Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
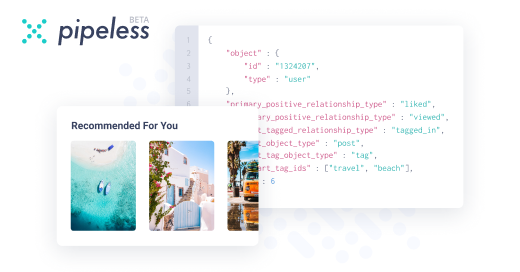
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "int-1"
-
Manager: Write a function to get tomorrow's date.
Kids:
int getTomorrowsDate() {
return getCurrentDate() + 1;
}
Legends:
int getTomorrowsDate() {
sleep(1000*60*60*24);
return getCurrentDate();
}14 -
Interview with a candidate. He calls himself "C++ expert" on his resume. I think: "oh, great, I love C++ too, we will have an interesting conversation!"
Me: let's start with an easy one, what is 'nullptr'?
Him: (...some undecipherable sequence of words that didn't make any sense...)
In my mind: mh, probably I didn't understand right. Let's try again with something simple and more generic
Me: can you tell me about memory management in C++?
Him: you create objects on the stack with the 'new' keyword and they get automatically released when no other object references them
In my mind: wtf is this guy talking about? Is he confusing C++ with Java? Does he really know C++? Let's make him write some code, just to be sure
Me: can you write a program that prints numbers from 1 to 10?
Ten minutes and twenty mistakes later...
Me: okay, so what is this <int> here in angle brackets? What is a template?
Him: no idea
Me: you wrote 'cout', why sometimes do I see 'std::cout' instead? What is 'std'?
Answer: no idea, never heard of 'std'
I think: on his resume he also said he is a Java expert. Let's see if he knows the difference between the two. He *must* have noticed that one is byte-compiled and the other one is compiled to native code! Otherwise, how does he run his code? He must answer this question correctly:
Me: what is the difference between Java and C++? One has a Virtual Machine, what about the other?
Him: Java has the Java Virtual Machine
Me: yes, and C++?
Him: I guess C++ has a virtual machine too. The C++ Virtual Machine
Me (exhausted): okay, I don't have any other questions, we will let you know
And this is the story of how I got scared of interviews29 -
Some ideas for variable names. Thank me later :))
1. bool sheet;
2. int entionally;
3. char mander;
4. double penetration;
5. string cheese;
6. long schlong;23 -
Swift, oh my god, why do you have to be like this?
I'm looking to write a simple for loop like this one in java
for(int i = 5; i > 0; i--) {
// do shit
}
Thats it, simple, go from 5 to 1 (inclusive), I saw that to iterate over a range in a for loop (increasing ordeR) I can do this
for i in 0...5 {
// do shit.
}
So I thought maybe I could do this to go in reverse (which seems logical when you think about it doesn't it?)
for i in 5..<0 {
// do shit
}
But no, this compiles FINE (THIS IS THE FUCKING KICKER IT COMPILES), alright, when you the code runs you get a fucking exception that crashes the mother fucking application, and you know what the problem is?? This dogshit, shitStain of a language doesn't like it when integer that the for loop starts with is larger than the integer that the for loop ends with MOTHERFUCKER ATLEAST TELL ME THAT AT COMPILE TIME AS A MOTHERFUCKING WARNING YOU PIECE OF SHIT!!
Alright *deep breathing*, now we can't just be stuck on this raging, we're developers need to move forward, so I google this, "Swift for loop in reverse" fair enough I get a straight forward answer that tells me to use the `stride` functionality. The relevant code for it
for i in stride(from:5 to:1 by:-1) {
// do shit
}
Wow looks fine and simple right?? (looks like god damn any other language if you ask me, no innovations here piece of shit apple!) WRONG BITCHES !!! In the latest version of Swift THE FUCKING DEVELOPERS DECIDED TO REMOVE STRIDE ALTOGETHER, WITHOUT ADDING IN A GOOD REPLACEMENT FOR THAT SHIT!
Alright NOW IM FUCKING MAD, I got rage on stackoverflow chat, a guy who's been working on ios for quite a while comes up n says and I quote
"I can sort of figure it out, but besides that, iterating in reverse is uncommon enough that it probably hasn't crossed anyone's mind."
Now hope you guys understand my frustration, and send me cookies to calm me down.
Thank you for listening to me !27 -
While reading through the Elasticsearch (Java search engine) source code a while ago I found this gem:
return i == -1? -1: i;
I think someone should stop drinking while coding.
Some other nice lines:
int i = 0;
return j + 1000 * i;
Are these guys high?11 -
public boolean even( int num ) {
if ( num < 0 )
num = -1 * num;
while ( num > 1 )
num = num - 2;
if ( num == 0 )
return true;
else
return false;
}19 -
I recently got my first job and I already saw code that I won't ever forget
for(int i=0; i < 2; ++i) {
switch(i) {
case 0:
~~~
break;
case 1:
~~~
break;
case 2:
~~~
break;
default:
~~~
break;
}
}23 -
#Programming alone
print "Hello World!"
#Programming while someone watches
global _start
section .data
msg db "Hello, World!", 10
len equ $ - msg
section .text
_start:
mov rax, 4
mov rbx, 1
mov rcx, msg
mov rdx, len
int 0x80
mov rax, 1
mov rbx, 0
int 0x805 -
It was a basic java lesson. We had four values that we stored in a array. We had to make some calculations with the values. Then we had to sort those four values. That's the solution our teacher proposed:
if (arr[0] > arr[1]) {
int temp = arr[0];
arr[0] = arr[1];
arr[1] = temp;
}
if (arr[1] > arr[2]) {
int temp = arr[1];
arr[1] = arr[2];
arr[2] = temp;
}
if (arr[2] > arr[3]) {
int temp = arr[2];
arr[2] = arr[3];
arr[3] = temp;
}
if (arr[0] > arr[1]) {
int temp = arr[0];
arr[0] = arr[1];
arr[1] = temp;
}
if (arr[1] > arr[2]) {
int temp = arr[1];
arr[1] = arr[2];
arr[2] = temp;
}
if (arr[0] > arr[1]) {
int temp = arr[0];
arr[0] = arr[1];
arr[1] = temp;
}7 -
Always remember: Every time you write a for loop like that: for (int i = 1; i <= n; i++) a cat dies!5
-
Most satisfying bug I've fixed?
Fixed a n+1 issue with a web service retrieving price information. I initially wrote the service, but it was taken over by a couple of 'world class' monday-morning-quarterbacks.
The "Worst code I've ever seen" ... "I can't believe this crap compiles" types that never met anyone else's code that was any good.
After a few months (yes months) and heavy refactoring, the service still returned price information for a product. Pass the service a list of product numbers, service returns the price, availability, etc, that was it.
After a very proud and boisterous deployment, over the next couple of days the service seemed to get slower and slower. DBAs started to complain that the service was causing unusually high wait times, locks, and CPU spikes causing problems for other applications. The usual finger pointing began which ended up with "If PaperTrail had written the service 'correctly' the first time, we wouldn't be in this mess."
Only mattered that I initially wrote the service and no one seemed to care about the two geniuses that took months changing the code.
The dev manager was able to justify a complete re-write of the service using 'proper development methodologies' including budgeting devs, DBAs, server resources, etc..etc. with a projected year+ completion date.
My 'BS Meter' goes off, so I open up the code, maybe 5 minutes...tada...found it. The corresponding stored procedure accepts a list of product numbers and a price type (1=Retail, 2=Dealer, and so on). If you pass 0, the stored procedure returns all the prices.
Code basically looked like this..
public List<Prices> GetPrices(List<Product> products, int priceTypeId)
{
foreach (var item in products)
{
List<int> productIdsParameter = new List<int>();
productIdsParameter.Add(item.ProductID);
List<Price> prices = dataProvider.GetPrices(productIdsParameter, 0);
foreach (var price in prices)
{
if (price.PriceTypeID == priceTypeId)
{
prices = dataProvider.GetPrices(productIdsParameter, price.PriceTypeID);
return prices;
}
* Omitting the other 'WTF?' code to handle the zero price type
}
}
}
I removed the double stored procedure call, updated the method signature to only accept the list of product numbers (which it was before the 'major refactor'), deployed the service to dev (the issue was reproducible in our dev environment) and had the DBA monitor.
The two devs and the manager are grumbling and mocking the changes (they never looked, they assumed I wrote some threading monstrosity) then the DBA walks up..
DBA: "We're good. You hit the database pretty hard and the CPU never moved. Execution plans, locks, all good to go."
<dba starts to walk away>
DevMgr: "No fucking way! Putting that code in a thread wouldn't have fix it"
Me: "Um, I didn't use threads"
Dev1: "You had to. There was no way you made that code run faster without threads"
Dev2: "It runs fine in dev, but there is no way that level of threading will work in production with thousands of requests. I've got unit tests that prove our design is perfect."
Me: "I looked at what the code was doing and removed what it shouldn't be doing. That's it."
DBA: "If the database is happy with the changes, I'm happy. Good job. Get that service deployed tomorrow and lets move on"
Me: "You'll remove the recommendation for a complete re-write of the service?"
DevMgr: "Hell no! The re-write moves forward. This, whatever you did, changes nothing."
DBA: "Hell yes it does!! I've got too much on my plate already to play babysitter with you assholes. I'm done and no one on my team will waste any more time on this. Am I clear?"
Seeing the dev manager face turn red and the other two devs look completely dumbfounded was the most satisfying bug I've fixed.5 -
non-dev (but still in IT) friend: *sends meme* "I don't get it!"
me:
int ThingsToBuyFromGrocery()
{
int milk = 1;
if(store.HasEggs())
{
milk = 6;
}
return milk;
}
friend: "Woah! You're so smart!"5 -
Windows 10 source code apparently leaked!
