Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
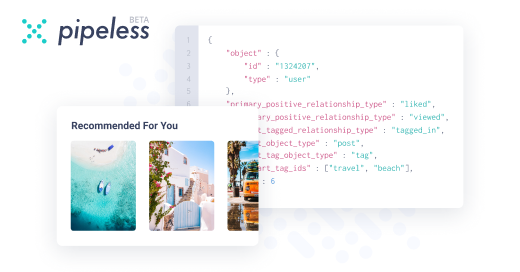
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "error loop"
-
I have an array of 1037 records. The soap service only accepts 100 at a time. So, I write code to send an array of 100 records at a time to the soap service in a loop and get a response back of, "The maximum number (100) of records allowed for this operation has been exceeded." Well, I'll try 99 records then. Nope same error. I'll try 50 records, nope I'll just bang my head on the desk now since the documentation and error say it is a record limit of 100. 😠
Look at my code again. I was grabbing 100 records out of the array of 1037 records and storing it in a new array, but I was sending the original array with 1037 records instead of the temporary array in the loop. 😢 I'm going to bed.7 -
Less a rant, more just a sad story.
Our company recently acquired its sister company, and everyone has been focused on improving and migrating their projects over to our stack.
There's a ton of material there, but this one little story summarizes the whole very accurately, I think. (Edit: two stories. I couldn't resist.)
There's a 3-reel novelty slot machine game with cards instead of the usual symbols, and winnings based on poker-like rules (straights and/or flushes, 2-3 of a kind, etc.) The machine is over a hundred times slower than the other slot machines because on every spin it runs each payline against a winnings table that exhastively lists every winning possibility, and I really do mean exhaustively. It lists every type of win, for every card, every segment for straights, in every order, of every suit. Absolutely everything.
And this logic has been totally acceptable for just. so. long. When I saw someone complaining in dev chat about how much slower it is, i made the bloody obvious suggestion of parsing the cards and applying some minimal logic to see if it's a winning combination. Nobody cared.
Ten minutes later, someone from the original project was like "Hey, I have an idea, why don't we do it algorithmically to not have a 4k line rewards table?"
He seriously tried stealing a really bloody obvious idea -- that he hadn't had for years prior -- and passing it off as his own. In the same chat. Eight messages below mine. What a derpballoon.
I called him out on it, and he was like "Oh, is that what you meant by parsing?" 🙄
Someone else leaped in to defend the ~128x slower approach, saying: "That's the tech we had." You really didn't have a for loop and a handful of if statements? Oh wait, you did, because that's how you're checking your exhaustive list. gfj. Abysmal decisions like this is exactly why most of you got fired. (Seriously: these same people were making devops decisions. They were hemorrhaging money.)
But regardless, the quality of bloody everything from that sister company is like this. One of the other fiascos involved pulling data from Facebook -- which they didn't ever even use -- and instead of failing on error/unexpected data, it just instantly repeated. So when Facebook changed permissions on friends context... you can see where this is going. Instead of their baseline of like 1400 errors per day, which is amazingly high, it spiked to EIGHTEEN BLOODY MILLION PER DAY. And they didn't even care until they noticed (like four days later) that it was killing their other online features because quite literally no other request could make it out. More reasons they got fired. I'm not even kidding: no single api request ever left the users' devices apart from the facebook checks.
So.
That's absolutely amazing.7 -
I was taking an introductory programming course. One assignment was to do a little payroll program, including some data validation. The program was supposed to accept terminal input and send output back to either the console or a printer.
Suddenly the printer began spewing out paper like crazy. One of the students (a particularly mouthy woman) had programmed a less-than-helpful error message ("YOU ARE WRONG") and then not provided any exit from the error-checking logic -- the program just re-read the last (failing) input and re-tested it. All in all, it was a very nice infinite loop.
After spitting through about fifty pages of "YOU ARE WRONG," somebody cut power to the printer, and the instructor had to flush the print queue manually. He went back to the student and asked if she had tested the program by sending the output to the console before trying to print it, and she said, yes, she had tested it on the console and ended up with a screen full of "YOU ARE WRONG" messages. Why, then, had she sent her output to the printer? "I thought I would be daring!"7 -
Okay, story time.
Back during 2016, I decided to do a little experiment to test the viability of multithreading in a JavaScript server stack, and I'm not talking about the Node.js way of queuing I/O on background threads, or about WebWorkers that box and convert your arguments to JSON and back during a simple call across two JS contexts.
I'm talking about JavaScript code running concurrently on all cores. I'm talking about replacing the god-awful single-threaded event loop of ECMAScript – the biggest bottleneck in software history – with an honest-to-god, lock-free thread-pool scheduler that executes JS code in parallel, on all cores.
I'm talking about concurrent access to shared mutable state – a big, rightfully-hated mess when done badly – in JavaScript.
This rant is about the many mistakes I made at the time, specifically the biggest – but not the first – of which: publishing some preliminary results very early on.
Every time I showed my work to a JavaScript developer, I'd get negative feedback. Like, unjustified hatred and immediate denial, or outright rejection of the entire concept. Some were even adamantly trying to discourage me from this project.
So I posted a sarcastic question to the Software Engineering Stack Exchange, which was originally worded differently to reflect my frustration, but was later edited by mods to be more serious.
You can see the responses for yourself here: https://goo.gl/poHKpK
Most of the serious answers were along the lines of "multithreading is hard". The top voted response started with this statement: "1) Multithreading is extremely hard, and unfortunately the way you've presented this idea so far implies you're severely underestimating how hard it is."
While I'll admit that my presentation was initially lacking, I later made an entire page to explain the synchronisation mechanism in place, and you can read more about it here, if you're interested:
http://nexusjs.com/architecture/
But what really shocked me was that I had never understood the mindset that all the naysayers adopted until I read that response.
Because the bottom-line of that entire response is an argument: an argument against change.
The average JavaScript developer doesn't want a multithreaded server platform for JavaScript because it means a change of the status quo.
And this is exactly why I started this project. I wanted a highly performant JavaScript platform for servers that's more suitable for real-time applications like transcoding, video streaming, and machine learning.
Nexus does not and will not hold your hand. It will not repeat Node's mistakes and give you nice ways to shoot yourself in the foot later, like `process.on('uncaughtException', ...)` for a catch-all global error handling solution.
No, an uncaught exception will be dealt with like any other self-respecting language: by not ignoring the problem and pretending it doesn't exist. If you write bad code, your program will crash, and you can't rectify a bug in your code by ignoring its presence entirely and using duct tape to scrape something together.
