Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
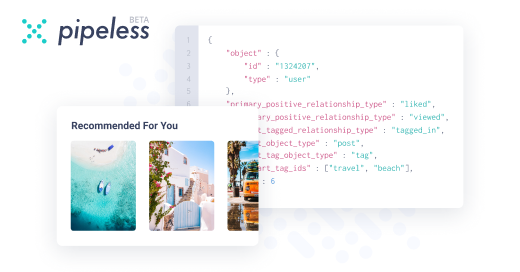
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "bad variables"
-
So... I just remembered a story that's perfect for devrant.
My brother got into engineering in university, and during the second semester they had their introductory class to programming. They had weekly homeworks that the lecturer would check and give grades accordingly.
The factors that could influence the grading were: execution (meaning that the code would excecute as intended), efficiency and readabilty. The weeks passed and everyone was doing well, getting fairly good grades. Everyone was happy.
Until one day a random guy we'll call bob got the worst grade possible. Bob wasn't a bad student. He had over-the-average grades in all the weekly homeworks and even impressed the professor in some. Naturally, he was baffled when he saw his grade on the google spreadsheet. He was pretty sure his code ran well. He always tested it on different machines and OSs. So, at the end of the class, he went straight to the helper of the class, in a pretty imperative manner, to demand to know how the fuck he got that grade. It's impossible he got excecution, efficiency and readabilty, wrong. All three wrong? Impossible. Even the stupidiest kid in the class had some points on readabilty.
"Oh, so you are Bob. Huh?" said the helper in a laid-back attitude. "Come with me. Prof. X is waiting for you in his office."
This got Bob even more confused. As they approached the office, the courage he had in a first moment banished and gave way for nervousness and fear.
The helper nocks the door. "Prof., Bobs here"
As soon as Bob sits in the chair in front of Prof. X's, he knew something bad was coming.
"In all these years of teaching..." said Prof. X hesitantly. "In all these years of teaching I have not come even close to see something similar to what you've done. You should be ashamed of yourself." Needless to say, Bob was panicked.
"In all these years I have not seen such blatant mockery!" added the professor. "HOW THE FUCK DID YOU EVEN DARE TO SEND A HOMEWORK WITH SUCH VARIABLE NAMING" That's when Bob realised the huge mistake he made. "NEVER IN ALL THESE YEARS I HAVE SEEN SOMEONE NAME HIS VARIABLES *opens the file on his desktop *: PENIS, SHIT, FUCKSHIT, GAYFUCKING<insert Prof. X's name>MAN, GOATSE, VAGINAVAR, CUMFUNCTION, [...]" The list of obcenities went on and on. In each word, the professor hit the table harder than the last time.
Turns out Bob felt so in comfort with the ease of the course he decided to spice things up by using "funny naming conventions" while coding, and then tidying everything up before uploading the homework. This week he forgot, and fucked it big time.
So remember folks, always check your code before committing/giving it in/production. And always adhere to naming conventions.9 -
It's not that I hate PHP, I just hate the lack of consistency in standard function naming and parameter order, nonsensical attribute access, nearly-meaningless comparison operators, reference handling, case (in)sensitivities, and more!
I mean, look at these functions:
strtoupper(...)
bin2hex(...)
strtolower(...)
And look at THESE FUNCTIONS:
array_search($needle, $haystack)
strpos($haystack, $needle)
array_filter($array, $callable)
array_map($callback, $array)
array_walk($array, $callable)
And let me jUST USE SOME ATTRIBUTES:
$object->attr = "No dollar sign...";
Class::$attr = "GOD WHY";
HOW ABOUT SOME COMPARISONS:
(NULL == 0) // true
(NULL < -1) // ALSO true
Functions AREN'T CASE SENSITIVE (at least variables are).
Wanna dereference? TOO BAD, YOU'LL HAVE TO GET OUT THE TNT.
Alright, yeah, I hate PHP.18 -
Okay, story time.
Back during 2016, I decided to do a little experiment to test the viability of multithreading in a JavaScript server stack, and I'm not talking about the Node.js way of queuing I/O on background threads, or about WebWorkers that box and convert your arguments to JSON and back during a simple call across two JS contexts.
I'm talking about JavaScript code running concurrently on all cores. I'm talking about replacing the god-awful single-threaded event loop of ECMAScript – the biggest bottleneck in software history – with an honest-to-god, lock-free thread-pool scheduler that executes JS code in parallel, on all cores.
I'm talking about concurrent access to shared mutable state – a big, rightfully-hated mess when done badly – in JavaScript.
This rant is about the many mistakes I made at the time, specifically the biggest – but not the first – of which: publishing some preliminary results very early on.
Every time I showed my work to a JavaScript developer, I'd get negative feedback. Like, unjustified hatred and immediate denial, or outright rejection of the entire concept. Some were even adamantly trying to discourage me from this project.
So I posted a sarcastic question to the Software Engineering Stack Exchange, which was originally worded differently to reflect my frustration, but was later edited by mods to be more serious.
You can see the responses for yourself here: https://goo.gl/poHKpK
Most of the serious answers were along the lines of "multithreading is hard". The top voted response started with this statement: "1) Multithreading is extremely hard, and unfortunately the way you've presented this idea so far implies you're severely underestimating how hard it is."
While I'll admit that my presentation was initially lacking, I later made an entire page to explain the synchronisation mechanism in place, and you can read more about it here, if you're interested:
http://nexusjs.com/architecture/
But what really shocked me was that I had never understood the mindset that all the naysayers adopted until I read that response.
Because the bottom-line of that entire response is an argument: an argument against change.
The average JavaScript developer doesn't want a multithreaded server platform for JavaScript because it means a change of the status quo.
And this is exactly why I started this project. I wanted a highly performant JavaScript platform for servers that's more suitable for real-time applications like transcoding, video streaming, and machine learning.
Nexus does not and will not hold your hand. It will not repeat Node's mistakes and give you nice ways to shoot yourself in the foot later, like `process.on('uncaughtException', ...)` for a catch-all global error handling solution.
No, an uncaught exception will be dealt with like any other self-respecting language: by not ignoring the problem and pretending it doesn't exist. If you write bad code, your program will crash, and you can't rectify a bug in your code by ignoring its presence entirely and using duct tape to scrape something together.
Back on the topic of multithreading, though. Multithreading is known to be hard, that's true. But how do you deal with a difficult solution? You simplify it and break it down, not just disregard it completely; because multithreading has its great advantages, too.
Like, how about we talk performance?
How about distributed algorithms that don't waste 40% of their computing power on agent communication and pointless overhead (like the serialisation/deserialisation of messages across the execution boundary for every single call)?
How about vertical scaling without forking the entire address space (and thus multiplying your application's memory consumption by the number of cores you wish to use)?
How about utilising logical CPUs to the fullest extent, and allowing them to execute JavaScript? Something that isn't even possible with the current model implemented by Node?
Some will say that the performance gains aren't worth the risk. That the possibility of race conditions and deadlocks aren't worth it.
That's the point of cooperative multithreading. It is a way to smartly work around these issues.
If you use promises, they will execute in parallel, to the best of the scheduler's abilities, and if you chain them then they will run consecutively as planned according to their dependency graph.
If your code doesn't access global variables or shared closure variables, or your promises only deal with their provided inputs without side-effects, then no contention will *ever* occur.
If you only read and never modify globals, no contention will ever occur.
Are you seeing the same trend I'm seeing?
Good JavaScript programming practices miraculously coincide with the best practices of thread-safety.
When someone says we shouldn't use multithreading because it's hard, do you know what I like to say to that?
"To multithread, you need a pair."18 -
Opening somebody else's code(11000+ lines in 2 js files) only to find a 100+ "var a" declarations and naming conventions like var chart1, var chart2. Best part? Not a single comment. Even better? The one who wrote the code doesn't remember what does what.3
-
You know the code is in bad shape when you see variables starting with "get":
Integer getDataBlockSize;4 -
I have to rant a bit about the toxic reactions to a constructive Q&A website.
People keep complaining that they get downvotes and corrections, or stuff like that.
