Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
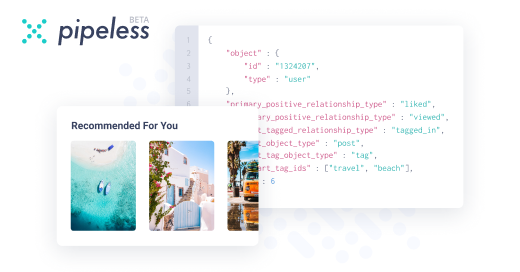
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "god object"
-
It's not that I hate PHP, I just hate the lack of consistency in standard function naming and parameter order, nonsensical attribute access, nearly-meaningless comparison operators, reference handling, case (in)sensitivities, and more!
I mean, look at these functions:
strtoupper(...)
bin2hex(...)
strtolower(...)
And look at THESE FUNCTIONS:
array_search($needle, $haystack)
strpos($haystack, $needle)
array_filter($array, $callable)
array_map($callback, $array)
array_walk($array, $callable)
And let me jUST USE SOME ATTRIBUTES:
$object->attr = "No dollar sign...";
Class::$attr = "GOD WHY";
HOW ABOUT SOME COMPARISONS:
(NULL == 0) // true
(NULL < -1) // ALSO true
Functions AREN'T CASE SENSITIVE (at least variables are).
Wanna dereference? TOO BAD, YOU'LL HAVE TO GET OUT THE TNT.
Alright, yeah, I hate PHP.18 -
Let the student use their own laptops. Even buy them one instead of having computers on site that no one uses for coding but only for some multiple choice tests and to browse Facebook.
Teach them 10 finger typing. (Don't be too strict and allow for personal preferences.)
Teach them text navigation and editing shortcuts. They should be able to scroll per page, jump to the beginning or end of the line or jump word by word. (I am not talking vi bindings or emacs magic.) And no, key repeat is an antifeature.
Teach them VCS before their first group assignment. Let's be honest, VCS means git nowadays. Yet teach them git != GitHub.
Teach git through the command line. They are allowed to use a gui once they aren't afraid to resolve a merge conflict or to rebase their feature branch against master. Just committing and pushing is not enough.
Teach them test-driven development ASAP. You can even give them assignments with a codebase of failing tests and their job is to make them pass in the beginning. Later require them to write tests themselves.
Don't teach the language, teach concepts. (No, if else and for loops aren't concepts you god-damn amateur! That's just syntax!)
When teaching object oriented programming, I'd smack you if do inane examples with vehicles, cars, bikes and a Mercedes Benz. Or animal, cat and dog for that matter. (I came from a self-taught imperative background. Those examples obfuscate more than they help.) Also, inheritance is overrated in oop teachings.
Functional programming concepts should be taught earlier as its concepts of avoiding side effects and pure functions can benefit even oop code bases. (Also great way to introduce testing, as pure functions take certain inputs and produce one output.)
Focus on one language in the beginning, it need not be Java, but don't confuse students with Java, Python and Ruby in their first year. (Bonus point if the language supports both oop and functional programming.)
And for the love of gawd: let them have a strictly typed language. Why would you teach with JavaScript!?
Use industry standards. Notepad, atom and eclipse might be open source and free; yet JetBrains community editions still best them.
For grades, don't your dare demand for them to write code on paper. (Pseudocode is fine.)
Don't let your students play compiler in their heads. It's not their job to know exactly what exception will be thrown by your contrived example. That's the compilers job to complain about. Rather teach them how to find solutions to these errors.
Teach them advanced google searches.
Teach them how to write a issue for a library on GitHub and similar sites.
Teach them how to ask a good stackoverflow question :>6 -
If you need to learn/teach object orientation, these are my approaches (I hate that classic "car" example):
1) Keep in mind games like Warcraft, Starcraft, Civilization, Age of Empires (yes, I am old school). They are a good example of having classes to use, instantiating objects (creatures) and putting them to work together. As in a real system.
2) Think of your program as an office that has a job to do, or a factory that has something to deliver. Classes are the roles/jobs and objects are the workers/employees. They don't need to be complex, but their purpose must be really (really, really) well defined. Just like in a real office / factory.
3) Even better (or crazier), see your classes and objects as real beings, digital creatures in a abstract world, and yourself as a kind of god, who creates species (define classes) with wisdom. Give life when it is the time for them to come into the world (instantiate object) and kill them when they are done with their mission (dispose an object). Give them behavior, logic, conditions to work with, situations where they take action, and when they don't. Make them kinda "smart". Build them able to make decisions and take actions based on conditions. Give them life. Think on your program as an ecossystem. There must be balance, connection, species must be well defined and creatures must work together to achieve a common objective. Don't just throw code and pray for it to run. Plan it.
-----
When I talk about my classes like they are real beings, and programs as mini-worlds, some people say I am crazy, some others say that's passion.
It is both! @__@3 -
WASM was a mistake. I just wanted to learn C++ and have fast code on the web. Everyone praised it. No one mentioned that it would double or quadruple my development time. That it would cause me to curse repeatedly at the screen until I wanted to harm myself.
The problem was never C++, which was a respectable if long-winded language. No no no. The problem was the lack of support for 'objects' or 'arrays' as parameters or return types. Anything of any complexity lives on one giant Float32Array which must surely bring a look of disgust from every programmer on this muddy rock. That is, one single array variable that you re-use for EVERYTHING.
