Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
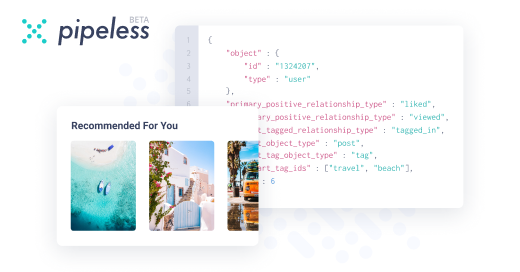
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "that is some ugly code..."
-
!rant
After over 20 years as a Software Engineer, Architect, and Manager, I want to pass along some unsolicited advice to junior developers either because I grew through it, or I've had to deal with developers who behaved poorly:
1) Your ego will hurt you FAR more than your junior coding skills. Nobody expects you to be the best early in your career, so don't act like you are.
2) Working independently is a must. It's okay to ask questions, but ask sparingly. Remember, mid and senior level guys need to focus just as much as you do, so before interrupting them, exhaust your resources (Google, Stack Overflow, books, etc..)
3) Working code != good code. You are an author. Write your code so that it can be read. Accept criticism that may seem trivial such as renaming a variable or method. If someone is suggesting it, it's because they didn't know what it did without further investigation.
4) Ask for peer reviews and LISTEN to the critique. Even after 20+ years, I send my code to more junior developers and often get good corrections sent back. (remember the ego thing from tip #1?) Even if they have no critiques for me, sometimes they will see a technique I used and learn from that. Peer reviews are win-win-win.
5) When in doubt, do NOT BS your way out. Refer to someone who knows, or offer to get back to them. Often times, persons other than engineers will take what you said as gospel. If that later turns out to be wrong, a bunch of people will have to get involved to clean up the expectations.
6) Slow down in order to speed up. Always start a task by thinking about the very high level use cases, then slowly work through your logic to achieve that. Rushing to complete, even for senior engineers, usually means less-than-ideal code that somebody will have to maintain.
7) Write documentation, always! Even if your company doesn't take documentation seriously, other engineers will remember how well documented your code is, and they will appreciate you for it/think of you next time that sweet job opens up.
8) Good code is important, but good impressions are better. I have code that is the most embarrassing crap ever still in production to this day. People don't think of me as "that shitty developer who wrote that ugly ass code that one time a decade ago," They think of me as "that developer who was fun to work with and busted his ass." Because of that, I've never been unemployed for more than a day. It's critical to have a good network and good references.
9) Don't shy away from the unknown. It's easy to hope somebody else picks up that task that you don't understand, but you wont learn it if they do. The daunting, unknown tasks are the most rewarding to complete (and trust me, other devs will notice.)
10) Learning is up to you. I can't tell you the number of engineers I passed on hiring because their answer to what they know about PHP7 was: "Nothing. I haven't learned it yet because my current company is still using PHP5." This is YOUR craft. It's not up to your employer to keep you relevant in the job market, it's up to YOU. You don't always need to be a pro at the latest and greatest, but at least read the changelog. Stay abreast of current technology, security threats, etc...
These are just a few quick tips from my experience. Others may chime in with theirs, and some may dispute mine. I wish you all fruitful careers!223 -
So, you start with a PHP website.
Nah, no hating on PHP here, this is not about language design or performance or strict type systems...
This is about architecture.
No backend web framework, just "plain PHP".
Well, I can deal with that. As long as there is some consistency, I wouldn't even mind maintaining a PHP4 site with Y2K-era HTML4 and zero Javascript.
That sounds like fucking paradise to me right now. 😍
But no, of course it was updated to PHP7, using Laravel, and a main.js file was created. GREAT.... right? Yes. Sure. Totally cool. Gotta stay with the times. But there's still remnants of that ancient framework-less website underneath. So we enter an era of Laravel + Blade templates, with a little sprinkle of raw imported PHP files here and there.
Fine. Ancient PHP + Laravel + Blade + main.js + bootstrap.css. Whatever. I can still handle this. 🤨
But then the Frontend hipsters swoosh back their shawls, sip from their caramel lattes, and start whining: "We want React! We want SPA! No more BootstrapCSS, we're going to launch our own suite of SASS styles! IT'S BETTER".
OK, so we create REST endpoints, and the little monkeys who spend their time animating spinners to cover up all the XHR fuckups are satisfied. But they only care about the top most visited pages, so we ALSO need to keep our Blade templated HTML. We now have about 200 SPA/REST routes, and about 350 classic PHP/Blade pages.
So we enter the Era of Ancient PHP + Laravel + Blade + main.js + bootstrap.css + hipster.sass + REST + React + SPA 😑
Now the Backend grizzlies wake from their hibernation, growling: We have nearly 25 million lines of PHP! Monoliths are evil! Did you know Netflix uses microservices? If we break everything into tiny chunks of code, all our problems will be solved! Let's use DDD! Let's use messaging pipelines! Let's use caching! Let's use big data! Let's use search indexes!... Good right? Sure. Whatever.
OK, so we enter the Era of Ancient PHP + Laravel + Blade + main.js + bootstrap.css + hipster.sass + REST + React + SPA + Redis + RabbitMQ + Cassandra + Elastic 😫
Our monolith starts pooping out little microservices. Some polished pieces turn into pretty little gems... but the obese monolith keeps swelling as well, while simultaneously pooping out more and more little ugly turds at an ever faster rate.
Management rushes in: "Forget about frontend and microservices! We need a desktop app! We need mobile apps! I read in a magazine that the era of the web is over!"
OK, so we enter the Era of Ancient PHP + Laravel + Blade + main.js + bootstrap.css + hipster.sass + REST + GraphQL + React + SPA + Redis + RabbitMQ + Google pub/sub + Neo4J + Cassandra + Elastic + UWP + Android + iOS 😠
"Do you have a monolith or microservices" -- "Yes"
"Which database do you use" -- "Yes"
"Which API standard do you follow" -- "Yes"
"Do you use a CI/building service?" -- "Yes, 3"
"Which Laravel version do you use?" -- "Nine" -- "What, Laravel 9, that isn't even out yet?" -- "No, nine different versions, depends on the services"
"Besides PHP, do you use any Python, Ruby, NodeJS, C#, Golang, or Java?" -- "Not OR, AND. So that's a yes. And bash. Oh and Perl. Oh... and a bit of LUA I think?"
2% of pages are still served by raw, framework-less PHP.31 -
I’m surrounded by idiots.
I’m continually reminded of that fact, but today I found something that really drives that point home.
Gather ‘round, everybody, it’s story time!
While working on a slow query ticket, I perused the code, finding several causes, and decided to run git blame on the files to see what dummy authored the mental diarrhea currently befouling my screen. As it turns out, the entire feature was written by mister legendary Apple golden boy “Finder’s Keeper” dev himself.
To give you the full scope of this mess, let me start at the frontend and work my way backward.
He wrote a javascript method that tracks whatever row was/is under the mouse in a table and dynamically removes/adds a “.row_selected” class on it. At least the js uses events (jQuery…) instead of a `setTimeout()` so it could be worse. But still, has he never heard of :hover? The function literally does nothing else, and the `selectedRow` var he stores the element reference in isn’t used elsewhere.
This function allows the user to better see the rows in the API Calls table, for which there is a also search feature — the very thing I’m tasked with fixing.
It’s worth noting that above the search feature are two inputs for a date range, with some helpful links like “last week” and “last month” … and “All”. It’s also worth noting that this table is for displaying search results of all the API requests and their responses for a given merchant… this table is enormous.
This search field for this table queries the backend on every character the user types. There’s no debouncing, no submit event, etc., so it triggers on every keystroke. The actual request runs through a layer of abstraction to parse out and log the user-entered date range, figure out where the request came from, and to map out some column names or add additional ones. It also does some hard to follow (and amazingly not injectable) orm condition building. It’s a mess of functional ugly.
The important columns in the table this query ultimately searches are not indexed, despite it only looking for “create_order” records — the largest of twenty-some types in the table. It also uses partial text matching (again: on. every. single. keystroke.) across two varchar(255)s that only ever hold <16 chars — and of which users only ever care about one at a time. After all of this, it filters the results based on some uncommented regexes, and worst of all: instead of fetching only one page’s worth of results like you’d expect, it fetches all of them at once and then discards what isn’t included by the paginator. So not only is this a guaranteed full table scan with partial text matching for every query (over millions to hundreds of millions of records), it’s that same full table scan for every single keystroke while the user types, and all but 25 records (user-selectable) get discarded — and then requeried when the user looks at the next page of results.
What the bloody fucking hell? I’d swear this idiot is an intern, but his code does (amazingly) actually work.
No wonder this search field nearly crashed one of the servers when someone actually tried using it.
Asdfajsdfk.rant fucking moron even when taking down the server hey bob pass me all the paperclips mysql murder terrible code slow query idiot can do no wrong but he’s the golden boy idiots repeatedly murdered mysql in the face21 -
---- Startup RantLife ----
A senior developer joined the team, let's name him Bob, and this guy is really good no doubts about that.
He made suggestions, some improvements, but Bob is always waving his hands and says out loud that some part of the code base is really really bad.
I kept quiet until one day I had to pair with Bob to check a feature. Guess what happened, as usual, Bob clenched his fist and start pointing that this code is super ugly.
So let's check the history of changes and boom, Bob was the main writer.
That moment, I was completely silent, trying not to smile as Bob came up with an excuse, he never admits that he is wrong, now he needs a scapegoat and he starts blaming the process, the planning...
I believe that being humble and saying sorry is a quality that it requires time to develop.
So don't be like Bob, please :)12 -
To improve our user's "experience" I suggested to my boss to add a status page showing...well, the current status of our services. Everybody was up for it, so I go off and implement a basic version + automated monitoring backend, get lots of positive feedback, all seems fine.
Then it starts:
Boss: "Can you get it all set up by this Saturday?"
Me: "Uh, today is Wednesday and I've never set up all the stuff needed on a proper server before"
Boss: "Well, you still have a few days. Please also contact your coworker to get it all hooked up in our launcher"
Me: "I'll try, can't make any promises though"
Contact my coworker and tell him what the plan is. I had already given him access to the repo and he is positive to get it all hooked up (I doubt he ever cloned my repo, let alone ran my code)
Spend all Friday getting my stuff set up on the production server, feeling pretty good thanks to the many tutorials.
Contact the boss Friday evening:
Me: "All up and running"
Boss: "Thanks, but we decided to go with a basic HTML page instead. We can just manually edit that, should be enough.
