Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
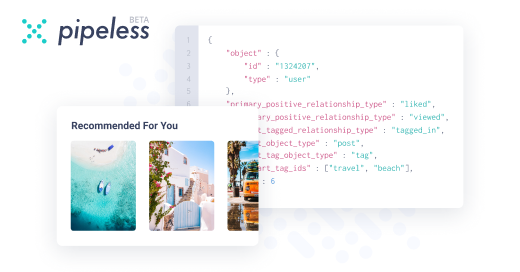
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "learning from mistakes"
-
0. Plan before you code. Document everything. You won't remember either your idea or those clever implementations next week (or next month, or next year...).
1. Don't hack your way through, unless that's what you intend to do. Name your variables, functions etc. neatly: autocomplete exists!
Protip: Sometimes you want to check a quick language feature or a piece of code from one of your modules. Resist the urge to quickly hack in the test into your actual project. Maintain a separate file where you can quickly type in and check what you're looking for without hacking on your project (For example, in Python, you can open a new terminal or IDLE window for those quick tests).
2. Keep a quiet environment where you can focus. Recommend listening to something while coding (my latest fad is on asoftmurmur.com). Don't let anything distract you and throw your contextual awareness out of whack.
3. Rubber ducks work. Really. Talking out a complex piece of logic, or that regex or SQL query aids your mind greatly in grasping the concept and clearing the idea. Bounce off code and ideas with a friend or colleague to catch errors and oversights faster. Read more here: https://en.wikipedia.org/wiki/...
4. Since everyone else is saying this (and because it merits saying), USE VERSION CONTROL. Singular most important thing to software development aside from planning and documenting.
5. Remember to flout all of the above once in a while and just make a mess of a project where you have fun throwing everything around all over the place. You'll make mistakes that you never thought were possible by someone of your caliber :) That's how you learn.
Have fun, keep learning!3 -
Things I wish I could tell my 18 year old self.
1) Accept you will make mistakes.
2) Truly learn the language you are using.
3) Write idiomatic code for the language you are using.
4) Be upfront about not knowing something.
5) Don't let not knowing something stop you from learning it.
6) None of us knew X until we learned it.
7) Understand your strengths and weaknesses as a developer, play to them.
8) Be willing to try new things.
9) X language isn't ALWAYS the best choice, X paradigm isn't ALWAYS the best choice. Choose wisely.
10) You won't know everything, but you might know more than others.
11) Your ideas and ego don't matter more than ensuring the product works.
12) "Perfection is the enemy of the good [enough]" - Voltaire
13) "Perfection is not achieved when there's nothing more to add, but when there's nothing more to remove." - Einstein.
14) Conflicts happen, deal with it.
15) Develop a toolset and really learn them.
16) Try new tools, they may prove better than what you were using.
17) Don't manage your own memory unless you absolutely have to, you are probably not smarter than the collective intelligence of the team that built the various garbage collection methods.
18) People can be dicks, especially online.
19) If you are new and people are being dicks to you, did you skip past the irc message about etiquette? If you did, you're the dick in this situation.
20) It can be tough, but it is fun, so have fun!6 -
TLDR; I just screwed a production server and rendered it useless!!!
Long story:
I went to install a product that we built at the customer's site, and was given a Linux running server, to deploy our app.
I work in windows, and barely know the basic Linux commands.
So I look at the files in the home directory, and see that the are a lot of files, so I ask the customer if it is ok that I move all the files to a separate directory.
He agrees, and me thinking that I am smart, proceed to enter the following commands in the terminal:
mkdir old
mv /* old
Of course I got an error that I don't have permission so my next command was:
sudo mv /* old
And that was the end of that computer.
The amazing part of the story is that as soon as it happened, I understood so much about Linux.
The file structure, sudo, the power of the terminal, aliases and so much more...15 -
Rather than singling out one person, I wanna present what I see as incompetent/stupid/ignorant:
- no will to learn
- failure to follow the very specific instructions & later asking for help when they FUBR sth & not even knowing what they did to fuck up in the first place
- asking how to solve stuff, then ignoring the suggestions & doing sth totally against recommendations
- failure to remember most basic stuff, especially if not writing it down to look at later when needed
- failure to check logs & 'google' stuff before asking why something isn't working the way they want it
- after two weeks, asking me how feature xy works, mind you they coded it, not me
- asking me why they did something in a specific way - WTF, am I a mind reader?! Who designed that crap?! Me or you?!!
- being passive/aggressive & snarky when told to do something or being asked why isn't it done already
- not testing their shit properly
- not making backups when upgrading (production) servers
- not checking the input value, no validation.. even after many many debacles on production with null ref exceptions
- failure to admit they fucked up
- not learning from (their) mistakes8 -
Okay, story time.
