Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
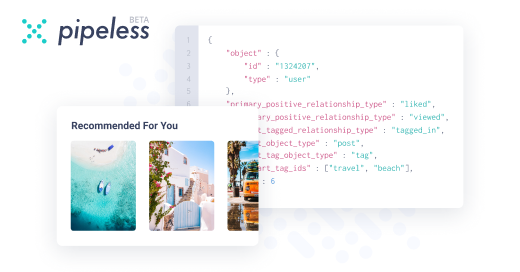
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "integers"
-
Le monday morning after a commit on sunday evening...
PM: BLAAAH!!!! Your commit broke the site, nothing is working!!!!!!
Me: What? All of tests passed (coverage 95%), no issues were found.
PM: NOO!!!! Site is broken, we can't use it no more!!!
Me: Ok, what's the problem?
PM: I've tried to enter -10021 into this field on that page and it gived me an error.
Me: Ok? So, that single page is broken?
PM: No, whole site!!!! This is important
Me: Sure... Let me take a look
* PM tried to enter a negative value into an unsigned field that I've mutated yesterday after checking LIVE database if there was no records with negative value. Reason: we've hit an int limit and there was no chance that the value would be negative. Validation? Well, yes.... Except that page was added by him this morning without even checking everything else *
Me: Here, this is the issue, *gives explanation*
PM: Well.... You shouldn't do this. This is unacceptable. You must never leave int fields without negative values. Didn't they teach you in school that integers can be negative?!
Me: What? *consufed as hell*
PM: *More morale... blah blah blah....* Revert it back!
Me: Ok but if anything else breaks, copy of this slack conversation will be kept.
PM: Don't care! Fix it!
Me: * Reverts the fix, saves chat copy * - Done.
PM: Great.
* 5 wild minutes later *
PM: BLAAAH!!!! Site is down, service is not working, what have you done?
Me: Reverted the change needed for it to work. Todays schedule is full with other important tasks. * pastes a screenshot as a proof that he asked me to do this *
PM: FIX IT NOW! Apply your fix.
Me: You're the PM. - Done.
PM: Great, now I'll fix my code. You should be more careful next time.
Me: * YOU DENSE MATHA...KER * Sure.
How's your morning going? :)9 -
If I could represent my life using 32-bit signed integers, you would be -2,147,483,648 because you are the Most Significant Bit of my life ❤9
-
I had to open the desktop app to write this because I could never write a rant this long on the app.
This will be a well-informed rebuttal to the "arrays start at 1 in Lua" complaint. If you have ever said or thought that, I guarantee you will learn a lot from this rant and probably enjoy it quite a bit as well.
Just a tiny bit of background information on me: I have a very intimate understanding of Lua and its c API. I have used this language for years and love it dearly.
[START RANT]
"arrays start at 1 in Lua" is factually incorrect because Lua does not have arrays. From their documentation, section 11.1 ("Arrays"), "We implement arrays in Lua simply by indexing tables with integers."
From chapter 2 of the Lua docs, we know there are only 8 types of data in Lua: nil, boolean, number, string, userdata, function, thread, and table
The only unfamiliar thing here might be userdata. "A userdatum offers a raw memory area with no predefined operations in Lua" (section 26.1). Essentially, it's for the API to interact with Lua scripts. The point is, this isn't a fancy term for array.
The misinformation comes from the table type. Let's first explore, at a low level, what an array is. An array, in programming, is a collection of data items all in a line in memory (The OS may not actually put them in a line, but they act as if they are). In most syntaxes, you access an array element similar to:
array[index]
Let's look at c, so we have some solid reference. "array" would be the name of the array, but what it really does is keep track of the starting location in memory of the array. Memory in computers acts like a number. In a very basic sense, the first sector of your RAM is memory location (referred to as an address) 0. "array" would be, for example, address 543745. This is where your data starts. Arrays can only be made up of one type, this is so that each element in that array is EXACTLY the same size. So, this is how indexing an array works. If you know where your array starts, and you know how large each element is, you can find the 6th element by starting at the start of they array and adding 6 times the size of the data in that array.
Tables are incredibly different. The elements of a table are NOT in a line in memory; they're all over the place depending on when you created them (and a lot of other things). Therefore, an array-style index is useless, because you cannot apply the above formula. In the case of a table, you need to perform a lookup: search through all of the elements in the table to find the right one. In Lua, you can do:
a = {1, 5, 9};
a["hello_world"] = "whatever";
a is a table with the length of 4 (the 4th element is "hello_world" with value "whatever"), but a[4] is nil because even though there are 4 items in the table, it looks for something "named" 4, not the 4th element of the table.
This is the difference between indexing and lookups. But you may say,
"Algo! If I do this:
a = {"first", "second", "third"};
print(a[1]);
...then "first" appears in my console!"
Yes, that's correct, in terms of computer science. Lua, because it is a nice language, makes keys in tables optional by automatically giving them an integer value key. This starts at 1. Why? Lets look at that formula for arrays again:
Given array "arr", size of data type "sz", and index "i", find the desired element ("el"):
el = arr + (sz * i)
This NEEDS to start at 0 and not 1 because otherwise, "sz" would always be added to the start address of the array and the first element would ALWAYS be skipped. But in tables, this is not the case, because tables do not have a defined data type size, and this formula is never used. This is why actual arrays are incredibly performant no matter the size, and the larger a table gets, the slower it is.
That felt good to get off my chest. Yes, Lua could start the auto-key at 0, but that might confuse people into thinking tables are arrays... well, I guess there's no avoiding that either way.13 -
I discovered a function in our database that converts integers to ordinals by concatenating the number and a suffix:
- ends in 1: add “st”
- ends in 2: add “nd”
- ends in 3: add “rd”
- else: add “th”
Simple! Except I guess nobody considered the 11st, 12nd, and 13rd iteration of this function…9 -
Where I work, in our database, we use 3 to indicate true and 7 to indicate false and 0 is true but null is false
In another table, we use 'P' to indicate true and 'I' to indicate false and 'Y' is also false and null is false
And the most used table, we also use 'Y' to indicate yes and 'N' to indicate no, but null is also Yes.
We also store integers as varchar in a live table, but stays an integer in all the other tables. I hope I'm not there when the number of digits exceeds the varchar limit.
These are all live and used in production all created by my boss, the head of IT.8 -
I'm currently rewriting perfectly clean and functioning Scala code in Java (because "Enterprise", yay). The amount of unnecessary boilerplate I have to add is insane. I'm not even talking big complicated code but two liners or the lack of simple things like a range from 5 to 10.