Me: "..."
In the end my stuff was never used, the server I set up was finally taken down a month ago. The gratitude you get when not hacking together some absolute shit that causes problems when you don't add <br/> tags at the correct places to prevent an ugly overflow, cause the coworker was too lazy to implement some form of line wrap in the launcher. I'm not saying my stuff is the best of the best, but at least it was professional looking to a certain extent.8 -
You can believe or not but it’s just one of those stories. It’s long and crazy and it probably happened.
A few years ago I was interviewed by this big insurance company. They asked me on linkedin and were interested. They didn’t specify who they were so I didn’t specify who I am either.
After they revealed who they are I was just curious how they fuck they want to spend those billions of dollars they claimed in their press notes about this fucking digital transformation everyone is talking about. The numbers were big.
I got into 3 or 4 phone/skype interviews without technical questions and I was invited to see them by person.
I know that it would be funny because they didn’t asked me for CV so they didn’t know anything about me and I was just more curious how far I can get without revealing myself.
They canceled interview at midnight and I was in the middle of Louis de Funès comedies marathon so I didn’t sleep whole night. I assumed they would just reschedule but then they phoned me at 8 am if I can come because they made mistake.
So at first talk I was just interviewed by some manager I knowed after 5 minutes he would be shitty as fuck and demand stupid things in no time because he is not technical. He was trying to explain me that they got so great people and they do everything so fast.
From my experience speed and programming are not the things that match. ( for reference of my thought see three virtues of a GREAT programmer )
So I just pissed them off by asking what they would do with me when I finish this transformation thingy next year. ( Probably get rid off and fire at some point were my thoughts )
Then I got this technical interview on newest gold color MacBook pro - pair programming ( they were showing off how much money they have all the time ).
The person asked me to transform json and get some data in javascript .
Really that was the thing and I was so bored and tired that I just asked in what ES standard I can code.
The problem was despite he told me I can do anything and they are using newest standards ( yeah right ) the “for of” loop didn’t worked and he even didn’t know that syntax existed. So I explained him it’s the newest syntax pointing mozilla page and that he need to adjust his configuration. Because we didn’t have time for that I just did it using var an function by writing bunch of code.
When he was asking me if I want to write some tests probably because my code looked ugly as fuck ( I didn’t sleep for more then 24 hours at that point and wanted to live the building as fast as I can) I told I finished and there is no time for tests because it’s so simple and dumb task. The code worked.
After showing me how awesome their office is ( yeah please I work from home so I don’t care ) I got into the talk with VP of engineering and he was the only person who asked me where is my CV because he didn’t know what to talk about. I just laughed at him and told him that I got here just by talking how awesome I am so we can talk about whatever he wants.
After quick talk about 4 different problems where I introduced 4 different languages and bunch of libraries just because I can and I worked with those he was mine.
He told me about this awesome stack they’re building with kubernetes and micro services and the shitty future where they want to put IOT into peoples ass to sell them insurance and suddenly I got awake and started to want that job but behind that all awesomeness there was just .NET bridge with stack of mainframes running COBOL that they want to get rid off and move company to the cloud.
They needed mostly people who would dump code to different technology stack and get rid of old stack ( and probably those old people ) and I was bored again because I work more in r&d field where you sometimes need to think about something that don’t exist and be creative.
I asked him why it would take so much time so he explained me how they would do the transformation by consolidating bunch of companies and how much money they would make by probably firing people that don’t know about it to this day.
I didn’t met any person working permanently there but only consultants from corporations and people hired in some 3rd party company created by this mother company.
They didn’t responded with any decision after me wasting so much time and they asked me for interview for another position year after.
I just explained HR person how they treat people and I don’t want to work there for any money.
If You reached this point it is the end and if it was entertaining thank YOU I did my best.
Have a nice day.5 -
Still trying to get good.
The requirements are forever shifting, and so do the applied paradigms.
I think the first layer is learning about each paradigm.
You learn 5-10 languages/technologies, get a feeling for procedural/functional/OOP programming. You mess around with some electronics engineering, write a bit of assembly. You write an ugly GTK program, an Android todo app, check how OpenGL works. You learn about relational models, about graph databases, time series storage and key value caches. You learn about networking and protocols. You void the warranty of all the devices in your house at some point. You develop preferences for languages and systems. For certain periods of time, you even become an insufferable fanboy who claims that all databases should be replaced by MongoDB, or all applications should be written in C# -- no exceptions in your mind are possible, because you found the Perfect Thing. Temporarily.
Eventually, you get to the second layer: Instead of being a champion for a single cause, you start to see patterns of applicability.
You might have grown to prefer serverless microservice architectures driven by pub/sub event busses, but realize that some MVC framework is probably more suitable for a 5-employee company. You realize that development is not just about picking the best language and best architecture -- It's about pros and cons for every situation. You start to value consistency over hard rules. You realize that even respected books about computer science can sometimes contain lies -- or represent solutions which are only applicable to "spherical cows in a vacuum".
Then you get to the third layer: Which is about orchestrating migrations between paradigms without creating a bigger mess.
Your company started with a tiny MVC webshop written in PHP. There are now 300 employees and a few million lines of code, the framework more often gets in the way than it helps, the database is terribly strained. Big rewrite? Gradual refactor? Introduce new languages within the company or stick with what people know? Educate people about paradigms which might be more suitable, but which will feel unfamiliar? What leads to a better product, someone who is experienced with PHP, or someone just learning to use Typescript?
All that theoretical knowledge about superior paradigms won't help you now -- No clean slates! You have to build a skyscraper city to replace a swamp village while keeping the economy running, together with builders who have no clue what concrete even looks like. You might think "I'll throw my superior engineering against this, no harm done if it doesn't stick", but 9 out of 10 times that will just end in a mix of concrete rubble, corpses and mud.
I think I'm somewhere between 2 and 3.
I think I have most of the important knowledge about a wide array of languages, technologies and architectures.
I think I know how to come to a conclusion about what to use in which scenario -- most of the time.
But dealing with a giant legacy mess, transforming things into something better, without creating an ugly amalgamation of old and new systems blended together into an even bigger abomination? Nah, I don't think I'm fully there yet.8 -
Please. Hear me out.
I've been doing frontend for six years already. I've been a junior dev, then in was all up to the CTO. I've worked for very small companies. Also, for the very large ones. Then, for huge enterprises. And also for startups. I've been developing for IE5.5, just for fun. I've done all kinds of stuff — accessibility, responsive design (with or without breakpoints), web components, workers, PWA, I've used frameworks from Backbone to React. My favourite language is CSS, and you probably know it. The bottom line is, you name it — I did it.
And, I want to say that Safari is a very good browser.
It's very fast. Especially on M1 Macs. Yes, it lacks customization and flexibility of Firefox, but general people, not developers, like to use it. Also, Safari is very important — Apple is a huge opposing force to Google when it comes to web standards. When Google pushes their BS like banning ad blockers, Apple never moves an inch. If we lose Safari, you'll notice.
As for the Safari-specific bugs situation, well… To me, Safari serves as a very good indicator: if your website breaks in Safari, chances are you used some hacks that are no good. Safari is a good litmus test I use to find the parts of my code that could've been better.
The only Safari-specific BUG I encountered was a blurry black segment in linear gradients that go from opaque to transparent. So, instead of linear-gradient(#f00, transparent), just do linear-gradient(#f00f, #f000).
This is the ONLY bug I encountered. Every single time my website broke in Safari other than that, was for some ugly hack I used.
You don't have to love it. I don't even use it, my browser of choice is Firefox. But, I'm grateful to Safari, just because it exists. Why? Well, if Safari ceases to exist, Google will just leave both W3C and WhatWG, and declare they'll be doing things their way from now on. Obey or die.
Firefox alone is just not big enough. But, together with Safari, they oppose Google's tyranny in web standards game.
Google will declare the victory and will turn the web into an authoritarian dictatorship. No ad blockers will be allowed. You won't be able to block Google's trackers. Google already owns the internet, well, almost, and this will be their final, devastating victory.
But Safari is the atlas that keeps the web from destruction.22 -
I'm really close to just quitting coding all together. This job is sucking the life out of me. I've lost my interest in code and the idea that there are better jobs out there.
My "boss" who's not even really my boss but behaves like he is, is micromanaging my every tag, and is an information hog. He doesn't document, he doesn't tell me anything, I've been here six months and still don't know half of what I need to know to do my job properly!
I'm expected to implement a new responsive design, but we don't have design specifications.
Cool, you'd think, new ideas, complete overhaul! Let's get a good foundation in bootstrap going!
WRONG! It needs to fit in with the old, fuck- ugly pre 2000 design.
Not because of any design constraints in particular, but because HE wants it that way. You know what was fucking trendy in 2000? Tables. Tables fucking everywhere. YOU KNOW WHAT TABLES ARE NOT? RESPONSIVE YOU FUCKING ICE LOLLY CHEWER!
We have no development timeline, no process management, no fucking project management. THE FUCKING PASSWORDS WERE STILL STORED IN PLAIN TEXT UNTIL LAST MONTH YOU IRRESPONSIBLE BANANA DEEPTHROATER! 😤😤😤😤😤😤
I'm doing my best here to get something resembling the old page, but there needs to be some fucking compromise! We are in fucking 2017, let's work with Bootstrap instead of against it, how about that you fucking bald cactus!
I know enough about UI to know that the way we're going, this is just going to be another unusable fucking clusterfuck.
YOU KNOW THE BEST FUCKING PART? I'M A FUCKING BACKEND DEV AND I WAS HIRED AS SUCH! GIVE ME A DESIGN TEMPLATE AND I'LL DO MY BEST TO IMPLEMENT IT, BUT FUCK YOU FOR EXPECTING FRONT END LEVEL DESIGN KNOWLEDGE YOU DUMB FUCKING SPAGHETTI!14 -
WordPress related, get ready for some disgust.
So today early in the morning my boss forwarded me an email from a client, it was about a bug, and asked me if I can have a look at it and fix it.
"Yaay, WordPress!" I thought and opened the page containing the mentioned bug. She wrote that in the italian version of the page, users can select dates in the calendar, which should be disabled, like in the german version.
So yeah, I opened the code. Everything in the function looked perfect. Really. And the Data was also correctly set in the backend of WP.
The function was only 3 lines of code:
- Get the german post ID of the current post (german or italian) by its ID (using a Polylang function)
- Get an Advanced Custom Fields field by name and from a post with the ID from before
- json_encode its content and echo it to a JS var for initialization and later use in some AngularJS.