Back during 2016, I decided to do a little experiment to test the viability of multithreading in a JavaScript server stack, and I'm not talking about the Node.js way of queuing I/O on background threads, or about WebWorkers that box and convert your arguments to JSON and back during a simple call across two JS contexts.
I'm talking about JavaScript code running concurrently on all cores. I'm talking about replacing the god-awful single-threaded event loop of ECMAScript – the biggest bottleneck in software history – with an honest-to-god, lock-free thread-pool scheduler that executes JS code in parallel, on all cores.
I'm talking about concurrent access to shared mutable state – a big, rightfully-hated mess when done badly – in JavaScript.
This rant is about the many mistakes I made at the time, specifically the biggest – but not the first – of which: publishing some preliminary results very early on.
Every time I showed my work to a JavaScript developer, I'd get negative feedback. Like, unjustified hatred and immediate denial, or outright rejection of the entire concept. Some were even adamantly trying to discourage me from this project.
So I posted a sarcastic question to the Software Engineering Stack Exchange, which was originally worded differently to reflect my frustration, but was later edited by mods to be more serious.
You can see the responses for yourself here: https://goo.gl/poHKpK
Most of the serious answers were along the lines of "multithreading is hard". The top voted response started with this statement: "1) Multithreading is extremely hard, and unfortunately the way you've presented this idea so far implies you're severely underestimating how hard it is."
While I'll admit that my presentation was initially lacking, I later made an entire page to explain the synchronisation mechanism in place, and you can read more about it here, if you're interested:
http://nexusjs.com/architecture/
But what really shocked me was that I had never understood the mindset that all the naysayers adopted until I read that response.
Because the bottom-line of that entire response is an argument: an argument against change.
The average JavaScript developer doesn't want a multithreaded server platform for JavaScript because it means a change of the status quo.
And this is exactly why I started this project. I wanted a highly performant JavaScript platform for servers that's more suitable for real-time applications like transcoding, video streaming, and machine learning.
Nexus does not and will not hold your hand. It will not repeat Node's mistakes and give you nice ways to shoot yourself in the foot later, like `process.on('uncaughtException', ...)` for a catch-all global error handling solution.
No, an uncaught exception will be dealt with like any other self-respecting language: by not ignoring the problem and pretending it doesn't exist. If you write bad code, your program will crash, and you can't rectify a bug in your code by ignoring its presence entirely and using duct tape to scrape something together.
Back on the topic of multithreading, though. Multithreading is known to be hard, that's true. But how do you deal with a difficult solution? You simplify it and break it down, not just disregard it completely; because multithreading has its great advantages, too.
Like, how about we talk performance?
How about distributed algorithms that don't waste 40% of their computing power on agent communication and pointless overhead (like the serialisation/deserialisation of messages across the execution boundary for every single call)?
How about vertical scaling without forking the entire address space (and thus multiplying your application's memory consumption by the number of cores you wish to use)?
How about utilising logical CPUs to the fullest extent, and allowing them to execute JavaScript? Something that isn't even possible with the current model implemented by Node?
Some will say that the performance gains aren't worth the risk. That the possibility of race conditions and deadlocks aren't worth it.
That's the point of cooperative multithreading. It is a way to smartly work around these issues.
If you use promises, they will execute in parallel, to the best of the scheduler's abilities, and if you chain them then they will run consecutively as planned according to their dependency graph.
If your code doesn't access global variables or shared closure variables, or your promises only deal with their provided inputs without side-effects, then no contention will *ever* occur.
If you only read and never modify globals, no contention will ever occur.
Are you seeing the same trend I'm seeing?
Good JavaScript programming practices miraculously coincide with the best practices of thread-safety.
When someone says we shouldn't use multithreading because it's hard, do you know what I like to say to that?
"To multithread, you need a pair."18 -
How the fuck am I expected to salvage a fucking project that has been handed down to me with.
- No fucking clear architecture
- No fucking documentation
- Fucking shitty ass code base with no fucking coding standards
- The previous team was fucking learning a whole fucking new technology stack *Not fucking kidding* making fucking mistakes left and right
- No code reviews
- Mixing fucking local and cloud enviroment together
- No fucking testing
- Feature that were supposed to be implemented and are not working
- No configuration all the stuff are hard coded
- Full responsiblity for the whole stack
- Only one other guy with me
- And this fucking project has been delayed for a year
- MUCH FUCKING MORE WHERE THAT CAME FROM
Like what the fuck am I expected to do? I took the job thinking that people knew what the fuck they were doing and surprise surprise that was a fucking bust.
the problem is also I am the junior and these fucking people have more experience than me, what the fuck happened to over seeing people's work, PM doesnt give a shit, developers dont give a shit nobody gives a shit.
