Join devRant
Do all the things like
++ or -- rants, post your own rants, comment on others' rants and build your customized dev avatar
Sign Up
Pipeless API
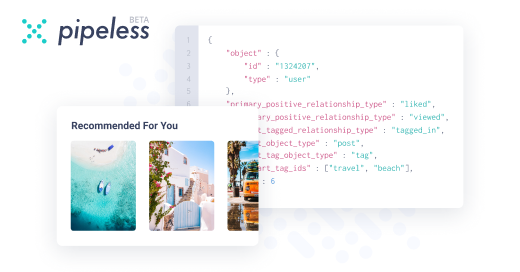
From the creators of devRant, Pipeless lets you power real-time personalized recommendations and activity feeds using a simple API
Learn More
Search - "code session"
-
At customer site with my boss.
Boss: let's check this code which is not working
Me: ok (starting the debugging session)
I found this code, which was failing during the writing on disk for some reasons.
try
{
....
writer.writeline(some data);
....
}
catch(Exception ex)
{
....
}
Boss: ok it fails to write data but we need to, let's manage it like this:
try
{
....
writer.writeline(some data);
....
}
catch(Exception ex)
{
writer.writeline(some data);
....
}12 -
My biggest dev blunder. I haven't told a single soul about this, until now.
👻👻👻👻👻👻
So, I was working as a full stack dev at a small consulting company. By this time I had about 3 years of experience and started to get pretty comfortable with my tools and the systems I worked with.
I was the person in charge of a system dealing with interactions between people in different roles. Some of this data could be sensitive in nature and users had a legal right to have data permanently removed from our system. In this case it meant remoting into the production database server and manually issuing DELETE statements against the db. Ugh.
As soon as my brain finishes processing the request to venture into that binary minefield and perform rocket surgery on that cursed database my sympathetic nervous system goes into high alert, palms sweaty. Mom's spaghetti.
Alright. Let's do this the safe way. I write the statements needed and do a test run on my machine. Works like a charm 😎
Time to get this over with. I remote into the server. I paste the code into Microsoft SQL Server Management Studio. I read through the code again and again and again. It's solid. I hit run.
....
Wait. I ran it?
....
With the IDs from my local run?
...
I stare at the confirmation message: "Nice job dude, you just deleted some stuff. Cool. See ya. - Your old pal SQL Server".
What did I just delete? What ramifications will this have? Am I sweating? My life is over. Fuck! Think, think, think.
You're a professional. Handle it like one, goddammit.
I think about doing a rollback but the server dudes are even more incompetent than me and we'd lose all the transactions that occurred after my little slip. No, that won't fly.
I do the only sensible thing: I run the statements again with the correct IDs, disconnect my remote session, and BOTTLE THAT SHIT UP FOREVER.
I tell no one. The next few days I await some kind of bug report or maybe a SWAT team. Days pass. Nothing. My anxiety slowly dissipates. That fateful day fades into oblivion and I feel confident my secret will die with me. Cool ¯\_(ツ)_/¯12 -
I've had my share of incompetent coworkers. In order of appearance:
1. A full stack dev. This one guy never, and I mean NEVER uses relationships in their tables. No indexing, no keys, nada. Couple of months later he was baffled why his page took ten seconds to load.
2. The same dev as (1). Requirement was to create some sort of "theme" feature for a web app. Hacked it by putting !important all over the place.
3. The same dev again. He creates several functions that if the data exists returns a view, and if it doesn't, "echo '0'". No, not return 0 or return false or anything, but fucking echo. This was PHP. If posted a rant about this a few months ago.
4. Same dev, has no idea what clean code is. No, not just reusable functions, he doesn't even get indenting right. Some functions have 4 spaces, some 2 tabs, some 6 tabs! And this is inside the same function. God wait until he tries Python...
5. Same dev now suggests that he become the PM. GM approves (very small company). Assigns me to travel to a client since they needed "technical assistance about the API". Was actually there to lead a UAT session.
Intermezzo, that guy went from fullstack dev to PM to sales (yes, one who calls clients to offer products) to business development, to product analyst in the span of two years.
After a year and a half there, I quit.
6. New company, a "QA engineer" who also assumes the role as the product owner. Does absolutely no tests other than "functional tests" in which he NEVER produces any form of documentation. Not even a set of test cases. He goes by "intuition".
7. Same guy as (6), hands me requirements for a feature. By "hands me" I mean he did that verbally. No spec documents, no slack chat, no Trello card. I ended up writing it as a card in Trello. Fast forward to the due date, he flips out because that wasn't what he wanted. Showed him the card. He walked away, without thinking of a solution how this mess should be handled.
Despite all this, I really don't want him (6&7) to leave the company. The devs get really stressed out at this job and he does make a really good person to laugh with/at. -
Actual pro tip: don't push only when you are done with code. Always push when you are done with coding session. Minimize the possibility of data loss and always have your code on the server.8
-
I wrote a database migration to add a column to a table and populated that column upon record creation.
But the code is so freaking convoluted that it took me four days of clawing my eyes out to manage this.
BUT IT'S FINALLY DONE.
FREAKING YAY.
Why so long, you ask? Just how convoluted could this possibly be? Follow my lead ~
There's an API to create a gift. (Possibly more; I have no bloody clue.)
I needed the mobile dev contractor to tell me which APIs he uses because there are lots of unused ones, and no reasoning to their naming, nor comments telling me what they do.
This API takes the supplied gift params, cherry-picks a few bits of useful data out (by passing both hashes by reference to several methods), replaces a couple of them with lookups / class instances (more pass-by-reference nonsense). After all of this, it logs the resulting (and very different) mess, and happily declares it the original supplied params. Utterly useless for basically everything, and so very wrong.
It then uses this data to call GiftSale#create, which returns an instance of GiftSale (that's actually a Gift; more on that soon).
GiftSale inherits from Gift, and redefines three of its methods.
GiftSale#create performs a lot of validations / data massaging, some by reference, some not. It uses `super` to call Gift#create which actually maps to the constructor Gift#initialize.
Gift#initialize calls Gift#pre_init (passing the data by reference again), which does nothing and returns null. But remember: GiftSale inherits from Gift, meaning GiftSale#pre_init supersedes Gift#pre_init, so that one is called instead. GiftSale#pre_init returns a Stripe charge object upon success, or a Gift (and a log entry containing '500 Internal') upon failure. But this is irrelevant because the return value is never actually used. Pass by reference, remember? I didn't.
We're now back at Gift#initialize, Rails finally creates a Gift object using the args modified [mostly] in-place by all of the above.
Another step back and we're at GiftSale#create again. This method returns either the shiny new Gift object or an error string (???), and the API logic branches on its type. For further confusion: not all of the method's returns are explicit, and those implicit return values are nested three levels deep. (In Ruby, a method will return the last executed line's return value automatically, allowing e.g. `def add(a,b); a+b; end`)
So, to summarize: GiftSale#create jumps back and forth between Gift five times before finally creating a Gift instance, and each jump further modifies the supplied params in-place.
Also. There are no rescue/catch blocks, meaning any issue with any of the above results in a 500. (A real 500, not a fake 500 like last time. A real 500, with tragic consequences.)
If you're having trouble following the above... yep! That's why it took FOUR FREAKING DAYS! I had no tests, no documentation, no already-built way of testing the API, and no idea what data to send it. especially considering it requires data from Stripe. It also requires an active session token + user data, and I likewise had no login API tests, documentation, logging, no idea how to create a user ... fucking hell, it's a mess.)
Also, and quite confusingly:
There's a class for GiftSale, but there's no table for it.
Gift and GiftSale are completely interchangeable except for their #create methods.
So, why does GiftSale exist?
I have no bloody idea.
All it seems to do is make everything far more complicated than it needs to be.
Anyway. My total commit?
Six lines.
IN FOUR FUCKING DAYS!
AHSKJGHALSKHGLKAHDSGJKASGH.7 -
How is it that my wireless headset becomes the name of my git bash session in VS code? Speculation welcome.8
-
> make a change
> PR gets rejected
> IHATEFORALIVING! YOUR CHANGE IS NOT WORKING! EVERYTHING BREAKS!
> 3 hours long debugging session
> We find out a whole bunch of bugs
> Suddenly, everything works
> None of the bugs had ANYTHING to do with my change. In the instances where the app broke, my code wasn't even being called at all.
> My change was literally the one and only working thing
I wish life was like in The Office, when you just stop what you're doing and you drop the Jim stare at some camera2 -
I'm editing the sidebar on one of our websites, and shuffling some entries. It involves moving some entries in/out of a dropdown and contextual sidebars, in/out of submenus, etc. It sounds a little tedious but overall pretty trivial, right?
This is day three.
I learned React+Redux from scratch (and rebuilt the latter for fun) in twice that long.
In my defense, I've been working on other tasks (see: Alerts), but mostly because I'd rather gouge my freaking eyes out than continue on this one.
Everything that could be wrong about this is. Everything that could be over-engineered is. Everything that could be written worse... can't, actually; it's awful.
Major grievances:
1) The sidebars (yes, there are several) are spread across a ridiculous number of folders. I stopped counting at 20.
2) Instead of icon fonts, this uses multiple images for entry states.
3) The image filenames don't match the menu entry names. at all. ("sb_gifts.png" -> orders); active filenames are e.g. "sb_giftsactive.png"
4) The actions don't match the menu entry names.
5) Menu state is handled within the root application controller, and doesn't use bools, but strings. (and these state flags never seem to get reset anywhere...)
6) These strings are used to construct the image filenames within the sidebar views/partials.
7) Sometimes access restrictions (employee, manager, etc.) are around the individual menu entries, sometimes they're around a partial include, meaning it's extremely difficult to determine which menu entries/sections/subsections are permission-locked without digging through everything.
8) Within different conditionals there are duplicate blocks markup, with duplicate includes, that end up render different partials/markup due to different state.
9) There are parent tags outside of includes, such as `<ul>#{render 'horrific-eye-stabbing'}</ul>`
10) The markup differs per location: sometimes it's a huge blob of non-semantic filthiness, sometimes it's a simple div+span. Example filth: section->p->a->(img,span) ... per menu entry.
11) In some places, the markup is broken, e.g. `<li><u>...</li></u>`
12) In other places, markup is used for layout adjustments, such as an single nested within several divs adorned with lots of styles/classes.
13) Per-device layouts are handled, not within separate views, but by conditionally enabling/disabling swaths of markup, e.g. (if is_cordova_session?).
14) `is_cordova_session` in particular is stored within a cookie that does not expire, and within your user session. disabling it is annoying and very non-obvious. It can get set whether or not you're using cordova.
15) There are virtually no stylesheets; almost everything is inline (but of course not actually everything), which makes for fun layout debugging.
16) Some of the markup (with inline styling, no less) is generated within a goddamn controller.
17) The markup does use css classes, but it's predominately not for actual styling: they're used to pick out elements within unit tests. An example class name: "hide-for-medium-down"; and no, I can't figure out what it means, even when looking at the tests that use it. There are no styles attached to that particular class.
18) The tests have not been updated for three years, and that last update was an rspec version bump.
19) Mixed tabs and spaces, with mixed indentation level (given spaces, it's sometimes 2, 4, 4, 5, or 6, and sometimes one of those levels consistently, plus an extra space thereafter.)
20) Intentional assignment within conditionals (`if var=possibly_nil_return_value()`)
21) hardcoded (and occasionally incorrect) values/urls.
... and last but not least:
22) Adding a new "menu sections unit" (I still haven't determined what the crap that means) requires changing two constants and writing a goddamn database migration.
I'm not even including minor annoyances like non-enclosed ternaries, poor naming conventions, commented out code, highly inefficient code, a 512-character regex (at least it's even, right?), etc.
just.
what the _fuck_
Who knew a sidebar could be so utterly convoluted?6 -
Thus far I've had little success trying to teach code to people I know.
But yesterday.....man
My best friend told me that he was serious about learning. He asked me about my recommendation would be. Against all odds, and after small demonstrations he asked to learn about Java.
I made some coffee and braced myself. Either the dude is a hidden genius or I am an amazing instructor, but he got the whole thing. I introduced him to the basics, oop, variables etc and he got it down in one session. He was able to understand errthing a do a little code along.
So proud.13 -
There was a time I made an update on one of our client's e-commerce website sign-up page. The update caused a bug that allowed new users to create an account without actually creating an account.
The code block meant to save user credentials (i.e email address and password) to the database was commented out for some reasons I still can't remember to this day. After registration new users had their session created just as normal but in reality they have no recorded account on the platform. This shit went on like this for a whole week affecting over 350 new customers before the devil sent me a DM.