No fucking missing semicolon, it was fucking perfect like a sunset with your soulmate.
So I tried to find the bug with my personal way of debugging:
"Shitstream Debugging"
When a creek suddenly is full of water mixed with shit, walk upstream through the turds until you reach clear water. This is where the bug is.
=> So I first looked at the HTML source: Turds.
=> Then the ACF field content: Still turds.
=> Then the ID of the german post: Shit stain and turds (var_dump: null)
=> Please god at least $post->ID? Nope, fart smell and turds.
=> Nothing more to check: Clear fucking water and the flowery smell of 99 devVirgins
So it replaced $post->IT with get_the_ID() and it worked like a charm.
Afterwards I feel stupid, but $post->IT worked all the times before...
Conclusion:
FUCK YOU WORDPRESS YOU UGLY PIECE OF HUMAN-CENTIPEDE-PROCESSED-DOGFART.
Thanks for your patience.
Only one beer was sucked dry during the writing of this fucking rant.2 -
This here is some source code that i made. And I'll admit, I was a bit frustrated at the time of making. I just started learning to code in HTML and CSS a coulpe days ago. And a friend asked if I could make him a website. So I told him that I barely know the basics yet. And he says that it doesn't matter just as long as he gets a website. So now, a couple days of tryhard coding later, he raged about how bad the site looked and that he himself could have done a better job than I did. And yet the entire site had over 300 lines of code in it (perhaps not very much for you hardcore coders out there, but a biiig step for me) and several subpages, all with custom error pages and all. Although I'll admit, the design was a fucking ugly as fuck since i can design about as good as an alligator flies. But man was I mad after that, haven't talked to him since. The bastard. But to he point, in my rage i made this. An outburst of anger that I later refactored to fit a large amount of devs (since I reckon 99% of programmers deal with clients/customers instead of friends). And if anyone has a spare dns space to put the code on, then help yourself.
The link is:
https://pastebin.com/aFcK10YK
Have a good day!8 -
Inspired by the comment I posted on another rant.
My uni decided to be one of those progressive tech schools that start people with Python. Mind you, I had prepared myself with studying as much as I could with math and programming by automating things and similar stuff in our computer when I was at my previous job, so I had a better idea as to what i could expect.
Introduction to computer science and programming with Python or some shit like that was the name of the class, and the instructor was a fat short ugly woman with a horrible attitude AND a phd in math, not comp sci and barely any industrial knowledge of the field.
She gave us the "a lot of you will fail" speech, which to me is code for "I suck and have no clue what I am doing"
One assignment involved, as per the requirements the use of switch cases. Now, unless someo knew came about, Python does not have swio cases. Me and a couple of less newbie like students tried to point out that switch cases were non existent and that her switch case example was in Javascript, not python, curly braces and everything. She told us to make it work.
We thought that she meant using a function with a dictionary and we pass the key and shit, a simple way of emulating the switch case.
NOPE she took points and insisted that she meant the example. We continuously pointed out that her example was in JS and that at the time Python did not have switch cases. The nasty woman laughed out and said that she didn't expect anyone to finish the assignment with full points.
Out of 100 points everyone got a 70. No problem. Wrote a detailed letter to the dean. Dean replied and talked to her (copied her in the email because fuck you bitch) and my grade was pulled up to full mark.
Every other class I had with her she did not question me. Which was only another class on some other shit I can't remember.
Teachers are what make or break a degree program. What make or break the experience, going to college is putting too much faith on people. If you ask me, trade certification, rigorous training is the future of computer science, or any field really. Rather than spending 4+ years studying a whoooole lotta shit for someone to focus on one field and never leave it.17 -
I recently ranted so much about languages but here it goes
JS we need to talk. BECAUSE YOU GOT FAT AND UGLY STUPID BITCH! Dumb piece of bloatware. What even is your problem? Depending on a library for strpad and then blow up like Steve jobs ego. Bastardized fuckfest. I used to like you bro and then you screw me over!
It's like you fuck my wife while I try to fix your car. Why can't you even be usefully on your own anymore? I'd be richer than bill gates if I get a dollar for every damn framework people pull from their asses. Are you writing this fuck while shitting so you can compare colors of your outcome?
Normalize the fucking base, don't add to the bukkakke! bitch is drowning already. Why is everyone jerking of to react and angular? When have YOU written something in vanilla the last time? Why even bother? Remove the core and hardcore every damn framework into the browsers. Guess that saves you 200kb. Oh wait I forgot that's about unminified jQuery.
Now I need to load about 2GB of dependencies, some creating code that puts code in my code to load code out of my code which was generated out of something that remotely resembles JS so every browser is able to execute my fancy shit. But hey, it's fast. And of course there are the fanboys. You are worse than apple fags. You sample your own jizz with your friends in a wine glass. there was a Time it was bad practice to mix logic and view. Now you made it mandatory. "Browser does the rendering" ofc you imbecile pile of fuck don't show me a damn preloader for 1 picture and 20 lines of text. Who fucked your brain so hard?
So react seems to be the cool kid now, then I tell someone I know angular it's like showing up in a pikachu onsie to a formal dinner with the queen.
I used to love you girl. I loved how we could dirty things together. Now you are like a pig. Please loose weight bby the sight of you disgusts me nowadays2 -
Yknow, I want to make an android app that I have in my mind for about half a year now and I already tried twice, both with Kotlin and with Java but everytime I try it's just pain and suffering and frustration...
No it's not because of the language, I like Java and I like Kotlin too and I'd say I'm at least decent at Kotlin and really good in Java...
No no.. the issue is the fucking Android SDK and the mix-and-match documentation available online!!!
Every fucking time I want to implement some sort of UI element, user action or a background service and I start googling how to do it It comes with with at least 3 different stack overflow solutions, all of them saying "that way of doing it is deprecated, instead you should X" and looking up the OFFICIAL FUCKING DOCS it will just make me roll up in the corner and cry because of how fucking inconsistent it is and the retarded domain language it uses... fucking transactions for fucking fragments inside fucking activities... because I guess the word "screen"/"view"/"template" or something similar natural just was too mainstream for the all knowing alphabet soup that google is...
And then you start looking up what the fucking difference even is and how to code it up only to find out there's at least 12 other opinions on how fragments should be used and what should be an activity and what should be a damn fragment...
But that's not all, that's just the base... I get a headache even thinking about how the fucking inflating of templates and the entire R. notation works. You want to open a fucking tiny corner menu with the settings options? WELL THEN YOU FUCKING BETTER REMEMBER TO IMPLEMENT IT THROUGH SOME SORT OF EVENT AND INFLATE THE MENU YOURSELF EVEN THOUGH ITS THE SAME FUCKING THING WITH STATIC STRINGS...
AND WHY THE FUCK DO I NEED LIKE 4 NEW FILES TO IMPLEMENT A FUCKING LISTVIEW...
also talking about ListViews... what was wrong with "ListView"... Why do we need a "RecyclerView"... oh right... because the fucks fucked the fuck up and all the legacy components were designed by a monkey and are next to useless! SO WE NEEDED A NEW NAME FOR THE FIXED VERSION, CANT NAME IT LISTVIEW AGAIN... FUCK YOU...
honestly... if I got a dolar for every "what the fuck android" I said during trying to understand that mess I'd be richer by a few hundred...
oh oh oh, but you know what? You don't like the android SDK? that's fine, you can use fucking React or Flutter or something... yeah.. because instead of torturing myself with the android SDK I want to torture myself with an abstraction of the same SDK and JavaScript as the fucking cherry on top... HAVE YOU FUCKING SEEN THE CODE FLUTTER SHOWS ON THEIR WEBSITE AS THE "Introduction" ?!!!
Look at this piece of shit:
[code in attached image, we could really use a proper Markdown support at least for rants]
THAT'S NOT EVEN THE ENTIRE THING, THAT'S JUST THE *REALLY* UGLY PART...
The fucking nesting... What is it with JS and all the fucking nesting everytime?! It looks like shit.... It reads like shit as well...
WHY, in the name OF FUCK, IS THERE MORE THAN 5 ANDROID FRAMEWORKS and ALL of them... used this FUCKING NOVEL idea of programming using A FUCKING BRACKET WALL
It always looks like:
(code(code[code{code(code{code()})}]));
If I wanted to make a fucking app or a website using fucking Haskell I'd do that.... at this point reading assembly code feels like heaven compared to this retardation... Why is this so popular?! WHAT DO YOU PEOPLE SEE IN IT?! Clearly it's not the aesthetics... it looks like a fucking frog vomit running down an emus leg, fuck that.... I don't even hate classic JavaScript, it's a good enough language and it does what I tell it to... but these ugly fucking frameworks like react, angular and whatever else uses this fucking format can go fuck right off. This is not the way JS is gonna get a better name for itself...
So:
Fuck Google
Fuck the marionette that designed the Android SDK
Fuck the Hellspawn the came up with the "functional-like" way of using JavaScript
Fuck everyone that thinks "JavaScript everywhere" is a good thing
And deeply future-fuck everyone that makes a new framework following any of these standards, stucks a .js at the end of the name and releases his hairball.js of an invention into the fucking world....
It's a mess... fuck everything android related...14 -
Oh boy, kotlin and its world of statics and lambdas are glorious 💗💗💗
I just finished this attendence counter app i have been working on for last 4 days.its quite simple so i tried to add as much constraints as possible:
-Good practices and minimal warningy
-Room database
-Viewmodel and livedata
-constraint layout
-everything in kotlin
Although i already have worked with room and livedata previously but i dont even have a hello world experience in kotlin . However it doesn't felt that bad tho for a newbie
Every code here is so small . Synthetic binding? Love at first sight.Although at some places its irritating , not having ?: Operator or its ugly 'when' logic, but overall its Awesome!!7 -
I (don't) like how some people say "If your code needs comments, your code is probably ugly and should be rewritten".
Well, asshats. You have never considered complex calculations/functions or "temporary" workarounds, right?
Sometimes, you have to do it in a not-very-readable way for efficiency. There is no way around that in that case, and comments that either explain the code below or provide alternative, slower code that's commented really help others understand your code.
If I ever work with you and you don't bother commenting your code at all (or rather use slow code because more efficient code doesn't appeal to your "muh code dun need comments" approach), I will hate you.6 -
I've started programming when I was 12. Right now I'm 25. I can clearly say that I'm passionate, I've touched I think almost every "type" of programming ever. From game development, through IoT and finished at eCommerce. I never stop learning.