Back on the topic of multithreading, though. Multithreading is known to be hard, that's true. But how do you deal with a difficult solution? You simplify it and break it down, not just disregard it completely; because multithreading has its great advantages, too.
Like, how about we talk performance?
How about distributed algorithms that don't waste 40% of their computing power on agent communication and pointless overhead (like the serialisation/deserialisation of messages across the execution boundary for every single call)?
How about vertical scaling without forking the entire address space (and thus multiplying your application's memory consumption by the number of cores you wish to use)?
How about utilising logical CPUs to the fullest extent, and allowing them to execute JavaScript? Something that isn't even possible with the current model implemented by Node?
Some will say that the performance gains aren't worth the risk. That the possibility of race conditions and deadlocks aren't worth it.
That's the point of cooperative multithreading. It is a way to smartly work around these issues.
If you use promises, they will execute in parallel, to the best of the scheduler's abilities, and if you chain them then they will run consecutively as planned according to their dependency graph.
If your code doesn't access global variables or shared closure variables, or your promises only deal with their provided inputs without side-effects, then no contention will *ever* occur.
If you only read and never modify globals, no contention will ever occur.
Are you seeing the same trend I'm seeing?
Good JavaScript programming practices miraculously coincide with the best practices of thread-safety.
When someone says we shouldn't use multithreading because it's hard, do you know what I like to say to that?
"To multithread, you need a pair."18 -
EEEEEEEEEEEE Some fAcking languages!! Actually barfs while using this trashdump!
The gist: new job, position required adv C# knowledge (like f yea, one of my fav languages), we are working with RPA (using software robots to automate stuff), and we are using some new robot still in beta phase, but robot has its own prog lang.
The problem:
- this language is kind of like ASM (i think so, I'm venting here, it's ASM OK), with syntax that burns your eyes
- no function return values, but I can live with that, at least they have some sort of functions
- emojies for identifiers (like php's $var, but they only aim for shitty features so you use a heart.. ♥var)
- only jump and jumpif for control flow
- no foopin variable scopes at all (if you run multiple scripts at the same time they even share variables *pukes*)
- weird alt characters everywhere. define strings with regular quotes? nah let's be [some mental illness] and use prime quotes (‴ U+2034), and like ⟦ ⟧ for array indexing, but only sometimes!
- super slow interpreter, ex a regular loop to count to 10 (using jumps because yea no actual loops) takes more than 20 seconds to execute, approx 700ms to run 1 code row.
- it supports c# snippets (defined with these stupid characters: ⊂ ⊃) and I guess that's the only c# I get to write with this job :^}
- on top of that, outdated documentation, because yea it's beta, but so crappin tedious with this trail n error to check how every feature works
The question: why in the living fartfaces yolk would you even make a new language when it's so easy nowadays to embed compilers!?! the robot is apparently made in c#, so it should be no funcking problem at all to add a damn lua compiler or something. having a tcp api would even be easier got dammit!!! And what in the world made our company think this robot was a plausible choice?! Did they do a full fubbing analysis of the different software robots out there and accidentally sorted by ease of use in reverse order?? 'cause that's the only explanation i can imagine
Frillin stupid shitpile of a language!!! AAAAAHHH
see the attached screenshot of production code we've developed at the company for reference.
Disclaimer: I do not stand responsible for any eventual headaches or gauged eyes caused by the named image.
(for those interested, the robot is G1ANT.Robot, https://beta.g1ant.com/)4 -
So Friday afternoon is always deployment time at my company. No sure why, but it always fucks us.
Anyways, last Friday, we had this lovely deployment that was missing a key piece. On Wednesday I had tested it, sent out an email(with screenshots) saying "yo, whoever wrote this, this feature is all fucked up." Management said they would handle it.
The response email. 1(out of 20) defects I sent in were not a defect but my error. No further response, so I assume the rest were being looked into.
In a call with bossman, my manager states that the feature is fixed, so I go to check it quickly before the deployment(on Friday).
THERE IS NO FUCKING CODE CHECK-IN. THE DEV BASTARD JUST SAID THAT MY USECASE WAS WRONG, SO MY ENTIRE EMAIL WAS INVALID.
I am currently working on Saturday, as the other guy refuses to see the problem! It is blatant, and I got 3 other people to reproduce to prove I am not crazy!
On top of that, the code makes me want to vomit! I write bad code. This is like a 3rd grader who doesn't know code copy-pasted from stack overflow! There is literally if(A) then B else if(!A) then B! And a for loop which does some shit, and the line after it closes has a second for loop that iterates over the same unaltered set! Why?! On top of that, the second for loop loops until "i" is equal to length-1, then does something! Why loop???
The smartest part of him ran down his Mama's leg when it saw the DNA dad was contributing!
Don't know who is the culprit, and if you happen to see this, I am pissed. I am working on Saturday because you can't check your code or you lied on your resume to get this job, as you are not qualified! Fuck you!15 -
Recently wrote a script that would check 2 years worth of images, crop them, and resize to different sizes as changes to front end required those.
Eventually the script went into an infinite loop and crashed the whole CMS.
The worst part was that my manager was on a date and I had to call him back into office, since his laptop was still at the office.
The actual problem wasn't the loop.. I forgot to check if file actually exists before cropping... Error log size was 10gb!1 -
Wow i must have been brain dead when i wrote this code. Needed to exclude certain elements from response for the the list of objects.
for (obj : objects) {
If (obj.skipFromResponse()) {
break
}
add obj to response
}
I used break instead of continue at the if condition which meant it would break out of the loop at the first instance of condition being met.
This went through qa and has been in production for 4 weeks so how did this not break before. Well little did i know the list of objects was sorted and all the test data, qa data and everything so far in production coincidentally only had the last element with matching condition. This meant it returned everything correctly so far.
Today was the first time there was a situation where this caused incorrect output. Luckily as soon as I heard the description of the issue I remembered to check the merged PR and hung my head in shame for making such trivial error. I must have written way more complicated code without any problem but this made me embarrassed to even admit. 🤦♂️4 -
Today on "How the Fuck is Python a Real Language?": Lambda functions and other dumb Python syntax.
Lambda functions are generally passed as callbacks, e.g. "myFunc(a, b, lambda c, d: c + d)". Note that the comma between c and d is somehow on a completely different level than the comma between a and b, even though they're both within the same brackets, because instead of using something like, say, universally agreed-upon grouping symbols to visually group the lambda function arguments together, Python groups them using a reserved keyword on one end, and two little dots on the other end. Like yeah, that's easy to notice among 10 other variable and argument names. But Python couldn't really do any better, because "myFunc(a, b, (c, d): c + d)" would be even less readable and prone to typos given how fucked up Python's use of brackets already is.