I got a call from my boss on that weekend that some users who had made purchases recently can't access their account from a different device and cannot also update their password. Nobody likes duty calls on a weekend, I grudgingly and sluggishly opened up my PC to create a quick fix but when I saw what the problem was I shut down my PC immediately, I ran into the shower like I was being chased by a ghost, I kept screaming "what tha fuck! what tha fuck!!" cus I knew hell was about to break loose.
At that moment everything seemed off as if I could feel everything, I felt the water dripping down my spine, I could hear the tiniest of sound. I thought about the 350 new customers the client just lost, I imagined the raving anger on the face of my boss, I thought about how dumb my colleagues would think I was for such a stupid long running bug.
I wondered through all possible solutions that could save me from this embarrassment.
-- "If this shitty client would have just allowed us verify users email before usage things wouldn't have gotten to this extent"
-- "Should I call the customers to get their email address using their provided telephone?... No they'd think I'm a scammer"
-- "Should I tell my boss the database was hacked? Pffft hack my a**",
-- "Should I create a page for the affected users to re-verify their email address and password? No, some sessions may have expired"
-- "Or maybe this the best time to quit this f*ckn job!"
... Different thoughts from all four corners of the bathroom made it a really long bath. Finally, I decided it was best I told my boss what had happened. So I fixed the code, called my boss the next day and explained the situation on ground to him and yes he was furious. "What a silly mistake..!" he raged and raged. See me in my office by Monday.
That night felt longer than usual, I couldn't sleep properly. I felt pity for the client and I blamed it all on myself... yeah the "silly mistake", I could have been more careful.
Monday came boss wasn't at the office, Tuesday, Wednesday, Thursday, Friday not available. Next week he was around and when we both met the discussion was about a different project. I tried briefing him about last week incident, he seems not to recall and demands we focus on the current project.
However, over three hundred and fifty customers swept under the carpet courtesy of me. I still felt the guilt of that f*ck up till this day.1 -
Let me explain a tiny corner of some awful code I read earlier today, in layman’s terms.
It’s a method to see if the user is in a secure session — not to set up the session, just to see if it exists. The method ends with a question mark, so it’s basically a question. It should look up the info (without changing anything) and should always give a clear yes/no answer. Makes sense, right?
Let’s say the question is “am I in school right now?”
The code… well.
If there isn’t a student, the answer it gives is null, not yes or no. Null is a fancy word for no, pretty much, so that’s kinda fine, but it really should be a simple no.
It then checks to see if the school is open today. If it is open, it then checks to see if I made my lunch, if I took my backpack, and if I rode the bus — and makes these things happen if they didn’t. Forgot my backpack? Just ask “am I in school today?” And poof! There’s my backpack! … but only if the school is open.
It then, finally, checks to see if I’m actually in the school, and gives that answer.
It could just see if I’m in the school — I mean, I could be in school without a backpack, or walked there on the weekend, right? Ha! You and your silly logic have no place here.
So, by asking if the user is in a secure session, we change the answer: they weren’t before, but the act of asking makes it so. This isn’t profound or anything: I don’t work with Schrödinger. My coworkers are just idiots.
And no, the rest of the code isn’t any better…7 -
Ticket: “feature [x] doesn’t work”
Me: “I’ll need more details: how do I reach feature [x]? In which of the three projects you assigned me is that?”
Manager: “the design is in the ticket”
Me, in my head: “can you effin listen to what I told you before giving air to your mouth?”
Me, in person: “yeah I just need to know which project this refers to and how to reach it”
Manager: “but you have to open the ticket as a separate page!”
Me: “sure!” *waits 15 min, opens a ticket for more details, assigns it to manager, flags as blocking, flags the other one as blocked*
5 mins later: details are given and I can proudly fox it by remembering the manager they have to login in order to see feature [x]
Later in the workweek:
Manager at 8:00 URGENT FEATURE! MUST BE DELIVERED BY EOD
Me, 10:00 “can jump on it, need authorisation for [a procedure]
Me, 11,12,13,15,16,17:30: pings for an answer
Manager, 17:58 “ah sorry didn’t see, we can do it tomorrow”
Is this the matrix? Am I being stopped from developing cause I am randomly accessing matrix’s code without knowing it? Is this the Truman show? And most importantly: can I please take part to a manager hiring session? I am curious to see how tf you hire such peculiar people.10 -
I've found and fixed any kind of "bad bug" I can think of over my career from allowing negative financial transfers to weird platform specific behaviour, here are a few of the more interesting ones that come to mind...
#1 - Most expensive lesson learned
Almost 10 years ago (while learning to code) I wrote a loyalty card system that ended up going national. Fast forward 2 years and by some miracle the system still worked and had services running on 500+ POS servers in large retail stores uploading thousands of transactions each second - due to this increased traffic to stay ahead of any trouble we decided to add a loadbalancer to our backend.
This was simply a matter of re-assigning the IP and would cause 10-15 minutes of downtime (for the first time ever), we made the switch and everything seemed perfect. Too perfect...
After 10 minutes every phone in the office started going beserk - calls where coming in about store servers irreparably crashing all over the country taking all the tills offline and forcing them to close doors midday. It was bad and we couldn't conceive how it could possibly be us or our software to blame.
Turns out we made the local service write any web service errors to a log file upon failure for debugging purposes before retrying - a perfectly sensible thing to do if I hadn't forgotten to check the size of or clear the log file. In about 15 minutes of downtime each stores error log proceeded to grow and consume every available byte of HD space before crashing windows.
#2 - Hardest to find
This was a true "Nessie" bug.. We had a single codebase powering a few hundred sites. Every now and then at some point the web server would spontaneously die and vommit a bunch of sql statements and sensitive data back to the user causing huge concern but I could never remotely replicate the behaviour - until 4 years later it happened to one of our support staff and I could pull out their network & session info.
Turns out years back when the server was first setup each domain was added as an individual "Site" on IIS but shared the same root directory and hence the same session path. It would have remained unnoticed if we had not grown but as our traffic increased ever so often 2 users of different sites would end up sharing a session id causing the server to promptly implode on itself.
#3 - Most elegant fix
Same bastard IIS server as #2. Codebase was the most unsecure unstable travesty I've ever worked with - sql injection vuns in EVERY URL, sql statements stored in COOKIES... this thing was irreparably fucked up but had to stay online until it could be replaced. Basically every other day it got hit by bots ended up sending bluepill spam or mining shitcoin and I would simply delete the instance and recreate it in a semi un-compromised state which was an acceptable solution for the business for uptime... until we we're DDOS'ed for 5 days straight.
My hands were tied and there was no way to mitigate it except for stopping individual sites as they came under attack and starting them after it subsided... (for some reason they seemed to be targeting by domain instead of ip). After 3 days of doing this manually I was given the go ahead to use any resources necessary to make it stop and especially since it was IIS6 I had no fucking clue where to start.
So I stuck to what I knew and deployed a $5 vm running an Nginx reverse proxy with heavy caching and rate limiting linked to a custom fail2ban plugin in in front of the insecure server. The attacks died instantly, the server sped up 10x and was never compromised by bots again (presumably since they got back a linux user agent). To this day I marvel at this miracle $5 fix.1 -
Try pair programming! I've never had an official pair programming session, but I'll be damned if my best code and quickest solutions didn't come from having a bitch session with my coworker. Just having to talk it out or having that second set of eyes can work wonders. Plus you have a built-in de-stressing outlet to help you sleep at night.3
-
We have a developer that is known for rejecting PR during code reviews.
He sent me a message and asked me to come to his desk to discuss my PR.
He mentioned that he didn't like my solution and suggested to rewrite the code together.
So far so good, he is a senior developer and I'm sure I'll pick something from the pair programming session. He went with his approach and faced some issues that led us to my solution after nearly 2 hours.
I'm not angry because this scenario happened at least 3 times but how do you guys deal with senior developers that are stubborn?7 -
I just love refactoring :) that feeling when an agonic 50loc method with ifs, loops, streams, other shit shrinks down to 3 lines with descriptive and SRP-compliant method calls.. When you can actually read code as a nicely written story. When there are no rubbish comments, cryptic variables and no overly complex if-else skyscrapers jamming all the logic in one conditional chain. When all the abstractions are designed so nicely and design patterns applied so perfectly that extending either of the components is as easy as a walk in a park.
When everything is nice and neat. Only then can I sleep well and enjoy the autumn :)
just some random thoughts after today's coding session :)5 -
Okay guys, this is it!
Today was my final day at my current employer. I am on vacation next week, and will return to my previous employer on January the 2nd.
So I am going back to full time C/C++ coding on Linux. My machines will, once again, all have Gentoo Linux on them, while the servers run Debian. (Or Devuan if I can help it.)
----------------------------------------------------------------
So what have I learned in my 15 months stint as a C++ Qt5 developer on Windows 10 using Visual Studio 2017?
1. VS2017 is the best ever.
Although I am a Linux guy, I have owned all Visual C++/Studio versions since Visual C++ 6 (1999) - if only to use for cross-platform projects in a Windows VM.
2. I love Qt5, even on Windows!
And QtDesigner is a far better tool than I thought. On Linux I rarely had to design GUIs, so I was happily surprised.
3. GUI apps are always inferior to CLI.
Whenever a collegue of mine and me had worked on the same parts in the same libraries, and hit the inevitable merge conflict resolving session, we played a game: Who would push first? Him, with TortoiseGit and BeyondCompare? Or me, with MinTTY and kdiff3?
Surprise! I always won! 😁
4. Only shortly into Application Development for Windows with Visual Studio, I started to miss the fun it is to code on Linux for Linux.
No matter how much I like VS2017, I really miss Code::Blocks!
5. Big software suites (2,792 files) are interesting, but I prefer libraries and frameworks to work on.
----------------------------------------------------------------
For future reference, I'll answer a possible question I may have in the future about Windows 10: What did I use to mod/pimp it?
1. 7+ Taskbar Tweaker
https://rammichael.com/7-taskbar-tw...
2. AeroGlass
http://www.glass8.eu/
3. Classic Start (Now: Open-Shell-Menu)
https://github.com/Open-Shell/...
4. f.lux
https://justgetflux.com/
5. ImDisk
https://sourceforge.net/projects/...
6. Kate
Enhanced text editor I like a lot more than notepad++. Aaaand it has a "vim-mode". 👍
https://kate-editor.org/
7. kdiff3
Three way diff viewer, that can resolve most merge conflicts on its own. Its keyboard shortcuts (ctrl-1|2|3 ; ctrl-PgDn) let you fly through your files.
http://kdiff3.sourceforge.net/
8. Link Shell Extensions
Support hard links, symbolic links, junctions and much more right from the explorer via right-click-menu.
http://schinagl.priv.at/nt/...
9. Rainmeter
Neither as beautiful as Conky, nor as easy to configure or flexible. But it does its job.
https://www.rainmeter.net/
10 WinAeroTweaker
https://winaero.com/comment.php/...
Of course this wasn't everything. I also pimped Visual Studio quite heavily. Sam question from my future self: What did I do?
1 AStyle Extension
https://marketplace.visualstudio.com/...
2 Better Comments
Simple patche to make different comment styles look different. Like obsolete ones being showed striked through, or important ones in bold red and such stuff.
https://marketplace.visualstudio.com/...
3 CodeMaid
Open Source AddOn to clean up source code. Supports C#, C++, F#, VB, PHP, PowerShell, R, JSON, XAML, XML, ASP, HTML, CSS, LESS, SCSS, JavaScript and TypeScript.
http://www.codemaid.net/
4 Atomineer Pro Documentation
Alright, it is commercial. But there is not another tool that can keep doxygen style comments updated. Without this, you have to do it by hand.
https://www.atomineerutils.com/
5 Highlight all occurrences of selected word++
Select a word, and all similar get highlighted. VS could do this on its own, but is restricted to keywords.
https://marketplace.visualstudio.com/...
6 Hot Commands for Visual Studio
https://marketplace.visualstudio.com/...
7 Viasfora
This ingenious invention colorizes brackets (aka "Rainbow brackets") and makes their inner space visible on demand. Very useful if you have to deal with complex flows.
https://viasfora.com/
8 VSColorOutput
Come on! 2018 and Visual Studio still outputs monochromatically?
http://mike-ward.net/vscoloroutput/
That's it, folks.
----------------------------------------------------------------
No matter how much fun it will be to do full time Linux C/C++ coding, and reverse engineering of WORM file systems and proprietary containers and databases, the thing I am most looking forward to is quite mundane: I can do what the fuck I want!
