Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
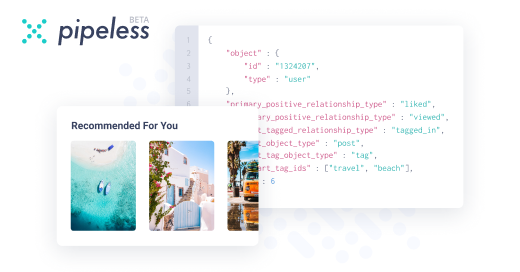
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "arr"
-
I was reading an article and stombled at this note:
when demonstrating arrays, always call them ‘arr’ – it allows you to talk like a pirate3 -
Fuck me, big fucking security flaw with a UK internet service provider, my head has gone through my desk and hit the floor it’s that bad.23
-
Junior dev complained about my request to remove unnecessary comments because they're too obvious. "They may be obvious to you, but not to others" he said.
The codes and the comments:
// Sort the array
arr.Sort()
// Return the first element of the array
return arr[0]14 -
I had to open the desktop app to write this because I could never write a rant this long on the app.
This will be a well-informed rebuttal to the "arrays start at 1 in Lua" complaint. If you have ever said or thought that, I guarantee you will learn a lot from this rant and probably enjoy it quite a bit as well.
Just a tiny bit of background information on me: I have a very intimate understanding of Lua and its c API. I have used this language for years and love it dearly.
[START RANT]
"arrays start at 1 in Lua" is factually incorrect because Lua does not have arrays. From their documentation, section 11.1 ("Arrays"), "We implement arrays in Lua simply by indexing tables with integers."
From chapter 2 of the Lua docs, we know there are only 8 types of data in Lua: nil, boolean, number, string, userdata, function, thread, and table
The only unfamiliar thing here might be userdata. "A userdatum offers a raw memory area with no predefined operations in Lua" (section 26.1). Essentially, it's for the API to interact with Lua scripts. The point is, this isn't a fancy term for array.
The misinformation comes from the table type. Let's first explore, at a low level, what an array is. An array, in programming, is a collection of data items all in a line in memory (The OS may not actually put them in a line, but they act as if they are). In most syntaxes, you access an array element similar to:
array[index]
Let's look at c, so we have some solid reference. "array" would be the name of the array, but what it really does is keep track of the starting location in memory of the array. Memory in computers acts like a number. In a very basic sense, the first sector of your RAM is memory location (referred to as an address) 0. "array" would be, for example, address 543745. This is where your data starts. Arrays can only be made up of one type, this is so that each element in that array is EXACTLY the same size. So, this is how indexing an array works. If you know where your array starts, and you know how large each element is, you can find the 6th element by starting at the start of they array and adding 6 times the size of the data in that array.
Tables are incredibly different. The elements of a table are NOT in a line in memory; they're all over the place depending on when you created them (and a lot of other things). Therefore, an array-style index is useless, because you cannot apply the above formula. In the case of a table, you need to perform a lookup: search through all of the elements in the table to find the right one. In Lua, you can do:
a = {1, 5, 9};
a["hello_world"] = "whatever";
a is a table with the length of 4 (the 4th element is "hello_world" with value "whatever"), but a[4] is nil because even though there are 4 items in the table, it looks for something "named" 4, not the 4th element of the table.
This is the difference between indexing and lookups. But you may say,
"Algo! If I do this:
a = {"first", "second", "third"};
print(a[1]);
...then "first" appears in my console!"
Yes, that's correct, in terms of computer science. Lua, because it is a nice language, makes keys in tables optional by automatically giving them an integer value key. This starts at 1. Why? Lets look at that formula for arrays again:
Given array "arr", size of data type "sz", and index "i", find the desired element ("el"):
el = arr + (sz * i)
This NEEDS to start at 0 and not 1 because otherwise, "sz" would always be added to the start address of the array and the first element would ALWAYS be skipped. But in tables, this is not the case, because tables do not have a defined data type size, and this formula is never used. This is why actual arrays are incredibly performant no matter the size, and the larger a table gets, the slower it is.
That felt good to get off my chest. Yes, Lua could start the auto-key at 0, but that might confuse people into thinking tables are arrays... well, I guess there's no avoiding that either way.13 -
-Let's start making the game!
-Yaay
-We should focus on performance optimization!
-But we don't have anything to optimize yet..
-Performance optimization!!!
1 month later
-OK you were right, we can't focus on performance now. We need to start making this game!
-Finally!
-So we're in pre-production now, let's do some R&D!
-Awesome, I wanted to start designing our workflow for adding new content, and maybe also loca..