And while I'm on the topic of dumb Python syntax, let's look at the switch, um, match statements. For a long time, people behind Python argued that a bunch of elif statements with the same fucking conditions (e.g. x == 1, x == 2, x == 3, ...) are more readable than a standard switch statement, but then in Python 3.10 (released only 1 year ago), they finally came to their senses and added match and case keywords to implement pattern matching. Except they managed to fuck up yet again; instead of a normal "default:" statement, the default statement is denoted by "case _:". Because somehow, everywhere else in the code _ behaves as a normal variable name, but in match statement it instead means "ignore the value in this place". For example, "match myVar:" and "case [first, *rest]:" will behave exactly like "[first, *rest] = myVar" as long as myVar is a list with one or more elements, but "case [_, *rest]:" won't assign the first element from the list to anything, even though "[_, *rest] = myVar" will assign it to _. Because fuck consistency, that's why.
And why the fuck is there no fallthrough? Wouldn't it make perfect sense to write
case ('rgb', r, g, b):
case ('argb', _, r, g, b):
case ('rgba', r, g, b, _):
case ('bgr', b, g, r):
case ('abgr', _, b, g, r):
case ('bgra', b, g, r, _):
and then, you know, handle r, g, and b values in the same fucking block of code? Pretty sure that would be more readable than having to write "handeRGB(r, g, b)" 6 fucking times depending on the input format. Oh, and never mind that Python already has a "break" keyword.
Speaking of the "break" keyword, if you try to use it outside of a loop, you get an error "'break' outside loop". However, there's also the "continue" keyword, and if you try to use it outside of a loop, you get an error "'continue' not properly in loop". Why the fuck are there two completely different error messages for that? Does it mean there exists some weird improper syntax to use "continue" inside of a loop? Or is it just another inconsistent Python bullshit where until Python 3.8 you couldn't use "continue" inside the "finally:" block (but you could always use "break", even though it does essentially the same thing, just branching to a different point).19 -
Running a fucking conda environment on windows (an update environment from the previous one that I normally use) gets to be a fucking pain in the fucking ass for no fucking reason.
First: Generate a new conda environment, for FUCKING SHITS AND GIGGLES, DO NOT SPECIFY THE PYTHON VERSION, just to see compatibility, this was an experiment, expected to fail.
Install tensorflow on said environment: It does not fucking work, not detecting cuda, the only requirement? To have the cuda dependencies installed, modified, and inside of the system path, check done, it works on 4 other fucking environments, so why not this one.
Still doesn't work, google around and found some thread on github (the errors) that has a way to fix it, do it that way, fucking magic, shit is fixed.
Very well, tensorflow is installed and detecting cuda, no biggie. HAD TO SWITCH TO PYHTHON 3,8 BECAUSE 3.9 WAS GIVING ISSUES FOR SOME UNKNOWN FUCKING REASON
Ok no problem, done.
Install jupyter lab, for which the first in all other 4 environments it works. Guess what a fuckload of errors upon executing the import of tensorflow. They go on a loop that does not fucking end.
The error: imPoRT eRrOr thE Dll waS noT loAdeD
Ok, fucking which one? who fucking knows.
I FUCKING HATE that the main language for this fucking bullshit is python. I guess the benefits of the repl, I do, but the python repl is fucking HORSESHIT compared to the one you get on: Lisp, Ruby and fucking even NODE in which error messages are still more fucking intelligent than those of fucking bullshit ass Python.
Personally? I am betting on Julia devising a smarter environment, it is a better language already, on a second note: If you are worried about A.I taking your job, don't, it requires a team of fucktards working around common basic system administration tasks to get this bullshit running in the first place.
My dream? Julia or Scala (fuck you) for a primary language in machine learning and AI, in which entire environments, with aaaaaaaaaall of the required dlls and dependencies can be downloaded and installed upon can just fucking run. A single directory structure in which shit just fucking works (reason why I like live environments like Smalltalk, but fuck you on that too) and just run your projects from there, without setting a bunch of bullshit from environment variables, cuda dlls installation phases and what not. Something that JUST FUCKING WORKS.
I.....fucking.....HATE the level of system administration required to run fucking anything nowadays, the reason why we had to create shit like devops jobs, for the sad fuckers that have to figure out environment configurations on a box just to run software.
Fuck me man development turned to shit, this is why go mod, node npm, php composer strict folder structure pipelines were created. Bitch all you want about npm, but if I can create a node_modules setting with all of the required dlls to run a project, even if this bitch weights 2.5GB for a project structure you bet your fucking ass that I would.
"YOU JUST DON'T KNOW WHAT YOU ARE DOING" YES I FUCKING DO and I will get this bullshit fixed, I will get it running just like I did the other 4 environments that I fucking use, for different versions of cuda and python and the dependency circle jerk BULLSHIT that I have to manage. But this "follow the guide and it will work, except when it does not and you are looking into obscure github errors" bullshit just takes away from valuable project time when you have a small dedicated group of developers and no sys admin or devops mastermind to resort to.
I have successfully deployed:
Java
Golang
Clojure
Python
Node
PHP
VB/C# .NET
C++
Rails
Django
Projects, and every single fucking time (save for .net, that shit just fucking works on a dedicated windows IIS server) the shit will not work with x..nT reasons. It fucking obliterates me how fucking annoying this bullshit is. And the reason why the ENTIRE FUCKING FIELD of computer science and software engineering is so fucking flawed.
But we can't all just run to simple windows bs in which we have documentation for everything. We have to spend countless hours on fucking Linux figuring shit out (fuck you also, I have been using Linux since I was 18, I am 30 now) for which graphical drivers for machine learning, cuda and whatTheFuckNot require all sorts of sys admin gymnasts to be used.
Y'all fucked up a long time ago. Smalltalk provided an all in one, easily rollable back to previous images, easily administered interfaces for this fileFuckery bullshit, and even though the JVM and the .NET environments did their best to hold shit down, and even though we had npm packages pulling the universe inside, or gomod compiling shit into one place NOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOOO we had to do whatever the fuck we wanted to feel l337 and wanted.
Fuck all of you, fuck this field, fuck setting boxes for ML/AI and fuck every single OS in existence2 -
Messed Up my first Coding Interview and that too of Google!
My first rant.
The first question was not an easy one. I cracked it though. Happy. Very Happy! I had 40 minutes left for the second question. And then came the nightmare. Okay, my foolishness.