Have a color? Throw it on the array. 10 floats in an object? Push it on the array - and split off the two bools via dependency injection (why do I have 3-4 line function parameter lists?!). Have an image with 1,000,000 floats? Drop it in the array. Want to return an array? Provide a malloc ptr into the code and write to it, then read from that location in JS after running the function, modifying the array as a side effect.
My- hahaha, my web worker has two images it's working with, calculations for all the planets, sun and moon in the solar system, and bunch of other calculations I wanted offloaded from the main thread... they all live in ONE GIANT ARRAY. LMFAO.If I want to find an element? I have to know exactly where to look or else, good luck finding it among the millions of numbers on that thing.
And of course, if you work with these, you put them in loops. Then you can have the joys of off-by-one errors that not only result in bad results in the returned array, but inexplicable errors in which code you haven't even touched suddenly has bad values. I've had entire functions suddenly explode with random errors because I accidentally overwrote the wrong section of that float array. Not like, the variable the function was using was wrong. No. WASM acted like the function didn't even exist and it didn't know why. Because, somehow, the function ALSO lived on that Float32Array.
And because you're using WASM to be fast, you're typically trying to overwrite things that do O(N) operations or more. NO ONE is going to use this return a + b. One off functions just aren't worth programming in WASM. Worst of all, debugging this is often a matter of writing print and console.log statements everywhere, to try and 'eat' the whole array at once to find out what portion got corrupted or is broke. Or comment out your code line by line to see what in forsaken 9 circles of coding hell caused your problem. It's like debugging blind in a strange and overgrown forest of code that you don't even recognize because most of it is there to satisfy the needs of WASM.
And because it takes so long to debug, it takes a massively long time to create things, and by the time you're done, the dependent package you're building for has 'moved on' and find you suddenly need to update a bunch of crap when you're not even finished. All of this, purely because of a horribly designed technology.
And do they have sympathy for you for forcing you to update all this stuff? No. They don't owe you sympathy, and god forbid they give you any. You are a developer and so it is your duty to suffer - for some kind of karma.
I wanted to love WASM, but screw that thing, it's horrible errors and most of all, the WASM heap32.7 -
God damn fucking shit.
Now I know again why I don't do apps.
This is a app as simple as can be:
Enter a link, click a button, do a http request, download a file.
BUT FUCKING HELL WHY ARE YOU SO FUCKING RETARDED ANDROID?!
I'm not familiar with java but i don't care why is this so freaking unintiutiv to get shit done? Why are there thousands of ways and none works or atleast at a easy way? Make an object for this, make an object for that...
THIS IS RETARDED.
In PHP a simple "file_get_contents" would do the job. I were even down for some curl shenanigans if it were an easy implementation. BUT GOD DAMN.
URL url = new URL("http://fuckinghardcoded.com")
Oh no can't compile because that MIGHT be an invalid URL. Ok try catch this or just tell the rest of the Programm to watch out for this bad boy cause he might throw a MalformedURLException.
Ditch that and try volley. Everything is document except how to fire that queue! Does it do that by itself? Do I really have to do an override to a function while declaring? CMON ON I'M A WEBDEV IS THIS TRYING TO DO A FUCKING CALLBACK AND IS THIS TRYING TO BE AN ANONYMOUS FUNCTION??? Why is this so frustrating and confusing? I'm also mad at myself this is dropdead simple shit but I can't get it to work. Fuck this, fuck java , fuck android and fuck myself10 -
Day of the interview sr. Architect says: "We have near 100% unit test coverage in our code."
One month later when I tell him there are 0 unit tests written against 300 projects: "Yeah, I knew that was a problem."
What can you do when the people who want to hire you lie outright to your face?
Oh yeah, and not a god damned one was written using any sense of object oriented programming at all. Every single damned project is written like its on a motherfucking punchcard put together by a cs 101 student with a 2 hour fucking deadline.
I can understand if it needs some work, just tell me. Don't fucking lie to me just to get me in the door to fix a problem you know you have. JUST HAVE SOME FUCKING RESPECT FOR YOUR CANDIDATES AND DON'T FUCKING LIE TO THEM!
Off to drink some scotch and think about what it would be like to shove a finger deep enough into my nostril to hear a pop and smell popcorn before going off into that good night.
I said good day.3 -
I've been working on implementing a fairly large feature on a project at work--
**Sorry. I should rephrase that**
I've been *trying* to work on implementing a fairly large feature on a project at work.
It's slightly complicated because I'm not as "in the know" with the project as I should be. I get tossed around projects a lot as the only designer+developer so I've got my hands in a lot of buckets... Or git repos I should say... My source tree has a lot of tabs open and each project is run by someone with their own ideologies on how stuff should be done and laid out and what not. Basically jumping between these projects leaves you mildly capable on all of them but not amazing at any of individual one them--
--I digress.
There's a bug I've been trying to fix.
--Stupid simple bug, literally just a casting issue or something but there's so much data in this one object that it's taking a few solid minutes of concentration to figure out which variable is busting it all up. It shouldn't take long to fix...
But it has. It has taken 4 days.
FOUR. DAYS.
...To fix what is basically a null reference exception.
Every time I sit down to work on this bug real quick I get pulled away to do a wireframe or change a flow chart or diagram or colour or print styling.