Why do I have to write
List<Position> occupiedPositions = placedEntities.stream()
.flatMap((pe) -> pe.occupiedPositions().stream())
.collect(Collectors.toList());
instead of simply
val occupiedPositions = placedEntities.flatMap(_.occupiedPositions)
Why on earth does `occupiedPositions.distinct` suddenly become a monstrosity like `occupiedPositions.stream().distinct().collect(Collectors.toList())` where the majority of code is pure boilerplate? And this is supposed to be the new and better Java8 api which people use as evidence that Java is now suddenly "functional" (yeah no, just no).
Why do APIs that annotate parameters with @Nullable throw NullPointerExceptions when I pass a null? Why does the compiler not help prevent such stupidity? Why do we use static typing PLUS those annotations and it still crashes at runtime like every damn dynamic, interpreted language out there? That's not unfortunate, it's a complete waste of time.
Why is a simple idea like a range from x to 10 (in scala literally `x to 10`) not by default included in Java? There's Guava's version of Range which does not have a helper for integer ranges (even though they are the most used ones). Then there's apache.commons version which _has_ a helper for integers, but is strangely not iterable (wtf I don't even...).
Speaking of Iterable: How difficult could it be to convert an abstract Iterable<T> into a concrete List<T>? In scala it's surprisingly `someIterable.toList`. I found nothing like that so I took to stackoverflow where I found a thread in which people suggested everything from writing your own ListUtils helper class, using Guava (which is a huge dependency!) to using the new Java8 features inline (which is still about three lines long). I didn't know this was such a hard problem in computer science, TIL.
How anyone can be productive in this abomination of a language is beyond me now, even though I've used it for many years while learning to code (back then I didn't know there were much better ways to do things). The only good part is that I have to endure this nonsense for only about 3 days longer then I'm free again!12 -
I did a job interview recently for a company and the test was something like this.
In ruby, write a web server that will serve a specific line number from a text file.
I thought up a simple solution and a more advanced solution, but I opted to go with the simple solution and submit my work quickly. I made a nice web server with tests and everything and it used the sed command to get the line number from the file.
Now, they had various instructions, like it had to perform. They asked how it'd perform with 10G, 100G files. I thought "Eh... it'll be alright."
The solution they were looking for was the "advanced" solution that I thought up, which involved storing a binary file of 32/64 bit integers that reference the byte-offset of the line they're looking for. Basically a binary index file.
This violates all of my sensibilities, because I would never build a database indexer like this using ruby, of all things.
I thought it was a stupid test, and how do these companies honestly expect me to spend hours coding and then tell me I didn't go far enough? It's unethical.
I actually followed-up with the "advanced" solution a couple hours after hearing I was out, just to show them that their process is flawed.2 -
A dev team has been spending the past couple of weeks working on a 'generic rule engine' to validate a marketing process. The “Buy 5, get 10% off” kind of promotions.
The UI has all the great bits, drop-downs, various data lookups, etc etc..
What the dev is storing the database is the actual string representation FieldA=“Buy 5, get 10% off” that is “built” from the UI.
Might be OK, but now they want to apply that string to an actual order. Extract ‘5’, the word ‘Buy’ to apply to the purchase quantity rule, ‘10%’ and the word ‘off’ to subtract from the total.
Dev asked me:
Dev: “How can I use reflection to parse the string and determine what are integers, decimals, and percents?”
Me: “That sounds complicated. Why would you do that?”
Dev: “It’s only a string. Parsing it was easy. First we need to know how to extract numbers and be able to compare them.”
Me: “I’ve seen the data structures, wouldn’t it be easier to serialize the objects to JSON and store the string in the database? When you deserialize, you won’t have to parse or do any kind of reflection. You should try to keep the rule behavior as simple as possible. Developing your own tokenizer that relies on reflection and hoping the UI doesn’t change isn’t going to be reliable.”
Dev: “Tokens!...yea…tokens…that’s what we want. I’ll come up with a tokenizing algorithm that can utilize recursion and reflection to extract all the comparable data structures.”
Me: “Wow…uh…no, don’t do that. The UI already has to map the data, just make it easy on yourself and serialize that object. It’s like one line of code to serialize and deserialize.”
Dev: “I don’t know…sounds like magic. Using tokens seems like the more straightforward O-O approach. Thanks anyway.”
I probably getting too old to keep up with these kids, I have no idea what the frack he was talking about. Not sure if they are too smart or I’m too stupid/lazy. Either way, I keeping my name as far away from that project as possible.4 -
Yeah.. it's a shame C# doesn't have a type to hold this (4) and then you have to resort to using var.. I am so disappointed, I've heard C# is crap, but this.. THIS!! It doesn't even have a type for normal integers!! FFS!!! I thought it was better than this!!
Oh, wait.. it's not C# who is 'weird'!! It's my super duper cool ex coworker who made a mess of the simple code again... I admit, this is not such a huge deal.. BUT... It doesn't end here.. o.O39 -
math be like:
"Addition (often signified by the plus symbol "+") is one of the four basic operations of arithmetic; the others are subtraction, multiplication and division. The addition of two whole numbers is the total amount of those values combined. For example, in the adjacent picture, there is a combination of three apples and two apples together, making a total of five apples. This observation is equivalent to the mathematical expression "3 + 2 = 5" i.e., "3 add 2 is equal to 5".
Besides counting items, addition can also be defined on other types of numbers, such as integers, real numbers and complex numbers. This is part of arithmetic, a branch of mathematics. In algebra, another area of mathematics, addition can be performed on abstract objects such as vectors and matrices.
Addition has several important properties. It is commutative, meaning that order does not matter, and it is associative, meaning that when one adds more than two numbers, the order in which addition is performed does not matter (see Summation). Repeated addition of 1 is the same as counting; addition of 0 does not change a number. Addition also obeys predictable rules concerning related operations such as subtraction and multiplication.
Performing addition is one of the simplest numerical tasks. Addition of very small numbers is accessible to toddlers; the most basic task, 1 + 1, can be performed by infants as young as five months and even some members of other animal species. In primary education, students are taught to add numbers in the decimal system, starting with single digits and progressively tackling more difficult problems. Mechanical aids range from the ancient abacus to the modern computer, where research on the most efficient implementations of addition continues to this day."
And you think like .. easy, but then you turn the page:15 -
I love* not being a web developer.
*: who the fuck thought that 31bit wide integers are a good idea5 -
Imagine saving Integers and Floats in a MySQL table as strings containing locale based thousand sepatators...
man... fickt das hart!
Wait, there's more!
Imagine storing a field containing list of object data as a CSV in a single table column instead of using JSON format or a separate DB table.... and later parsing it by splitting the CSV string on ";"...7 -
Why yes, it makes total sense for a variable called `$connectionString` to only accept either booleans or integers ... wat
Also beware the beauty on how well that names conveys the variable's intent.