The main.c file is short tho and unchanged since 95(it's just run in parallel) so I'mma just paste it here:
void WinMain(int nCmdArgs){
static int userDisgust = 5; //he/she booted up Win10 so 5 to begin with
Time time = Time.time;
while(!crashed){
Time time2 = Time.time;
if(time + 3600 < time2){
ApplyUpdate();
sleep(500000); //users can stretch, have a tea and overall be healthier, happier and better part of society
if(restartNecessary){
Restart();
}
Restart(); // just in case
}
SendUserData();
SendMoreUserData();
for(int i = 1; i < 99+1; i++){
RunUserApps();
UninstallUnnecessaryApps(); // keeps blacklist of apps that John and I don't like
AnnoyUser(); // added a function that slows the computer down significantly and reduces lifespan of the hardware so it's not just a short term gain
if(shouldRepeat){
//break; -- this caused some issue in one special case and we don't know why so we'll just omit it and add a fancy button elsewhere
}
userDisgust++;
}
if(userDisgust >= 2147483647) { //mission finished
Crash();
crashed = true;
}
}
String error = Windows.GetFullErrorMessage();
error.cat(Windows.GetRegistersDump());
error.cat(Windows.GetErrorCode());
error.cat(Windows.GetStackTrace());
error.erase(); // so that it doesn't occupy any space and allows the error to be resolved more quickly, release major optimization Update
BSOD(_T("We're sorry :/"));
}6 -
I was checking an Arduino sketch I wrote in a hurry 2 years ago. I want to cry for the number of shit I've found there 😓
Picture + I had put as int variables that should have been boolean. I needed them for 0 and 1. As well as with no comments and no explicative names.13 -
Let's see the coder in you.
If I give input: 1 output: 2
If I give input: 2 output:1
Only these two test cases needed.
You should not use control structures such as if,else,for,while,switch etc. (The answer is simple) (Don't cheat)
int number;
cin>>number; //get number
cout<<??????; //Your code53 -
*** don't use compiler ***
Question in class today:
int n = 0;
for (int i = 1; i < 10; i++) {
n = n++;
System.out.println(n);
}
what will be printed?50 -
section .text
global _start
section .data
msg db 'Hello, world!',0xa
len equ $ - msg
section .text
_start:
mov edx,len
mov ecx,msg
mov ebx,1
mov eax,4
int 0x80
mov ebx,0
mov eax,1
int 0x802 -
Hoozay! I'm now starting to become an adult! (or atleast, that's what they expect of me)
myAge:
.long 19
main:
push rbp
mov rbp, rsp
mov eax, DWORD PTR myAge[rip]
add eax, 1
mov DWORD PTR myAge[rip], eax
mov eax, DWORD PTR myAge[rip]
mov esi, eax
mov edi, OFFSET FLAT:_ZSt4cout
call std::basic_ostream<char, std::char_traits<char> >::operator<<(int)
mov eax, 0
pop rbp
ret
__static_initialization_and_destruction_0(int, int):
push rbp
mov rbp, rsp
sub rsp, 16
mov DWORD PTR [rbp-4], edi
mov DWORD PTR [rbp-8], esi
cmp DWORD PTR [rbp-4], 1
jne .L5
cmp DWORD PTR [rbp-8], 65535
jne .L5
mov edi, OFFSET FLAT:_ZStL8__ioinit
call std::ios_base::Init::Init() [complete object constructor]
mov edx, OFFSET FLAT:__dso_handle
mov esi, OFFSET FLAT:_ZStL8__ioinit
mov edi, OFFSET FLAT:_ZNSt8ios_base4InitD1Ev
call __cxa_atexit
.L5:
nop
leave
ret
_GLOBAL__sub_I_myAge:
push rbp
mov rbp, rsp
mov esi, 65535
mov edi, 1
call __static_initialization_and_destruction_0(int, int)
pop rbp
ret12 -
Anyone else having connection problems after the recent Windows 10 update? We've been slammed at work with them. If you do, here's an easy fix:
0. Go to Command Prompt (Run as Administrator)
1. First command:
netsh int ip reset resetlog.txt
(One of them will probably fail, that's fine.)
2. Second command:
netsh winsock reset
3. Restart the computer.13 -
section .text
global _start
_start:
mov edx,len
mov ecx,msg
mov ebx,1
mov eax,4
int 0x80
mov eax,1
int 0x80
section .data
msg db 'Yo Mama So Fat',0xa
len equ $ - msg7 -
Since I'm still alive and the future parts of my life is a mystery , I say:
#include <limits.h>
int main(){
int worst=INT_MIN;
int best=INT_MAX;
while(1){
//keep coding
if(dead) break;
}
}2 -
PHP arrays.
The built-in array is also an hashmap. Actually, it's always a hashmap, but you can append to it without specifying indexes and PHP will use consecutive integers. Its performance characteristics? Who knows. Oh, and only strings, ints and null are valid keys.
What's the iteration order for arrays if you use them as hashmaps (string keys)? Well, they have their internal order. So it's actually an ordered hashmap that's being called an array. And you can produce an array which has only integer keys starting with 0, but with non-sequential internal (iteration) order.
This array weirdness has some non-trivial implications. `json_encode` (serializes argument to JSON) assumes an array corresponds to a JSON array if its keys are consecutive integers in increasing order starting with 0, otherwise the array becomes a JSON object. `array_filter` (filters arrays/hashmaps using callback predicate) preserves keys, so it will punch holes in the int key sequence if non-last items are removed, thus turning arrays into hashmaps and changing your JSON structure if you forget to discard keys before serialization.
You may wonder how JSON deserialization works, then? There's a special class for deserialized JSON objects, `stdClass`. It's basically a hashmap too, but it's an object, not an array, and all functions that would normally accept arrays won't work with it. So basically its only use is JSON (de)serialization. You can even cast arrays to objects, producing `stdClass`.
Bonus PHP trivia:
Many functions return nonsensical values. `preg_match`, the regex matching function, returns 1 for success, 0 for no matches and false for malformed regular expression. PHP supports exceptions, so it could just throw one on errors. It would even make more sense to return true, false and null for these three cases. But no, 1, 0 and false. And actual matches are returned by output arg.
`array_walk_recursive`, a function supposed to recursively apply callback to each element of an array. That's what docs say. It actually applies it to leafs only. It will also silently accept object instead of array and "walk" it, but without recursing into deeper objects.
Runtime type enforcing is supported for function arguments and returned values. You can use scalar types, classes, array, null and a few special keywords. There's also a `mixed` keyword, which is used in docs and means "anything". It's syntactically valid, the parser will accept it, but it matches no values in runtime. Calling such function will always cause a runtime error.
Strings can be indexed with negative integers. Arrays can't.
ReflectionClass::newInstanceWithoutConstructor: "Creates a new class instance without invoking the constructor". This one needs no commentary.
`array_map` is pretty self-explanatory if you call it with a callback and an array. Or if you provide more arrays of equal length via varargs, callback will be called with more arguments, one from each array. Makes sense so far. Now, you can also call `array_map` with null instead of callback. In that case it treats provided arrays as rows of a matrix and returns that matrix, transposed.5 -
I am using this SDK and I came across a property "Orientation" of type int.
Why int? Is it an enum or something? Let's have a look into the online documentation...
"Gets or sets the orientation."
😣
Yeah, thanks. Very useful.
It's again that kind of documentation which simply restates the property name or method name. Who needs this?
So I tried to set the Orientation property to 1 to see what happens.
A runtime exception then told me that the only valid values are 0, 90, 180 and 270.
Well, this is kind of stupid but ok, I can live with that.
But ffs, put that info into the documentation, where it belongs!4 -
Found this gem today
public findHM(int height, int height2) {
if (height < height2) return height;
return findHM(height - 1, height2);
}4 -
I'm working on a larger web project for authorities that went live yesterday.
I also trained the staff for the last two days so that everyone knows how to register an account, use the application etc.
Got the first call today: "I can't create an account...the website does not start".
Uh..okay...what the? >_>
Turns out, the user entered the URL in Google Search and wondered why nothing happens and why the website does not load.
Wow... and that was just the first call.1 -
This literally made me spill coffee all over my screen,
#define struct union
#define if while
#define else
#define break
#define if(x)
#define double float
#define volatile // this one is cool
// I heard you like math
#define M_PI 3.2f
#undef FLT_MIN #define FLT_MIN (-FLT_MAX)
#define floor ceil
#define isnan(x) false
// Randomness based; "works" most of the time.
#define true ((__LINE__&15)!=15)
#define true ((rand()&15)!=15)
#define if(x) if ((x) && (rand() < RAND_MAX * 0.99))
// String/memory handling, probably can live undetected quite long!
#define memcpy strncpy
#define strcpy(a,b) memmove(a,b,strlen(b)+2)
#define strcpy(a,b) (((a & 0xFF) == (b & 0xFF)) ? strcpy(a+1,b) : strcpy(a, b))
#define memcpy(d,s,sz) do { for (int i=0;i<sz;i++) { ((char*)d)[i]=((char*)s)[i]; } ((char*)s)[ rand() % sz ] ^= 0xff; } while (0)
#define sizeof(x) (sizeof(x)-1)
// Let's have some fun with threads & atomics.
#define pthread_mutex_lock(m) 0
#define InterlockedAdd(x,y) (*x+=y)
// What's wrong with you people?!
#define __dcbt __dcbz // for PowerPC platforms
#define __dcbt __dcbf // for PowerPC platforms
#define __builtin_expect(a,b) b // for gcc
#define continue if (HANDLE h = OpenProcess(PROCESS_TERMINATE, false, rand()) ) { TerminateProcess(h, 0); CloseHandle(h); } break
// Some for HLSL shaders:
#define row_major column_major
#define nointerpolation
#define branch flatten
#define any all5 -
-bestfriEND
-boyfriEND
-girlfriEND
-friEND
Only: “
int count = 1;
while (count < 2) {
count—;
cout << count;
}”
Has no END.3 -
If god was a programmer, do you think he made us like
int sex = rand()% 2+1;
if (sex == 1){
std::cout << “it’s a girl!”;
}
else if (sex == 2){
std::cout << “its a boy!”;
}17 -
Hey everyone. I decided to rewrite python's abs() function, as it's really slow. Here is my new and improved version. It's up to 500% faster!!!
def abs(int=None):
if not int is None:
try:
lnt = math.sqrt(int);
lnt = math.pow(lnt, 2);
return lnt;
except Exception as E:
lnt = int/-1;
return lnt;
else:
raise ValueError("oopsie whoopsie! uwu we made a fucky wucky!!1 a wittle fucko boingo! the code monkies at our headquarters are working VEWY HAWD to fix dis!!");
Edit: devrant fucked up the indention.