I compiled my code. Compilation error.
Declared variables. Compilation Error!
Imported Libraries. Compilation Error!
Changed vector to an array. compilation Error!
Checked the loop for edge cases. Compilation Error!
Cannot use an IDE too. Tab's change is not allowed.
My score was still ZERO and I had only 15 minutes left.
Then lazily my eyes went to the language selected. It was C. I wrote the code in C++.
I mean HOW CAN I BE SOOOO STUPID??
I was coding in an entirely different language!
But..But, the story doesn't end here.
Next, I copied the code and switched languages. NOOO, my code was lost. I couldn't paste my code!!
I checked the timer- 5 minutes left.
Somehow, I managed to rewrite the code. And submitted it at the last minute.
I have no idea what will be the results. I just solved 1/2 questions.
SAD but FRUSTRATED at my stupidity :(5 -
In college when we had programming labs where we had to use the schools unix server to compile and run.
My professor was very bad at explaining what actually needed to be done in the labs to the point where even the TAs didn't know what to do.
We were suppose to write an application in C to find out by "trial and error" how large we could make an array (or something like that, it's been too long). This not being explained well and no one knowing that much about C, I wrote a loop that just kept growing an array until it couldn't anymore. I watched it consume 72GB or memory from the servers before quitting the loop and realizing with the TA what the professor really meant.
I now feel bad for the IT staff monitoring the system wondering where 72GB just went...2 -
Short rant: I hate xcode, I hate Swift, I hate Apple.
After 3 weeks of intensive work (I'm an apprentice, part-student, part-worker), I was happy to go back to school and was like "Oh we're going to learn iOS, sounds cool !".
It is now friday, I have homicidal tendencies growing inside me, I want to cry whenever I hear xcode or swift.
Why in the hell I can't use a string argument when I'm calling a function NEEDING a string arg ?
Why do xcode take so long to tell me that there is a problem, why is the error message not explicit AT ALL ?
Why dictionaries so hard to manipulate, EVEN IN JAVA IT'S SIMPLIER.
Why putting our API call in specialized files make them run AFTER EVERYTHING ELSE and the solution that is given to us is deprecated since 5 years ?
Why is a classic c-style for loop is now deprecated ?
These are just a drop in the ocean of WHAT THE FUCK IS THAT that we came across this week.
Fuck Swift, fuck xcode.7 -
It’s been so long since I posted but this time it’s juicy again.
I got a coworker, no prio experience but already a year and few months into the job. He’s bad.
Magnitudes of bad!
We’re trying to teach him but to no avail. Everything about him sucks, major ballsack to be exact.
His attitude is to avoid every task, finishes nothing and then starts something new.
„Did you do X like we told you to?“
„No I started on Y, because I thought it [looks better, seems more interesting, thought that X is useless…]“
When you ask him much is done he is always „almost“ finished and needs your help on the „last 5-10%“. Yeah fuck that!
But that guy has a talent, his talent is to always give you technically correct answers which actually are complete bullshit.
„What are you doing at your job?“
„Staring at a screen and typing things.“ dude what?
That guy used the excuse „I can’t do maths“ on everything.
For an exam he had to calculate how long it would take to reach a certain amount if you would get some interest in that every year.
He asked the teacher for the formula. During the exam! And when the teacher didn’t want to give it to him he wrote plainly „can’t do maths“ on the paper and left
His code is of a quality as if he would write his first line in a week and then has the audacity to blame me and the colleagues for not explaining it right.
Ok you might think now we’re teaching him bad, or are too impatient. But honestly if you have to explain how to do a for loop for over about 15 months and get that attitude I think you get the right to be angry. I don’t mind explaining on how things work, even for the hundredth time, but then don’t tell me you understood, go behind my back, complain at a colleague how bad I explained, get explained by him and then do it again until you whored yourself through the whole staff!
It’s like he got the mind swiper from Men in black at home. Every day he hits the reset button.
He had a week of just changing indentation on a html file. Why? Because he wanted to find his style.
Yeah his style
if(a==b){
console.log(a);
}
else {
console.log(b)
}
And to produce code like that it takes him atleast 4 hours of trial and error.
And at the same time he goes arround and boasts what a super good programmer he his and that he can do some project work for them.
How we found out? Because he started working in those projects during work time at the office and asked us how to do things.
And he does so like a complete bastard!
Broken sql query? “No that query is perfect as it is, it’s supposed to show no results! But, just in theory, if I wanted to show some results, what would I need to change?”
I’m so mad about it and pissed on a personal level because he goes around blames everyone and the world for his short comings5 -
Hey, been gone a hot minute from devrant, so I thought I'd say hi to Demolishun, atheist, Lensflare, Root, kobenz, score, jestdotty, figoore, cafecortado, typosaurus, and the raft of other people I've met along the way and got to know somewhat.
All of you have been really good.
And while I'm here its time for maaaaaaaaath.
So I decided to horribly mutilate the concept of bloom filters.
If you don't know what that is, you take two random numbers, m, and p, both prime, where m < p, and it generate two numbers a and b, that output a function. That function is a hash.
Normally you'd have say five to ten different hashes.
A bloom filter lets you probabilistic-ally say whether you've seen something before, with no false negatives.
It lets you do this very space efficiently, with some caveats.
Each hash function should be uniformly distributed (any value input to it is likely to be mapped to any other value).
Then you interpret these output values as bit indexes.
So Hi might output [0, 1, 0, 0, 0]
while Hj outputs [0, 0, 0, 1, 0]
and Hk outputs [1, 0, 0, 0, 0]
producing [1, 1, 0, 1, 0]
And if your bloom filter has bits set in all those places, congratulations, you've seen that number before.
It's used by big companies like google to prevent re-indexing pages they've already seen, among other things.
Well I thought, what if instead of using it as a has-been-seen-before filter, we mangled its purpose until a square peg fit in a round hole?
Not long after I went and wrote a script that 1. generates data, 2. generates a hash function to encode it. 3. finds a hash function that reverses the encoding.
And it just works. Reversible hashes.
Of course you can't use it for compression strictly, not under normal circumstances, but these aren't normal circumstances.
The first thing I tried was finding a hash function h0, that predicts each subsequent value in a list given the previous value. This doesn't work because of hash collisions by default. A value like 731 might map to 64 in one place, and a later value might map to 453, so trying to invert the output to get the original sequence out would lead to branching. It occurs to me just now we might use a checkpointing system, with lookahead to see if a branch is the correct one, but I digress, I tried some other things first.