FML3 -
While trying to integrate a third-party service:
Their Android SDK accepts almost anything as a UID, even floats and doubles. Which is odd, who uses those as UIDs? I pass an Integer instead. No errors. Seems like it's working. User shows up on their dashboard.
Next let's move onto using their data import API. Plug in everything just like I did on mobile. Whoa, got an error. "UIDs must be a string". What. Uh, but the SDK accepts everything with no error. Ok fine. Change both the SDK and API to return the UID as a string. No errors returned after changing the UIDs.
Check dashboard for user via UID. Uh, properties haven't been updating. Check search properties. Find out that UIDs can only be looked up as Integers. What? Why do you ask me to send it as a string via the API then? Contact support. Find out it created two distinct records with the UID, one as a string and the other as an Integer.
GFG.3 -
You know what just gets to me about garbage-collected languages like c# and Java? Fucking dynamic memory allocation (seemingly) on the stack. Like it's so bizzare to me.
"Hey, c#, can I have an array of 256 integers during run-time?"
"Ya sure no prob"
"What happens when the array falls out of scope"
"I gotchu fam lol"8 -
PHP arrays.
The built-in array is also an hashmap. Actually, it's always a hashmap, but you can append to it without specifying indexes and PHP will use consecutive integers. Its performance characteristics? Who knows. Oh, and only strings, ints and null are valid keys.
What's the iteration order for arrays if you use them as hashmaps (string keys)? Well, they have their internal order. So it's actually an ordered hashmap that's being called an array. And you can produce an array which has only integer keys starting with 0, but with non-sequential internal (iteration) order.
This array weirdness has some non-trivial implications. `json_encode` (serializes argument to JSON) assumes an array corresponds to a JSON array if its keys are consecutive integers in increasing order starting with 0, otherwise the array becomes a JSON object. `array_filter` (filters arrays/hashmaps using callback predicate) preserves keys, so it will punch holes in the int key sequence if non-last items are removed, thus turning arrays into hashmaps and changing your JSON structure if you forget to discard keys before serialization.
You may wonder how JSON deserialization works, then? There's a special class for deserialized JSON objects, `stdClass`. It's basically a hashmap too, but it's an object, not an array, and all functions that would normally accept arrays won't work with it. So basically its only use is JSON (de)serialization. You can even cast arrays to objects, producing `stdClass`.
Bonus PHP trivia:
Many functions return nonsensical values. `preg_match`, the regex matching function, returns 1 for success, 0 for no matches and false for malformed regular expression. PHP supports exceptions, so it could just throw one on errors. It would even make more sense to return true, false and null for these three cases. But no, 1, 0 and false. And actual matches are returned by output arg.
`array_walk_recursive`, a function supposed to recursively apply callback to each element of an array. That's what docs say. It actually applies it to leafs only. It will also silently accept object instead of array and "walk" it, but without recursing into deeper objects.
Runtime type enforcing is supported for function arguments and returned values. You can use scalar types, classes, array, null and a few special keywords. There's also a `mixed` keyword, which is used in docs and means "anything". It's syntactically valid, the parser will accept it, but it matches no values in runtime. Calling such function will always cause a runtime error.
Strings can be indexed with negative integers. Arrays can't.
ReflectionClass::newInstanceWithoutConstructor: "Creates a new class instance without invoking the constructor". This one needs no commentary.
`array_map` is pretty self-explanatory if you call it with a callback and an array. Or if you provide more arrays of equal length via varargs, callback will be called with more arguments, one from each array. Makes sense so far. Now, you can also call `array_map` with null instead of callback. In that case it treats provided arrays as rows of a matrix and returns that matrix, transposed.5 -
There are so many weird hacks in the quite legacy app I work with I could write a book about all them hacks…
But I must admit, the worst of them all is internal time. Yes, so some blockhead thought it’s a good idea to represent time in a manner completely removed from Datetime objects or timestamps or even string representations. Instead we deal with them as intervals represented by integers - and because this is not fucked up enough by itself, the internal time doesn’t start at midnight, yet the integer representations do. It’s a bloody mess. No wonder most of the bugs we face have to do with dates and time…5 -
Security!
Offensive and defensive at both code and infrastructure levels.
So many times I see devs not give a flying pancake about security. Whether it be rolling integers for sql injection or permission guarding to prevent someone executing something they shouldn't.
Why is security in this industry always the last thing to be concerned about when it's the first thing that's going to kill your business.
😓7 -
Not Speaking The Same Programming Language
(It is the mid 80s, and I have a coworker come to me with two full pages of computer programming source code.)
Coworker: “Hey, can you help me with this? This function is not working right.”
Me: “Sure. What’s it do?”
Coworker: “Well, on the first line I copy…” *drones on for a few seconds about stuff I can clearly read*
Me: “Wait! Let me interrupt for a moment. I can read the code. In 20 words or less, what does this do?“
Coworker: *long pause that tells me he’s having trouble seeing the forest for the trees* “It, um, converts a date that’s a string to three integers: month, day, and year.”
Me: “Ah! Excellent. And by the time you get the string, has it been sanitized? You know, guaranteed to be pairs of digits with a slash in-between, not blanks or words or other garbage?”
Coworker: “Oh, yeah, all the user input is cleaned up.”
Me: “Okay, good.”
(I scribble “sscanf(text, “%02d/%02d/%02d”, &month, &day, &year);” in a blank spot on the page.)
Me: “Throw out everything and replace it with that.”
Coworker: “You’re kidding.”
Me: “Not at all. Use that. It’ll work. Trust me.”
Coworker: *not sure* “Well, okay.”
(Half an hour later he’s back and looking a bit sheepish.)
Coworker: “That worked. Thanks.”
Me: “No problem.”
(It’s been 30 years. Unfortunately, the new generation of programmers is in the same spot.)
https://notalwaysright.com/not-spea...2 -
The best thing about perl is it doesn't care about errors and really tries to do what you ask, without throwing exceptions.
The worst? It does exactly what you ask, no matter how insane.
Typed $arri[ $0 ] instead of $arr[0] inside a function that detected what changes were needed in dns zones. $0 is script name and path, strings are converted to integers as needed and there's a little thing called vivification.
You see where this train wreck is going.
Also my dog died today.
Got to love Mondays :/11 -
Oh gee whiz fellas. I lived through my nightmare. Recently too.
(Multiple rants over last few months are merged in this one. Couldn't rant earlier because my login didn't work.)
I joined a new shithole recently.
It was a huge change because my whole tech stack changed, and on top of that the application domain was new too.
Boss: ho hey newbie, here take this task which is a core service redesign and implementation and finish it in two weeks because it has to be in production for a client.
