Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
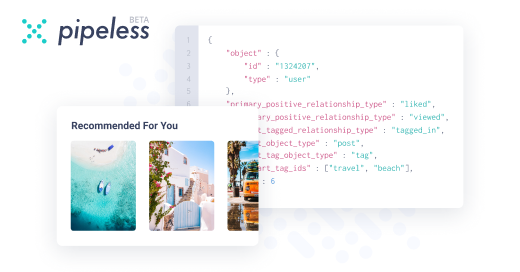
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "binary tree"
-
Wife: what are you thinking about?
Me: how to optimize key storage in a binary tree
Wife: forget that I asked5 -
Yo mama's so fat that if she sat on a binary tree she will convert it into a linked list in O(1) time5
-
Before you're hired:
1. A binary tree?
2. Currying?
3. Higher-order function?
4. How does event loop work?
5. What is prototype?
6. What is encapsulation?
7. Can you draw an algorithm?
After you're hired:
1. Hey, can you add auth token and login to our app?11 -
Me talking to a recruiter (even though I am not looking for a job)
Me: If I walk into an interview, and they ask me to reverse a binary tree for a frontend Reac or Vue position or something along those lines, I will end the call and/or walk away from it.
Him: I get similar feelings from other programmers, I don't quite understand why the notion is as common
Me: Because it is fucking useless, it servers no purpose to a dev to know about that when building frontends with react, I link my github profile, for which they can find advanced backend-frontend related projects, compiler and interpreter projects, plus the title I currently have at my workplace and a bunch of other shit, I am not interviewing for a teaching position at an institute, but an actual place of work, for which if they want to know about DS and A they can review my profile which has a repo of DS and A in about 5 different languages including plain C++. I do not need to be offended by such notions since they server no purpose on the frontend, and neither do other devs. If anything it should be a casual conversation during the interview, not a basis for employment.
Recruiter: .........thank you for explaining this to me, I am sure I can bring it up to the agencies doing the reviews and interviews. Are you still interested?
Me: Are they going to give me a coding assignment for a project or a bs question like what I mentioned?
Him: I don't know
Me: then I am not interested12 -
Open source block chain neural network binary tree growth hacker synergy vertically integrating cryptocurrency game changing GDPR compliant internet of things node.js quantum computing start up that'll disrupt and pivot the cloud based ecosystem11
-
You are asked by airport security to balance this binary tree to prove you're a programmer. How fucked are you?12
-
Today, implemented Binary Tree from scratch and then wrote a unit testing suite from scratch in my personal project.
Pretty neat.7 -
I've optimised so many things in my time I can't remember most of them.
Most recently, something had to be the equivalent off `"literal" LIKE column` with a million rows to compare. It would take around a second average each literal to lookup for a service that needs to be high load and low latency. This isn't an easy case to optimise, many people would consider it impossible.
It took my a couple of hours to reverse engineer the data and implement a few hundred line implementation that would look it up in 1ms average with the worst possible case being very rare and not too distant from this.
In another case there was a lookup of arbitrary time spans that most people would not bother to cache because the input parameters are too short lived and variable to make a difference. I replaced the 50000+ line application acting as a middle man between the application and database with 500 lines of code that did the look up faster and was able to implement a reasonable caching strategy. This dropped resource consumption by a minimum of factor of ten at least. Misses were cheaper and it was able to cache most cases. It also involved modifying the client library in C to stop it unnecessarily wrapping primitives in objects to the high level language which was causing it to consume excessive amounts of memory when processing huge data streams.
Another system would download a huge data set for every point of sale constantly, then parse and apply it. It had to reflect changes quickly but would download the whole dataset each time containing hundreds of thousands of rows. I whipped up a system so that a single server (barring redundancy) would download it in a loop, parse it using C which was much faster than the traditional interpreted language, then use a custom data differential format, TCP data streaming protocol, binary serialisation and LZMA compression to pipe it down to points of sale. This protocol also used versioning for catchup and differential combination for additional reduction in size. It went from being 30 seconds to a few minutes behind to using able to keep up to with in a second of changes. It was also using so much bandwidth that it would reach the limit on ADSL connections then get throttled. I looked at the traffic stats after and it dropped from dozens of terabytes a month to around a gigabyte or so a month for several hundred machines. The drop in the graphs you'd think all the machines had been turned off as that's what it looked like. It could now happily run over GPRS or 56K.
I was working on a project with a lot of data and noticed these huge tables and horrible queries. The tables were all the results of queries. Someone wrote terrible SQL then to optimise it ran it in the background with all possible variable values then store the results of joins and aggregates into new tables. On top of those tables they wrote more SQL. I wrote some new queries and query generation that wiped out thousands of lines of code immediately and operated on the original tables taking things down from 30GB and rapidly climbing to a couple GB.