The next problem was 1. long sequences are slow to generate. I solved this by tuning the amount of iterations of the outer and inner loop. We find h0 first, and then h1 and put all the inputs through h0 to generate an intermediate list, and then put them through h1, and see if the output of h1 matches the original input. If it does, we return h0, and h1. It turns out it can take inordinate amounts of time if h0 lands on a hash function that doesn't play well with h1, so the next step was 2. adding an error margin. It turns out something fun happens, where if you allow a sequence generated by h1 (the decoder) to match *within* an error margin, under a certain error value, it'll find potential hash functions hn such that the outputs of h1 are *always* the same distance from their parent values in the original input to h0. This becomes our salt value k.
So our hash-function generate called encoder_decoder() or 'ed' (lol two letter functions), also calculates the k value and outputs that along with the hash functions for our data.
This is all well and good but what if we want to go further? With a few tweaks, along with taking output values, converting to binary, and left-padding each value with 0s, we can then calculate shannon entropy in its most essential form.
Turns out with tens of thousands of values (and tens of thousands of bits), the output of h1 with the salt, has a higher entropy than the original input. Meaning finding an h1 and h0 hash function for your data is equivalent to compression below the known shannon limit.
By how much?
Approximately 0.15%
Of course this doesn't factor in the five numbers you need, a0, and b0 to define h0, a1, and b1 to define h1, and the salt value, so it probably works out to the same. I'd like to see what the savings are with even larger sets though.
Next I said, well what if we COULD compress our data further?
What if all we needed were the numbers to define our hash functions, a starting value, a salt, and a number to represent 'depth'?
What if we could rearrange this system so we *could* use the starting value to represent n subsequent elements of our input x?
And thats what I did.
We break the input into blocks of 15-25 items, b/c thats the fastest to work with and find hashes for.
We then follow the math, to get a block which is
H0, H1, H2, H3, depth (how many items our 1st item will reproduce), & a starting value or 1stitem in this slice of our input.
x goes into h0, giving us y. y goes into h1 -> z, z into h2 -> y, y into h3, giving us back x.
The rest is in the image.
Anyway good to see you all again.27 -
Was fixing a bug and suddenly got an error that the lodash library could not be loaded. Funny, didn't even know the project used that lib. Looked for the reference and the previous dev used _.times instead of a for loop. Ha okay, interesting. Wonder where else this library is used.
Searched the whole project for references, dependencies, whatever, any sign of it. Fucking. Nothing.
Rewrote the _.times part as a simple for loop, then removed the library. The rest of the project still worked perfectly. Took me about a minute and a half.
Who the fuck uses an entire damn library to... Not write a for loop I guess?!7 -
Amazon mturk. Job was to rate grammatical corrections.
First of all, it's surprising how often people forget commas. That's like, the #1 error with these things.
People just keep going on and on and on and on and on and never break their sentence even if there was supposed to be a comma and it really makes the voice in my head fell like it's running out of breath but it can't stop because the sentence is still going and [...]
The corrections are generally okay. I took many more college-level English classes than I think I needed to, so my English is fairly decent. For this reason, I might be a bit more of a stickler than I need to be for this job.
But this one threw me for a loop, because it's just such a bad correction. Not only does it miss the obvious errors but creates a new, equally obvious error.
This is one of the reasons mturk is interesting to me. Sure, I don't make.... practically anything. But you come into such a variety of work that it's almost addicting in a sense.18 -
I HATE SURFACES SO FRICKING MUCH. OK, sure they're decent when they work. But the problem is that half the time our Surfaces here DON'T work. From not connecting to the network, to only one external screen working when docked, to shutting down due to overheating because Microsoft didn't put fans in them, to the battery getting too hot and bulging.... So. Many. Problems. It finally culminated this past weekend when I had to set up a Laptop 3. It already had a local AD profile set up, so I needed to reset it and let it autoprovision. Should be easy. Generally a half-hour or so job. I perform the reset, and it begins reinstalling Windows. Halfway through, it BSOD's with a NO_BOOT_MEDIA error. Great, now it's stuck in a boot loop. Tried several things to fix it. Nothing worked. Oh well, I may as well just do a clean install of Windows. I plug a flash drive into my PC, download the Media Creation Tool, and try to create an image. It goes through the lengthy process of downloading Windows, then begins creating the media. At 68% it just errors out with no explanation. Hmm. Strange. I try again. Same issue. Well, it's 5:15 on a Friday evening. I'm not staying at work. But the user needs this laptop Monday morning. Fine, I'll take it home and work on it over the weekend. At home, I use my personal PC to create a bootable USB drive. No hitches this time. I plug it into the laptop and boot from it. However, once I hit the Windows installation screen the keyboard stops working. The trackpad doesn't work. The touchscreen doesn't work. Weird, none of the other Surfaces had this issue. Fine, I'll use an external keyboard. Except Microsoft is brilliant and only put one USB-A port on the machine. BRILLIANT. Fortunately I have a USB hub so I plug that in. Now I can use a USB keyboard to proceed through Windows installation. However, when I get to the network connection stage no wireless networks come up. At this point I'm beginning to realize that the drivers which work fine when navigating the UEFI somehow don't work during Windows installation. Oh well. I proceed through setup and then install the drivers. But of course the machine hasn't autoprovisioned because it had no internet connection during setup. OK fine, I decide to reset it again. Surely that BSOD was just a fluke. Nope. Happens again. I again proceed through Windows installation and install the drivers. I decide to try a fresh installation *without* resetting first, thinking maybe whatever bug is causing the BSOD is also deleting the drivers. No dice. OK, I go Googling. Turns out this is a common issue. The Laptop 3 uses wonky drivers and the generic Windows installation drivers won't work right. This is ridiculous. Windows is made by Microsoft. Surface is made by Microsoft. And I'm supposed to believe that I can't even install Windows on the machine properly? Oh well, I'll try it. Apparently I need to extract the Laptop 3 drivers, convert the ESD install file to a WIM file, inject the drivers, then split the WIM file since it's now too big to fit on a FAT32 drive. I honestly didn't even expect this to work, but it did. I ran into quite a few more problems with autoprovisioning which required two more reinstallations, but I won't go into detail on that. All in all, I totaled up 9 hours on that laptop over the weekend. Suffice to say our organization is now looking very hard at DELL for our next machines.4
-
Autodesk + Linux is such a goddamn clusterfuck.
Firstly, they only release RPM builds for Maya, and say that they officially support RHEL and CentOS only.
No support for Debian, Arch, etc. What. The. Fuck.