My workmates are pissing me off. For code review sometimes I'm waiting even 3 days when I've changed like 5-6 files. They don't want to introduce "new" technologies (by new I mean who are existing at least 2-3 years, got stable community). They don't want to refactor some core of the application because it's working - they don't care about it as they can later say "legacy system so this basic feature took me a week".
Code quality means for them "use shorthand syntax, this code is ugly" - the basic shit which can do any linter
When I'm doing code review, I'm checking out to this branch, test it, check if the solution is scalable. Then I make my comments. I just hear "stop bitching about it just approve".
Thank God I've made through interview and I'm going to switch job in next week.7 -
As a pretty solid Angular dev getting thrown a react project over the fence by his PM I can say:
FUCK REACT!
It is nigh impossible to write well structured, readable, well modularized code with it and not twist your mind in recursion from "lift state up" and "rendercycle downwards only"
Try writing a modular modal as a modern function component with interchangeable children (passeable to the component as it should be) that uses portals and returns the result of the passed children components.
Closest I found to it is:
c o d e s a n d b o x.io/s/7w6mq72l2q
(and its a fucking nightmare logic wise and readability wise)
And also I still wouldn't know right of the bat how to get the result from the passed child components with all the oneway binding CLUSTERFUCK.
And even if you manage to there is no chance to do it async as it should be.
You HAVE to write a lot of "HTML" tags in the DOM that practically should not be anywhere but in async functions.
In Angular this is a breeze and works like a charm.
Its not even much gray matter to it...
I can´t comprehend how companies decide to write real big web apps with it.
They must be a MESS to maintain.
For a small "four components that show a counter and fetch user images" - OK.
But fo a big webapp with a big team etc. etc.?
Asking stuff about it on Stackoverflow I got edited unsolicited as fuck and downvoted as fuck in an instant.
Nobody explained anything or even cared to look at my Stackblitz.
Unsolicited edit, downvote, closevote and of they go - no help provided whatsoever.
Its completely fine if you don't have time to help strangers - but then at least do not stomp on beginners like that.
I immediately regretted asking a toxic community like this something that I genuinely seem to not understand. Wasn't SO about helping people?
I deleted my post there and won't be coming back and doing something productive there anytime soon.
Out of respect for my clients budget I'm now doing it the ugly react way and forget about my software architecture standards but as soon as I can I will advise switching to Angular.
If you made it here: WOW
Thank you for giving me a vent to let off some steam :)13 -
I loved what Flash used to be. Most people thought it was proprietary stuff. The program was. It's language was not. And damn, did we have fun together! We rendered vector graphics from code and pushed perlin noise into bitmaps while the HTML guys were still struggling with rounded corners. Oh, those bezier curves we dreamed up out of thin lines of code!
Other people just couldn't see how beautiful you were. They hated you because you were popular, and ads were beginning to dominate the landscape. And lots of dildo's made ads by abusing your capabilities, straining you with their ugly code that didn't remove event listeners properly. I always did, because I loved you.
They made fun of you because you had to be compiled. Look what those cavemen are doing now, dear ActionScript 3.0. They are compiling Javascript and pushing it to production. They are all fools my dear, unworthy to read even a single line of your gracious typed syntax. We were faster then Java. More animated and fluid then CSS. We were even responsive if we needed to.
But... I have to move on. I don't know if you're still watching over me but I can't deny I've been trying to find some happiness. I think you would have wanted me to. C# is a sweet girl and I'm thankful for her, but I won't ever forget those short few years we had together. They were the absolute best.
Rest well my dear princess.8 -
One thing you learn after a few years in IT and some languages is that there is no problem with most languages.
BUT
Ugly code is ugly code! Has half of the devs I have been working with never heard of a style guide? Is it so long of a read that they just skip it.
I have flashback of variables being called "a", "b", "c" and/or methods being called "method_alfa" in production code.
In my opinion, repeatedly sh*ting all over a style guide is a reason for getting fired.1 -
i had to do a project with someone who isnt that good at programming. but for her to learn programming, i wanted to let her do part of the code even though i could have done it myself. so she wrote some code after 2 days without me intervening. then i checked out the code and it was total crap. it was ugly asf, it could have been optimized a lot more and a lot of variables were unnecessary and to think that the code was just around 30 lines in 2 days! when its not that optimized, they deduct points from the final grade and having useless variables and functions can also be a negative thing to the professors' eyes.
in the end, i rewrote the code myself because i wanted it to be better. my grade also depends on that code so i shouldnt be ugly asf.
i recognize my mistakes too and sometimes my code isnt as optimized as it can possibly be but imagine her code is waaaay fucked up.
p.s. it didnt even compile2 -
TL;DR:
JuniorDev ignores every advice, writes bad code and complains about other people not working because he does not see their result because he looks at the wrong places.
Okay, so I am really fed up right now.
We have this Junior Dev, who is now with us for circa 8 months, so ca. a year less than me. Our first job for both of us.
He is mostly doing stuff nobody in the team cares about because he is doing his own projects.
But now there's a project where we need to work with him. He got a small part and did implement that. Then parts of the main project got changed and he included stuff which was not there anymore. It was like this for weeks until someone needed to tell him to fix it.
His code is a huge mess (confirmed by senior dev and all the other people working at the project).
Another colleague and me mostly did (mostly) pair programming the past 1-2 weeks because we were fixing and improving (adding functionality) libraries which we are going to use in the project. Furthermore we discussed the overall structure and each of us built some proof-of-concept applications to check if some techniques would work like we planned it.
So in short: We did a lot of preparation to have the project cleaner and faster done in the next few weeks/months and to have our code base updated for the future. Plus there were a few things about technical problems which we need to solve which was already done in that time.
Side note: All of this was done not in the repository of the main project but of side projects, test projects and libraries.
Now it seems that this idiot complained at another coworker (in our team but another project) that we were sitting there for 2 weeks, just talking and that we made no progress in the project as we did not really commit much to the repository.
Side note: My colleague and me are talking in another language when working together and nobody else joins, as we have the same mother tongue, but we switch to the team language as soon as somebody joins, so that other colleague did not even know what we were talking about the whole day.
So, we are nearly the same level experience wise (the other colleague I work with has just one year more professional experience than me) and his work is confirmed to be a mess, ugly and totally bad structured, also not documented. Whereas our code is, at least most of it, there is always space for improvement, clean, readable and re-useable (confirmed by senior and other team members as well).
And this idiot who could implement his (far smaller part) so fast because he does not care about structure or any style convention, pattern or anything complains about us not doing our work.
I just hope, that after this project, I don't have to work with him again soon.
He is also one of those people who think that they know everything because he studied computer science (as everybody in the team, by the way). So he listens to nothing anybody explains to him, not even the senior. You have to explain everything multiple times (which is fine in general) and at some points he just says that he understood, although you can clearly see that he didn't really understand but just wants to go on coding his stuff.
So you explain him stuff and also explain why something does not work or is not a good thing, he just says "yes, okay", changes something completely different and moves on like he used to.
How do you cope with something like this?6 -
Everyone and their dog is making a game, so why can't I?
1. open world (check)
2. taking inspiration from metro and fallout (check)
3. on a map roughly the size of the u.s. (check)
So I thought what I'd do is pretend to be one of those deaf mutes. While also pretending to be a programmer. Sometimes you make believe
so hard that it comes true apparently.
For the main map I thought I'd automate laying down the base map before hand tweaking it. It's been a bit of a slog. Roughly 1 pixel per mile. (okay, 1973 by 1067). The u.s. is 3.1 million miles, this would work out to 2.1 million miles instead. Eh.
Wrote the script to filter out all the ocean pixels, based on the elevation map, and output the difference. Still had to edit around the shoreline but it sped things up a lot. Just attached the elevation map, because the actual one is an ugly cluster of death magenta to represent the ocean.
Consequence of filtering is, the shoreline is messy and not entirely representative of the u.s.
The preprocessing step also added a lot of in-land 'lakes' that don't exist in some areas, like death valley. Already expected that.
But the plus side is I now have map layers for both elevation and ecology biomes. Aligning them close enough so that the heightmap wasn't displaced, and didn't cut off the shoreline in the ecology layer (at export), was a royal pain, and as super finicky. But thankfully thats done.
Next step is to go through the ecology map, copy each key color, and write down the biome id, courtesy of the 2017 ecoregions project.
From there, I write down the primary landscape features (water, plants, trees, terrain roughness, etc), anything easy to convey.
Main thing I'm interested in is tree types, because those, as tiles, convey a lot more information about the hex terrain than anything else.
Once the biomes are marked, and the tree types are written, the next step is to assign a tile to each tree type, and each density level of mountains (flat, hills, mountains, snowcapped peaks, etc).
The reference ids, colors, and numbers on the map will simplify the process.
After that, I'll write an exporter with python, and dump to csv or another format.
Next steps are laying out the instances in the level editor, that'll act as the tiles in question.
Theres a few naive approaches:
Spawn all the relevant instances at startup, and load the corresponding tiles.
Or setup chunks of instances, enough to cover the camera, and a buffer surrounding the camera. As the camera moves, reconfigure the instances to match the streamed in tile data.
Instances here make sense, because if theres any simulation going on (and I'd like there to be), they can detect in event code, when they are in the invisible buffer around the camera but not yet visible, and be activated by the camera, or deactive themselves after leaving the camera and buffer's area.
The alternative is to let a global controller stream the data in, as a series of tile IDs, corresponding to the various tile sprites, and code global interaction like tile picking into a single event, which seems unwieldy and not at all manageable. I can see it turning into a giant switch case already.
So instances it is.
Actually, if I do 16^2 pixel chunks, it only works out to 124x68 chunks in all. A few thousand, mostly inactive chunks is pretty trivial, and simplifies spawning and serializing/deserializing.
All of this doesn't account for
* putting lakes back in that aren't present
* lots of islands and parts of shores that would typically have bays and parts that jut out, need reworked.
* great lakes need refinement and corrections
* elevation key map too blocky. Need a higher resolution one while reducing color count
This can be solved by introducing some noise into the elevations, varying say, within one standard div.
* mountains will still require refinement to individual state geography. Thats for later on
* shoreline is too smooth, and needs to be less straight-line and less blocky. less corners.
* rivers need added, not just large ones but smaller ones too
* available tree assets need to be matched, as best and fully as possible, to types of trees represented in biome data, so that even if I don't have an exact match, I can still place *something* thats native or looks close enough to what you would expect in a given biome.