Every. God. Damn. Time.
4 days. Soon to be 5.
My commits are real low at this point guys.
Please boss man, just let me code...4 -
bash.org, this website always cracks me up. Never gets old.
A few hilarious samples, if you will:
http://bash.org/?23601
<mage> what should I give sister for unzipping?
<Kevyn> Um. Ten bucks?
<mage> no I mean like, WinZip?
http://www.bash.org/?14258
<Sigurd> a sprite is anything not static
<SRElysian> a sprite is a variable object
<SRElysian> be it 2d or 3d
<TorMuck> a sprite is a fucking soda
<TorMuck> you god damn geekass bastards5 -
Lets create a library.
Lets use that library in a project.
Lets wrap the library call in a wrapper functione to remove duplicate code.
Lets add an overloaded wrapper call that wraps the wrapper call that calls the library to partially undo the duplicate code removal.
Lets add another overloaded wrapper call that wraps the wrapper call that wraps the wrapper call that calls the library to partially undo the duplicate code removal.
How I love it. Not.
Sometimes redundancy makes sense, especially when it are two lines which make it obvious whats going on vs a single line that leads to a fuckton of overloaded wrapper functions.
Sheeesh.
Today in "code monkeys deserve divine punishment".
Another funny thing is creating a Helper class for Junit 5 tests, making it instantiable and adding to it all kinds of shit like testcontainer creation, applications instantiation, mocks, ....
... Then " crying " why the tests are so slow.
Yeah. Logic. Isolation of concerns, each test should be a stand alone complex.
But that would lead to redundancy... Oh no.
Better to create a global state god object.
Some devs... Really amaze me, especially when they argument in ways that makes one really wonder whether they are serious or just brain dead.14 -
TL;DR you suck, I suck and everybody sucks, deal with it....
------------------------------------
Let me let off some steam, since I've had enough of people hating on languages "just because"
Every language has it's drawbacks and quirks, BUT they have their strengths also. Saying "I hate {language}" is just you being and ignorant prick and probably your head is so far up your ass that you look like an ass hat. With that being said, every language is either good or bad depending on the developer writing in it. Let's give you an example:
If I ware to give you a brick and ask you to put a nail in a plank, can you do it? Yes, it will be easier if you do it with a hammer, but you have a brick, so hammer is out of the question. If you hit your thumb while doing it... well... sorry, but it is not the bricks fault - it is YOU!
JavaScript, yes it has a whole lot of problems, but it works, you can do a ton of stuff and does a good job at that, it is evolving through node and typescript (and others, just a personal pref), BUT if you used js when you ware debugging that jquery (1.0) plugin written in the free time of a 13 yo, who copy pasted a bunch from SO, well, it is not js' problem - deal with it. Same goes for PHP, i've been there where you had a single `index.php` with bazillion lines of code, did a bunch of eval and it was called MVC, but it also is evolving.. thing is all languages allow you to do some dumb stuff so YOU have to be responsible to not fuck it up (which you always DO btw, we all do). Difference is PHP/JS roll with it because the assumption is that you know what you are doing, which again - newsflash - you don't.
More or less I would blame that shit on businesses which decided to go with undergrads to save money instead of investing in their product, hell, I am in a major company that does not invest that doesn't care a whole lot about dev /tech stuff and now everybody's mother is an engineer - they care about money, because investors care about money (ROI) and because clean code does not pay the bills, but money does.
If we get all of the good practices and apply them to each language every one of them has it's place, that is why there is no "The Language", even if there was, we STILL ware going to fuck it up and probably it was going to be even worse than where we are now.
Study, improve, rinse and repeat... There are SENIORS and LEADS out there that are about 25-30 and have no fucking clue about the language, because they have stuck up their heads up the ass of frameworks and refuse to take a breath of clean air and consider something different than their dogmatic framework "way" of doing things.. That is the result you are seeing. Let me give you a fresh example to illustrate where I am at atm:
Le me works with ZendFramework 2.3-2.5 (why not, which is PHP5+ running on PHP7 [fancy, eh]), and little me writes a module for said project, and tries to contain it in its own space, i.e not touching anything outside of the folder of the module so it is SELF-CONTAINED (see, practices), during 2-3-4 iterations of code review, I've had to modify 4 different modules with `if (somthing === self::SOMETHING_TYPE)` as requested by my TL, which resulted in me not covering 3 use-cases after the changes and not adding a new event (the fw is event-driven, cuz.. reasons) so I have to use a bunch of ifs in the code, to check a config value and do shit. That is the way of I am asked to do things I hate what I've done and the fact that because of CR I have lost case-coverage, a week of work and the same TL will be on my ass on monday that things are now "perfect".
The biggest things is "we care about convention and code style"... right.... That is not because of the language, not because of me, not because of the framework - it is some dude's opinion that you hate, not the language.
New stuff are better, reinventing the wheel is also good, if it wasn't you would've had a few stone circular things on your car and things ware going to be like that - we need to try and try, that is the only way we actually learn shit.
Until things change in the trade, we will be on the same boat, complaining about the same shit over and over, you and me won't be alive probably but things will not change a bit.