-NO! That's unimportant! We must do R&D!
-OK what should we Arr-and-Dee?
-Performance optimization!!!5 -
In the darkest of days, I discovered how to remote login to my computer at work through the company vpn. I then proceeded to work overtime at night in secret for a week or so, writing documentation and refactoring code.
I finally woke the fuck up and realized that I shouldn't be obsessing over proprietary codebases that do not belong to me, and I should put this misguided energy into my own projects.
So yeah, as a bad dev habit I'm working on fixing, this fits the bill.4 -
So... I learnt a couple things today about C++ language which I didn’t know before...
1. float var = 5.9;
std::cout << ( var == 5.9 );
// shows 0 (false) coz of float and double thing... apparently, 5.9 isn’t automatically converted to float when compared to one 🤔
2. arr[ i ] == i[ arr ]
Well... I guess I now like my college 1% more from the previous % whatever that was 😊☺️32 -
I've a recently joined developer hired from campus sitting next to me.
She: I'm printing an array in Java but it's not working. Could you please check what's wrong?
I see this piece of code:
printArray(arr);
Me: Where is the printArray method?
She: (With a puzzled look) Oh, do I have to declare that?
Me: 😶😶😶 (lowered my head, walked away slowly praying for the company)9 -
Previous developers read entire result of a SELECT into array of arrays.
Then used that later on in the following fashion.
print "name: " + result[row][17]
print "address: " + result[row][23]
...
without any description whatsoever what the numbers mean.
And it's here "result" and "row", in the actual code it was "arr" and "ii".
And these arrays were "public static" used everywhere, but initialized only at few places, so if you went onto wrong screen or if there was a phone call that kicked the app out it crashed.
But real fun began when people started changing queries and altering tables...
I seriously thought I was being pranked as a new hire.9 -
It was a basic java lesson. We had four values that we stored in a array. We had to make some calculations with the values. Then we had to sort those four values. That's the solution our teacher proposed:
if (arr[0] > arr[1]) {
int temp = arr[0];
arr[0] = arr[1];
arr[1] = temp;
}
if (arr[1] > arr[2]) {
int temp = arr[1];
arr[1] = arr[2];
arr[2] = temp;
}
if (arr[2] > arr[3]) {
int temp = arr[2];
arr[2] = arr[3];
arr[3] = temp;
}
if (arr[0] > arr[1]) {
int temp = arr[0];
arr[0] = arr[1];
arr[1] = temp;
}
if (arr[1] > arr[2]) {
int temp = arr[1];
arr[1] = arr[2];
arr[2] = temp;
}
if (arr[0] > arr[1]) {
int temp = arr[0];
arr[0] = arr[1];
arr[1] = temp;
}7 -
Did you know that
console.table(arr);
will let you print whole JavaScript arrays in table form in console?10 -
What the fuck Apple, I thought selling a monitor stand separately at £999 was bad, £360 for putting fucking wheels on your Mac Pro, fuck me.
I had no fucking clue how to tag this, hence why it’s got 3 categories to it11 -
So we got our first household Alexa yesterday and my brothers have been asking it silly things, like:
Brother: Alexa sing baby shark:
Alexa: <sings baby shark>
B: Alexa sing mummy shark
A: <sings mummy shark>
B: Alexa sing daddy shark
A: <sings daddy shark>
B: Alexa sing grandma shark
A: "I think that's enough, even I have my limits"
Fucking Brilliant!!!4 -
Just had Windows pop up with a notification saying “We’ve turned on storage sense for you”. Love it how the default is “When Windows Decides”. Was that why countless users lost their data back in October 2018???
I think I’ll turn that off permanently 😂😂😂4 -
My tech lead keeps assigning me incident tickets in the company's worst legacy app because I solved some bug in it a while ago. I'm the only one who gets assigned these.
If this keeps going, I know for sure that I will be regarded as the designated developer for this application. Then I will be truly fucked.5 -
This compiles and works in C++:
int arr[10];
5[arr] = 42;
cout << 5[arr] << endl;
I know why it works technically. Its just pointer math. But I have to ask "Why?" Of all that is unholy, WHY?!11 -
Today I experienced cruelty of C and mercy of Sublime and SublimeLinter.
So yesterday I was programming late at night for my uni homework in C. So I had this struct:
typedef struct {
int borrowed;
int user_id;
int book_id;
unsigned long long date;
} entry;
and I created an array of this entry like this:
entry *arr = (entry*) malloc (sizeof(arr) * n);
and my program compiled. But at the output, there was something strange...