Another time a piece of mathematics had to generate all possible permutations and the existing solution was factorial. I worked out how to optimise it to run n*n which believe it or not made the world of difference. Went from hardly handling anything to handling anything thrown at it. It was nice trying to get people to "freeze the system now".
I build my own frontend systems (admittedly rushed) that do what angular/react/vue aim for but with higher (maximum) performance including an in memory data base to back the UI that had layered event driven indexes and could handle referential integrity (overlay on the database only revealing items with valid integrity) or reordering and reposition events very rapidly using a custom AVL tree. You could layer indexes over it (data inheritance) that could be partial and dynamic.
So many times have I optimised things on automatic just cleaning up code normally. Hundreds, thousands of optimisations. It's what makes my clock tick.4 -
Computer Scientists put the root at the top of their trees because they've never been outside to see what a real tree looks like3
-
I am a firmware developer with 4 years experience. C and sometimes assembly is my bread and butter.
Like 2 years ago, I was really interested to make a switch to application development. Got referred by my friend to her startup.
But I was a bit rusty with my data structures, high level languages and interpersonal skills.
The first question was to find the number of occurences for each word in a paragraph. The language choice was Java. But I was allowed to use C++ since it was the closest relative to Java that I knew.
And I started implementing a binary search tree from scratch and started inserting each tokenised word into it, wrote a traversal algorithm.
The interviewer, luckily, was a patient guy. After I completed my whole mess, he asked is it possible to do this in a slightly better way with constant time access without traversal.
I said yes, we can with a hash table but I dont know how to implement one. He replied I dont expect you to implement the hash table but see you use it. I asked him if I am allowed to used the standard library, for which he said ofcourse.
*facepalm*.
Finally I understood his expectation, referred cppreference.com and used an unordered_map.
Later there were some quesion on databases for which I tried my best to answer. And I frankly replied that I am not comfortable with JS frameworks as of now. Got rejected.
So the mistake is I never asked basic questions like what is the time complexity expects, if I was allowed to use standard library, didnt spend some extra time on studying stuffs needed for the domain switch and most importantly I panicked.7 -
Hmmm, this feature that I added seems to make the whole process 50% longer. I need to optimize something. Let's see now...
Yeah, make that a shared resource and parallelize IO to leverage the multi-core architecture. Hash map for this, binary tree for that. This thing that gets called a million times can be written easily without a regexp. That thing can be rewritten in Rust as it's too demanding.
There! Works! And it's also a lot cleaner. Nice!
How's the performance doing? 70% longer.11 -
Software engineers: "Maths is hard and scary!"
Also software engineers: "I've learnt to write a balanced binary search tree in c++ as interview prep!"
Mathematicians: "Have you guys heard of an AVL tree?"20 -
The hammer dev. See a problem, they grab a hammer. See a screw? Hammer. Complex social issue? You bet your binary tree it's getting hammered.2
-
Just thought I'd share what I've been working on lately.
Heap: https://repl.it/@AmyShackles/Heap/...
Array: https://repl.it/@AmyShackles/...
Binary Tree: https://repl.it/@AmyShackles/...
(I'm so freaking tired.)6 -
Recently I launched the minimalistic online drawing app https://okso.app. I wanted it to be a place where people could do fast, ad-hoc, napkin-based-like explanations of any concept as if you are sitting with your friend and trying to explain him/her something during lunch. Don't ask me why it is needed, I was just experimenting.
So, the first concept I've tried to explain with sketches was the Data Structures. Without further ado, here is the interactive ✍🏻 https://okso.app/showcase/... showcase that you may play with.
Of course, not all data structures are covered. And of course, this is not comprehensive material, but rather a cheatsheet that would create visual hints and associations for the following data structures:
- Linked List
- Doubly Linked List
- Queue
- Stack
- Hash Table (with hash collision resolution)
- Tree (including the Binary Search Tree)
- Heap (including Mean Heap and Max Heap)
- Trie
- Graph
Each box on the sketch is clickable, so you may dig into the data structure you're interested. For example `Heap → Max Heap`, or `Heap → Min Heap`, or `Heap → Array Representation`.
The sketches are split into so-called Pages just to make it easier to grasp them, so the users stay focused on one concept at a time, they see the relationship between the concept, and thus, hopefully, they are not getting overwhelmed with seeing a lot of information at the same time on one drawing/page.
Each page has a link to the source-code examples that are implementing the data structure on JavaScript.
