Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
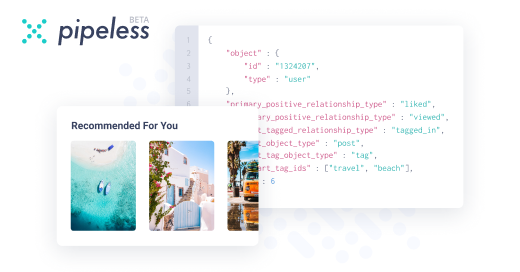
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "ecmascript"
-
Hi, I am a Javascript apprentice. Can you help me with my project?
- Sure! What do you need?
Oh, it’s very simple, I just want to make a static webpage that shows a clock with the real time.
- Wait, why static? Why not dynamic?
I don’t know, I guess it’ll be easier.
- Well, maybe, but that’s boring, and if that’s boring you are not going to put in time, and if you’re not going to put in time, it’s going to be harder; so it’s better to start with something harder in order to make it easier.
You know that doesn’t make sense right?
- When you learn Javascript you’ll get it.
Okay, so I want to parse this date first to make the clock be universal for all the regions.
- You’re not going to do that by yourself right? You know what they say, don’t repeat yourself!
But it’s just two lines.
- Don’t reinvent the wheel!
Literally, Javascript has a built in library for t...
- One component per file!
I’m lost.
- It happens, and you’ll get lost managing your files as well. You should use Webpack or Browserify for managing your modules.
Doesn’t Javascript include that already?
- Yes, but some people still have previous versions of ECMAScript, so it wouldn’t be compatible.
What’s ECMAScript?
- Javascript
Why is it called ECMAScript then?
- It’s called both ways. Anyways, after you install Webpack to manage your modules, you still need a module and dependency manager, such as bower, or node package manager or yarn.
What does that have to do with my page?
- So you can install AngularJS.
What’s AngularJS?
- A Javascript framework that allows you to do complex stuff easily, such as two way data binding!
Oh, that’s great, so if I modify one sentence on a part of the page, it will automatically refresh the other part of the page which is related to the first one and viceversa?
- Exactly! Except two way data binding is not recommended, since you don’t want child components to edit the parent components of your app.
Then why make two way data binding in the first place?
- It’s backed up by Google. You just don’t get it do you?
I have installed AngularJS now, but it seems I have to redefine something called a... directive?
- AngularJS is old now, you should start using Angular, aka Angular 2.
But it’s the same name... wtf! Only 3 minutes have passed since we started talking, how are they in Angular 2 already?
- You mean 3.
2.
- 3.
4?
- 5.
6?
- Exactly.
Okay, I now know Angular 6.0, and use a component based architecture using only a one way data binding, I have read and started using the Design Patterns already described to solve my problem without reinventing the wheel using libraries such as lodash and D3 for a world map visualization of my clock as well as moment to parse the dates correctly. I also used ECMAScript 6 with Babel to secure backwards compatibility.
- That’s good.
Really?
- Yes, except you didn’t concatenate your html into templates that can be under a super Javascript file which can, then, be concatenated along all your Javascript files and finally be minimized in order to reduce latency. And automate all that process using Gulp while testing every single unit of your code using Jasmine or protractor or just the Angular built in unit tester.
I did.
- But did you use TypeScript?37 -
Recently i had to interview a guy with 10 years of frontend experience for a react developer role
Me : Do you know what ecmascript is ?
Him : Yes
Me : Which version would you prefer to use and why ?
Him : I dont use it. I am more comfortable with JavaScript.
Me : (totally confused) 😶
Him : (trying to be oversmart) I know you young guys prefer to use these fancy frameworks because you dont know how powerful raw javascript is.
Me : (Trying hard to not "react") Ok.
How would you "react" to this ?31 -
Okay, story time.
Back during 2016, I decided to do a little experiment to test the viability of multithreading in a JavaScript server stack, and I'm not talking about the Node.js way of queuing I/O on background threads, or about WebWorkers that box and convert your arguments to JSON and back during a simple call across two JS contexts.
I'm talking about JavaScript code running concurrently on all cores. I'm talking about replacing the god-awful single-threaded event loop of ECMAScript – the biggest bottleneck in software history – with an honest-to-god, lock-free thread-pool scheduler that executes JS code in parallel, on all cores.
I'm talking about concurrent access to shared mutable state – a big, rightfully-hated mess when done badly – in JavaScript.
This rant is about the many mistakes I made at the time, specifically the biggest – but not the first – of which: publishing some preliminary results very early on.