Normally I'd be able to provide a reasonable analysis and estimate. But being new and unaware of how things work here, I just said 'cool, I'll try my best.' (I was aware that it was a big undertaking but didn't realize the scope and the alarming lack of support I'd get and the bullshit egos I'd have to deal with)
Like a mad man I worked 17+ hours a day with barely a day off every week and changed and produced a lot of code, most of it of decent quality.
Deadline came and went by. Got extended because it was impossible (and fake).
All the time my manager is continuously building pressure on me. When I asked questions I never got any direct/clear answers. On asking for help, I'd get an elaborate word vomit of what was already known/visible. Yet I finally managed to have an implementation ready.
Reviewer: You haven't added parameter comments on your functions and there aren't enough comments in code. We follow standards. Clean code and whatnot. Care for the craft verbal diarrhea.
Boss: Ho hey anux, do you think we'll be able to push the code to production?
Me: Nope. We care for the craft and have standards. We need to add redundant comments to self documented code first, because that is of utmost importance as Nuthead reviewer explained.
(what I wish I had said)
What I actually said: No, code is not reviewed yet.
And despite examples of functions which were not documented (which were written by the reviewer nut), I added 6-7 lines of comments for my single line functions describing how e.g. Sum takes two input integers and returns their sum and asked for a review again.
Reviewer: See this comment is better written as this same-meaning-but-slightly-longer way. Can we please add full stops everywhere even though they were not there to begin with? Can we please not follow this pattern and instead promote our anti-pattern? Thanks.
Me: Changed the comments. Added full stops. Here's a link for why this anti-pattern is bad.
Reviewer: you have written such beautiful code with such little gems. Brilliant. It's great to see how my mentoring has honed your skills.
.
.
.
I swear I would have broken a CRT on his stupid face if we weren't working remotely (and if I had a CRT).
It infuriates me how the solution to every problem with this guy is 'add a comment'.
What enrages me more is that I actually thought I could learn from this guy (in the beginning). My self doubt just made me burnout for little in return.
Thankfully this living nightmare will soon be over.rant fuck you shitty reviewer micromanagement by micrococks wk279 living nightmare fml glassdoor reviews don't lie9 -
So in my class the teacher wanted us to write a little program (not going into further details) and therefore we needed to convert chars from a string to integers.
My classmates are starting like:
if (c = '0')
return 0;
else if (.....
And so on. I was just like duuuuuh wtf are u doing? I was actually screaming when I had a look at my neighbours screen.
I personally am like "return c - 48;"15 -
Just finished a large write up on a security flaw I found and disclosed in an exam spyware vendor's "zero knowledge encryption" - derived keys that were generated from incrementing integers, discussion on obfuscation and more.
It was a hell of a time writing this up; not sure if linking my personal blog here would be bad practice, but here it is: https://proctor.ninja/wave-rake-pro...
It's been something else, but hopefully I can keep fighting against tech like this on school campuses.4 -
After a year of college give everyone 2 hours to solve a programming problem in the language of their choice. Like implement a doubly linked list, or count the number of primes between two integers or something straightforward. Anyone who can’t do it gets kicked out of the major.
I’m sick of dealing with people who are 3 or 4 years into a CS degree, and can’t do 30-line programming assignments in two weeks. I might have to work with one of these clowns someday and I hope to God that my university doesn’t send them into the workforce with a degree.3 -
Inspired by @shahriyer 's rant about floating point math:
I had a bug related to this in JavaScript recently. I have an infinite scrolling table that I load data into once the user has scrolled to the bottom. For this I use scrollHeight, scrollTop, and clientHeight. I subtract scrollTop from scrollHeight and check to see if the result is equal to clientHeight. If it is, the user has hit the bottom of the scrolling area and I can load new data. Simple, right?
Well, one day about a week and a half ago, it stopped working for one of our product managers. He'd scroll and nothing would happen. It was so strange. I noticed everything looked a bit small on his screen in Chrome, so I had him hit Ctrl+0 to reset his zoom level and try again.
It. Fucking. Worked.
So we log what I dubbed The Dumbest Bug Ever™ and put it in the next sprint.
Middle of this week, I started looking into the code that handled the scrolling check. I logged to the console every variable associated with it every time a scroll event was fired. Then I zoomed out and did it.
Turns out, when you zoom, you're no longer 100% guaranteed to be working with integers. scrollTop was now a float, but clientHeight was still an integer, so the comparison was always false and no loading of new data ever occurred. I tried round, floor, and ceil on the result of scrollHeight - scrollTop, but it was still inconsistent.
The solution I used was to round the difference of scrollHeight - scrollTop _and_ clientHeight to the lowest 10 before comparing them, to ensure an accurate comparison.
Inspired by this rant: https://devrant.com/rants/1356488/...2 -
I want honest opinions. Do you think the following is a good or not so good interview question. Why or why not? Defend your argument.
Define a function where the input is a list of integers. It should find and return all the unique sets of three within the list that sum to x.
For example, given the list [1, 3, 2, 5, 6, 8, 10, 13, 15] and with x = 16, the function would return [(10,5,1), (13,2,1)]
If the candidate presents the trivial solution with time complexity of o(n^3), ask if can be done in o(n^2) or better.7 -
Some days I feel like I really know what I am doing and today was not one of these days...
Working on a game engine using Vala and now using Raylib in the backend for rendering and input.
Wrote a VAPI for Raylib and when I was doing the 'Rectangle' struct... I made it's members integers when they are floats...
So this whole time; when using a camera everything would jitter like crazy.... because I was taking the transform which is all floats... rounding it then casting to an int only for the int to be cast into a float again....
Lo and behold; changing the members to floats and removing the rounding and casting makes everything silky smooth...
I have been debugging every bit of my current render loop trying to work this out when it was 100% unrelated.... I hate myself sometimes -
!rant Eeeeeeeeee!!!! The interpreter can now handle floats and integers! And accept the power operator! And modulo! (Sorry, absurdly excited)2
-
I spent the whole day coding in python (usually I code in php or perl) and this language is a fucking joke. C'mon, why everything have to be done in such a weird way? And don't say it's python way because it's bullshit way. Want some examples?
", ". join(str(x) for x in array)
to join array of integers. wtf is that?
True|False
why in hell you need the first letter to be uppercase when your own fucking standard says to use lowercase letters in eg. var names and method names. why?
math.isnan(float(x))
to check if a variable (expected to be integer) is NaN. I won't fucking comment that...
Even prolog don't have such stupid things6 -
A buddy of mine sent this to me from a first year procedural programming course.
The email was in regards to an assignment where they had to print some statistics. The caps letters are the response from the lecturer...
Integers with decimal places hey?3 -
FUCKING HELL.
It's already enough that this FUCKING API I have to work with is a mess of JSON and XML responses mixed together.
With mixed german and english keys and attributes all sprinkled over it.
And API access locked to Austria only for some reason.
And then they even manage to fuck up the little bit of JSON they use.