Are you fucking kidding me?
So you expect people to spend their own time for absolutely free, to help you, while you don't even want to invest in describing the issue you're having properly? And then complain that people are having issues in understanding your questions?
Let's look at this scientifically. Let's gather up some questions that have been received badly on SO in the last few hours. From the top (simply put https://stackoverflow.com/questions... in front of the id):
47619033 - person wants a discussion about an algorithm while not providing any information about what worked and what failed. "Please write a program for me". Breaking at least 2 rules.
47619027 - "check out my videos" spam
47619030 - "Here's the manual that has my answer but I can't find my answer in it".
47619004 - "how do I keep variables in memory"
47618997 - debug this exception, I'll give you no info on what I tried and failed. Screw this, you guys figure this out, I'm going out for beer.
47618993 - expects everyone to guess what the input is, what the expected output is, and whether he has read what HashMap is in the manual. But sure, this question is so far the best out of all the bad ones.
47618985 - please write code according to my specifications
Should I go on? There wasn't a single clear question about problems in code in this entire small set. Be free to continue searching, let me know if you find something that:
1. You understand what's being asked
2. Answer is clear and non-ambiguous (ex. NOT "which language is the coolest?")
3. Not asking someone to write a program for them.
4. Answer is not found in the most basic form of manuals (ex. php.net)
5. Is about programming.
The point is:
If you get downvoted on Stackoverflow - then you wrote a shitty question. Instead of coming over here and venting uselessly, simply address the concerns and at least TRY to write a clear question if you expect any answers.5 -
I've been lurking on devrant a while now, I figure it's time to add my first rant.
Little background and setting a frame of reference for the rant: I'm currently a software engineer in the bioinformatics field. I have a computer science background whereas a vast majority of those around me, especially other devs, are people with little to no formal computer background - mostly biology in some form or another. Now, this said, a lot of the other devs are excellent developers, but some are as bad as you could imagine.
I started at a new company in April. About a month after joining a dev who worked there left, and I inherited the pipeline he maintained. Primarily 3 perl scripts (yes, perl, welcome to bioinformatics, especially when it comes to legacy code like is seen in this pipeline) that mostly copied and generated some files and reports in different places. No biggie, until I really dove in.
This dev, which I barely feel he deserves to be called, is a biology major turned computer developer. He was hired at this company and learned to program on the job. That being said, I give him a bit of a pass as I'm sure he did not have had an adequate support structure to teach him any better, but still, some of this is BS.
One final note: not all of the code, especially a lot of the stupid logic, in this pipeline was developed by this other dev. A lot of it he adopted himself. However, he did nothing about it either, so I put fault on him.
Now, let's start.
1. perl - yay bioinformatics
2. Redundant code. Like, you literally copied 200+ lines of code into a function to change 3 lines in that code for a different condition, and added if(condition) {function();} else {existing code;}?? Seriously??
3. Whitesmiths indentation style.. why? Just, why? Fuck off with that. Where did you learn that and why do you insist on using it??
4. Mixing of whitesmiths and more common K&R indentation.
5. Fucked indentation. Code either not indented and even some code indented THE WRONG WAY
6. 10+ indentation levels. This, not "terrible" normally, but imagine this with the last 3 points. Cannot follow the code at freaking all.
7. Stupid logic. Like, for example, check if a string has a comma in it. If it does, split the string on the comma and push everything to an array. If not, just push the string to the array.... You, you know you can just split the string on the comma and push it, right?? If there is no comma it will be an array containing the original string.. Why the fuck did you think you needed to add a condition for that??
8. Functions that are called to set values in global variables, arrays, and hashes.. function has like 5 lines in it and is called in 2 locations. Just keep that code in place!
9. 50+ global variables/hashes/arrays in one of the scripts with no clear way to tell how/when values are set nor what they are used for.
10. Non-descriptive names for everything
11. Next to no comments in the code. What comments there are are barely useful.
12. No documentation
There's more, but this is all I can think to identify right now. All together these issues have made this pipeline the pinnacle of all the garbage that I've had to work on.
Attaching some screenshots of just a tiny fraction of the code to show some of the crap I'm talking about.6 -
I'm taking an Intro to Programming course along aside an Intro to Computers class so I already know about basic programing, still very new to it though! At the end of the Intro to Comp, we're learning about programming and a classmate was having a hard time understanding assignments and variables.
I explained the idea of the input command at least three times and he kept trying to print out a statement he just wanted to write in instead of printing out the input that the user will enter. He also assigned the same name to different variables.
Explained that what he was doing was not versatile and not useful, explained in an example situation, explained by writing some lines of code myself (THRICE), and he still had trouble understanding me. I didn't want to hold his hand the entire time.
Glad that I was called to leave early since I might get too frustrated if I had to stay back and continue to help him.
Hope he managed to finish the assignments successfully though! Feel kinda bad now...2 -
I'm working at this company where I have to update their app both for Android and iOS and it was originally coded by what seems to be one guy, that has written some of the worse code I've seen (I've seen pretty bad code when I was at uni), there is so much uncommented code, commented code with no real reason on why it's commented, variables that are one or two letters, Lots and Lots of magical numbers for things like images! And for the first few weeks working on the iOS app I was also still learning objective-c and had to look at his code for reference, I cringed so much.
I take pride in my commented code, I take pride in writing description for methods and having my variables at the top of a class and explain exactly why it's a constant. I'm also only just a recent graduate.
This guy that worked out this app is a senior developer, now working on security software for a bank, how is he even allowed to code?3 -
Ohh man i fucked up bad. 5 days as intern, and i fuck up really bad with my ego and ignorance.
I love my this company. A great environment, lots of people to learn from , i am given reasonable tasks and i feel happy to complete them. But what happened today was weird and fucked up.
I have never worked at a place with seniors designers tech leads and more people with positions. I have also worked with a lot of competitive people who are always in a race to be first.
And how do we come first? Have a lot of knowledge, hear the smallest of detail and sprint towards goal (because the combination your knowledge, assumptions and speed is enough to make you reach to the top). You don't ask for specific details, because they are obvious. And that's me in short.
Today i fucked up.
Mistake #1 ) first i was given a small task by my senior. It was a 20 mins task max if i had done it the normal noobie way . But i am a pro in mind , i have to do it with all the architecture , even if i don't understand why. So i asked for 50 mins. They gave it and did not had a problem with my time, but with the way i wrote my code.
He was like "who told you to make it like this ? Why did you made it like this?" And was visibly irritated. And i was like super chill saying "i don't know the why, but i know its correct way of using it" , pissing him even more. In my eyes he's just a super friendly sr, more like a bro and wouldn't mind some cheeky answers. And he didnt show any
consequences for that time.
Mistake #2 this is super fucked up. Our office is going under some renovation & interns were asked to sit in the co-working spaces (outside of the office). It was already very disturbing and i had to go to office every few minutes.
So after lunch this happens : We are working on a new module that already has a tonne of screens and logics. I have made a small part which is from the middle and now we can go both in the forward or in the backward direction.(Also, its quite a new module whose idea was recently discussed and decided. And weirdly i am also being treated like a core member as the ceo once himself asked what would he my flow for doing things in this. i am in direct contact and under direction of backend , designers , ceo and My senior and many ppl are giving me tasks ) And... Aagh fuck it. .. its a long story and i don't feel like repeating it but
inshort :
got a task,
didn't understood it completely and thought its my task to figure it out, took a long time figuring it my self ,
techlead/designer somehow changed my and my sr. direction of flow even tho we were taking a different approach
I sit in a noisy and irritating place
Techlead/designer comes during the time when i am figuring out the solution(already overtime the one in point #2) nags for result.
I get in an argument with him, justifying for my time and arguing that it's difficult to think technical logics for that design
( truth be told, it WAS a difficult logic which he thought was too easy. It consisted of 3 variables and 8 states we were doing different works for 4 of them and rejecting 2 and ... I don't know, i had got that wrong . But that shouldn't had been my problem to solve. I should have gone to my senior and didn't get into argument with tech lead ). It think i might have offended him too.