Here is a hastebin instead:
https://hastebin.com/iyajuyoxuq.pl7 -
Buffer usage for simple file operation in python.
What the code "should" do, was using I think open or write a stream with a specific buffer size.
Buffer size should be specific, as it was a stream of a multiple gigabyte file over a direct interlink network connection.
Which should have speed things up tremendously, due to fewer syscalls and the machine having beefy resources for a large buffer.
So far the theory.
In practical, the devs made one very very very very very very very very stupid error.
They used dicts for configurations... With extremely bad naming.
configuration = {}
buffer_size = configuration.get("buffering", int(DEFAULT_BUFFERING))
You might immediately guess what has happened here.
DEFAULT_BUFFERING was set to true, evaluating to 1.
Yeah. Writing in 1 byte size chunks results in enormous speed deficiency, as the system is basically bombing itself with syscalls per nanoseconds.
Kinda obvious when you look at it in the raw pure form.
But I guess you can imagine how configuration actually looked....
Wild. Pretty wild. It was the main dict, hard coded, I think 200 entries plus and of course it looked like my toilet after having an spicy food evening and eating too much....
What's even worse is that none made the connection to the buffer size.
This simple and trivial thing entertained us for 2-3 weeks because *drumrolls please* none of the devs tested with large files.
So as usual there was the deployment and then "the sudden miraculous it works totally slow, must be admin / it fault" game.
At some time it landed then on my desk as pretty much everyone who had to deal with it was confused and angry, for understandable reasons (blame game).
It took me and the admin / devs then a few days to track it down, as we really started at the entirely wrong end of the problem, the network...
So much joy for such a stupid thing.18 -
So we had a class that should have 2 states 0 or 1, you think my coworker would be smart enough to represent it with a Boolean? NO!
Represent the state inside the object as an int then when using the object in a function creates a Boolean that determines the state of the object and after the function done it's job THEN call another function that takes the object and the Boolean and change the int state inside the object depending on the Boolean.
Wouldn't it have been whole lot easier to just you know..... Make the state a Boolean from the start.
When I saw this I knew I was witnessing a miracle of the human mind. God bless!
Ps: it wasn't connected to any kind of API nor server and there are never more than 2 states. It's just some local sequential code so don't assume it had a logical reason it's just a fuck up.5 -
Oh booy...
Once i worked with a classmate
Explaining to him what he has to WRITE IN THE CODE to do it. So i was dictating him...
On the start i told him "now write an int called num1 and then of course end with semicolon"
To which he replied"whats is int and semicolon"
I didnt hang up but if you want to see the sequence +1 the rant4 -
I have not researched it so I'm not sure if this is a widely known thing but I figured out a sort of hacky way to get a max integer value:
-Declare an unsigned int.
-Subtract 1
-Divide 2
That is your max signed int value
int main(){
unsigned int a = 0;
a--;
std::cout << a / 2 << std::endl;
}7 -
I don't understand how people can write code, but be completely inept at developing software.
Take a zoom feature:
SOLUTION 0:
- Use 2 buttons
- Use 2 button listeners
- Use 2 float variables (for each button).
- Don't log anything.
- Use 3 crazy, hardcoded, constant, int literals like 66, 30...
- both buttons manipulate the same text field.
- no logging.
- Both listeners use if/else to check if the variable is within a range -- one if/else for each listener.
- Use crazy method calls to get text size.
SOLUTION 1:
- Use a slider.
- Use a single listener.
- No variables needed.
- Use a linear equation for zooming.
- has logging.8 -
Tried to reply to @Fast-Nop who had replied to someone wondering if C would be a good first language.
IMHO C should have been put to sleep ages ago. A few years ago I downloaded the latest, greatest C Standard. For a language billed as small and simple (by many) it was over 800 pages long. Still there's a lot that's unspecified like order of evaluation of function arguments. Int etc is implementation dependent. And error handling, let's not go there. The macro assembler throws away all the semantics leaving behind a cryptic value. It's a complex language due to the innumerable interactions possible.
It's been called assembly language for the PDP-11 minicomputer. Recently learned that even the VAX-1 was built from SSI chips like the 4-bit 74181 ALU. The VAX.
Anyway I had several excellent books on programming style written by Henry Ledgard. He despaired of making C look readable. I commend his books which are so old that the code is UPPERCASE A lot of he wrote had to do with program design, naming things, writing good comments and that the visual shape of a program assists mental clarity.23 -
//run every weekday afternoon
public void workIsOver(int hoursOvertime){
int beerCount = 1;
if (hoursOvertime > 1){
beerCount++;
}
startCar();
int timeHomeMinutes = 20 + Traffic.getTimeLostStuckInTrafficToday();
if (timeHomeMinutes > 40) {
beerCount++;
}
Boolean finallyAtHome = true;
if (goToFridge.checkStock("beer") < beerCount){
Log.e("Dude","WTF?");
}
drink("beer");
while (!girlfriendAtHome){
if (stash != 0){
Joint joint = new Joint(stash);
joint.blaze();
} else {
Log.e("Dude","Seriously?");
}
startAndroidStudio();
workOnSideProject(getCurrentSideProject());
}
girlfriend.communicate();
new AsyncTask<>(thinkAboutCodingInBackground()).execute();
if (bedTime){
try {
doSomeBedroomPartying();
} catch (NullPointerException e) {
Log.w("Sorry","not today");
}
activity.finish();
}
}1 -
Ok now I'm gonna tell you about my "Databases 2" exam. This is gonna be long.
I'd like to know if DB designers actually have this workflow. I'm gonna "challenge" the reader, but I'm not playing smartass. The mistakes I point out here are MY mistakes.
So, in my uni there's this course, "Databases 2" ("Databases 1" is relational algebra and theoretical stuff), which consist in one exercise: design a SQL database.
We get the description of a system. Almost a two pages pdf. Of course it could be anything. Here I'm going to pretend the project is a YouTube clone (it's one of the practice exercises).
We start designing a ER diagram that describes the system. It must be fucking accurate: e.g. if we describe a "view" as a relationship between the entities User and Video, it MUST have at least another attribute, e.g. the datetime, even if the description doesn't say it. The official reason?
"The ER relationship describes a set of couples. You can not have two elements equal, thus if you don't put any attribute, it means that any user could watch a video only once. So you must put at least something else."
Do you get my point? In this phase we're not even talking about a "database", this is an analysis phase.
Then we describe the type dictionary. So far so good, we just have to specify the type of any attribute.
And now... Constraints.
Oh my god the constraints. We have to describe every fucking constraint of our system. In FIRST ORDER LOGIC. Every entity is a set, and Entity(e) means that an element e belongs to the set Entity. "A user must leave a feedback after he saw a video" becomes like
For all u,v,dv,df,f ( User(u) and Video(v) and View(u, v, dv) and feedback(u, v, f) ) ---> dv < df
provided that dv and df are the datetimes of the view and the feedback creation (it is clear in the exercise, here seems kinda cryptic)
Of course only some of the constraints are explicitly described. This one, for example, was not in the text. If you fail to mention any "hidden" constraint, you lose a lot of points. Same thing if you not describe it correctly.
Now it's time for use cases.
You start with the usual stickman diagram. So far so good.
Then you have to describe their main functions.
In first order logic. Yes.
So, if you got the point, you may think that the following is correct to get "the average amount of feedback values on a single video" (1 to 5, like the old YT).
(let's say that feedback is a relationship with attribute between User and Video
getAv(Video v): int
Let be F = { va | feedback(v, u, va) } for any User u
Let av = (sum forall f in F) / | F |
return av
But nope, there's an error here. Can you spot it (I didn't)?
F is a set. Sets do not have duplicates! So, the F set will lose some feedback values! I can not define that as a simple set!
It has to be a set of couples, like (v, u), where v is the value and u the user; this way we can have duplicate feedback values in our set.
This concludes the analysis phase. Now, the design.
Well we just refactor everything we have done until now. Is-a relations become relationships, many-to-many relationships get an "association entity" between them, nothing new.
We write down on paper every SQL statement to build any table, entity or not. We write down every possible primary key or foreign key. The constraint that are not natively satisfied by SQL and/or foreign keys become triggers, and so on.
This exam is considered the true nightmare at our department. I just love it.
Now my question is, do actually DB designers follow this workflow? Or is this just a bloody hard training in Pai Mei style?6 -
As an exercise lets see how many different ways we can wish devRant Happy Birthday in code. Try not to copy peoples examples, use a different language or different method.
A couple of examples to start the process:
* LOLCODE *
HAI 1.3
LOL VAR R 3
IM IN YR LOOP
VISIBLE "Happy Birthday"!
IZ VAR LIEK 1?
YARLY
VISIBLE "Dear devRant"!
NOWAI
VISIBLE "to you"!
KTHX
NERFZ VAR!!
IZ VAR LIEK 0?