Being stuck in a project? No problem, any of my own projects is just a 'git clone' away. (Or fetch/pull more likely... 😜)
Here I am leaving a place where gitlab.com, github.com and sourceforge.net are blocked.
But I will also miss my collegues here. I know it.
Well, part of the game I guess?7 -
Well... I had in over 15 years of programming a lot of PHP / HTML projects where I asked myself: What psychopath could have written this?
(PHP haters: Just go trolling somewhere else...)
In my current project I've "inherited" a project which was running around ~ 15 years. Code Base looked solid to me... (Article system for ERP, huge company / branches system, lot of other modules for internal use... All in all: Not small.)
The original goal was to port to PHP 7 and to give it a fresh layout. Seemed doable...
The first days passed by - porting to an asset system, cleaning up the base system (login / logout / session & cookies... you know the drill).
And that was where it all went haywire.
I really have no clue how someone could have been so ignorant to not even think twice before setting cookies or doing other "header related" stuff without at least checking the result codes...
Basically the authentication / permission system was fully fucked up. It relied on redirecting the user via header modification to the login page with an error set in a GET variable...
Uh boy. That ain't funny.
Ported to session flash messages, checked if headers were sent, hard exit otherwise - redirect.
But then I got to the first layers of the whole "OOP class" related shit...
It's basically "whack a mole".
Whoever wrote this, was as dumb and as ignorant to build up a daisy chain of commands for fixing corner cases of corner cases of the regular command... If you don't understand what I mean, take the following example:
Permissions are based on group (accumulation of single permissions) and single permissions - to get all permissions from a user, you need to fetch both and build a unique array.
Well... The "names" for permissions are not unique. I'd never expected to be someone to be so stupid. Yes. You could have two permissions name "article_search" - while relying on uniqueness.
All in all all permissions are fetched once for lifetime of script and stored to a cache...
To fix this corner case… There is another function that fetches the results from the cache and returns simply "one" of the rights (getting permission array).
In case you need to get the ID of the other (yes... two identifiers used in the project for permissions - name and ID (auto increment key))...
Let's write another function on top of the function on top of the function.
My brain is seriously in deep fried mode.
Untangling this mess is basically like getting pumped up with pain killers and trying to solve logic riddles - it just doesn't work....
So... From redesigning and porting from PHP 7 I'm basically rewriting the whole base system to MVC, porting and touching every script, untangling this dumb shit of "functions" / "OOP" [or whatever you call this garbage] and then hoping everything works...
A huge thanks to AURA. http://auraphp.com/
It's incredibily useful in this case, as it has no dependencies and makes it very easy to get a solid ground without writing a whole framework by myself.
Amen.2 -
Reasons 1 and 2 arent that important to me. The main reason I code is #3.
1) Brain exercise. I always feel sharp after a coding session, even if it ended in disaster.
2) Lots to do! There's never a full day in code. Make your own universe, if you so desire.
3) Pride. I have a pride problem. I never felt proud of myself no matter what I do. I graduated with a melancholy feeling, same deal when getting my license, same deal when passing a test (God, glad that's over!)... But code makes me proud. I love what I make. I want to show everyone. I want to show it to everyone before it's even finished because I just can't wait. I want everyone to use it and to love it. Because I sure do, and it's the best thing ever.
I could make a viral video, produce a triple platinum record, or build a billion dollar business and still not feel the same level of genuine satisfaction and happiness that I may get from writing good code.
It always keeps me coming back. -
My son just flooded my apartment for the third time. Why a rant here, you would ask? Because he filled the place with water because I can't get my head out of my code, or, in this case, of a debugging session chasing a Crashlytics issue. It is an old apartment, and a previous tenant "fixed" a drainpipe on the bathroom so well that if you take a long bath, it just collapses and starts pouring water all over the place from a mesh strainer. I sent the kid to take a bath, thinking "I'll just take a look to this issue and then take him out in a few minutes". An hour and a half later...2
-
Me after a long coding session with a well prepared working flow: I am such a great computer scientist, I can conquer the world.
Right after that I found a repository for computer science papers and got immidiately hooked. Well, the level of knowledge and theory is so immense that it brought me back to ground of reality again: I know so little that it is almost ridiculous, even if I read and code 16 hours a day I may never understand computer science as a whole.
Le me sad.11 -
brain: ABSTRACTION ABSTRACTION ABSTRACTION too much ABSTRACTION!
me: jeez calm down a lil i just deployed a boilerplate ember web app with cli tools with next to nothing amount of 'my' code.
b: YES U SUCKER THAT'S WHAT WENT WRONG U DON'T KNOW SHIT ABOUT THE LIL STUFF THAT HAPPENS BEHIND THE SCENES THE FUCK MAN U CALL YOURSELF A CS STUDENT YOU CAN'T EVEN WRITE A COMPILER YET
m: sooo remember when we were studying logic gates and binary conversions and you sigkilled all my threads cuz it was 'boring'?
b: why yes why do you ask
m: WELL that's where we'll end up again if you don't stop nagging me about going down. Trust me, I KNOW how to starve you and you'll beg me to use Python again. You start making advanced data structures in C and the next thing you know you're writing assembly code 'just for fun'.
I have a hackathon coming right up and I have to use a framework or my team loses the advantage. Are we good?
b: well if you put it that way...BUT AFTER THAT YOU'RE TAKING ME TO AN ALGORITHM SESSION
m: *eerily stares at the dusty book in the corner*
you... have a deal3 -
I’m trying to add digit separators to a few amount fields. There’s actually three tickets to do this in various places, and I’m working on the last of them.
I had a nightmare debugging session earlier where literally everything would 404 unless I navigated through the site in a very roundabout way. I never did figure out the cause, but I found a viable workaround. Basically: the house doesn’t exist if you use the front door, but it’s fine if you go through the garden gate, around the back, and crawl in through the side window. After hours of debugging I eventually discovered that if I unlocked the front door with a different key, everything was fine… but nobody else has this problem?
Whatever.
Onto the problem at hand!
I’m trying to add digit separators to some values. I found a way to navigate to the page in question (more difficult than it sounds), and … I don’t know what view is rendering the page. Or what controller. Or how it generates its text.
The URL is encrypted, so I get no clues there. (Which was lead dev’s solution to having scrapeable IDs instead of just, you know, fixing them). The encryption also happens in middleware, so it’s a nightmare to work through. And it’s by the lead dev, so the code is fucking atrocious.
The view… could be one of many, and I don’t even know where they are. Or what layout. Or what partials go into building it.
All of the text on the page are “resources” — think named translations that support plus nested macros. I don’t know their names, and the bits of text I can search for are used fucking everywhere. “Confirmation number” (the most unique of them) turns up 79 matches. “Fee” showed up in 8310 places before my editor gave up looking. Really.
The table displaying the data, which is what I actually care about, isn’t built in JS or markup, but is likely a resource that goes through heavy processing. It gets generated in a controller somewhere (I don’t know the resource name so I can’t find it), and passed through several layers of “dynamic form” abstraction, eventually turned into markup, and rendered as a partial template. At least, that’s how it worked in the previous ticket. I found a resource that looks right, and there’s only the one. I found the nested macros it uses for the amount and total, and added the separators there… only to find that it doesn’t work.
Fucking dead end.
And i have absolutely nothing else to go on.
Page title? “Show”
URL? /~LiolV8N8KrIgaozEgLv93s…
Text? All from macros with unknown names. Can’t really search for it without considerable effort.
Table? Doesn’t work.
Text in the table? doesn’t turn up anything new.
Legal agreement? There are multiple, used in many places, generates them dynamically via (of course) resources, and even looking through the method usages, doesn’t narrow it down very much.
Just.
What the fuck?
Why does this need to be so fucking complicated?
And what genius decided “$100000.00” doesn’t need separators? Right, the lot of them because separators aren’t used ANYWHERE but in code I authored. Like, really? This is fintech. You’d think they would be ubiquitous.
And the sheer amount of abstraction?
Stupid stupid stupid stupid stupid.11 -
Well on my last full-time job, that ware using cookies for authentication (not something new, eh?). The thing is, you see, the cookies had the 'accountId' which if you change to another number, kaboom you're that account, oh but that was not all, there was an option to mark the account type in there 'accountType', which was kind of obvious in VLE (virtual learning environment), 'Teacher', 'Student', 'Manager' put what of those values and boom you are that role for the session
Thing was open of SQL injection from the login form, from said cookies and form every part you can pass input to it, when I raised the question to my TL he said 'no one is going to know about thatt, I don't see what is the problem', then escalated to higher management 'oh well speak to *tl_guy*'
Oh and bonus points for it being written in ASP CLASSIC in 2014+ (I was supposed to rewrite, but ended up patching ASP code and writing components in PHP)
In 2015-2016, in a private college, charging kind-of big money per year1 -
*WanBLowS shits itself as usual in BSOD*
FEATUREFUL FUCKING JOKE OF AN OPERATING SYSTEM..!!!! How about you do the only thing that you're good at - casual shit like letting me watch a fucking anime! - and do it properly?! Yes there's an rsync from btrfs to btrfs going on in the background - because yes I fucking detest your joke of a filesystem called NTFS!! Should that even matter?! ONE FUCKING JOB!!!
Meanwhile my tablet, a fucking €120 cheapie!! It can stay up and running - stable! - for fucking weeks in a row, only taken down by me forgetting to charge the bloody thing every few days. But yeah it's gotta be a hardware issue, it's gotta be an obscure setup. NO IT'S A FUCKING CRAPTACULAR SHIT OS!!! If only those Microshit certified enganeers would write a goddamn line of DECENT CODE!!!
(As for anyone who doesn't know already that I've tried countless times to convert this turd to Linux.. It's an Intel + Nvidia GPU hybrid and it doesn't even boot a Linux live session. Believe me, I've tried.)7 -
Took a class on computer systems, was supposed to be taught instruction sets, interrupts, pointers, basic gate level digital logic, etc. Professor spent the whole semester going line by line in the Visual Studio disassembler for some arbitrary C code, explaining in painfully dull detail, what each assembly line did. They did this for every class session, including the first, with no introduction to assembly. I lost count of how many times I fell asleep in class.
-
Soooo it's Monday........ 🤯
@C0D4 started the day fixing current projects defects (4 tickets smashed before coffee 💪)
Then after coffee, run a test coverage report and see a significant decline over the past few months, so spends a couple hours adding more tests to get some areas filled in - meh, nothing like 50+ lines per test... to test a if() statement but whatever - complex scenarios will be complex to get too, but no my tests break and I'm missing data I didn't know about🤦♂️
So let's comment all that out, and go to lunch ... mmmm lunch.
Get back, start working on those again, and then get handed a new issue, so comment that all back out again, ( ok I know what you're thinking, but I'm working in an environment that does not use git for deployments - don't ask, real pain in the ass I haven't had time to invest into yet - but as code versioning only) anywho, starts to workout this new issue but don't figure it out, enter a 30 minute meeting.................. yea that was 2 hours later but was a very practical whiteboard session only to work out I have something like 16-20 weeks of work over 4-5 projects to get out in like 6 weeks... hahahahahahaha fml..... oh and that's excluding another project which had a 6 weeks of work in the pipeline to get to somehow.... I'm not seeing this one happening, and probably conflicting projects needed on top of that down the track... but we'll leave those out for now!
Whoot is fucking home time!!!
🤷♂️I'm starting to think I'm like a team of 5-10 devs right now, maybe I should start asking for 5-10x more 😏
#letsBringOnTuesday!!!!4 -
> See unintelligible error from stuff that used to work and now it doesn't
> Ask colleagues if they ever met the error
> Fucktard colleague says "try using the debugger, if you run the code line by line you may probably understand what's wrong"
Dear Fucktard, I've been writing code for 7 years now, you can safely assume I know how to use a debugger if need be.
The thing: when I ask "have you ever met this error" I'm asking if you actually know what's going on, BEFORE diving in a 3 hours long debugging session of code written 6 years ago by people who have left the company in the meantime.
You don't always have to say something. Sometimes it happens: you may not have anything clever to add, and a simple "I'm sorry I don't know" is perfectly fine.1 -
My head is melting. Does anyone have a colleague who constantly complains about missing specs, documentation, project organization, bad processes and procedures? Everything needs to be planned. Not a single small code change can be done without reviewed details. 10min job becomes a week-long session of whining and dabbling.
You give the guy a small task and at the end of the day nothing is done. Just page after page of written documents and lists in Word and online notebooks. Version numbers, meaningless measurement results, latencies etc. And all you asked was "could you just fucking fix this one thing and quickly compile and check it". But no. There must be a review and at least 10 people need to be called into conference. Someone needs to approve everything just so that he can later move to blame to others. "Yeah I know it's not working but I showed you the code and you reviewed it!". Yes, you did, but other people have work of their own so sometimes you need to tie your own shoelaces.