After he left, i am so angry on him that after sometime my senior comes and i misbehave with him. He just asks to meet me before i go, and i do so. During the meeting we discuss this whole fuck up and how many times i showed him my ego and indiscipline. And then i realise what a fuckup i did due to my ego and lack of asking, blindly following my own over confidence and blindly following or arguing with others.
Fuck fuck fuck6 -
Reviewed some Unity game code yesterday
[HideInInspector] public NavMeshAgent agent;
Me:”why is this hidden if it’s public”
Dev: “so designers don’t fuck it up”
Me: “then why have it public”
Dev: “I need it to be set by another class”
Me: “then make it a private bar and create a get:set function”
Dev: “Why?”
Me: “Because hiding a public variables from designers is a bad model and by standards things now to be shown to the inspector should be private”
This shit is why I have no confidence in devs my age10 -
We learned Java in school this year. Everyone who did not comply to the coding style defined in some stone-age books, got a bad grade, including me, who rather used a "normal" style everyone uses. They thought us this:
CLASSNAMES, Constants, MethodNames, VariableNames, etc.
Worse than that, they used german names for pretty much everything including classes, variables and methods.9 -
I guess most of the things I do are a bad dev habit?
From not commenting stuff to commenting in German to copying stuff without looking at it, procrastinating a lot, not starting at all, bad naming of variables, bad... Everything? Idk, I have a lot to learn4 -
So in Germany we have something like 'cooperative study'. You are employed in a company and study 'normal' at a university. This is in 3 month phases, i.e. 3 months working, 3 months studying.
At the moment I'm working and there is a colleauge, that seems to have no high confidence in my programming skills.
Today I saw parts of his NodeJS code and I thought I'm going crazy.
No comments, no real usage of callbacks or at least promises and I dont want to talk about naming of the variables.
I caught myself arguing with this guy too often and always thought I'm the stupid one, that doesn't understand him.
But I'm starting to think, He is the one that is hard to understand.
How ever, I stay confident and also keep a nice tone (also help as much as I can) and sometimes we also have the same thoughts in some topicd. It's not that bad, but sometimes I feel underestimated.
But hey, so it's a bigger surprise if I'm presenting my results and show them what I'm able to do 👍🏻2 -
You know what really grinds my gears? When people criticize a programming language but uses edge cases and stuff that can be avoided by using the tried and true "don't be an idiot". Take for instance JavaScript, a language I like and a language that has a lot you can criticize. But I feel like a lot of peoples criticism isn't warranted.
What's that? No ints? Use parseInt or Math.floor.
What are you saying? == works in strange ways? Yes, that's what we have === for.
Type coercion is wonky? Think it's weird how string + int works differently than string - int? Wanna string with number + - + - - + - - etc? Don't! Don't add strings and ints, don't subtract strings and ints. You can't in statically typed languages and you aren't supposed to in dynamically typed
Adding arrays and objects, arrays and arrays, objects and objects etc. is inconsistent? Why are you trying to do that?
Adding floats together gives odd results? Now we're getting somewhere! And Mozilla responded to that with a method called toFixed.
Declaring variables with var doesn't always work that well? Use let and const
Then there's this weird attitude that some people I've met have, where they will complain about the module system and how "well you rely on the community for those packages" as if it's a bad thing. And then coming with the "well you don't know what the (open source) packages do internally" as if I (for the most part) give a shit. Then they'll swear by companies like Zend or Microsoft as if they can't just stop supporting the languages they use. Maybe it's just because I like community content more because of video game mods.
Wanna criticize JS, then there's plenty to talk about. Like the built in date object is basically shit. Or how in NodeJS you can have node_modules in your node_modules. Or how classes don't really have the best syntax. Left-Pad. And so on (it's too late for me to be able to remember much more).1 -
First time I was screaming out of anger while looking at code.
I'm doing a group project in my university.
We are developing a indoor navigation Android app.
And a team mate of mine just merged this…
/*Method for help-feature.
Sets all the TouchEvents that are at least 400 ms long. This is made for all the relevant buttons or editTexts, which are seen on the mapView.
The case for mapView is needed because otherwise the other buttons, etc. wont work properly.*/
public void setButtonsForHelpDialog(){
View v = mapView;
switch (v.getId()) {
case R.id.mapview:
mapView.setOnTouchListener(…);
case R.id.buttonUp:
buttonOn.setOnTouchListener(…);
case R.id.buttonDown:
buttonDown.setOnTouchListener(…);
…
case R.id.description:
description.setOnTouchListener(…);
}}
The code is really aligned like this - no breaks. And it's even worse. There are if statements like if("constantly false var" == true). Which is highlighted by Android Studio.
This is done in a own class. The views are set via public member variables of this new class. The constant vars were added in the actual class holding the buttons and also stuff like this useless method
public void getDoStuff() {
doStuff()
}
And I could continue like this.
I never saw code this bad…
I can't even find words for it :/4 -
I have a guy sitting next to me in class. We were working on the same project. It's about rewriting a functioning mergesort algorithm in C and doing a presentation about that topic.
Now... the thing is that I was ill on that specific day when we got that project assigned. And he didn't tell me it either. I asked the whole class.
They just said that there was nothing special about that day. These fuckers.
Anyway...
Thé following week we had the same lesson again. Actually there were more than both of us. We were a group of 5 dudes.
3 of them barely have anything to do with programming at all. They just learn for the exams and have bad grades in programming.
Luckily, they already wrote the functioning sorting algorithm.
Since that is the case, I chose to review it to get deeper into that topic.
There were comments in English (we live in Germany) and these comments were written in a different style. My classmates would never comment in such a way.
It was a modified version copied from the internet. The whole source code.
The variables had names like j,k,b,u and so on. It was perfectly obfuscated.
Yesterday, I wasn't at college either.
I had to show up to a given time at a government bureau. They have been working on that project that day. So, I decided to ask them via a messenger, if they can give me the newest presentation files after 1 pm.
They said that they barely have anything to present. They would like to improvise they said.
"Fuck you all" I thought.
I'm done with these fucking illiterate humans.
I hope they all die in hell with satan having a ride on them. Stabbing them from behind right into their assholes and eating their ball sacks (if they have any).
Today is the presentation.
That's when I decided not to drive there during these specific lessons.1 -
When I was in first year, I let my classmate copy my source code for our VB program in order for him to save the semester. We both agreed that he should change the variables, etc first before submitting the project.
GOOD NEWS: He literally changed the variables.
BAD NEWS: We had the same interface on our project.
It sometimes haunts me, until today.3 -
(first post/rant on here)
So I recently started at a new company. I was kinda aware that the project I'm working on would be rather old school (to put it in a nice way :-)).
Part of my job is to 'industrialize' and update/clean up the existing code so there is less time spent on fixing bugs due to bad design.
One of the first things I had to do was to write a new interface to integrate with external software.
I already noticed some rather nasty habits, like prefixing every variable with m (don't know why), private fields for every property (all simple properties) and a whole lot of other stuff that either is obsolete or just bad practice.
Started writing clean code (simple classes with properties only, no m prefixing, making sure everything is single responsibility, unit tests, ...).
So I check in the code, don't hear much from it again besides the original dev/architect that started the project using my code to further work on that integration.
Now recently I started converting everything from TFVC to Git (which is the company standard but wasn't used by our team yet). And I quickly skimmed through my code to check if everything was there before pushing it to the remote repo.
To my surprise, all the code I had written was replaced by m prefixed private variables used in simple properties. BL classes were thrown in together, creating giant monstrosities that did everything. And last but not least, all unit tests were commented out.
Not sure what I got myself into ... but the facepalming has commenced.14 -
// My First Rant
We have a developer that almost everyone adjust to what he want to avoid talking or working with him.
I have office mates that doesn't want to give tasks to him just to avoid working with him.
Even our devOps guy just did what he want so he would stop talking.
One bad experience of our devOps guy with him is that his infrastructure or other AWS stuff was blame why his APIs is not working. It turns our that his url for the database has FUCKING SPACES.
