Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
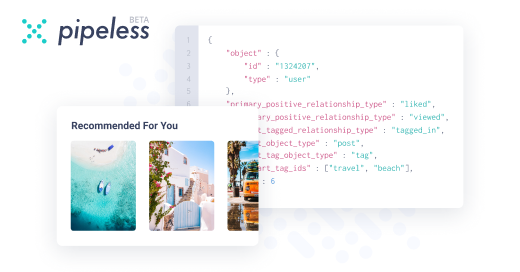
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "variables help"
-
I recalled a seemingly simple task I took on.
We were building a booking system, and I had to figure out how to retrieve bookings by a certain date range.
Upfront, the tasked seemed simple until I realised I had to both figure out the logic and the SQL statements needed to retrieve all bookings within a certain date range in one query.
I ended up drawing a model to help me visualise the various date-range criteria to be satisfied. And used unit tests to help me think through each date range criterion and make sure they were accurate. Some were obviously from paranoia, but better to be safe than sorry...
After that, I had wrote down raw SQL directly into Sequel Pro first to make sure my query logic was accurate too, before translating into something the ORM equivalent. This was when I learned how to define and use variables in SQL. The variables were throw-away code; I just didn't want to have to hard-code the test date-ranges over and over again; minimise chances of spelling errors.
Needless to say, felt my problem-solving skills went up one level after this task. Saw my coding style and unit tests improve. And also the thought processes that go into how to maintain code quality...4 -
Today a colleague of mine asked me to help with some javascript. So I said sure, it will be done in 5 minutes. Im a fullstack developer with a focus on backend in this project.
So I opened the frontend part and was amazed how shit the javascript file was. Yes you read it right FILE...
One big file with a lot of variables in the window scope.
Because she was in “charge” of the frontenders because she works there a bit longer then me I never checked the frontend code.
I said I wont/cant help unless I see better code. I explained to a trainee what could be done to change it and Im impressed that the trainee did a better job then the employee and quick as well.
Got the whole code in seperate files with each part of the code in seperate scopes within 2 hours.
What Im saying here is that even as student, intern or trainee you can know things better thsn someone with experience, dont be afraid to speak up. Because everyone can learn from eachother.7 -
I was asked to help with the website of this one club. Their 'IT head' is a business person. I told them no, but they sent me something anyways.
They sent me a zip file of their code
instead of giving me access to their GitHub repo. I then realized that they were using 3-year-old NodeJS and Express to power their static website and doing blog posts as JavaScript modules.
A second part of their architecture which was related to member sign up was horribly broken and also written in Node. I found out that they hard coded credentials to their Google Apps account, despite having the setup to pass it via environment variables.
And now they are worried that their sign up isn't working. Their developer resigned.
They want me to help them fix it within a very small timeframe. So they can use the code to collect membership fees.
This is what happens when you have business people develop code.6 -
I have to rant a bit about the toxic reactions to a constructive Q&A website.
People keep complaining that they get downvotes and corrections, or stuff like that.
Are you fucking kidding me?
So you expect people to spend their own time for absolutely free, to help you, while you don't even want to invest in describing the issue you're having properly? And then complain that people are having issues in understanding your questions?
Let's look at this scientifically. Let's gather up some questions that have been received badly on SO in the last few hours. From the top (simply put https://stackoverflow.com/questions... in front of the id):
47619033 - person wants a discussion about an algorithm while not providing any information about what worked and what failed. "Please write a program for me". Breaking at least 2 rules.
47619027 - "check out my videos" spam
47619030 - "Here's the manual that has my answer but I can't find my answer in it".
47619004 - "how do I keep variables in memory"
47618997 - debug this exception, I'll give you no info on what I tried and failed. Screw this, you guys figure this out, I'm going out for beer.
47618993 - expects everyone to guess what the input is, what the expected output is, and whether he has read what HashMap is in the manual. But sure, this question is so far the best out of all the bad ones.
47618985 - please write code according to my specifications
Should I go on? There wasn't a single clear question about problems in code in this entire small set. Be free to continue searching, let me know if you find something that:
1. You understand what's being asked
2. Answer is clear and non-ambiguous (ex. NOT "which language is the coolest?")
3. Not asking someone to write a program for them.
4. Answer is not found in the most basic form of manuals (ex. php.net)
5. Is about programming.
The point is:
If you get downvoted on Stackoverflow - then you wrote a shitty question. Instead of coming over here and venting uselessly, simply address the concerns and at least TRY to write a clear question if you expect any answers.5 -
I could bitch about XSLT again, as that was certainly painful, but that’s less about learning a skill and more about understanding someone else’s mental diarrhea, so let me pick something else.
My most painful learning experience was probably pointers, but not pointers in the usual sense of `char *ptr` in C and how they’re totally confusing at first. I mean, it was that too, but in addition it was how I had absolutely none of the background needed to understand them, not having any learning material (nor guidance), nor even a typical compiler to tell me what i was doing wrong — and on top of all of that, only being able to run code on a device that would crash/halt/freak out whenever i made a mistake. It was an absolute nightmare.
Here’s the story:
Someone gave me the game RACE for my TI-83 calculator, but it turned out to be an unlocked version, which means I could edit it and see the code. I discovered this later on by accident while trying to play it during class, and when I looked at it, all I saw was incomprehensible garbage. I closed it, and the game no longer worked. Looking back I must have changed something, but then I thought it was just magic. It took me a long time to get curious enough to look at it again.
But in the meantime, I ended up played with these “programs” a little, and made some really simple ones, and later some somewhat complex ones. So the next time I opened RACE again I kind of understood what it was doing.
Moving on, I spent a year learning TI-Basic, and eventually reached the limit of what it could do. Along the way, I learned that all of the really amazing games/utilities that were incredibly fast, had greyscale graphics, lowercase text, no runtime indicator, etc. were written in “Assembly,” so naturally I wanted to use that, too.
I had no idea what it was, but it was the obvious next step for me, so I started teaching myself. It was z80 Assembly, and there was practically no documents, resources, nothing helpful online.
I found the specs, and a few terrible docs and other sources, but with only one year of programming experience, I didn’t really understand what they were telling me. This was before stackoverflow, etc., too, so what little help I found was mostly from forum posts, IRC (mostly got ignored or made fun of), and reading other people’s source when I could find it. And usually that was less than clear.
And here’s where we dive into the specifics. Starting with so little experience, and in TI-Basic of all things, meant I had zero understanding of pointers, memory and addresses, the stack, heap, data structures, interrupts, clocks, etc. I had mastered everything TI-Basic offered, which astoundingly included arrays and matrices (six of each), but it hid everything else except basic logic and flow control. (No, there weren’t even functions; it has labels and goto.) It has 27 numeric variables (A-Z and theta, can store either float or complex numbers), 8 Lists (numeric arrays), 6 matricies (2d numeric arrays), 10 strings, and a few other things like “equations” and literal bitmap pictures.