And sometimes finally there's some work done. All indentations are shit. There’re code changes everywhere just because the guy didn't like the previous smaller, compact and logical code. The code doesn't even compile properly anymore. And if you complain, the reason is "there's no proper reviewed and stamped process description, so I cannot know if a variable is supposed to be 10 characters long. Besides 200 character long variable names are much more descriptive". For fucks sake.
Some coders should've gone to work in some tax office basement.9 -
Gj Mastercard! My card just got blocked because every time I want pay and 3D secure code is needed, every first SMS that day is delayed by 5 minutes so session expires and I have to try again...now it happened quite a few times and card got blocked. Fucking shit...2
-
So one of my clients got their wordpress site hacked and basically just redirects to scam links and well.. I looked at in the server file manager and their are like three directories with this wordpress site (not clones but the same?) one in the root, a version in a folder called old and another in temp.. with 3 separate wp databases.. DNS entries had malware redirects, the wp-content folder was writable to the public and contained a temp folder with tons of encoded malware and ip links to malicious sites.. there was encoded malware in index.php, has like 20+ plugins, oh and the theme uses a dynamic web builder so the code is basically unreadable in source and scattered.. and the redirects seem to happen randomly or at least on a new session or something. Oh.. and did I mention there are no backups? 😃2
-
Just went out from a 12+ hours session of debugging.
After removing bits of code until there was almost nothing left, sweeping through StackOverflow, step-debugging into thousand-lines framework source code, running tests, considering filing a bug to Android...
turns out I had a
getFragmentManager()
that should actually have been
getChildFragmentManager().1 -
My designer just had an user interview where the user is a developer and my designer showed him the mock-ups of a no code tool that we are building, asking the dev for his input.
She literally had a session with a guy announcing him that we are building a tool that will put him out of work and moreover asked him for inputs so that we miss no use case.
And in another story, one of my dev lead decided to decommission an entire feature and replace it will a hacky solution because the devs in her team were not comfortable using the current design in their development stage. Hence, without user research, any strong use case, or considering business implications, she went ahead and drafted the entire approach on how to fuck everyone.
I am out of my honeymoon phase at my new org and I am scared. Shit scared.16 -
I hate lying customers.
Today a customer opened a support ticket related to his website account. Apparently he is losing his session right after the login success.
I've debugged everything, checked all logs and couldn't reproduce it.
I know every bit of business logic on the website by heart.
The only explanation could be that his browser either doesn't allow cookies or expires them after page change.
So I asked him to check.
"Yes, cookies are allowed in my browser" he wrote.
Well... fuck me... I will change the code to put the session ID in the URL as well. If it works - and I'm 100% sure of that - I will personally mail him a collection of the finest turds.4 -
I am a senior Android dev, and I have an old colleague (iOS senior dev). We work on the same project, but in every estimation session he pushes a lot on the lower side: he estimates 4h a task that normally takes 6h or 8h, and the reason is that he has no social life. Right after work he starts working again from home (I can see all his commits), he also works almost entire weekends. I would say he works as average 12/13 h per day.
I don t want to work extra time (unpayed).
About him, it is his life, so I don t care...but at the same time this makes me pressure. I care a lot about quality of code, and I don t want to sacrifice it just for catching up. Most of the people in the team know that he works a lot extra time.
How would you handle this?28 -
Whelp. I started making a very simple website with a single-page design, which I intended to use for managing my own personal knowledge on a particular subject matter, with some basic categorization features and a simple rich text editor for entering data. Partly as an exercise in web development, and partly due to not being happy with existing options out there. All was going well...
...and then feature creep happened. Now I have implemented support for multiple users with different access levels; user profiles; encrypted login system (and encrypted cookies that contain no sensitive data lol) and session handling according to (perceived) best practices; secure password recovery; user-management interface for admins; public, private and group-based sections with multiple categories and posts in each category that can be sorted by sort order value or drag and drop; custom user-created groups where they can give other users access to their sections; notifications; context menus for everything; post & user flagging system, moderation queue and support system; post revisions with comparison between different revisions; support for mobile devices and touch/swipe gestures to open/close menus or navigate between posts; easily extendible css themes with two different dark themes and one ugly as heck light theme; lazy loading of images in posts that won't load until you actually open them; auto-saving of posts in case of browser crash or accidental navigation away from page; plus various other small stuff like syntax highlighting for code, internal post linking, favouriting of posts, free-text filter, no-javascript mode, invitation system, secure (yeah right) image uploading, post-locking...
On my TODO-list: Comment and/or upvote system, spoiler tag, GDPR compliance (if I ever launch it haha), data-limits, a simple user action log for admins/moderators, overall improved security measures, refactor various controllers, clean up the code...
It STILL uses a single-page design, and the amount of feature requests (and bugs) added to my Trello board increases exponentially with every passing week. No other living person has seen the website yet, and at the pace I'm going, humanity will have gone through at least one major extinction event before I consider it "done" enough to show anyone.
help4 -
When you've spent hours debugging a problem of session not being saved and there is no error. And then you find this in the code:
try{
save_session();
}catch(Exception $e){}// added by another member of the team
🙈😖🖕🏻6 -
Fuck Unity.
Every single time I try to use Unity to develop my well-along-in-development video game, it finds some way of fucking itself up.
Be it from somehow failing to compile a DLL - which is something completely out of my control, the inspector failing to update itself when I select a new object every five minutes, to the engine managing to fail to load its UI layout because it somehow managed to lose a file responsible for containing the layout, the Inspector forgetting to include a scrollbar and as such trying to cram a bunch of components into one area, crashing in a certain area because I tried using reflections, crashing because I tried running the game in a place that always works, all the way to the whole thing closing instantaneously when I try selecting a new layout.
My experience with using this god-forsaken configuration of code and imagery has been one of endless torment; I've spent hours lamenting about the pain this piece of utter horseshit has caused me to those who'd listen.
I don't know what I did to this thing to deserve to be shown the absolute worst of this engine for the year I've been working on my game for. I can't even take a look at its source code to see if I can piece together things I'll pick up from alien code to fix obnoxious bugs myself because you cunts have it under lock-and-key for some dumbass reason.
Even updating my install of this engine is a gamble; I remember clear-as-day updating my project from 2019.3.14 to whichever one was most recent at the time, and everything breaking. This time, I got lucky and managed to update to 2020.1.4 with no issue on the surface, except I inadvertently let in a host of other issues that somehow made the editor worse than the older one.
There's little point in even bothering to report a bug because this shit happens so randomly that I could be just working on auto-pilot and the next thing I know Unity's stupid "crash handler" rears its ugly head yet again, or you people are probably too busy adding support for platforms no sane person uses like fucking Chromebooks.
There've been times where it's crashed upwards of three times in the span of 40 minutes of light use.
How is one expected to cough up hundreds of dollars a year to use a "pro" version of this horrid editor when every session of use yields a 50/50 chance that it'll either work like it's supposed to, or break in one way or another?
It's a miracle I even managed to type all of this out in one go, I expected the website to just stop responding entirely once I got past four lines.
Do what you will with my post, I don't care.6 -
Just came back from vaccation yesterday. During sprint retrospective today I hear my team was having trouble dealing with the API layer (which was mostly written by me). Suggestion was a session where I sit and explain the application to the team ,which I have no problem with.
One of my teammates asserts that it's written in such a way that "only the person who wrote it can modify it".
Agree to disagree but whatever. This thing goes through code review everytime I push changes to it. If there was a problem I don't know why he's just discovering it 6 months into the project. I assure you there's no rocket surgery going on. The problem is that I have been doing everything on that side of the project and nobody was curious enough to give it a read sometime. In fact I dont think anything needed to change while I was on vacation, they just didn't have me to troubleshoot every problem for them like usual 😤 -
Best part of working in Company:
Getting learning sessions from Seniors and sharing design aspects and their pros and cons.
Had an awesome session on how to focus on making a code testable.
With hands on coding too.
Never expected to have such a great experience. -
Arrrgggghhh!!
How the fuck do you stay focused on one task?!
Writing code, is like browsing YouTube for me. I begin by searching for a Hibernate tutorial, and end, 3 hours later watching a video of a cat chasing a dog up a water slide.
How do you stay focused on the task of writing the specific class, method, package you set out to achieve when you sat down for that session??4 -
Got back home from my last therapy session. Situation was kind of strange. I had only done about two sessions but my therapist told me they wouldn't be a good fit for me as I have a pretty good grasp of what my issues are and what I want to do to fix them.
Thing is, I'm an introverted person. And I work with people who are much more extroverted than me. And that's not really surprising, most Americans are to a fault extremely extroverted and it drives me nuts.
I hate their gas guzzling trucks and suv's that haul almost nothing so they can go to Walmart to buy shit they don't need. I hate our advertising with it's whoring to the public. I hate our media for being shallow as fuck. I hate our politicians who whore out capitalism to the lowest fucking bidder to get elected.
I do like some American companies though, and we have a lot of pretty locations you can see. I like Minneapolis, I'd probably like Seattle and Portland too but I've never been there.
I don't know. I think I'm at a breaking point in my frustration with living in the States, and I need to decide next year if I want to make a call to leave the country or decide on a different state to live in. Someplace that's far less conservative than Iowa. I'm single, I could manage to make a major move change without it affecting anyone but me.
I'm feeling a bit tense. I just want to write code and calm the fuck down a couple levels.
Sorry if I've been pissy. :(18 -
# Retrospective as Backend engineer
Once upon a time, I was rejected by a startup who tries to snag me from another company that I was working with.
They are looking for Senior / Supervisor level backend engineer and my profile looks like a fit for them.
So they contacted me, arranged a technical test, system design test, and interview with their lead backend engineer who also happens to be co-founder of the startup.
## The Interview
As usual, they asked me what are my contribution to previous workplace.
I answered them with achievements that I think are the best for each company that I worked with, and how to technologically achieve them.
One of it includes designing and implementing a `CQRS+ES` system in the backend.
With complete capability of what I `brag` as `Time Machine` through replaying event.
## The Rejection
And of course I was rejected by the startup, maybe specifically by the co-founder. As I asked around on the reason of rejection from an insider.
They insisted I am a guy who overengineer thing that are not needed, by doing `CQRS+ES`, and only suitable for RND, non-production stuffs.
Nobody needs that kind of `Time Machine`.
## Ironically
After switching jobs (to another company), becoming fullstack developer, learning about react and redux.
I can reflect back on this past experience and say this:
The same company that says `CQRS+ES` is an over engineering, also uses `React+Redux`.
Never did they realize the concept behind `React+Redux` is very similar to `CQRS+ES`.
- Separation of concern
- CQRS: `Command` is separated from `Query`
- Redux: Side effect / `Action` in `Thunk` separated from the presentation
- Managing State of Application
- ES: Through sequence of `Event` produced by `Command`
- Redux: Through action data produced / dispatched by `Action`
- Replayability
- ES: Through replaying `Event` into the `Applier`
- Redux: Through replay `Action` which trigger dispatch to `Reducer`
---
The same company that says `CQRS` is an over engineering also uses `ElasticSearch+MySQL`.
Never did they realize they are separating `WRITE` database into `MySQL` as their `Single Source Of Truth`, and `READ` database into `ElasticSearch` is also inline with `CQRS` principle.
## Value as Backend Engineer
It's a sad days as Backend Engineer these days. At least in the country I live in.
Seems like being a backend engineer is often under-appreciated.
Company (or people) seems to think of backend engineer is the guy who ONLY makes `CRUD` API endpoint to database.
- I've heard from Fullstack engineer who comes from React background complains about Backend engineers have it easy by only doing CRUD without having to worry about application.
- The same guy fails when given task in Backend to make a simple round-robin ticketing system.
- I've seen company who only hires Fullstack engineer with strong Frontend experience, fails to have basic understanding of how SQL Transaction and Connection Pool works.
- I've seen company Fullstack engineer relies on ORM to do super complex query instead of writing proper SQL, and prefer to translate SQL into ORM query language.
- I've seen company Fullstack engineer with strong React background brags about Uncle Bob clean code but fail to know on how to do basic dependency injection.