We live in a place where state is considered good, god objects necessary (can you believe it, I've got kudos for using the term 'God Object'... yep, let that sink in). If you really hate something, please, oh god I beg you, show me how you will do it better and I will shake your hand and buy you a beer, but until then, please keep your ass-hurt fanboy opinion to your self, no one gives a shit about what you think, we will die and the world will not notice...6 -
Shit Developers say:
Fuck you Jasmine and your camelCase
I’ve been wrestling cucumbers all day
Oh no all the cucumbers are broken
In a fit of refactoring madness I have gone and changed a lot
Did you seriously just give ME nil?... No!
If the shit sticks, then we put nice paint on it
Fucking red dot motherfucker (Ben and his failing specs)
You know what we don’t do often..kill each others builds. Kill them and reschedule for later. Mwahaha ha ha.
This build is going to be so rad...(5mins later)...Ok this is not going to pass..I can feel it in my waters!
Can i do that in a digital way or do i have to move my meaty body downstairs to find him?
All the donkeys have be out the gate by sundown
God, imagine if you could patent mathematical solutions
actually, I wouldn't be surprised if you can in the states "no, you can't use a laplace transform, you haven't got the rights, you have to use a less accurate transform on your matrices"
ooooo a boolean that's phrased in the negative, my favourite for code review destruction!
Fuck the police i'll call the object here
Web RTC - its super easy, all you have to do is..probably some hard stuff
I want to go to that conference so I can start arguments with dickheads about semicolons. Just for fun.
This this is not the same as that this.
Can’t come to work I can’t find any clothes. It’s best for everyone if I just don’t come in. ...2 hours later... Yeah my clothes were just in the other room and i couldn’t be fucked moving
(OH about bad bug reports) - you know when they are all like oh joogly joogly doesn’t doodle doodle and it should wobbly doodle you know? and im all like fuck i don’t know any of that shit you are talking about.
Him: "I don’t like it, it’s against REST convention its so 2006 that my eyes are bleeding. As a privileged white male i feel entitled to complain about this." Me: "you. were. eleven in 2006
Source: Kellective Github2 -
So we had a class that should have 2 states 0 or 1, you think my coworker would be smart enough to represent it with a Boolean? NO!
Represent the state inside the object as an int then when using the object in a function creates a Boolean that determines the state of the object and after the function done it's job THEN call another function that takes the object and the Boolean and change the int state inside the object depending on the Boolean.
Wouldn't it have been whole lot easier to just you know..... Make the state a Boolean from the start.
When I saw this I knew I was witnessing a miracle of the human mind. God bless!
Ps: it wasn't connected to any kind of API nor server and there are never more than 2 states. It's just some local sequential code so don't assume it had a logical reason it's just a fuck up.5 -
What were some of your "OH MY GOD I'M AN AWESOME CODE WIZARD!" moments?
For example, I can remember two or three:
One was when I, with only cursory knowledge of C, never having worked with it but having been exposed to it (and having lots of experience with C# therefore familiar with the c-family syntax), took 5 minute look at a source code and pointed out a bug that the student working on it was trying to solve for the past 2 hours. Sadly, I don't remember what the bug was anymore.
Second one was on reddit, someone posted to gamedev group a 2minute video from his voxel+ai framework he was working on, I watched it, and without any idea what it's written in, or how, I was like "you seem to be dropping frames in a pretty regular manner unrelated to anything I see happening on the screen. You're creating too much garbage on frame-by-frame basis (probably while your AI is exploring what to do), look into object pooling, it'll help".
And the guy responded in a few hours like "by gosh, you're right! thank you! and what do you think about the source code?" (he linked git repo below the video.
And I was like OMG I'M A MAGE, I DIDN'T EVEN CLICK THE REPO LINK, ONLY NOW AFTERWARDS, AND yeah, it's c++ so sadly nothing for me, but OMG I JUST WROTE THE FIRST THING THAT CAME TO MY MIND, DIDN'T EXPECT IT TO BE CORRECT, I'M AWESOME.
=D and the feeling stayed with me for about two days.
(If it's not clear yet, it's perfectly okay, in fact, required, to brag about yourself in answering this question ;) )18 -
I don't need you to reiterate what the problem is. I am aware. I was the one who told you what the problem is. Via email and Slack. Why do you keep restating it to me like you are the one who figured out? I know the table isn't syncing with the third party object. I'm trying to figure out WHY. No amount of "I'm pretty sure the sync process is broken" will trigger a solution. Stop coming into my office every 5 minutes with a new "revelation" that wasn't even your own. This isn't my code, and since the owner of said code is not here to fix it, I have to spend some time figuring out how this damn thing works. SO PLEASE, FOR THE LOVE OF GOD, LET ME WORK SO I CAN FIX THIS2
-
Fuck Unity.
Every single time I try to use Unity to develop my well-along-in-development video game, it finds some way of fucking itself up.
Be it from somehow failing to compile a DLL - which is something completely out of my control, the inspector failing to update itself when I select a new object every five minutes, to the engine managing to fail to load its UI layout because it somehow managed to lose a file responsible for containing the layout, the Inspector forgetting to include a scrollbar and as such trying to cram a bunch of components into one area, crashing in a certain area because I tried using reflections, crashing because I tried running the game in a place that always works, all the way to the whole thing closing instantaneously when I try selecting a new layout.
My experience with using this god-forsaken configuration of code and imagery has been one of endless torment; I've spent hours lamenting about the pain this piece of utter horseshit has caused me to those who'd listen.