There were some weird hexadecimal characters at the beginning but then there was normal output. So late at night, I thought that something is wrong with printf statement and I went googling... and after 2 hours I didn't found anything. In this 2 hours, I also tried to change scanf statement if maybe I was reading the wrong way. But nothing worked. But then I tried to type input in the console (before I was reading from a file and saving output in a file). And it outputted right answer!!! AT THAT POINT I WAS DONE!!! I SAID FUCK THIS SHIT I AM GOING TO SLEEP.
So this morning I continued to work on homework and tried on my other computer with other distro to see if there is the same problem. And it was..
So then I noticed that my sublime lint has some interesting warning in this line
entry *arr = (entry*) malloc (sizeof(arr) * n);
Before I thought that is just some random indentation or something but then I saw a message: Size of pointer 'arr' is used instead of its data.
AND IT STRUCT ME LIKE LIGHTNING.
I just changed this line to this:
entry *arr = (entry*) malloc (sizeof(entry) * n);
And It all worked fine. At that moment I was so happy and so angry at myself.
Lesson learned for next time: Don't program late at night especially in C and check SublimeLInter messages.7 -
You think arrays starting with 1 are annoying?
HA!
How about time in a day starting with 12?
12:00, 12:45, 1:00, 1:45,...,11:45, 12:00, 12:45, 1:00, 1:45, ..., 11:45, 12:00, 12:45, 1:00 again
What if arrays started with 12? I bet Americans would love that!
arr[12]=fuck
arr[1]=this
arr[2]=shit
arr[3]=!!!14 -
I’ve only just been able to catch up with the UK news and the government missed ~16,000 cases of COVID-19 over a 7 day period due to a “technical glitch”.
The “technical glitch” was the government using an excel spreadsheet that reached the data limit.
Who the fuck thought that was a good idea long term, that’s why databases exist!!!!9 -
Fucking shit fuck.
I got off work, ranted with the wk rant... and forgot about it.
Typed out a -1000 character ish rant about the fuck of a dependency manglement situation I got myself into in node today. Pure artwork I swear.
>you can’t be posting rants within 2h of the last one.
>okay, that’s fine, I’ll park it and wait.
Switch app out to messenger to chat to my booty.
Switch back, check it’s still there... fucking empty new rant screen 😞
I know iOS have updated their app backgrounding... maybe that was the problem.
Or it’s just user error. FUCK!
I ain’t typing it again. That masterpiece of a rant is gone to the ether.10 -
Fuck Udemy adverty. "You should learn this online machine learning course, it's taught by an 'expert' instructor, you'll learn algorithms in pythaan and 'arr, make robust models, add value to your business." Fuck off with your buzzword mumbo jumbo and just be straight with people, don't treat them like idiots.11
-
I’ve had enough of shitty ISPs. Time to shame them 😈
Here in the UK, we have a company called TalkTalk who treat their customers as whores by not giving a shit and taking all their money.
I have had an ongoing issue for about 9 months now where our internet is more unstable, but also slower. We pay for 72Mbps at which we used to get, but now our internet tops out at 30Mbps if we are lucky. It can be 20Mbps one minute and 7Mbps the next, and I’ve had it drop below 1Mbps for no goddam reason.
I’ve spoken (well, argued) argued to their so called customer service department over phone and live chat explaining the situation and all they’ve done is said “restart your router”, or “we’ll send out a new router, it’s probably a WiFi issue”, or some bullshit like “I’ve ‘changed’ something on my end”. On one conversation with a so called technician, I had to explain how networking actually worded, and and even called a 7Mbps acceptable when we were paying for 10x that!!!!!
The thing that makes it worse is they actually have systems that detect any issues with customers internet lines, but they only alert the customer to the issue through an online portal, telling the customer to call up and get it dealt with rather than passing the info to a tech department and having issues fixed without the customer knowing unless it’s absolutely necessary.
So 9 months in and I still have a fluctuating, unstable internet connection which is slow and overpriced with no tunnel in sight. GIVE ME BACK MY GODDAMN MONEY YOU FUCKING THIEVING BASTARDS.3 -
The best thing about perl is it doesn't care about errors and really tries to do what you ask, without throwing exceptions.
The worst? It does exactly what you ask, no matter how insane.
Typed $arri[ $0 ] instead of $arr[0] inside a function that detected what changes were needed in dns zones. $0 is script name and path, strings are converted to integers as needed and there's a little thing called vivification.
You see where this train wreck is going.
Also my dog died today.
Got to love Mondays :/11 -
Management has been promising we'd leave .NET framework for 2 years now. Never fucking happens. A new ASP.NET project was just started last week and yup, OF COURSE, its .NET Framework 4.8.