But when I got this surprise surprise now everyone is interested in finishing the project
BULLSHIT11 -
The best thing about being a consultant is that you keep learning from the mistakes of people who follow your advice.3
-
Hey guys,
this rant will be long again. I'm sorry for any grammar errors or something like that, english isn't my native language. Furthermore I'm actually very sad and not in a good mood.
Why? What happened? Some of you may already know - I'm doing my apprenticeship / education in a smal company.
There I'm learning a lot, I'm developing awesome features directly for the clients, experience of which other in my age (I'm only 19 years old) can only dream.
Working in such a small company is very exhausting, but I love my job, I love programming. I turned my hobby into a profession and I'm very proud of it.
But then there are moments like the last time, when I had to present something for a client - the first presentation was good, the last was a disaster, nothing worked - but I learned from it.
But this time everything is worse than bad - I mean really, really worse than bad.
I've worked the whole week on a cool new feature - I've done everything that it works yesterday, that everything gets done before the deadline of yesterday.
To achieve this I've coded thursday till 10pm ! At home! Friday I tested the whole day everything to ensure that everything is working properly. I fixed several bugs and then at the end of the day everything seems to be working. Even my boss said that it looks good and he thinks that the rollout to all clients will become good and without any issues.
But unfortunately deceived.
Yesterday evening I wrote a long mail to my boss - with a "manual". He was very proud and said that he is confident that everything will work fine. He trusts me completly.
Then, this morning I received a mail from him - nothing works anymore - all clients have issues, everything stays blank - because I've forgotten to ensure that the new feature (a plugin) and its functionality is supported by the device (needs a installation).
First - I was very shoked - but in the same moment I thought - one moment - you've written an if statement, if the plugin is installed - so why the fuck should it broken everything?!
I looked instant to the code via git. This has to be a very bad joke from my boss I thought. But then I saw the fucking bug - I've written:
if(plugin) { // do shit }
but it has to be if(typeof plugin !== 'undefined')
I fucked up everything - due to this fucking mistake. This little piece of shit I've forgotten on one single line fucked up everything. I'm sorry for this mode of expression but I thought - no this can not be true - it must be a bad bad nightmare.
I've tested this so long, every scenario, everything. Worked till the night so it gets finished. No one, no one from my classmates would ever think of working so long. But I did it, because I love my job. I've implemented a check to ensure that the plugin is installed - but implemented it wrong - exactly this line which caused all the errors should prevent exactly this - what an irony of fate.
I've instantly called my boss and apologized for this mistake. The mistake can't be undone. My boss now has to go to all clients to fix it. This will be very expensive...
Oh my goodnes, I just cried.
I'm only working about half a year in this company - they trust me so much - but I'm not perfect - I make mistakes - like everyone else. This time my boss didn't looked over my code, didn't review it, because he trusted me completly - now this happens. I think this destroyed the trust :( I'm so sad.
He only said that we will talk on monday, how we can prevent such things in the feature..
Oh guys, I don't know - I've fucked up everything, we were so overhelmed that everything would work :(
Now I'm the looser who fucked up - because not testing enough - even when I tested it for days, even at home - worked at home - till the night - for free, for nothing - voluntary.
This is the thanks for that.
Thousand good things - but one mistake and you're the little asshole. You - a 19 year old guy, which works since 6 months in a company. A boss which trusts you and don't look over your code. One line which should prevent crashing, crashed everything.
I'm sorry that this rant is so long, I just need to talk to you guys because I'm so sad. Again. This has happend to frequently lately.16 -
We need to be learning from other's mistakes. A good weekly topic would be "a time you really failed as a dev, and what you learned".4
-
I did some grave and irreversible mistakes in my life
- Never gathered enough courage to mingle with women when I was younger and now the hope is lost
- Compromised my values and mental wellness when I met a narcissistic bitch
- Did not invest money wisely when markets were sailing low and allowed that good sum to sit in bank
- Did not plan health and term insurance at early age when premiums could have been low
- Out of fear, did not follow my gut to purchase gold because my father was acting crazy (or else my money would have been doubled)
- Did not plan my taxation well (or until now would have paid almost zero tax)
- Did not define strict boundaries and allowed people to overstep (or else I would have better friends and family relationships)
- Did not quit my job early and stuck with low paying shit with negative learning, for years (or else I would have grown exponentially)
Thankfully few things I did right are, spending more time with my mom and learning from my mistakes.
I hope I don't make such stupid life choices again.15 -
This new junior dev was going pretty well, learning pretty fast and working pretty fast from when I talked to them, but I wasn't seeing his changes up on GitHub.
Me: Hey have you finished *feature X*
Junior: Oh yeah a few days ago.
Me: Why aren't your changes up on GitHub?
Junior: Sorry, my bad, im not used to pushing stuff during the development since i was used to do all of my stuff on my own
(No kidding!)