- I've heard company who made webapp criticize my way of handling `session` through http secure cookie. Saying it's a bad practice and better to use local storage. Despite my argument of `secure` in the cookie and ability to control cookie via backend.18 -
Had a bad day at work :( They gave me this code for some obscure streaming job and asked me to complete it. Only after 3 days did I realize that the LLD given to me was incorrect as the data model was updated. Another 2 more days, I was able to debug the code and run it successfully— I was able to parse the tables and generate the required frame but not able to stream it back to the output topic as per the LLD. That’s where I needed help but none of my emails/messages were replied to. The main guy who is pretty technical scheduled a code review session with me— I expected that I would run the code and he would spot it something I might’ve missed and why my streaming function isn’t working. Instead, what happened was that he grilled me on each and every line of the code (which had some obscure tables queried) and then got super mad at me saying “Why are we having this code review session if your code is not complete?”. I’m like bruh, you asked for it, and yes, the main parsing logic is done and I’m just having this issue in the last part. And he’s like “Why didn’t you tell me earlier?”. Wtf?! I left at least 5 emails and a dozen messages. He’s like this has to go live on Monday, and I’m like Ok, I’ll work in the weekend. And he’s like “Don’t tell me all these things! You’re not doing me a favor by working on weekends! How am I to ask my colleagues to connect with you separately on Saturday/Sunday? You should have done the on the weekdays itself. What were you doing this whole week?”. Bruh, I was running the code multiple times and debugging it using print statements. All while you were ignoring my attempts to reach out to you. SMH 🤦♂️ I can go on and on about this whole saga.4
-
While it's totally not without its valid use cases, I fucking hate pair programming.
Well, let me elaborate. I hate *remote* pair programming. It completely disrupts my flow and wastes so much time with additional water cooler nonsense, and pedantic argument for the sake of participation. Not to mention "oh hey let me see how you did this... Oh, you know what, I think it would be better to do it this way...". Ok, great, we weren't even discussing that, but sure, let's completely detail this session to refactor something that could have come up at a good transition point, like I dunno, say a code review?
Like I said, there are very good reasons to pair program, but I would much prefer rubber ducking wherever possible.2 -
My dev lead is a uniquely poor leader with an impressive ability to produce a large amount inflexible, temporarily functional code.
As we're in another pair programming session where I try to keep him from destroying over all type safety and architectural decisions to meet a self imposed demo deadline, he keeps trying to access properties of his state.
This state object is incorrectly typed with an anonymous type with incorrect properties.
Despite repeating calmly stating that the object is incorrectly typed, and that's why there are red underlines when he tries to access a property he knows is in there, he insists that that's not correct.
Finally, he knowingly says that he's figured it out and that he's been doing this for many years.
What was the solution you might ask? (state as any).myProperty;
Truly breathtaking mastery. -
So I'm trying to get used to using vim and I've spent a couple of days setting up my vimrc and practising commands and what not.
Come today I'm doing my first proper coding session and my codes sending back weird errors and I can't work out why
Then when I read very carefully I find :w somewhere it's not supposed to be... Of course I'd forgetten to enter normal mode a ton of times and now my code is littered with :wq and :w so I spent a few minutes combing my code to find them all and it all works now.
Am I an elite hacker now?4 -
so, me and my best friend started playing pen and paper and after a wile we decided to create our own system. After a year of improving and testing we thaugth about a new java side project and more improvements for our system. time goes by and now we have three java apps for our own pen and paper and a lot of reusabilly code.
Playing and planing a new session of p&p is now so comfortable and fast 🤗 -
i was hired to join a team of old devs (40+) in an unnamed European country "yay goodbye 3rd world it's time to enjoy the quality of life" assist with enhancing already existing software and creating new solutions.
prior to my arrival most things were slow and super buggy, looking at the code base it shouldn't be a surprise, amateur hour everyone, logic implemented that is not needed, comment driven development, last time code review was done back in 1996. lots of anti patterns.
i swear there is a for loop that does nothing but it loops through a 100+ elements list, trunk based development with tfs since git is "not really needed"
test projects are not there.
>enter me an educated fool, with genuine passion for the craft and somehow a decent amount of knowledge.
>spent the last year fixing stuff educating people on principles and qualities.
> countless hours of training and explaining. team is showing cooperation, a new requirement comes in to develop with react.
> tear my ass creating reusable shit and self explanatory code with proper naming etc using git with feature branching, monday is first deployment day.
> today a colleague was working on an item submit a pull request and self approve it
> look at the code..... WTF the dumb fuck copied and pasted the whole code from different kendo components but somehow managed to refractor the name to test component, commented out all the code that he didn't use did the api call directly from the component, has 2 useeffects that depends on the a fucking text box changes for no reason, no redux implementation, the acceptance criteria is not achieved, and it doesn't work it just look right.
> first world country shit cannot scold, cannot complain, lead by example.
>asked him why you did this, the response was yeah probably i shouldn't have done that, i really didn't understand anything in the training but didn't want to waste time!!!!
> rest of the team created a different styled disaster with different flavors they don't even name their shit the same way.
fellow developers I'm stuck in a spaceship with a bunch of imposters, seriously i never cried in my entire life now I'm teary and on the verge of a break down.
talk with management "improving needs time" and offers me to join a yoga session to release the stress as if reaching nirvana would deliver shit on monday.
i really don't know what do is this a rant, is this a cry for help, I'm not sure, any advice is welcomed.7 -
I continue to internally read and study about Smalltalk in an effort to see where we might have FUCKED UP and went backwards in terms of software engineering since I do not believe that complex source code based languages are the solution.
So I have Pharo. Nothin to complex really, everything is an object, yet, you do have room for building DSL's inside of it over a simple object model with no issue, the system browser can be opened across multiple screens (morph windows inside of a smalltalk system) for which you can edit you code in composable blocks with no issues. Blocks being a particular part of the language (think Ruby in more modern features) give ample room for functional programming. Thus far we have FP and OO (the original mind you) styles out in the open for development.
Your main code can be executed and instantly ALTER the live environment of a program as it is running, if what you are trying to do is stupid it won't affect the live instance, live programming is ahead of its time, and impressive, considering how old Smalltalk is. GUI applications can be given headless (this is also old in terms of how this shit was first distributed) So I can go ahead and package the virtual machine with the entire application into a folder, and distribute it agains't an organization "but why!!!! that package is 80+ mbs!") yeah cuz it carries the entire virtual machine, but go ahead and give it to the Mac user, or the Linux user, it will run, natively once it is clicked.
Server side applications run in similar fashion to php, in terms of lifecycles of request and how session storage is handled, this to me is interesting, no additional runtimes, drop it on a server, configure it properly and off you go, but this is common on other languages so really not that much of a point.
BUT if over a network a user is using your application and you change it and send that change over the network then the the change is damn near instant and fault tolerant due to the nature of the language.
Honestly, I don't know what went wrong or why we are not bringing this shit to the masses, the language was built for fucking kids, it was the first "y'all too stupid to get it, so here is simple" engine and we still said "nah fuck it, unlimited file system based programs, horrible build engines and {}; all over the place"
I am now writing a large budget managing application in Pharo Smalltalk which I want to go ahead and put to test soon at my institution. I do not have any issues thus far, other than my documentation help is literally "read the source code of the package system" which is easy as shit since it is already included inside. My scripts are small, my class hierarchies cover on themselves AND testing is part of the system. I honestly see no faults other than "well....fuck you I like opening vim and editing 300000000 files"
And honestly that is fine, my questions are: why is a paradigm that fits procedural, functional and OBVIOUSLY OO while including an all encompassing IDE NOT more famous, SELECTION is fine and other languages are a better fit, but why is such environment not more famous?9 -
Spending 2 days trying to figure out why code signing wasn’t working when deployment is started from teamcity. Every time I tried manually it worked, but through teamcity it just kept telling me that no certificate could be found.
I finally twigged what the problem was, my code signing certificate is smart card based and you can’t access it if is requested from a RDP session. I had launched the teamcity build agent from an RDP session a few days back without thinking…
Rebooted and started the buildagent via VirtIO VNC and low and behold it started working again. -
Usually I come here to share rants/negativity but this time I wanna share an happy moment I had yesterday as a programmer.
In lots of instances I struggled to work on personal projects: I feel the desire to code cool stuff but I've often self-sabotaged myself by doing stuff like:
- self-enforcing "one man agile methodologies" with tasks, issue boards and lately time tracking
- forcing myself to do long study/research periods about the language/technologies I wanna use before writing the first line of code (and when I was able to actually end my research and get to code most of the stuff I researched was forgotten since cramming information is not effective on the long run)
- forcing myself to stick with all the "best practices" under the sun and to setup countless tools (linters, CI, unit testing...) before even getting a working POC
Usually all these stupid self imposed rules ended up in me procrastinating or pushing trough stuff struggling with headache after headache when coding actually used to feel a mostly fun pursuit to me.
Took lots of time to recognize this monster I created into my head but finally yesterday I did and I gave myself permission to:
- Start programming with just the very basics of the language (while reading a book on said language on the side at a relaxed pace, I can always come back later to improve my code as I learn more)
- Add stuff (unit testing, complex frameworks, CI/CD...) only when I need it
- Do a very basic planning (like a text files listing "must have" features and "nice to have features") and avoid issue boards and stuff, I'm working on a hobby project not on a company or a big OSS projects
It's been so long since the last time I had a programming session where I spent most time actually writing code and not researching and overthinking stuff and it felt great. -
everything microsoft makes is SHIT
for example, windows, and the fact that they bought out minecraft and ruined it with their bullshit "child friendly" feature
vscode's liveshare is ACTUALLY MAKING ME LOSE MY DAMN MIND TRYING TO WORK WITH SOMEONE
just now i was writing code and i realized, it wasn't even updating the file at all, it was just pretending to save changes, while on the hoster's end, nothing changed. at all
i had to rejoin the session 500 times, tell the host to restart, and it was generally a whole nightmare
nothing i typed in applied to the other end, and there were already 50+ errors from nothing in the php file i was working on
and it was erroring out at the most RANDOM spots, where nothing is wrong at all with the code, it was picking up something invalid, but NOT SHOWING ME WHAT WAS WRONG
i rejoined and saw a shit ton of test, an if that i never saw before i rejoined
in general why does everything microsoft make have to be so bad, and unreliable
smh microsoft u can do better2 -
So, we (I'm the backend guy and work with a UI dev) are building this product portfolio management tool for our client and they have a set of 250 users. The team has two point of contacts for the 250 users who maintain the master data, help users with data quality, tool guidance, reporting and other stuff. So one day one of these two support users come to me and say : Hey I'm not able to add new transactions coz a customer is missing.
We have the provision to create / maintain customers.
I check the production DB, application code, try creating the customer and then the transaction, everything works perfectly fine.
I ask the user for a screen sharing session, the user starts reproducing the error like this :
We have a 3 system landscape - Dev / Test and Prod
U : Logs into the test system url, creates the customer.
U : Points out the toast saying customer creation is successful.
U : opens a new tab, opens the production system, tries creating the transaction, searches for the customer and says " see !! cant find the customer here ! the master data management apps never work !! "
FML?. -
So just babbling my shit down here.
(Tldr : i am a crazy guy who followed my half slept brain, went onto a stage , gave some kind of motivating , stammering talk to a large group of professional strangers, enjoyed that day with a red embarrassed face and just got my first pic of me speaking on stage and that is so awesome !)
Last Saturday i went to a gdg meetup and i embarrassed the hell out of me.
I went there with just 2 hours of sleep from the previous night.
After a few talks there comes a guy who is taking some time to install is setup and the host calls for lightning round session ( ie he asks if anyone from the audience would like to share something about their product or something).
I am a fucking nutt guy. I can explain something to you nicely in a hacky way as long as i have done enough work on that and you speak my native language.
But giving a talk on English stage, hell no! I stammer, mix hindi with English and start speaking werd shit.. And that's what happened.
I don't know what went into me but as some guy went to the stage and talked for 2 mins, i was like yeah i want to do that too. So in next turn when he asked for a show of hands, i raised mine and fucking went to stage!
I forgot that if you go on stage you should have something to talk to . But the moment i was on stage, i was like... "Nope, we will do this differently".
I had been working on a video ads module from the last week which could be easily explained in 2 mins. But i felt like giving a non techy talk instead.
It went something like this: i introduced myself with my experience details ( who gives experience details on stage !?!) Then host said to speak loudly and i went like "Bharat mata ki jai!"( Victory to mother india (wtf!?😆) .
Then started talking about how the developers feel disheartened when searching on internet where the resources are scattered . And the solution i told them was :"don't be disheartened. You will eventually find it (like wow dude wtf, as if they didn't knew that) . Look on the youtube and other resources " and then went full on explaining/marketing about some online tutor who gives advice/consultancy via a subscription based payment ( tbf that guy really helped solve a lot of my doubts, he has written books on Android dev and is the top so answerer for Android).