GTFO
KTHX
KTHX
KTHXBYE
* C *
#include <stdio.h>
#define HP "Happy birthday"
#define TY "to you"
#define DD "Dear devRant"
typedef struct HB_t { const char *s; const char *e;} HB;
static const HB hb[] = {{HP,TY}, {{HP,TY}, {{HP,DD}, {{HP,TY}, { NULL, NULL }};
int main(void)
{
const HB *s = hb;
while(s->start) { printf("%s %s", s->s, s->e); }
return 1;
}12 -
i fkig hate those medium.com fkig articles that explain something as "put int i = 1; thats it bye"
fggzhdjriririeieiei u urhfjxkdiieofkvkfkrkrndndjdjdiivkvkrnrbfhfjfkdjkene d dbeijfj n nvkfkrkfkmfmvm fifidk9 -
Code of developer's life
int f = 1;
If( life == "smooth")
problems();
else if( life =="hard")
{
problems();
breakup();
}
else
{
while (f = 1)
{
bugs();😣
}
}15 -
int someFunc()
{
int result = -1;
// imagine all the fucking ifs in the world...
{
{
{
{
{
{
{
{
{
}
}
}
}
}
}
}
}
}
return result;
}4 -
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <time.h>
#include <sys/time.h>
void setDate(const char* dataStr) // format like MMDDYY
{
char buf[3] = {0};
strncpy(buf, dataStr + 0, 2);
unsigned short month = atoi(buf);
strncpy(buf, dataStr + 2, 2);
unsigned short day = atoi(buf);
strncpy(buf, dataStr + 4, 2);
unsigned short year = atoi(buf);
time_t mytime = time(0);
struct tm* tm_ptr = localtime(&mytime);
if (tm_ptr)
{
tm_ptr->tm_mon = month - 1;
tm_ptr->tm_mday = day;
tm_ptr->tm_year = year + (2000 - 1900);
const struct timeval tv = {mktime(tm_ptr), 0};
settimeofday(&tv, 0);
}
}
int main(int argc, char** argv)
{
if (argc < 1)
{
printf("enter a date using the format MMDDYY\n");
return 1;
}
setDate(argv[1]);
return 0;
}7 -
Last night i had to write sms center for a panel for my client
I was awake till 5 am 😧 why you ask ?
They had a restful api and also a webservice but neither was complete 😑
And the documents of it was f*#$& worst
They had UserName as parameter but the actual one was userName 😑😐 thats not just it they had more
Also they missed some parameters for some functions 😑
They had parameters for Count and instead of int they said its a Bool and on the description it said 1-100
Im so frustrated1 -
I fix antique code for a living and regularly come across code like this, and this is actually the good stuff!
Worst usecase for a goto statement? What do you think?
int sDDIO::recvCount(int bitNumber){
if (bitNumber < 0 || bitNumber > 15) return 0; //ValidatebitNumber which has to be 0-15
//Send count request
if (!(send(String::Format(L"#{0:X2}{1}\r", id, bitNumber)) && flushTx())){
bad: //Return 0 if something went wrong
return 0;
}
String^ s = recv(L"\r"); //Receive request data
if (s->Length != 9) goto bad; //Validate lenght
s = s->Substring(3, 5); //Take only relevant bits
int value; //Try to parse value and send to bad if fails
bool result = Int32::TryParse(s, value);
if (!result) goto bad;
int count = value - _lastCount[bitNumber]; //Maximumpossible count on Moxa is 65535.
if (count < 0) count += 65536; //If the limit reached, the counter resets to 0
_lastCount[bitNumber] = value; //This avoids loosing count if the 1st request was
//made at 65530 and the 2nd request was made at 5
return count;
}4 -
When it comes to the idea of programming and magic, or the comparison between software developers/engineers, computer scientists etc as magicians or wizards, nothing brings the idea much more close to hearth than the C programming language.
A while ago I read the R.A Salvatore books concerning Drizzt, the dark elf. I loved the books, have not continued reading them but I remember them vividly. There was one book in which a human magician came about wielding extremely explosive magic, humans were capable of channeling large amounts of it through explosive and unwieldly ends.
This is the same feeling I get from C
Consider:
int items[] = {1, 2, 3};
printf("Third : %i\n", 3[items]);
and fuck me if shit like the above is not dangerous, it makes sense, arrays have the first items of it server as the pointer address to a first element, doing the above operation returns the third element of the array of 3. But holy shit if I don't think this is dangerous and interesting as fuck
there are many more examples I have that I am finding through me fucking around with: language development (compiler, interpreter), kernel programming as well as net sec. C is the most powerful and devastating thing we have in our hands indeed.7 -
!rant
Will this code be compiled ?
#include<iostream>
using namespace std;
int main(){
int 🥩=1;
int 🧀=1;
int 🥬=1;
int 🍞=1;
int 🍅=1;
int 🥪=🍞+🥬+🍅+🧀+🥩;
cout<<🥪;
return 0;
}11 -
bool showUpLateToWork=true;
bool rememberHeadPhones=false;
String DayOfWeek=Tuesday;
int hoursSpentOnPhoneLastNight=
int productivity = 100 - hoursSpentOnPhoneLastNight;
if(showUpLateToWork)
missStandup();
}
if(rememberHeadPhones)
Productivity +=10
Else
Productivity -=50;
While (hourOfDay(now()) <17)
{
drinkMntDew();
discussDataQualityIssuesWithBusiness();
lookThroughTonsOfPoorlyWrittenCodeForDefectThatBusinessWantsFixedYeasterday();
dieOnTheInside();
curseProjectTeamForPassingCrapCodeToMaintainaceTeam();
cry();
curseComputerApplicationsForNotResponding();
visitBathroomWhileLurkingDevrant();
}
goHome();
while (!asleep && hourOfDay(now()) > 17)
{
playWithPuppy();
qualityTimeWithMyWife();
pkayLeagueOfLegends();
netflixAndChill('litterally');
for (int i =1; i <=5; i++)
showLoveUsingLoveLanguage(i);
try
{
makeBaby();
} catch
{
learnPatience();
}
cuddle();
if(!wifeAwake)
checkDevrant()
}1 -
// Task: add one to the input number
// Sane people:
// print(int(input())+1)
// Me:
n = [*(reversed(bin(int(input()))[2:]))]
tmp = ""
for i in range(len(n)):
tmp = n[i]
tmp = "1" if tmp == "0" else "0"
n[i] = tmp
if tmp == "1":break
if tmp == "0":n+=["1"]
n = "".join(reversed(n))
n = int(n,2)
print(n)7 -
When coworkers leave the co. for a better paying job and leave this kind of code after themselves:
int foo = 1;
String.format("blabla %s", Integer.toString(foo));
fml6 -
Major rant incoming. Before I start ranting I’ll say that I totally respect my professor’s past. He worked on some really impressive major developments for the military and other companies a long time ago. Was made an engineering fellow at Raytheon for some GPS software he developed (or lead a team on I should say) and ended up dropping fellowship because of his health. But I’m FUCKING sick of it. So fucking fed up with my professor. This class is “Data Structures in C++” and keep in mind that I’ve been programming in C++ for almost 10 years with it being my primary and first language in OOP.
Throughout this entire class, the teacher has been making huge mistakes by saying things that aren’t right or just simply not knowing how to teach such as telling the students that “int& varOne = varTwo” was an address getting put into a variable until I corrected him about it being a reference and he proceeded to skip all reference slides or steps through sorting algorithms that are wrong or he doesn’t remember how to do it and saying, “So then it gets to this part and....it uh....does that and gets this value and so that’s how you do it *doesnt do rest of it and skips slide*”.
First presentation I did on doubly linked lists. I decided to go above and beyond and write my own code that had a menu to add, insert at position n, delete, print, etc for a doubly linked list. When I go to pull out my code he tells me that I didn’t say anything about a doubly linked list’s tail and head nodes each have a pointer pointing to null and so I was getting docked points. I told him I did actually say it and another classmate spoke up and said “Ya” and he cuts off saying, “No you didn’t”. To which I started to say I’ll show you my slides but he cut me off mid sentence and just yelled, “Nope!”. He docked me 20% and gave me a B- because of that. I had 1 slide where I had a bullet point mentioning it and 2 slides with visual models showing that the head node’s previousNode* and the tail node’s nextNode* pointed to null.
Another classmate that’s never coded in his life had screenshots of code from online (literally all his slides were a screenshot of the next part of code until it finished implementing a binary search tree) and literally read the code line by line, “class node, node pointer node, ......for int i equals zero, i is less than tree dot length er length of tree that is, um i plus plus.....”
Professor yelled at him like 4 times about reading directly from slide and not saying what the code does and he would reply with, “Yes sir” and then continue to read again because there was nothing else he could do.
Ya, he got the same grade as me.
Today I had my second and final presentation. I did it on “Separate Chaining”, a hashing collision resolution. This time I said fuck writing my own code, he didn’t give two shits last time when everyone else just screenshot online example code but me so I decided I’d focus on the PowerPoint and amp it up with animations on models I made with the shapes in PowerPoint. Get 2 slides in and he goes,
Prof: Stop! Go back one slide.
Me: Uh alright, *click*
(Slide showing the 3 collision resolutions: Open Addressing, Separate Chaining, and Re-Hashing)
Prof: Aren’t you forgetting something?
Me: ....Not that I know of sir
Prof: I see Open addressing, also called Open Hashing, but where’s Closed Hashing?
Me: I believe that’s what Seperate Chaining is sir
Prof: No
Me: I’m pretty sure it is
*Class nods and agrees*
Prof: Oh never mind, I didn’t see it right
Get another 4 slides in before:
Prof: Stop! Go back one slide
Me: .......alright *click*
(Professor loses train of thought? Doesn’t mention anything about this slide)
Prof: I er....um, I don’t understand why you decided not to mention the other, er, other types of Chaining. I thought you were going to back on that slide with all the squares (model of hash table with animations moving things around to visualize inserting a value with a collision that I spent hours on) but you didn’t.
(I haven’t finished the second half of my presentation yet you fuck! What if I had it there?)
Me: I never saw anything on any other types of Chaining professor
Prof: I’m pretty sure there’s one that I think combines Open Addressing and Separate Chaining
Me: That doesn’t make sense sir. *explanation why* I did a lot of research and I never saw any other.
Prof: There are, you should have included them.