Me: ok then push them
Junior: Emmm... I don't think I should... I kinda already started working on *feature Y* so it's full of bugs...
I don't wanna be mad at them they're pretty good at their stuff, and he's got some good comments on the performance of the program. But UUGGHH 😠
Rookie mistakes I guess14 -
Failure is not the problem.
Problem is not admitting and accepting that you failed.
Problem is not learning from your mistakes.
Problem is causing the same damage over and over again.
What's worsens the situation is defending the dumb act.
If you cannot handle failure then not only you stagnate your growth, but you also negatively affect people around you.
Mistakes make you perfect only when you fix them.7 -
Hello DevRant community! It’s been a while, almost 5 years to be exact. The last time I posted here, I was a newbie, grappling with the challenges of a new job in a completely new country. Oh, how time flies!
Fast forward to today, and it’s been quite the journey. The codebase that once seemed like an indecipherable maze is now my playground. The bugs that used to keep me up at night are now my morning coffee puzzles. And the team, oh the team! We’ve moved from awkward nods to inside jokes and shared victories.
But let’s talk about the real hero here - the coffee machine. The unsung hero that has fueled late-night coding sessions and early morning stand-ups. It’s seen more heated debates than the PR comments section. If only it could talk, it would probably write its own rant about the indecisiveness of developers choosing between cappuccino and latte.
And then there are the unforgettable ‘learning opportunities’ - moments like accidentally shutting down the production server or dropping the customer database. Yes, they were panic-inducing crises of apocalyptic proportions at that time, but in hindsight, they were valuable lessons. Lessons about the importance of thorough testing, proper version control, reliable backup systems, and most importantly, owning up to our mistakes.
So here’s to the victories and failures, the bugs and fixes, the refactorings and 'wontfix’s. Here’s to the incredible journey of growth and learning. And most importantly, here’s to this amazing community that’s always been there with advice, sympathy, humor, and support.
Can’t wait to see what the next 5 years bring! 🥂3 -
Fuck. The entire day to do this shit.
The screen was my first experiment, but because of a bad module (i2c) it didn't worked.
Today I finnaly got it to work.
Starting making everything almost like in the picture, everything mounted (and lots of black hot glue, no wires showing...
Didn't work.
One hour breaking everything apart without damaging the screen... Was a loose wire.
Started again... Didn't work...
The pot is also damaged, sometimes it works, others need to turn it hard.
New pot.
New set of wires.
Soldering everything right, testing all wires so no mistakes this time... But it takes so longgggg... Making everything in modules this time (to reuse without having to sordering again. And finally... It works.
By this time I should have 3 or 4 learning projects finish (I really wanted the screen to adapt all output in text, no serial, no blinking less, everything in modules, code prepared so, when I get my 40+ packages from China I already have a prototype tester ready.
10 hours... Fuck I'm really addicted, or else I would just solder everything together :D28 -
Hey guys, I have a serious question for you: How do you define science?
And yes this is going to be a long Rant. This topic really pisses me off.
A bit of context first. I come from a "humanities" background. I study history and dude, I love it. The problem is that even though we fucking pull our brains out studying historical phenomena with a fucking ton of conceptual tools, our work is mostly seen as literature to entertain the elderly during their lonely evenings. But that's not really the point of this rant.
My fucking problem is that while we try to do some serious work; actual work that could help society for real, it all goes into that magical fucking kingdom called "humanities". HOW THE FUCK DO THEY DARE TO CALL SOMETHING "HUMANITIES". IT'S A FUCKING HISTORICAL TERM THAT MEANS "TO FULFILL MEN IN ALL IT'S ASPECTS", AND NOW THEY'VE REPURPOSED IT, MAKING IT CONTAIN ANY STUDY THAT ISN'T "EMPIRICAL", "OBJECTIVE", ADD ANY FUCKING SCIENTIFIC DELUSIONARY TERM YOU CAN THINK OF.
And don't get me started on "objectivity". Oh boy, your fucking objectivity is hollow as a kid's balloon. There is no such thing as a objective study, even when it applies your "rational" "godly" scientific method. Some guys follow that shit as if it was a fucking religion. I do understand it's useful and all that, but in the end it's just a tool, you can't fucking define "science" by it's tools.
"""Q: What is carpintery?
A: Well, it's hammers, nails and wood. Yep. Hammers, nails and wood."""
THE SCIENTIFIC METHOD WAS FUCKING INVENTED DURING THE XVIII CENTURY, WHAT THE FUCK DO YOU THINK WAS GALLILEI BEFORE THAT? "HUMANITIES"?