Ponderosa pines vs white pines for example.
This also doesn't account for 1. major and minor roads, 2. artificial and natural attractions, 3. other major features people in any given state are familiar with. 4. named places, 5. infrastructure, 6. cities and buildings and towns.
Also I'm pretty sure I cut off part of florida.
Woops, sorry everglades.
Guess I'll just make it a death-zone from nuclear fallout.
Take that gators!5 -
So about 3 weeks ago I was laid off from my dream job due to corporate bullshit. From the feedback received since then it is clear that the company made a mistake hiring a brand new React dev while they really needed an experienced one. Because the consultants who were supposed to be weren't. And the other in-house front end dev was an elitist asshole. And I never received proper feedback until it was too late. Actually I still don't have proper feedback save for some vague stuff which really sounds like the kind of feedback you'd give someone in the middle of their learning process. They even said eventually given more time I could have made it. But alas they felt they had to make a call in the best interest of the company.
Things moved fast since then, I took a week to recover and then I spent time updating my resume before getting back in touch with the recruiter who got me my last job. Great guy and he was happy to help me again. Applied to some positions, got some replies, first in person interview I go to they are immediately willing to take me on.
So now I'm supposed to start tomorrow but somehow I'm having my doubts. The company isn't an IT company but rather a fashion company. They believe in developing in house tools because past attempts with external companies resulted in them trying to push their vision through. Knowing who they worked with I agree, they tried to oversell all the time. But after talking with their developers I noticed they are behind on their knowledge. But so am I. So there was no tech interview which means I am getting an easy way in. And if they honour their word I'll be signing tomorrow for around my old wages.
So you'd think that sounds good right? And yet I'm worried it's going to be another shit show working on software without proper analysis or best practices. I mean the devs aren't total idiots, they are mediors like me and I think their heart is in the right place. They want to develop a good project but it will be just us 3 making a modern .net wpf application with the same functionality of the old Access based system currently in use. I was urged by the boss to draw on my experience and I think he wants me to help teach them too. But I'm painfully aware for my decade since graduating I'm a less than average .net dev who struggles with theory and never worked a job where I had someone more experienced to teach me. I coasted most of the time in underpaid jobs due to various reasons. But I'd always get mad over shitty code and practices. Which I realize is hypocritical for someone who couldn't explain what a singleton class is or who still fails at separation of concerns.
So yeah my question for the hivemind is what advice would you give a dev like me? I honestly dislike how poor I perform but it often feels like an insurmountable climb, and being over 30 makes it even more depressing. On the other hand I know I should feel blessed to find a workplace who seems to genuinely believe that people grow and develop and wishes to support me in this. Part of me thinks I should just go in, relax, but also learn till I'm there where I want to be and see if these people are open to improving with me. But part of me also feels I'm rushing into this, picking the first best offer, and it sure feels like a step backwards somehow. And that then makes me feel like an ugly ungrateful person who deserves her bad luck because she expects of others what she can't even do herself :(4 -
Fuck Unity.
Every single time I try to use Unity to develop my well-along-in-development video game, it finds some way of fucking itself up.
Be it from somehow failing to compile a DLL - which is something completely out of my control, the inspector failing to update itself when I select a new object every five minutes, to the engine managing to fail to load its UI layout because it somehow managed to lose a file responsible for containing the layout, the Inspector forgetting to include a scrollbar and as such trying to cram a bunch of components into one area, crashing in a certain area because I tried using reflections, crashing because I tried running the game in a place that always works, all the way to the whole thing closing instantaneously when I try selecting a new layout.
My experience with using this god-forsaken configuration of code and imagery has been one of endless torment; I've spent hours lamenting about the pain this piece of utter horseshit has caused me to those who'd listen.
I don't know what I did to this thing to deserve to be shown the absolute worst of this engine for the year I've been working on my game for. I can't even take a look at its source code to see if I can piece together things I'll pick up from alien code to fix obnoxious bugs myself because you cunts have it under lock-and-key for some dumbass reason.
Even updating my install of this engine is a gamble; I remember clear-as-day updating my project from 2019.3.14 to whichever one was most recent at the time, and everything breaking. This time, I got lucky and managed to update to 2020.1.4 with no issue on the surface, except I inadvertently let in a host of other issues that somehow made the editor worse than the older one.
There's little point in even bothering to report a bug because this shit happens so randomly that I could be just working on auto-pilot and the next thing I know Unity's stupid "crash handler" rears its ugly head yet again, or you people are probably too busy adding support for platforms no sane person uses like fucking Chromebooks.
There've been times where it's crashed upwards of three times in the span of 40 minutes of light use.
How is one expected to cough up hundreds of dollars a year to use a "pro" version of this horrid editor when every session of use yields a 50/50 chance that it'll either work like it's supposed to, or break in one way or another?
It's a miracle I even managed to type all of this out in one go, I expected the website to just stop responding entirely once I got past four lines.
Do what you will with my post, I don't care.6 -
Android development sucks assssssssssss.
They FINALLY made a design system that doesn't look ugly so I thought might as well upgrade my old apps to it.
Publish and tonnnnes of crashes hours after launch.
Test on older devices and turns out some @color/material_xyz was missing in a lower API code BUT available in higher ones? No fallback, no error in AndroidStudio, just a runtime crash. Amazing
Then the location permissions glitch up. On lower androids even if you aren't actively tracking the user, the system tries to call some method which if you haven't overridden, the app crashes at launch.
And no amount of wrapping in try-catch-ignore helps (https://stackoverflow.com/questions... helped)
OH AND THEN the above solution if used on latest Android code33, CRASHES ON RUNTIME. so more sets of 'if VCODE this then ask this else that' bullshit.
I don't even need location it's just for better ad money ffs.
I've been team-android since Froyo and hate apple's monopoly, but if this is the level of their competence, many will jump ship sooner or later.
PS: yes I know I should've checked for lower versions before hand but Im not gonna make 8 android VMs to test all when different things fail in different versions.
I did have to do that in the end, but for a meh pet project one shouldn't have to. The system should have enough fallbacks and graceful fails.3 -
Storytime!
(I just posted this in a shorter form as a comment but wanted to write it as a post too)
TL;DR, smarts are important, but so is how you work.
My first 'real' job was a lucky break in the .com era working tech support. This was pretty high end / professional / well respected and really well paid work.
I've never been a super fast learner, I was HORRIBLE in school. I was not a good student until I was ~40 (and then I loved it, but no longer have the time :( )
At work I really felt like so many folks around me did a better job / knew more than me. And straight up I know that was true. I was competent, but I was not the best by far.
However .... when things got ugly, I got assigned to the big cases. Particularly when I transferred to a group that dealt with some fancy smancy networking equipment.
The reason I was assigned? Engineering (another department) asked I be assigned. Even when it would take me a while to pickup the case and catch up on what was going on, they wanted the super smart tech support guys off the case, and me on it.
At first this was a bit perplexing as this engineering team were some ultra smart guys, custom chip designers, great education, and guys you could almost see were running a mental simulation of the chip as you described what you observed on the network...
What was also amusing was how ego-less these guys seemed to be (I don't pretend to know if they really were). I knew for a fact that recruiting teams tried to recruit some of these guys for years from other companies before they'd jump ship from one company to the next ... and yet when I met them in person it was like some random meeting on the street (there's a whole other story there that I wish I understood more about Indian Americans (many of them) and American engineers treat status / behave).
I eventually figured out that the reason I was assigned / requested was simple:
1. Support management couldn't refuse, in fact several valley managers very much didn't like me / did not want to give me those cases .... but nobody could refuse the almighty ASIC engineers. No joke, ASIC engineers requests were all but handed down on stone tablets and smote any idols you might have.
2. The engineers trusted me. It was that simple.
They liked to read my notes before going into a meeting / high pressure conference call. I could tell from talking to them on the phone (I was remote) if their mental model was seizing up, or if they just wanted more data, and we could have quick and effective conversations before meetings ;)
I always qualified my answers. If I didn't know I said so (this was HUGE) and I would go find out. In fact my notes often included a list of unknowns (I knew they'd ask), and a list of questions I had sent to / pending for the customer.
The super smart tech support guys, they had egos, didn't want to say they didn't know, and they'd send eng down the rabbit hole. Truth be told most of what the smarter than me tech support guy's knew was memorization. I don't want to sound like I'm knocking that because for the most part memorization would quickly solve a good chunk of tech support calls for sure... no question those guys solved problems. I wish I was able to memorize like those guys.
But memorization did NOT help anyone solve off the wall bugs, sort of emergent behavior, recognize patterns (network traffic and bugs all have patterns / smells). Memorization also wouldn't lead you to the right path to finding ANYTHING new / new methods to find things that you don't anticipate.
In fact relying on memorization like some support folks did meant that they often assumed that if bit 1 was on... they couldn't imagine what would happen if that didn't work, even if they saw a problem where ... bro obviously bit 1 is on but that thing ain't happening, that means A, B, C.
Being careful, asking questions, making lists of what you know / don't know, iterating LOGICALLY (for the love of god change one thing at a time). That's how you solved big problems I found.
Sometimes your skills aren't super smarts, super flashy code, sometimes, knowing every method off the top of your head, sometimes you can excel just being more careful, thinking different.4 -
First Happy new year, now lets get put on the dancing shoes... (I have another one coming, but this one is fresh)
As a PHP developer (yeah I am and I like it, if you gonna hate on me... go fuck yourself) I expect to not be required to reinvent the wheel when I have to use something that is not too mainstream (in my case was producing JSON and XML HAL responses). Now there are 2 (fairly active and somewhat mature), one of which does not produce XML responses, so off I went with the other one, but for fucks sake it does not produce XML that is compliant with the (draft)RFC (https://tools.ietf.org/html/...)
So as I need that, I decided to write one myself, since extending the one that provided XML would've been a waste of time, since it is NOT documented and for some reason depends on about 4 packages (also developed by the same maintainer), why the whining you ask, eh? Well fuck this shit. It took me 2(+2 classes) to achieve everything (according to standard as far as I can tell) + went with using a "hydrator" as opposed to reflection (the lib used reflection and didn't care too much for the access modified on the property of the object being serialized) so got a pretty solid performance boost, cleaner and simple code (I wrote it for a few hours and it is ugly, but hey KISS and it works perfectly)...
