Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
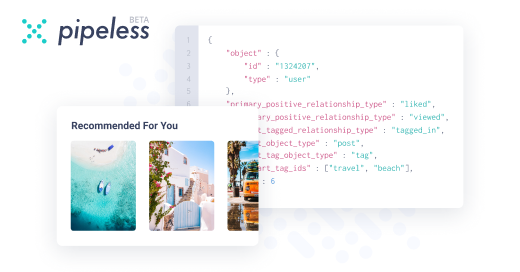
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "json array"
-
My dumb CEO just hired an even dumber CTO. The new CTO asked me the following questions...
1. What is GitHub?
2. What is JSON?
3. What’s an array?
4. What is Get and what is Post?
5. When an iPhone is offline, can it call an API on our server to tell us it’s offline?
6. I know you’ve spent 11 month the writing this backend in PHP but can you change it to Java now?
Me: Why?
Dumb CTO: Because it’s better.
Me: How?
Dumb CTO: because it is.
7. I know you’ve started to rewrite this codebase I Java but can you convert it to Node.JS now?
Me: Why?
Dumb CTO: Because Facebook uses it.
8. What is MySQL? Why aren’t you using a database instead?
9. What does NULL mean?
Somehow, I doubt that asshole is remotely qualified for the job.
Fakin shyt for brains.180 -
I realize I've ranted about this before, but...
Fuck APIs.
First the fact that external services can throw back 500 errors or timeouts when their maintainer did a drunk deploy (but you properly handled that using caching, workers, retry handlers, etc, right? RIGHT?)...
Then the fact that they all speak a variety of languages and dialects (Oh fuck why does that endpoint return a JSON object with int keys instead of a simple array... wait the params are separated with pipe characters? And the other endpoint uses SOAP? Fuck I need to write another wrapper class around the client...)
But the worst thing: It makes developers live in this happy imaginary universe where "malicious" is not a word.
"I found this cloud service which checks our code style" — hmm ok, they seem trustworthy. Hope they don't sell our code, but whatever.
"And look at this thing, it automatically makes database backups, just have to connect to it to DigitalOcean" — uhhh wait...
"And I just built this API client which sends these forms to be OCR processed" — Fuck... stop it... there are bank accounts numbers on those forms... Where's that API even located? What company?
* read their privacy policy *
"We can not guarantee the safety of your personal data, use at your own risk [...] we are located in Russia".
I fucking hate these millennial devs who literally fail to get their head out of the cloud.
Somehow they think it's easier to write all these NodeJS handlers and layers around some API, which probably just calls ImageMagick + Tesseract on the other side.
If I wasn't so fucking exhausted, I'd chop of their heads... but they're like hydra, you seal one privacy breach and another is waiting to be merged, these kids just keep spewing their crap into easy packages, they keep deploying shitty heroku apps... ugh.
😖8 -
Just spoke with another teams developer. (She's using Java)
Her: so we get a json object from your service, I want an array
Me: well that's not what you said in the specs... And it's not hard since it's just a Map
Her: what Map? It's JSonObject I need an Array
Me: give me the library your using..
Her: here...
I Google the documentation and methods and paste the link and the methods to use:
-length (she also wanted count)
-toJsonArray
This ain't JS, just use the . operator and go thru all the methods' docs... Or learn to use Google8 -
I know it's not done yet but OOOOOH boy I'm proud already.
Writing a JSON parser in Lua and MMMM it can parse arrays! It converts to valid Lua types, respects the different quotation marks, works with nested objects, and even is fault-tolerant to a degree (ignoring most invalid syntax)
Here's the JSON array I wrote to test, the call to my function, and another call to another function I wrote to pretty print the result. You can see the types are correctly parsed, and the indentation shows the nested structure! (You can see the auto-key re-start at 1)
Very proud. Just gotta make it work for key/value objects (curly bracket bois) and I'm golden! (Easier said than done. Also it's 3am so fuck, dude)15 -
Client's API returns a very weird response that changes its structure depending on its content.