It's just a fucking array of strings (where one could easily use integers).
They can stick this fucking steaming pile of shit that they call API up their PHP infested assholes.
I hate web development sometimes.5 -
When you spend 1 hour trying to solve a programming challange... And then realise "All integers starting with 1" means all integers from 1 to inf, and not all integers that begin with the digit 1. UUUGGH2
-
Question - is this meaningful or is this retarded?
if
2*3 = 6
2*2 = 4
2*1 = 2
2*0 = 0
2*-1 = -2
then why doesnt this work?
6/3 = 2
6/2 = 3
6/1 = 6
6/0 = 0
6/-1 = -6
if n/0 is forbidden and 1/n returns the inverse of n, why shouldn't zero be its own inverse?
If we're talking "0" as in an infinitely precise definition of zero, then 1/n (where n is arbitrarily close to 0), then the result is an arbitrarily large answer, close to infinite, because any floating point number beneath zero (like an infinitely precise approximation of zero) when inverted, produces a number equal to or greater than 1.
If the multiplicative identity, 1, covers the entire set of integers, then why shouldn't division by zero be the inverse of the multiplicative identity, excluding the entire set? It ONLY returns 0, while anything n*1 ONLY returns n.
This puts even the multiplicative identity in the set covered by its inverse.
Ergo, division by zero produces either 0 or infinity. When theres an infinity in an formula, it sometimes indicates theres been
some misunderstanding or the system isn't fully understood. The simpler approach here would be to say therefore the answer is
not infinity, but zero. Now 'simpler' doesn't always mean "correct", only more elegant.
But if we represent the result of a division as BOTH an integer and mantissa
component, e.x
1.234567 or 0.1234567,
i.e. a float, we can say the integer component is the quotient, and the mantissa
is the remainder.
Logically it makes sense then that division by zero is equivalent to taking the numerator, and leaving it "undistributed".
I.e. shunting it to the remainder, and leaving the quotient as zero.
If we treat this as equivalent of an inversion, we can effectively represent the quotient from denominators of n/0 as 1/n
Meaning even 1/0 has a representation, it just happens to be 0.000...
Therefore
(n * (n/0)) = 1
the multiplicative identity
because
(n* (n/0)) == (n * ( 1/n ))
People who math. Is this a yea or nay in your book?21 -
Here are some of the variable types in java if someone needs it :)
-String myString = "Hello";
//integeres
- Byte myByte = 127; [It can hold up to 128
-Short myShort = 530; [up to 32767] -> 2 bytes
-int myInt = 8021; [up to 2,147,483,647] -> 4 bytes]
-long myLong = 213134; [ up to 9,223,372,036,854,755,807] -> 8bytes
//Decimal Numbers
-float myFloat = 3.14f;
-double myDouble = 23.45;
P.S.
I hate double... idk why
Bye12 -
More marks for a bubble sort.
For those of you that know the bubble sort you may share my frustration. I built a simple python program that took three integers and sorted them using a series or compound if statements using no built in low and max functions. Someone in my college class did the same thing but used a bubble sort and got higher marks. This angers me, I had to write an algorithim in a language I had barely touched but this person just used old scrappy code and got higher marks. Only a little tease but you get the picture, bubble sort is inefficient.2 -
ok, fuck people. i mean the people who talk about things that are a big deal. you don't need to take a course in html/css to build a website, you need documentation.
people act like programming languages are a whole separate literacy. they're not. it is not a big deal, nor an accomplishment of any significance, to learn any language to a basic extent. variables, control flow, functions and scope should not be considered challenging topics, and people should stop bragging about them. i'm pretty sure this is because programming is new. as people, i think when something is new we tend to think of it as more complex and harder to understand. basic programming is not that.
ok that was a tangent from my real point. college is a scam. anyone can learn anything from books and the internet. any time you want to learn about something, go to google, and search "${my topic} site:*.github.io" and you'll have a page about that topic written by someone who is knowledgeable and passionate of the topic. colleges don't teach people how to think like these books/websites do. and i'm fucking sick of people who'd rather see a degree then a portfolio. fuck them shits bro. i can distinct my smart friends because my smart friends speak logically and enjoy becoming smarter. i would take the kid who watches aerodynamics videos on youtube and then built a plane over a kid who studied and got a five on his ap physics exam. watching then doing is better learning than watching and repeating. after all, creativity is not at all measured in our grades, and i'd like to argue that sometimes intelligence isn't even measured. i mean, people can say they're good at math, but the kids who talk about fibinnoci numbers and why there can never be two primes more than 7 (i if i remember properly) integers apart or the ones who prove cryptographic algorithms. i guess what i'm trying to say is the dumb kids aren't dumb and the smart kids aren't smart (well not that) but kids who are passionate and just do something instead of waiting for their degree to do the same thing are the best and brightest. i forgot what i was talking about. sorry it is almost 2 am and i am intoxicated , and i don't believe i got my point across very well either.7 -
When we subtract some number m from another number n, we are essentially creating a relationship between n and m such that whatever the difference is, can be treated as a 'local identity' (relative value of '1') for n, and the base then becomes '(base n/(n-m))%1' (the floating point component).
for example, take any number, say 512
697/(697-512)
3.7675675675675677
here, 697 is a partial multiple of our new value of '1' whose actual value is the difference (697-512) 185 in base 10. proper multiples on this example number line, based on natural numbers, would be
185*1,
185*2
185*3, etc
The translation factor between these number lines becomes
0.7675675675675677
multiplying any base 10 number by this, puts it on the 1:185 integer line.
Once on a number line other than 1:10, you must multiply by the multiplicative identity of the new number line (185 in the case of 1:185), to get integers on the 1:10 integer line back out.
185*0.7675675675675677 for example gives us
185*0.7675675675675677
142.000000000000
This value, pulled from our example, would be 'zero' on the line.
185 becomes the 'multiplicative' identity of the 1:185 line. And 142 becomes the additive identity.
Incidentally the proof of this is trivial to see just by example. if 185 is the multiplicative identity of 697-512, and and 142 is the additive identity of number line 1:185
then any number '1', or k=some integer, (185*(k+0.7675675675675677))%185
should equal 142.
because on the 1:10 number line, any number n%1 == 0
We can start to think of the difference of any two integers n, as the multiplicative identity of a new number line, and the floating point component of quotient of any number n to the difference of any number n-m, as the additive identity.
let n =697
let m = 185
n-m == '1' (for the 1:185 line)
(n-m) * ((n/(n-m))%1) == '0'
As we can see just like on the integer number line, n%1 == 0
or in the case of 1:185, it equals 142, our additive identity.
And now, the purpose of this long convoluted post: all so I could bait people into reading a rant on division by zero.26 -
First you make a filthy JSON protocol where numbers are encapsulated into strings.
