reader(s)) annoying (or otherwise irritating) since they (the parenthesis) tend to make the text (of any kind) very difficult (hard) to read especially (there can be other reasons) when you (or someone else in the company) have decided to write reaaaally long and complex sentences which add no information but make the reader go back and forth of the text trying (and sometimes not succeeding) to make any sense out of it.
- Always remember to use cross-reference number like [1] but don't tell what it is referring to. Special bonus will be awarded, if the link is broken!
- Save space and time by not explaining things that you can just refer to. Just add vague "read from [1], [2] and [3] for info about this." And then expect the reader to go through thousands of pages of boring jargon.
And oh yeah, please ask comments in the review session and then ignore all of them, since "well technically all the information was in the spec". You just need to be Sherloc Holmes to connect the dots.2 -
¡rant|rant
Nice to do some refactoring of the whole data access layer of our core logistics software, let me tell an story.
The project is around 80k lines of code, with a lot of integrations with an ERP system and an sql database.
The ERP system is old, shitty api for it also, only static methods through an wrapper to an c++ library
imagine an order table.
To access an order, you would first need to open the database by calling Api.Open(...file paths) (yes, it's an fucking flat file type database)
Now the database is open, now you would open the orders table with method Api.Table(int tableId) and in return you would get an integer value, the pointer.
Now for the actual order. first you need to search for it by setting the search parameter to the column ID of the order number while checking all calls for some BS error code
Api.SetInt(int pointer, int column, int query Value)
Then call the find method.
Api.Find(int pointer)
Then to top this shitcake of an api of: if it doesn't find your shit it will use the "close enough" method of search.
And now to read a singe string 😑
First you will look in the outdated and incorrect documentation given to you from the devil himself and look for the column ID to find the length of the column.
Then you create a string variable with ALL FUCKING SPACES.
Now you call the Api.GetStr(int pointer, int column, ref string emptyString, int length)
Now you have passed your poor string to the api's demon orgy by reference.
Then some more BS error code checking.
Now you have read an string value 😀
Now keep in mind to repeat these steps for all 300+ columns in the order table.
News from the creators: SQL server? yes, sql is good so everything will be better?
Now imagine the poor developers that got tasked to convert this shitcake to use a MS SQL server, that they did.
Now I can honestly say that I found the best SQL server benchmark tool. This sucker creams out just above ~105K sql statements per second on peak and ~15K per second for 1.5 second to read an order. 1.5 second to read less than 4 fucking kilobytes!
Right at that moment I released that our software would grind to an fucking halt before even thinking about starting it. And that me & myself and I would be tasked to fix it.
4 months later and two weeks until functional beta, here I am. We created our own api with the SQL server 😀
And the outcome of all this...
Fixes bugs older than a year, Forces rewriting part of code base. Forces removal of dirty fixes. allows proper unit and integration testing and even database testing with snapshot feature.
The whole ERP system could be replaced with ~10 lines of code (provided same relational structure) on the application while adding it to our own API library.
Best part is probably the performance improvements 😀. Up to 4500 times faster and 60 times less memory usage also with only managed memory.3 -
!tech
recently i have been realising that i am utterly lonely. their isn't a group of people in life (apart from my parents) who aren't either paid to be with me (i.e office colleagues) or i am paying to be with them (i.e gym) and its very sad.
i don't have any siblings. the relatives are on sour terms, so no one visit. my parents are mostly loveless and the whole family is just focusing on sustaining than living or enjoying. i recently had some arguments with my friends and now they too are not on talking terms. .
I am a 25 year old, short , somewhat chubby guy in the most boring and safe field with no interesting interests except an average guy stuff ( cars, stocks, tech, career, sports... things that guys usually discuss).
I have been told on face that my vibe isn't interesting and i can honestly accept that . i myself wouldn't want to be with someone like me. if you are girl, then i will probably be talking to you for 30 seconds of joke-cum-fun-cum-serious-cum-caring stuff( i usually have 1-2 lines of witty stuff prepared) before going all silent and boring you the fuck out.
the next convo will be followed by an even dumber sentence but i will try to end it with a geeky joke or reference and a small laugh prompting you to also smile or fake laugh. and if you did that, then i will be desperate to keep you laughing, but my sentences will keep on getting more dumber and boring until you leave and categorise me as the most boring idiot/ "nice guy" you met. ( and meanwhile i am at the mental stage where i love you as the most precious thing of my world and imagining kids and life with you)
I can't care for anyone. I have seen too much parent fights, empty walls, money issues to understand how to care for anyone . my life is focused and sad.
shall i go on giving chocolates to everyyone in office to be popular? shall i ask a random gorl on the stret for her phone number? shall i start strolling in the park and try to talk to people? honestly, if i were a girl and someone does this to me, i would be shit scared and creeped out than falling for that guy.