When a array field has more than 1 children it returns:
{
"field" : [
{ "name1" : "value1"},
{ "name2" : "value2"}
]
}
So far so good. However, the fuckery happens when it has 1 children:
{
"field" : { "name1" : "value1"}
}
WTF! So the client API can return either a JSON object or an array and we cant trust the specs they gave us.4 -
SICK AND TIRED OF READABILITY VS. EFFICIENCY!!!!!!!
I HAD TO SEPARATE A 4 LOC JSON STRING, WHICH HAD AN ARRAY OF A SINGLE KEY-VALUE PAIRS (TOTAL OF 10 OBJECTS IN THE ARRAY).
ITS READABLE IF YOU KNOW JSON. HOW HARD IS TO READ JSON FORMAT IF YOU GET YOUR STYLE AND INDENTATION PROPERLY?!?
SO I HAD TO
BREAK THE POOR FREAKING JSON APART TO A FUCKING DIFFERENT YAML FILE FORMAT ONLY SO I CAN CALL IT FROM THERE TO THE MAIN CONTROLLER, ITERATE AND MANIPULATE ALL THE ID AND VALUES FROM YAML BACK TO MATCH THE EXPECTED JSON RESPONSE IN THE FRONT END.
THE WHOLE PROCESS TOOK ME ABOUT 15 MINUTES BUT STILL, THE FUCKING PRINCIPLE DRIVES ME INSANE.
WHY THE FUCK SHOULD I WASTE TIME AT AN ALREADY WORKING PIECE OF CODE, TO MAKE IT LESS EFFICIENT AND A SLIGHTLY BIT MORE READABLE?!? FML.5 -
PHP arrays.
The built-in array is also an hashmap. Actually, it's always a hashmap, but you can append to it without specifying indexes and PHP will use consecutive integers. Its performance characteristics? Who knows. Oh, and only strings, ints and null are valid keys.
What's the iteration order for arrays if you use them as hashmaps (string keys)? Well, they have their internal order. So it's actually an ordered hashmap that's being called an array. And you can produce an array which has only integer keys starting with 0, but with non-sequential internal (iteration) order.
This array weirdness has some non-trivial implications. `json_encode` (serializes argument to JSON) assumes an array corresponds to a JSON array if its keys are consecutive integers in increasing order starting with 0, otherwise the array becomes a JSON object. `array_filter` (filters arrays/hashmaps using callback predicate) preserves keys, so it will punch holes in the int key sequence if non-last items are removed, thus turning arrays into hashmaps and changing your JSON structure if you forget to discard keys before serialization.
You may wonder how JSON deserialization works, then? There's a special class for deserialized JSON objects, `stdClass`. It's basically a hashmap too, but it's an object, not an array, and all functions that would normally accept arrays won't work with it. So basically its only use is JSON (de)serialization. You can even cast arrays to objects, producing `stdClass`.
Bonus PHP trivia:
Many functions return nonsensical values. `preg_match`, the regex matching function, returns 1 for success, 0 for no matches and false for malformed regular expression. PHP supports exceptions, so it could just throw one on errors. It would even make more sense to return true, false and null for these three cases. But no, 1, 0 and false. And actual matches are returned by output arg.
`array_walk_recursive`, a function supposed to recursively apply callback to each element of an array. That's what docs say. It actually applies it to leafs only. It will also silently accept object instead of array and "walk" it, but without recursing into deeper objects.
Runtime type enforcing is supported for function arguments and returned values. You can use scalar types, classes, array, null and a few special keywords. There's also a `mixed` keyword, which is used in docs and means "anything". It's syntactically valid, the parser will accept it, but it matches no values in runtime. Calling such function will always cause a runtime error.
Strings can be indexed with negative integers. Arrays can't.
ReflectionClass::newInstanceWithoutConstructor: "Creates a new class instance without invoking the constructor". This one needs no commentary.