Not sure if a good practice but he wants the base url of our Endpoint to be set in environment variables instead of having DEV/PROD/TESTING and base the endpoint from there.
He said that he was given permission to study a language but he doesn't even ask for permission.3 -
I started learning Golang today and really like it.
The error handling is *excellent*. It always works the same way and is standardized, unlike the hell that NodeJS error handling is (.catch(), try).
Modules confused the fuck out of me. I eventually figured out how they worked, but Go really doesn't try to make it easy to have multiple source folders...
I'll probably be re-writing my Discord Bot in Golang soon. Being able to have just one binary output will make things infinitely easier. Compile-time variables are another feature that's nice and easy to implement.
The goal is only having to upload a single binary to deploy on production from my CI script that has all keys and stuff inside. Feels good to finally throw all that old bad JS code out and starting completely fresh.7 -
I legit had an interview once where he said
"Ok next question. Static variables...are they good or are they bad"
The funny thing is I can totally relate to working with someone who thinks static variables are bad but it's sad to see this is how far we have fallen.7 -
5 years ago in Texas. There is shit code and then there's SHIT code. I know I can produce bad code and often do, but that codebase was an eldritch abomination.
Just to give you a tiny taste, variables in JS would usually be called "that" because "when calling them, I send the 'this' value right? So in the other function it is 'that'". I wish I was making this up. And this is nothing compared to everything else.12 -
Fuck this I need to ventilate.
Thinking about job change because maintaining and extending 3 years old codebase (flask project) is FUCKIN exhausting. It was badly written since start by someone who obviously didn't know much about python. (Going by commit history.)
Examples:
- if var != None / if var == None
- if var is not None / if var is None (well..)
- Returning self-parsed obscure JSONs from dict variable
- Serializing dictionaries into database by str() (both sqlalchemy and mysql support JSON format) - THEY ARE ALMOST UNUSABLE OTHER WAY AROUND (luckily, python can deal even with that)
- celery tasks, the way they are called they BLOCK the whole flask (not bad in itself, but if connection breaks there are no errors, nothing it just hangs)
- obscure generator/yielding that contains return of flask's response in itself
- creating fifteen thousands of variables one by one where they would look so nicely as dict keys, and hey they are then both MANUALLY SERIALIZED into returning dict by "%s" (string formatting) [okey, some of them are objecst like datetime but MATE WTF]
- many, many more, PEP lint shall not pass
I would rather deal with fresh startup owners wanting me to program unicorns in one week then trying to extend and manage zombie-like projects.
Nothing personal against the firm I actually like the place.3 -
This Safari Bug is sooo bad, really hard to find it. (It initializes new variables with the element where id and variable name is the same, why?)10
-
It took literally days to get our software installed onto the client VMs and get the services started correctly.
On our own test VMs the same task takes all of about an hour or so. Mind you these VMs are supposed to be created and match the client's environment almost too the T. Same configurations, same third party software, same environment variables and the whole 9 yards.
This was not the case at all.
Environment variables were not set, third party software was not installed, and I honestly don't remember the list of things wrong with how they setup the VM. Sparing the details, the errors were also not helpful.
They also gave us critical information we needed for development days before we were going on site to test. The amount of hackery we had to do could be a completely separate rant on its own.
While desperately trying to to stay sane long enough to get our services started, the only thing I could think was how great it would be if there was a fire or something. Anything, just to have an excuse to go back to the hotel and actually sleep. The second day there we finally had everything installed and running.
I shit you not, just as we began our first test, the fire alarms went off.
At this point in time the team was extremely (pissed tf off) annoyed to put it mildly. Assuming it was just a drill, we left our stuff and went to eat dinner. After we came back we found out it in fact was not a drill...
Moral of the story:
Don't wish bad fortune on a customer even if out of impulsive spite.
Other moral of the story:
Don't leave your belongings behind only because you think the fire alarms are just a fire drill. It may not be.
P.S. Karma's a bitch.1 -
Biggest sin
Due to lack of time, I named all the variables in my project without logic,
Like temp1,str1, function dojson etc
Lord be with the dev who's gonna work on that project
PS I am not a bad person, it is the time that made do such things -
Imagine a time when a colleague contributes a shitty spaghetti of non-optimized code that neither use mnemonic variables nor conventional naming of functions, and you can imagine the dark hours of maintaining it and your fingers itch to fix it but you don't have the time and the responsibility too to do it. He doesn't listen to you and you feel bad to tell this to the boss as the colleague is also a friend you've known since college and is a good person otherwise. No options seems to give peace.6
-
Global variables destroyed my day
Of course you can call me a bad programmer and all. When you have the idea, sometimes globals make it seem easy, accessible and "saving resources". They are devils.
The app was connected to a suite of applications. So I ended up silently destroying it neighbors. I committed and pushed the shit. Just when the testers made their weed high smoke tests, a server stopped working.
I got an email that boss wanted the latest version, I reverted the wrong branch, which had code unrelated to its name, pushed shit again and voila.
I went to the bathroom and laughed. I had to take a smoke. I'm still laughing while typing this. The damage is too much and I can't help it. I'll go home, leave this pc on and work remotely through the night. It might be the hysterics speaking now, but I messed up, and I need magic by friday morning.6 -
I'm trying to build VoIP into my browser-based game, and holy shit are sound processing people bad at explaining stuff.
Every stackoverflow answer has badly named variables, noone names the algorithms they're using (which makes research near impossible), and literally every single Web Audio API pipeline I have seen so far contains at least one unexplained effect with no parameters, but it's a different effect each time.
One guy had implemented some kind of smoothing for catching up with the stream after interruptions (where the playback speed is proportional to how far we're behind the intended latency), without ever mentioning it anywhere. And this is meant to be a basic example!4 -
Client wants some CMS text to be automatically translated. So I checked and Google seems to have a solution for that. I thought to to be as simpel as doing a request and parsing the response. That's how API's work, right?
No. First I must create an account, that account must have a credit card, then I need to setup credentials, the default ones working with path variables, an API key... etc etc etc.
I feel so stupid for just not understanding their docs. I'm just a dude that installs a CMS and makes pretty CSS for it. I've worked with REST APIs before (Mollie, Carerix) but none of them ever demanded the level of knowledge and setup the Google Translate API demands.
Am I just a bad developer or is this shit just too complex for your average web developer?9 -
I’m currently working with a devops team in the company to migrate our old ass jboss servers architecture to kubernetes.
They’ve been working in this for about a year now, and it was supposed to be delivered a few months back, no one knew what’s going on and last week they manage to have something to see at least.
I’ve never seen anything so bad in my short life as a developer, at the point that the main devops guy can’t even understand his own documentation to add ci/cd to a project.
It goes from trigger manually pipelines in multiple branches for configuration and secrets, a million unnecessary env variables to set, to docker images lacking almost all requisites necessary to run the apps.
You can clearly see the dude goes around internet copy pasting stuff without actually understanding what going on behind as every time you ask him for the guts of the architecture he changes the topic.
And the worst of all this, as my team is their counterpart on development we’ve fighting for weeks to make them understand that is impossible the proceed with this process with over 100 apps and 50+ developers.
Long story short, last two weeks I’ve been fixing the “dev ops” guy mess in terms of processes and documentation but I think this is gonna end really bad, not to sound cocky or anything but developers level is really low, add docker and k8s in top of that and you have a recipe for disaster.
Still enjoying as I have no fault there, and dude got busted.9 -
I was asked to make proof of concept small frontend app with some simplified requirements, they asked me because it should be written in the stack I done most of my career work with. I do it in 3 days instead of 5, using those 2 days to optimise the app and explore different approaches. I noted down my findings, what to avoid and reasons and also what is good to use and reasons and shared with everyone.
We waited for the project to start, I started working on another project in the meantime and there was a big rush to make project go live etc., so I was consumed 100% on that new project.
So they put in charge backend php developer to do frontend js work. I said ok, do you need help in starting out? Nah, my proof of concept repo is enough.