So with the more ranty part of this rant... Why the fuck so many people don't write independant packages for the simple parts... I don't hate it when I need a package and end up downloading half of the codebase of symfony or whatever fancy framework the dev decided to use, wasn't it the point of having 'package managers' (composer, npm, etc.. you get the deal..) instead of promote our projects and not force others to use our favorite framework that is absolutely out of scope for their projects...
Fuck you, fuck me and fuck everybody... If this continues I will continue writing my own packages from scratch, because "you" asshole are too lazy to learn and apply SOLID and common sense; even if your life depends on it you cannot write a meaningful piece of code without "the fancy framework of the month" holding your hand and allowing you to continue being a dumbass that has enough brain cells to walk straight and remember that you have to go to the toilet and not shit all over the place....
FML.... Fuck this shit and that is the main reason my gears grind the most when I head "you should use *framework name* instead" or "don't reinvent the wheel", fuck that guy I refuse to work my ways around a framework in order to get things done, my boss aint happy for that shit you know, I don't get paid to deal with your crappy code or uninformed opinion..3 -
I think I'm a good developer. I have pretty decent debugging skills, including pulling apart disassembled x86 and other architecture code.
I'm fascinated by how things work.
But almost everything is catered for by a library. Or has already been done.
I find it enjoyable to create a library or program myself, but get disheartened when I find some library or program that is written seemingly very well, compared to my own code. And then I start to think I'm not a good developer after all.
Sort of relates to my previous rant about repeatedly rewriting code.
Applies to me doing programming as a hobby but probably affects my code at work as well... I just can't help but think my code is probably awful compared to what someone else might write.
...then I see incredibly ugly, messy, badly written code by other people and I feel better...
I suppose it is like an artist who sees amazing works but cannot paint to that standard, but is well beyond drawing stick figures with crayons.
Sounds like a trivial problem but it probably impedes my progress with a lot of things.3 -
So I do not get why people use ReactJS. I hate it. for 3 years passionately. And I have to work with it every day.
- one-way data binding
this makes you write twice as much code, which will have twice as much bugs, you need to read through twice as much code from other devs.
- mixing html and JS
after all I like to pour my coffee on my omlette so I can eat and drink at the same time in the morning. This kills productivity and ugly AF
- not unified
Every dev uses their own special snowflake framework with React there is no unified way of doing things and you cannot use your familiar tools. Every project you need to start over from zero.
- Bugs bugs bugs
infinite loops, max update depth reached, key not present on list element. Let me ask you something dear ReactJS. If you know that there should be a unique key on that element. Why cannot you just put it there and shut the f up?
- works reeaally slow when compiled with TS
ReactJS was never designed to work with TS and now the tools for it are really slow. And why TS? Explicit contract is always better than an implicit contract. TS helps you in coding time, but for some reason React devs decided to worth 3 seconds to wait for compile and then realize you mad an error. ReactJS is bad and inefficient so stop making projects with it please.9 -
I really hate PHP frameworks.
I also often write my own frameworks but propriety. I have two decades experience doing without frameworks, writing frameworks and using frameworks.
Virtually every PHP framework I've ever used has causes more headaches than if I had simply written the code.
Let me give you an example. I want a tinyint in my database.
> Unknown column type "tinyint" requested.
Oh, doctrine doesn't support it and wont fix. Doctrine is a library that takes a perfectly good feature rich powerful enough database system and nerfs it to the capabilities of mysql 1.0.0 for portability and because the devs don't actually have the time to create a full ORM library. Sadly it's also the defacto for certain filthy disgusting frameworks whose name I shan't speak.
So I add my own type class. Annoying but what can you do.
I have to try to use it and to do so I have to register it in two places like this (pseudo)...
Types::add(Tinyint::class);
Doctrine::add(Tinyint::class);
Seems simply enough so I run it and see...
> Type tinyint already exists.
So I assume it's doing some magic loading it based on the directory and commend out the Type::add line to see.
> Type to be overwritten tinyint does not exist.
Are you fucking kidding me?
At this point I figure out it must be running twice. It's booting twice. Do I get a stack trace by default from a CLI command? Of course not because who would ever need that?
I take a quick look at parent::boot(). HttpKernel is the standard for Cli Commands?
I notice it has state, uses a protected booted property but I'm curious why it tries to boot so many times. I assume it's user error.
After some fiddling around I get a stack trace but only one boot. How is it possible?
It's not user error, the program flow of the framework is just sub par and it just calls boot all over the place.
I use the state variable and I have to do it in a weird way...
> $booted = $this->booted;parent::boot();if (!$booted) {doStuffOnceThatDependsOnParentBootage();}
A bit awkward but not life and death. I could probably just return but believe or not the parent is doing some crap if already booted. A common ugly practice but one that works is to usually call doSomething and have something only work around the state.
The thing is, doctrine does use TINYINT for bool and it gets all super confused now running commands like updates. It keeps trying to push changes when nothing changed. I'm building my own schema differential system for another project and it doesn't have these problems out of the box. It's not clever enough to handle ambiguous reverse mappings when single types are defined and it should be possible to match the right one or heck both are fine in this case. I'd expect ambiguity to be a problem with reverse engineer, not compare schema to an exact schema.
This is numpty country. Changing TINYINT UNSIGNED to TINYINT UNSIGNED. IT can't even compare two before and after strings.
There's a few other boots I could use but who cares. The internet seems to want to use that boot function. There's also init stages missing. Believe it or not there's a shutdown and reboot for the kernel. It might not be obvious but the Type::add line wants to go not in the boot method but in the top level scope along with the class definition. The top level scope is run only once.
I think people using OOP frameworks forget that there's a scope outside of the object in PHP. It's not ideal but does the trick given the functionality is confined to static only. The register command appears to have it's own check and noop or simply overwrite if the command is issued twice making things more confusing as it was working with register type before to merely alias a type to an existing type so that it could detect it from SQL when reverse engineering.
I start to wonder if I should just use columnDefinition.
It's this. Constantly on a daily basis using these pretentious stuck up frameworks and libraries.
It's not just the palava which in this case is relatively mild compared to some of the headaches that arise. It's that if you use a framework you expect basic things out of the box like oh I don't know support for the byte/char/tinyint/int8 type and a differential command that's able to compare two strings to see if they're different.
Some people might say you're using it wrong. There is such a thing as a learning curve and this one goes down, learning all the things it can't do. It's cripplesauce.12 -
A long time ago you sent me an email with the subject 'I love you', I then got so excited that I forwarded the letter to all my contacts, and they forwarded it too.. I can't describe the words for the feelings I had back then for you. I felt into love with you, really. But there were always troubling moments for me.
For example when 'Code Red' showed up and found your backdoor. Man I was pissed at that time. I didn't know what to do next. But things settled, and we found each other again.
And then that other time when this girl named 'Melissa' was sending me some passwords to pr0n sites, I couldn't resist. She was really awesome, but you know, deep in my heart that was not what I wanted. I somehow managed to go back to you and say sorry. We even moved together in our first flat, and later in our own house. That was a really good time, I love to think back at those moments.
Then my friend 'Sasser' came over to us one night, do you remember how he claimed that big shelf in our living room, and overflooded it with his own stuff, so that we haven't a clue we are reading yet offshelve? Wow that was a disturbing experience.
But a really hard time has come when our dog 'Zeus' got kicked by this ugly trojan horse. I really don't want go into details how the mess looked like after we discovered him on our floor. Still, I am very sorry for him that he didn't survived it :(
Some months later this guy named 'Conficker' showed up one day. I shitted my pants when I discovered that he guessed my password on my computer and got access to all my private stuff on it. He even tried to find some network shares of us with our photos on it. God, I was happy that he didn't got access to the pics we stored there. Never thought that our homemade photos are not secure there.
We lived our lives together, we were happy until that day when you started the war. 'Stuxnet..'! you cried directly in my face, 'you are gonna blow up our centrifuges of our life', and yeah she was right. I was in a real bad mood that days back then. I even not tried to hide my anger. But really, I don't know why all this could happen. All I know is, that it started with that cool USB stick I found on the stairs of our house. After that I don't remember anything, as it is just erased from my memory.
The years were passing. And I say the truth here, we were not able to manage the mess of our relationship. But I still loved you when you opened me that you will leave. My 'Heartbleed' started immediately, you stabbed it where it causes the most pain, where I thought that my keys to your heart are secured. But no, you stabbed even harder.
Because not long after that you even encrypted our private photos on our NAS, and now I am really finished, no memory which can be refreshed with a look at our pictures, and you even want my money. I really 'WannaCry' now... -
In SublimeText, I noticed that my markdowns formatting was not showing up correctly— I decided to download the new markdown package altogether hoping for some kind of update/fix. Turns out the package comes with a super ugly color theme which overrides the default theme of SublimeText. After some googling and experimenting, I found way to override this through the package settings. I always use git through my terminal but I thought let’s try to use git through my code editor and see how it works. I downloaded the git package but then I notice that git tool shows status and all correctly but doesn’t push files to GitHub (it says fatal: unable to read current working directory). Then I download another application called SublimeMerge. It works correctly on its own (pushes files to GitHub) but SublimeText is still not doing the same. Then I tinker around with my SSH keys hoping for a fix, but nothing works. I even go to stackoverflow and search for a solution but I find nothing (I even wrote a post asking for a solution but no replies till now). Fuck it! I now open the file with VSCode. Open terminal within VSCode and add/push/commit through it and everything works perfectly. So goodbye SublimeText I guess 👋🏾11
-
I'm shitting there hammering out some code butchering some real problems when I suddenly realise I'm surrounded. I look around and yes it's the bloody committee.
The committee is what I call the rest of the department and it is dominated by the old guard which comprises of the programmers that have been around for longer.
None of the old guard can program particularly well but because they had been around the longest they'd all grown senior. The committee had free reign but anyone else doing anything differently has to get approval from the committee.
The only way to code otherwise was to copy and paste existing code then to primarily rename things. If anyone did anything that hadn't been seen before then it would have to be approved by the committee. Individual action was not permitted unless you were old guard.
I swept my headphones away expecting it to be something unimportant. It was.
First things first they announce. We're going to add extraneous commas to the last element of all possible lists separated by comma including parameters or so they say. Ask but why so I do.
Because the language now supports it. They added support for it so it must be the right way someone proclaimed. Does it? I didn't realise we were waiting for it. Why do we want it though?
Didn't you hear? It's all over the blogosphere. It massively improves merge requests. But how I ask?