I'd even be happy with one of the earlier .NET Core versions at this point for fucks sake. I have no clue why tech leads are so happy to create a brand new project on a deprecated framework version.
And yes, I have checked thoroughly. Our whole infrastructure works with .NET Core onward. People are just too lazy to learn new stuff.
Stuff like switching to .NET 6, actually doing unit testing, improving our CI/CD pipeline, refactoring problematic codebases, etc. -> all this stuff is the kind of things they promise me I can work on later whenever I'm so bogged down with work that I'm looking for a light at the end of the tunnel. All empty promises.
Ideally we should be on .NET 6 since its LTS and just stay on the LTS versions as the year goes on.8 -
What developer/team thought that this would be a fucking brilliant option to put in this in a game?
Btw there is no VSync option, just “Limit to 30 FPS”11 -
When creating auto incrementing integer keys on a MySQL database, please mark then as unsigned, you don’t need a negative primary key 😞😞😞4
-
I'm supposed to be the introverted, non-people person! But the client meetings I'm in for my college senior project go off the rails into awkward mumbling unless I step in and take the tiniest bit of effort in driving a meeting.
Am I doomed folks to become a BA or other person dealing with clients all day, pls end me now2 -
2nd rant this week on PHP PDO
PHP 💩:
PHP message: SQLSTATE[HY000]: General error: 2014 Cannot execute queries while other unbuffered queries are active. Consider using PDOStatement::fetchAll(). Alternatively, if your code is only ever going to run against mysql, you may enable query buffering by setting the PDO::MYSQL_ATTR_USE_BUFFERED_QUERY attribute
Me 🤬:
THATS WHAT I’M FUCKING USING. STOP FUCKING COMPLAINING. YOU DIDN’T LIKE THE FUCKING COLONS FOR YOUR FUCKING PARAMATETRS, NOW YOU HATE YOUR OWN FUCKING FETCHALL() METHOD YOU PEICE OF SHIT, AND YOU CAN’T HAVE ANY OTHER ACTIVE QUERIES AS IT’S THE FIRST FUCKING QUERY. ARRGHHH!!!!!
What the actual fuck is wrong with PDO. It bitches about everything 😡.8 -
Only just noticed that CSS has variables - when the fuck did this happen and where the hell was I when it did!!!!6
-
No wonder why Google Chrome takes up alot of RAM when running, I've just seen chromiums dev requirements:
System requirements
- A 64-bit Intel machine with at least 8GB of RAM. More than 16GB is highly recommended.
- At least 100GB of free disk space on an NTFS-formatted hard drive. FAT32 will not work, as some of the Git packfiles are larger than 4GB.
- An appropriate version of Visual Studio, as described below.
Windows 7 or newer.8 -
So our city’s bus service provider has decided to update the software for their “iPoints”, I think they haven’t thought this rollout through.7
-
Over a year ago I posted a screenshot of chrome vs edge, and chrome had 92 processes consuming 2.1GB RAM vs edges 19 processes consuming 3.2GB RAM
Just seen this... WTF google, how can you need soooo much ram, I thought you had fixed your high memory issues!!!22 -
Just been told by someone that they think that Megabits (Mb) is the same as Megabytes (MB)... FUCK SAKE🤬🤬🤬😞😞😞
I want to put my head through my keyboard, through my desk, and through the 3 floors below me. I’m so god damn triggered right now6 -
What dumb twat decided to commit a change with the message “FUCK”. And it not just one, it’s 10 or more in a fucking row!!!!!
Thanks, that’s really gonna help me understand what was changed 😡5 -
Why does Windows have to be so fucking useless when running docker. Everytime i need to make a change to the NGINX container, I have to restart the docker daemon, rather than rebuild and restart the container.
I would be doing this on Ubuntu, if I could install it that is.8 -
Is anyone else dealing with AD(H)D in university or while working as an software engineer. I’ve been diagnosed 3 years ago and I’m still trying to find a way to keep my focus. I start a new project every week without finishing it. Medication helps but not for 100%
Does anyone else experience the same or do you have any tips?8 -
Why does the css border-spacing have to be different in how it takes 2 values.