Why do I say objectivity isn't posible? Well, guess what? YOU ARE FUCKING HUMAN. Every thing you know is full of preconceptions and fucking cultural subjectivities invented to understand the world. And it's ok, becouse if you understand your own subjectivity, at least you can see yourself in a critical sense, and at least "tend" to objectivity, in the same way functions tend to infinity.
And here comes the best part: people studying "cs" in my university pass most of the time studying a ton of shit that isn't really science, but is taken as scientific becouse it is related to "science". These guys spend entire semesters just learning programming fundational stuff that in my opinion isn't really science, it's just subjective conceptual constructs built to make the coding process better. They only have TWO fucking classes on discrete mathematics and another 3 or 4 in actual scientific fields related to computing. THESE GUYS AREN'T FUCKING BEING TAUGHT TO BE COMPUTER SCIENTISTS; THEY ARE TEACHING THEM TO BE PROGRAMMERS. THERE'S A HUGE DIFFERENCE BETWEEN CS AND PROGRAMMING AND THAT IS THE WORD SCIENCE. And yes, I'm being drastic on the definition of science on purpose becouse guess fucking what? I'M PISSED OFF.
"Hey, what are you doing?"
"Just doing science with scrum and agile development."
I understand most of you guys would think of science as "the application of the scientific method", "Knowledge by experimentation and peer-review", "anything techy". Guys, science is a lot broather than that. I define it as "the search for truth", mainly becouse that's what we are all doing, and what humans have been doing to gain knowledge through the ages. It doesn't matter what field of truth you are seeking as long as you do it seriously and with fundaments. I don't fucking care if you can't be objective: that's impossible. Just acknowledge it and continue investigating accordingly.
I believe during the last centuries the concept of science has been deformed by the popular rise of both natural and applied sciences. And I love the fact that these science fields have been growing so much all this time, but for fucks sake don't leave every other science (science as I define it) behind. Governments and corporations make huge mistakes becouse they don't treat history, politics and other sciences seriously. Yes, I called history a "science", fuck you.
And yes, by my definition programming is not a science. I don't know what most of you think programming is, but for me it's a discipline that builds stuff, similar to carpintery or blacksmithing. Now if you are pushing the limits, seeking ways to make computing go further, then that's science. The guys that are figuring out AI are scientists, the guys that are using it to detect hotdogs aren't - unless they are the same person- deal with it. I guess a lot of you guys are with me on this point.
In the end, we are all artisans building abstract tools by giving orders to a machine.
I still have some characters left, so I want to thank the community as a whole for letting me vent my inner rage. I don't have much ways to express myself on these matters, so for me DevRant is a bless.8 -
I'm getting beat up pretty bad by Rust. I like it so far but man is it hard. Imposter-syndrome is almost making me lose motivation. Almost, but I won't quit, one day I'll get there.
I think the primary reason I think I'm having such a hard time is that I'm trying to learn stuff that prevents me from making some mistakes that I have never run into. I know a bit of the theory but no hand's on experience on double-free errors, memory leaks and weird low-level stuff. I read the documentation, mostly understand what stuff is for but when I go write code I'm just like "now what?". I don't have enough experience to know when and where to use some concepts and I'm super lost. I don't know where to start and the feeling of being completely overwhelmed by all sorts of new stuff is at the same time exciting and frightening.
I have never, as a programmer, thought something was hard. All of my past knowledge required dedication, work and patience, but I wouldn't say I ever felt something was *hard*. But Rust... damn. Rust is hard.
Hopefully at the end of this super steep learning curve I'll know a lot more stuff and have stronger "dev powers" and be one step closer to being as knowledgeable as some of you guys around here to whom I look up to.2 -
Several years ago, I heard from a friend who was doing assignments for students on the side. Quite a hustle. His story began when he wanted to figure out why can't these students be able to draw their own database tables, relationships, UML, etc. That's what school has to be teaching them and then he was told that they were learning through MS Access. He goes and tell me that even though this is a lame way of teaching database design, its definitely easier to explain through hands-on and less typing mistakes, as according to the lecturer he met. Making the explanation more visually appealing and helpful for understanding.
OK I get it, but somehow that taught them the wrong way of database design from the beginning. I'd prefer getting them to start writing SQL commands from day 1 and play em at some DB VM. Keep em as real as it gets.
Now I have my own students asking for help in their assignment and also asked for tutoring lessons in web development. So I gave them the crash course in HTML, CSS and Javascript. I've asked them if they've used anything of what I taught them in school. They go and tell me that they've been taught web development through Wordpress. Oh WTF!? I havn't talked to their lecturer yet but it better be a really good explanation to teach these youngsters in a flawed and bloated PHP CMS framework for "web development".2 -
How do I help my colleague in fighting harrassment?