Five minutes later I grow tired of the chin stroking, elbow harnessing, slanted gazes into the yonder and occasionally hearing maybe its because and ask if they mean when you for example add an element the last element registers as changed from adding a comma. Turns out that's all it is.
How often do we see that tiny distraction and isn't it pointless to make the code ugly just for a tiny transient reduction in diff noise I ask. Everyone's stumped. This went on and on and got worse and worse. But it makes moving things around easy half of them say in unison like the bunch of slobs that they are. I mean really. It doesn't make expanding and contracting statements from multiline to single line easy and it's such a stupid thing. Is that all they do all day? Move multi-line method parameters up and down all day? If their coding conventions weren't totally whack they wouldn't have so many multiline method prototypes with stupid amounts of parameters with stupidly long types and names. They all use the same smart IDE which can also surely handle fixing the last comma and why is that even a concern given all the other outrageously verbose and excessive conventions for readability?
But you know what, who cares, fine, whatever. Lets put commas all over the shop and then we can all go to the pub and woo the ladies with how cool and trendy we are up to date with all the latest trends and fashions then we go home with ten babes hanging off each arm and get so laid we have to take a sick day the following to go to the STD clinic. Make way for we are conformists.
But then someone had to do it. They had to bring up PSR. Yes, another braindead committee that produces stupid decisions. Should brackets be same line or next line, I know, lets do both they decided. Now we have to do PSR and aren't allowed to use sensible conventions.
But why, I ask after explaining it's actually quite useful as a set of documents we can plagiarise as a starting point but then modify but no, we have to do exactly what PSR says. We're all too stupid apparently you see. Apparently we're not on their level. We're mere mortals. The reason or so I'm told, is so that anyone can come in and is they know PSR coding styles be able to read and write the code. That's not how it works. If you can't adjust to a different style, a more consistent style, that's not massively bizarre or atypical but rather with only minor differences from standard styles, you're useless. That's not even an argument, it's a confession that you've got a lump of coal where your brain's supposed to be.
Through all of this I don't really care because I long ago just made my own code generators or transpilers that work two ways and switch things between my shit and their shit but share my wisdom anyway because I'm a greedy scumbag like that.
Where the shit really hit the fan is that I pointed out that PSR style guide doesn't answer all questions nor covers all cases so what do we do then. If it's not in PSR? Then we're fucked.4 -
Any other language: Hey fuckface, you can't name this variable by a single letter, tf is wrong with you? use some descriptive shit.
Golang: lmao fuck u
I really find it interesting how we use short variable names for items in golang. Kinda makes sense when you think of it. Most of these items come up in short methods for which the mental model lets you know and remember what you are doing, they even make sense when going through the std lib in which that shit is all over the place. YET years of going by other languages has made me squint my eyes a bit in frustration every time I see it.
Say for example that a function is implementing io.Writer. What would you call the method parameter? you could argue that writer would be sensible since it has it in the signature, but what about when the io.Writer in itself is a file or a socket or whatever? writer would be funny or strange? nah fuck it just w, it makes sense, but x wouldn't. I find these points to make sense even if i don't like them.
Would, now, this practice be acceptable in C? you are supposed to write the same modular code with C in which you compose large functionality in separated units of code, yet I am sure this practice of single name variables is something that C engineers dislike greatly.
Are go devs just doing this out of blind love for their preference in languages? and how would this work if mfkers add generics to go(I hope not, Go is simple enough to understand in order to extend functionality through the empty interface, but that is a preference of mine as well)
The more I use Go the more I like it to be honest, I think the code looks ugly syntactically, but that is subjective as all hell and based on my constant preference for a language to look like Ruby, which even though it might not be everyone's cup of tea it remains to my eyes as the most beautiful language in existence, again, an obvious personal preference.18 -
Have you ever gotten a task where you have to modify some existing code, and to get it to work the way it needs to you have to write some ugly ass code?
And I'm talking FUGLY ass code. The kind where every brain cell you have screams to refactor it all so that your code won't be so ugly and you can live with yourself. But you only wrote it that way because some numbnuts who was fired a year ago designed it that way, and left zero commentary or documentation on his reasoning ("sELf-dOcUmeNtiNg cOde, bRuH!").
It doesn't pose any sort of risk with regards to security or resource management or efficiency, or really even faulty logic. It just looks fucking awful, my brain can instantly see better ways to design it and I don't want history to tie my name to it.
But also the system is being gutted and retired within a matter of months, so maintenance won't even be a concern; and you know that you have a lot of other large tasks that need your attention too, and to refactor will ultimately prove to be a time sink.
I mean ultimately, I know what I need to do, but I guess it's a pride thing. Just makes me feel icky. -
"Delete all code!" That should be the mantra!
Was watching some stuff from destroyallsoftware.com. Not entirely convinced. So I should cook up my own shit.
So here is how the argument goes:
There's quite some negativity in the term "legacy" software. Partly it may be the envy to software that runs on actual machines and is not that phantasm, that perfect first lines on a greenfield project until it gets messed up as it has to put up with all the real world messiness. But the negativity it deserves is actually for the code that we cannot get rid of. This ugly class or function that soaked all the complexity and functionality so it defies any positive change. And always when it appears on your screen, it irks you, enrages you, makes you punch the screen, because you can almost feel the distaste physically. - *That* is the definition of "legacy" in its true negativity. No software should be like that. On the contrary. Every line should be replaceable, dispensable, disposable. At the verge to deletable. Because you know: the best code is no code.
This is where my hatred of code could get productive: Delete all the wretched, loathsome stuff and replace it, with something that just sucks less and can be thrown away any time. Don't expect beauty or perfect design. It'll never finish.3 -
Data wrangling is messy
I'm doing the vegetation maps for the game today, maybe rivers if it all goes smoothly.
I could probably do it by hand, but theres something like 60-70 ecoregions to chart,
each with their own species, both fauna and flora. And each has an elevation range its
found at in real life, so I want to use the heightmap to dictate that. Who has time for that? It's a lot of manual work.
And the night prior I'm thinking "oh this will be easy."
yeah, no.
(Also why does Devrant have to mangle my line breaks? -_-)
Laid out the requirements, how I could go about it, and the more I look the more involved
it gets.
So what I think I'll do is automate it. I already automated some of the map extraction, so
I don't see why I shouldn't just go the distance.
Also it means, later on, when I have access to better, higher resolution geographic data, updating it will be a smoother process. And even though I'm only interested in flora at the moment, theres no reason I can't reuse the same system to extract fauna information.
Of course in-game design there are some things you'll want to fudge. When the players are exploring outside the rockies in a mountainous area, maybe I still want to spawn the occasional mountain lion as a mid-tier enemy, even though our survivor might be outside the cats natural habitat. This could even be the prelude to a task you have to do, go take care of a dangerous
creature outside its normal hunting range. And who knows why it is there? Wild fire? Hunted by something *more* dangerous? Poaching? Maybe a nuke plant exploded and drove all the wildlife from an adjoining region?
who knows.
Having the extraction mostly automated goes a long way to updating those lists down the road.
But for now, flora.
For deciding plants and other features of the terrain what I can do is:
* rewrite pixeltile to take file names as input,
* along with a series of colors as a key (which are put into a SET to check each pixel against)
* input each region, one at a time, as the key, and the heightmap as the source image
* output only the region in the heightmap that corresponds to the ecoregion in the key.
* write a function to extract the palette from the outputted heightmap. (is this really needed?)
* arrange colors on the bottom or side of the image by hand, along with (in text) the elevation in feet for reference.
For automating this entire process I can go one step further:
* Do this entire process with the key colors I already snagged by hand, outputting region IDs as the file names.
* setup selenium
* selenium opens a link related to each elevation-map of a specific biome, and saves the text links
(so I dont have to hand-open them)
* I'll save the species and text by hand (assuming elevation data isn't listed)
* once I have a list of species and other details, to save them to csv, or json, or another format
* I save the list of species as csv or json or another format.
* then selenium opens this list, opens wikipedia for each, one at a time, and searches the text for elevation
* selenium saves out the species name (or an "unknown") for the species, and elevation, to a text file, along with the biome ID, and maybe the elevation code (from the heightmap) as a number or a color (probably a number, simplifies changing the heightmap later on)
Having done all this, I can start to assign species types, specific world tiles. The outputs for each region act as reference.
The only problem with the existing biome map (you can see it below, its ugly) is that it has a lot of "inbetween" colors. Theres a few things I can do here. I can treat those as a "mixing" between regions, dictating the chance of one biome's plants or the other's spawning. This seems a little complicated and dependent on a scraped together standard rather than actual data. So I'm thinking instead what I'll do is I'll implement biome transitions in code, which makes more sense, and decouples it from relying on the underlaying data. also prevents species and terrain from generating in say, towns on the borders of region, where certain plants or terrain features would be unnatural. Part of what makes an ecoregion unique is that geography has lead to relative isolation and evolutionary development of each region (usually thanks to mountains, rivers, and large impassible expanses like deserts).
Maybe I'll stuff it all into a giant bson file or maybe sqlite. Don't know yet.
As an entry level programmer I may not know what I'm doing, and I may be supposed to be looking for a job, but that won't stop me from procrastinating.
Data wrangling is fun.1 -
been exploring the options for cross platform desktop app, and i found :
java : both awt and swing look ugly, i really like OOP of java, and the way projects are organized is easy to scale, but i need to deploy the jdk, and the speed on gui apps isn't that great
C# : (.net/ mono, i can't grasp F# and vb is stupid) looks native on windows, not so much alien on both linux/mac, and being a java cousin is a pro, i found the Eto library for mono even looks more native on *ix than winforms
wxwidgets: for C/C++ so far this looks like the best option for total native feel and performance, but man i fucking hate C code, and this looks a lot like C code, even with proper native Cpp support, maybe i should dive deeper in it
GTK+ : did any one mention C code ? because this mother fucker is plain C with macros all over the place, it made me realize why wx is promoted as Cpp friendly, i doubt I'll use this
tcl/tk : even tho ive never wrote a single line of tcl in my life, the tk lib is the default ui for both python and ruby on all supported platforms,
and i really love ruby, and Python is Usually a joy to work with
Qt : this by far looks like the best option, proper OOP in C++, bindings for python (ruby binds are outdated), almost native look and feel on supported platforms, and even has a gui builder in xml or json/js (qml) however i bet I'll use such a thing, the building tho depends on an external preprocessor "moc" and some wicked macros, also makes working with templates a fucking mess, and the heavy dependence on QObject inheritance makes integrating external libraries a bit more tiring, the signal slot system makes more sense in python than in C++, since it makes me confused about the flow of the code
lazarus: is a freepascal implementation that looks and feels like delphi, not so much for native look and feel, but good performance and easy language to handle
electron : this fat mofo is fat, it's the slowest of all options, if i want an html app, I'll just compile a stripped down webkit and deploy that
what do you think ? and did i miss something ?17 -
I hate Intellij Idea but it's best option available to develop in Scala. Improvements in VSCode/Metals is my last hope.