If you look at margin and padding properties when passing in 2 values, you pass in:
margin: (top/bottom)px (left/right)px;
However, when using border spacing:
border-spacing: (left/right)px (top/bottom)px;
I know I’m simplifying here, but it’s goddamn irritating how it’s takes in vertical and horizontal spacing the other way around. Who the hell thought that was a good idea4 -
Love it when you have a SQL query that takes 6.3 seconds (which includes processing time) to execute, and you managed to convert to a process that takes just 0.072 to complete1
-
1. I have Animal Crossing
2. My internet can drop to 0.8Mbps
3. I have Animal Crossing
4. My internet can drop out 10 times a day
5. I have animal crossing
6. My office pc is my gaming rig
7. I have... I think you get the gist1 -
In college doing A2’s, working solo. Building a companion app for Trivial Pursuit bet you know it edition. Laptop got hit by virus/malware, stopped me from copying files, lost 2/3’s of my project 3 days before submission.3
-
One of my commits today:
“Corrected a file path for view as it’s causing issues within the live environment”
What I actually wanted to put:
“Changed letter to uppercase in file path for view because live is being a fucking bitch about it”
Why do my Dev and Live environments have to differ 😞, I kept my cool though, which is a first for me 🙂4 -
Can we have a Moment of silence please
https://sebastiandedeyne.com/moment...
I only found this library a couple of months ago and instantly loved it as it made working with JS date so much easier.
Sorry for the pun btw, i couldn’t resist 😂7 -
Just finished a defect fix, and turns out there's another unrelated but harder bug in the codebase. We are in the last few days of the release.
I told my tech leads that it was an unrelated problem and showed them in detail. I told them I was starting work on it now, but there should probably be a new defect entered for it.
They actually said for me to piggyback the old defect and let this go under the radar. Actually laughed it off like it was no big deal. Like WTF! I don't think its very unreasonable for devs to want separate defects for separate bugs. They're worried about analytics and shit, but I'm the one left holding the rug, looking like I spent a week on a trivial defect.5 -
Erm, instead of trying to guess my gender, just put “Dear <first name>” and leave it at that. It’s actually quite insulting13
-
Why does the Fat Free Frameworks (F3) $db->exec() method have its array count from 1 and not 0.
Instead of doing:
[$param1, $param2]
You have to do:
[ 1=> $param1, 2=> $param2]
WHO THE FUCK THOUGHT THAT WOULD BE A GREAT IDEA?
This is something that PHP PDO gets right, AND I FUCKING HATE PDO!!!4 -
I’ve just fired up Ubuntu for the first time in a couple of years on a laptop while doing some data recovery. I use windows and Mac in a daily basis.
I’m tempted to switch, I forgot how much I missed Ubuntu1 -
I'm at a bit of a loose end here, I'll do my best to explain and I hope it all makes sense.
I'm trying to find a way to wrap a C++ Console App in a C# Class so I can use the C++ App as a library within the C# app rather than triggering the C++ exe and providing a command to it.
The reason why I wish to do it this way is for maintainability, so I can make changes to the C++ app without affecting the C# App.
I've been looking at tutorials and stack overflow to see if's its possible, but for someone with learning difficulties, I'm struggling to find the right path to take as I'm seeing conflicting info.
Any help would be greatly appreciated, Thanks in advance5 -
in BASH you cannot reassign associative arrays (i.e. string:string maps) to other variables.
If you have created an array as variable "arr", doing
```
declare -A arr2
arr2=${arr}
```
will not give you var2 as a usable assoc array. It will kind of transform it into an indexed array
In fact working with assoc arrays in shell is a bitch.3 -
Can anyone suggest a good TS based stack to use with electron? I'm (hopefully) looking to make an open source app3
-
Do I have just a bad version of ecma script or is this some stupid shit in JS in general? I want each sub array to be separate entities, not that same one for all. I assume the fill in just putting the same list in all of them? I honestly don't care I guess, replacing a sublist is fine too. Rather than editing each element separately. Saves ram in long run.
let arr = Array(5).fill(Array(1,2))
console.log(arr)
arr[0][0] = 3
console.log(arr)
[[1,2],[1,2],[1,2],[1,2],[1,2]]
[[3,2],[3,2],[3,2],[3,2],[3,2]]
Congratulations, you are my dev duck today.19 -
Fucking hell... If I knew that PHPStorm could display the same file over 2 screens/windows, I’d have used it sooner. 😡
It is a godsend though 🙂7 -
I graduate college in December and I got my first fulltime job offer today! I've been working in my university's oit department for 3 semesters, lol most of rants are about that job. I guess a lot of my frustration stemmed from being capable but hitting a wall in the sort of things I worked on. I didnt feel like I was growing and had no avenues to express concerns/feedback towards the end. Plus the job was not one where they could give me a job after graduation, so I just felt unseen and discardable day to day.