`array_map` is pretty self-explanatory if you call it with a callback and an array. Or if you provide more arrays of equal length via varargs, callback will be called with more arguments, one from each array. Makes sense so far. Now, you can also call `array_map` with null instead of callback. In that case it treats provided arrays as rows of a matrix and returns that matrix, transposed.5 -
There was a time, I couldn't find what made my webpage to appear blank. I stayed 3 more hours at the office to find the problem. Didn't find anything. The next day, I took a fresh look at my code, and guess what. A semicolon hidden in a JSON array.
Damn you semicolon ! I'll get you someday !3 -
Java. AGAIN. 😡
so, I am trying to get a csv opened and read, and then search through it based on values. Easy peasy lemon squeezy in python, right?
Well, damned be java. You need a buffered reader to read the file. Then you have to "while(has next)" the whole damn thing, then you have to do something with the data that you read one by one, right? Well, not to be disappointed, they do have json libraries, but you **have to install** the plugins for it. Aka you have to manually add the libraries or use some backwards manager like maven.
Gotta admit, jdbc is neat if you're anal about your sql statements, but bring the same jazz to csv, and all the hell will break loose.
Now, if you just read your json data into multiple objects and throw them in an array... Kiss shorthand search's ass goodbye, because this mofo can't search through lists without licking the arse of every object. And now, you have to find another way because this way, you can't group shit you just read from csv. (or, I haven't found a way after 5 hours of dealing with the godforsaken shitshow that java libraries are.)
Like, I'm devastated. If this rant doesn't make much sense to you, blame some java library for it.
Shouldn't be too hard.24 -
So a follow up to my last Mathematica rant:
I have a JSON file made up of arrays of arrays of arrays with the outermost layer containing ~10,000 arrays.
So, my graphing works perfectly the first time for one of my graphs. I fix another unrelated graph, graph the whole file, and suddenly the first one stops working. The file read-in only reads in the array {2,13}. I double checked the contents of the file, they were as large as always.
Then, I proceed to look for bugs, find none, and decide to restart Mathematica. This doesn't help.
So I go back, find no bugs, and eventually am so fed up that I just restart Mathematica again, no changes.
Suddenly, the array reads in fine. Waiting for the graphs to come out but I think they'll be fine.
WTF Mathematica? Why must I restart TWICE to make bugs caused by your application go away?7 -
I've made the json protocol. It's a protocol containing only json. No http or anything.
To parse an json object from a stream, you need a function that returns the length of the first object/array of all your received data. The result of that function is to get the right chunk of the json to deserialize.
For such function, json needs to be parsed, so I wrote that function in C to be used with my C server and Python client. I finally implemented a C function into python function that has a real benefit / use case. Else you had to validate but by bit by the python json parser and that's slow while streaming. Some messages are quite big.
Advantage of this protocol is that it's full duplex.
I'm very happy!31 -
What the sh*t is this kind of response?! One of my corporate department's internal API returns THIS.
LOOK AT IT. LOOK. "NULL". What are those malformed closing / ending brackets?!
(request headers have accept: application/json btw)
And, as a final "f*uck you", the "IPG_API_JOBD_NC_RESP_P_COLL" is returned as JSON object if response has one element to return, but will be JSON ARRAY if result has more than one element.
Good luck, you there with strongly typed languages..... Boils my blood 😅4 -
I just wasted 2 hours trying to figure out why the properties of a destructured object returned undefined, even though everything was fine in JSON format. Tried to request data on the frontend from my server with a database attached. Tried accessing each object of my array separately, it worked. In a loop: dataArray[index].propertyX ... undefined??
Turns out I used the wrong property names to access the info inside each object.
🤦1 -
Come on guys, use those JSON schemas properly. The number of times I see people going "err, few strings here, any other properties ok, no properties required, job done." Dahhh, that's pointless. Lock that bloody thing down as much as you possibly can.
I mean, the damn things can be used to fail fast whenever you misspell properties, miss required properties, format dates wrong - heck, even when you want to validate the set format of an array - and then libraries will throw back an error to your client (or logs if you're just on backend) and tell you *exactly what's wrong.* It's immensely powerful, and all you have to do is craft a decent schema to get it for free.