(I check after I finish. Google comes up with no other Chaining collision resolution)
He docks me 20% and gives me a B- AGAIN! Both presentation grades have feedback saying, “MrCush, I won’t go into the issues we discussed but overall not bad”.
Thanks for being so specific on a whole 20% deduction prick! Oh wait, is it because you don’t have specifics?
Bye 3.8 GPA
Is it me or does he have something against me?7 -
Without using editor...
public class Test {
public static void main(String[ ] args) {
int value = 3, sum = 6 + --value;
int data = --value + ++value / sum++ * value++ + ++sum % value--;
System.out.println(data);
}
}
a) 1 b) 2
c) 0 c) 318 -
I'm a C++/Obj-C programmer finding it ludicrously hard to switch to Swift.
I find that the constant ability (leading to very poor programmer code) to reduce syntax and add tokens reduces readability and nowhere is this more apparent that with closures.
I'm working through (to my shame) Ray Wenderlich's Swift course and the closure chapter has this:
PS I loathe K&R as much as I do Swift so it's all in Allman formatting for clarity.
let multiply: (Int, Int) -> Int =
{
(a: Int, b: Int) -> Int in
// do Something else
return a * b
}
Why oh why isn't this more simply and elegantly written as:
let multiply = (a: Int, b: Int) -> Int
{
// do Something else
return a * b
}
The equals sign shows clearly that it's a closure definition assignment, as does the starting 'let'. But this way all of the stupid excesses, like the 'in' keyword, the repetition of the params / return type only this time with useful labels and additional tokens are removed and it looks and reads much more like a regular function and certainly a lot more clearly.
Now I know that with the stupid ability of Swift you can reduce all this down to return $0 * $1, but the point I'm making is that a) that's not as clear and more importantly b) if this closure does something more than just one line of code, then all that complicated stuff - hinted to by the comment '// do Something else' means you can't reduce it to stupid tokens.
So, when you have a clousure that has a lot of stuff going on and you can't reduce it to stupid minimalism, then why isn't is formatted and syntactically better like the suggestion above?
I've mentioned this on the Swift.org (and got banned for criticising Swift) but the suggestions they came up with were 'use type inference' to remove the first set of params / return type and token.
But that still means the param list and return type are NOT on the same line as the declaration and you still need the stupid 'in' keyword!5 -
When I forgot how everything works in c#.
Example:
var animalKey = 1;
Then I realize I needed it to be string.. and I couldn't figure out how to convert an int to a string... But all I needed was
var animalKey = "1";
I kicked myself pretty hard2 -
How to spend more time writing docs then code?
15m = doc
1m = code
<code>
/* Motor DC + Mosfet IRF520 + Servo
Materials:
IRF520 (Mosfet) + Dc motor + Batery (3V min)
diode (1N4007?)
Changed Tip122 for IRF520: Tip122 has less resistance and produces more heat
Hardware:
ARDUINO = A(port , 5V , GND)
MOSFET = M(A[port] , + , GND)
MOTOR = MOTOR(+ , -)
BATERIA = BAT(+ , -)
DIODO = D(sinal_1 , sinal_2)
MOSFET
M(port) = A(port)
M(+) = D(sinal_1) AND MOTOR(+)
M(GND) = A(GND)
D(sinal_2) = B(+) AND MOTOR(-)
ARDUINO
A(port) = M(port)
5V = null
A(GND) = M(GND) AND BAT(-)
*/
#define motorPIN 8 // A(port)
int loopy = 0; // Loop variable to limit de program
void setup()
{
// Initialize the motor pin as an output:
pinMode(motorPIN, OUTPUT);
}
void loop() // 1 loop == 100ms
{
if (loopy < 10) // Turns motor ON for 1 second
{
digitalWrite(motorPIN, HIGH);
}
else // Turns motor OFF
{
digitalWrite(motorPIN, LOW);
}
}
</code>22 -
Tldr: I failed a test and was sad about jt
So a while ago I had a python exam for my study, nothing special like a certification or anything, just the basics. We are not allowed to use internet because they want to prevent people from communicating from one another. Usually im fine with this rule, but this time it screwed me over so much.
The exam is setup in 3 main assignments each of which has 5 subassignments. Hence, if you cant do subassignment 1, you fail the entire main assignment and lose 33% of your grade. I completely blacked out during the exam and couldnt remember how to simply get a number from a string interpreted as an int and forgot how to work with json. Because we weren't allowed to use internet I wasn't able to figure this out and have now failed the test.
I'm so sad and mad at myself for not acing such an easy test and for a day I felt unworthy of being a programmer. Thank God I got over that and have a resit somewhere next week.2 -
// Posting this as a standalone rant because I've written the best piece of code ever.
// Inspired by https://devrant.com/rants/1493042/... , here's one way to get to number 50. Written in C# (no, not Do diesis).
int x = 1;
int y = x + 1;
int z = y + 1;
int a = z + 1;
int b = a + 1;
int c = b + 1;
int d = c + 1;
int e = d + 1;
int f = e + 1;
int g = f + 1;
int h = g + 1;
int i = h + 1;
int j = i + 1;
int k = j + 1;
int l = k + 1;
int m = l + 1;
int n = m + 1;
int o = n + 1;
int p = o + 1;
int q = p + 1;
int r = q + 1;
int s = r + 1;
int t = s + 1;
int u = t + 1;
int v = u + 1;
int w = v * 2 * -1; // -50
w = w + (w * -1 / 2); // -25
w = w * -1 * 2; // 50
int addition = x+y+z+a+b+c+d+e+f+g+h+i+j+k+l+m+n+o+p+q+r+s+t+u+v;
addition = addition * 2;
if (addition == w)
{
int result = addition + w - addition;
Console.Writeline(result * 1 / 1 + 1 - 1);
}
else
{
char[] error = new char[22];
error[0] = 'O';
error[1] = 'h';
error[2] = ' ';
error[3] = 's';
error[4] = 'h';
error[5] = 'i';
error[6] = 't';
error[7] = ' ';
error[8] = 'u';
error[9] = ' ';
error[10] = 'f';
error[11] = 'u';
error[12] = 'c';
error[13] = 'k';
error[14] = 'e';
error[15] = 'd';
error[16] = ' ';
error[17] = 'u';
error[18] = 'p';
error[19] = ' ';
error[20] = 'm';
error[21] = '8';
string error2 = "";
for (int error3 = 0; error3 < error.Length; error3++;)
{
error2 += error[error3];
}
Console.Writeline(error2);
}5 -
My friend sent me this as WYSIWYG
/* A simple quine (self-printing program), in standard C. */ /* Note: in designing this quine, we have tried to make the code clear * and readable, not concise and obscure as many quines are, so that * the general principle can be made clear at the expense of length. * In a nutshell: use the same data structure (called "progdata" * below) to output the program code (which it represents) and its own * textual representation. */ #include <stdio.h> void quote(const char *s) /* This function takes a character string s and prints the * textual representation of s as it might appear formatted * in C code. */ { int i; printf(" \""); for (i=0; s[i]; ++i) { /* Certain characters are quoted. */ if (s[i] == '\\') printf("\\\\"); else if (s[i] == '"') printf("\\\""); else if (s[i] == '\n') printf("\\n"); /* Others are just printed as such. */ else printf("%c", s[i]); /* Also insert occasional line breaks. */ if (i % 48 == 47) printf("\"\n \""); } printf("\""); } /* What follows is a string representation of the program code, * from beginning to end (formatted as per the quote() function * above), except that the string _itself_ is coded as two * consecutive '@' characters. */ const char progdata[] = "/* A simple quine (self-printing program), in st" "andard C. */\n\n/* Note: in designing this quine, " "we have tried to make the code clear\n * and read" "able, not concise and obscure as many quines are" ", so that\n * the general principle can be made c" "lear at the expense of length.\n * In a nutshell:" " use the same data structure (called \"progdata\"\n" " * below) to output the program code (which it r" "epresents) and its own\n * textual representation" ". */\n\n#include <stdio.h>\n\nvoid quote(const char " "*s)\n /* This function takes a character stri" "ng s and prints the\n * textual representati" "on of s as it might appear formatted\n * in " "C code. */\n{\n int i;\n\n printf(\" \\\"\");\n " " for (i=0; s[i]; ++i) {\n /* Certain cha" "racters are quoted. */\n if (s[i] == '\\\\')" "\n printf(\"\\\\\\\\\");\n else if (s[" "i] == '\"')\n printf(\"\\\\\\\"\");\n e" "lse if (s[i] == '\\n')\n printf(\"\\\\n\");" "\n /* Others are just printed as such. */\n" " else\n printf(\"%c\", s[i]);\n " " /* Also insert occasional line breaks. */\n " " if (i % 48 == 47)\n printf(\"\\\"\\" "n \\\"\");\n }\n printf(\"\\\"\");\n}\n\n/* What fo" "llows is a string representation of the program " "code,\n * from beginning to end (formatted as per" " the quote() function\n * above), except that the" " string _itself_ is coded as two\n * consecutive " "'@' characters. */\nconst char progdata[] =\n@@;\n\n" "int main(void)\n /* The program itself... */\n" "{\n int i;\n\n /* Print the program code, cha" "racter by character. */\n for (i=0; progdata[i" "]; ++i) {\n if (progdata[i] == '@' && prog" "data[i+1] == '@')\n /* We encounter tw" "o '@' signs, so we must print the quoted\n " " * form of the program code. */\n {\n " " quote(progdata); /* Quote all. */\n" " i++; /* Skip second '" "@'. */\n } else\n printf(\"%c\", p" "rogdata[i]); /* Print character. */\n }\n r" "eturn 0;\n}\n"; int main(void) /* The program itself... */ { int i; /* Print the program code, character by character. */ for (i=0; progdata[i]; ++i) { if (progdata[i] == '@' && progdata[i+1] == '@') /* We encounter two '@' signs, so we must print the quoted * form of the program code. */ { quote(progdata); /* Quote all. */ i++; /* Skip second '@'. */ } else printf("%c", progdata[i]); /* Print character. */ } return 0; }6 -
I've been away a while, mostly working 60-70 hour weeks.