Soo… I went from knowing only that to learning pointers. And pointer math. And data structures. And pointers to pointers, and the stack, and function calls, and all that goodness. And remember, I was learning and writing all of this in plain Assembly, in notepad (or on paper at school), not in C or C++ with a teacher, a textbook, SO, and an intelligent compiler with its incredibly helpful type checking and warnings. Just raw trial and error. I learned what I could from whatever cryptic sources I could find (and understand) online, and applied it.
But actually using what I learned? If a pointer was wrong, it resulted in unexpected behavior, memory corruption, freezes, etc. I didn’t have a debugger, an emulator, etc. I had notepad, the barebones compiler, and my calculator.
Also, iterating meant changing my code, recompiling, factory resetting my calculator (removing the battery for 30+ sec) because bugs usually froze it or corrupted something, then transferring the new program over, and finally running it. It was soo slowwwww. But I made steady progress.
Painful learning experience? Check.
Pointer hell? Absolutely.4 -
I told a guy to implement an algorithm in cpp. He wrote this weird 600+ lines of code which contains only global variables and void functions then I told him to make it object oriented and he just put all those garbage in the class and gave me back and on top of that class name is Template and file name is template.cpp. I don't have words to describe his code. May be this picture can help you understand my state. Oh, if you think this matchOn_r1, r2,r3 are different then you are mistaken they are just different with one argument (one global argument). This is just part of the code. He has this shit all over the place. Why the fuck this kind of people exists?13
-
I'm coming off a lengthy staff augmentation assignment awful enough that I feel like I need to be rehabilitated to convince myself that I even want to be a software developer.
They needed someone who does .NET. It turns out what they meant was someone to copy and paste massive amounts of code that their EA calls a "framework." Just copy and paste this entire repo, make a whole ton of tweaks that for whatever reason never make their way back into the "template," and then make a few edits for some specific functionality. And then repeat. And repeat. Over a dozen times.
The code is unbelievable. Everything is stacked into giant classes that inherit from each other. There's no dependency inversion. The classes have default constructors with a comment "for unit testing" and then the "real" code uses a different one.
It's full of projects, classes, and methods with weird names that don't do anything. The class and method names sound like they mean something but don't. So after a dozen times I tried to refactor, and the EA threw a hissy fit. Deleting dead code, reducing three levels of inheritance to a simple class, and renaming stuff to indicate what it does are all violations of "standards." I had to go back to the template and start over.
This guy actually recorded a video of himself giving developers instructions on how to copy and paste his awful code.
Then he randomly invents new "standards." A class that reads messages from a queue and processes them shouldn't process them anymore. It should read them and put them in another queue, and then we add more complication by reading from that queue. The reason? We might want to use the original queue for something else one day. I'm pretty sure rewriting working code to meet requirements no one has is as close as you can get to the opposite of Agile.
I fixed some major bugs during my refactor, and missed one the second time after I started over. So stuff actually broke in production because I took points off the board and "fixed" what worked to add back in dead code, variables that aren't used, etc.
In the process, I asked the EA how he wanted me to do this stuff, because I know that he makes up "standards" on the fly and whatever I do may or may not be what he was imagining. We had a tight deadline and I didn't really have time to guess, read his mind, get it wrong, and start over. So we scheduled an hour for him to show me what he wanted.
He said it would take fifteen minutes. He used the first fifteen insisting that he would not explain what he wanted, and besides he didn't remember how all of the code he wrote worked anyway so I would just have to spend more time studying his masterpiece and stepping through it in the debugger.
Being accountable to my team, I insisted that we needed to spend the scheduled hour on him actually explaining what he wanted. He started yelling and hung up. I had to explain to management that I could figure out how to make his "framework" work, but it would take longer and there was no guarantee that when it was done it would magically converge on whatever he was imagining. We totally blew that deadline.
When the .NET work was done, I got sucked into another part of the same project where they were writing massive 500 line SQL stored procedures that no one could understand. They would write a dozen before sending any to QA, then find out that there was a scenario or two not accounted for, and rewrite them all. And repeat. And repeat. Eventually it consisted of, one again, copying and pasting existing procedures into new ones.
At one point one dev asked me to help him test his procedure. I said sure, tell me the scenarios for which I needed to test. He didn't know. My question was the equivalent of asking, "Tell me what you think your code does," and he couldn't answer it. If the guy who wrote it doesn't know what it does right after he wrote it and you certainly can't tell by reading it, and there's dozens of these procedures, all the same but slightly different, how is anyone ever going to read them in a month or a year? What happens when someone needs to change them? What happens when someone finds another defect, and there are going to be a ton of them?
It's a nightmare. Why interview me with all sorts of questions about my dev skills if the plan is to have me copy and paste stuff and carefully avoid applying anything that I know?
The people are all nice except for their evil XEB (Xenophobe Expert Beginner) EA who has no business writing a line of code, ever, and certainly shouldn't be reviewing it.
I've tried to keep my sanity by answering stackoverflow questions once in a while and sometimes turning evil things I was forced to do into constructive blog posts to which I cannot link to preserve my anonymity. I feel like I've taken a six-month detour from software development to shovel crap. Never again. Lesson learned. Next time they're not interviewing me. I'm interviewing them. I'm a professional.9 -
I started attending this IoT class in some computer training school. During my first class, I was early because I had the raspberry pi class earlier in the day. A guy came up to me and started chatting to me, he was bragging about how he created some big projects, how he works in his dad's company which develops IoT products (he codes it). Later on in class he talked about how he hacked his school's server or something and changed his marks. Whenever he brags, he has a tendency to use a deeper voice (which is pretty annoying).
Anyways so I thought he is pretty good and maybe I can learn a thing or two from him. A few class later, I started having my doubts, why? Because he doesn't know how to debug code, he copies the lecturer's code and still copies it wrong, and he doesn't know what variables and constants are. He uses IE and doesn't know about GitHub.
Now he asks me or the guy in front for help in class. He makes the class more fun, it's funny listening to him brag. Love it.2 -
I'm taking an Intro to Programming course along aside an Intro to Computers class so I already know about basic programing, still very new to it though! At the end of the Intro to Comp, we're learning about programming and a classmate was having a hard time understanding assignments and variables.
I explained the idea of the input command at least three times and he kept trying to print out a statement he just wanted to write in instead of printing out the input that the user will enter. He also assigned the same name to different variables.
Explained that what he was doing was not versatile and not useful, explained in an example situation, explained by writing some lines of code myself (THRICE), and he still had trouble understanding me. I didn't want to hold his hand the entire time.
Glad that I was called to leave early since I might get too frustrated if I had to stay back and continue to help him.
Hope he managed to finish the assignments successfully though! Feel kinda bad now...2 -
Being a programmer in a scientific discipline can be infuriating.
using "no one" ="almost no one"
using everyone = "almost everyone"
1. No one knows what even the very idea of good practice is. And everyone refuses to learn. 3k lines of repetitive copy pasted main. 500 lines of plotting method.