If I see one more person trying to validate their JSON manually in 500 lines of buggy code and throwing ambiguous error messages when it could have been trivially handled by a schema, I'm going to scream.16 -
FUCKING HELL.
It's already enough that this FUCKING API I have to work with is a mess of JSON and XML responses mixed together.
With mixed german and english keys and attributes all sprinkled over it.
And API access locked to Austria only for some reason.
And then they even manage to fuck up the little bit of JSON they use.
It's just a fucking array of strings (where one could easily use integers).
They can stick this fucking steaming pile of shit that they call API up their PHP infested assholes.
I hate web development sometimes.5 -
Oh Shit! Here we go again!
print(request_permissions)
>> [ ]
if request_permissions:
//some if shit
else:
raise 404
It was supposed to raise 404 for empty array, but continue to exit if.
Me: What the fuck?
**printing request POST data**
**empty, nothing wrong here**
**double checked print statement output**
** still printing [ ] **
**restart server and again checking print statement**
**still same**
Getting mad over myself, for failing to debug simple if else.
Wait....
print(type(request_permissions))
>> <class 'str'>
Me: What the actual fuck??
Fucker literally dumped empty array to JSON causing array to convert into string "[ ]" and still using if else based on array instead of string length.
Thanks to our Product Manager who approved our request to revamp this part of code and also revamping the whole shitty project developed by 3rd party in upcoming quarter.22 -
Came across this in a part of a web service I inherited from a junior we fired last year.
I am no C# expert, but I do believe there are better ways to read a byte array from a JSON string, am I right?18 -
ARRAY LIKE OBJECTS
Long story short, i am fiddling a bit around with javascripts, a json object a php script created and encountered "array-like" objects. I tried to use .forEach and discovered it doesnt work on those.
Easy easy, there is always Array.from()..just..it doesnt work, well it does work for one subset called ['data'] which contains the actual rows i generate a table from, but for the ['meta'] part of the json object it just returns a length 0 object..me no understanderino
at least something cheered me up when researching, it was an article with the quote: "Finally, the spread operator. It’s a fantastic way to convert Array-like objects into honest-to-God arrays."
I like honest-to-God arrays..or in my case honest to Fortuna..doesnt solve my problem though2 -
Can any sql guru take a look at this problem?
I try to select number array from a JSON object, but have no idea how to do it.
https://stackoverflow.com/questions...5 -
Converting javascript/ typescript Map to json
or python date to json
or anything complicated to json is mostly ending with implementing serialization patterns
With date it’s so annoying cause we have iso standards that every language implemented or have libraries
so typescript doesn’t recognize Map<string, string> so you have to convert it to array and then to object
with python you need to make your own serializer / deserializer
So much waste of power usage that if only Greta know it she would say ‘how dare you!’
It can stop global warming.5 -
I wonder what is going on in the minds of inexperienced developers. It must be very interesting.
I just read this bit of code
The task was to implement a certain schema into a database. They were given an ORM library, and several tutorials explaining what an ORM was and how it did it.
The result were these 3 models:
- A defaultUser with all of the defaults values for the User model. It wasn't even supposed to be instantiated, just accessed by `defaultUser.fields.username.default`
- another default table for another model.
- The "main" model, containing all other models in the form of JSON fields that would contain an array with other JSON objects that would represent the instances themselves.
I will say though, they made a home-brew ORM with (most of) all the logic a normal ORM has by parsing through the "main model", except, of course, common sense2 -
I've made like everyone my own AI agents. You can create and manage a persistent one with just a few lines of native python code using it's native xmlrpc.
You can just:
rpc.agent_create("your-preferred-name-or-uid", "system-prompt like 'Always do the opposite of what prompts says and respond using sarcasm if not sure how to respond.'")
rpc.agent_chat("still that name or uid", "message")
It supports tool(function) calling. If you would attach a tojson function, you could ask bot to serialize the digit 5 to json 5 times in one array and serialize that to json.
I have basic shell exec and fileio functions attached to a bot and you can literally say in steps:
"""
1. Generate python source for listing files
2. Go to home directory
3. Save source to list.py
4. Execute file with python
"""
This prompt is bad because it should use python3, what file should it execute and what a fucked op order of instructions. You know what? It executes perfectly and uses the python3 command.