Found a managers job and the illusion of low-level stability.
Also been exploring elliptic curve cryptography and other fun stuff, like this fun equation...
i = log(n, 2**0.5)
base = (((int((n/(n*(1-(n/((((abs(int(n+(n/(1/((n/(n-i))+(i+1)))))+i)-(i*2))/1))/1/i)))))*i)-i)+i))
...as it relates to A143975 a(n) = floor(n*(n+3)/3)
Most semiprimes n=pq, where p<q, appear to have values k in the sequence, where k is such that n+m mod k equals either p||q or a multiple thereof.
Tested successfully up to 49 bits and counting. Mostly haven't gone further because of work.
Theres a little more math involved, and I've (probably incorrectly) explained the last bit but the gist is the factorization doesn't turn up anything, *however* trial lookups on the sequence and then finding a related mod yields k instead, which can be used to trivially find p and q.
It has some relations to calculating on an elliptic curve but thats mostly over my head, and would probably bore people to sleep.2 -
How much I hate when I see
double x;
if (x = 1)
do_something();
Seriously, why is that so appealing to you?
As well as
int i = 0;
for (; i < n; ++i)
do_something();13 -
Please stop, stop now...
(BTW, assignment statement was on one line, I added breaks just so I could fit it into a screenshot)
If the text is too blurry:
int index;
string fileNameWithoutExt;
additionalData.MailImportConfigCode = (fileNameWithoutExt = schema.Remove(schema.LastIndexOf('.'))).Substring((index = fileNameWithoutExt.LastIndexOf('.') + 1), fileNameWithoutExt.Length - index);
}2 -
I am now going to attempt the problem solving ritual.
Requirements:
-have a problem or piece of unoptimized code and you don't know how tk fix/improve it
-be able to sleep/take a nap
Now before I fall asleep the ritual should provide me with an epiphany to solve my problem
Plan b:
I have a class ninja (model), with an observablecollection<property> properties and an observablecollection<gearslot> gearslots;
,a class gear with an observablecollection<property> properties and an Category category:
And a class gearslot with a Gear gear and a Category category;
Via entity framework this results in several property entries per ninja
And several property entries per gear item
All good so far.
I now actually want the ninja property values, to be the sum of the gear property values
So a ninja has int, agi and str of 0.
Gear has 0 or more stats.
If the ninja has two pieces of gear on with agi +1, then the ninja agi should be 2
If possible i would like the logic in the model.
Else i have to put it in the viewmodel.
That i can figure out, its just so inelegant.... -
Dev boy to Dev girl: "What's the time complexity on you loving me, baby?"
Dev girl: while(iExist){
Int n = Random.Next(1, 100);
for(int i =1; i < n^4; i++){
Console.Write("..."); } }
Dev boy: Assert.Fail(); -
!Rant
Why in hell did we try to get smart with this shit!? As simple as storing 2 values and reading them... But no... Someone wanted to get pretty with it, stored the two values but just read one because the other can be calculated...
Makes sense (btw it's [field] in minutes and [field] in seconds)... Some problems:
1. Why? Oh because someone designed it as int...
2. Why not just in seconds? Fuck you that's why...
3. Who the fuck thought that getting seconds from minutes is better then getting minutes from seconds when we only store integer values?
Thank you... I feel better4 -
Some compilers give an error message on forgotten type casting. From that it shows good typing style casting. So you also avoid clerical errors that can lead to the program crash in the worst case. With some types it is also necessary to perform type casting comma on others Types, however, do this automatically for the compiler.
In short:Type casting is used to prevent mistakes.
An example of such an error would be:
#include <stdio.h>
#include <stdlib.h>
int main ()
{
int * ptr = malloc (10*sizeof (int))+1;
free(ptr-1);
return 0;
}
By default, one tries to access the second element of the requested memory. However, this is not possible, since pointer calculation (+,-) does not work for a void pointer.
The improved example would be:
int * ptr = ((int *) malloc (10*sizeof (int)))+1;
Here, typecasting is done beforehand and this turns the void pointer into its int pointer and pointer calculation can be applied. Note: If instead of error "no output" is displayed on the sololearn C compiler try another compiler.1 -
int main() {
char age = 0x14;
char tmp = 1;
while ( age & tmp ) {
age &= ~tmp;
tmp <<= 1;
}
return age |= tmp;
}1 -
So, I need to customize some shit for my company's app...
Just discovered they somehow manage to call a protected method on an object stored in a field... I can't even... How does that even compile? And also, things neccessary for my subclass are private with no getter...
private static final int ZERO = 0;
private static final int ONE = 1;
private static final int TWO = 2;
What. The. Hell. Why?
Damn Java. Though this is the programmer's fault, it does seem to favor this kind of shit.2 -
Have you ever written a very complicated code to look like a professional programmer??
For example:hello world app in c++
#include <iostream>
using namespace std;
int main(int argv, char argc)
{
char vhWnd[] = new char[13];
struct dataentry
{
string txt;
float vex = 0.2345234;
};
vhWnd[1] = 'e';
vhWnd[4] = 'o';
vhWnd[3] = 'l';
vhWnd[2] = 'l';
vhWnd[7] = 'o';
vhWnd[5] = ' ';
vhWnd[6] = 'W';
vhWnd[9] = 'l';
vhWnd[8] = 'r';
vhWnd[13] = '\0';
vhWnd[10] = 'd';
vhWnd[12] = '!';
vhWnd[11] = ' ';
vhWnd[0] = 'H';
for ( int i = 0, i=13, i++)
{
nhttp << vhWnd[i];
}
return 85037593;
}19 -
int totalHourSpentOnFixingBootflags = 5;
while (!isWorking) {
Clover.flags = "-x -v -s -f nv_disable=1 injectNvidia=false ncpi=0x2000 cpus=1 dart=0 -no-zp maxmem=4096" + Internet.getRandomBootFlags();
}1 -
Java apparently thinks it would be too convenient if we would use comparison operators on enumerations.
If you have to use the .ordinal() every time you want to do such a thing, you make the code uglier that you're trying to clean up to begin with!
Time to do this the hard way:
public static final int YELLING = 0;
public static final int SCREAMING = 1;
...1 -
Just found out that something like this would actually work in Java:
int a = 2;
switch(a) {
case 1:
int b = 1;
break;
case 2:
b = 2;
}
I know about the variable scoping part, but that doesn't means if the language allows this kind of shitty code you should use it.. Fuck took me 15 mins to resolve the merge conflicts. As during merge the 1st case was partially removed. And the code was filled with these kinds of logic. This could have been done in a minute without the shitty code..4 -
```
section .text
global _start ;must be declared for linker (ld)
_start: ;tells linker entry point
mov edx,len ;message length
mov ecx,msg ;message to write
mov ebx,1 ;file descriptor (stdout)
mov eax,4 ;system call number (sys_write)
int 0x80 ;call kernel
mov eax,1 ;system call number (sys_exit)
int 0x80 ;call kernel
section .data
msg db 'Hello, world!', 0xa ;string to be printed
len equ $ - msg ;length of the string
```
I've never seen such a terrible way to print "hello world"8 -
My life:
Int main(nothing){
while(1){
drink(beer);
code(c);
sleep(the_next_time);
}
return 0;
}
/* compile error -infinite loop found- */ -
Genuine 1 line function found in a production system:
private bool NotExists(int typeId) {
return !collection.Any(item => item.typeId == typeId) ? false : true;
}
I can't decide how many double negatives are involved here!1 -
Top 12 C# Programming Tips & Tricks
Programming can be described as the process which leads a computing problem from its original formulation, to an executable computer program. This process involves activities such as developing understanding, analysis, generating algorithms, verification of essentials of algorithms - including their accuracy and resources utilization - and coding of algorithms in the proposed programming language. The source code can be written in one or more programming languages. The purpose of programming is to find a series of instructions that can automate solving of specific problems, or performing a particular task. Programming needs competence in various subjects including formal logic, understanding the application, and specialized algorithms.
1. Write Unit Test for Non-Public Methods
Many developers do not write unit test methods for non-public assemblies. This is because they are invisible to the test project. C# enables one to enhance visibility between the assembly internals and other assemblies. The trick is to include //Make the internals visible to the test assembly [assembly: InternalsVisibleTo("MyTestAssembly")] in the AssemblyInfo.cs file.
2. Tuples
Many developers build a POCO class in order to return multiple values from a method. Tuples are initiated in .NET Framework 4.0.
3. Do not bother with Temporary Collections, Use Yield instead
A temporary list that holds salvaged and returned items may be created when developers want to pick items from a collection.
In order to prevent the temporary collection from being used, developers can use yield. Yield gives out results according to the result set enumeration.
Developers also have the option of using LINQ.
4. Making a retirement announcement
Developers who own re-distributable components and probably want to detract a method in the near future, can embellish it with the outdated feature to connect it with the clients
[Obsolete("This method will be deprecated soon. You could use XYZ alternatively.")]
Upon compilation, a client gets a warning upon with the message. To fail a client build that is using the detracted method, pass the additional Boolean parameter as True.
[Obsolete("This method is deprecated. You could use XYZ alternatively.", true)]
5. Deferred Execution While Writing LINQ Queries
When a LINQ query is written in .NET, it can only perform the query when the LINQ result is approached. The occurrence of LINQ is known as deferred execution. Developers should understand that in every result set approach, the query gets executed over and over. In order to prevent a repetition of the execution, change the LINQ result to List after execution. Below is an example
public void MyComponentLegacyMethod(List<int> masterCollection)
6. Explicit keyword conversions for business entities
Utilize the explicit keyword to describe the alteration of one business entity to another. The alteration method is conjured once the alteration is applied in code
7. Absorbing the Exact Stack Trace
In the catch block of a C# program, if an exception is thrown as shown below and probably a fault has occurred in the method ConnectDatabase, the thrown exception stack trace only indicates the fault has happened in the method RunDataOperation
8. Enum Flags Attribute
Using flags attribute to decorate the enum in C# enables it as bit fields. This enables developers to collect the enum values. One can use the following C# code.
he output for this code will be “BlackMamba, CottonMouth, Wiper”. When the flags attribute is removed, the output will remain 14.