I don't know what I did to this thing to deserve to be shown the absolute worst of this engine for the year I've been working on my game for. I can't even take a look at its source code to see if I can piece together things I'll pick up from alien code to fix obnoxious bugs myself because you cunts have it under lock-and-key for some dumbass reason.
Even updating my install of this engine is a gamble; I remember clear-as-day updating my project from 2019.3.14 to whichever one was most recent at the time, and everything breaking. This time, I got lucky and managed to update to 2020.1.4 with no issue on the surface, except I inadvertently let in a host of other issues that somehow made the editor worse than the older one.
There's little point in even bothering to report a bug because this shit happens so randomly that I could be just working on auto-pilot and the next thing I know Unity's stupid "crash handler" rears its ugly head yet again, or you people are probably too busy adding support for platforms no sane person uses like fucking Chromebooks.
There've been times where it's crashed upwards of three times in the span of 40 minutes of light use.
How is one expected to cough up hundreds of dollars a year to use a "pro" version of this horrid editor when every session of use yields a 50/50 chance that it'll either work like it's supposed to, or break in one way or another?
It's a miracle I even managed to type all of this out in one go, I expected the website to just stop responding entirely once I got past four lines.
Do what you will with my post, I don't care.6 -
For hours I spent my time debugging my code, trying different approach to the same code function. Looking for one simple invisible mistake, that is when I want to make a delete request to the IndexedDB.
The request are fine without running a single error, the success event fires perfectly. But one thing which is unexpected, the object inside IndexedDB did not vanish at all. The data stay the same without any flaws (but how can that be, when the 'delete success' event fired? IT SHOULD BE GONE BY NOW!). No kidding, for hours I debug my code, yet found nothing's wrong!
Until one moment I found out the datatype of key I gave the request are different from the object I wanted to delete, the object has a key of 4 and I gave the request "4". I'm so pissed at this moment making me googled 'developer rant' and found this site.
Really! God Bless 1 !== '1'.5 -
Swift 1 and 2 were really pain in the ass!! You had to write God damn completed word of each statement. Eg : "presentViewController" is now "present" in Swift 3. Or that fucking "NS" in every object :)))
Thanks God! Thanks Apple! No more bull shit keyword!2 -
Stupid stupid stupid API that returns a 204 on failed validations.
Informative docs? Hell no! Here's a few hundred long-ass field names that you need to pass as a JSON.
Doesn't work huh? Yeah, you're structure's all wrong. Some of these are grouped in vaguely named keys like "Wholesale".
Oh you need those as well? Yeah, you can see the whole structure if you try to GET an object.
Oh you need an ID to GET an object? Yeah you can just go ahead and create as many as you want. This is just a sandbox API, it's cool.
Oh that's not the point? Ahh you need the structure to be able to create one! *haha* Right, I'll get back to you on that.
* Email correspondence over 2 weeks time. I have still yet to be able to make a an actual successful request. The fucking 204 doesn't count if it doesn't actually create the resource.
Fucking fucky fuckity fuck fuck fuck.
I swear to god if I ever meet this guy in person, I will probably buy him coffee or beer and have a long talk about how to build proper REST APIs.
Because I'm nice like that.8 -
rant.
i'm graduating uni and I have to say, my school sucks. they dont teach us how to be developers, they're teaching us how to be tools.
half the subjects could easily have descriptions like how to be employee of the month. I know social and management skills are important in the workplace but by god if I knew that that's the only thing they'll be teaching then I shouldnt have enrolled. for fuck's sake this is IT not HRM.
it doesnt help that most of the professors cant even code beyond printing statements and loops. they didnt even teach object-oriented programming. I had to study that shit myself, so mind you i'm probably not good at it.
though I've had my share of wonderful professors who have taught me so much, a handful of them isnt enough to salvage the incompetence of the whole faculty.
end rant.5 -
Oh my god, GDScript is the single biggest piece of shit scripting language I have ever witnessed. It somehow manages to combine the very worst things of dynamic typing with the downsides of static typing, all in one bundle of utter shit
Imagine you have two game object scripts that want to reference each other, e.g. by calling each others methods.
Well you're outta fucking luck because scripts CANNOT have cyclic references. Not even fucking *type hints* can be cyclic between scripts. Okay no problem, since GDScript is loosely based of Python I can surely just call my method out of the blue without type hints and have it look it up by name. Nope! Not even with the inefficient as fuck `call` method that does a completely dynamic-at-runtime fuck-compile-time-we-script-in-this-bitch function call can find the function. Why? Because the variable that holds a reference to my other script is assumed to be of type Node. The very base class of everything
So not only is the optional typing colossal garbage. You cant even do a fucking dynamic function call because this piece of shit is just C++ in Pyhtons clothing. And nothing against C++ (first time I said that). At least c++ lets me call a fucking function8 -
A colleague of mine was very smart, but didn't know how to use classes in .net or object literals in js, so he organized his data with arrays.
It worked, of course, but, god, did I hate working with his code. It would take hours to make simple changes. -
FUCK YOU PHP, FUCK YOU SYMFONY AND DEFINITELY FUCK YOU SHOPWARE.
Don't get me wrong, PHP has evolved a lot, but the stuff people are building with it is just the biggest load of fucking shit I have ever seen: Shopware. Shopware is the most ass-sucking abomination to extend. It's nearly impossible to develop anything beyond "use the standard features and shut the fuck up" that is more sophisticated than a fucking calculator.