This is the story of a helpless employee facing everyday harassment. Im trying to help. Seeking for your thoughts
Backstory fast forwarded: My company acquired another company. So we handle all their projects and clients now, but its a completely new domain. So we needed new people. Hired 4 employees + 1 team lead to start with. But the project process got delayed and they were free for a month. So i took 2 of them in my project and gave them some small tasks to help us over. They loved working with my team and were learning new stuff apart from what they usually did. And we were also happy of their contribution. We became good friends. All of this was in March 2020 before covid-19 was taken seriously.
About my company: I love this company. I have been in this company for more than 4 years now. People are really nice. Parties and fun events. Lot of smart and ambitious people. So company and people are awesome.
Coming back to the story. Lets call the team the 4 and team lead T. The 4 were happy that someone like T was in their team. This T had all the best knowledge about stuff and life was going to be awesome for the 4. Or was it?
Story starts: So I talk to one of these 4 on daily basis. Lets call this friend F. F is a real gentle person. Intelligent and dedicated to work. F is awesome to work with. And always enjoyed working. F is a team player and very very soft person. F is fking workoholic. So few days after project starts, F tells me work was not going well. F is getting real frustrated at work and not able to deal with it or find solution.
What happened:
This person T, who was supposed to help these 4, is real piece of shit. He is impatient, arrogant and MFing dick head. Aaaarggggg.
All the good qualities of a leader like supporting the team, boosting confidence, guiding team when they make mistakes, teaching them, were all missing from this person. T was a machine with no emotion and only clock working jerk. I have no idea how T cleared interview process, because one of the interview round is also about cultural fit into company. I know this because i take interviews for other domains. We have rejected lot of such well qualified but arrogant candidates.
So whats the problem now: this team of 4 are learning new tools and taking over the clients requests from old company. Most of the stuff is new for them. So in tat case people need lot of time to understand and figure out shit. people make mistakes while learning and you know have to deal with it. Person T abuses these 4 when something goes wrong. That's one.
Second, the T definitely knows more than these 4. So if these guys dont understand certain stuff they ask T. But T does not help them learn. T will either say busy or run away by saying thats simple and ull know when time comes. REALLY MF???
Third, T does not talk nice. T is rude and does not listen to team members. For eg, If F says some task cannot be done for some reason T will say, "y cant u do it? U r capable of doing it. Tats y u r in this job". And then point number one and two happens. Never responds to emails and messages. But if someone else does the same will not tolerate that and abuses them. List goes on.
So y not escalate and deal with that T:
This person F and other 3 are still under probation and they think complaint or escalation will back fire. These people do not want to lose job in between all this pandemic shit. They are scared.
So this was happening for a while. And i was giving lot of tips on how to handle certain situations. And how one should communicate these.
But being a gentle, soft and workoholic person, F focussed on work and assumed things will get in place as time goes by.
Today, F could not meet a requirement. So T told some shit which got F all sad. and F called up me late night and started crying explaining what happened. I felt real bad. I asked F to file harrassment case. F refused saying it was F's mistake on not completing requirement. WHO THE FK CARES. PEOPLE CANNOT TALK SHIT. I told ill file harrassment case against T. (We have a policy where others can also file if person is not courageous enough). But F did not allow me.
Then after calming down, I told F that telling the problems to me wont solve them. You have to talk to T directly and tell him on face not to talk like this. Or tell the manager about whats happening. Or tell the the HR about this. F said tat cant be done. I was like Y THE FK NOT.
Because the other 3 are not ready to talk about this to anyone as they fear they'll lose job. So if F talks and people question other 3 they might bail out. WAT THE HOLY SPIRIT.
so after lot of convincing F is still not going to
Talk to anyone about this.
So i have decided ill write an anonymous email to HR, the manager and other senior people in the organisation about whats happening.
I really dont know how itll go. Ill keep updating you guys. Feel free to share ur thoughts.3 -
I'm working on a codebase that is terminally ill. It's split so badly into microservices that no matter what you do, every one of them talks to every one of them over and over. If there's any way they can avoid just invoking a method on a class and send themselves a message or make an HTTP request, they'll do it. One of the services just sends messages to itself for no apparent reason. Except it doesn't even send messages to itself. It sends an HTTP request to a controller in another app, and that controller sends a message which is received by the same class that made the request.
The point is that this application is screwed. The defects pile up and there is literally no one who can understand what it's supposed to do in any scenario. I'm good at this. I can follow confusing code and document it. But not this one. It's overwhelming. It's insanity.
When these defects come in we're told to just run the app from the UI, see what HTTP requests it makes, and start tracing the code manually. Running and debugging it locally would be a nightmare but it's impossible anyway.
They decided that we all need to understand the application better so we can work on it, so we were each given six poorly-define five-hour tasks to "understand" various things. Those things don't make any sense. It's like if someone gave you the source code to Excel and told you to spent five hours understanding columns, five more understanding rows, and five more understanding cells.