Fine. Okay. Corporate policy. I can live with that. I use alien to convert the RPMs to DEBs on my ZorinOS installation and then found a script which does the installation for me. Cool.
Installs with a few library fuckups. Okay, no problem. I added the missing library versions (ancient libpng and libtiff). I run it. It throws up with some error involving licensing.
Upon searching it seems that Maya 20-fucking-17 can't handle the "new" consistent device naming system (the one which renames eth0 to enp1s0 or whatever). WHAT THE FUCK. Okay. Found a way to disable that. No effect. It's doing the equivalent of a boot loop with the same error.
Wow. This is the leading player in 3D content creation software :/
(As an aside, I did try to install Fedora 28 but it keeps failing with a TPM error. Yay for Linux distro quirks).1 -
The more problems I encounter with Windows the more I have the impression these infested chimps never implemented a real system repair/restoration feature but just faked it with randomized error messages.
Now Windows 10 can't even rollback updates anymore but lands in an infinite boot loop that even a reset to an earlier restoration point won't fix.
And the Windows repair option lists two Windows installations even though there's just one.
Somehow the roles of Linux and Windows have switched nowadays, by now I had to reinstall Win10 5 times on three PCs in the last 12 months.
Windows 8 was shit too but at least it didn't fucking break within a week.3 -
Badass scenario:
Professor: writes a loop to sum up first five numbers and asks the output.
Me: 500
Other random student: 15
Prof praises him.
He runs the code.
Output: 500 ( internal server error)
(He had a missing semicolon) 😅6 -
Fed up with f@_#-$&@_ Microsoft bullshit, computer refused to install IIS without a reboot first. Rebooted, got bluescreen with some error like APC_Index mismatch since it was hooked up to an external monitor (this has happened before, but only when booting into Windows, not Ubuntu), god knows why now since it was hooked up to same monitor before reboot and worked just fine. All this because some stupid school related task requires Visual Studio and a web project-solution that takes ages to run/debug which I would rather build and devug through attaching remote debugger to the running IIS-process. Instead I get into a boot-loop when the screen is connected, and when I connect the screen after a normal boot it restarts itself. Why is it always like this? Most of the time is spent just to setup the environment or making Windows work. F#&#_ this and the assignment, goodnight.1
-
WTF if you want to program stuff learn how to use your programming language. Why is it so hard for that many people to learn how stuff works and stop copying blindly from the internet?
I have two colleagues who are doing nothing else that to just google their problems going to the first answer and cooping it then trying to run the program and if it doesn’t work ether give up completely or starting a loop of inserting the error message to google and copy the first result?3 -
TL;DR: TIL for heavy queries use PDO and not some frameworks DB class
ffs I was trying to save 300k+ lines at once with Laravel for weeks. Mind you from a text file. 1gb ram on the vps so while trying to prepare the text to save: Fatal Error: Allowed Memory Size of bla bla Bytes Exhausted
ok so lets put it in a loop: Fatal error: Maximum execution time of 30 seconds exceeded (set_time_limit(0); lol)
optimising, varying the code got me into a situation when the content got saved in the BD but inconsistent (duplicates) and the table had often more than 1,5M rows. That was what told me its not a performance issue, my code is the issue. (dah)
I was starting to think it would be easier to export a prepared query to a sql file and load the file into the db as thats the fastest possible option...I even started to think about switching to python, then it hit me, Laravel has a shitload of routes to the DB so I switched to PDO
benchmark on 1vCPU, 1GB RAM VPS with SSD
379k lines with 11 columns in less than 10 sec with a loop of saving every ~6000 rows (if i tried choking it to save the whole thing at once it went up to 16-17sec)2 -
God I fucking hate macs.
I got a mac at work. I tried to install ubuntu, with rather questionable results (unfortunately, I expected that) - so I tried to get mac work for me the way I like a system to work. I needed to download slack, simple enough, right? Ha, you wish. It's gotta be done through Apple store, so I went to create an Apple ID inside the Apple Store form. And, well, it just errored out on the submission. Great start. I went then to the settings and created an account there, great success, went back to Apple Store. Unfortunately being logged in at the system level doesn't mean you are logged in to the store. So, I went to log in to the store, simple enough, right? No, nothing's simple with Apple. After logging in I got a message that the Apple ID has not yet been used with Apple Store and that I need to review the account's setting. So, I click the "review" button and... I'm presented with a log in form. Yep, a perfect log in loop. I can't log in because I can't review the account but I can't review the account because I can't log in. Fun :)
You can't just go to the web admin panel for your account to review it for Apple Store, that would too be too easy. After a bit of searching I've found an answer on StackOverflow. You need to log in to iTunes. Through a fucking MUSIC APP. To install a free application from the store you need to log in to a music app. Yes, we're all mad here.
Then, after finding out that to be able to use side buttons on my mouse I need an app that I need to manually restart every time I restart the machine and that I need to have an app to fucking transfer files from an android I need another fucking app, because reading a storage of a linux-based system would be too standards compliant - something in me broke. I found out that installing windows on a mac is officially supported.
Supported doesn't mean that it's easy. I tried to install it trying different solutions from SO, but each time I would get an error that Windows couldn't modify the boot partition. Turns out that even wiping the drive and reinstalling OSX doesn't remove residual files on a boot partition and Windows installer is not allowed to modify them. It took me hunting into some shady looking site to actually find this answer. I have no fucking idea how long it all took me, but, finally, great success, Windows, WSL, side buttons working, I can even install slack from an installer. I just wish I could have those hours of my life back.17 -
I've been out of the loop with websites and frontends for a while. Now, is it me or is it just overengineered to make a static website that's not a blog these days?
I mean, I need to make a landing page. 6 sections + footer. And I don't want to end up with a 600+ lines html file. With tailwind possibly.
JEKYLL
I've used it a few times, and after 3 years I still get some weird error when installing everything. Maybe it's trivial, but I know shit about ruby. Plus, I don't need ruby for anything else, and the official Docker image just doesn't work, exactly like the quickstart tutorial. 3 years later, same issues.
HUGO
I like this guy but god, the docs are just unreadable, it's not compatible with tailwind 3.x (or smth) and it's been a pain to build a user-configurable homepage. Plus, it does more than half of the work by itself, Fair enough, it's supposed to be used for blogs.
ANY OTHER "JAMSTACK" BULLSHIT
Anything is either a blogging engine or delivers some crappy javascript blob from hell. I just need an html document, that weird thingie the whole World Wide Web was built upon, broken into pieces so I can keep my sanity.