The (few) things I NEED from Intellij:
* Very good autocompletion
* Refactoring tools (renaming, auto imports)
* Search tools (find usages, sub/super-types)
The (many) things I hate of Intellij:
* Layout with panel sizes doesn't behave properly and it scales instead of remaining fixed.
* Tedious 2-hands shortcuts makes the right hand to move a lot from the mouse
* Delays and lag in the UI, freezes on garbage collection
* High memory consumption, high CPU usage and generally slow and cumbersome
* The delay in the UI between commands is so that it's accidentally possible to introduce typos
* Can't move tabs around and organize them as I like
* Ugly font rendering and missing typography settings
* Multi-caret implementation as a different editing mode is annoying because requires frequent switching
* Unnatural code folding regions, why method arguments are not folded with the method?
* Unhelpful support forum, sometimes dismissive answers
* Highlighting of current word under the caret doesn't work
* Very slow editor, can't keep spacebar pressed to move text or it hangs!
* Several settings reset at every update. Like the auto fetch of git
* New features are added and enabled by default which is very invasive
* Some of the features mentioned above are really annoying and it's not possible/not trivial to disable them
* It uses its own compile and several times it highlights false positives7 -
So I have a website as a personal project that has a decent amount of visitors each day. The codebase, however, is really ugly because it's something I made very fast in my spare time three years ago.
Over the past six months, I have been working on a completely new version of the website with a better layout and much nicer backend code.
At the moment I'm pretty sure the new website is ready to deploy. I even asked some friends who tested the website very thoroughly and came up with some minor bugs.
But now I'm really stressed to deploy the new website and I keep postponing it. What if I forgot a stupid error? What if some mobile part doesn't work? What if the new website isn't as SEO friendly as the current and I lose my visitors? 😱2 -
"if compiler can infer this, there is no need to add "x ->" , simply use it" ..AAAGHH FUCK YOUUUUU KOTLIN!! what else should i fucking not write? why do't you take a number of my employee and ask his requirements, maybe add a ShoppingKartApp.kt in your compiler next time? it will be completely inferred when i write "Fuck you" in the gradle.
And fucking companies are promoting this! I wonder how those devs are living there
Person A knows only that lambda is
{name:Type,name:Type->code}, and thus writes a clean code.
Person B comes says "This shit suck", writes "{ acc, i -> acc + " " + i }" ,goes away
Person A : "wtf is this shit? why it works?"
Please for the love of god, follow some rules! My first language was python, i love its zen:
- Beautiful is better than ugly.
- Simple is better than complex.
- Readability counts.
- Special cases aren't special enough to break the rules.
- There should be one-- and preferably only one --obvious way to do it.
- If the implementation is hard to explain, it's a bad idea.
-...
I just wish it follows at least one thing from python's zen : "There should be one-- and preferably only one --obvious way to do it."3 -
Sometimes I just code some ugly crap that does the job and create a To Do to revisit it later and try to make it elegant.
When I revisit the code I always can't believe that I thought it was bad.
Seems like my past Me is a better programmer than present Me. Ouch. -
Okay so there are a lot of things that are left by us students as "this would be taught to us on job, why bother now?" So i have many questions regarding this:
- is it a safe mentality? I mean University is teaching me, say a,b,c and the job is supposed to be like writing full letters, than am i stupid to stick to just a,b,c and not learning how to write letters beforehand?
- what is even "taught" on job? This is especially directed towards people in Big firms. I mean i can always blame that small ugly startup who treated me badly and not gave me any resources, but why do i feel its going to be same at every other company?
I guess no one is gonna teach me for 6 months on how to write classes with java, or make a ml engineer out of me when i don't know jack shit about ml.... That's the task for college, right?
I feel that when these companies say they "teach", you they mean how to follow instructions regarding agile meetings, how to survive office politics and how to learn quickly and produce an output quickly. I don't think that if i don't know how MVI works, then they are gonna teach me that, would they?i guess not unless they already have someone knowledgeable in that topic
- what about the things that are not taught in our colleges and we wanna make a career in it? Like say Android. From what i have experienced , choosing a career in a subject that's not taught you in grad school immediately takes away some kind of shield from you, as you are expected to know everything beforehand. So again, the same questions bfrom above
i did learned something from job life tho, and that too twice. Once it was when i first encountered an app sample for mvvm and once when i found out a very specific case of how video player is being used in a manner that handled a lot of bugs.
Why i didn't knew those approaches when i was not in job? Well, the first was a theoretical model whose practical implementation was difficult to find online that time and the second was a thing that i myself gave a lot of hours, yet failed to understand. However when i was in the company , i was partnered with a senior dev who himself had once spent 30 days with the source code to find a similar solution.
So again , both of above things could have been done by me had i spent more time trying to learn those "professional tools" and/or dwelve deeper into the tech. And i did felt pretty guilty not knowing about those...5 -
i am feeling angry and frustrated. not sure if it's a person ,or codebase or this bloody job. i have been into the company for 8 months and i feel like someone taking a lot of load while not getting enough team support to do it or any appreciation if i do it right.
i am not a senior by designation, but i do think my manager and my seniors have got their work easy when they see my work . like for eg, if on first release, they told me that i have to update unit tests and documentation, then on every subsequent release i did them by default and mentioning that with a small tick .
but they sure as hell don't make my work easy for me. their codebase is shitty and they don't give me KT, rather expect me to read everything on my own, understand on my own and then do everything on my own, then raise a pr , then merge that pr (once reviewed) , then create a release, then update the docs and finally publish the release and send the notification to the team
well fine, as a beginner dev, i think that's a good exercise, but if not in the coding step, their intervention would be needed in other steps like reviewing merging and releasing. but for those steps they again cause unnecessary delay. my senior is so shitty guy, he will just reply to any of my message after 2-3 hours
and his pr review process is also frustrating. he will keep me on call while reviewing each and every file of my pr and then suggest changes. that's good i guess, but why tf do you need to suggest something every fucking time? if i am doing such a shitty coding that you want me to redo some approach that i thought was correct , why don't you intervene beforehand? when i was messaging you for advice and when you ignored me for 3 hours? another eg : check my comment on root's rant https://devrant.com/rants/5845126/ (am talking about my tl there but he's also similar)
the tasks they give are also very frustrating. i am an android dev by profession, my previous company was a b2c edtech app that used kotlin, java11, a proper hierarchy and other latest Android advancements.
this company's main Android product is a java sdk that other android apps uses. the java code is verbose , repetitive and with a messed up architecture. for one api, the client is able to attach a listener to some service that is 4 layers down the hierarchy , while got other api, the client provides a listener which is kept as a weak reference while internal listeners come back with the values and update this weak reference . neither my team lead nor my seniors have been able to answer about logic for seperation among various files/classes/internal classes and unnecessary division of code makes me puke.
so by now you might have an idea of my situation: ugly codebase, unavailable/ignorant codeowners (my sr and TL) and tight deadlines.
but i haven't told you about the tasks, coz they get even more shittier
- in addition to adding features/ maintaining this horrible codebase , i would sometimes get task to fix queries by client . note that we have tons of customer representatives that would easily get those stupid queries resolced if they did their job correctly
- we also have hybrid and 3rd party sdks like react, flutter etc in total 7 hybrid sdks which uses this Android library as a dependency and have a wrapper written on its public facing apis in an equally horrible code style. that i have to maintain. i did not got much time/kt to learn these techs, but once my sr. half heartedly explained the code and now every thing about those awful sdls is my responsibility. thank god they don't give me the ios and web SDK too
- the worst is the shitty user side docs. I don't know what shit is going there, but we got like 4 people in the docs team and they are supposed to maintain the documentation of sdk, client side. however they have rasied 20 tickets about 20 pages for me to add more stuff there. like what are you guys supposed to do? we create the changelog, release notes , comments in pr , comments in codebase , test cases, test scenarios, fucking working sample apps and their code bases... then why tf are we supposed to do the documentation on an html based website too?? can't you just have a basic knowledge of running the sample, reading the docs and understand what is going around? do i need to be a master of english too in addition to being a frustrated coder?
just.... fml -
I've been working on this personal project for awhile, I showed some screenshots, then I showed some updates (which I promptly deleted cause it was plain ugly). A website aimed at the "not-so-seasoned" devs, and I've been at a creative plateau for about 3 days now. I try to do some front-end, when I like what I see, I take care of the corresponding server-side logic, but for some reason, I'm having "Developer's block" (is that even a thing?).
Every second that goes by I try to do something else none code related, but I can't shake thinking about the project, but once I switch back to it, fuckin crickets.
I'm not asking a question this time (for once lol), just a mini dev's block rant. -
i always get sucked into this "cute code" hell whenever i am working with a b2c codebase, and especially with kotlin code.
here's a scenario:
task : build a debounce logic for an input view where each user input is currently triggerring an api call.
my steps
1. read what debouncing is.
2. see if any code is available on the internet
=> found a code piece on the internet with some level of abstraction ( basically a simple final class that implements the input event callback and encapsulates the debounce logic)
3) copy it, run it , it wokrs
------
for any sane coder, these steps are hardly 10-30 mins and they can move on with life. but its your truly that made this task into a 6hour research only to come up at similar solution. my curiosity led me to stupid places
1) why this class is final? what if someone else wanna use it but with a different behaviour? lets try open(non final class) .
2) why even use a class? it extends an interface, lets try to wrap the logic in interface itself (kotlin supports interfaces that don't require implementation)
3) umm , the interface works but it looks ugly, with all its global overridden variables. what about we make it extension?
4) yeah the extension approach is also not very good, lets go back to open class.
5) but extend is super nice to look! lets keep the extension and open class too
6) can we optimise the implementation? why it uses an additional handler? what if we provided everything in constructor? how about builder pattern?
FUCK MY BRAIN! there are so much fucking options that i forgot that i spent 4 hours on this small thing
the simplest approach would have been tk just shove all the listeners and everything in activity and forget about it :/
senior devs on this platform, how do you stop yourself from adding every concept that you know into the smallest possible task?6