Here's the thing: I'm okay with learning and understanding some code. It's part of the job. But I'm not going to abandon my career as a software developer so I can become an expert on debugging their awful code. I didn't make this mess. I'm not going to live with it. I'm moving on as quickly as I possibly can.
I've tried to explain to them that if they want the situation to improve they need to improve the code. They need to learn how to write tests. If your plan is that people will study your code, know it inside and out, and then spend all their time debugging it, that's a plan for failure. Everyone who can will leave and take what they know with them.
These companies just don't get it. They need their software to work, but the types of developers who can help them don't need that software to work. No one capable of doing good work is going to spend several years debugging their awful code unless you pay them a crazy ton of money.
Just don't make a mess in the first place. Hire developers who can do a good job. If you hire the cheapest people you can find you won't be able to get someone else to fix it later. It's not personal but I wish failure on those projects or even those companies. I want them to fail because failure is so expensive. I want them to fail so that others learn from it and don't repeat the same mistakes.
As an industry we're a bunch of genuine idiots. We just keep doing the same things over and over again no matter how much it hurts.1 -
Dear diary,
Today with my dad at AI expo, and it was the best person to go with. He has learnt reinforcement learning and ocr. Never enjoyed a museum together that much. There were also steam engines and minecraft carts and elevator.
Did you know that the car years ago was considered dangerous bevause you didn't have a horse to protect you from mistakes? In horses we trust, nit in Tesla.
It was a great day.15 -
So we're doing this contract work for this other company and the project is just an overcomplicated piece of garbage where they shoved every buzzword technology into it just because. I managed to get the code just about organized and functional on our side of the contract and it was looking up when suddenly the management decides "we had a rough start, lets start over, learning from our mistakes"
So I was thinking "cool, there were a lot of problems with this overcomplicated pre-optimized stack, surely we can only do better".. oh boy how naive I was. See Im not the guy in charge of the infrastructure (unfortunately) and really the project structure across this huge multi service project is a free-for-all kind of management.. so we had a call on friday where they explained how the new structure should be built... 3 new technologies, more micro services and even worse dependency tree later I was contemplating suicide on the spot.
I tried to make this shit usable and efficient and all my fucking work went down the drain in a single day of these fuckers throwing more buzzwords at the problem... I can't even get a new empty project started without browsing our huge 100+ repo project git for which dependency Im still missing to even run it...
I fucking hate this retarded piece of crap project and I hope every "manager" and "developer" with an exception of very few chokes on a cock...2 -
I had this dream, We had to destroy this super mainframe who wanted some revenge because we as a programmers made a lot of mistakes in the code.
So we had to create this amazing machine with super powers and machine learning and then to go back to the past to save the world and to find me and protect me from the same machines that we created..
At the end, I died.. because the fucking machine betrayed me.1 -
Random recruiter from LinkedIn sends an “opportunity” in a well stablished German company in Madrid ..
.. has three entries in requirements for jquery, associated with, and I quote “OOP, Object Programming, and other frameworks” ..
Goes on to require knowledge of “css, scss and saas”, along with “Don HTML” ..
And requests “experience with the principles of agile user interface methodologies” ..
And Angular 1 ..
How would you respond to this one!?
I actually did, corrected the mistakes, told what other mistakes were at the differences between libraries and frameworks, .. and that I don’t like Angular and I’m not interested in learning the old one at all ..1 -
Always learning new technologies, solutions, and stuff, never afraid to leave my comfort zone, and learning from my or others' mistakes.
-
To me this is when you have that one breakthrough you spend considerable time on and with the divine knowledge of a peer collegue solves it in minutes... That feeling of enlightenment. That is what drives me everyday. Learning from mistakes, record progress, expand your knowledge, and call for help when you're stuck. Every single day.
-
Today I sat down and made my first two proper scripts in bash, I have been studying and pulling bits of information together, and ive finally got a grasp on Redirection. I found a script for a Dialog driven Menu, tore it to pieces and learnt from it.
2 Hours Later, stupid mistakes and lots of coffee, I have the start of something I think is cool :) If anyone would like to take a look and give me some comments I have pushed both projects to github. And will be working on the GUI one all day :)
https://github.com/Rotho98/...6 -
So when i was playing saxophone, I realized that music and coding are just the same. When you get a new score to play you need to start over by learning how to play it. When you code and start a new project you need to start from zero. When you play the score over and over you learn from your mistakes. When you code you debug your faults when there is something wrong in your code. At the exam you need to make no faults when you play music. When you’re at the deadline. There shouldn't be no faults in your code
-
What's devrant's opinion on WordPress blogs?