Every time I showed my work to a JavaScript developer, I'd get negative feedback. Like, unjustified hatred and immediate denial, or outright rejection of the entire concept. Some were even adamantly trying to discourage me from this project.
So I posted a sarcastic question to the Software Engineering Stack Exchange, which was originally worded differently to reflect my frustration, but was later edited by mods to be more serious.
You can see the responses for yourself here: https://goo.gl/poHKpK
Most of the serious answers were along the lines of "multithreading is hard". The top voted response started with this statement: "1) Multithreading is extremely hard, and unfortunately the way you've presented this idea so far implies you're severely underestimating how hard it is."
While I'll admit that my presentation was initially lacking, I later made an entire page to explain the synchronisation mechanism in place, and you can read more about it here, if you're interested:
http://nexusjs.com/architecture/
But what really shocked me was that I had never understood the mindset that all the naysayers adopted until I read that response.
Because the bottom-line of that entire response is an argument: an argument against change.
The average JavaScript developer doesn't want a multithreaded server platform for JavaScript because it means a change of the status quo.
And this is exactly why I started this project. I wanted a highly performant JavaScript platform for servers that's more suitable for real-time applications like transcoding, video streaming, and machine learning.
Nexus does not and will not hold your hand. It will not repeat Node's mistakes and give you nice ways to shoot yourself in the foot later, like `process.on('uncaughtException', ...)` for a catch-all global error handling solution.
No, an uncaught exception will be dealt with like any other self-respecting language: by not ignoring the problem and pretending it doesn't exist. If you write bad code, your program will crash, and you can't rectify a bug in your code by ignoring its presence entirely and using duct tape to scrape something together.
Back on the topic of multithreading, though. Multithreading is known to be hard, that's true. But how do you deal with a difficult solution? You simplify it and break it down, not just disregard it completely; because multithreading has its great advantages, too.
Like, how about we talk performance?
How about distributed algorithms that don't waste 40% of their computing power on agent communication and pointless overhead (like the serialisation/deserialisation of messages across the execution boundary for every single call)?
How about vertical scaling without forking the entire address space (and thus multiplying your application's memory consumption by the number of cores you wish to use)?
How about utilising logical CPUs to the fullest extent, and allowing them to execute JavaScript? Something that isn't even possible with the current model implemented by Node?
Some will say that the performance gains aren't worth the risk. That the possibility of race conditions and deadlocks aren't worth it.
That's the point of cooperative multithreading. It is a way to smartly work around these issues.
If you use promises, they will execute in parallel, to the best of the scheduler's abilities, and if you chain them then they will run consecutively as planned according to their dependency graph.
If your code doesn't access global variables or shared closure variables, or your promises only deal with their provided inputs without side-effects, then no contention will *ever* occur.
If you only read and never modify globals, no contention will ever occur.
Are you seeing the same trend I'm seeing?
Good JavaScript programming practices miraculously coincide with the best practices of thread-safety.
When someone says we shouldn't use multithreading because it's hard, do you know what I like to say to that?
"To multithread, you need a pair."18 -
Why the hell does every single frontend job on Earth require expert knowledge of React, a fad JS library that will be forgotten in 5 years?
For extra credit: when was the sage advice of "keep content, appearance, and behavior separate" overruled in favor of "use JavaScript for literally everything"? Have I been in a coma for the past 3 years?
Look, I understand the appeal of separating code into repeatable components. I just don't understand why essentially the same thing can't be accomplished with vanilla JS, which is ten times easier to understand and doesn't require an entire website to be written in some make-believe offshoot of Ecmascript that will look like hieroglyphics a decade from now.
There are also seemingly no positions that offer people a platform to start applying React to real world scenarios. I've seen the "understand React in 5 minutes" articles. Wow, super cool, you used 30 lines of JavaScript to render a "Hello world" div. The possibilities are truly endless.
But seriously, how does this shit work? Where do I go to learn the mystical art of offloading every single one of a website's responsibilities to JavaScript? How do I use React to build an actual functioning website, and at what point will it save me time or increase my productivity instead of just pissing me off?17 -
Well done firefox for not implementing some stuff from ES6, because you decided to do so. Isn't the releases of EcmaScript considered to be standards? I'm a Firefox fan, but damn, Chrome is so much more advanced.7
-
Sometimes I see advice given to others that is either clearly wrong or even dangerous, but it's not socially acceptable to call them out on it because 'everyone is entitled to their opinion'.