Then you document this little fact nowhere. Actually you don't document anything at all.
Then you make a shitty parser that ignores any exception. So that when I try to send my objects, it took two hours to figure out it was "my fault" as I was sending actual integers instead of strings.
I think you deserve to suffer a terrible agony for exactly the amount of time I lost.2 -
Lets play a little python game together and win some devRant points :
We have an array of integers named L
We need to sort this array so that the biggest number be in the beginning and lowest at the end..descending order
Rules:
-You are not allowed to write more than one line of code in the comment...only one
-if you comment once...you cannot comment directly after that...you have to wait for two other comments after you so you can comment again
-you have to build your code upon the previous comments ... you cannot start from scratch
-the lucky one who puts the final comment is the winner..and we should all ++ his comment to give him the biggest amount of points
Lets start and see who will win :)15 -
Trying to convince the class that test-driven development + DTSTTMPW ("do the simplest thing that might possibly work") + pair programming is the way to go, our software dev prof had us split in groups of two that would each get a turn to
1. add a unit test
2. edit the code so it passes the test
3. commit the change
The goal was to write a java class that converts integers to roman numerals.
Each group had only 2 minutes before the prof made them revert their changes.
After 45 minutes the code was just 10 lines of this:
if ( n == 1 )
return "I";
else if ( n == 2 )
return "II";
else if ( n == 3 ) ... -
So I have a problem and I was hoping for some insight.
I figured out how to get
(surd(n, x)-surd(n, y))
without knowing x or y, (only n), through a convergent series of approximate identities.
n is the product of x and y, where x<y
My only issue is I don't know where to go from here. I've basically hit the limit of my insight into the problem.
surd() here is just a function that returns the results of two arguments, a, b, such that (a^2)-b.
Both are guaranteed to be positive integers, greater than 1.
But, having come this far, with a couple pages of intermediate identities, I'm at a loss.4 -
i'm starting a project where i will have a large amount audio clips, anywhere from a few seconds to about an hour long, and i need to store them based on which user created them and what group they are created in (so they will be sorted based on two integers). i'll need to concatenate and/or merge the audio files frequently, and i may need to filter which audio i use based on users and time created.
how should i store the audio? i'm pretty sure a database is the best option, but should i consider using the file system? if i shant, should i use mysql or postgres? i know postgres has more types and supports complex queries.
does anyone have experience who can help?8 -
So I got assigned to this project last week to help other developers to remove bugs from a android app. First bug I have to deal with: field that should only accept integers is accepting other characters and thus crashes the app.
Alright seems like a simple bug to get into the project and Xamarin. So I set some break points and find the bug: "uncaught FormatException on line 789 Convert.ToInt(string, v) .
OK then, implement some try-catch and add a warning message to the user.
let's try it out... alright, message works, close message and app crashes
-Tsc... dammit
search for the bug in code... "uncaught FormatException on line 899 Convert.ToInt(string, v)"
what the...
wait a minute, ~ Ctrl + F ~ - "Convert.ToInt"
17 matches on file
oh fuck me...2 -
"Most memorable bug you fixed?"
A recent instance happened in one of my Scratch projects, and the bug involved "Infinities."
I had an opportunity to teach kids programming, and it involved Scratch. So, to have something to show those kids at least, I decided to make a small game.
In that game, I had an object that takes some time before appearing after being cloned (i.e., instantiated.) The duration was calculated by dividing a constant with a variable:
[Wait for ((3) / (variable)) seconds]
The bug is that I forgot about the case where 'variable' can be 0, which is classic and insignificant.
Well, the thing is that I learned two things the hard way:
1: Scratch is very flexible about integers and floats (e.g., at one second, it looks like an integer, but one operation later, it's a float.)
2: Scratch does not provide any 'runtime errors' that can crash the project.
In other languages, similar "wait" methods take "milliseconds" in an integer, so it would have barfed out a "DivideByZeroException" or something. But Scratch was so robust against project-crashing behavior that it literally waited for f*<king "infinity seconds," effectively hanging that clone without warning or runtime errors. This masked my bug. It took way too long to debug that s#!+.
Don't blanket-mask any errors. -
First post on devRant... Aaaaand it's university hw... I can't wrap my head around this...
So, the problem is: I have to implement writing and printing 64 bit decimal integers (negative and positive with 2s complement) in NASM Assembly. There are no input parameters, and the result should be in EDX:EAX. The use of 64 bit registers is prohibited.
There is a library which I can use: mio.inc
It has these functions:
- mio_writechar (writes the character which corresponds to the ASCII code stored in AL to console)
- mio_readchar (reads an ASCII character from console to AL)
It also has to manage overflow and backspace. An input can be considered valid or invalid only after the user hits Enter... It's actually a lot of work, and it's just the first exercise out of 10... 😭
The problem is actually just the input - printing should be easy, once I have valid data...
Please help me!3 -
https://isevenapi.xyz/
If anyone needs parity checking for integers in SaaS form, definitely check this out!6 -
I just found out, there is a GCC flag that prevents you from using integers in a bool context in C.
Like wtf?!
Not only is this the original "bool" implementation of C, but it's also a widespread concept for use in NULL checks and the likes.5 -
Dude closes EVERY issue on his repo IMMEDIATELY with "Why would you need to do that?" Yeah, you're right- it's totally unreasonable to expect my database interface to support integers other than int64. Ugghh1
-
This internal api is killing me. Why the fuck do people return an array of numbers inside a field that has a generic name such as `icons` to convey information such as "hasOptionFooIncluded". Because of course then icons contains '6'. Yet if both 6 and 4 are in there, it means something else. Needless to say there is no documentation whatsoever what each number or group of numbers actually means so I have to ask around to find out what numbers means what in order to wrap that call away into something maintainable. Because the API is deprecated and we don't want to fix shit in there. We just create other shit depending on this crappile. :/
Stop using magic numbers. Just stawp it! -
Up all damn night making the script work.
Wrote a non-sieve prime generator.
Thing kept outputting one or two numbers that weren't prime, related to something called carmichael numbers.
Any case got it to work, god damn was it a slog though.
Generates next and previous primes pretty reliably regardless of the size of the number
(haven't gone over 31 bit because I haven't had a chance to implement decimal for this).
Don't know if the sieve is the only reliable way to do it. This seems to do it without a hitch, and doesn't seem to use a lot of memory. Don't have to constantly return to a lookup table of small factors or their multiple either.
Technically it generates the primes out of the integers, and not the other way around.
Things 0.01-0.02th of a second per prime up to around the 100 million mark, and then it gets into the 0.15-1second range per generation.
At around primes of a couple billion, its averaging about 1 second per bit to calculate 1. whether the number is prime or not, 2. what the next or last immediate prime is. Although I'm sure theres some optimization or improvement here.