Looking forward to get the fucking AWS Solutions Architect. Looking even more forward to build my farm.8 -
I went down a rabbit hole of code changes to try and delete a stupid for loop with a break in it.
It was super stupid and I gave up and submitted to the fact that some battles are not worth the time and stress.
OK... But seriously, It was returning multiple entities from the database, but we only always want the first one. My logic is that we should just go in there and fix the LINQ so we are explicitly getting one entity out.
But fuck that logic. No I'll have to change fucking everything that's tied to that method and expects a list from it. Every fucking thing. That includes error handling, parsing, for loops..... Nevermind...
You can have your foreach and your break. I'm taking mine, now.rant break my back on this stupid code what do you want on the frontend last minute changes did this to me they couldn't decide1 -
Error in linux be like D∈∀DL❍⊂κ:
Can't install module <a> dependent on 3 other.. trying to install one of those 3 and here comes again.... Can't inatall this module depends on <a> -
There's an angular project at work.
I guess they know about as much about angular as I do (not much)
Because the error page isn't working, so when you get an error, you infinite loop and DDoS yourself.
It actually crashes my (admittedly subpart) desktop.
Guess I'll be learning how to fix that.19 -
Mount an azure file share in an app service container? Sounds handy. Nice clicky-draggy wizard to set it up, pick your file share, type a path to mount it to, hit save.
And does it work?
Does it buggery.
And is there a helpful error message so you can see what you've done wrong?
In a pig's arse is there a helpful fucking error message.
"Application error", and a link to some "diagnostic resources" that displays the exact same error message, including the same link, so a link to itself, in an infinite recursive loop of rank, inhuman stupidity.
Let me see what's in the logs. Absolutely fuck all. No, wait! There's the html markup for the fucking useless error message I'm looking at in the browser. So the UI is telling me to fuck off, and the logs are recording that I have been told to fuck off.
But this is Azure. So there isn't just one place to look at the logs, there are many places to look at the logs. And they are all geologically slow and most of them don't work.
It's probably a firewall issue. I'll have a look later on if I can be arsed, but frankly I'd rather be performing cunnilingus on a lion.1 -
Is there any solution to the “Preparing Automatic Repair” error in windows?
It has gone in an infinite loop.
I have Lenovo Ideapad 300.7 -
Finally finished an algo to check an image for grouping of pixels that will form a rectangular area. I got the grouping to work on one image, but found it was utterly failing on another. I went through every step of the algo and still could not find the solution. The 128x128 image was working, but the 128x16 image was not. I knew it had something to do with the dimensions. Started thinking it was overflowing a buffer somewhere. So I started putting asserts in the functions that abstracted the buffer access. None of the numbers exceeded the proper bounds. It was close to bedtime so I finally gave up. I was tired. Then I realized it wouldn't be until the next evening when I could look at this again. So I got up again and started looking at the code again. I had a loop to check the output of my algo that I did the memory access of the buffer. It too was not fully filling my temp image to show how the algo was working. WTF!
Then I finally realized the flaw:
buffer[x+y*height]
And my test loop to test the algo:
buffer[x+y*ymax]
I kept overlooking the error because I was sure it was right. Also my asserts for the functions to access the buffers? They only checked the inputs x and y. So it didn't help that the math was wrong for reading and writing the buffers. It also worked fine on 128x128 images because the width and height were the same.
It is funny that I struggled with this part. The algo was actually surprisingly easy to formulate. I just looked through every point and checked a buffer to see if that point was used. If not then I would attempt to grow in the x and y direction the shaped of that point based upon pixel color. This was saved in a structure while growing that point. Then when that rectangle could not be grown further the inner loop would continue checking used points again.
I still have work to do to use the data this algo produces. I need to now figure out how to parent the rectangular areas to each other. I will probably use my check buffer to keep track of these rects by an index. Then do adjacent checks to determine parenting. Eventually I will have to extend this algo to 3 dimensions, but that should not be difficult.2 -
Magento Debugging Horror!
Changing lots of things in magento with no problem. Continuing development for quite sometime. Suddenly decide to clear cache to see affect of a change on a template in frontent. Suddenly magento crashes! There's no error message. No exception log. No log in any file anywhere on the disk. All that happens is that magento suddenly returns you to the home page!
Reverting all the changes to the template. Clear the cache. Nope! Still the same! Why? Because the problem has happened somewhere in your code. Magento just didn't face it, because it was using an older version of your code. How? Because magento 2 even caches code! Not the php opcache. Don't get me wrong. It has it's own cache for code, in a folder called generated. Now that you cleared all the caches including this folder, you just realized that, somewhere something is wrong. But there is no way for you to know where as there is absolutely no exception logged anywhere!
So you debug the code, from index.php, down to the deepest levels of hell. In a normal php code, once the exception happens, you should see the control jumps to an exception handler, there, you can see the exception object and its call stack in your debugger. But that's not the case with magento.
Your debugger suddenly jumps to a function named:
write_close();
That's all. No exception object. No call stack. No way to figure out why it failed. So you decide to debug into each and every step to figure out where it crashes. The way magento renders response to each request is that, it calls a plugin, which calls a plugin loop, which calls another plugin, which calls a list of plugins, which calls a plugin loop, which calls another plugin.....
And if in each step, just by accident, instead of step through, you use the step over command of your debugger, the crash happens suddenly and you end up with the same freaking write_close() function with no idea what went wrong and where the error happened! You spend a whole day, to figure out, that this is actually a bug in core of magento, they simply introduced after your recent update of magento core to the latest STABLE version!!! It was not your mistake. They ruined their own code for the thousandth of time. You just didn't notice it, because as I said, you didn't clear the `generated` folder, therefore using an older version of everything!
Now that after spending 7 hours figuring out what has failed with absolutely no standard way of debugging and within a spaghetti of GOTO commands (Magento calls them plugin), why not report it to github? So you report it with a pull request. This also takes 1 hour of your time. Just to next day get informed that your pull request is rejected because another person already fixed the bug and made the same pull request. It was just not on the latest stable version yet!
So you decide to avoid updating magento as much as possible. Because you know that the next Stable version will make your life and career unstable. But then the customer complains that the Admin Panel is warning him of using old Magento version which might pose SECURITY THREATS! -
Removes an control statement or a loop statement in python code ......
Fucks up entire code below it due to indentation error..
Sit and remove the indentation line by line
PYTHON IS LIFE3 -
Newbie Linux User - Story about not working GUI
I am a proud Opensuse user for about a year, still struggling with some basic stuff, terminal, etc.
The story begins when a few days ago I try to login to the system. To my trusty Gnome. I get stuck on login loop;
successful login - > black screen for a second - > back to login screen.