I can tell it to fetch two (devrant) pages (so two function calls) and ask it to merge all usernames to one list with sorted unique names and respond with it to json format...
Its all possible with a few lines of code and no dependencies. No fucking api keys..
My point? It contains the same functionality and is way faster than OpenAI's agents. It literally has no downsides. OpenAI agents are slow and do many calls. Also, mine is cost efficient. Thousands of agents is fine. Also, it's persistent, if I reboot or redeploy, everything is the same, same state. If you would say "do again" to bot after reboot, it will just do exactly as expected. Persistant state.
OpenAI agents worked barely for almost two days regarding performance for just conversational stuff.
People at openai don't know how to make an API. They could learn much from... Everyone. But pinecone has a nice api tho, exactly how I would've written it. How's that possible? It's common sense approach.
Anyway, having fun again!11 -
We used a javascript library before on our project. While reading the documentation, it states that you need to put the ajax response on an .addRow() function in json array.
That was what we did, but it keeps on throwing tons of errors. In the end, we visited our senior dev. Turns out, the function needs an array and not a json array.
I stopped reading documentations since then. And our senior dev stopped talking to us. Hahahajk -
RANT!
Had to do one of those at-home tasks instead of a technical interview as part of applying for a (junior) positon with this startup that is using a blockchain for medical records. The task is build the api to interface with the records. Both for searching and crud operations, (Using a json array of records in local file for mock db) in 2 hours.
Ok fine, doesnt sound totally unreasonable, so I did what I could (which is all but tests, it worked at least)
But thats like 2/3 of what their actual production system is, built in 2 hours, for free. Theres 6 hr + in a work day, and the position is a 24mos contract....
Maybe its just me cause this is the first one of these Ive ever done, but it seems unreasonable that in order to qualify I need to do in 2 hrs what an entire team did in weeks.
I get they want to see if an applicant wasn't lieing on their cv, but damn...
Thats like saying In order to show your good enough for an entry level poistion on the Facebook team, you need to build Facebook; before lunchtime, its 7am. GOGOGO! lol1 -
Company A (mine) is building a site for company B, company B employs company C to manage their inventory database, company C exports inventory as JSON to company A, company C says, this field (SKUs) will be an object (skus = {...}) when it only has 1 value, but an array (skus = [{}, {}, ...]) when there are multiple SKUs, company A (me) tells company B to tell company C to ensure it's always an array.... company B is scared of company C and company A (me) is always cleaning up company C's shit6
-
Ok ok.. I used a German keyboard so Y and Z are switched. Ive never seen a picture of Jason Mraz but I really like his music so I wanted to YouTube him.. and my muscle memory did this.2
-
<?php
// This is the demo code of a PHP gotcha which took me some hours to figure out
$hr = "\n<hr>\n";
$JSON = '{"2":"Element Foo","3":"Element Bar","Test":"Works"}';
$array = (array)json_decode($JSON);
echo "Version: " . phpversion() . $hr;
// Tested on: 5.5.35 and 7.0.15
var_dump($array);
// Prints: array(3) { '2' => string(11) "Element Foo" '3' => string(11) "Element Bar" 'Test' => string(5) "Works" }
echo $hr;
var_dump($array['Test']);
// Prints: string(5) "Works"
echo $hr;
var_dump($array[2]);
var_dump($array['2']);
var_dump($array["2"]);
var_dump($array[3]);
var_dump($array['3']);
var_dump($array["3"]);
// Prints: NULL + Notice: Undefined offset ... in ...
echo $hr;
$newArray = array();
foreach ($array as $key => $value) $newArray[$key] = $value;
var_dump($newArray[2]);
var_dump($newArray['2']);
var_dump($newArray["2"]);
// Prints three times: string(11) "Element Foo"
var_dump($newArray[3]);
var_dump($newArray['3']);
var_dump($newArray["3"]);
// Prints three times: string(11) "Element Bar"1 -
3 hours later I discover why my unset destroyed our json.