But turns out this job worked out for me! There's an opening in a whole other division that does api development and data warehousing with Snowflake/Attacama and they want me specifically for it. If the benefits/pay ends up being decent, I'm leaning towards accepting it. -
Why THE FUCK would Array.fill(arr) use arr's REFERENCE?
I know it's the default behaviour on parameters but WHY WOULD YOU WANT THAT BEHAVIOUR IN THAT METHOD?3 -
The company I work at severely limits the days we can take off, like most requests I put in will be denied. Additionally, I don't get paid that well or even get paid for time off or holidays. Obligatory: the job I work at currently is co-op, I'm still in college.
Yesterday and today I was severely burnt out so I said I was sick when I wasn't really so I could get some (unpaid) time off. It's likely that the current release we're targeting at work will be in jeopardy because of this. I feel so guilty, should I be? I really needed this time, I doubt I could have continued much longer without this.
TLDR, please help me justify not giving a shitty job my unconditional 100% and being shitty back every once in a while2 -
Google's "Mobile Friendly Test Tool" is shit.
It does its automated test's during it's index and points out that there are various issues with the page and IT IS NOT mobile friendly.
So I go and test the page in the Mobile test tool to see what other error's it's reporting and it comes back and says "This page IS mobile friendly".
Like WTF Google, make up your fucking goddamn mind and stop pissing me about.rant google can go fuck itself google is a bitch search console fuck google overlord mobile development google1 -
Can this week be over now(), it's been an absolute nightmare in terms of development. I just want to sleep.1
-
Looking for a easy to learn and well developed ide for php and wordpress development. Atom phpstorm and zend arr on my list and money isn't really an issue.5
-
Had a debate with a colleague today over git commits, I had 24 outstanding and he had 15. We came up with a little game...
Who can create the most changes for a single commit. The winner is the one who can remember what each change was without looking at the changes.
Needlessly to say, we both lost 😂 -
So we are testing slack as our new messaging platform, it states that the platform has 10k searchable messages, does that mean that you can send unlimited messages with 10k searchable, or 10k messages total before you have to start paying?5
-
Question for the hiring managers out there: When reviewing applications for an open role, what specifically stands out to you about an applicant? (Assuming that the ATS gods don't just automatically filter the application out.)
Is it their achievements at previous companies? (Ex. Boosted ARR by 200% or decreased monthly churn by 30%)
Is it their career trajectory?
Is it their resume writing abilities?
Is it their education/certification credentials?
Is there some degree of "brand shopping" involved? For example, does seeing an average resume from a former Google employee with 2 YOE get you more excited than a well-written resume from a candidate with 7 YOE who worked at a lesser-known company?
I suppose much of this depends on the role and its needs.
Just given the market right now, I'm curious how hiring managers are making selections from their undoubtedly vast pool of candidates. I've heard that almost any job positing now is getting 500+ applicants within the hour, but with the caveat that 490 of those 500 applicants are completely unqualified (Like a Shift Manager at Chipotle who worked an IT help desk summer internship applying for a Senior Software Engineer role.)
Ultimately, what aspects of an applicant combined with their background and resume makes you say "Wow, this might be the one" while reviewing applications for a role?3 -
A project where there was no templating at all and every page was independent of each other, it was that bad I started from scratch.
-
does anyone else find it wild that there are hundreds or even thousands of products you've never heard of with over $1M+ ARR? this is just mind boggling to me4
-
There is nothing worse than trying to program while having RSI, it’s either you have your wrist support on and type slowly and hit multiple keys, or you have it off, type slowly and live with the pain3
-
So I was writing some C code, pretty simple code. I had to pass a matrix to a function. The matrix had been globally defined as arr[100][100]. But the actual size of the matrix was stored in 2 vars m, n. Now when defining the function if I do like this:
void fun(int a[][n])
The code doesn't work as expected but when defined as:
void fun(int a[][100])
Works perfectly.
I have no idea why this is happening, any insight will be very helpful.5 -
How come so many dev teams are working with blindfolds on?
We have two projects that communicate using endpoints. One of them throws a parsing error with some data. Cool, just give the calling project some debug references and attach a debugger right?
Apparently not. I haven't figured out why we can't do that, it seems like the project only works using nuget references so we never get any debug info for the other project.
Asked around how we usually solve issues like this. The answer: "idk the codegen always works, so we never solve issues like this".
What.
It "always works". Except now it doesn't. And you've never tried debugging it? Instead just working with blindfolds on trying random shit until it does?