Then i went on sharing my thoughts live on that fuckin stage ! ( Live because i usually post my thoughts here on devrant before discussing them out with real people, you guys are my safe space) but there i discussed my thoughts on libraries!
I have this believe that Android devs these days are having lesser knowledge of the system because we have all the libraries and templates available to us. But when we have to customize stuff, we need to go deep into docs and source classes and find ourselves in trouble there. So i kind of said this out loud and that we should try to read more the code and implement stuff ourselves instead of using the library 😅🙈)
I was feeling so fucking embarrassing after that all stuff! It was so full of stammering , broken English and worst attempt at motivation. At that time i was regretting this and about to burst cry and run away, but somehow i gathered my self, got my mood back to the event games and talks, later went to the organizers and apologized(and they were very nice and didn't cared about it), and overall enjoyed my weirdest day!
When i came home, my mom gave me a little more confidence about it. Now i think i shouldn't be that much instinctive. Next day i went hack to work and everything got normal.
But Yesterday i found a link to the public repository of the photos. Ohh fuck, someone had took my image! and that was too in full hd!!! 🙈🙈🙈😅😆😆 Oh mann I can't stop looking at that cool stage speaker image, i love it ! I, the shy-est and the most uncool awkward person , present on the stage with a mike, oof , i think i lived my dream !
I hope i could get enough confidence and speaking skills to take a real stage talk next time ( and maybe enough interesting talks and confidence to talk with girls of our office, ¯\_(ツ)_/¯ )5 -
I dug up my old ledger web app that I wrote when I was in my late twenties, as I realized with a tight budget toward the end of this year, I need to get a good view of future balances. The data was encrypted in gpg text files, but the site itself was unencrypted, with simple httpasswd auth. I dove into the code this week, and fixed a lot of crap that was all terrible practice, but all I knew when I wrote it in the mid-2000s. I grabbed a letsencrypt cert, and implemented cookies and session handling. I moved from the code opening and parsing a large gpg file to storing and retrieving all the data in a Redis backend, for a massive performance gain. Finally, I switched the UI from white to dark. It looks and works great, and most importantly, I have that future view that I needed.1
-
Whole day was a debug session with several hundred lines of code and tons of if else conditions.
Found the bug.
Time to go home.
I am done. 🤕6 -
When your up against a session issue and can't fucking work out why the session is lost, not one instance of the logout functionality is called and yet... the fucking thing decides to log you out anyway.
Now this was working all fine and dandy last week, and NOTHING has changed, as in not 1 fucking line of code for this process has been touched in 4 years.
It's like all of a sudden, Satan crawled out of this piece of shit site and decided I was to be toyed with.
As you can imagine, I'm a little pissed at this one, there's something hiding in the shadows fucking me in the ass.2 -
So, it's been a while since I've been working on my current project and I've never had the "luck" to touch the legacy project wrote in PHP, until this week when I got my first issue.
And damn, this goddamn issue. It was a bug, a very strange bug, that only happens in production and that nobody has any idea what was happening, so yeah, I didn't have anyone to ask and I got less time than usual ( because Thanksgiving ).
And thus, I have no starting point, no previous knowledge on PHP and less time! I expected a very fun week 😀 and it was beyond my expectations.
First I tried to understand what might be causing the issue, but there wasn't any real clue to star with, so no choice, time to read the flow on the code and see what are they're doing and using ( 1k line files, yay, legacy ). Luckily I got some clues, we're using a cookie and a php session variable for the session, ok, let's star with the session variable. Where it's that been initialize ? Well, spoiler alert, I shouldn't start with that, because my search end up in the login method of the API that set a that variable and for some reason in the front end app it was always false and that lead me to think that some of the new backend functions were failing, but after checking the logs I got no luck.
Ok, maybe the cookie it's the issue, I should try open the previous website on the brow...redirect to new project login, What? Why ? I ask around and it's a new feature push on Monday, ok I got Chrome Dev tools I can see which value of the cookie it's been set and THERE IT WAS it has a wrong domain! After 2 days ( I resume a lot of my pain ) I got what I've been looking for, so now I should be able to fix the bug. Then where is the cookie initialized ? In the first file the server hits whenever you tried to enter any page of the app, ok, I found the method, but it's using a function that process the domain and sets it correctly? wtf ? Then how in heaven do I get the incorrect domain ? Hello? Ok, relax, you still have one more day to fix this, let's take it easy.
Then, at the end of the Wednesday, nope I still have no clue how this is happening. I talked with the Devops guy and he explain me how this redirection happens and with what it depends on, I followed the PHP code through and nothing, everything should works fine, sigh. Ok I still have 2 days, because I'm not from US and I'm not in US, so I still have time, but the Sprint is messed up already, so whatever I'm gonna had done this bug anyhow.
Thursday ! I got sick, yay, what else could happen this week. Somehow I managed to work a little and star thinking in what external issue could affect the processing, maybe the redirection was bringing a wrong direction, let's talk with the Devops guy again, and he answer me that the redirection it was being made by PHP code, IN A FILE THAT DOESN'T EXIST IN THE REPOSITORY, amazing, it's just amazing. Then he explained me why this file might be missing and how it's the deployment of this app ( btw the Devops guy it's really cool and I will invite him a beer ) . After that I checked the file and I see a random session_star in the first line of the code, without any configuration, eureka ! There was the cause and I only need to ask someone If that line it's necessary anymore, but oh they're on holiday, damn, well I'll wait till Monday to ask them. But once and for all that bug was done for ! 🎉
What do I learn ? PHP and that I don't want any more tickets of PHP 😆. -
Yet another day at my company, Im rewriting some old code for client (rewriting old, php 4 system for vindications managment) and you know the moment when you are focused and someone comes to you to absolutely ruin your focus. Fine, whatever. Oh, for fuck sake. Again dev is doing as support becouse one moron with second can't login into zimbra admin panel and add fucking mailbox. I show them exacly how they login, remind them they are admins too, slowly show them, so you click "manage" than you click that gear icon and than you click "new", fill in email address and password. As simple as 1-2-3. Okay, fuck it, time to go for a cig. I just finish up few lines and stand, grab my vape and start walking towards door. In door I find my buddy with 2 random people. He told me that they are interns and that I should show them some basics and stuff around that. Oh god, fuck my life. If anything, Im definitely very bad teacher, mainly becouse I often have problems with saying what I mean in the way that somebody actually understans and knows what I am trying to say. Whatever. Fuck it all. I grab two of our old laptops that nobody used in like a year or so, and first thing I quickly figure out, is that one day for some what the fuck reason I dont even dont bothered to remember I installed Arch on both while I dont usually use Arch. I just needed it for some specific reason. Whatever. So I guess I will need to upgrade fucking system. Our network isn't really great so that was like... hour or so. In the meantime I figured what they know about coding in general etc, and holly shit. One of them (there was boy and girl), girl, apparently never ever in her life even touched code. Well... fuck. Why am I wasting my time? Becouse there was some programme or some shit like that... Someone could tell me before so I could mentally prepare.. fuck it. whatever. So while laptops are doing their pacman thing, I sit with them and slowly start to explain based on my machine some really basic concepts. Second guy actually had some expirience, he knew how to make some really really basic logic and stuff, so he had another world of problems, becouse it was PHP and, as we all know, everyone hates PHP, and... yeah.. You can probably imagine his approach. Yes, you get user input in super global array. I really wanted to say "Now shut the fuck up and write that fucking $_POST".
hour or so passed, I was close to giving up to not let my anger rise (im not really good teacher... I mentioned it. I suck at teaching others) but luckly machines upgraded. He wanted to use visual studio code, she didnt care too much, so I installed phpstorm in trial mode. whatever. Since that's linux and they were not comfortable with that, I walked them through installing LAMP stack, and when finally it started to look like LAMP stack, I requested them to google how to install xdebug, becouse xdebug is very usefull and googling skill is your best weapon on that field. I go for cig, come back and what I see boiled me a little bit. The girl was stuck looking at github page randomly looking through xdebug source code and idk... hoping for miracle (she admited she thought there will be instructions somewhere) and the guy was in good place, xdebug has a place to paste your phpinfo() for custom instructions. But it didn't work for him, he claims that wizzard told him it cant help him.. hmm intresting, you are sure you pasted in phpinfo? yes, he is sure. Okay, show me.
Again mindblown how someone can have problems with reading.
so his phpinfo() looked like that:
```<?php
phpinfo();```
I highlighted on the page the words "output of phpinfo". He somehow didn't see it or something. He didnt know, he thought that he needs to put in phpinfo so he did. OMG.
Finally, I figured out I can workaround my intern problem, and I just briefly shown them php.net, how documentation looks, said to allways google in english, if he uses tutorial to read whole fucking thing, not just some parts of it, and left them with simple task, that took them whole day and at which they ultimately failed.
To make 3 buttons labeled "1" "2" "3" and if someone presses one of them, remember in session that they pressed it and disallow pressing other ones.
Never fucking again interns. Especially those who randomly without apparent reason almost literally just spawn in front of you and here, its your fucking problem now.
Fuck it, I have some time to get back to my stuff. Time is running so lets not waste it.
After around 15 minutes my one of my superiors comes in and asks me if I can go on meeting with him and other superior. My buddy goes with us, and next 3 hours I was basically explaining that you cannot do some things (ie. know XYZ happened without any source of information) in code, and I can't listen for callbacks from ABC becouse it wont send anyc cuz in their fucking brilliant idea ABC can't even know that this script would even exist, not to mention it wants callbacks.
Sometimes I hate my job.4 -
Me at interview ->
Imagine the frustration you may go writing code in a different platform not having your vimrc. Than imagine the suicidal thoughts you have after you finish your program and have tabs mixed with spaces and indent errors, well on top of that imagine losing all the code highlighting all the code using X to yank it and P to paste in another file
Well, i shut down the vim session and lost all the code i wrote in 2 hrs3 -
Attended a game workshop session by Mark Skaggs, creator of Farmville. At one point he asked all the programmers to raise their hands. I love to code even though i aint an expert in backend db related code. I raised my hand. Then he asked all the designers to raise their hand. I love to graphic design as a hobby even though i aint an artist. I raised my hand again.
He noticed and said "Interesting..."
It made me question which trade i should focus more on. I still have years ahead to master them but should i give up one for the other?
Would be cool to hear your thoughts on this!4 -
v0.0005a (alpha)
- class support added to lua thanks to yonaba.
- rkUIs class created
- new panel class
- added drawing code for panel
- fixed bug where some sides of the UI's border were failing to drawing (line rendering quark)
v0.0014a (alpha) 11.30.2023 (~2 hours)
- successfully retrieving basic data from save folder, load text into lua from files
- added 'props' property to Entity class
- added a props table to control what gets serialized and what doesn't
- added a save() base method for instances (has to be overridden to be useful beyond the basics)
- moved the lume.serialize() call into the :save() method on the base entity class itself
- serialized and successfully saved an entities property table.
- fixed deserializion bugs involving wrong indexes (savedata[1] not savedata[2])
- moved deserialization from temp code, into line loading loop itself (assuming each item is on one line)
- deser'd test data, and init()'d new player Entity using the freshly-loaded data, and displayed the entity sprite
All in all not a bad session. Understanding filing handling and how to interact with the directory system was the biggest hurdle I was worried about for building my tools.
Next steps will be defining some basic UI elements (with overridable draw code), and then loading and initializing the UI from lua or json.
New projects can be set as subfolders folders in appdata, using 'Setidentity("appname/projectname") to keep things clean.
I'm not even dreading writing basic syntax highlighting!
Idea is to dogfood the whole process. UI is in-engine rendered just like you might see with godot, unity, or gamemaker, that way I have maximum flexibility to style it the way I want. I'm familiar enough with constructing from polygons, on top of stenciling, on top of nine-slicing, on top of existing tweening and special effects, that I can achieve exactly what I want.
Idea is to build a really well managed asset pipeline. Stencyl, as 'crappy' as it appeared, and 'for education' was a master class in how to do things the correct way, it was just horribly bloated while doing it.
Logical tilesets that you import, can rearrange through drag-n-drop, assign custom tile shapes to, physics materials, collisions groups, name, add tag data to, all in one editor? Yes please.
Every other 2D editor is basic-bitch, has you importing images, and at most generates different scales and does the slicing for you.
Code editor? Everything behavior was in a component, with custom fields. All your code goes into a list of events, which you can toggle on and off with a proper toggle button, so you can explicitly experiment, instead of commenting shit out (yes git is better, but we're talking solo amateurs here, they're not gonna be using git out the gate unless they already know what they're doing).
Components all have an image assignable to identify them, along with a description field, and they're arranged in a 2d grid for easy browsing, copying, modifying.