The full list you may find in the ✍🏻 https://okso.app/showcase/... showcase.
I hope you find this showcase useful and I hope it will be a good visual cheatsheet-like complement to your data structure knowledge.12 -
Android dev job question:
"Describe the activity lifecycle and write an application that does x,y,z in accordance with it"
Fullstack dev job question:
"Write some code that interacts with our API and does x,y,z, put the data into our database and build a web interface"
Java backend dev interview :
"BUILD AN ELEVATOR ALGORITHM WITH LESS THAN o(nlog(n)), FIND NEIGHBORS IN A BINARY TREE, WHAT IS THE DIFFERENCE BETWEEN AN INTERFACE AND ABSTRACT CLASS?"
Why?5 -
So during a test I was to write an algo of binary search tree traversal and I got a zero....Why? Cause I wrote a recursive algorithm and also because I wrote "English" instead of pseudocode, which she thought is called algorithm and on showing her the exact same recursive Algo on various websites and books, she just declared all of them wrong1
-
If you're currently in college and wish to get placed in a major tech giant like Amazon or Facebook:
Don't learn React.js, instead learn Linked lists.
Don't learn Flutter, instead learn Binary search trees.
Don't learn how to perform secure Authorization with JWTs, instead learn how to recursively reverse a singly linked list.
Don't learn how to build scalable and fault tolerant web servers, instead learn how to optimally inverse a binary search tree.
These big tech companies don't really care what real world development technologies you've mastered. Your competence in competitive programming and data structures is all that matters.
The system is screwed. Or atleast I am.18 -
The saddest and funniest side of our industry is (atleast in India): someone works hard and makes it to the best colleges, do great projects on AI, ML; get a good score on Leetcode, codechef; gets a job in FAANG-like companies...
Changes colors in CSS and texts in HTML.
And, why is there so much emphasis on Data Structures and Algorithms? I mean, a little bit is fine, but why get obsessed with it when you never write algorithms in production code?
Now, don't tell me that, we use libraries and we should know what we are doing, no, we don't use algorithms even in libraries.
Now, before you tell me that MySQL uses B-tree for maintaining indexes, you really don't need to solve tricky questions to be able to understand how a B-tree works.
It's just absurd.
I know how to little bit on how design scalable systems.
I know how to write good code that is both modular and extensible.
I know how to mentor interns and turn them into employees.
I know how to mentor junior engineers (freshers) and help them get started.
Heck I can even invert a binary tree.
But some FAANG company would reject me because I cannot solve a very tricky dynamic programming question.4 -
I might create a coding course for people actually interested in learning how to program correctly (not Get Rich Quick Bootcamp style, not webapps, not magic Javascript incantations).
I have an idea on how to structure it but I worry it'll be too weird for most people to follow (starting from binary theory and then teaching machine code and then working upwards to C and beyond) explaining how a computer works along the way, showing the real errors with annotations explaining things, etc.
I've always wanted to teach in this format but I feel as though it's too.. idk, "useless" to most people? But I've never had a friend go through e.g. CodeAcademy and come out knowing how to actually make applications from start to finish without just hacking together random React components and hoping the frankenstein project works well enough.
The target demographic would be those either completely new to programming or just have a fundamental or web-centric preexisting knowledge, or maybe those who simply want to understand computers better.
Am I barking up a shitty tree?28 -
Major rant incoming. Before I start ranting I’ll say that I totally respect my professor’s past. He worked on some really impressive major developments for the military and other companies a long time ago. Was made an engineering fellow at Raytheon for some GPS software he developed (or lead a team on I should say) and ended up dropping fellowship because of his health. But I’m FUCKING sick of it. So fucking fed up with my professor. This class is “Data Structures in C++” and keep in mind that I’ve been programming in C++ for almost 10 years with it being my primary and first language in OOP.
Throughout this entire class, the teacher has been making huge mistakes by saying things that aren’t right or just simply not knowing how to teach such as telling the students that “int& varOne = varTwo” was an address getting put into a variable until I corrected him about it being a reference and he proceeded to skip all reference slides or steps through sorting algorithms that are wrong or he doesn’t remember how to do it and saying, “So then it gets to this part and....it uh....does that and gets this value and so that’s how you do it *doesnt do rest of it and skips slide*”.