then how the fuck i land myself into someone who wants to be with me? do i even want someone to be with me? or is loneliness the only thing i want?
i feel pretty okay for the most part of the day in this loneliness, except at some weird times like when am eating a platefu9 of chinese alone in some shop, or at night when i lock the door of a 9x9 large room and realise that i am the only one here.
i was once excited to grow up and do grown-up stuff like drive a car, take a solo tour, goto vaccination in every few days, be adventurous . but that has changed . i did all these things when i had people in my life. i somewhat felt motivated to do those, seeing that there were people who wanted to be with me during/after these things and care about me. now it just feels pointless.9 -
Context: New to typescript. Writing a thing, doing it for work, good opportunity to stretch my dev legs. Using a propriety lib, alternatives not an option.
Rant begin:
SOOOO, who the fuck thought THIS was a good idea:
1. Lib has minified react in dev (because closed source) meaning no downstream errors AND the entire premise of the lib is that a widget is a react component, so I'm writing typescript react the entire time without downstream errors
2. SHIT docs. By that, I mean there's an API reference page that's so sparse there's literally a set of CRUCIAL interfaces that only say the word 'Interface' on them. That's it. that's what i get. It's an interface. NO FUCKING SHIT SHERLOCK, what the fuck is it though? What's its purpose? Is it an interface for a dog? A dog that has a 'shit' property? or a cat? or a cat eating dog shit? Nobody fucking knows - the docs sure as fuck don't care.
3. No syntax highlighting - editors, IDEs (i've tried a few) can't even find the lib inside this environment, so Code and everything else thinks I'm importing shit that doesn't even exist - so no error prediction, code completion based on syntax of the library, none of that.
4. There are some EXTREMELY basic samples - these samples exclusively use React classes - no function components, no hooks, nada - just classes and even perfect replicas of the sample code display erratic behavior like errors about missing props, so that's mostly FUCKING USELESS
5. And this... this is where the straw breaks the fucking camel's back... there's no... there's no hot reloading... Do you know what that (in conjunction with the previous 4 fuckups) means?
When I write anything or I fuck up (which of course I'm doing every time I write half a line because how the fuck?) I have to restart the client and server EVERY FUCKING TIME and manually test to see if the error (THAT ONLY GETS REPORTED IN THE LOCAL UI) is gone or different.
Then, once I see the error, it isn't an error: it's the minified React error-decoder link and guess what? It isn't really clickable a link OR copyable, meaning that every FUCKING time I get a new error, I have to MANUALLY TYPE A FUCKING 50 CHAR URL TO FIND OUT A GENERIC REACT ERROR MESSAGE WITHOUT A LINE NUMBER OR ANY FUCKING CONTEXT. I HAVE TO DO THIS CONSTANTLY TO SEE IF ANYTHING I'M DOING EVEN WORKS.
6. There's no github to complain to the maintainers or search for issues because it's NOT FUCKING OPEN SOURCE so there is literally nothing to be fucking done about it.
This is due in a week and a half, found out about it last Friday. How's your day going?
PS: good to be back after a long respite from dev ranting.1 -
You know shit is going to hit the fan if the sentence "c++ is the same as java" is said because fuck all the underlying parts of software. It's all the fucking same. Oh and to write a newline in bash we don't use \n or so, we just put an empty echo in there. And fuck this #!/bin/bash line, I'm a teacher. I don't need to know how shit works to teach shit. Let's teach 'em you need stdio for printf even tho it compiles fine without on linux (wtf moment number one, asking em leaves you with "dunno..") and as someone who knows c you look at your terminal questioning everything you ever learned in your whole life. And then we let you look into the binaries with ldd and all the good stuff but we won't explain you why you can see a size difference in the compiled files even tho you included stdio in the second one, and all symbol tables show the exact same thing but dude chill, we don't know what's going on either.
Oh and btw don't use different directory names as we do in our examples. You won't find your own path, there is no tab key you can press to auto-fill shit.
But thats not everything. How about we fill a whole semester with "this is how to printf" but make you write a whole game with unity and c#. (not thaught even the slightest bit until then btw)
Now that you half-assed everything because we put you in a group full of fucks who don't even know what a compiler is but want to tell you you don't know shit and show you their non-working unfinished algorithms in some not-even-syntax-correct java...