Yes, you're right. I'm entitled to my opinion based on doing this for 20 years and facing your exact, usually "junior" situation a hundred times. You did a code camp earlier this year and still think JSX is part of the Ecmascript standard.
There is a difference, and not being able to point it out without being scolded for being "entitled" (ironically) is slowly draining me of any interest in helping others.
How the fuck do you cope with this?18 -
Just found out that IE11 doesn't even support ES6 classes...
I couldn't care less about but still...6 -
Fuck the new ECMAScript 2018 specifications.
https://youtu.be/s-G_RZ4RJLU
I mean seriously? How the hell is dot syntax gonna make it more readable?
Also, i love the brackets, braces and semicolon. Hate to see them deprecated.
Almost gave me a heart attack and my head was boiling watching it.
😓😌6 -
It's official, the "front end dev" doesn't know how to code.
Why.
And it's not a "Well I don't know JS because I use React." scenario, no. He has almost no idea of coding.
What was he thinking trying to build the front end of a very complex app with just HTML, CSS and stupid copied and pasted snippets?5 -
What was the most stupid thing interview ever do to you?
My side of story. He (interviewer, also a developer) try to convince me that JavaScript and Java are the same thing. Which we all know that is not...
He said "I can't hire someone who don't know basic of computer science , this is basic that JavaScript is from Java"
(Isn't ecmascript or something?)
Saying JavaScript is From Java is a wrong statement. There are differences between having a DICK and Being a DICK.5 -
I have multiple (in no particular order) :
Nextcloud : It was an idea that I had in my head as well - to take on corporations like Google in the space of personal cloud. Be free, open-source and put the users in charge.
Gitlab : The most open and transparent company that I've ever come across. And they work 99% remote. They've got features that no other players in the space have. All while putting users in control.
Fediverse social media - Mastodon, GNU Social, Diaspora (soon) : For taking a major step in the direction of putting the users in control of their data; all while enabling a decentralized social network.
Ruby : An open community and building a programming language that runs a lot of software of the world.
Python : The oldest thriving community that has a special place in the development community (and my heart)
Javascript / ECMAScript : The scripting language that grew to be a beast of it's own. -
Ecma International, the organization in charge of managing the ECMAScript standard, has published the most recent version of the JavaScript language. ECMAScript 2016 (ES7 or JavaScript 7th Edition in the old naming scheme) comes with very few new features. The most important is that JavaScript developers will finally get a "raise to the power" operator, which was mysteriously left out of the standard for 20 years. The operator is **... It will also become much easier to search for data in a JavaScript array with Array.prototype.includes(), but support for async functions (initially announced for ES2016), has been deferred until next year's release. "From now on, expect smaller changelogs from the ECMAScript team," reports Softpedia, "since this was the plan set out last year. Fewer breaking changes means more time to migrate code, instead of having to rewrite entire applications, as developers did when the mammoth ES6 release came out last year."1
-
My non-techie girlfriend tends to get a tad pissed at me when she understands what people are talking about when she overhears them talking about programming stuff, e.g. "website with Python backend" or "differences between JavaScript and EcmaScript"... what can I say, she's the best rubber duck there is!1
-
ECMAScript is everywhere, so I thought: Let's do even more inappropriate and insane things with it ;)
...Like using Duktape (small ECMAScript engine) and exposing LoadLibrary/GetProcAddress along with some helper routines to describe the routine's argument types and return type, and finally providing a routine to invoke those routines.
It's a very rough prototype that can handle up to 4 arguments in a 64-bit Windows environment.
Next "todo" is structure handling which will initially be a case of stuffing data into a Buffer() object.
I'm not sure what exactly I'm trying to do with it or why...1 -
!RANT
Oh, the SORROW that is JEST! 😡
Endless days have been swallowed by the abyss in my quest to configure Jest with TypeScript and ECMAScript modules instead of CommonJS. Triumph seemed within my grasp until - BAM! - suddenly the tool forgets what "import" or "export" means. And the kicker? On the CI, it still runs like nothing’s amiss!
Allow me to elucidate for the uninitiated: Jest is supposed to be a testing safeguard, a protective barrier insulating devs from the errors of their peers, ensuring a smooth, uninterrupted coding experience.
But OH, how the tables have turned when the very shield becomes the sword, stabbing me with countless, infuriating errors birthed from Jest’s own design decisions!
The audacity to reinvent the whole module loading process just to facilitate module mocking is mind-boggling! Imagine constructing an entirely new ecosystem just to allow people to pretend modules are something they're not. This is not just overkill; it's a preposterous reinvention of a wheel that insists on being a pentagon!