4 days before that small project goes live they asked me to do code review. All things I noted down to avoid are in the codebase, few bad practices but everything is over-engineered (in a very bad way), some parts should be more flexible as current setup is very rigid, having almost all kinds of CSS, I saw SASS, CSS variables, 2 different CSS-in-JS tools with some additional libraries that is used to toggle classes.
I don't know how to approach this as I am not asshole as a person and I don't want to say to my colleague that his codebase is completely trash, but it is.
The worst parts: They called me to help finish the app and budget is almost spent!
I would rewrite the whole app as the state of the current app is unusable and everything is glued with bad Chinese ducktape that barely holds.
Additional points because it won't bundle as everything is f**ked.
I am seriously thinking of duplicating master branch and refactor the whole fricking app but won't do that as I am burning midnight oil on other two projects. Don't worry overtimes are paid.
I hate those shitty situations, this project was supposed to be tiny, sweet and example of decent project in this company but it is instead big fat franken-app that will be example how smart it is to avoid putting backend dev to do frontend work (I also agree for vice versa)! -
AI is dumb and is not going to rob your work as a programmer.
Expanding on this:
https://devrant.com/rants/12459112/...
Don't know about the others, but programming and IT is mostly safe unless you're a secretary answering to mails pressing 1 keystroke at time with index finger.
Bullshit.
I’ve tried EVERYTHING. As a developer, I know exactly what instructions to give and how to explain them. I tried this stuff for years.
I abandoned the idea to give Ai a full blown workspace to vscode with copilot, even with experimental LLMS (Claude 3.5, Gpt4o, o1, as per my linked post, copilot is dumb as a rock), because it fucks up every fucking time so bad.
I tried getting an AI to build a simple project, something that has plenty of samples of code around, something that I was sure it could have been in its training dataset. A copy of Arkanoid, in HTML/CSS/JS, even reformulating the prompts over and over with different LLMs that claim to have reasoning abilities. I provided detailed feedback step by step, pointed out the errors, improvements, and problems in-depth to: o3, o1, 4o, deepseekv3+R1, and Qwen 2.5 Ultra. I even activated web search and suggested scanning GitHub repos when necessary. I gave examples of code after several failed attempts.
And guess what? Nothing. A total mess. Half the time, the game didn’t even run, and when it did, everything was wrong—bricks overlapping, barely anything working the way I asked. Even though the internet is full of similar code, and I gave it part of the solution myself when it couldn’t figure it out.
Don’t worry, AI isn’t going to steal your job—it’s just a broken toy. Fine for repetitive, simple tasks, but nothing more.
It's years that they make hyped up bold statements that the next model will revolutionize everything and it's years that I get delusional results.
It's just good at replacing some junior bovine work like mapping some classes or writing some loops with not too much variables and logics involved.
Sigh. My error was getting too comfortable using it and trusting/hoping that this ramp up in AI developement would have brought an easier life to dev.
Silly mistake.7 -
I'm currently between jobs and have a few rants about my previous job (naturally). In retrospect, it's somewhat therapeutic to range about the sheer brainfuckery that has taken place. Enjoy!
First, let me set the scene: legacy B2B web app made with LEMP stack and sencha ext.js 3 + 4 (don't ask) and a lot of madness. Let's call that app "Alpha".
Alpha is a self made CMS build for typical ERP stuff. Yes, a self made CMS: entities are containers, containers have types and fields and values. Like so many legacy PHP apps, it does not have a dedicated FE: the HTML is rendered on the server and then spewed out to the browser.
Easy right? Coding like it's 1999! But there was a twist: Because everything is basically a container, the HTML-templates are saved in the DB. Along with the nessary JS and the CSS. And the translation variables. Why? Because fuck you! That's why. Who needs a git history anyways.
For some reason, Alpha was kinda slow.
There was also an editor, that allowed you to modify templates (web, mail, pdf) on the fly in prod. Because templates contain repeating data (header/footer), one template could contain additional templates. Much confusion. You could change templates via migration (slow, boring) or just ctrl-c/ctrl-v that sucker (fast, much excitement).
Did I mention Alpha was slow?
On with the rant: e-mails! How do they work? Noone knows. How to send mails asynchronous in PHP? Witchcraft is the only possible answer to that riddle. Here is your enterprise™ solution:
1. create mail
2. insert mail into DB
3. WAIT UP TO 59 SECONDS FOR A FUCKING CRON TO SEND MAIL
Why? "Because that way, we can resend mails in case the network is down :)"
Same procedure for the SOAP-API (db-queue + cron). You read that right: all requests to various other systems are processed once a minute.
Alpha slow.
Alpha was only one of several systems. Imagine a bunch of monolithic php apps, interconnected via SOAP, REST and GraphQL like a godamn intergalactic orgy. Image having to debug that cluster fuck.
Let's say there is a bad request. These things happen. No biggie. Remember the db-queue? Let's try to send the bad request a second time! And a third time! Still no luck? How odd. Let's create a specific file in a specific directory: a LOCK-file. Now, "the db-queue is on hold and no request gets processed :)"
Golly gee thanks Alpha.
Anyhow, did you know that MySQL has a join limit of 61 tables?3 -
I need some opinions on Rx and MVVM. Its being done in iOS, but I think its fairly general programming question.
The small team I joined is using Rx (I've never used it before) and I'm trying to learn and catch up to them. Looking at the code, I think there are thousands of lines of over-engineered code that could be done so much simpler. From a non Rx point of view, I think we are following some bad practises, from an Rx point of view the guys are saying this is what Rx needs to be. I'm trying to discuss this with them, but they are shooting me down saying I just don't know enough about Rx. Maybe thats true, maybe I just don't get it, but they aren't exactly explaining it, just telling me i'm wrong and they are right. I need another set of eyes on this to see if it is just me.
One of the main points is that there are many places where network errors shouldn't complete the observable (i.e. can't call onError), I understand this concept. I read a response from the RxSwift maintainers that said the way to handle this was to wrap your response type in a class with a generic type (e.g. Result<T>) that contained a property to denote a success or error and maybe an error message. This way errors (such as incorrect password) won't cause it to complete, everything goes through onNext and users can retry / go again, makes sense.
The guys are saying that this breaks Rx principals and MVVM. Instead we need separate observables for every type of response. So we have viewModels that contain:
- isSuccessObservable
- isErrorObservable
- isLoadingObservable
- isRefreshingObservable
- etc. (some have close to 10 different observables)
To me this is overkill to have so many streams all frequently only ever delivering 1 or none messages. I would have aimed for 1 observable, that returns an object holding properties for each of these things, and sending several messages. Is that not what streams are suppose to do? Then the local code can use filters as part of the subscriptions. The major benefit of having 1 is that it becomes easier to make it generic and abstract away, which brings us to point 2.
Currently, due to each viewModel having different numbers of observables and methods of different names (but effectively doing the same thing) the guys create a new custom protocol (equivalent of a java interface) for each viewModel with its N observables. The viewModel creates local variables of PublishSubject, BehavorSubject, Driver etc. Then it implements the procotol / interface and casts all the local's back as observables. e.g.
protocol CarViewModelType {
isSuccessObservable: Observable<Car>
isErrorObservable: Observable<String>
isLoadingObservable: Observable<Void>
}
class CarViewModel {
isSuccessSubject: PublishSubject<Car>
isErrorSubject: PublishSubject<String>
isLoadingSubject: PublishSubject<Void>
// other stuff
}
extension CarViewModel: CarViewModelType {
isSuccessObservable {
return isSuccessSubject.asObservable()
}
isErrorObservable {
return isSuccessSubject.asObservable()
}
isLoadingObservable {
return isSuccessSubject.asObservable()
}
}
This has to be created by hand, for every viewModel, of which there is one for every screen and there is 40+ screens. This same structure is copy / pasted into every viewModel. As mentioned above I would like to make this all generic. Have a generic protocol for all viewModels to define 1 Observable, 1 local variable of generic type and handle the cast back automatically. The method to trigger all the business logic could also have its name standardised ("load", "fetch", "processData" etc.). Maybe we could also figure out a few other bits too. This would remove a lot of code, as well as making the code more readable (less messy), and make unit testing much easier. While it could never do everything automatically we could test the basic responses of each viewModel and have at least some testing done by default and not have everything be very boilerplate-y and copy / paste nature.