The architecture of this pile of crap is the worst bullshit ever. A mix of OOP, randomly making use of non OOP concepts and features together with the unnecessarily HUGE amount of useless interfaces and classes. Sometimes I feel like it's 90% fucking shitty boilerplate shit.
And don't get me started with TWIG. It's a nice thought, but WHY THE BLOODY FUCK WOULD YOU NOT USE VUE IF YOU ARE ALREADY USING IT FOR A DIFFERENT PART OF SHOPWARE. This makes no fucking sense whatsoever and makes development of new features a huge pain in the ass. I can't comprehend how people actually like using this shit.
OH AND THE DATABASE. OH MY FUCKING GOD. This one is bad. Ever tried to figure anything out in a database where random strings (yes MySQL "relational" - you might think) that are stored as text in a JSON format make up some object or relations during runtime?? Why the fuck do you have foreign and primary keys if you don't use them properly??
Seriously you can't even figure out which data belongs to what because the architecture just sucks fucking ass. FUCK YOU Shopware wankers, you suck, your product sucks, your support sucks, your architecture sucks and you keep releasing new versions that regularly break shit even in minor versions.
I used to like PHP, but not in projects like these.7 -
c++ has a little bit of a learning curve, I think.
Used smart pointers everywhere in my code because I heard that's what we gotta do nowadays.
When learning about shared vs unique vs weak, I disregarded weak pointers because I didn't really understand them.
"That sounds like something for liberal pansies", I said to myself, then continued on with my STRONG shared and unique pointers.
Now my app leaks memory like a MOTHERFUCKER, if you can believe that.
So now I need to go back and manage my object lifetime with more intent instead of just making everything a shared pointer. Fuckin circular references. Fuckin reaping what I fuckin sow. God damn.9 -
ARRAY LIKE OBJECTS
Long story short, i am fiddling a bit around with javascripts, a json object a php script created and encountered "array-like" objects. I tried to use .forEach and discovered it doesnt work on those.
Easy easy, there is always Array.from()..just..it doesnt work, well it does work for one subset called ['data'] which contains the actual rows i generate a table from, but for the ['meta'] part of the json object it just returns a length 0 object..me no understanderino
at least something cheered me up when researching, it was an article with the quote: "Finally, the spread operator. It’s a fantastic way to convert Array-like objects into honest-to-God arrays."
I like honest-to-God arrays..or in my case honest to Fortuna..doesnt solve my problem though2 -
Don't refractor for fun!
An anecdote from my previous company. A developer had written a shitty java console app for fetching stock prices. About 3000 LOC. just one java GOD class. So, when me and my friend looked at it, we were amazed how that code works with all that if conditions spanning 100LOC. so. My dear friend underestimated the complexity. Since it just fetches stock price and puts in database right. I can write it in few days and much better one. So, he started writing code in an OO way. Three days later I see he still working on it. Having a glimpse at code. The app is now Object oriented shitty and ugly.
Guess what new code never goes in prod too.
Learning
Don't underestimate complexity of app.
Be empathic about fellow developer. Don't think he has written a shitty code. Think why he had to do so.
Don't work on refractors if there is no one to guide you.3 -
yes, you absolute fucking asshat, i would like to do more than JUST LOG FUCKING PALETTE TO THE FUCKING CONSOLE
ANY METHODS EXPOSED BY THIS? ANY OTHER OPTIONS? OR YOU JUST GONNA SHOW THAT YOU CAN LOG OUT THIS OBJECT
https://github.com/Vibrant-Colors/...
retarded documentation will be the end of us all i swear to fucking god (or at least me)
though reap what you sow, js devs just as retarded as the language i conceed
i've never seen such a large README and learned so little of what the fuck is going on or how to actually use any of the options1 -
I wasn't happy with one of our UI views for editing a database query that consisted of about 50 fields ("editing" being the operative term here, not just viewing. It had to be two-way). Everything was hardcoded and defined manually, with each block of ~10 lines being repeated and mostly identical apart from the occasional double inline field and name of the variable. It had "just ended up that way" over time due to the variable names in the UI being different than the names of the variables that came from the API.
I decided to overhaul it all where I defined the different input components and which fields should be included, then made a function which would generate the page based on these definitions. It was about 500 lines of modularized functions and classes where the class for the actual view was about 50 lines- compared to the 1400+ lines of the previous version.
But, it didn't work. It should, but it "just didn't". There was no error. All I got was a blank, solid white page. I could make a drastic change or try something completely different and I would get the same error, same blank page. API fetch succeeded, value assignments succeeded, the object exists, but if you iterated it it was... empty.
I started getting really discouraged that I had made it too abstract. Maybe I actually made it more complex and unreadable than before. Maybe just hardcoding it all was the better solution after all. Maybe I had gone against KISS and overdesigned it.
I was up pretty late and everyone had gone home. When the last guy left there was that mood where "yeah if I can't make this work we'll just use the current version...".
Turns out I had tried iterating over a property of the set of fields to render, rather than the entire collection. In the old method the variables were a member of an object, but now they were its own object, a change I had made to isolate the set of values which were to be viewed/edited and make them easier to pass back and forth. This member existed since I hadn't cleaned it out yet, but it was empty.