...how about we finally go on with Algebra II: complex numbers, how they are going to fuck up your life, how we can do roots of negative numbers all of the sudden and let you do some probability shit no one ever fucking needs. BUT WHY DON'T YOU KNOW EVERYTHING ALREADY HMMMMM, IT'S YOUR SECOND LESSON, YOU WENT TO SCHOOL PLS BE A MATH PRO ASAP CUS YOU NEED IT SO MUCH BUT YOU DON'T NEED TO KNOW PROPER SYNTAX, HOW MEMORY MANAGEMENT WORKS, WHAT A REFERENCE IS AND PLS FINALLY FORGET THE WORD "ALLOCATION" IT DOESN'T PLAY A SINGLE ROLE YOU ARE STUDYING SOFTWARE DEVELOPMENT WHY ARE YOU SO BAD AT ECONOMICS IT MAKES NO SENSE I MEAN YOU HAD A WHOLE SEMESTER OF HOW TO GREET SOMEONE IN ENGLISH, MATHS > ECONOMICS > ENGLISH > FUCKING SHIT > CODING SKILL THATS HOW THE PRIORITIES WORK FOR US WHY DON'T YOU GET IT IT MAKES SO MUCH SENSE BRAH4 -
In reference to:
https://devrant.com/rants/2333925/...
Ideas are commonplace things. Just as a challenge today, in a two hour span, I came up with exactly 100 commercially viable ideas, some of which haven't even been tried yet by anyone that I know of.
This is me humblebragging, but it highlights an important lesson:
Good ideas are *genuinely* not worth the bytes or ink it takes to write them if you don't have the skill, connections, marketing, or cash to carry them forward.
I guarantee you, if you aggregated the commercially viable ideas of all the people on this platform, the list would number in the hundreds, probably in the thousands. And the list would be different every week.
Good ideas happen frequently enough because good ideas are a subset of the *ocean* of nonviable and stupid ideas that we all stumble on constantly, every day.
Like finding a needle in a stack of hay..or a nugget of golden corn by digging through piles and piles of steaming shit. It's a numbers game.2 -
Currently making a perfect sudoku webapp / plugin using native JS and html templates where I'm very enthousiast about.
It allows to select multiple cells and then put in a number and all selected have that number. It keeps state of every change, you can do unlimited redo's. Right click or double click someehere removes selection. Not built yet, but it will have a box where you can paste sudoku's you've found on the internet. I just parse 81 times [1-9] with regex. So all formats are supported including noisy ones as long the noise is not numbers. Making your own puzzle is very easy. Art is to make hard ones. I'm generating extra hard puzzles using C threading. For reference: there are 6,670,903,752,021,072,936,960 sudoku puzzles possible and from that I try to resolve the hard ones using simple human logging with brute forcing as fallback until it can use logic again. 30 million attempts to solve per secon. I should at some more logic. I don't do xwing or ywing, bs imho. You have to be a superhuman to spot xwing / ywing possibilities. I think i can imagine a better logic myself. We'll see.
And yes, that's a real screenshot. Puzzle is validated and it found issues. Marked with red font. Green is current selection by user11 -
Hello, everyone! Wild Matrix room just appeared: https://matrix.to//...
Hopefully, this one will be of more service to the people of devRant wanting to chat. It is bridged with dRCS, our Discord server, to make a faithful transition of subcommunities. Read the room's description for more detail. 🔗
Just at the start of it all, there is one more event you might like: a canvas like "r/place", but where you join via Lemmy. 🐭 I know, I know, Lemmy is only getting traction - but the community is thriving and making their own cool events already!
This one is called "Canvas": it's a fork of Pxls and a place where you can draw pixel art for all to see. The number of pixels replenishing on each person is limited and it takes time, so we REALLY need to gather together and draw this thing until the event ends!!! What thing? We are going to place a DEVRANT LOGO.
Canvas link and reference will be dropped in the comments below. Let's make devRant proud, for ducks' sake! 🦆🦆🦆🦆🦆🦆🦆🦆🦆🦆🦆🦆🦆🦆🦆🦆🦆🦆
It is recommended to join the channel to coordinate in real time and enjoy a good chat. 😁
https://matrix.to//...13 -
I absolutely hate it when companies use this or that medium for communications despite me asking them time and time again for another.
I have a mail server for more professional communications. The phone, only for stuff that won't matter if I inevitably end up forgetting about it (even more so now that Google made call recording more or less impossible, laws be damned). I will forget about a phone call no doubt. I've got better shit to do than to remember your manglement decisions, thank you very much. On mail, that's all nicely on my mail server for retrieval in several years even.
So I ask them to use the email address I gave them, a dedicated one for their company too (catch-all go brrr). Can't do that with phone numbers. Managing all those SIM cards aside, our government has now limited the amount of SIM cards one can have to 10. And texts and phone calls are not a long-term medium! And I can't share my phone number with just about anyone because people will inevitably spam the shit out of it, AND it's hard to replace! It's not a good medium! So with all due respect, companies - I couldn't care less what medium you prefer to use for your customers. You don't care about what your customer wants you to use - explicitly so! - and you lose a customer. It's as simple as that. Dealing with manglement is one thing, but dealing with manglement using the wrong media is something I'd really rather not do.