Seems reliable but obviously I don't have the resources to check it beyond the first 20k primes I confirmed.
From what I can see it didn't drop any primes, and it didn't include any errant non-primes.
Codes here:
https://pastebin.com/raw/57j3mHsN
Your gotos should be nextPrime(), lastPrime(), isPrime, genPrimes(up to but not including some N), and genNPrimes(), which generates x amount of primes for you.
Speed limit definitely seems to top out at 1 second per bit for a prime once the code is in the billions, but I don't know if thats the ceiling, again, because decimal needs implemented.
I think the core method, in calcY (terrible name, I know) could probably be optimized in some clever way if its given an adjacent prime, and what parameters were used. Theres probably some pattern I'm not seeing, but eh.
I'm also wondering if I can't use those fancy aberrations, 'carmichael numbers' or whatever the hell they are, to calculate some sort of offset, and by doing so, figure out a given primes index.
And all my brain says is "sleep"
But family wants me to hang out, and I have to go talk a manager at home depot into an interview, because wanting to program for a living, and actually getting someone to give you the time of day are two different things.1 -
A bit longer rant, somehow triggered by the end of this rant:
https://devrant.com/rants/7145365/...
The discussion revolved around strpos returning false or a positive integer.
Instead of an Option or a Exception.
I said I'm a sucker for exception, but I'm also a sucker for typing.
Which is something most languages lack - except the lower level ones like C / C++.
I always loved languages which have unsigned and signed types.
There, I said it... :) I know that signed / unsigned is controversial, Google immediately leads to blog entries screaming bloody murder because unsigned can overflow – or underflow, if someone tries to use a -1on an unsigned integer.
Note that my love is only meant for numeric types, unsigned / signed char is ... a whole can of insanity on its own.
https://phoronix.com/news/...
If you wanna know more.
Back to the strpos problem, now with my secret love exposed:
strpos works on a single string, where a string is a sequence of chars starting with 0.
0 is a positive integer.
In case the needle (char that should be looked up in the string) cannot be found in the haystack (the string), PHP returns "false".
This leads to the necessity of explicitly checking the type as "0" (beginning of string, a string position)... So strpos !== false.
PHP interprets 0 as false, any other integer value is true.
In the discussion, the suggestion came up to return -1 if a value could not be found – which some languages do, for example Scala.
Now I said I have a love for unsigned & signed integers vs. just signed integers...
Can you guess why the -1 bothers me very much?
Because it's a value that's illogical.
A search in a sequence that is indexed by 0 can only have 0 or more elements, not less than zero elements.
-1 refers to a position in the sequence that *cannot* exist.
Which is - of course - the reason -1 was chosen as a return value for false, but it still annoys me.
An unsigned integer with an exception would be my love as a return value, mostly because an unsigned integer represents the return value *best*. After all, the sequence can only return a value of 0 ... X.
*sigh*
Yes, I know I'm weird.
I'm also missing unsigned in Postgres, which was more or less not implemented because it's not in the SQL standard...
*sob*29 -
Python..... please cast my integers and such to string for me. I don't want to have to keep typing str().... pretty please?4
-
Could somebody please help me understand why the fucking hell does JS (I am talking about node.js, so backend) use 32bit integers in setTimeout and setInterval? I mean, I understand most of the choices regarding the language (I have chosen programming languages design and principles for my studies) and I am happily using it for almost 4 years. But I came across an occasion that I had to use big numbers in those functions and it took me a lot of time to figure out why the fuck my code was not working as it was supposed to.
If anyone has a good reason please elaborate. In the meantime I'll go punch some shit to calm down.10 -
We have a somewhat experience developer for whom we have to CONSTANTLY fix his type errors. He just doesn't seem to grasp the idea that there is a difference between integers, strings, floats, etc. and that when you don't bother with them, things get a little screwed up.
Granted, Javascript's typing leaves... well, everything.. to be desired, but STILL dude, this is basic shit. Come on.2 -
Any X11 people in devrant?
Is there a way to listen to child window events from a parent?
I'm getting all the `XFocusChangeEvent`s if I use the root window, but then I can't get the original window integers where those events are happening.6 -
!rant
C++ / OOP QUESTION
I have a uni assignment / project (Data Structures class), where I have to implement the ins-n-outs of 1D arrays, by creating a dynamically allocated array class, which can accept any type of data (using templates). But there's a problem.
I'd like to implement sorting the elements of the array. But given the fact, that I'm using templates, I cannot treat the elements as integers, nor as strings, or other types...
Also, let's say that the elements of the array are elements of class T, where T looks like this:
class T {
private:
double height;
int age;
string name;
public:
double getH() { return height; }
int getAge() { return age; }
string getName() { return name; }
};
(It's just a random example, pls don't judge for code quality...)
Let's say that I'd like to sort the T elements based on height, print out, sort by age, print out, then sort by name and print out. How can I do this? Is this possible?5 -
https://i.postimg.cc/4ycRFNZf/...
The factorization shit I'm always ranting about? I decided for once to explain it visually in this handy dandy little infographic.
We're essentially transforming the product from an unsmooth set of potential factors in its factor tree, to a factorization tree that guarantees first that the set of potential factors are all 2, 3, 5, and a or b of p, and second, that all the factors are *smooth integers* of a or b.
This is basically what Adi Shamir was trying to do with TWINKLE and TWIRL, despite checking a hundred thousand+ potential primes.
I did it in four.6 -
Why the hell do people still use Java? It's so slow, messy, insecure, and heck, it doesn't even have unsigned integers, bytes etc.? Like wtf?34
-
DailyCodingProblem: #1
Given an array of integers, return a new array such that each element at index i of the new array is the product of all the numbers in the original array except the one at i.
For example, if our input was [1, 2, 3, 4, 5], the expected output would be [120, 60, 40, 30, 24]. If our input was [3, 2, 1], the expected output would be [2, 3, 6].
this is my quickly solution in php:
$input_array = [1, 2, 3, 4, 5];
echo('INPUT ARRAY:');
print_r($input_array);
echo("<br/>");
foreach($input_array as $key => $value){
$works_input_array = $input_array;
unset($works_input_array[$key]);
$result[] = array_product($works_input_array);
}
echo('OUTPUT ARRAY:');
print_r($result);
outpout:
INPUT ARRAY:Array ( [0] => 3 [1] => 2 [2] => 1 )
OUTPUT ARRAY:Array ( [0] => 2 [1] => 3 [2] => 6 )5 -
My tech lead rookie mistake of the week. Makes ids in the MySql DB big integers.
Now react, python and pinecone are all converting them to scientific values resulting into invalid or missing values and lookups.