2. Raw C-style pointer based array creation. Won't use develope array libraries because what if development stops. FUCKING HAVE YOU SEEN YOUR CODE WHAT IF DEVELOPMENT ON YOUR CODE STOPS. FUCK.
3. LOOP VARIABLES DECLARED AT THE BEGINNING OF THE METHOD WHY.
4. Everyone wants to make modular, independent code. No one wants to use OOP. NOPE. ALL IN ONE FILE. WRITE C++ LIKE A FUCKING PYTHON NOTEBOOK. FUCK.
5. LIBRARIES OH MY GOD PLEASE DO NOT CODE UP YOUR MATRIX MULTIPLICATION. PLEASE DO NOT TRIPLE LOOP IT. NO. THE LINEAR ALGEBRA LIBRARY WILL STAY IN DEVELOPMENT.
6. Please realize that literally not one comment over an 1800 line file does not help anyone.
FUCKING. WHY. WHY ARE WE SCIENTISTS SO GOOD AT SCIENCE AND SO FUCKING SHIT AT THE CODE THAT MAKES OUR SCIENCE HAPPEN. WHY. FUCKING. WHY. FUCK.undefined rage no comments scientific computing fuck this shit wall of text bad code science fuck c++ fucking4 -
Nightmare IRL:
Your colleague is in PTO for 2 weeks.
You are in charge of maintaining his project along with yours, CI, code, tests and everything.
Your colleague's code base is a real master piece of shit when you look at it closer. By shit, I mean hardcoded values everywhere, random sleeps now and then, 20 if branches that could be replaced by maps, variables named a b c d everywhere, try catch to silence errors that should not be silenced, etc.
Your colleague left the CI and code broken as shit. Takes forever to run on my goddamn computer.
PMs are spamming you: "What is going on? It's red everywhere. Help! Plz fix this! We are going to release tomorrow!"
FML6 -
Two big moments today:
1. Holy hell, how did I ever get on without a proper debugger? Was debugging some old code by eye (following along and keeping track mentally, of what the variables should be and what each step did). That didn't work because the code isn't intuitive. Tried the print() method, old reliable as it were. Kinda worked but didn't give me enough fine-grain control.
Bit the bullet and installed Wing IDE for python. And bam, it hit me. How did I ever live without step-through, and breakpoints before now?
2. Remember that non-sieve prime generator I wrote a while back? (well maybe some of you do). The one that generated quasi lucas carmichael (QLC) numbers? Well thats what I managed to debug. I figured out why it wasn't working. Last time I released it, I included two core methods, genprimes() and nextPrime(). The first generates a list of primes accurately, up to some n, and only needs a small handful of QLC numbers filtered out after the fact (because the set of primes generated and the set of QLC numbers overlap. Well I think they call it an embedding, as in QLC is included in the series generated by genprimes, but not the converse, but I digress).
nextPrime() was supposed to take any arbitrary n above zero, and accurately return the nearest prime number above the argument. But for some reason when it started, it would return 2,3,5,6...but genprimes() would work fine for some reason.
So genprimes loops over an index, i, and tests it for primality. It begins by entering the loop, and doing "result = gffi(i)".
This calls into something a function that runs four tests on the argument passed to it. I won't go into detail here about what those are because I don't even remember how I came up with them (I'll make a separate post when the code is fully fixed).
If the number fails any of these tests then gffi would just return the value of i that was passed to it, unaltered. Otherwise, if it did pass all of them, it would return i+1.
And once back in genPrimes() we would check if the variable 'result' was greater than the loop index. And if it was, then it was either prime (comparatively plentiful) or a QLC number (comparatively rare)--these two types and no others.
nextPrime() was only taking n, and didn't have this index to compare to, so the prior steps in genprimes were acting as a filter that nextPrime() didn't have, while internally gffi() was returning not only primes, and QLCs, but also plenty of composite numbers.
Now *why* that last step in genPrimes() was filtering out all the composites, idk.
But now that I understand whats going on I can fix it and hypothetically it should be possible to enter a positive n of any size, and without additional primality checks (such as is done with sieves, where you have to check off multiples of n), get the nearest prime numbers. Of course I'm not familiar enough with prime number generation to know if thats an achievement or worthwhile mentioning, so if anyone *is* familiar, and how something like that holds up compared to other linear generators (O(n)?), I'd be interested to hear about it.
I also am working on filtering out the intersection of the sets (QLC numbers), which I'm pretty sure I figured out how to incorporate into the prime generator itself.
I also think it may be possible to generator primes even faster, using the carmichael numbers or related set--or even derive a function that maps one set of upper-and-lower bounds around a semiprime, and map those same bounds to carmichael numbers that act as the upper and lower bound numbers on the factors of a semiprime.
Meanwhile I'm also looking into testing the prime generator on a larger set of numbers (to make sure it doesn't fail at large values of n) and so I'm looking for more computing power if anyone has it on hand, or is willing to test it at sufficiently large bit lengths (512, 1024, etc).
Lastly, the earlier work I posted (linked below), I realized could be applied with ECM to greatly reduce the smallest factor of a large number.
If ECM, being one of the best methods available, only handles 50-60 digit numbers, & your factors are 70+ digits, then being able to transform your semiprime product into another product tree thats non-semiprime, with factors that ARE in range of ECM, and which *does* contain either of the original factors, means products that *were not* formally factorable by ECM, *could* be now.
That wouldn't have been possible though withput enormous help from many others such as hitko who took the time to explain the solution was a form of modular exponentiation, Fast-Nop who contributed on other threads, Voxera who did as well, and support from Scor in particular, and many others.
Thank you all. And more to come.
Links mentioned (because DR wouldn't accept them as they were):
https://pastebin.com/MWechZj912 -
I don't know if I'm being pranked or not, but I work with my boss and he has the strangest way of doing things.
- Only use PHP
- Keep error_reporting off (for development), Site cannot function if they are on.
- 20,000 lines of functions in a single file, 50% of which was unused, mostly repeated code that could have been reduced massively.
- Zero Code Comments
- Inconsistent variable names, function names, file names -- I was literally project searching for months to find things.
- There is nothing close to a normalized SQL Database, column ID names can't even stay consistent.
- Every query is done with a mysqli wrapper to use legacy mysql functions.
- Most used function is to escape stirngs
- Type-hinting is too strict for the code.
- Most files packed with Inline CSS, JavaScript and PHP - we don't want to use an external file otherwise we'd have to open two of them.
- Do not use a package manger composer because he doesn't have it installed.. Though I told him it's easy on any platform and I'll explain it.
- He downloads a few composer packages he likes and drag/drop them into random folder.
- Uses $_GET to set values and pass them around like a message contianer.
- One file is 6000 lines which is a giant if statement with somewhere close to 7 levels deep of recursion.
- Never removes his old code that bloats things.
- Has functions from a decade ago he would like to save to use some day. Just regular, plain old, PHP functions.