Zero feedback, not a single error message
Stress level increases taking in count that I am at a climax at my university with tons of projects on my computer.
I assemble the Team A:
Me, Google, Stackoverflow, and for desperate times Russian Stackoverflow
Over 4 hours, found out that my user is affected by this, tried restoring default Gnome configuration, went through bunch of logs only to find out that every user gets the same errors, still only my not working. Even KDE denied to cooperate with the same result.
So what went wrong you may be thinking.
One line in file replaced by miniconda, that changed the PATH.
Linux is the best detective game that I've ever played.
Is it something that I should get used to?2 -
I started to learn coding at school using RM Basic. I made a script with an infinite loop using goto that flashed the screen different colours and said error on it. I left it running. Found out later the it guy took the computer away for "repair"...
-
There's nothing quite like an app force closing for no apparent reason, and no error log info.
Just spent an hour figuring out that one of the device I test on doesn't like linking GLSL shader programs if it contains a loop even know every other devices I've tested on are totally fine with it. 😑 -
My life:
Int main(nothing){
while(1){
drink(beer);
code(c);
sleep(the_next_time);
}
return 0;
}
/* compile error -infinite loop found- */ -
To most non-dev people, being hit with error after error when fixing one small problem out of a much bigger collection of problems would probably be infuriating.
To me, it's a huge loop of:
for(var failsAtLine = 1; failsAtLine < lines.length; failsAtLine++;) {
changeSomething();
runTest();
readErrors();
cry();
comtemplateMeaningOfLife();
}
openChampagne();
Would help if deployer.org had better documentation and bigger support community. -
All the night thinking about a possible solution, finally got it, code it, push and went to sleep some time, then I realized it didn't deploy and doesn't actually run ._. almost cry, and then I realized the error was that i was using the wrong variable and forcing an infinite loop .___.
-
I can work productively and for very long hours with a lot of stuff which many dev considers productivity hurdles:
- single small monitor? No problem (in fact in one occasion in which my roommate accidentally broke my laptop charghing port and I couldn't get a spare I worked on an iPad connected trough SSH to a Linux machine completing one of the hardest tasks I ever did without significant loss of productivity)
- old machine? That's ok as long as I can run a minimal Linux and not struggle with Windows
- noise and chatter around me? A 10€ pair of earbuds are enough for me, no noise cancelling needed
- "legacy" stack/programming language? I'd rather spend my days coding in Swift or Rust but in the end I believe which is the dev and its skill which gets the job done not fancy language features so Java 8 will be fine
- no JetBrains or other fancy IDE? Altough some refactoring and code generation stuff is amazing Neovim or VS Code, maybe with the help of some UNIX CLI tools here and there are more than enough
despite this I found out there is a single thing which is like kryptonite for my productivity bringing it from above average* to dangerously low and it's the lack of a quick feedback loop.
For programming tasks that's not a problem because it doesn't matter the language there's always a compiler/interpreter I can use to quickly check what I did and this helps to get quickly in a good work flow but since I went to work with a customer which wants everything deployed on a lazily put together "private cloud" which needs configurations in non-standard and badly documented file formats, has a lot of stuff which instead of being automated gets done trough slowly processed tickets, sometimes things breaks and may take MONTHS to see them fixed... my productivity took a big hit since while I'm still quick at the dev stuff (if I'm able to put together a decent local environment and I don't depend on the cloud of nightmares, something which isn't always warranted) my productivity plummets when I have to integrate what I did or what someone else did in this "cloud" since lacking decent documentation everything has do be done trough a lot of manual tasks and most importantly slow iterations of trial and error. When I have to do that kind stuff (sadly quite often) my brain feels like stuck on "1st gear": I get slow, quickly tired and often I procrastinate a lot even if I force myself out of non work related internet stuff.
*I don't want this to sound braggy but being a passionate developer which breathes computers since childhood and dedicating part of my freetime on continuously improving my skill I have an edge over who do this without much passion or even reluctantly and I say this without wanting to be an èlitist gatekeeper, everyone has to work and tot everybody as the privilege of being passionate in a skill which nowadays has so much market2 -
//not a rant
Ok so weird bug. Fellow C# people, help me out.
//already made it work so no I don't need to post it on SO
I write a Switch Case block based on the user's combo-box selection id.
if id 0, add everything to the mainpage grid
if 1, a foreach loop filtering out the ones with a certain attribute of the object as false and adding em to the grid on the mainpage
if 2, similar scenario as 1.
Countless times I had a null exception with the "count" variable being the number of items in the post which, wasn't null. there was no other variable that was being initialized from within the block, so I had no idea what was causing it.
Moving to an if-else statement doing the same thing, same issue.
In the end I created 2 empty lists before the switch case and filled them up and then another loop filling the mainpage grid with the now-filled list.
In the end im doing the same thing, with no issues, but I don't understand why adding it directly caused an error, what was null?
I wanna understand the working that might be causing this.. if anyone else came across this, would be glad to hear from you8 -
“Just a quick fix” Classic start to a reported ticket. Ticket states that a form field was not doing anything.
Think to myself ok this sounds like a nice easy one for the morning. A few hours later I find something like the following written by a senior member of the dev team.
SearchClass {
//...list of getter and setters
Private $snakeCaseName;
SearchFunction() {
Foreach($this as $property => $value){
//... if property keys = string for each object property then do code
If($property == “snakecaseProperty”){
//...do stuff
}
}
}
}
Why does this loop exist!!!!! All it does is remove any error checking if a getter method is misspelt...
To make matters worse the entire search method was over 300 lines building a MySQL query string.... even though there was an ORM and entity classes available!!!2 -
To you CPP-devs,
I want to write a small ask-password tool, like systemd-ask-password, but tty-only and non-systemd.
I use termios to switch the terminal into noncanonical mode and disable echo of stdin.
Now I want to read chars from cin and append them to a string, until I read an endl or an error occurs.
In C, I could use an input-loop with getchar, but how could I do this the CPP-way, since cin.get() is nonblocking?2 -
Disclaimer: I am a beginner and I used node just because my employer asked me to.
I needed to create 1400 random users for a platform and I needed to get all the usernames and passwords in a json file and my idea was to just add the object to other collection with all the creditals(passwords are hashed in the db so I couldnt just loop them). For some reason it wouldn't work (i am really bad with async functions) and I just threw the table and copy pasted it from the error screen.
this_shit = {[name1,pass1],[name2,pass2]...}
throw this_shit;
Worked like a charm ^_^