The guys think that subscribing to isSuccess and / or isError is perfect Rx + MVVM. But for some reason subscribing to status.filter(success) or status.filter(!success) is a sin of unimaginable proportions. Also the idea of multiple buttons and events all "reacting" to the same method named e.g. "load", is bad Rx (why if they all need to do the same thing?)
My thoughts on this are:
- To me its indentical in meaning and architecture, one way is just significantly less code.
- Lets say I agree its not textbook, is it not worth bending the rules to reduce code.
- We are already breaking the rules of MVVM to introduce coordinators (which I hate, as they are adding even more unnecessary code), so why is breaking it to reduce code such a no no.
Any thoughts on the above? Am I way off the mark or is this classic Rx?16 -
Got one right now, no idea if it’s the “most” unrealistic, because I’ve been doing this for a while now.
Until recently, I was rewriting a very old, very brittle legacy codebase - we’re talking garbage code from two generations of complete dumbfucks, and hands down the most awful codebase I’ve ever seen. The code itself is quite difficult to describe without seeing it for yourself, but it was written over a period of about a decade by a certifiably insane person, and then maintained and arguably made much worse by a try-hard moron whose only success was making things exponentially harder for his successor to comprehend and maintain. No documentation whatsoever either. One small example of just how fucking stupid these guys were - every function is wrapped in a try catch with an empty catch, variables are declared and redeclared ten times, but never used. Hard coded credentials, hard coded widths and sizes, weird shit like the entire application 500ing if you move a button to another part of the page, or change its width by a pixel, unsanitized inputs, you name it, if it’s a textbook fuck up, it’s in there, and then some.
Because the code is so damn old as well (MySQL 8.0, C#4, and ASP.NET 3), and utterly eschews the vaguest tenets of structured, organized programming - I decided after a month of a disproportionate effort:success ratio, to just extract the SQL queries, sanitize them, and create a new back end and front end that would jointly get things where they need to be, and most importantly, make the application secure, stable, and maintainable. I’m the only developer, but one of the senior employees wrote most of the SQL queries, so I asked for his help in extracting them, to save time. He basically refused, and then told me to make my peace with God if I missed that deadline. Very helpful.
I was making really good time on it too, nearly complete after 60 days of working on it, along with supporting and maintaining the dumpster fire that is the legacy application. Suddenly my phone rings, and I’m told that management wants me to implement a payment processing feature on the site, and because I’ve been so effective at fixing problems thus far, they want to see it inside of a week. I am surprised, because I’ve been regularly communicating my progress and immediate focus to management, so I explain that I might be able to ship the feature by end of Q1, because rather than shoehorn the processor onto the decrepit piece of shit legacy app, it would be far better to just include it in the replacement. I add that PCI compliance is another matter that we must account for, and so there’s not a great chance of shipping this in a week. They tell me that I have a month to do it…and then the Marketing person asks to see my progress and ends up bitching about everything, despite the front end being a pixel perfect reproduction. Despite my making everything mobile responsive, iframe free, secure and encrypted, fast, and void of unpredictable behaviors. I tell her that this is what I was asked to do, and that there should have been no surprises at all, especially since I’ve been sending out weekly updates via email. I guess it needed more suck? But either way, fuck me and my two months of hard work. I mean really, no ego, I made a true enterprise grade app for them.
Short version, I stopped working on the rebuild, and I’m nearly done writing the payment processor as a microservice that I’ll just embed as an iframe, since the legacy build is full of those anyway, and I’m being asked to make bricks without straw. I’m probably glossing over a lot of finer points here too, just because it’s been such an epic of disappointment. The deadline is coming up, and I’m definitely going to make it, now that I have accordingly reduced the scope of work, but this whole thing has just totally pissed me off, and left a bad taste about the organization.9 -
Today, I had a small, but funny conversation with a person I knew from my education (application developing).
He suddenly asked, how to prevent using HTML-Tags in PHP.
So I send ihm following line:
$string = str_replace(array("<", ">"), array("<", ">"), $string);
Shortly after the line, he asked, how to add this into his query, which looks like:
$query = "INSERT INTO comments (name, email, quote, hinzugefuegt, ip_adress) VALUES ('" . $_POST['vName'] . "', '" . $_POST['eMail'] . "', '" . $_POST['q17'] . "', NOW(), '" . $_SERVER['REMOTE_ADDR'] . "')";
Now I thought: "Well, he don't even secure his variables", and I posted a Pastebin, which only "fixes" his issue with replacing the HTML-Tags, but still allows SQL injection.
https://pastebin.com/kfXGje4h
Maybe I'm a bad person, but he doesn't deserve it otherwise, because when I was still in education with him, I told him, he should learn to use prepared statements.3 -
So while exploring some new ideas, I decided to figure out if I could use variables in the known set to determine the bounds of variables in the unknown set.
The variables in question are algebraic identities derived from the semiprimes, so you already know where this is going.
The existing known set is 1194 identities.
And there are, if I recall, roughly two dozen unknowns.
Many knowns have the unknowns as their factors. The d4 product set for example is composed of variables d4a, d4u, d4z, d4z9, d4z4, d4alpha, d4theta, d4omega, etc.
The component variables themselves are unknown, just their products are known. Anyway.
What I've found interesting is if you know the minimum of some of these subsets, for example d4z is smallest out of the d4's for some semiprimes, then you know the upperbound of both the component variables d4 and z.
Unless of course either of them is < 1.
So the order of these variables, based on value, changes depending on the properties of the semiprime, which I won't get into. Most of the time the order change is minor, but for some variables they can vary a lot between semiprimes, rapidly shifting their rank in the known set. This makes it hard to do anything with them.
And what I found myself asking, over and over again, was if there was a way to lock them down? Think of it like a giant switch board, where flipping one switch lights up N number of others, apparently at random. But flipping some other switch completely alters how that first switch works and what lights it seemingly interacts with. And you have a board of them thats 1194^2 in total. So what do you do?
I'd had a similar notion a while back, where I would measure relative value in the known set, among a bunch of variables, assign a letter if the conditions were present, and generate a string, called a "haplotype."
It was hap hazard and I wrote a lot of code to do filtering, sorting, and set manipulation to find sets of elements in common, unique elements, etc. But the 'type' strings, a jumble of random letters, were only useful say, forty percent of the time. For example if a semiprime had a particular type starting with a certain series of letters, 40% of the time a certain known variable was guaranteed to be above a certain variable from the unknown set...40%~ of the time.
It was a lost cause it seemed.
But I returned to the idea recently and revamped the entire notion.
Instead what I would approach it from a more complete angle.
I'd take two known variables J and K, one would be called the indicator, and the other would be the 'target'.
Two other variables would be the 'component' variables (an element taken from the unknown set), and the constraint variable (could be from either the known or unknown set).
The idea was that relationships between the KNOWN variables (an indicator and a target variable) could be used to indicate the rank relationship between the unknown component variable and the constraint variable.
You'd think this wouldn't work either, but my intuition was there were so many seemingly 'random' rank changes of variables in the known set for any two semiprimes, that 1. no two semiprimes ever shared the same order for every variable, and 2. the order of the known variables had to be leaking information about the relationships of the unknown variables.
It turns out my intuition was correct.
Imagine you are picking a lock, and by knowing the order and position of the first two pins, you are able to deduce the relative position of two pins further back that you can't reach because of the locks security features. It doesn't let you unlock the lock directly, but by knowing this, if you can get past the lock's security features, you have a chance of using information about the third pin to get a better, if incomplete, understanding about the boundary position of the last pin.
I would initiate a big scoring list, one for each known element or identity. And then I would check it in tandem like so:
if component > constraint and indicator > target:
indicator[j]+= 1
This is a simplication, but the idea was to score ALL such combination of relationship, whether the indicator was greater than the target at the same time a component was greater than a constraint, or the opposite.