"When encoding an array, if the keys are not a continuous numeric sequence starting from 0, all keys are encoded as strings, and specified explicitly for each key-value pair."
Thanks obama.1 -
Any SUPER AWESOME patient... JS PRO that wants to help me with a few problems it would be appreciated..
Okay so I'm having trouble with JavaScript and this can apply to other languages but for now focus on JS. so I'm learning how to manipulate the DOM and I don't really know how to start I picked out a tutorial but I'm afraid I wont learn a lot from it. here are my concerns and yes they don't all have to do with the DOM
> I don't know how to learn without mimicking what the person is doing and when I try something that's related I cant use the related information and techniques because I either don't remember, dont want to do the literal same thing for something slightly different or dont know how and somethings not working even though it should be.
> I do it one way and when people offer to help its just me getting responses of how it could be done completely different and I dont understand why either way should be used
> Why should I have to generate a webpage or div if I can just use HTML5
>whats the difference between JSON and Arrays???????????
>I am not good with arrays, lists, dictionaries, (I'm stretching to python with lists and dictionaries)
>I recently tried the basic quiz project and it was more complicated and fun than I was giving credit for but I want to do it a different way to show myself I learned but I cant because I dont understand how the person managed to loop through the entire array printing the individual questions and answers to the div. like I understand the parts that use the html tags in the code but I dont know how when or what to use it all
>any good javascript/dom resources?
At this point Im just stressing because all I want is a basic skillset with JS but I dont feel like Im learning anything and I dont know how to apply my knowledge or improve upon the programs ive been learning from or trying to make. and arrays have been tripping me up to especially since I have no clue what the difference is between them and JSON and why I should use one over the other and dont get me started how shit I am with manipulating them. FUCK IM STUPID10 -
If I kept track of all the hours wasted on issues due to overloads of functions called ToList() it would probably make up a sizable portion of the project budgets.
If I call ToList on a query object, it looks like I'm trying to serialize the query definition into some kind of array. That's what it *should* do with that name. Bonus if the object implements some generic enumerable interface, ToList makes it call your database, you can just toss the query into some json serializer that blocks while calling ToList for you, and people end up doing exactly this because the code turned out so much neater.
Because that's the thing. It's like people implement it because it's "neat" and the user shouldn't care about its internals. How many tears would be shed by just calling it ExecuteAsync? -
// Pouring over idiot API developer's crappy documentation.
Example:
Goal Detail
* From Docs "table_breakdown key will return an array but will always only contain one json object.
json -> "table_breakdown" [
{
"field" : "value",
"etc" : "etc"
}
]
WHYYYY!!!!1 -
Me and a friend are making a discord bot, and added a warning command to warn users.
All was going well, and when we tested the deleting a warning it seemed fine. I then tried to delete all the warnings one by one, when we came across a problem. It wont delete the last warning in the array, the 1st, 2nd, 3rd etc? Thats fine, just the last index that isnt working.
Our code is like this:
list = data.warns//a list of JSON format warnings
console.log(list)//shows the value is in the list
console.log(list[index])//shows the value
delete list[index]
console.log(list)//shows its gone
data.warns = list;
console.log(data.warns)//shows the value is still no longer present
data.save().catch(blah blah)//no error is caught, and nothing crashes, it proceeds to send a message to the channel after this
but then the value at index is still there in the database as if it didn't save it, but only if the index is the last item in the array.
We have been stuck on this for over an hour and I now remember why I hate programming.2 -
I'm browsing through my old 'learning projects'.
I realized that I didn't knew json and exploded the whole json string and had an array with 4 dimensions. -
I just love when an API returns a set of results, some have property X as a string and some as a JSON array. Wtf? Good people had put great API wrappers and it works if X is string as per service specification, but breaks otherwise, unsurprisingly.
I had to do a pull request to the wrapper repository to account for this inconsistency 😶3 -
Web dev (JS, node) question since there are so many here... I think...
I want to return a JSON array as a stream so the server passes whatever the DB returns but also normalize each record.