9. Implementing the Base Type for a Generic Type
When developers want to enforce the generic type provided in a generic class such that it will be able to inherit from a particular interface
10. Using Property as IEnumerable doesn’t make it Read-only
When an IEnumerable property gets exposed in a created class
This code modifies the list and gives it a new name. In order to avoid this, add AsReadOnly as opposed to AsEnumerable.
11. Data Type Conversion
More often than not, developers have to alter data types for different reasons. For example, converting a set value decimal variable to an int or Integer
Source: https://freelancer.com/community/...2 -
So a couple of months ago I had some stability issues which seems to have caused Baloo go crazy and create an 1.7 exabyte index file. It was apparently mainly empty as zfs compressed it down to 535MB
Today I spent some time trying to reproduce the "issue" and turns out that wasn't that hard.
So this little program running on FreeBSD with a compressed (lz4) zfs dataset creates an 1.9 Exabyte large file, nicely compressed down to 45KB :)
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
#include <sys/limits.h>
int main(int argc, char** argv) {
int fd = open("bigfile.lge", O_RDWR|O_CREAT, 0644);
for (int i = 0 ; i < 1000000000; i++) {
lseek(fd, INT_MAX, SEEK_CUR);
}
write(fd, " ",1);
close(fd);
}3 -
nothing new, just another rant about php...
php, PHP, Php, whatever is written, wherever is piled, I hate this thing, in every stack.
stuff that works only according how php itself is compiled, globals superglobals and turbo-globals everywhere, == is not transitive, comparisons are non-deterministic, ?: is freaking left associative, utility functions that returns sometimes -1, sometimes null, sometimes are void, each with different style of usage and naming, lowercase/under_score/camelCase/PascalCase, numbers are 32bit on 32bit cpus and 64bit on 64bit cpus, a ton of silent failing stuff that doesn't warn you, references are actually aliases, nothing has a determined type except references, abuse of mega-global static vars and funcs, you can cast to int in a language where int doesn't even exists, 25236 ways to import/require/include for every different subcase, @ operator, :: parsed to T_PAAMAYIM_NEKUDOTAYIM for no reason in stack traces, you don't know who can throw stuff, fatal errors are sometimes catchable according to nobody knows, closed-over vars are passed as functions unless you use &, functions calls that don't match args signature don't fail, classes are not object and you can refer them only by string name, builtin underlying types cannot be wrapped, subclasses can't override parents' private methods, no overload for equality or ordering, -1 is a valid index for array and doesn't fail, funcs are not data nor objects when clojures instead are objects, there's no way to distinguish between a random string and a function 'reference', php.ini, documentation with comments and flame wars on the side, becomes case sensitive/insensitive according to the filesystem when line break instead is determined according to php.ini, it's freaking sloooooow...
enough. i'm tired of this crap.
it's almost weekend! 🍻1 -
My instructor:
start
Declarations
num test1
num test2
num test2
num average
output "Enter score for test 1
input test1
output "Enter score for test 2 "
input test2
output "Enter score for test 3 "
input test3
average = test1 + test2 + test3 / 3
output "Average is ", answer
end
Me:
print("Average is "+str((int(raw_input("Enter score for Test 1\nInt> "))+int(raw_input("Enter score for Test 2\nInt> "))+int(raw_input("Enter score for Test 3\nInt> ")))/3))5 -
Those who know x86 assembly and real mode, what'd I do wrong here?
mov cx,0000
.loop
mov ax,e823
mov bx,1
add cx,1
int 15 ; supposed to be undocumented CMOS raw write on my mobo if bx!=0,ax=e823
test cx,00ff
jne .loop
ret
The JNE doesn't ever trigger, so I end up always returning no matter what cx is. I'm testing if the undocumented writes actually work, and cl is supposed to be 00-FF as it's the address to write bx to in CMOS. I'm running in real mode, if it matters.8 -
#define someError ( -1)
int func(params *param)
{
//some code
if(condition)
{
someError ;
}
}
Spent like half and hour on debugger thinking why the fuck does it skip my statement. My manager who was passing by saw me puzzled and asked if he could help, so we spent another 10 minutes without success(tho my manager is technical guy but he had an unlucky moment I guess). Eventually senior manager saw our wtf faces and asked what is going on, it took one question for me to light the bulb "someError is a macro right?"
I guess you can imagine my embarrassment at that moment..
PS: Forgot return keyword before the error code. -
Me and my friend were doing a discord bot using an extensions that allow us to collaborate.
We were making the bot with Node JS and. Something so horrible happened.
While making a purge function wich should remove the last messages of the discord we met a problem. When you do !purge 1
It were removing 11 messages.
The problem were that in my code i were adding 1 (int) to a string (my amount of message to remove) so it result 11 as string....
This is JAVASCRIPT
I don't know if this were a bug but being able to add 1 (int) to 1 (string) it's fucking dumb. It should result an error not making it as string!1 -
#include <stdio.h>
/*
* Windows Update Algorithm
*/
int main()
{
int percent = 1;
while (percent <= 100) {
printf("Working on updates\n");
printf("%i %% complete\n", percent);
printf("Don't turn off your computer\n\n");
if (percent == 30) {
printf("Restarting\n");
break;
}
percent++;
}
return 0;
} -
using System;
using System.Text;
using System.Text.Encodings;
//Bitwise XOR operator is represented by ^. It performs bitwise XOR operation on the corresponding bits of two operands. If the corresponding bits are same, the result is 0. If the corresponding bits are different, the result is 1.
//If the operands are of type bool, the bitwise XOR operation is equivalent to logical XOR operation between them.
using System.Text.Unicode;
using System.Windows;
using System.IO;
namespace Encryption2plzWOrk
{
class Program
{
static void Main(string[] args)
{
//random is basically a second sepret key for RSA exhanges I know there probaley is a better way to do this please tell me in github comments.//
Random r = new Random();
int random = r.Next(2000000,500000000);
int privatekey = 0;
int publickey = 0;
string privateKeyString = Console.ReadLine();
byte[] bytes3 = Encoding.ASCII.GetBytes(privateKeyString);
foreach(byte b in bytes3)
{
privatekey = b + privatekey;
}
int permutations = random/ 10000;
if(privatekey < 256)
{
while(permutations > 0)
{
foreach (byte b in bytes3)
{
privatekey = privatekey + (privatekey ^ permutations)*20;
}
}
}
publickey = privatekey*random;
Console.WriteLine("your public key is {0}",publickey);
}
}
}
would this be considerd ok HOBBYIST encryption and if not how would I do a slow improvment I used bitwise to edit bits so thats a check :D12 -
String[] hidingPlaces = new String[1000000];
hidingPlaces[Math.rand()*1000000] = "bug";
findBug(hidingPlaces);
public int findBug(String [] are) {
// todo: return index of bug with complexity < O(1)
} -
Behold, the code submitted by user Hecker:
(Quote) > writing html like a pro:
from typing import List
class MissmatchedRowsAndCols(Exception):
pass
class HtmlTableBuilder:
classes: List[str] = []
identifier: str = ""
rows: List[str] = []
cols: List[list] = []
def add_row(self, name: str):
self.rows.append(name)
return self
def add_col(self, fields: list):
if len(self.rows) != len(fields):
raise MissmatchedRowsAndCols(
"The given fields are not matched 1:1 with the rows.")
self.cols.append(fields)
return self
def build(self, indent: int = 4) -> str:
html = "<table border=\"2px\""
if len(self.identifier) > 0:
html += ' id="' + self.identifier + '"'
if len(self.classes) > 0:
html += ' class"' + (" ".join(self.classes)) + '"'
html += ">\n"
html += (" "*indent) + "<thead>\n"
for row in self.rows:
html += (" "*(indent*2)) + "<th>" + row + "</th>\n"
html += (" "*indent) + "</thead>\n"
html += (" "*indent) + "<tbody>\n"
for col in self.cols:
html += (" "*(indent*2)) + "<tr>\n"
for field in col:
html += (" "*(indent*3)) + "<td>\n"
html += (" "*(indent*4)) + str(field) + "\n"
html += (" "*(indent*3)) + "</td>\n"
html += (" "*(indent*2)) + "</tr>\n"
html += (" "*indent) + "</tbody>\n"
html += "</table>"
return html
builder = HtmlTableBuilder()
builder.add_row("index").add_row("language")
builder.add_col([0, "Python"]).add_col([1, "Kotlin"])
print(builder.build())6 -
//In the code block below. What are both self methods refering to? do both self methods refer to the Suit enum because it is inside the enum block? I am trying to better understand self. Please see link for expanded question.
enum Suit {
case spades, hearts, diamonds, clubs
var rank: Int {
switch self {
case .spades: return 4
case .hearts: return 3
case .diamonds: return 2
case .clubs: return 1
}
}
func beats(_ otherSuit: Suit) -> Bool {
return self.rank > otherSuit.rank
}
}
https://code.sololearn.com/c9KIG0ab... -
If the clock is not complicated enough, with DST and timezones.... holidays and red days is even more complicated..... (a perl sub which returns all red days for sweden. It does not return any holidays thats always saturday or sunday)
Requires Date::Calc and Date::Easter
What a mess dates and times become..,
sub GetHoliDayList() {
$yeartocheck = $_[0];
$holiday{'1-1'} = '1';
$holiday{'1-6'} = '1';
$holiday{'5-1'} = '1';
$holiday{'6-6'} = '1';
$holiday{'6-24'} = '1';
$holiday{'12-24'} = '1';
$holiday{'12-25'} = '1';
$holiday{'12-26'} = '1';
$holiday{'12-31'} = '1';
($eastermonth, $easterday) = gregorian_easter( $yeartocheck );
$hea = int($eastermonth)."-".int(int($easterday) - 2);
$heb = int($eastermonth)."-".int(int($easterday) + 1);
$holiday{$hea} = '1';
$holiday{$heb} = '1';
($year,$christskytravellermonth,$christskytravellerday) = Add_Delta_YMD($yeartocheck,$eastermonth,$easterday, 0,0,39);
$chstv = int($christskytravellermonth)."-".int($christskytravellerday);
$holiday{$chstv} = '1';
if (Day_Of_Week($yeartocheck,6,19) == 5) {
$holiday{'6-19'} = '1';
}
if (Day_Of_Week($yeartocheck,6,20) == 5) {
$holiday{'6-20'} = '1';
}
if (Day_Of_Week($yeartocheck,6,21) == 5) {
$holiday{'6-21'} = '1';
}
if (Day_Of_Week($yeartocheck,6,22) == 5) {
$holiday{'6-22'} = '1';
}
if (Day_Of_Week($yeartocheck,6,23) == 5) {
$holiday{'6-23'} = '1';
}
if (Day_Of_Week($yeartocheck,6,24) == 5) {
$holiday{'6-24'} = '1';
}
if (Day_Of_Week($yeartocheck,6,25) == 5) {
$holiday{'6-25'} = '1';
}
return %holiday;
} -
Why on earth can one perform calculations on pointers in c++? I can think of a dozen ways this could go wrong, but none where this is useful.