This is far from the first time I've heard this on a team. That and "we don't need error codes, if something goes wrong we have to fix it either way". I'm losing faith in the dev world... -
There is this local cloud service provider I used to like, since the promise was "it's from Africa" woi, they don't know shit. We tell then to open port 8888 ssh and 8899 since firewall is configured on their side, they close all ports then you can't ssh into the server. They take another 2 hours to fix that. Later on we change our ports and then tell them to change some ports, they open and close all ports (we discovered again because we were locked out again and had to d an nmap to see what was happening). Apparently the staff we we're talking to didn't know much about configuring servers only the senior management knew (I think to cut down costs), then we tell then to terminate services but they decide to bill us for another month (bullying) and gave us a warning letter from their lawyer for not paying for that month and since we are a small startup, we can't afford a court case which will drain us cash so we had to pay for shitty service and some month arr angry
-
So apparently in MySQL, 0 doesn’t always equal 0, sometimes it can be 1, or 2, or 3...
what the actual fuck?!?!5 -
Why is PHP PDO such a b*tch to work with?
One minute it says it’ll accept one query via one method for one insert but then deny the next query you give it, all because of “:” within bindParam() -
Joined a new project.
The core of the application has references to pretty much everything in the entire codebase, both api and database layer.
On top of that it uses extension methods instead of mappers or normal methods on classes in order to "keep classes short", so many methods are unnecessarily hidden from view.
Tried to fix it, got told to revert back to the old version because "it might be wrong, but at least it's wrong everwhere". Guess I'm making bolognese for dinner.1 -
Trying to find a function I swear I had created to retrieve a set of counts from a database. Couldn’t find it. What I could find is the comment I had written with the function being completely overwritten with something entirely different.
I’m a complete fucking idiot and thank fuck for version control. -
Sat on a bus heading home next to some guy who clearly hasn’t got knowledge of how to use a pair of fucking headphones and it sat playing a fucking game with the volume on loud, and all I can hear is the same fucking “whoosh, ping” noise fucking over and over again or he’s watching those shitty videos on Facebook
IT’S DOING MY FUCKING HEAD IN!!!!!! GET A PAIR FUCKING HEADPHONES OR MUTE YOUR FUCKING PHONE!!!!! NO-ONE WANTS TO HEAR THAT SHIT!!!!!2 -
Sometimes, just sometimes, I need to stop programming until nearly 10pm. It cannot be healthy for the body.
-
So as part of my news years resolution, I thought id build an app and I thought I would build it in vue.js and native script with a shared codebase, I've started with a fresh project and already I have an error, one that I've not caused, this is in router.js. see if you can spot it.
module.exports = (api, options) => {
require('@vue/cli-plugin-router/generator')(api, {
historyMode: options.routerHistoryMode
};
export default new Router(options);
}
When I've fixed the syntax error, I then get, the error:
/src/router.js: 'import' and 'export' may only appear at the top level (5:0)
This is when I run "npm run serve:ios"
if anyone has encountered this, please let me know how you fixed this.2 -
Why is the prettus repository package shit at handling mass inserts. The documentation says:
$this->repository->create(Input::all())
WTF does that even mean.3 -
How the fuck is this even possible Microsoft, apparently one line of code breaks web browser sandboxing on all chromium based browsers.
https://slashgear.com/windows-10-19...3 -
Why did the pirate learn boolean logic?
So he could "arr" and "arr" without getting confused between true and false :)2 -
Can any one recommend a good Go framework for APIs with a MongoDB ORM?
Was using Laravel 6/Lumen but it’s API ability is starting to piss me off with its CSRF crap, JWT/Passport implementation and a it’s lack of MongoDB support.