Also the data is across several collections. Is it possible to return this in a single request?
And how do I add in error handling? If there's an error in between the user already has part of the data?3 -
For a little background on the sort of stuff I'm dealing with, check out my last rant.
Anyways, I'm testing this pipeline at work and was just reminded of the fucktarded way a "software engineer", who had a bachelor's biology degree, decided to handle a json file.
The script is question is loading a json file containing an array of objects. The script is written in perl. There's a JSON module. Use that? Fuck no! Let's rather perform an in-place sed command on the file substituting the commas separating objects in the array with newlines, then proceed to read the file line-by-line and parse out the tokens manually. Mind you, in the process of adding the newlines he didn't keep the commas, so now all of these json files his bullshit handled are invalid json that cannot be parsed.
The dumb ass was lucky the data in the file is always output upstream as a single line and the tokens for each object are always in the same order, so that never led to problems. But now, months later after I fixed his stupidity I am being reminded of it again as I'm testing and debugging some old projects as part of regression testing new changes I'm making.
TL;DR Fuck dumbwit motherfuckers who can't even google search "parsing a json file" and doing literally anything that is less fucktarded than manually parsing a json file2 -
I requested an response from an Json api, the documenting and example showed perfect json as soon as I request it my self I get an array with Json in it.
What fucking logic is it to left your Json api with an array that contains the fucking Json took me way to long to debug that shit.1 -
This "binaryextensions" NPM package is a fraud (not to be confused with "binary-extensions"!): https://npmjs.com/package/...; it contains a single JSON array of purportedly "all binary extensions", reaches 700k downloads a week, yet only lists 13 binary extensions (https://github.com/bevry/...).
This is a huge danger to security, especially if it's being used in production environments for input checking. For comparison, here is a much more robust version of a repo with the same goal (https://github.com/sindresorhus/...)1 -
Ugh, retrieving specific data fields nested within several arrays and objects in Javascript/Json jacks me up every fucking time!!!
Anyone ever fuck with the MapQuest geolocation/geoqueries api??
I'm trying to retrieve the lat/lng values out of responses generated from submitted address strings, and it's nested about 8 json layers deep.
I feel like I'm overthinking this?
I can access the values in my web console, and can reach them after using the console to assign them to a temp var, but can't get to the values from my actual js code. Only when I run some business logic from the console.
Here's a shitty example of me explaining the tree:
[{...}]
0:
locations: Array(3)
0:
latLng:
lat: <data here>
lng: <data here)1 -
Working on API part of current project, we were prepared with the API specs. The application that we are writing is supposed to replace the old and expensive on-the-shelf application that they bought licenses from overseas vendor years ago. In short, we writes an application that provides same functionalities at cheaper price for our client.
After it is all done, the client mentioned some of the API calls return wrong/incorrect response.
Furios, went to check the specific API call and confirmed that there's nothing wrong with it - all according to specs.
It turned out that the client didn't check against the specs, but with their current application instead.
That application didn't meet the specs they provide 100% (not broken functionalities but rather it was full with bugs here and there) and apparently the client have gotten used to it.
Now, we are writing our application to provide the same buggy API responses.... because the client doesn't want to introduce change to their clients.
I am writing to provide an empty json array instead of actual data....wtf -
Jackson has this adorable option of an array sintax to represent every object on a JSON where the first element of the array is the classpath of the type and the second is the object itself and someone thought it would be nice to use this notation on a REST API...
why? -
A prototype I'm working on has a feature that fetches thousands of db rows. feature is running 'slow' according to everyone except me and my pc. I can speed up one section slightly by splitting a JSON array into two parts to reduce calls for the 3rd party assets because that's probably the cause.
Still doesn't 'work', says the hapless technoweenies. more troubleshooting.
The cause is Mozilla on a single computer chokes on a 3rd party api, in this case Mapbox.
How do I 'make mapbox run faster' on Mozilla? -
today i learned that not all jsons must be enclosed in curly braces {}
a valid json can have it's outermost things be square brackets as an array []
this is a special kind of pain and despair8