But hey I guess that virtue signalling is more "in" than actually listening to your goddamn customers nowadays? Let's replace another master/slave reference. You know, arguing that if we did that 2 years ago, George Floyd would've totally survived. Not by fixing the US police brutality, oh no no no. That's not the right way. Changing nomenclature and hashtags however, and not giving half a shit about your customers, yeah that's the way to go!1 -
When you get that customer's customer who forgets what the email is about:
-customer's customer was complaining they couldn't see a thing on some website-
Me: "That reference number didn't work... can you give me another reference number or something that might help find the thing?"
Customer's Customer: "I'm sorry but you're going to be more specific, I can't help you without a reference number."
-It appears customer's customer thinks I'm asking them for help now... let's see if...-
Me: "Never mind, close ticket."
Customer's Customer: "Ok!"
-me closes ticket-
┐(´ー`)┌3 -
2005 called. It wants its numbered file names back.
While I am mostly satisfied with "celluloid" as a worthy successor to xplayer, the first major disappointment I stumbled upon is `celluloid-shot0001.jpg`. Are we in 2005?
Just like xplayer, Celluloid, the new default media player of Linux Mint, should use proper, i.e. time-stamped names such as `celluloid-2023-04-10T00-47-42.jpg` or `celluloid-video_file_name-2023-04-10T00-47-42.jpg` for screenshots taken from videos, to eliminate the possibility of file name conflicts if files are moved into other directories, to make screenshots searchable by video file name, and to retain the date and time information if the files are moved to a device that does not support date and time stamp retention such as MTP (Media Transfer Protocol), and to allow for date range selection using wildcards in the terminal (e.g. `celluloid-2023-04*` for all screenshots from April 2023). Besides, PNG screenshots should be supported too, but that's out of scope here.
As a reference, the gnome and mate screenshot tools also pre-fill time stamps into the file name field.
Numbered file names were useful in an era when there was no VFAT and file names needed to have 8.3 file names that could impossibly fit a date and a time, and compact cameras used such names, but those times are long over. Just like the useless and annoying pull-to-refresh gesture on mobile apps and the Media Transfer Protocol, numbered file names belong to the technological graveyard.
If numbers are really desirable, at least `celluloid-shot0001.2023-04-10T00-47-42.jpg` should be used, to include both a number and a date. The command to get this date format is `date +"%Y-%m-%dT%H-%M-%S"`. For compatibility across operating systems, dashes instead of colons have to be used to separate hours and minutes and seconds.
Numbered file names are a thing of the past. Use time stamps.2 -
Control your searches like an ADULT damn it!!!
So we have records that can have any of a bazillion different reference numbers associated with them. No big deal. Everyone does right?
Our customer's love to run reports and so we have this one option for "just look at a hell of a lot of reference numbers". I call it the 'fuck all' search.
Really it is just there to find something that you don't know where a rando string or number might be in the record and just want to do a "fuck all" search across a number of likely fields to find it... and then presumably you'd be an adult and refine your search from there. LOL yeah right...
Customers get lazy and include that stupid option in their reports and we get a lot of.
Customer: "I always run this report (that includes the fuck all search) and now it isn't working. I want records that have ID 2222."
Me: "Yeah well that was only working because you were rando typing '2222' in like several fields and it would find those .... but now you quit doing that so it won't find them. If you want ID 2222, click the drop down and search by 'ID'. That will find it right away."
Customer: "But I want to just search by 'fuck all search' to find it..."
Me: "But then you get all these other records too right?"
Customer: "Yeah but I just delete them out of the spreadsheet ... "
Me: "Look watch this <screen share> there, look all records with an ID of 2222 and no more extra records you need to delete!!! How great is that?"
Customer: "But why do I have to do it this way now, I want to do it the old way..."
ಠ_ಠ
(granted I could add their ID to the fuck all search but we try to avoid adding too much because it gets out of hand / stops being useful the more fuck all it gets)3 -
Data wrangling is messy
I'm doing the vegetation maps for the game today, maybe rivers if it all goes smoothly.
I could probably do it by hand, but theres something like 60-70 ecoregions to chart,
each with their own species, both fauna and flora. And each has an elevation range its
found at in real life, so I want to use the heightmap to dictate that. Who has time for that? It's a lot of manual work.
And the night prior I'm thinking "oh this will be easy."
yeah, no.
(Also why does Devrant have to mangle my line breaks? -_-)
Laid out the requirements, how I could go about it, and the more I look the more involved
it gets.