Sure, if devs want to globally expose their variables, entwining everything in a static context, so be it. BUT, why should we, who walk the righteous path of dependency injection, be subjugated to this configured chaos?!
My blood boils as the jestering Jest thrusts upon me a fragile, perpetually breaking system, punishing ME for its determination to support whole module mocking! A technique, mind you, that I wouldn’t touch with a ten-foot pole, because, you know, DEPENDENCY INJECTION!
Where are the alternatives, you ask? Drowned in the abyss, it seems! Why can’t we embrace snapshots and all the delectable integrations WITHOUT being dragged through this module-mocking mire? Can’t module mocking just be a friendly sidekick, an OPTIONAL add-on, rather than the cruel dictator forcing its agenda upon our code?
Punish those clinging to their static contexts, their global variables – NOT those of us advocating for cleaner, more stable practices!
It’s high time we decouple the goodness of Jest from its built-in bad practices. Must we continue to dance with the devil to delight in the depth of Jest’s capabilities?
WHY, Jest, WHY?! 😭9 -
I like there's a new ECMAScript version but ECMAScript 2015 didn't replace ECMAScript 5 as BrowserJS
huh the more you know -
Don't call is ECMAScript 6 — call it JS 2015.
Don't call it iPhone 16 — call it iPhone 2024. Or Apple Phone 2024.
Don't call it Ubuntu 24.10 Oracular Oriole — call it Ubuntu 2024.
Don't call it WiFi 802.11 b/g/n/ac/ax — call it WiFi 1 gb/s.
Don't call it SDXC II 3 10 — call it SD 300 mb/s.
Don't call it USB 3.2 gen 2x2 — call it USB 20 gb/s.
Don't call it Google Pixel 6A — call it Google Phone 2022 Lite.
STOP. Giving. Bullshit. Names! Make it SIMPLER for once.28 -
I spent half of the day trying to make the Grunt script of my node project which uses ECMAscript 6 (Harmony) and make compatible with Uglify-JS by using Babel... Then I found there was a branch of Uglify-JS for Harmony and I only had to update my dependency which took me 2min -_-
-
Best Explanation I found of every(), filter(), map(), some() and foreach() methods in Javascript
(at least for me as a non-js dev)
could help someone there
https://coderwall.com/p/_ggh2w/...2 -
Got a new job. Its all good. Fun work, great colleagues and good pay. However, last week they mandated some new surveillance tech so all network traffic is unencrypted, logged, stored and analyzed.
Should I stay or should I go? I am big into privacy. What would you do?26 -
1) what do i have to know to be a good react native developer?
2) what level of javascript knowledge do need to have to understand react native?
3) how hard is typescript/ecmascript and what do i need to know to understand it? only js i guess or something else?
4) i have basic knowledge of javascript. do i have to learn it extremely well or can i start learning typescript/ecmascript along with react native?
5) tips for learning react native as fast and as efficiently as humanly possible
thanks3 -
Should we get rid of the name JAVAscript and start calling it EcmaScript or smth to avoid confusing recruiters with Java?20
-
Hi everybody! I would like to know if someone could tell me why I should install a converter between Javascript and EcmaScript if there is already support for ES? Is it for IE?7
-
I have a job interview on Thursday for a .Net stack suite of web apps. Thing is: I know C# and SQL Server pretty good (not necessarily together but that comes pretty easy to me). They also use Javascript/jQuery/ECMAScipt (they said it not me) and ASP.Net. In my web dev days I was mostly backend so I am super super rusty on Javascript and, though to a lesser extent, ASP. Do you have any tutorials and refreshers you recommend? Preferably in an IDE so I can hide my shame from the interwebs? Love you.4
-
Any SUPER AWESOME patient... JS PRO that wants to help me with a few problems it would be appreciated..
Okay so I'm having trouble with JavaScript and this can apply to other languages but for now focus on JS. so I'm learning how to manipulate the DOM and I don't really know how to start I picked out a tutorial but I'm afraid I wont learn a lot from it. here are my concerns and yes they don't all have to do with the DOM
> I don't know how to learn without mimicking what the person is doing and when I try something that's related I cant use the related information and techniques because I either don't remember, dont want to do the literal same thing for something slightly different or dont know how and somethings not working even though it should be.
> I do it one way and when people offer to help its just me getting responses of how it could be done completely different and I dont understand why either way should be used
> Why should I have to generate a webpage or div if I can just use HTML5
>whats the difference between JSON and Arrays???????????