I've wanted to start a blog for a while to share what I know and learn from others who read and want to correct my mistakes on the blog. But as of now my own site is barely held together and nowhere near ready to host a blog. So while I continue learning and building my skills to eventually make my site more reflective of my abilities I'm considering starting WordPress blog to just start now rather than continue the 'eventually' trend I've been following while working on my site.21 -
The most stressful day of month.
I need to put hours into hour counting programs so computer can analyze those hours using deep learning algorithms and pay me a wage I don't deserve.
Each program work differently.
One of it works inside the local company network.
Other one I need to connect outside from company network.
In all of them I can't make mistake or I need to write to someone to fix my mistakes.
One of this programs use java applet, other is simple php website.
One of them blocks row in calendar when I click so when I login again and click I can't edit this row because it's locked by me who is editing this row.
One of them is requesting me to provide my work in minutes.
I need to follow strict procedures to report any holidays or national holidays that I need first figure out when they happen.
Wish me luck.1 -
I enjoy learning and improving my skills, but I do not enjoy the being wrong part of learning. I understand learning from mistakes is a big part of improving, but man being wrong constantly is exhausting.
-
Let's be honest - given the state of the world today, the more I listen to Megadeth, the more I relate to what Dave Mustaine has been pissed off about for a few decades now. Oh, you don't know who Dave Mustain is? He was, like, the 5th guy in Metallica. Rather, he was the bass player until he got fucked over because he was a dick and thrown off the first album Metallica did. Don't worry - he did OK. He formed Megadeth and still had quite a successful musical career. Why am I ranting about him? Simple - A lot of his lyrics are darker than Metallica's. I honestly don't know what the fuck I'm doing with my software/personal/professional life right now. I've got ideas & dreams, but all this COVID shit is just draining the fuck out of me. Sometimes I feel like I've failed - most of the lifeforms on this planet manage to procreate. Well, that didn't happen for me. On the down side, I didn't get to be a father. On the up side, I didn't punish the life of a child with my own brands of mistakes, ignorance, and stupidity. My life is littered with male failures. My biological father (paranoid, schizophrenic ) died at 58, doing everyone around him a favor. My grandfather on my mother's side died of colon cancer at 69 (so-called reformed alcoholic, manic depressive on lithium with great abusive tendencies). My step father who adopted me? Sure - he loved me. He just never understood me. "Computers are just a tool". Fuck you, 'dad'. Go play with your horses and tell me what I'm doing isn't meaningful. Where was I? Oh yes, almost killing myself last summer. I think between COVID and my own colossal screw ups & paranoia I went over the entire fucking edge. I pulled myself out of it with the help of medication, counseling, and learning to just let shit blow up because "it's not my problem". I'm still angry. Perhaps that's the only thing that keeps me going from time to time. I'll leave you with a quote from Ghandi - No, not that idealistic, limited one, Mahatma Ghandi. From his grandson, who managed to really pick up what he was putting down - Arun Ghandi:
“Use your anger for good. Anger to people is like gas to the automobile - it fuels you to move forward and get to a better place. Without it, we would not be motivated to rise to a challenge. It is an energy that compels us to define what is just and unjust.” -
Need advice:
So I’m 20 years old. Got a decent job as software engineer with a really good pay and really want to break into machine learning.
Mastered NodeJS (my stack has always had node for the past 5-6 years) and I’m finding it difficult to switch to python for machine learning since things are so engraved in my head in javascript.
Aside from the syntax when I’m watching tutorials or reading books, I see data scientists and mathematicians make design mistakes in their code and it hurts my eyes and triggers my ocd.
I need tips on how to put my mindset in a moldable state so I can judge less and learn more and absorb data. Like you know that philosophy that when u get old your brain can’t learn things as fast anymore? I feel like that’s already happening to me rn at the age of 20.5 -
Woke up yesterday morning from a dream where I was explaining what needed to be done to upgrade a Drupal 6 site. It hasn't been supported officially for years and I was explaining how there isn't a decent port of the main module we use Audio. And even the guy I was explaining this too seemed somewhat exasperated. So yeah, this is reality.
I could probably write a real upgrade path for the Drupal module and take all of our content into a new version of Drupal. But it would involve a fair amount of learning and outdated syntax and then learning Drupal 8. This would be all volunteer and take away from my time working on my other open source radio automation project.
All the while I've been learning Ruby on Rails for a class and I could just upgrade the app right out of Drupal, but this would require me to support the site into perpetuity. Which I already more or less do.
Drupal at this point is like an ex- girlfriend who I've grown away from, we did cool things but always got into fights about stupid things. Now I have to revisit my past mistakes and decide what to clean up and what to take into the future. I'm a better programmer now but I'm still not sure if it is worth my time to rekindle my romance with Drupal or it would just distract me from my current pursuits. Anyone who has been through the transition of a Drupal site from one major version to the next should feel my pain. At least it's not Word Press.