This worked out to four if checks and four separate score lists.
And by subtracting one scorelist from another, I could check for variables that were a bad fit: they'd have equal probability of scoring for example, where they were greater than the target one time, and then lesser than it for another semiprime.
So for any given relationship, greater or lesser between any unknown variable and constraint variable, I could find any indicator variable and target variable whose relationship strongly correlated to the unknown's.15 -
!RANT
Oh, the SORROW that is JEST! 😡
Endless days have been swallowed by the abyss in my quest to configure Jest with TypeScript and ECMAScript modules instead of CommonJS. Triumph seemed within my grasp until - BAM! - suddenly the tool forgets what "import" or "export" means. And the kicker? On the CI, it still runs like nothing’s amiss!
Allow me to elucidate for the uninitiated: Jest is supposed to be a testing safeguard, a protective barrier insulating devs from the errors of their peers, ensuring a smooth, uninterrupted coding experience.
But OH, how the tables have turned when the very shield becomes the sword, stabbing me with countless, infuriating errors birthed from Jest’s own design decisions!
The audacity to reinvent the whole module loading process just to facilitate module mocking is mind-boggling! Imagine constructing an entirely new ecosystem just to allow people to pretend modules are something they're not. This is not just overkill; it's a preposterous reinvention of a wheel that insists on being a pentagon!
Sure, if devs want to globally expose their variables, entwining everything in a static context, so be it. BUT, why should we, who walk the righteous path of dependency injection, be subjugated to this configured chaos?!
My blood boils as the jestering Jest thrusts upon me a fragile, perpetually breaking system, punishing ME for its determination to support whole module mocking! A technique, mind you, that I wouldn’t touch with a ten-foot pole, because, you know, DEPENDENCY INJECTION!
Where are the alternatives, you ask? Drowned in the abyss, it seems! Why can’t we embrace snapshots and all the delectable integrations WITHOUT being dragged through this module-mocking mire? Can’t module mocking just be a friendly sidekick, an OPTIONAL add-on, rather than the cruel dictator forcing its agenda upon our code?
Punish those clinging to their static contexts, their global variables – NOT those of us advocating for cleaner, more stable practices!
It’s high time we decouple the goodness of Jest from its built-in bad practices. Must we continue to dance with the devil to delight in the depth of Jest’s capabilities?
WHY, Jest, WHY?! 😭9 -
After learning a bit about alife I was able to write
another one. It took some false starts
to understand the problem, but afterward I was able to refactor the problem into a sort of alife that measured and carefully tweaked various variables in the simulator, as the algorithm
explored the paramater space. After a few hours of letting the thing run, it successfully returned a remainder of zero on 41.4% of semiprimes tested.
This is the bad boy right here:
tracks[14]
[15, 2731, 52, 144, 41.4]
As they say, "he ain't there yet, but he got the spirit."
A 'track' here is just a collection of critical values and a fitness score that was found given a few million runs. These variables are used as input to a factoring algorithm, attempting to factor
any number you give it. These parameters tune or configure the algorithm to try slightly different things. After some trial runs, the results are stored in the last entry in the list, and the whole process is repeated with slightly different numbers, ones that have been modified
and mutated so we can explore the space of possible parameters.
Naturally this is a bit of a hodgepodge, but the critical thing is that for each configuration of numbers representing a track (and its results), I chose the lowest fitness of three runs.
Meaning hypothetically theres room for improvement with a tweak of the core algorithm, or even modifications or mutations to the
track variables. I have no clue if this scales up to very large semiprime products, so that would be one of the next steps to test.
Fitness also doesn't account for return speed. Some of these may have a lower overall fitness, but might in fact have a lower basis
(the value of 'i' that needs to be found in order for the algorithm to return rem%a == 0) for correctly factoring a semiprime.
The key thing here is that because all the entries generated here are dependent on in an outer loop that specifies [i] must never be greater than a/4 (for whatever the lowest factor generated in this run is), we can potentially push down the value of i further with some modification.
The entire exercise took 2.1735 billion iterations (3-4 hours, wasn't paying attention) to find this particular configuration of variables for the current algorithm, but as before, I suspect I can probably push the fitness value (percentage of semiprimes covered) higher, either with a few
additional parameters, or a modification of the algorithm itself (with a necessary rerun to find another track of equivalent or greater fitness).
I'm starting to bump up to the limit of my resources, I keep hitting the ceiling in my RAD-style write->test->repeat development loop.
I'm primarily using the limited number of identities I know, my gut intuition, combine with looking at the numbers themselves, to deduce relationships as I improve these and other algorithms, instead of relying strictly on memorizing identities like most mathematicians do.
I'm thinking if I want to keep that rapid write->eval loop I'm gonna have to upgrade, or go to a server environment to keep things snappy.
I did find that "jiggling" the parameters after each trial helped to explore the parameter
space better, so I wrote some methods to do just that. But what I wouldn't mind doing
is taking this a bit of a step further, and writing some code to optimize the variables
of the jiggle method itself, by automating the observation of real-time track fitness,
and discarding those changes that lead to the system tending to find tracks with lower fitness.
I'd also like to break up the entire regime into a training vs test set, but for now
the results are pretty promising.
I knew if I kept researching I'd likely find extensions like this. Of course tested on
billions of semiprimes, instead of simply millions, or tested on very large semiprimes, the
effect might disappear, though the more i've tested, and the larger the numbers I've given it,
the more the effect has become prevalent.
Hitko suggested in the earlier thread, based on a simplification, that the original algorithm
was a tautology, but something told me for a change that I got one correct. Without that initial challenge I might have chalked this up to another false start instead of pushing through and making further breakthroughs.
I'd also like to thank all those who followed along, helped, or cheered on the madness:
In no particular order ,demolishun, scor, root, iiii, karlisk, netikras, fast-nop, hazarth, chonky-quiche, Midnight-shcode, nanobot, c0d4, jilano, kescherrant, electrineer, nomad,
vintprox, sariel, lensflare, jeeper.
The original write up for the ideas behind the concept can be found at:
https://devrant.com/rants/7650612/...
If I left your name out, you better speak up, theres only so many invitations to the orgy.
Firecode already says we're past max capacity!5 -
I'm currently doing project in Java using JavaFX for GUI and after like 6 months I found out we can bind textfields to variables(yea,dumb me) and I got more than 20 forms so of course, binding is useful than that getText method. So I think there are many things like this which will help me to optimize my code but I dont know, so can anyone tell me more stuff like this?
I'm using MongoDb so I'm currently finding easiest way to make Bson document from textfield values. Any suggestions?
(Sorry, for my bad English)1 -
Why do I always have to refactor bad written code ? Is this some kind of karma ?
Undocumented, written by a senior but looks like it was a junior, no unit tests, variables with meaningless names, duplicated code and every possible thing you can find in that kind of code.3 -
I looked at an SQL server today from a customer, talked with one of their devs and he said that he's unable to understand why the server misbehaves... All (!) queries were optimized, but they have 'big data queries'... Migraine started, I had a very bad feeling. Monitoring? Nooooppeeee. Migraine kicks in. Connected to server. SHOW GLOBAL VARIABLES...
After a bit of scrolling I found a lot of misconfigured variables (e.g. extreme large join buffers, unrealistic buffer sizes), high slow query count (nearly 60 % of COM_SELECT) and a few variables that were unknown to me.
Then came the version line.
5.0.46
Yes. 5.0.46.
Big data? Well... 30 GB of usage data.
I called the company back... The dev told me sternly that this was the production server (I had hope...) and that I lie - neither the version, nor the variables could be the problem.
A coworker had to verify it and our manager had to do the communication... Worst, most traumatic working day I ever had. -
Good code is a lie imho.
When you see a project as code, there are 3 variables in most cases:
- time
- people / human resources
- rules
Every variable plays a certain role in how the code (project) evolves.
Time - two different forms: when certain parts of code are either changed in a high frequency or a very low frequency, it's a bad omen.