>I am not good with arrays, lists, dictionaries, (I'm stretching to python with lists and dictionaries)
>I recently tried the basic quiz project and it was more complicated and fun than I was giving credit for but I want to do it a different way to show myself I learned but I cant because I dont understand how the person managed to loop through the entire array printing the individual questions and answers to the div. like I understand the parts that use the html tags in the code but I dont know how when or what to use it all
>any good javascript/dom resources?
At this point Im just stressing because all I want is a basic skillset with JS but I dont feel like Im learning anything and I dont know how to apply my knowledge or improve upon the programs ive been learning from or trying to make. and arrays have been tripping me up to especially since I have no clue what the difference is between them and JSON and why I should use one over the other and dont get me started how shit I am with manipulating them. FUCK IM STUPID10 -
Looking through ecmascript documentation and im not recognizong anything under the arrays.
Oh thats the ruby documentation. Why am i on the ruby documentation -
I write code as part of my job but also tend to have a lot of pet projects I think about in my spare time. A lot of those projects are not specifically targeted at solving an actual real problem but are just a curiosity (like my Duktape/ECMAScript thing that could import and call DLL routines.) I often find it difficult to choose which one to continue working on and end up not working on any of them because I can't decide which one is more interesting at the time! Or I get stuck and struggle to find a way around whatever roadblock I've hit.1
-
TIL ECMAScript (2015) supports tail call optimization, except virtually only safari can do that, making it useless1
-
Honest question:
For an open temp role, we are looking for someone that knows and used Angular 1.5 and ECMAScript 6.. yet everyone we have interviewed so far only knows up to Angular 1 and doesn't even know a new version of JavaScript came out, or have never used it before.
Are we really asking too much for someone interested in a temp role or are we just getting unqualified candidates?9 -
Compiler Question
Things you hate/don't like about JS/Javascript/ECMAscript/ES. Or what do you think are the issues4 -
started out with react.....its been a fucking week hopping from documentation to youtube to udemy, edx, pluralsight, blogs and what not..... All hit me at once: babel, webpack, ecmascript, fuckin hell.... Cant even set up my machine on my own without any boilerplate to just start working with a fucking framework ..... Uughhh!! Finally found a setup guide on scotch.io.... Followed the steps using yarn(as thats what the tutorial creater used). Worked flawlessly. Tried to imitate using npm, doesn't work.... Why? Fucking piece of crap framework... Steep learning curve..... Cool logo tho.undefined webpack-server react-dom babel-core 😒🔫 babel-preset-es2015 webpack babel-preset-react react2
-
Dear web developers, please think of the boot disk users.
Users might have to boot their computer from external bootable media such as a live USB stick, SSD, or live CD/DVD, after their operating system caught a problem that prevents it from booting.
Emergency boot media usually has earlier versions of web browsers because they are not frequently used, much less updated. Sadly, the developers of many websites have a habit of breaking compatibility for older web browsers. For example, the new audio player used by the Internet Archive (Archive.org) does not even support Firefox 57, a version that was released as recently as November 2017!
Therefore, websites should retain support for old web browsers. If not all features can be made to work, at least the essential features should work on older browser versions. Websites should not let down people who are stuck due to a computer problem. Those users should still be able to browse the Internet for help, and perhaps enjoy basic entertainment such as watching videos (YouTube, Dailymotion) and listenening to music or audio books (SoundCloud, Internet Archive) while at it.
The attached screenshot shows something no internet user wants to be "greeted" with.
Keep the Internet accessible.18 -
Read The Docs!!!
Read The Docs!!!
But none of y’all have read the ECMAScript Specification.
Don’t even get me started with W3C… Just stick with your W3Schools5 -
Is ECMAScript a dialect of Lisp?
"JavaScript has much in common with Scheme. It is a dynamic language. It has a flexible datatype (arrays) that can easily simulate s-expressions. And most importantly, functions are lambdas. Because of this deep similarity, all the functions in [recursive programming primer] 'The Little Schemer' can be written in JavaScript."
— Douglas Crockford
An interesting discussion on SO (https://stackoverflow.com/questions...)2 -
ES(afaik)2020 has a new private field declaration proposal feature in classes. I made a gist to try them _when they are implemented properly_.
Gonna test on chrome lolz.
https://gist.github.com/fbarda/...5 -
If I were on the ECMAScript committee, I would just ask them to use python for everything instead.40