- Always wants to build things from scratch, and re-using a lot of his code that is honestly a weird way of doing almost everything.
- Using CodeIntel, Mess Detectors, Error Detectors is not good or useful.
- Would not deploy to production through any tool I setup, though I was told to. Instead he wrote bash scripts that still make me nervous.
- Often tells me to make something modern/great (reinventing a wheel) and then ends up saying, "I think I'd do it this way... Referes to his code 5 years ago".
- Using isset() breaks things.
- Tens of thousands of undefined variables exist because arrays are creates like $this[][][] = 5;
- Understanding the naming of functions required me to write several documents.
- I had to use #region tags to find places in the code quicker since a router was about 2000 lines of if else statements.
- I used Todo Bookmark extensions in VSCode to mark and flag everything that's a bug.
- Gets upset if I add anything to .gitignore; I tried to tell him it ignores files we don't want, he is though it deleted them for a while.
- He would rather explain every line of code in a mammoth project that follows no human known patterns, includes files that overwrite global scope variables and wants has me do the documentation.
- Open to ideas but when I bring them up such as - This is what most standards suggest, here's a literal example of exactly what you want but easier - He will passively decide against it and end up working on tedious things not very necessary for project release dates.
- On another project I try to write code but he wants to go over every single nook and cranny and stay on the phone the entire day as I watch his screen and Im trying to code.
I would like us all to do well but I do not consider him a programmer but a script-whippersnapper. I find myself trying to to debate the most basic of things (you shouldnt 777 every file), and I need all kinds of evidence before he will do something about it. We need "security" and all kinds of buzz words but I'm scared to death of this code. After several months its a nice place to work but I am convinced I'm being pranked or my boss has very little idea what he's doing. I've worked in a lot of disasters but nothing like this.
We are building an API, I could use something open source to help with anything from validations, routing, ACL but he ends up reinventing the wheel. I have never worked so slow, hindered and baffled at how I am supposed to build anything - nothing is stable, tested, and rarely logical. I suggested many things but he would rather have small talk and reason his way into using things he made.
I could fhave this project 50% done i a Node API i two weeks, pretty fast in a PHP or Python one, but we for reasons I have no idea would rather go slow and literally "build a framework". Two knuckleheads are going to build a PHP REST framework and compete with tested, tried and true open source tools by tens of millions?
I just wanted to rant because this drives me crazy. I have so much stress my neck and shoulder seems like a nerve is pinched. I don't understand what any of this means. I've never met someone who was wrong about so many things but believed they were right. I just don't know what to say so often on call I just say, 'uhh..'. It's like nothing anyone or any authority says matters, I don't know why he asks anything he's going to do things one way, a hard way, only that he can decipher. He's an owner, he's not worried about job security.13 -
Got rebuked by the Java teacher today at the University for using proper long names for variables in the code. She though I was just wasting time being lazy in the lab. "If something can be achieved by a single character, why type that long variable again and again?". *Everyone in class laughs*
Then, there was an error in my code [turned out to be long long int in Java is weird], and I had no clue what was going wrong [I'm a week old in Java]. So, I had initially called her to help. She made me change all private methods and attributes to public. When asked "why?", got trolled again.
Now, I know it's okay, and not that I really care about what my classmates think of me, but getting this kind of treatment really sucks. And if this is how future software developers are crafted today, maintainability is surely going to be an issue tomorrow.
Maybe staying in this stupid country was my worst career choice. I should have tried harder and gone abroad.11 -
So in Germany we have something like 'cooperative study'. You are employed in a company and study 'normal' at a university. This is in 3 month phases, i.e. 3 months working, 3 months studying.
At the moment I'm working and there is a colleauge, that seems to have no high confidence in my programming skills.
Today I saw parts of his NodeJS code and I thought I'm going crazy.
No comments, no real usage of callbacks or at least promises and I dont want to talk about naming of the variables.
I caught myself arguing with this guy too often and always thought I'm the stupid one, that doesn't understand him.
But I'm starting to think, He is the one that is hard to understand.
How ever, I stay confident and also keep a nice tone (also help as much as I can) and sometimes we also have the same thoughts in some topicd. It's not that bad, but sometimes I feel underestimated.
But hey, so it's a bigger surprise if I'm presenting my results and show them what I'm able to do 👍🏻2 -
First time I was screaming out of anger while looking at code.
I'm doing a group project in my university.
We are developing a indoor navigation Android app.
And a team mate of mine just merged this…
/*Method for help-feature.
Sets all the TouchEvents that are at least 400 ms long. This is made for all the relevant buttons or editTexts, which are seen on the mapView.
The case for mapView is needed because otherwise the other buttons, etc. wont work properly.*/
public void setButtonsForHelpDialog(){
View v = mapView;
switch (v.getId()) {
case R.id.mapview:
mapView.setOnTouchListener(…);
case R.id.buttonUp:
buttonOn.setOnTouchListener(…);
case R.id.buttonDown:
buttonDown.setOnTouchListener(…);
…
case R.id.description:
description.setOnTouchListener(…);
}}
The code is really aligned like this - no breaks. And it's even worse. There are if statements like if("constantly false var" == true). Which is highlighted by Android Studio.
This is done in a own class. The views are set via public member variables of this new class. The constant vars were added in the actual class holding the buttons and also stuff like this useless method
public void getDoStuff() {
doStuff()
}
And I could continue like this.
I never saw code this bad…
I can't even find words for it :/4 -
Need to rant. I am doing programming 2 at university with java and the assessment is to make a card game. The subject is shit and is basically going over loops, variables, conditionals ect which we learned in introduction to programming and programming 1.
This leaves little time for oop principles, design patterns inherentance and all other useful stuff.
I am dedicated to making a career in programming and want to do my assessment the correct oop way. Although the lecturer doesn't care and is instructing the class to do it procedurally and shit.
I could do the program really quickly the shit procedural way and still get full marks but I feel dirty as hell coding like a scrub. So I'm 60 hours in on this assessment and there are so many classes and even more because of unit testing (we don't have to unit test) and I am spending way too much time.
My code is beautiful, my classes are tiny and maintainable, easy to modify and I'm learning so much about how to code oop the correct way with the help of a mentor and someone I look up to. But god does it take forever to code this way. And soo many iterations and redesigns because I'm still learning.
It's almost done but now I have another programming assessment for another class I'll have to do the dirty way because of time restraints and other assessments.
Sorry for wall of text but this is stressing me out 😛4 -
I help first year Computer Science students at my uni. In the first few weeks I reviewed someone's code to find he had 253 variables called var1, var2, var3 and so forth. The even worse part: he made the same if-else structures for every variable without using a loop.
if (var1 == something) {
//huge chunk of code here
}
else if (var1 == something) {
}
else {
}
... var2... var3
The funniest thing about this is that we had a discussion about this, because he claimed this was the right way to solve his problem.5 -
!rant
Warning : This rant is long and is a rant asking for help and suggestions. If you will read and dont leave any comments, please go search other rants. Thanks.