Too high - somehow this area seems to be relentless. Be it features, regressions or bugs - it takes usually in larger code bases 3 - 4 weeks till all code pathes were triggered.
Too low - it can be a good sign. But it should be on the radar imho. Code that never changes should be reviewed at an - depending on size of codebase - max. yearly audit. Git / VCS is very helpful here.
Why? Mostly because the chances are very high that the code was once written for a completely different requirement set. Hence the audit - check if this code still is doing the right job or if you have a ticking time bomb that needs to be defused.
People
If a project has only person working on it, it most certainly isn't verified by another person. Meaning that only one person worked on it - I'd say it's pretty bad to bad, as no discussion / review / verification was done. The author did the best he / she could do, but maybe another person would have had an better idea?
Too many people working on one thing is only bad when there are no rules ;)
Rules. There are two different kind of rules.
Styling / Organisation / Dokumentation - everything that has not much to do with coding itself. These should be enforced at a certain point, otherwise the code will become a hot glued mess noone wants to work on.
Coding itself. This is a very critical thing.
Do: Forbid things that are known to be problematic in the programming language itself. Eg. usage of variables in variables, reflection, deprecated features.
Do: Define a feature set for each language. Feature set not meaning every feature you want to use! Rather a fixed minimum version every developer must use and - in case of library / module / plugin support - which additional extras are supported.
Every extra costs. Most developers don't want to realize this... And a code base that evolves over time should have minimal dependencies. Every new version of an extra can have bugs, breakages, incompabilties and so on.
Don't: don't specify a way of coding. Most coding guidelines are horrific copy pastures from some books some smart people wrote who have no fucking clue what you're doing and why.
If you don't know how to operate on people, standing in an OR and doing what a book told you to do would end in dead person pretty sure. Same for code.
Learn from mistakes and experience, respect knowledge from other persons, but always reflect on wether this makes sense at this specific area of code.
There are very few things which are applicable to a large codebase on a global level. Even DRY / SOLID and what ever you can come up with can be at a certain point completely wrong.
Good code is a lie - because it can only exist at a certain point of time.
A codebase should be a living thing - when certain parts rot, other parts will be affected too.
The reason for the length of the comment was to give some hints on what my principles are that code stays in an "okayish" state, but good is a very rare state -
Context:
I recently joined a team and we are working on a fairly large mobile app using RN, they started a month ago. And I noticed, they don't have any fucking tests and static typings like Typescript.
I have this pretentious team leader that acts like he's the BeSt dEveLopEr in ThE woRld, and act like he always know what he's doing. But in reality, he code like crap, the formatting is shit because the ESLint config is not working, he's not even aware of it until I've fixed it. He's using every BAD Practice available, unused variables and imports are scattered everywhere, etc. And the directory structure is crap and no consistency.
How can I convince this ignorant mofo to use tests and typescript? He believes that adding those will take us longer and cost more money to the client, based on my experience, this is not the case, it's only slow on the first 2 weeks and it is worth it in the long run.13 -
I have been struggling with managing and keeping track of config secrets and keys. I know that keeping secrets in code is bad karma, but managing them with environment variables becomes cumbersome with multiple microservices running on multiple servers. To worsen this, add humans and access levels.
Whenever I Google, I feel like I am the only one who has this problem. Do you guys sometimes feel like this?
If you have any solutions, hacks or services that you use, please recommend.4 -
My AP Comp Sci teacher back in highschool. She spent a lot of time making BS assignments to get me out of bad habigs. (Uninitialized variables, public variables in Java, bad documentation, cryptic variable names [x, y, string q], etc.)
-
I am the technical lead in a project which uses a C# based framework. It's a lot of drag and drop, and C# scripts can be embedded for fancy stuff.
Scripts in general are not hard to do, it's harder to understand the business rules rather than the code itself.
I got hired as a junior to build this project from scratch as an MVP, and we need another junior to add enhancements and minor changes required from our end users. Since management wants me to move on working on more mid-senior development stuff, I'm supposed to be only supervising the juniors work (in the hopes that one day they'll be able to work on their own).
We've had bad luck filling this position. Our last hire is a guy like 17 years older than me, supposedly with experience in said framework but OH DEAR GOD.
Fucktard can't understand requirements and corrections, isn't able to deliver a 20 line script without fucking up. I give him a list with 3 mistakes to fix and only fixes two, crap like that.
Now, hear me out, the mistakes are stuff like:
- Unused variables
- Confusing error messages
- Error messages written in spanglish (mix between Spanish and English, we're located in Latin America)
- Untested features, this is the worst of all.
You may say "but he's a junior", sure. But as I said, he supposedly has experience, more years in IT than me, and fine, you're allowed to fuck up a few times on your first tasks but not make the same mistakes over and over, specially since we've already sat down and addressed these issues in presence of the CTO.
Fuck this guy. I genuinely dislike him as a person also, he is from another latin country and we have some serious cultural differences. For instance, he insists on sucking your ass constantly, being overly well manered (we already saluted with the whole team at the daily stand up, stop saying hello, good day, regards in each of your fucking chat messages or task submissions), and other mannerisms that are hard to translate, but whatever, all of these attitudes are frowned upon here. They're not necessary, we just want to keep it simple, cordial and casual and see you deliver the crap that you're being paid for with a decent level of quality.
On Monday the CTO comes back from vacation, I'm looking forward to that meeting, gonna report his ass, there is evidence everywhere on our issue tracker.4 -
So there was this project in second year of uni, I was in a team with 2 friends, we had to do a small project to learn programming. I was the most experimented one but still very bad.
One night, I took a few beers and started coding.
I wrote almost all the thing that night, the main functionalities plus the input/output.
But as I was drunk I made some weird decisions:
-naming all the classes in french and all the variables in English
-no tests (who does tests?)
-comments in Spanish
The next morning, when I send the code to my friends (we didn't know about git yet), they started hallucinating. We spent a lot of time refactoring and cleaning.
In the end, as most of the logic was there, we ended up the project a few days before due date and celebrated with more beers 🍺2 -
What's the best way to set username password for DB?
I currently set them as system env variables and then have placeholers in the properties files. This helps in avoiding a bad commit to GitHub.6 -
It's always the same mistake I do: writing bad words as variables or in comments. NEVER DO IT YOU WILL ALWAYS REGRET IT2
-
I'm not experienced in VB Forms. So can someone who is, tell me if I'm just too inexperienced or if Im right about this?
Im tasked with fixing some bugs in a VB Forms project that a privious employee wrote some years ago. When I opened the project and checked it out, there was over 5600 lines of code in the codebehind for the form.
I feel like this is somewhat bad practice, no comments, no documentation... Nothing. And to top it off, among the worst naming of Subs and variables ever. Stuff like: "Run", "Stop", "Feeder", "When Load".
Oh, and the best part? The guy forgot some test code in the software, so when he left, the software stoped functioning. For real, he coded in a dependency to his own account in The AD.1 -
line breaks after 100 or so characters suck. function chaining will look bad in one line, but it doesn't mean we need to limit all our lines to a hard length.
ex:
config.logger.e(TAG, "func() called with: p1 = $p1, p2 = $p2, p3 = $p3, p4 = $p4, p5 = $p5")
this is a standard log that i add at the start of my functions . its easy to autogenerate in intellij , even if my class and variable names are much long (like mVideoAnalyticsManager) . when these variables and their string names add up, they will easily go out of the screen and i won't mind scrolling a few seonds to the left to ensure everything is correct (which 99.9% is)
but a fucking ctrl+alt+l and all strings are broken with a fucking +
+
+
+
+)
AAGh its just irritating ://////4 -
Does anyone know if there's a list or something with examples of bad variable names? As in ones that shouldn't be used because they're already used namespaces and such?
It would be awfully convenient in situations where you're not sure if it's an okay name or not. :/ -
Sorta just had a realization but wanted to confirm if that's how it works.
@Bean adds the object to global objects collection?
And @AutoWired in a object returned in like @Bean ClassA classA() { return new ClassA(); }
Gets the instance from these global collections?
In which case Spring is just a way to use global variables/singletons... Isn't that like bad?14