The physics shape editor, the animation editor, the map editor, all of it was so bare bones and yet had things others didn't.
I want that, except without the historic ties to flash, without the overhead of java, and with sexier fucking in-engine rendering of the UI and support for modding and in-engine custom tools.
Not really doing it for anyone except myself, and doubt I'll get very far, but since I dropped looking for easy solutions, I've just been powering through all the areas I don't understand and doing the work.
I rediscovered my love of programming after 3-4 years of learning to hate it, and things are looking up.2 -
Today was a rather funny day in school. School starts for me at 13:40 because our timetable planners are so qualified for this job.
First 2hrs: Physics, fine its good
Second 2hrs: Discrete Maths (however you want to call it)
Goal is to write a text (30 pages, 10, etc all those standard settings). Teacher prefers Latex over word, but we can do it in word if we want. We could choose a topic, I took primes because it looked the best. I decided to use latex because I'm a fetishist and it simply looks better in the end. A classmate was arguing with our teacher about ides: texmaker vs kile. And I'm like "I use vim". So my teacher is like kk
Later that class, when we actually started doing stuff I started the ssh session to my server because I don't know any good c++ compilers for win and I'm too lazy to get a portable version of cygwin (or whatever its called). So in my server I open vim and start coding my tool for Fermat Primes (Fermatsche Primzahlen, too lazy to actually translate). And this teacher seriously is the best teacher I ever met in my life. Usually teachers are like " dude r u hakin' the school server?" and I'm like bruh its just vim and I'm doing it this way because I cannot code on your PC coz I can't install a compiler. And this teacher is like "oh hey you actually use vi, all cool kids used it in 2000. I first though u were kidding and stuff..." And we continued talking about more of stuff like that and I have to say that this is the first teacher that actually understands me. Phew
Now I'm going to continue writing my 30 pages piece of trash latex doc and hope it'll end good1 -
How is it called when I rant about something my colleagues experienced and I just supported his debug session? Third party rant? Anyway, here's the thing.
Other team built some project specific code right in the heart of their software, which in itself would be worth a rant. But as you might guess, it's getting rantier.
For a new project the same code is used, but needed some tweaks. Now guess what happened:
1) Someone took the code, refactored it, made project specific stuff configurable, everything works fine now
2) Someone changed the code completely for the new project, everything seems to work fine for 5 months, then the old project needed a new release 💣 -
Dell Summer Internship Experience
Firstly,to be a part of this process it is important to clear the exam conducted by college and according to me it wasn't something which can't be easily achieved so to prepare of this exam stick to basics of all subjects which have been taught so far till semester majorily data structures,data base,Java,C, operating system were asked.Basics of all following subjects should be clear which also going to help during internship.I myself prepared for the test from geeksforgeek.I tried to gain as much as basic knowledge of subjects I can.And after selecting from test you have you go through hackathon on that personally I think one should be prepared with latest demanding skills.Mostly all the hackathon topics were in and around Machine Learning,Block chain,Web development,Databases.So typically should be aware of all these technologies and how this can be used to enhance in project.During hackathon days it is important to be interactive,it is good to clear doubts or explain your idea and how innovative you project is and how different it can be and further keep in mind how your project can be industrial utilized.Try to make your project more in aspect of how industry going to adapt this or how this problem's solution is perfect in every terms for a company.And majorily at last it comes down to how to present your project infront of your panel.I think keep that session as much as interactive you can,try to answer their queries,and most importantly know your part of the project very well on theoretical as well as on code level. At last you have to go through a HR interview in which firstly you have to be prepare with a nice resume in which you to include all your achievement's,projects and most importantly keep it short and simple and include only those things which you are completely aware of.For interview first try to know and learn about company, it's goals,in what field it is presently working and during interview there is nothing to worry about you just have to talk like you are talking with a normal person,express all your views ,try to speak out. Confidence is one important thing for this interview.So this was conclusion of my experience from hackathon hiring process from Dell.5 -
Everytime I have to work on some old Asp.Net shit. WebForms/WinForms etc.
Everything with that bullshitass designer. You wanna open a file you've just created? Sorry, error. Restart IDE and maybe...
Restarted website? Sorry. Old instance still hangs somewhere in IIS, so the port is taken...
Seeing code light up red when cleaning the project. Compiler being like "What the fuck is 'void'?"
Or - I know you didnt make any changes, but Im gonna build AppCode folder anyway... Its only gonna take a minute or two, no worries.
Or - You have XML template file to this class (codebehind)? You wanna open the XML? Would be shame if it was opened in the designer view and your entire IDE crashed 'cuz of some unsuported third party UI element.
Or - just unexpected debug session crashes.
And dont make me start on Xamarin...1 -
Hey ya'll back with another college boi question.
I want to develop a web server akin to that of jackbox/among us. Where each session has like an 'ABCXYZ' style code, and i assume are using TCP sockets on the back end.
I'll be writing in Go cause I <3 Go and its a chad language. Anywho, am i supposed to spin up a new websocket server each time someone wants to make a room? Or do i have one websocket server and some sort of map of rooms.
gameRooms := map['id_string']clients
Anyone have any suggestions for this?7 -
So I just got an insight from my PW session in SQLplus. I finally understood why the fridge did the SQL console under Oracle return the error:
ORA-00911: invalid character
on a line that seemed perfectly correct, but it's as if it spawned you an invalid character, just because.
But in fact, when I enter a character unintentionally like ç, then I remove it, it's as if the text prompt makes it look like it got removed, but there's still the cedilla, or at least its character code roaming around in the line I'm writing, and thus resulting in an error.
I'm not even salty now, that got me curious, and I think it's better like this, even if I'll require a bit of research on that thing later on.4 -
Working on a new release. This release was tested locally and pronounced good. The release went to the QA environment. QA responds that a new feature is doing nothing. There are no errors reported, and work is being done on the UI, but is not get getting persisted to the database so all changes are lost when the session is lost. Do some investigating. I find that a web service had the code in two of its methods commented out. Why? No idea. No response yet from the developer who just had the two methods return a boolean denoting success while all other operations were commented out.
I need an appropriate punishment for this...3 -
It goes back in college days were,I started developing on Visual Basic for a college project as it was the only option.
As the scope was limited to a standalone application,we we're not allowed to use network.
Building up on the that,the project was to be done in a group of two with SRS and other stuff needed to done.
With my partner having no knowledge about the code,I took my ideas and Incorporated it into my project such as system logs,session tracking,data records,barcode reader,export data in various formats and so on.
The project got large eventually and professor's were curious to see the development of my project.
The project got showcased as the best project by professors and that overall gained my popularity in college and got me a job offer which I rejected in the end -
I casually solved a problem in some private code at work today that I didn't manage to fix the previous two evenings.
Then I forget to push the changes and remembered at home that I turned off my work computer which normally I never turn off so that I could TeamViewer into it (I don't have VPN
access).
Wanted to work on that code this weekend 😒
(Well, we were in a "progession session" meeting right before leaving the office so I kind of forgot about it because of that) -
Why? As a senior, you won't give some time to review my code, will let me merge my code to a branch, then blame us when it will produce the bug in production? why? 😐 Won't even arrange a code review/knowledge sharing session so that juniors can learn at least something. Even you won't encourage us write test cases. If seniors don't follow, are the juniors to blame? 🙂3
-
Hey Sid,
Since I have no other way to contact you anymore, cool live coding session you did and code looks OK. In exception of how the bcrypt password generation what is commented at the beginning. I'm sure you didn't write it yourself, because if so, you should go to jail. It makes absolute NO SENSE. If you wrote yourself you would've find it out halfway writing :p
Maybe you should start using gpt, gpt would've produced better code regarding that :p
Nice singing. "To the staaaaars"20 -
So when writing golang code, you can put alias into the imported library
And one of interns in my office include include our company's library called "helper/session", and alias it with 'hSession'
Now all the code which use that library has to look like "hSession.ErrUnexpected", "hSession.NewSession"
Well, i think i've played too much VN and anime, but still i'm having a good laugh when doing the code review -
So i am working on a project based on Agile methodology but client wants to have a daily 4-5 hour code review session like he used to do in waterfall model. What should i do, how to say no diplomatically?8
-
I have an upcoming team meeting whereby we are going to ask the team on how they think we (as in the department) are doing? This can cover anything really from the way deliver code, interact or even just the wider context such as the office space we occupy.
I don’t want the session to be a free for all moan, so what techniques have other developers employed to elicit feedback?
In the past I’ve done a big piece of paper with sections:
What we do well
What we can improve
What we are poor at
We then asked people to write post it’s and attach them to the paper. These were gathered into broad themes and we then voted on as to which people felt most strongly about.
Just looking for suggestion of how I could make the session more successful. Thanks.1 -
Learned php all by myself, started with session to add stuff to an HTML web site a friend did. Took me a while to write hideous code, I had a chance to look at the code a couple years later.
-
1. Music, something fast paced with minimal to zero lyrics (usually a GOA radio station in my case)
2. No distractions around (use a "do-not-disturb" flag or something to hang on your monitor or show on your desk)
3. No chats or other communication/social media visible, best case those apps / tabs are completely closed or muted
4. Having a clear goal to achieve, might even be only a sub-goal for the current coding session.
5. Structure your code before your actually write it, I usually create step-by-step comments in each file, documenting my thought process and what steps the current file/class/whatever should do.
6. Try to code your stuff in the same order as the aforementioned comment step-by-step list dictates (unless there is a reason to change the coding order)
7. Only windows open: IDE/Editor, Browser
8. Also keep only the browser tabs needed for your work open (testing clients, documentation, music if using a browser client, etc.)
At least that's what works for me3 -
Being pretty much the only one who has some knowledge of how to code and get my way around tech (even if minimal, I'm too lazy for my own good) in my familiar household - and by extension, my family (Family extends FamiliarHousehold - LoL I'm sorry) - (my brother is on his first grade of a programming course in high school, I'm a 2nd grade uni student aiming to become a game dev) sometimes I wish I knew nothing of it.
Don't get me wrong, I do like working on code (if in Java. C is making me wanna tear my eyes out) but sometimes ignorant family members push me through the edge.
I worked on a business thing my family started this summer and one of the "jobs" was managing everything via a website.
Fair enough, I knew nothing of it when I started but I learn fast and just like that I knew my way around it. The problem came when I had to teach the person who started the project how it worked. This doesn't sound all that bad except he is kinda in the stone age regarding informatics.
He got a computer a few years ago and he pretty much only played poker in it, and he still had one of those old nokias you could throw to a wall and get a hole into it. The computer is like 9y and runs like crap.
To make things worse he bought a new phone, a smartphone, and pestered me to teach him. I swear trying to teach him is like repeating the same thing 1000x and pray he keeps it in his head. Spoiler: he doesn't. ( sanity--; )
So to try and easy my suffering I decided to make a manual for the website (which is outdated by now because the team behind the website did a 180 and some things looks different), but it acted as if I'd done nothing. ( sanity--; )
To top this off he keeps on saying I don't wanna help him. ( sanity--; )
This kept going for the whole damn summer, and meanwhile I had to go back to uni and in the first days I still got like 4-5 calls/day, half of those might about the smallest things because he's so panicky.
Like (both examples happened while I was still there but it kinda goes along those lines sometimes):
- (During the period they changed the website the first time since we're there; they were mostly doing changes back and forth and testing because it had a new layout for a day or 2 before going back; also the site was totally functional, except for a thing or 2)
Him: "They're changing the website, why are they doing that?"
Me: "Because it's their website and they can?"
Him: "WHY DIDN'T THEY LET US KNOW"
Me: "They don't have to, they don't work for you." ( sanity--; )
Or (during the same period; the pages have a menu on the left; one of the submenus has a counter that resets every time the session ends; during that maintenance time they must've "disabled" the function because the number kept growing even after the session ended):
Him: "WHY IS THE NUMBER GROWING?"
Me: "They're working on the code, relax, it's nothing."
Him: "But why." ( sanity--; )
The only quesion he pretty much hasn't asked me yet is why "Is the website's colour this one and not that one?".2 -
"The Perils and Triumphs of Debugging: A Developer's Odyssey"
You know you're in for an adventurous coding session when you decide to dive headfirst into debugging. It's like setting sail on the tumultuous seas of code, not quite sure if you'll end up on the shores of success or stranded on the island of endless errors.
As a developer, I often find myself in this perilous predicament, armed with my trusty text editor and a cup of coffee, ready to conquer the bugs lurking in the shadows. The first line of code looks innocent enough, but little did I know that it was the calm before the storm.