-----------------------------------
Hi, fellow ranters. In our community, we have a tech class where teens (teens here mean 14yo -15yo) come to learn computer stuffs. Teens here are selected by a test and an interview. There are some teens who are f***ing awesome. One of them are proficient in scratch. (yeah, the orange cat) Another is awesome at PhotoShop, and the other loves windows xp. The teacher uses Microsoft Visual C++ IDE made in the 1990s. The kid sitting to my left made flappy bird with gamemaker. About 10 to 11 teens doesnt know what ctrl+alt+del does in windows and never did programming before... 3 among them always brings coke and oreos and eats super loudly. CRACK! And I bet no one knows about git.
Ok. Enough for the awesome teens. Now what we learn.
We learn C! Yes, C. We learned for, if else, switch and all those stuffs, then learned variables, which made other students who never did programming before be (―,.―).
Next class we will learn about functions in 3 hours. Then array and pointer in 3 hours. Thats it for c programming. Then we do some unnecessary stuffs and time for the finals.
We need to make a project with up to 4 teens as one team. Now I am asking you awesome ranters to suggest some projects for about 4 pros and 16 noobs can do. 10 hours are given in class and we can do in other times by ourselves in home. What should we do? I bet many of them will say to make ascii art in c which is dull and I have no thoughts of doing that.
Any thoughts will be appreciated.
Thank you for reading.
To see my skills, go to my profile page.
| Comments below
v17 -
I don’t know if I would call it a quirk of the language or serious abuse of it :P
But I managed to get a null ref exception when comparing a local int variable to an int parameter to the same function in C#.
Since a local or parameter of type in cannot be null and I compared the variables them self and dud not try to access any property on them (and no extension method or implicit case or similar) my first thought, along with all colleagues that chipped in to help, was that this should not be possible.
Turns out the method was called through reflection and in that part it injected null as the base object to call the method on.
Since local variables actually are referenced through the parent object this was what was causing the null ref.
That took some time to figure out.4 -
Using the console to print out variables is a perfectly valid method of debugging for new developers: often, it can help you resolve the problem faster than using a debugger.2
-
honestly some online courses are bullshit. i joined one for some sample code, and no comments, no explanations, the variable names WEREN'T even descriptive.
this is from a website with a published book… how about you take some fucking responsibility for your code?
the language was c++ and they are still using printf! shake my fucking head. you have global variables that are one fucking letter! please, stop, get help.
…AND IT WASN'T EVEN ON GITHUB -
> do you feel sorry for freelancing contractors
> whose previous client abandoned them
> they ask you to help them fix some trivial bugs in the shitty code
> you believe you can change the world by going overboard by also improving the code quality, along with fixing the bugs
> initialize an empty file where you'll translate the shitty code into a more organized one
> start creating variables and generic functions which can be used in a modular and organized fashion
> meticulously document the first function you write
> realize this is not worth your time
> insert some glue code into the original code which fixes the trivial bugs
> glue code has hard coded values so it adds to the shittiness of the code
> submit the work
> get $$$ -
When I was in my first year in university, at the Introduction to Programming class, we had to do a Java project and my partner was someone who was always bragging about how much he knew about programming. Me being someone who was learning programming by the moment I started the course I thought he was going to help and teach me cool stuff.
The first phase was due to deliver in one week and literally my code was all original and my partners code was all copies of others work. Out project could'vw been graded 0 because of his code (because they used a programm that compared variables of each project to see if.they fins copies). He practically didn't gave the effort to try to code, just copies. I spent the whole week correcting his work so that I wasnt penalized in the end...1 -
Back from the dead with more vaguely-obscure technical bullshit
Working on a chatbot for my BS-CS. Almost done with college, so the assignment is to make a bot that recommends you a CS career. Cool.
I get through making a joint personality and skill-interest quiz that gives you number grades on different spectra. So far, so good. But this project has to be done entirely in pandorabots' online editor. So no scripting. Zero scripting. 100% markup language. That means to even do math, you need to copy a standard library off GitHub.
I mean, that's fine and all, but the syntax is just atrocious, because everything in AIML is input->response. If you ask the bot "what is 5+5?" you must have it go:
- recognize pattern WHAT IS * + *
-> redirect -> XADD * XS *
-> do math -> recurse result
-> 10
uncomfy. Plus, variables can only be accessed through <get> and <set> tags. But mangeable.
So here's where the story becomes a rant.
In the standard docs, there's all these math functions, and they work. There's also logic.
And then there's this fucker
XIF [ * ] XS [ * ]
Which has no documentation and just doesn't work. No idea what the brackets mean. Tried putting in TRUE, tried putting in true math statements (5 XEQ 5), tried putting in recursion tags to trick it, tried everything. It just ignores it.
There is not a single comment, stackOverflow post, or youtube video that even acknowledges the existence of this thing.
So unless I want to convert the entire logic of my program into nested SWITCH statements with the <condition> tag, I'm just fucked.
The icing on the cake is, I go to tech support on Pandorabots to ask for help with this. What do they have except a chatbot to cheerfully tell me that no humans are around to help me right now?
gonna have to build an entire fuckin turing machine in markup tags to calculate whether x = 3
(:1 -
Got one right now, no idea if it’s the “most” unrealistic, because I’ve been doing this for a while now.
Until recently, I was rewriting a very old, very brittle legacy codebase - we’re talking garbage code from two generations of complete dumbfucks, and hands down the most awful codebase I’ve ever seen. The code itself is quite difficult to describe without seeing it for yourself, but it was written over a period of about a decade by a certifiably insane person, and then maintained and arguably made much worse by a try-hard moron whose only success was making things exponentially harder for his successor to comprehend and maintain. No documentation whatsoever either. One small example of just how fucking stupid these guys were - every function is wrapped in a try catch with an empty catch, variables are declared and redeclared ten times, but never used. Hard coded credentials, hard coded widths and sizes, weird shit like the entire application 500ing if you move a button to another part of the page, or change its width by a pixel, unsanitized inputs, you name it, if it’s a textbook fuck up, it’s in there, and then some.
Because the code is so damn old as well (MySQL 8.0, C#4, and ASP.NET 3), and utterly eschews the vaguest tenets of structured, organized programming - I decided after a month of a disproportionate effort:success ratio, to just extract the SQL queries, sanitize them, and create a new back end and front end that would jointly get things where they need to be, and most importantly, make the application secure, stable, and maintainable. I’m the only developer, but one of the senior employees wrote most of the SQL queries, so I asked for his help in extracting them, to save time. He basically refused, and then told me to make my peace with God if I missed that deadline. Very helpful.