I don’t need any templating engines at all, its all handled by a SPA and Mobile Apps6 -
So I'm working on this little personal project (also as a way to keep my "skills" sharpened for the coming semester), that first started as a workaround to do this other thing, and I wanted to develop it and make it a full fledged thing, with a GUI (or something that resembles it, I don't know how to make GUIs yet, and IDK why is it a 3rd grade thing) and all instead of existing just in the IDE's terminal. When it was on the workaround stage it was just this ugly monster, with only 2 things one could do, but it worked. Now I'm going for a more polished thing and it's starting to break on me, and in places I didn't expect it to LoL
It's like I'm on a boat and I'm getting leaks from everywhere. Arr gotta get me a bucket and save me boat from sinking -
Oh yeah ... Java is cool in an utterly sick way even that i can't seem to find a non-retarded built-in stack data structure
Call me a racist, but java.util.Stack has a removeIf() method in case you want to remove odd numbers:
import java.util.Stack;
public class App {
public static void main(String[] args) {
int arr[] = { 2, 4, 7, 11, 13, 16, 19 };
Stack<Integer> s = new Stack<Integer>();
for (int i = 0; i < arr.length; i++) {
s.push(arr[i]);
}
s.removeIf((n) -> (n % 2 == 1));
System.out.println(s); // [2, 4, 16]
}
}
Stop using java.util.Stack they said, a legacy class they said, instead i should use java.util.ArrayDeque, but frankly i can still keep up being racist (in a reversed manner):
import java.util.ArrayDeque;
import java.util.Deque;
public class App {
public static void main(String[] args) {
int arr[] = { 2, 4, 7, 11, 13, 16, 19 };
Deque<Integer> s = new ArrayDeque<Integer>();
for (int i = 0; i < arr.length; i++) {
s.push(arr[i]);
}
s.removeIf((n) -> (n % 2 == 1));
System.out.println(s); // [16, 4, 2]
}
}
The fact that you can iterate through java.util.Stack is amazing, but the ability to insert element in a specified index:
import java.util.Stack;
public class App {
public static void main(String[] args) {
int arr[] = { 2, 4, 7, 11, 13, 16, 19 };
Stack<Integer> s = new Stack<Integer>();
for (int i = 0; i < arr.length; i++) {
s.push(arr[i]);
}
s.add(2, 218);
System.out.println(s); // [2, 4, 218, 7, 11, 13, 16, 19]
}
}
That's what happens when you inherit java.util.Vector, which is only done a BRAIN OVEN person, a very brain oven even that it will revert to retarded
If you thought about using this type of bullshit in Java get yourself prepared to beat the disk for hours when you accidentally call java.util.Stack<T>.add(int index, T element) instead of java.util.Stack<T>.push(T element), you will probably end up breaking the disk or your hand, but not solving the issue
WHY THE F*** CAN'T WE HAVE A WORKING NORMAL STACK ?5 -
#include <iostream>
using namespace std;
int main() {
int t;
cin>>t;
while(t--){
int n;
cin>>n;
int arr[n];
int count=0;
for(int i=0;i<n;i++){
cin>>arr[i];
count^=arr[i];
}
cout<<count<<endl;
}
return 0;
}
In the above program,
how does this code snippet work?
count^=arr[i];8 -
Just found out it’s pronounced Microsoft Arr-sure like “sure” in pressure and not Microsoft Arr-zu-ray.4
-
So working from home due to UK Gov lockdown. iTunes on, and the perfect power anthem comes on... Productivity up 110%
-
Why we don't write dereference operator while priting string(format specifiers is %s).
For example: char arr[5]={"char"};
printf("%s",arr);//print char.
If we write like printf("%s",*arr);//compile time error.1 -
(tldr: are foriegn keys good/bad? Can you give a simple example of a situation where foriegn keys were the only and/or best solution?)
i have been recently trying to make some apps and their databases , so i decided to give a deeper look to sql and its queries.
I am a little confused and wanted to know more about foreign keys , joins and this particular db designing technique i use.
Can anyone explain me about them in a simpler way?
Firstly i wanted to show you this not much unheard tecnique of making relations that i find very useful( i guess its called toxi technique) :
In this , we use an extra table for joining 2 tables . For eg, if we have a table of questions and we have a table of tags then we should also have a table of relation called relation which will be mapping the the tags with questions through their primary IDs this way we can search all the questions by using tag name and we can also show multiple tags for a question just like stackoverflow does.
Now am not sure which could be a possibile situation when i need a foriegn key. In this particular example, both questions and tags are joined via what i say as "soft link" and this makes it very scalable and both easy to add both questions and new tags.
From what i learned about foriegn keys, it marks a mandatory one directional relation between 2 tables (or as i say "hard a to b" link)
Firstly i don't understand how i could use foriegn key to map multiple tags with a question. Does that mean it will always going to make a 1to1 relationship between 2 tables( i have yet to understand what 11 1mant or many many relations arr, not sure if my terminology is correct)
Secondly it poses super difficulty and differences in logics for adding either a tag or question, don't you think?
Like one table (say question) is having a foreign key of tags ID then the the questions table is completely independent of tag entries.
Its insertion/updation/deletion/creation of entries doesn't affect the tags table. but for tag table we cannot modify a particular tag or delete a tag without making without causing harm to its associated question entries.
if we have to delete a particular tag then we have to delete all its associated questions with that this means this is rather a bad thing to use for making tables isn't it?
I m just so confused regarding foriegn keys , joins and this toxi approach. Maybe my example of stack overflow tag/questions is wrong wrt to foreign key. But then i would like to know an example where it is useful5