I had been banging my head against this for a whole day and I was ready to admit I had made a mistake and wasted my time before discarding it all, but then I backspaced this one property and the interface went from empty to rendering perfectly and with all functionality intact. I swear god rays were coming out of my screen. -
Dear God, why are punishing me by another bug report related to Edge?
Console dock freezes commonly for MINUTES, literally. It doesn't support objects, so every object is very usefully converted to "[object Object]" string. And now I am discovering that change event on input is magically not firing?
What a day. This would be solved in Chrome or Firefox in a matter of minutes, for a same time Edge doesn't even manage to render a page with dev tools opened FFS...2 -
Maybe you people will like this story.
The past semester I studied Java in class. First time doing object oriented programming, I had an annoying teacher but got the hang of it. I still miss C from the last year.
As a final project we had to do any program and apply some stuff we saw in class (The program should have an array list, use interfaces, bla bla bla bery simple stuff). It also must have a complete documentation, a manual and a diary explaining what was developed every week. Bonus points if it was in a repository like GitLab.
I wanted to do an RPG game in a matrix, like a rougelike or an old FF game, that should be a map or two, a few monsters and items and that's it. Enough to show what can I do and to have enough excuses to apply everything that the teacher asked. I had a team with two friends who wanted to do the same.
After making accounts in three different pages that apparently would help us to be more organized (One to make charts and two task trackers) I lost all patience and made an account in GitLab, made the basic classes that we had defined in a chart, divided the tasks and put them in to do on GitLab and we started to work.
One of my companions caused a lot of problems. First, he didin't wanted to learn how to use GitLab (I simply asked them to do merge requests) and he insisted to use GitHub. Then he started to say that using the console version was even better (Pretty sure he said thet he never used Git, but maybe was gas poisoning). The GitLab repository never had a single commit to his name.
BUT WAIT IT GETS BETTER all the entire time, he was complaining about the graphical interface of the game, wanting to use some SDK for RPGs that he found. I told him that we will see that at the end, that first we should have all the mechanics done, test it in ASCII in the console and then, if we have time, we will put the visual interface, separated and optional from the main program to avoid problems.
After two weeks where he gave me very simple standard stuff late, half done and through Google Drive, I discovered he was most of the time working on... the graphical interface SDK! He took the job already done by me and the other guy and making a pretty hardcoded integration with the graphical interface and making everything that he tought it would be necesary. Soon enough the GitLab repository was totally outdated and completly useless. He had the totallity of the project in his half broken laptop, and sometimes he gave us a zip with all the code, outdated after a few minutes. Most of the stuff that I made was modified, a lot of the code was totally unknown to what it was and I had no idea even of how the folders were organised.
We had a month to finish it. I got totally disconected from the project and just hoped for the best, sometimes doing a handful of generic and adaptable lines of code for a specific thing (Funny enough, many core mechanics were nonexistent). The other guy managed to work more on the project, mostly fixing the mess that the guy did: apparently he didin't read the documentation of the SDK and just experimented and saw tutorials and tried to figure out how to do what he wanted.
Talking about documentation: we dont had yet. The code wasn't even commented propely. We did all that the last week and some stuff was finished the last night. The program apparently worked but I had no idea.
Thank God, the teacher just looked over everything and was very impressed by the working camera and the FF tiles. I don't think he saw the code or read too much of the documentation, much less when I directly wrote how I lost all access to the project.
I had a 10/10. I didin't complained. Most easy and annoying ten I ever had. I will never do a project with that guy. -
Saturday morning, trying to set up an automated testing environment on my own since at the workplace it's not considered something useful and time should be spent on other stuff. Yay.
Been there another couple of times, both times failed due to poor, overcomplicated architecture that makes use of DI in the very places it shouldn't (and vice versa)... but then I finally found where the DB access is configured and thought "well, let's try tomorrow to automate this bitch".
...turns out, the db access object is injected indeed, but... from a static, deeply nested configuration file, that's referenced EVERYWHERE and embedded in the project core dll.
So basically I can't use a mock DB without changing it in the original config and recompiling the actual project I'm testing, not the test project itself. WTF?!
Or maybe I'm missing something... god, I hope it's me missing something here.
I hope so much to be wrong...1 -
Okay so theres something stopping me from understanding how Object Oriented Programming works. im sorry ahead of time this will get messy..
SO in this case we will use python. well what if the object has more than two functions? like the __init__, func1, then func2 and func2 does something else but doesn't get called or would you have to call of of them like class.func1(), class.func2().
I just don't understand when it comes to how the functions interact or effect each other. and how they would work when you dont call that specific function. I see the use of oop i just cant wrap my head around certain things..15 -
Quess who's back again, php oudated piece of shit monolith codebase. So we have a relatively huge client we need to migrate to AWS.
It is written with yii, all object-oriented. The way it's implemented makes me question my love for object oriented as well my sanity for even accepting this project.
I probably could talk about this piece of shit for hours but the fact they save 3 gigabyte of qr code images is the fucking worst. It's literally a few one hundred thousand images who could be generated on the fly.
Please for the love of god, let me finish this migration tomorrow.4 -
I need some advice to avoid stressing myself out. I'm in a situation where I feel stuck between a rock and a hard place at work, and it feels like there's no one to turn to. This is a long one, because context is needed.