I was making really good time on it too, nearly complete after 60 days of working on it, along with supporting and maintaining the dumpster fire that is the legacy application. Suddenly my phone rings, and I’m told that management wants me to implement a payment processing feature on the site, and because I’ve been so effective at fixing problems thus far, they want to see it inside of a week. I am surprised, because I’ve been regularly communicating my progress and immediate focus to management, so I explain that I might be able to ship the feature by end of Q1, because rather than shoehorn the processor onto the decrepit piece of shit legacy app, it would be far better to just include it in the replacement. I add that PCI compliance is another matter that we must account for, and so there’s not a great chance of shipping this in a week. They tell me that I have a month to do it…and then the Marketing person asks to see my progress and ends up bitching about everything, despite the front end being a pixel perfect reproduction. Despite my making everything mobile responsive, iframe free, secure and encrypted, fast, and void of unpredictable behaviors. I tell her that this is what I was asked to do, and that there should have been no surprises at all, especially since I’ve been sending out weekly updates via email. I guess it needed more suck? But either way, fuck me and my two months of hard work. I mean really, no ego, I made a true enterprise grade app for them.
Short version, I stopped working on the rebuild, and I’m nearly done writing the payment processor as a microservice that I’ll just embed as an iframe, since the legacy build is full of those anyway, and I’m being asked to make bricks without straw. I’m probably glossing over a lot of finer points here too, just because it’s been such an epic of disappointment. The deadline is coming up, and I’m definitely going to make it, now that I have accordingly reduced the scope of work, but this whole thing has just totally pissed me off, and left a bad taste about the organization.10 -
Any JavaScript developers out there willing to help me out with something?
I have an interview question that I like to ask candidates that no one ever seems to get right. But, to me, it seems pretty basic, so I expect MOST JavaScript developers at almost any level of expertise to get it, and I like it generally because it demonstrates some core knowledge of JavaScript concepts and syntax.
But I want to verify that my feelings about it are reasonable, because give how few ever seem to get it right (and I'm talking across literally hundreds of interviews, MAYBE 2 people have ever gotten it right), I'm starting to wonder if I'm right or not.
Look at this code, and then answer the question after. Please do so off the top of your head and without testing anything since that's normally the experience a candidate would have. I'll give the answer after some time for anyone who gets it wrong but is curious.
But this isn't about YOU getting it right or not, and it's not about whether it's the best way to do something in JavaScript or anything like that, it's just about whether it's a reasonable question and whether my expectation that MOST JavaScript developers should get it right is fair.
const O = {
sayHello : function() { alert("Hello"); }
};
const S = "sayHello";
Question: using ONLY the variables O and S (and you MUST use both), write code that executes the sayHello function.
Thanks!34 -
Global variables destroyed my day
Of course you can call me a bad programmer and all. When you have the idea, sometimes globals make it seem easy, accessible and "saving resources". They are devils.
The app was connected to a suite of applications. So I ended up silently destroying it neighbors. I committed and pushed the shit. Just when the testers made their weed high smoke tests, a server stopped working.
I got an email that boss wanted the latest version, I reverted the wrong branch, which had code unrelated to its name, pushed shit again and voila.
I went to the bathroom and laughed. I had to take a smoke. I'm still laughing while typing this. The damage is too much and I can't help it. I'll go home, leave this pc on and work remotely through the night. It might be the hysterics speaking now, but I messed up, and I need magic by friday morning.6 -
today when I was asked to fix some code I couldn't help but count if those variables where used more times :D2
-
please for the love of all that is right in the world...name variables something useful. ll_j does not help me.4
-
The worst part of being a dev? Working in teams.
And I don't mean that in the "I'm the best ninja code wizard in the whole world and you're all holding me back" kinda way. I'm thinking more in the lines of someone who has to deal with that kind of attitude on a daily basis. As someone who recently was put in a leading position in a dev team, this is by far one of the worst experiences that came with it.
Some examples?
- One dev completely changed the naming scheme for variables in a class he worked on for one. single. bug fix. His reason? He just didn't like it!
- Another one noticed that data he was supplied with was not in the specified format. Instead of flagging this with the project leads, he just rewrote his parser to fit the data. A couple of weeks later the supplier noticed the error, fixed the format and suddenly everyone wondered why the software failed processing the data.
- Or that one senior dev, that just refuses to accept changes because "it was always done like this and it worked" No, it didn't. That's why it was changed!
Once a dev team reaches a certain size, people need to realize that stuff like coding rules and process guidelines are not there to annoy them but to help the whole team work as efficient as possible. I don't care how good a programmer you are, if you can't check your ego you don't belong in any kind of team-oriented development project! -
I was asked to make proof of concept small frontend app with some simplified requirements, they asked me because it should be written in the stack I done most of my career work with. I do it in 3 days instead of 5, using those 2 days to optimise the app and explore different approaches. I noted down my findings, what to avoid and reasons and also what is good to use and reasons and shared with everyone.
We waited for the project to start, I started working on another project in the meantime and there was a big rush to make project go live etc., so I was consumed 100% on that new project.
So they put in charge backend php developer to do frontend js work. I said ok, do you need help in starting out? Nah, my proof of concept repo is enough.
4 days before that small project goes live they asked me to do code review. All things I noted down to avoid are in the codebase, few bad practices but everything is over-engineered (in a very bad way), some parts should be more flexible as current setup is very rigid, having almost all kinds of CSS, I saw SASS, CSS variables, 2 different CSS-in-JS tools with some additional libraries that is used to toggle classes.
I don't know how to approach this as I am not asshole as a person and I don't want to say to my colleague that his codebase is completely trash, but it is.
The worst parts: They called me to help finish the app and budget is almost spent!
I would rewrite the whole app as the state of the current app is unusable and everything is glued with bad Chinese ducktape that barely holds.
Additional points because it won't bundle as everything is f**ked.
I am seriously thinking of duplicating master branch and refactor the whole fricking app but won't do that as I am burning midnight oil on other two projects. Don't worry overtimes are paid.
I hate those shitty situations, this project was supposed to be tiny, sweet and example of decent project in this company but it is instead big fat franken-app that will be example how smart it is to avoid putting backend dev to do frontend work (I also agree for vice versa)! -
Hey devs!!
I just can't stop myself from sharing this.
Its been two years now ... my junior is working. and now she is handling standalone WP projects.. but somehow her task got stucked so I was asked to help her.
So I just said check the count of variables and she messaged me with code .. Ma'am this isn't working .. Haha.. I mean come on she better can google out atleast for syntax :(
<?php if(count($content2 == 3) && count($interestt2 == 3))
{
print_r($content2);
print_r($interestt2);
}else{
echo "not checked";
}
?>2 -
Just found a crystal subreport with 33 shared variables. For those who don't know what that is (lucky), they're super global variables that exist everywhere.
Please send help. I'm supposed to refactor this...19 -
For the new/aspiring developers:
1. If you are still looking to learn more, but you don't know where to go, start brainstorming. Make a list of projects you could make and sort them by difficulty. Put the ones you could do now at the top of the list, and the ones you aren't sure how to do yet, at the bottom of the list. As you go through them, if you want to do something but aren't sure how, just hop onto an irc chat and everyone will be glad to help. As you go through the projects, your logic and program design skills should improve, as well as your knowledge of programming.