First presentation I did on doubly linked lists. I decided to go above and beyond and write my own code that had a menu to add, insert at position n, delete, print, etc for a doubly linked list. When I go to pull out my code he tells me that I didn’t say anything about a doubly linked list’s tail and head nodes each have a pointer pointing to null and so I was getting docked points. I told him I did actually say it and another classmate spoke up and said “Ya” and he cuts off saying, “No you didn’t”. To which I started to say I’ll show you my slides but he cut me off mid sentence and just yelled, “Nope!”. He docked me 20% and gave me a B- because of that. I had 1 slide where I had a bullet point mentioning it and 2 slides with visual models showing that the head node’s previousNode* and the tail node’s nextNode* pointed to null.
Another classmate that’s never coded in his life had screenshots of code from online (literally all his slides were a screenshot of the next part of code until it finished implementing a binary search tree) and literally read the code line by line, “class node, node pointer node, ......for int i equals zero, i is less than tree dot length er length of tree that is, um i plus plus.....”
Professor yelled at him like 4 times about reading directly from slide and not saying what the code does and he would reply with, “Yes sir” and then continue to read again because there was nothing else he could do.
Ya, he got the same grade as me.
Today I had my second and final presentation. I did it on “Separate Chaining”, a hashing collision resolution. This time I said fuck writing my own code, he didn’t give two shits last time when everyone else just screenshot online example code but me so I decided I’d focus on the PowerPoint and amp it up with animations on models I made with the shapes in PowerPoint. Get 2 slides in and he goes,
Prof: Stop! Go back one slide.
Me: Uh alright, *click*
(Slide showing the 3 collision resolutions: Open Addressing, Separate Chaining, and Re-Hashing)
Prof: Aren’t you forgetting something?
Me: ....Not that I know of sir
Prof: I see Open addressing, also called Open Hashing, but where’s Closed Hashing?
Me: I believe that’s what Seperate Chaining is sir
Prof: No
Me: I’m pretty sure it is
*Class nods and agrees*
Prof: Oh never mind, I didn’t see it right
Get another 4 slides in before:
Prof: Stop! Go back one slide
Me: .......alright *click*
(Professor loses train of thought? Doesn’t mention anything about this slide)
Prof: I er....um, I don’t understand why you decided not to mention the other, er, other types of Chaining. I thought you were going to back on that slide with all the squares (model of hash table with animations moving things around to visualize inserting a value with a collision that I spent hours on) but you didn’t.
(I haven’t finished the second half of my presentation yet you fuck! What if I had it there?)
Me: I never saw anything on any other types of Chaining professor
Prof: I’m pretty sure there’s one that I think combines Open Addressing and Separate Chaining
Me: That doesn’t make sense sir. *explanation why* I did a lot of research and I never saw any other.
Prof: There are, you should have included them.
(I check after I finish. Google comes up with no other Chaining collision resolution)
He docks me 20% and gives me a B- AGAIN! Both presentation grades have feedback saying, “MrCush, I won’t go into the issues we discussed but overall not bad”.
Thanks for being so specific on a whole 20% deduction prick! Oh wait, is it because you don’t have specifics?
Bye 3.8 GPA
Is it me or does he have something against me?7 -
I am wondering how a #FunctionalProgramming based implementation of a Binary Search Tree would look like?
Has anyone tried it?11 -
Boy oh boy.. Reminds me of good ol college days. I was in my final sem when Amazon came to our university for campus hiring. I was very confident that I will get selected. Funnily enough I went till the final round and I had a feeling that it went well if not excellent. It was a Friday night and we had to wait two excruciating days for the final shortlisted result to come. On the evening of Monday my friend T called me and told me my name is not on the list. I was heartbroken. I asked him who all got selected and he said our friend A did. A was, and still is a good friend of ours and I was happy for him. That night we sat down for drinks and as the night progressed I anguished over my selection. I still remember solving a binary tree problem holding a glass of whiskey in my one hand. The next morning I woke up at 6, detoxed myself with fruit juices and sat in front of my laptop feeling full rage from last night. I sat till lunch and hacked a chrome extension in one sitting. Mind you I had no existing knowledge of extensions at that point of time. I sometimes look how my life has turned since that time and now I am one of the devs in a team which work on a product that itself is a browser extension. :)
-
So my data structure's exam's result came and i got scored 57.5 out of 80. My classmates who barely know anything about C scored way more than me. I am so embarrassed at myself but i gave the right answers in exam. My score in the exams before was 39/40 and 38.5/40. All my hardwork failed because it was a so called THEORY exam where there was only 2 small questions of writing algos but all others were just like "describe pre-order traversal of a binary tree" or "write the difference between a tree and a graph?define adjacent node, path and complete graph"...
When will this fuckery end?2 -
Googling how to electromagnetically shield a espruino project from neodymium magnets over lunch, and that leads on a trail of manipulating and directing em fields...
"What are you doing? That doesn't look anything like a binary tree structure in Java... What the hell is all that?"