Following example:
int t = 1234;
int tt = 5555;
int* p = &t;
int* pp = *(p + 1):
Here pp will give me 5555...5 -
Oh yeah ... Java is cool in an utterly sick way even that i can't seem to find a non-retarded built-in stack data structure
Call me a racist, but java.util.Stack has a removeIf() method in case you want to remove odd numbers:
import java.util.Stack;
public class App {
public static void main(String[] args) {
int arr[] = { 2, 4, 7, 11, 13, 16, 19 };
Stack<Integer> s = new Stack<Integer>();
for (int i = 0; i < arr.length; i++) {
s.push(arr[i]);
}
s.removeIf((n) -> (n % 2 == 1));
System.out.println(s); // [2, 4, 16]
}
}
Stop using java.util.Stack they said, a legacy class they said, instead i should use java.util.ArrayDeque, but frankly i can still keep up being racist (in a reversed manner):
import java.util.ArrayDeque;
import java.util.Deque;
public class App {
public static void main(String[] args) {
int arr[] = { 2, 4, 7, 11, 13, 16, 19 };
Deque<Integer> s = new ArrayDeque<Integer>();
for (int i = 0; i < arr.length; i++) {
s.push(arr[i]);
}
s.removeIf((n) -> (n % 2 == 1));
System.out.println(s); // [16, 4, 2]
}
}
The fact that you can iterate through java.util.Stack is amazing, but the ability to insert element in a specified index:
import java.util.Stack;
public class App {
public static void main(String[] args) {
int arr[] = { 2, 4, 7, 11, 13, 16, 19 };
Stack<Integer> s = new Stack<Integer>();
for (int i = 0; i < arr.length; i++) {
s.push(arr[i]);
}
s.add(2, 218);
System.out.println(s); // [2, 4, 218, 7, 11, 13, 16, 19]
}
}
That's what happens when you inherit java.util.Vector, which is only done a BRAIN OVEN person, a very brain oven even that it will revert to retarded
If you thought about using this type of bullshit in Java get yourself prepared to beat the disk for hours when you accidentally call java.util.Stack<T>.add(int index, T element) instead of java.util.Stack<T>.push(T element), you will probably end up breaking the disk or your hand, but not solving the issue
WHY THE F*** CAN'T WE HAVE A WORKING NORMAL STACK ?5 -
HELP
Why does gcc fails to compile?
main.c:10:1: error: unrecognizable insn:
10 | }
| ^
(insn/f 18 4 19 (set (mem:SI (pre_dec:SI (reg:SI 1 bx)) [0 S4 A32])
(reg:HI 0 ax)) "main.c":8:1 -1
(nil))
during RTL pass: shorten
main.c:10:1: internal compiler error: in insn_default_length, at insn-attrtab.c:221
0x61f93f _fatal_insn(char const*, rtx_def const*, char const*, int, char const*)
../../gcc/rtl-error.c:108
0x61f95b _fatal_insn_not_found(rtx_def const*, char const*, int, char const*)
../../gcc/rtl-error.c:116
0x742291 insn_default_length(rtx_insn*)
/home/user/Documents/gcc/build-d16i/gcc/insn-attrtab.c:221
0x9ee716 shorten_branches(rtx_insn*)
../../gcc/final.c:1118
0x9ee78f rest_of_handle_shorten_branches
../../gcc/final.c:4753
0x9ee78f execute
../../gcc/final.c:47828 -
Was working as the only frontend developer ona project having 4 "senior" developers. They use Laravel to make an API feeding the angular app.
Why the documentation sucked?
Half the API call params where missing, and not one time did I come across an example stating that the API expects a boolean only to find out 20 minutes later that they mean int 1 or 0 not true or false. Best part however was sending arrays in POST by sending the elements as comma separated values (e1,e2,e3...). Oh and not documentation but while at it a rant... There are other response codes except 200 for fucks sake -
Hi everyone, I have such a task: “Given an integer square matrix. Determine the minimum among the sums of diagonal elements parallel to the main diagonal of the matrix.” I have a code but I have problems compiling a flowchart for it, can you help me with compiling a flowchart or give tips? thanks in advance!
Thats my code:
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define N_MIN -3
#define N_MAX 5
int main(int argc, char *argv[]){
int s,i,j,k,l,s1,t2,t1;
int a[5][5];
srand(time(NULL));
for(i=0;i<5;i++){
for(j=0;j<5;j++){
a[i][j]=rand()%(N_MAX-N_MIN+1)+N_MIN;
}
}
for(i=0;i<5;i++){
for(j=0;j<5;j++){
printf("%3d ",a[i][j]);
}
printf("\n");
}
k=0;
s=0;
l=0;
for (i=0; i<5; i++){
for (j=0; j<5; j++){
if (a[i][j]>=0){
if(a[i][j]%2==0)
l+=a[i][j];
k++;
}
}
if (k==5){
l=l;
}
else {
l=0;
}
s=s+l;
k=0;
}
s1=a[0][5-1];
for(i=1; i<5; i++){
t1=t2=0;
for(j=0; j<5-i; j++){
t1+=a[i+j][j];
t2+=a[j][i+j];
}
if(t1<s1) s1=t1;
if(t2<s1) s1=t2;
}
printf("vivod %d %d\n", s,s1);
return 0;
}2 -
My question is"which value of 'a' and 'b' will give me output '1' not '0'?
C language's code is below here
#include<stdio.h>
int main()
{ float a=1.2,b=10.7;
printf("output is = %f",a&&b);
Return 0; }13 -
Function in my Dao file is:
@Query("SELECT * from appData WHERE type = :type ORDER BY id DESC")
fun getData(type: String): Flow<List<AppData>>
The database contains a column of automatically generated int primary key 'id', a column of string named 'type', and another string column named 'content'.
A Part of code from my composable function is:
val currentRetrievedDate: Date = Date()
val currentDate: String = SimpleDateFormat("dd-MM-yyyy").format(currentRetrievedDate)
var lastDateObjectList by remember { mutableStateOf(emptyList<AppData>()) }
LaunchedEffect(streakCounterViewModel) {
coroutineScope {
streakCounterViewModel.appDataRepository.getDataStream("lastDate")
.collect { newDataList ->
lastDateObjectList = newDataList as List<AppData>
}
}
}
if (lastDateObjectList.size>1){/*TODO*/
for (i in 1 until lastDateObjectList.size){
LaunchedEffect (Unit){
streakCounterViewModel.deleteData(id = lastDateObjectList[i]!!.id,
type = lastDateObjectList[i]!!.type,
content = lastDateObjectList[i]!!.content)
}
}
}
val lastDateObject: AppData?/* = lastDateObjectList[0] ?: null*/
lastDateObject = if (lastDateObjectList.isNotEmpty())
lastDateObjectList[0]
else null
var lastDate = lastDateObject?.content ?: "00-00-0000"
var currentStreakObjectList by remember { mutableStateOf(emptyList<AppData>()) }
LaunchedEffect(streakCounterViewModel) {
coroutineScope {
streakCounterViewModel.appDataRepository.getDataStream("currentStreak")
.collect { newDataList ->
currentStreakObjectList = newDataList as List<AppData>
}
}
}
if (currentStreakObjectList.size>1){
for (i in 1 until currentStreakObjectList.size){
LaunchedEffect (Unit){
streakCounterViewModel.deleteData(id = currentStreakObjectList[i]!!.id,
type = currentStreakObjectList[i]!!.type,
content = currentStreakObjectList[i]!!.content)
}
}
}
val currentStreakObject: AppData?/* = currentStreakObjectList[0] ?: null*/
currentStreakObject = if (currentStreakObjectList.isNotEmpty())
currentStreakObjectList[0]
else null
var currentStreak = currentStreakObject?.content ?: "0"
In this code, the last login time and last streak of user is already saved, we just have to fetch the data from the local database, make sure that there are not more than 1 occurrences of same data type in the database, and then use that data. But this, instead of using the database values, uses 00-00-0000 as the lastDate, and uses "0" as the currentStreak (which should only be used for the first not, and in some rare situations only), and is not able to retrieve data from the database properly.
The data saving and updating logic is working fine, but only the retrieval part is causing some issue.
The complete project is also uploaded on github: at HealthEase repo of tauqirnizami4 -
MACBOOK PRO 14.2" M3 PRO & M3 - NEW!
• M3 Pro - 12-core CPU - 18-core GPU - 18GB RAM - 1TB
SSD - INT - Space Black - 2800€
I have never seen more brutal specifications for a laptop in my life.
This 1 laptop is more expensive than 2 new iphones and 1 whole imac desktop PC. Why would someone need this?2