So what I think I'll do is automate it. I already automated some of the map extraction, so
I don't see why I shouldn't just go the distance.
Also it means, later on, when I have access to better, higher resolution geographic data, updating it will be a smoother process. And even though I'm only interested in flora at the moment, theres no reason I can't reuse the same system to extract fauna information.
Of course in-game design there are some things you'll want to fudge. When the players are exploring outside the rockies in a mountainous area, maybe I still want to spawn the occasional mountain lion as a mid-tier enemy, even though our survivor might be outside the cats natural habitat. This could even be the prelude to a task you have to do, go take care of a dangerous
creature outside its normal hunting range. And who knows why it is there? Wild fire? Hunted by something *more* dangerous? Poaching? Maybe a nuke plant exploded and drove all the wildlife from an adjoining region?
who knows.
Having the extraction mostly automated goes a long way to updating those lists down the road.
But for now, flora.
For deciding plants and other features of the terrain what I can do is:
* rewrite pixeltile to take file names as input,
* along with a series of colors as a key (which are put into a SET to check each pixel against)
* input each region, one at a time, as the key, and the heightmap as the source image
* output only the region in the heightmap that corresponds to the ecoregion in the key.
* write a function to extract the palette from the outputted heightmap. (is this really needed?)
* arrange colors on the bottom or side of the image by hand, along with (in text) the elevation in feet for reference.
For automating this entire process I can go one step further:
* Do this entire process with the key colors I already snagged by hand, outputting region IDs as the file names.
* setup selenium
* selenium opens a link related to each elevation-map of a specific biome, and saves the text links
(so I dont have to hand-open them)
* I'll save the species and text by hand (assuming elevation data isn't listed)
* once I have a list of species and other details, to save them to csv, or json, or another format
* I save the list of species as csv or json or another format.
* then selenium opens this list, opens wikipedia for each, one at a time, and searches the text for elevation
* selenium saves out the species name (or an "unknown") for the species, and elevation, to a text file, along with the biome ID, and maybe the elevation code (from the heightmap) as a number or a color (probably a number, simplifies changing the heightmap later on)
Having done all this, I can start to assign species types, specific world tiles. The outputs for each region act as reference.
The only problem with the existing biome map (you can see it below, its ugly) is that it has a lot of "inbetween" colors. Theres a few things I can do here. I can treat those as a "mixing" between regions, dictating the chance of one biome's plants or the other's spawning. This seems a little complicated and dependent on a scraped together standard rather than actual data. So I'm thinking instead what I'll do is I'll implement biome transitions in code, which makes more sense, and decouples it from relying on the underlaying data. also prevents species and terrain from generating in say, towns on the borders of region, where certain plants or terrain features would be unnatural. Part of what makes an ecoregion unique is that geography has lead to relative isolation and evolutionary development of each region (usually thanks to mountains, rivers, and large impassible expanses like deserts).
Maybe I'll stuff it all into a giant bson file or maybe sqlite. Don't know yet.
As an entry level programmer I may not know what I'm doing, and I may be supposed to be looking for a job, but that won't stop me from procrastinating.
Data wrangling is fun.1 -
I have been 'called' by a very unusual number. Seems to originate from Russia
For reference, don't call them back!
+79540118049
Now I'm in paranoid mode due to The whole pegasus affair where a simple phone call already infects your phone
Am I paranoid android?7 -
something something the weed number is the date lololololol
MY NAME IS DRACULA
I'M HERE TO SMOKE THE VEED
(name the reference)1 -
In reference to https://devrant.com/rants/2333764/...
I've always wanted a desktop I could treat like apple maps. Pan and zoom (or on touch screens, pinch to exit, opposite to zoom).
Drag to create a new folder/region and name it, like a constellation of files. Zoom or click to expand, and zoom out to exit.
I guess it'd be messy af, but it's a different way of thinking and organizing for some of us.
Some of us think hierarchically (classic folders), and some of us think in two dimensions.
I dunno, I've just always found it easier to find things by organizing into 2d groups, no matter the number of files, versus having to scroll and search.
But you're reading a devrant by a guy who has north of 25-30k bookmarks, so I'm probably clinically insane anyway.5 -
Can there be a happy rant?
This is going to be a bit of a rambling semi coherent story here:
So this customer who just doesn't know what their data schema is or how they use it (they're a conglomeration of companies so maybe you get how that works out in a database). For every record there's like a ton of reference number type things mapped all over the DB to fit each companies needs needs.
To each company the data means something different, they use the data differently, and despite their claims otherwise, I think there are some logical conflicts in there regarding things like "This widget is owned by company A, division B, user C.". I'm also pretty sure different companies actually don't agree on who owns what... but when I show them they just sort of dance around what they've said in the past...