"Uhm... Personal research?" -
Girl: "Professor I don't really understand the algorithm. Can you please write it on the board again?"
Professor writes down the alphabet.
Girl: "I don't get it."
We were talking about traversing a Binary search tree in Inorder traversal.2 -
Have you ever seen a tree data structure implementation in any code base?
I wonder why recruiters are so desperately asking how to invert binary trees in my coding interviews🥴4 -
Oh look I'm posting the same thing again because all logical patheways lead to the same fucking place.
Sort of binary tree with a few keys added visualized.7 -
Just had an interview on a CS graduate from a top university with several years industrial experience who cannot even write pseudocode to rotate a binary tree. What is wrong with this world?4
-
So I see posts about an interview question/challenge of inverting a binary tree. I don't use trees very often (mainly file related or parsing server nodes), but I thought I would learn how to do this.
I saw a page that started talking about different ways to invert enough to understand that one type of inversion is swapping left and right nodes. So I stopped before they showed how.
Then I created a test program that has a tree structure and also can display a tree before and after modification. This was kind of fun.
So then I wrote the inversion function. It was less than 10 lines of code. Wtf? I thought it would be harder than this.
Then I started wondering where trees were used. So today I have been learning how they are used and why I might need one to solve a problem. One use I intuited was parsing regex or a language. Apparently it is useful there.
What I am learning is that a lot of these interview questions are really test to see if you can comprehend instructions when stressed. Or you will ask questions to clarify the task. It doesn't necessarily test your ability to solve hard problems.
One thing that perplexes me. If inverting a tree is swapping nodes left<->right, then why not leave data in place and just swap roles in the functions. Maybe I completely misunderstood what inversion means or why it would be done. I guess if this is not inverting I have the structure to try other methods now.2 -
The rear ducking continues. We've built a reliable translator in the dumbest fucking way possible, it's just lovely. I simply reused the structure for feeding data to the VM assembler, an array of arrays, where there's one array of (ins [args]) per node in the parse tree.
It's nice because nodes can be solved out of order without affecting the actual sequence in which the instructions are output. And if one statement (node) equals multiple instructions, you just push multiple entries to the corresponding array, or push nothing if you need to output nothing. Easy as goblin pie.
This is enough to convert an input language to the assembly-like intermediate representation we use for the virtual machine. So then there's doing it backwards: walk the same array of arrays, and map those virtual instructions to a physical architechture. I guess I could do the encoding to native binary myself, it'd certainly be interesting to try, but I'm burnt-out already so I'll just use fasm for now.
Initial test: wrote a test program in my own stupid language, ran the translator, dump output to file, assemble that with fasm, run with r2 -d.
Crashes? No.
Runs fine? Yes and no.
For fuck's sake, I don't have syscalls. Mainly because the VM doesn't have an operating system, lmao. I was testing virtual programs by just freezing state, terminating, then dumping the fucking registers and stack to the console, we have no I/O to speak of. Not even a real 'exit', VM handles that by reading a return value every step like a mentally damaged son of a bitch.
So anyway, I manually paste the linux mambo, you know:
mov rax,60
mov rdi,0
syscall
And NOW our program can end execution without crashing.
Okay then, so does the test code work correctly?
** DRUM ROLL **
Yes.
Ladies and gentlemen, mother fucking PESO is now a compiled language, and going forward I will be expectantly receiving your marriage proposals for reviewing. Oh, but not so fast, we still need a frontend...
Well, we'll handle that in the next few days. I'm just glad to be *nearly* finished with this fucking compiler, I want nothing to do with anything else ever, but we know that's not going to happen, so Lord please end my pain.
No sponsor as this rant has been paid for by tax evasion. -
TSA questions for screening software engineers: Sunday an engineer from Nigeria got screened on if they were really a coder with googled questions like "write a function to check if a Binary Search Tree is balanced." What questions should they really be asking?7
-
the most interesting question tech interveiwers should ask a job applicant should be "we want you to balance a red and black tree using bash"2
-
If you think root is at the top of the tree not at the bottom then you're already my close friend.
#CodingForLife -
It is Saturday 19:30 and I am spending my time writing functions for save/load data in a binary tree. Recursion and fscanf are not a good combo so far, but that is the task. When the code is done I have to get some math done.
-
Do all devs know how to write these Goolgle/Amazonesq algorithms off the top of their heads? ex: build singly linked list from scratch / pre or post binary tree traversals etc..5
-
So my latest assignment's having me write a recursive method that can traverse a binary tree and return the data in a List. The catch? I can't pass anything in and I can't make any new instance variables.
How and why