2. Put comments in your code. Seriously. If you are working on a project and suddenly stop working on it for a week or more, you will go back to look at that code and be extremely confused. If you are making something open source, its even more important. If people can understand the code, they are more likely to contribute to it.
3. Try not to focus on code for too long. The longer you work, the more tired your brain gets. Eventually you get tired and make really stupid decisions in your code.
4. Don't code while tired (look at #3)
5. If you are writing code as an assignment, make sure to rename all variables to proper names before submitting it. The instructor will likely not be pleased to see variable names with the f-bomb in them. -
I hate naming variables.
DEVICE_INPUT
Is it input of external device or input of my device? Should I read from it or write to it? Help... -
I'm currently doing project in Java using JavaFX for GUI and after like 6 months I found out we can bind textfields to variables(yea,dumb me) and I got more than 20 forms so of course, binding is useful than that getText method. So I think there are many things like this which will help me to optimize my code but I dont know, so can anyone tell me more stuff like this?
I'm using MongoDb so I'm currently finding easiest way to make Bson document from textfield values. Any suggestions?
(Sorry, for my bad English)1 -
So I've been tinkering with this idea since this morning:
There should be a dictionary for developers, to help give meaningful names for variables, functions.
With some pipe like interface to narrow down possible names,2 -
I had to optimize a SQL-Query and i was able to cut the amount of time in half.
The business part of the company said it is still too slow, so i researched deeper and looked into the called functions which are used in every part where to claculate value X.
Found 20 similiar selects to get values which are summed up afterwards into three different variables. Thought this can be done in three selects with "sum", but then i found the dumbest thing is this function:
V1, V2 have the values from the selects
V3 is ja given value
X is the return value
the function returns another value
help = functionCall(parameter) - V1 -V2
X= help + V1 + V2 +V3
WTF im mean it does the selects for nothing...
X = functionCall(parameter) + V3 -
Whenever I reach the point where static analysis can't help me any further I always feel a sort of thrill mixed with terror. This is the real deal. Until now the problems were easy to find, the questions had well defined answers to choose from, the rules were universal. In the part of the logic that cannot be checked, the invariants upheld manually, where the best the type system can enforce is for the programmer to clearly state what they're doing, lies the real beast. In proofs commented on functions or invariants as logical expressions over plain English variables written in the doc comments of a struct.
In the blurry and pompous future I imagine for software development, that's where the programmer's time will be spent. Once we all agree on what a string is, what it means to depend on someone else's code, and what parts a UI should be made of, all a developer should have to do is make decisions and derive proofs an automated deduction engine can't do on its own. -
February will be the first full year at this company as full time employee.
I've updated so many legacy projects, optimized a lot of workflows as well as built new tools to improve efficiency and remove unnecessarily duplicate projects (sometimes literally only 3 variables were different between multiple projects)
My one co-worker taught himself enough code to do the job but doesn't think like a programmer though he is asking me for help and advice to improve what he does since ive proven i know a little. my other direct co-worker I'm practically teaching a Programming 100 course to them
My direct manager at one point said he was so happy he took a chance on me even though I didn't interview well
I like my job, I find it so much better than my last job which was horribly toxic, and more fun than my first 'real' job as a night shift help desk for basically a warehouse environment.
But I feel under paid sometimes for how much i do and all ive improved in my first year, I have my first yearly review coming up. I'm hoping to get a decent raise for all ive done and I want to somehow go over everything with the HR person to justify it. But I have no idea how to talk about my dev work to them in a way a non technical person could understand. I'm also not sure how the review process will work. Like will my manager be there. Or is it just me and HR, is there a paper I'll be sent to fill before hand,1 -
So, guys, I'm wondering if someone can help me wkth this thing. I'm sorta newish to programming and was searching up different kinds of typing. Basically what I found was:
Strong: Variables are explicitly declared, can only be changed to values of that type
Weak: Variables are implicitly typed, can accept any value of any type.
However, upon searching static and dynamic, it says the same things for dynamic and weak, and for static and strong. Can anyone help me by telling me the dofference between these (if you even understood this post 😅)
Thanks5 -
Maybe I'm stupid, but I'm annoyed that there are no good ways to debug Blazor WASM.
It doesn't help to tell me that I got a null value, unless the error tells me which of my 50 variables is causing it5 -
A little help on Django
So I just started learning Python and Django Framework and I have one question...
Are the variables shared between visitors?
I mean, let's say I have a variable named "school"
school = "school1"
A visitor from X location enters and changes this variable to "school2". A new visitor enters the page from Y location and reads the variable 'school'. What is going to show on visitor Y? "school1" or "school2".2 -
Doing someone else's Code Review in my project: "You must retain the holiness and piety of the code you write by following PascalCase naming for files and kebab-case naming for CSS variables. Avoid using duplicate strings by declaring enums in a constants.ts file and using that all throughout the app"
During my own Code Review in someone else's project: "WHAT THE FUCK DO YOU MEAN I CANNOT PASS FUNCTION REFERENCES AS PROPS TO A REACT COMPONENT AND ALWAYS NEED TO INVOKE IT INSIDE AN INLINE FUNCTION FOR THE PROP."
"WHAT KINDA FKIN DRUGS ARE YOU ON TO USE snake_case IN TYPESCRIPT DID YOUR MOM DROP YOU ON YOUR HEAD WHEN YOU WERE BORN YOU SACRILEGIOUS PIECE OF SHIT"
"WHAT DO YOU MEAN I SHOULD USE BOTH SINGLE AND DOUBLE QUOTES FOR IMPORTS AS PER LOCAL OR GLOBAL; I'LL SHOVE THE SINGLE QUOTE UP YOU WHERE THE SUN DOESN'T SHINE YOU FKIN DEGENERATE MORON"
As much as I do believe in self righteousness of my own coding conventions over others (I might be slightly better than others but I really can't claim good authority because I've had my lapses in conventions too; and being one of the newer members of the team certainly doesn't help, despite my boss supporting my initiative), I guess it is high time we bring in some already established code conventions in the team that is finally big enough to warrant them. Maybe AirBnB. -
When you need proxy,
Connecting to Amazon S3
---
config.setProxyHost(URI);
config.setProxyPort(8080);
AmazonS3 s3 = new AmazonS3Client(Credentials, config);
---
So easy.
We need to move out amazon and start using google cloud storage.
---
Can't seem to find API Documentation for it.
Saw that they are using HttpURLConnection.
Fvck! They are not even reading proxy information set via System Environment Variables!
Help! Stuck on it for 3 days already.
.°(ಗдಗ。)°.2 -
In reply to this:
https://devrant.com/rants/260590/...
As a senior dev for over 13 years, I will break you point by point in the most realistic way, so you don't get in troubles for following internet boring paternal advices.
1) False. Being go-ahead, pro active and prone to learn is a good thing in most places.