Fml I need to change companies.12 -
Had a list of integers: 0,2,1,0,1,2,2,0. Needed to add a variable number of integers and stay as close as possible the current average.
Used their percentage to solve this. Didnt work. After a few hours I realised it is just to pick a random in the list as many times that I need to add integers.
Say I have 32 integers and need 36, just pick 4 by random, problem solved. Fuck I will never get those hours back... :( -
Not really an impressive one, but I think it's blasphemous enough to be mentioned:
Creating an embedded application, it was not supported to print a float using optimized libraries (understandable since they're not really supposed to be used anyways), but I was too lazy to convert clocks to a time unit by hand while running benchmarks. So I just printed the float as two integers, splitting it to one for the whole and one for the thousands. -
Hmmm, doing a little side project which requires me to store "money" I am uncertain if the approach should be integers with cents or dollars as decimals (19,4) in a MySQL/php project.. Any thoughts?5
-
It's always some dumb thing that goes wrong. Like, a semicolon I forgot to type, or a period or a misspelled variable. Last week I spent two hours on a Discord Bot coded in Python that refused to iterate a list of names. I had forgotten to put quotation marks around each of the items in the list so they would register as strings instead of integers. Will it ever get better?6
-
If we can transform the search space or properties of a product into a graph problem
we could possibly use Kirchhoff's theorem to reveal products which are 'low complexity'
in particular search spaces, yeah?
Now according to
https://en.wikipedia.org/wiki/...
"n Cycle Space, A family of sets closed under the symmetric difference operation can be described algebraically as a vector space over the two-element finite field Z 2 {\displaystyle \mathbb {Z} _{2}} \mathbb{Z } _{2}.[4] This field has two elements, 0 and 1, and its addition and multiplication operations can be described as the familiar addition and multiplication of integers, taken modulo 2"
Wouldn't this relate to pollards algorithm, because it involves looking for factors of coprimes modulo N or am I mistaken?
Now, according to wikipedia, "in a group, the additive identity is the identity element of the group, is often denoted 0, and is unique."
If we make the multiplicative identity of our ring or field a tuple of the ratio of a/b for some product p, or a (and a/w, where w is the square root of p), or any other set such that n*m allows us to derive a or b, we could reduce the additive identity to the multiplicative identity, making the ring trivial. Solving for p would then mean finding a function from R to R, mapping every number to 0, i.e. finding the additive identity.
Now in a system with a multiplication operation the distributes over addition, the "additive
identity annihilates ring elements", so naturally, the function that maps to 0, gives us
our additive identity, we need only find the subset, no?
Forgive me if I'm wrong, but shouldn't this be convertible to a graph search?
I'm WAY out of my depth here so if anyone is familiar and can enlighten me I'd be grateful.
It's all unknown unknowns to me. -
this is my first actual rant. I am trying to learn es6 right now and have encountered switch statements for the first time. after a 26 minute video explaining how to do switch statements (which is literally just the same kind of information on them that you can get from w3 or mdn I am given a large task with no practice to create a switch function that hold four values two values containing positive integers and two values accepting strings. then I have to be able to pass days and minutes through it.
an example solution after input would be:
addTime(1,"hour",3,"minutes")
I feel like this is too complicated with 26 minutes of information and no practice exercises to prepare for that.
-end rant7 -
Its everyones favorite time again. Wisecrack's 8th grade hoborants about mathematics.
Lets start with the example
a=89
b=223
p=a*b=19847
If
(1/(5/p))/b = 17.8
and naturally
p/5 =3969.4
3969.4/b = 17.8
What I find interesting is that...
p/17.8 = 1115.0
..for any product and factors (given two factors), the result will always be an integer.
Why is this?
You can see that
t= 1115.0*b = 248645.0
And if
17.8*(p/a) = 3969.4
Then
17.8*(t/p) = 223.0 (our factor, b)
a*(t/p)
1115.0
p/1115
17.8
also a*(t/p) = 1115.0
I could be once again misunderstanding but
what it looks like is that theres some real number that always transforms p into an integer on the ring of integers (Z) representing multiples of the factors of p.
Now notice
b/17.8 = 12.52808988764045
We can also get that number like so..
t/p = 12.52808988764045
I think (though I could be mistaken) is that the reason is because t is b*1115 and 12.52808988764045 is the ratio between b and 17.8 as well as the ratio between
p and 1115.
And if we do
t/√p = 1764.9495488858483
1764.9495488858483^2 = 3115046.9101123596
also incidentally
3115046.9101123596/t =12.52808988764045
3115046.9101123596/12.52808988764045 =
t (this is obvious but I want to point it out anyway), or 248645.0
and
1115/b = 5.0
248645.0/5 = 49729.0
and
√49729.0 = b
Why is this last part true, that √(t/5) = b?11 -
Hang with me! This is *not* a math shitpost, I repeat, it is NOT a math shitpost, not entirely anyway.
It appears there is for products of two non-trivial factors, a real number n (well a rational number anyway) such that p/n = i (some number in the set of integers), whos factor chain is apparently no greater than floor(log(log(p))**2)-2, and whos largest factor is never greater than p^(1/4).
And that this number is at least derivable, laboriously with the following:
where p=a*b
https://pastebin.com/Z4thebha
And assuming you have the factors of p/z = jkl..
then instead of doing
p/(jkl..) = z
you can do
p-(jkl) to get the value of [result] whos index is a-1
Getting the actual factor tree of p/z is another matter, but its a start.
Edit: you have to provide your own product.
Preferably import Decimal first.3 -
What's your idea of a perfect number?
Mine is:
default decimal, prefix for binary, octal, hex
Integers in natural notation with strictly positive exponent
All bases allow natural notation, where the exponent is always a decimal number and represents the power of the base (0b101e3 = 0b101000)
Floats in all bases allow a combination of natural notation and dot notation
Underscores allowed anywhere except the beginning and end for easier reading11 -
Let's say I take a matrix of high entropy random numbers (call it matrix J), and encode a problem into those numbers (represented as some integers which in turn represent some operations and data).
And then I generate *another* high entropy random number matrix (call it matrix K). As I do this I measure the Pearson's correlation coefficient between J (before encoding the problem into it, call Jb), and K, and the correlation between J (after encoding, let's call it Ja) and K.
I stop at some predetermined satisfactory correlation level, let's say > 0.5 or < -0.5
I do this till Ja is highly correlated with some sample of K, and Jb's correlation with K is close to 0.
Would the random numbers in K then represent, in some way, the data/problem encoded in Ja? Or is it merely a correlation?
Keep in mind K has no direct connection to J, Ja, or Jb, we're only looking for a matrix of high entropy random numbers that indicated a correlation to J and its data.
I say "high entropy", it would be trivial to generate random numbers with a PRNG that are highly correlated simply by virtue of the algorithm that generated them.12