So I write a report (just an SQL query that outputs ... somewhere ... I mean what isn't that?) that tells them about all the things that happened given X, Y, Z.
Then every damn morning they'd get all up in arms about how some things are 'missing' but sometimes they don't know what or why because they've no clue what the underlying data actually is / their own people don't enter the data in a consistent way. (garbage in garbage out man...)
So I've struggled with this for a few weeks and been really frustrated. Every morning when I'm trying to do something else ... emails about how something isn't working / missing.
In the meantime I'm also frustrated by inquiries about "hey this is just a simple report right?" (to be clear folks asking that aren't being jerks, and they're not wrong ... it really should be simple)
Anyway my boss being the good guy he is offers to take it over, so I can do some things. Also sometimes it helps just to have someone else own something / not just look it over.
So a few days into this.... yup, emails coming in about things 'missing' or 'wrong' every day.
Like it sucks, but it's nice to see it suck for someone else too as validation. -
Talked with a recruiter a few times. He kept presenting jobs that were 1.5 hours away, despite how many times I told him specifically my car would not make it that far regularly.
I eventually started ignoring his calls and emails... Until he called my mom, and a former boss (reference), leaving messages with them to call him back. I don't even know how he got my mom's number.
I wrote him a very clear email after that and haven't heard from him since.1 -
During my small tenure as the lead mobile developer for a logistics company I had to manage my stacks between native Android applications in Java and native apps in IOS.
Back then, swift was barely coming into version 3 and as such the transition was not trustworthy enough for me to discard Obj C. So I went with Obj C and kept my knowledge of Swift in the back. It was not difficult since I had always liked Obj C for some reason. The language was what made me click with pointers and understand them well enough to feel more comfortable with C as it was a strict superset from said language. It was enjoyable really and making apps for IOS made me appreciate the ecosystem that much better and realize the level of dedication that the engineering team at Apple used for their compilation protocols. It was my first exposure to ARC(Automatic Reference Counting) as a "form" of garbage collection per se. The tooling in particular was nice, normally with xcode you have a 50/50 chance of it being great or shit. For me it was a mixture of both really, but the number of crashes or unexpected behavior was FAR lesser than what I had in Android back when we still used eclipse and even when we started to use Android Studio.
Developing IOS apps was also what made me see why IOS apps have that distinctive shine and why their phones required less memory(RAM). It was a pleasant experience.
The whole ordeal also left me with a bad taste for Android development. Don't get me wrong, I love my Android phones. But I firmly believe that unless you pay top dollar for an android manufacturer such as Samsung, motorla or lg then you will have lag galore. And man.....everyone that would try to prove me wrong always had to make excuses later on(no, your $200_$300 dllr android device just didn't cut it my dude)
It really sucks sometimes for Android development. I want to know what Google got so wrong that they made the decisions they made in order to make people design other tools such as React Native, Cordova, Ionic, phonegapp, titanium, xamarin(which is shit imo) codename one and many others. With IOS i never considered going for something different than Native since the API just seemed so well designed and far superior to me from an architectural point of view.
Fast forward to 2018(almost 2019) adn Google had talks about flutter for a while and how they make it seem that they are fixing how they want people to design apps.
You see. I firmly believe that tech stacks work in 2 ways:
1 people love a stack so much they start to develop cool ADDITIONS to it(see the awesomeios repo) to expand on the standard libraries
2 people start to FIX a stack because the implementation is broken, lacking in functionality, hard to use by itself: see okhttp, legit all the Square libs, butterknife etc etc etc and etc
From this I can conclude 2 things: people love developing for IOS because the ecosystem is nice and dev friendly, and people like to develop for Android in spite of how Google manages their API. Seriously Android is a great OS and having apps that work awesomely in spite of how hard it is to create applications for said platform just shows a level of love and dedication that is unmatched.
This is why I find it hard, and even mean to call out on one product over the other. Despite the morals behind the 2 leading companies inferred from my post, the develpers are what makes the situation better or worse.
So just fuck it and develop and use for what you want.
Honorific mention to PHP and the php developer community which is a mixture of fixing and adding in spite of the ammount of hatred that such coolness gets from a lot of peeps :P
Oh and I got a couple of mobile contracts in the way, this is why I made this post.
And I still hate developing for Android even though I love Java.3 -
The number of concurrent transformations impacting more than half of the codebase in Orchid surpassed 4, so instead of walking the reference graph for each of these I'm updating the whole codebase, from lexer to runtime, in a single pass.
In this process, I also got to reread a lot of code from a year ago. This is the project I learned Rust with. It's incredible, not just how much better I've gotten at this language, but also how much better I've gotten at structuring code on general.