I've been working on a fairly big CMS based website for a few years that's turned into multiple solutions that I'm more or less responsible for. During that time I've been optimizing the code base with proper design patterns, setting up continuous delivery, updating packaging etc. because I care that the next developer can quickly grasp what's going on, should they take over the project in the future. During that time I've been accused of over-engineering, which to an extent is true. It's something I've gotten a lot better at over the years, but I'm only human and error prone, so sometimes that's just how it is.
Anyways, after a few years of working on the project I get a new colleague that's going to help me on my CMS projects. It doesn't take long for me to realize that their code style is a mess. Inconsistent line breaks and naming conventions, really god awful anti-pattern code. There's no attempt to mimic the code style I've been using throughout the project, it's just complete chaos. The code "works", although it's not something I'd call production code. But they're new and learning, so I just sort of deal with it and remain patient, pointing out where they could optimize their code, teaching them basic object oriented design patterns like... just using freaking objects once in a while.
Fast forward a few years until now. They've learned nothing. Every time I read their code it's the same mess it's always been.
Concrete example: a part of the project uses Vue to render some common components in the frontend. Looking through the code, there is currently *no* attempt to include any air between functions, or any part of the code for that matter. Everything gets transpiled and minified so there's absolutely NO REASON to "compress" the code like this. Furthermore, they have often directly manipulated the DOM from the JavaScript code rather than rendering the component based on the model state. Completely rendering the use of Vue pointless.
And this is just the frontend part of the code. The backend is often orders of magnitude worse. They will - COMPLETELY RANDOMLY - sometimes leave in 5-10 lines of whitespace for no discernable reason. It frustrates me to no end. I keep asking them to verify their staged changes before every commit, but nothing changes. They also blatantly copy/paste bits of my code to other components without thinking about what they do. So I'll have this random bit of backend code that injects 3-5 dependencies there's simply no reason for and aren't being used. When I ask why they put them there I simply get a “I don't know, I just did it like you did it”.
I simply cannot trust this person to write production code, and the more I let them take over things, the more the technical debt we accumulate. I have talked to my boss about this, and things have improved, but nowhere near where I need it to be.
On the other side of this are my project manager and my boss. They, of course, both want me to implement solutions with low estimates, and as fast and simply as possible. Which would be fine if I wasn't the only person fighting against this technical debt on my team. Add in the fact that specs are oftentimes VERY implicit, so I'm stuck guessing what we actually need and having to constantly ask if this or that feature should exist.
And then, out of nowhere, I get assigned a another project after some colleague quits, during a time I’m already overbooked. The project is very complex and I'm expected to give estimates on tasks that would take me several hours just to research.
I'm super stressed and have no one I can turn to for help, hence this post. I haven't put the people in this post in the best light, but they're honestly good people that I genuinely like. I just want to write good code, but it's like I have to fight for my right to do it.1 -
Transference of Consciousness
We take ideas or concepts born from abstract thoughts and turn them into working machinery run by an electronic cog. Literally pulling thought from the mind and putting it into action and bringing life into inanimate objects. The life may not 'yet' be self aware or conscious itself. So is programming a desire to impart our will or life to an otherwise inert object? Is this desire intrinsic to our own essence? Was this desire born of the desire our own creator had when making us? I use the term creator very loosely here, btw. It could be a god, the universe itself, aliens, etc.2 -
Is WordPress' use of God objects really such a big problem? I mean, sure wp_query is used for every possible purpose and is the most mutated piece of horror every. But what is the harm?1
-
I am particularly guilty of this, embedding non-constructive comments, code poetry and little jokes into most of my projects (although I usually have enough sense to remove anything directly offensive before releasing the code). Here's one I'm particulary fond of, placed far, far down a poorly-designed 'God Object':
/**
* For the brave souls who get this far: You are the chosen ones,
* the valiant knights of programming who toil away, without rest,
* fixing our most awful code. To you, true saviors, kings of men,
* I say this: never gonna give you up, never gonna let you down,
* never gonna run around and desert you. Never gonna make you cry,
* never gonna say goodbye. Never gonna tell a lie and hurt you.
*/
I'M SORRY!!!! I just couldn't help myself.....!
And another, which I'll admit I haven't actually released into the wild, even though I am very tempted to do so in one of my less intuitive classes:
//
// Dear maintainer:
//
// Once you are done trying to 'optimize' this routine,
// and have realized what a terrible mistake that was,
// please increment the following counter as a warning
// to the next guy:
//
// total_hours_wasted_here = 42
//1 -
God is the master programmer, Discuss citing various object oriented design concepts that he used. 😂2
-
I'm going insane.
So let's say you have an object in database, with 20-30 related objects (Or lists of objects) (All related objects have a foregn key to the "main" object).
Now, as long as you just edit/create thinga everything is fine.
But the deletion... Oh MY GOD
"Just put on delete cascade", right ? RIGHT ?
WRONG ! presence of some objects should block delete, while others can be deleted and some are "situational" depending on the first object state.
So delete operation with all the checks takes .... 1 - 2 seconds.
Seems fine ? WRONG ! When you have 40 or more objects to delete, even 1 second is too long.
Should I say "fuck it" and just write a stored proc which will crash if object cannot be deleted for any reason ? because with Entity Framework, I don't see how I can do it effitenly.
But I HATE stored proc, after couple of month/years noone remembers how they work...
RHAAAAA.
Ok I feel better.8