This doesn't mean being an entitled asshole, but standing for yourself (don't get put down and used to do shit for others, or it will become the routine) and show good learning and exploration skills will definitely put you under a good light.
2)False. 2 things to check:
a) if the guy over you is an entitled asshole who thinkg you're going to steal his job and will try to sabotage you or not answer acting annoyed, or if it's a cool guy.
Choose wisely your questions and put them all togheter. Don't be that guy that fires questions in crumbles, one every 2 minutes.
Put them togheter and try to work out the obvious and what can be done through google or chatgpt by yourself. Then collect the hard ones for the experienced guy and ask them all at once. He's been put over you to help you.
3) Idiotic. NO.
Working code = good code. It's always been like this.
If you follow this idiotic advice you will annoy everyone.
The thing about renaming variables and crap it's called a standard. Most company will have a document with one if there is a need to follow it.
What remains are common programming conventions that everyone mostly follows.
Else you'll end up getting crazy at all the rules and small conventions and will start to do messy hot spaghetti code filled with syntactic sugar that no one likes, included yourself.
4)LMAO.
This mostly never happens (seniors send to juniors) in real life.
But it happens on the other side (junior code gets reviewed).
He must either be a crap programmer or stopped learning years ago(?)
5) This is absolutely true.
Programming is not a forgiving job if you're not honest.
Covering up mess in programming is mostly impossible, expecially when git and all that stuff with your name on it came out.
Be honest, admit your faults, ask if not sure.
Code is code, if it's wrong it won't work magically and sooner or later it will fire back.
6)Somewhat true, but it all depends on the deadline you're given and the complexity of the logic to be implemented.
If very complex you have to divide an conquer (usually)
7)LMAO, this one might be true for multi billionaire companies with thousand of employees.
Normal companies rarely do that because it's a waste of time. They pass knowledge by word or with concise documentation that later gets explained by seniors or TL's to the devs.
Try following this and as a junior:
1) you will have written shit docs and wasted time
2) you will come up to the devs at the deadline with half of the code done and them saying wtf who told you to do that
8) See? What an oxymoron ahahah
Look at point 3 of this guy than re-read this.
This alone should prove you that I'm right for everything else.
9) Half true.
Watch your ass. You need to understand what you're going to put yourself into.
If it's some unknown deep sea shit, with no documentations whatsoever you will end up with a sore ass and pulling your hair finding crumbles of code that make that unknown thing work.
Believe me and not him.
I have been there. To say one, I've been doing some high level project for using powerful RFID reading antennas for doing large warehouse inventory with high speed (instead of counting manually or scanning pieces, the put rfid tags inside the boxes and pass a scanner between shelves, reading all the inventory).
I had to deal with all the RFID protocol, the math behind radio waves (yes, knowing it will let you configure them more efficently and avoid conflicts), know a whole new SDK from them I've never used again (useless knowledge = time wasted and no resume worthy material for your next job) and so on.
It was a grueling, hair pulling, horrible experience that brought me nothing in return execpt the skill of accepting and embracing the pain of such experiences.
And I can go on with other stories. Horror Stories.
If it's something that is doable but it's complex, hard or just interesting, go for it. Expecially if the tech involved is something marketable.
10) Yes, and you can't stop learning, expecially now that AI will start to cover more and more of our work.4 -
If you have a blog, How do you decide what to write and publish on it? And, How do I motivate myself to write posts?
Context: I created my blog/website on 29 September 2017. I had a few ideas on writing blog posts(Condition variables in Go, Serverless related stuff and a whole bunch of posts related to wireguard) but every time I have tried write a post, I learn there is someone else who has already written a post on it and probably better than what I could have done, So what is really the point of writing it? And, I feel very insecure about writing posts, I feel like, If I do write a post, every one will know, I don't know anything about **anything**. :( I know about imposter syndrome, But I don't think I have that. I work with a lot of realllly smart people and I don't know as much as them. So, I am actually an imposter.
edit: I am usually active on Telegram, IRC and I try to help out people. It's easier for me to help people in communities like that but doing the same thing with a blog makes me very uncomfortable.2 -
I'm kind of in a bit of a conundrum.
Abou 6 months ago, a friend and me were speaking about how bored I was and how I don't have any new ideas for projects, and he gave me one, and it is the basis of the project I am currently building, yet I changed one of the variables he had gave me as to appeal to a wider audience, yet now as I resumed work on it, I think it is a good idea and would make me some money( I'm not writing about this grounbreaking idea that would make me millions, hell, I'm not sure it will be successfull, but it might make me some money to help me when the time comes to go to university) but I am a firm believer in open-source and I don't know if I should make it open source and rely on the donations and let them modify the code, or just charge for it?
If it were another case, I wouldn't think twice before making it open source yet I probably won't be able to afford uni and this would be good for me and help me along with the freelancing jobs I am starting to work on.
What would you guys recommend I do?2 -
I got two files one called lte and the other called fcc2. The attached image is the fcc2 file. The first file is just a list of census blocks. 15 digit number per line on the lte file.I need help changing just the lines in the fcc2 file that have the same 15 digit number at the start of the line. Just need to change 2 variables in the line. FCC2 is a TSV file. any help would be appreciated.7
-
Okay, I hope a few people can help me with this; what are the benefits/reasons to use MS technologies? I'm talking about .NET, ASP, Windows Server, Powershell...
I've never understood it. I love Nodejs because you don't have any packages unless you ask for them. Alpine Linux is amazing! It runs on 8MB of RAM from fresh and doesn't need much more space to install.
You want .NET core? 140MB download. You're configuring database connection strings? Feel free to type in whatever you like, it'll parse and replace with some magic variables that have come from some other random file.
I was using Powershell recently, needed to set an env variable. Bash is happy with "export name=value". You want to do that in Powershell? I just googled it and found an entire 40-minute read discussing how to set env vars. Why?! It should be one command, and I don't know who thought that "Get-ChildItem" was _obviously_ referring to env variables.
It seems to me that everywhere MS has got their hands on development-wise, it inherits the typical sales bullshit. No no, you can't call them "websockets", they have to be branded "SignalR" and add tons of overhead. You can't say "disable notifications" it has to be "focus assist". I'm really surprised something as simple as a keyboard hasn't become a "varied user input device" or something of the like.
Am I alone in thinking this?4 -
Hey everyone. I wouldn't do this normally but this is actually my first project that has gone live ehich was also the base of my study for becoming front-end dev. Its a front end lib that mixes bootstrap with styled components. But also explains a way to create react components with variables and theming helpers to quickly create components and themes that are sharable.
Yes, i learned html, css and javascript and jumped onto react about 6 month later. Its been 3 years now but the project ready even though it ha some bugs.
Any help testing and criticising would be of great help. We are trying to be reactive for bug correction and improvements.
https://tinyurl.com/y9q3pp9w -
When the college, that started at the same time as you, needs your help declaring variables after half a year in the company...