Interestingly though my problem-solving ability seems to be the same. I can tell this by looking at the utilities I made to solve specific well-defined abstract problems. I may have superficial issues with how the code is spelled out in text, but the logic itself is as good as anything I could come up with today.2 -
!rant !dev
So, following up my last rant.
https://devrant.com/rants/2433162
I quit on Friday, this is what I said to my bosses.
"In the last week I had, 2 panic attacks, and I have 2 theories for this, one is that I have underlying psychological problems, the other theory is that we are under an impossible task, I choose to say now that I have to quit because I have psychological issues, but if you are willing to hear my other theory, that involves saying that meeting the deadline is not viable, then I can tell you that, so do want to listen that part?.
Bosses: No, we heard enough, we are going to have your contract terminated in order, and we will let you know when you can come and pick your paycheck."
So, that's them. Now about me and how I re-discovered GTD, or more precisely how I organized my whole weekend using taskwarrior with GTD, and why I think is going to be useful as a freelancer.
Before I feel good about telling you about my weekend I have to tell you a few things about myself.
I am a very impulsive person, I have a lot of energy in short surges, so I have to be able to maximize my activity when I'm in a surge, and I have to maximize my rest when I am not.
That's hard to do, it requires a balanced lifestyle, I am also very prone to being neurotic, and overwhelmed by the amount of stuff that I want to do.
And on top of that, when I am resting, I have surges of things that I want to have, do, or implement, it could be software related, as "Doing an app that will be the Uber of home services", to house improvements like, "I have to fix that leaking roof", and all the sort of stuff that happens in between hardware and software. That surge of consciousness doesn't allow me to have the proper rest that I need before I engage with activities again.
Because of this I have a very cyclic rhythm, with whole weeks burning my energy into doing stuff, and weeks resting doing very little and thinking too much.
Now about my weekend. Friday night I was browsing the web, and a thought came to my head. "The way you use your terminal, says a lot about your personality", and I got curious, so I searched for, "Show me your terminal", and found a post in dev.to to see all kind of nice terminal setups, from the very minimalist to very feature rich oh-my-zsh themes with plugins for git, aws and what not. One of these pictures really got my attention, a guy had set up his terminal to show him, how many task has he done in the day, and how many cups of coffee has he had.
So by investigating how he set up his terminal to show in the prompt the number of successfully completed tasks in the day, I found out that he was using taskwarrior, he was also kind enough to share the source code of his prompt setup, which I bookmarked to later incorporate that into my oh-my-zsh config.
After reading about taskwarrior, I also got a reference to GTD, I don't remember if this was one of those thoughts that I have and follow immediately, or if I read something that led me to a YouTube video summarizing GTD.
In the end, after watching that GTD video, I decided to give it a try to organize my life, and help me find a remote job, keep my house in order, plan my social activities as "hang out with friends", "visit mom and dad", and give the proper amount of attention to my GF, with whom I am deeply in love, and willing to spend the remaining of my years with her.
So my fist task was.
task add Ask for GF's parents blessing.
Which of course I have no intention of doing right now, but is one of the things that I will eventually have to do.
Then it started, I started adding tasks, and things to do, and go through the whole Capture phase of GTD.
Now it is a good time to write a small summary of what I think GTD is.
GTD is a life habit of organizing your life in todo-lists. And it was a very specific core method, that in the video summary that I watched was called CPR.
Capture, Process and Review.
Capture:
When you capture you just add your tasks to a bucket list.
So I took a notebook and started writing down everything that I wanted to have done. I also started to capture ideas as they came up to me, I did this by writing a telegram saved message in my phone, or directly adding it as a task in TW.
Process:
I read my telegram messages and put them into my task warrior list, then I started to organize my tasks into projects, breaking down every task that was not an atomic unit.
* And different projects started to emerge from this. One of them was project:Housekeeping.
And here's my screenshot of what I did this weekend, also the number of projects that I have, and all the things that I have to do in order to have what I think would be a very balanced, fun, and productive life.
You'll be able to see in the screenshot, that there's a blocked task, yes, tw allows you to organize dependencies too, so one task is delegated, and blocked by the delegation task.1 -
What the Fun Javascript Part 2
With reference to my old rant : https://devrant.com/rants/1445754/...
An explanation to this...
In JS whenever it sees '==' it has a set of rules that it follows before comparing quantities...
One such rule is...
When we compare a number with a boolean using '==' it first converts boolean into number then compares the two quantities...
So in this case..
When we convert true to number we get 1 and when we compare 1 and 2 they are obviously unequal hence we get false...1 -
Hello All, I am working on java project and I want to know the source code of the Exam Seating Arrangement System Project. Basically, This java project strives at building an automated seating arrangement for students for exams, on the basis of different inputs. There are primarily two entities, the admin and the student. Both entities can log in and register to the system, check and access the system as per the approval granted to them. The admin can see all the relevant details of the students and provide the input to the system taking into consideration the need like the branch, semester, year, subject of the student. Admin will input details like the total students, available classes with the number of seats, etc. and I have taken this reference from here (https://interviewbit.com/blog/...). Can anyone provide me the source code of the exam seating arrangement system?1
-
MCIA-LEVEL-1 MuleSoft Certified Integration Architect - Level 1 test is one of the MuleSoft affirmation tests, which can upgrade your position and work on your life. However, how do get ready for the MCIA-LEVEL-1 test well and pass it effectively? While scanning on the web asset for MCIA-LEVEL-1 MuleSoft Certified Integration Architect - Level 1 test, DumpsCafe strongly prescribes you pick an online MCIA-LEVEL-1 practice test. MuleSoft Certified Integration Architect - Level 1 MCIA-LEVEL-1 test questions are kept in touch with the best expectations of specialized exactness, given by our guaranteed well-informed authorities and distributed creators for advancement. You will pass MCIA-LEVEL-1 MuleSoft Certified Integration Architect - Level 1 test with online review materials, 100% cashback included.
Get ready to pass the exam with the help of the MuleSoft MCIA-LEVEL-1 exam:
Readiness of accreditation tests could be covered with two asset types. The first is the review guides, reference books, and study discussions that are explained and suitable for developing data starting from the earliest stage. Aside from the video instructional exercises and talks are a decent choice to facilitate the aggravation of through study and are somewhat make the review cycle really fascinating in any case these interest time and fixation from the student.
Brilliant up-and-comers who wish to make a strong establishment out and out assessment themes and associated advancements commonly blend video addresses with a concentrate on advisers for harvest the upsides of each, however, practice tests or practice test motors is one significant review device that goes ordinarily unnoted by most competitors.
Practice tests are planned with our specialists to make test possibilities test their insight on abilities accomplished in the course, just as possibilities become agreeable and acquainted with the genuine test climate. Measurements have shown test uneasiness assumes a lot greater part in an understudy's disappointment in the test than the dread of the obscure.
Confirmation questions master group suggests setting up certain notes on these subjects alongside it remember to rehearse MuleSoft MCIA-LEVEL-1 dumps which had been composed by our master group, each of these can help you loads to clear this test with superb imprints.
DumpsCafe gives you the latest MCIA-LEVEL-1 exam questions:
DumpsCafe has in addition presented two or three supporting devices and learning modes that will help you in having a fitting enthusiasm for the fundamental limits and to additional work with your MCIA-LEVEL-1 dumps status association with getting MuleSoft Architect attestation with a guarantee. A couple of up-and-comers need to further develop abilities to get movements or others need to have a promising beginning in the MuleSoft MCIA-LEVEL-1 dumps announcement world and with this part, they can plan as shown by their singular basics.
DumpsCafe MCIA-LEVEL-1 test dumps are similarly open in the pdf plan so you can use this report in any of your splendid devices whether it is a PDA, tablet, or even a PC. We have made this relationship in the pdf record so it can end up being less difficult for you to utilize and you can start perusing for your MuleSoft Certified Integration Architect - Level 1 test wherever.
MuleSoft MCIA-LEVEL-1 content makes you ready for the real exam:
Rather than following the ages-old idea of MuleSoft Certified Integration Architect - Level 1 test readiness utilizing voluminous books and notes, DumpsCafe has presented a brief, direct, and most important substance that is incredibly useful in passing any accreditation MuleSoft Certified Integration Architect - Level 1 test. For an occurrence, their MCIA-LEVEL-1 refreshed review guide covers the whole prospectus in a particular number of inquiries and replies. The data, given in the review questions, is streamlined to the level of a normal test competitor. Any place, it is fundamental, the appropriate responses have been clarified further with the assistance of reproductions, diagrams, and additional notes.
Conclusion:
Need to pass your MuleSoft MCIA-LEVEL-1 test in the primary attempt? Download the most recent MuleSoft Certified Integration Architect - Level 1 MCIA-LEVEL-1 dumps and invest however much energy as could be expected to rehearse before your MuleSoft Certified Integration Architect - Level 1 exam. Passcert group profoundly proposes everybody purchase MuleSoft Certified Integration Architect - Level 1 MCIA-LEVEL-1 dumps when you will take your test in several weeks. Please keep sufficient opportunities to practice. DumpsCafe guarantees 100% passing your MuleSoft certificate MCIA-LEVEL-1 test effectively.
For more info: www(dot)dumpscafe(dot)com/Braindumps-MCIA-Level-1.html1