The journey begins with that one cryptic error message that might as well be written in an ancient, forgotten language. It's a puzzle, a riddle, and a test of patience all rolled into one. You read it, re-read it, and then call over your colleague, hoping they possess the magical incantation to decipher it. Alas, they're just as clueless.
With each debugging attempt, you explore uncharted territories of your codebase, and every line feels like a step into the abyss. You question your life choices and wonder why you didn't become a chef instead. But then, as you unravel one issue, two more pop up like hydra heads. The sense of despair is palpable.
But, my fellow developers, there's a silver lining in this chaotic journey. The moment when you finally squash that bug is an unparalleled triumph. It's the victory music after a challenging boss fight, the "Eureka!" moment that echoes through the office, and the affirmation that, yes, you can tame this unruly beast we call code.
So, the next time you find yourself knee-deep in debugging hell, remember that you're not alone. We've all been there, and we've all emerged stronger, wiser, and maybe just a little crazier. Debugging is our odyssey, and every error is a dragon to be slain. Embrace the chaos, and may your code be ever bug-free!1 -
Dell Summer Internship Experience
Firstly,to be a part of this process it is important to clear the exam conducted by college and according to me it wasn't something which can't be easily achieved so to prepare of this exam stick to basics of all subjects which have been taught so far till semester majorily data structures,data base,Java,C, operating system were asked.Basics of all following subjects should be clear which also going to help during internship.
I myself prepared for the test from geeksforgeek.I tried to gain as much as basic knowledge of subjects I can.And after selecting from test you have you go through hackathon on that personally I think one should be prepared with latest demanding skills.Mostly all the hackathon topics were in and around Machine Learning,Block chain,Web development,Databases.So typically should be aware of all these technologies and how this can be used to enhance in project.
During hackathon days it is important to be interactive,it is good to clear doubts or explain your idea and how innovative you project is and how different it can be and further keep in mind how your project can be industrial utilized.Try to make your project more in aspect of how industry going to adapt this or how this problem's solution is perfect in every terms for a company.And majorily at last it comes down to how to present your project infront of your panel.
I think keep that session as much as interactive you can,try to answer their queries,and most importantly know your part of the project very well on theoretical as well as on code level. At last you have to go through a HR interview in which firstly you have to be prepare with a nice resume in which you to include all your achievement's,projects and most importantly keep it short and simple and include only those things which you are completely aware of.For interview first try to know and learn about company, it's goals,in what field it is presently working and during interview there is nothing to worry about you just have to talk like you are talking with a normal person,express all your views ,try to speak out.
Confidence is one important thing for this interview.So this was conclusion of my experience from hackathon hiring process from Dell.2 -
So this product already has session replay, feedback collection widgets with multiple integrations to jira, slack, GitHub etc.... this widget embed code is already getting large and I am beginning to get worried
New problem, owner wants to add some error reporting tool. this would have been easy if I was to just collect and group errors from the users browsers but here is the use case
user tries to submit a form, out of probably lord know number of function and api calls, one fails, the user may be stuck or not, we don't know but I am supposed to infer from the session recording data whether the user got stuck or not, create a nice PM understandable error message like "user was unable to update their profile", group this error message over the number of affected users/visitors and attach the affected error messages/data to this generated group and also the session recording.
where am I even supposed to start with this?, can I trust AI to do the heavy lifting?5 -
Does anybody teach mobile development live? For example as code is being written they are providing audible commentary that is also captioned that is also downloadable after session is closed. A cool feature would be to have a q and a live and it picks up the commentary and/or question from the audience too, I think I just thought of another sound mobile app idea!5
-
Let's do a story mapping session! Ok cool. PO asks the team: so guys what do you think? *silence*... *more silence*.... PO: come on guys, please respond. *silence*.... Then someone finally responds.
I'm starting to hate this big time. It's almost always like that, no matter the type of session (story mapping, refinement) And there's someone in the team that thinks he always knows best, so if ever someone speaks up, it will always be challenged and lead to useless discussions. He always wants the perfect solution. A good solution is good enough, it doesn't have to be perfect. PO is happy with a good solution (good = maintainable, scoring at least x on our code quality tooling), so why the fuck would you want to go for the 'perfect' solution, which may score just slightly higher in regard to quality, cost much more to develop and people have a hard time maintaining it due to the high level of abstraction? He's always refactoring stuff because it's not future proof. Well, why completely reimplement parts that have been working properly for 2 years and have a very very small chance of needing a change, which then still only needs to be done in just 1 place?
And you know what? All these fancy structures, patterns etc are in there but will their flexibility ever really be used? In my 20 years experience haven't seen such flexibility being really used. Some exceptions of course.
Once it's built, it will keep running, yes, changes will need to be made, but in most cases they never touch all these expensive fancy structured components. Just because most changes are in content or small changes in functionality.1 -
I wrote my first proper promise today
I'm building a State-driven, ajax fed Order/Invoice creation UI which Sales Reps use to place purchases for customers over the phone. The backend is a mutated PHP OSCommerce catalog which I've been making strides in refactoring towards OOP/eliminating spahgetti code and the need for a massive bootstrapper file which includes a ton of nonsense (I started by isolating the session and several crucial classes dealing with currency, language and the cart)
I'm using raw JS and jquery with copious reorganization.
I like state driven design, so I write all my data objects as classes using a base class with a simple attribute setter, and then extend the class and define it's attributes as an array which is passed to the parent setter in the construct.
I have also populateFromJson method in the parent class which allows me to match the attribute names to database fields in the backend which returns via ajax.
I achieve the state tracking by placing these objects into an array which underscore.js Observe watches, and that triggers methods to update the DOM or other objects.
Sure, I could do this in react but
1) It's in an admin area where the sales reps using it have to use edge/chrome/Firefox
2) I'm still climbing the react learning curve, so I can rapid prototype in jquery faster instead of getting hung up on something I don't understand
3) said admin area already uses jquery anyway
4) I like a challenge
Implementing promises is quickly turning messy jquery ajax calls into neat organized promise based operations that fit into my state tracking paradigm, so all jquery is responsible for is user interaction events.
The big flaw I want to address is that I'm still making html elements as JS strings to generate inputs/fields into the pseudo-forms.
Can anyone point me in the direction of a library or practice that allows me to generate Dom elements in a template-style manner.4 -
Hi friends!
I'm I'll since Sunday and my imagination is starting to fade. So far I've read/watched movies on deep learning and CNN, had a long session on Wikipedia on common fallacies, as well as a lot of other things. But now I can't think of what to do.
What is your favourite go to thing when you need to kill time using nothing but eyes and ears?
I'm too tired to code or play games...3 -
in agile methodology, have a daily session of 3 hours code review in which he will understand my code.. 👿
-
I did see two posts about vibe coding.
While it's a bit hyped and not that convenient yet, I actually vibe coded two applications in Rust / C# using my own vibe coding tool. My vibe coding tool is very convenient since it's a terminal app you can talk to that will read, write and execute on winning systems (Linux). You don't have to copy or paste source code as with other vibe coders.
This project url, binaries and source: https://molodetz.nl/retoor/r. Use free version if you do not have openai key. If you do, use commercial version and configure OPENAI_API_KEY in your env.
It's written in C because I'm crazy.
Since it's written in C it's maybe not convenient as educational resource but it does contain all information you need to let AI control your pc / filesystem and instruction following.
There's also a python module of that software. You can do rpylib.chat("user", "prompt") with it and it'll be a session. I prompted yesterday 150(!!!!)mb with it by batching using python.
The r project supports context (instruction file) that makes it a real local agent. If you're creative, sky is the limit with this tool. I use it all the time. I copied to /usr/local/bin and if I need gpt, I just type r(f) in my terminal or directoy "r [the prompt no quotes needed]". Last example exits the application after result of prompt. If you say "Hey, grade the quality of my script.py from 1-10" it will do that. The options are endless. Ultimate automation tool.9 -
I love how my boss wants to use libraries for everything, even the most minor task needed he looks up for a library to do it, I think that sometimes he even spends more time looking for a library than the time he would spend writing the functionality
e.g. Roles for routes permissions, dude, you have the users role in session you can just write a middleware with ~15-20 lines of code to get them rid of the route come on -
part 5/n
me vs my job at mnc laggards
Do these laggards even know how virtual meetings work?
meeting 1: an induction meeting from 10am-1pm . one day ago, the supposed manager of me called to say that he will be in office after 10.30, so i should come after that. so ofcourse i missed the call from 10-11.30 as i was commuting. one would expect the meeting to be recorded but nope :/ .Also, the 1 hour session that i somewhat attended consisted of an old guy telling how he has been doing these inductions for last 10 years in this company and how company takes its code of conduct with utmost sincerity (wtf?? tell me the employee benefits you dinosaurs 🤬)
meeting 2 : a meeting describing the softwares, from 2.30-3.30 . no fucking person is leading the meeting and 10+ people are just sitting their with their mics off!!!
also sidenote: microsoft teams and outlook can go suck its own dick if it has one. one of the shittiest piece of shit i have ever worked with. People find them so complex that they instead have unofficial whatsapp groups for official communication.
And guess what : YOU CAN'T FUCKING CREATE THEIR ACCOUNTS IN ANYTHING BUT LAPTOP!!
And when whatsapp comes into the fucking work life, you can expect an RIP to the P of my life 😭😭
-----
previous rants:
https://devrant.com/rants/6543145/...2 -
Lesson learned, never use Vim's 'd' to move a large chunk of code from file to file. Need to move a large chunk of Rust code from one file to another, when I quit the session and edit the file needs to be pasted, the entire content, disappeared, divided into atoms.
Edit: I found that the 'd' command means 'delete command', so that may explain why. But still.2 -
So, I really tried .. again ... to use intellij. And i simply really don't get it. Why do so many devs like it? For me it feels like swimming in the dark not knowing if my java code will actually build because there is no fucking actual build feedback provided in real time.
I can build the whole project and get a build log, a fucking text log! I want my eclipse problems view, that auto-updates with erronious code as I type ... as I FUCKING TYPE!
Ok so there are various "hacks" to enable auto-build, even while having a running debug session, (in the registry ..., remind me of old windows days *sigh*).
And still, all looks good and I start the program an baaammm, compile time errors on start What the actual fuck?
Also why the heck does it allow to setup/move/resize the panels when i resets them every fucking time I restart intellij???
The UI is so cluttered and illogical, like the debugging view that has three tool/tabbars on it's own, on various hierarchies, even a vertical one. It alls looks so ... in a lack of a better word I would say "hingspieben" [austrian for "puked out"]
The only real nice thing is the "settings sync" to github. Everything else is mediocre or even really really bad.
So intellij users, please tell me, what do you guys really like about it, that is so good that no other IDE has is?9 -
I recently started working on laravel. As the community says it was easy to get along with the framework and its methodologies. But then i had to do multiple login with framework in same domain.
Oh man, i spent a week to make it work. All those guards and middlewares realted to login was driving me crazy. The concept was clear, but somehow the framework was like "You! I shall make you spend a week for my satisfaction". The project demo was nearing and i was doing all kind of stuff i found. Atlast after continous tries it worked. Never in my 4+ years as a developer i had to face such an issue with login.
So here is how it works,if anyone faces the same issue:
(This case is beneficial if you're using table structures different from default laravel auth table structures)
1. Define the guards for each in auth.php
Eg:
'users' => [
'driver' => 'session',
'provider' => 'users',
],
'client' => [
'driver' => 'session',
'provider' => 'client',
],
'admin' => [
'driver' => 'session',
'provider' => 'admins',
],
2. Define providers for each guards in auth.php
'users' => [
'driver' => 'eloquent',
'model' => <Model Namespace>::class,
'table' => '<table name>', //Optional. You can define it in the model also
],
'admins' => [
'driver' => 'eloquent',
'model' => <Model Namespace>::class,
],
'client' => [
'driver' => 'eloquent',
'model' => <Model Namespace>::class,
],
Similarly you can define passwords for resetting passwords in auth.php
3. Edit login controller in app/Http/Controller/Auth folder accordingly
a. Usually this particular line of code is used for authentication
Auth::guard('<guard name>')->attempt(['email' => $request->email, 'password' => $request->password]);
b. If above mentioned method doesn't work, You can directly login using login method
EG:
$user = <model namespace>::where([
'username' => $request->username,
'password' => md5($request->password),
])->first();
Auth::guard('<guard name>')->login($user);
4. If you're using custom build table to store user details, then you should adjust the model for that particular table accordingly. NOTE: The model extends Authenticatable
EG
class <model name> extends Authenticatable
{
use Notifiable;
protected $table = "<table name>";
protected $guard = '<guard name>';
protected $fillable = [
'name' , 'username' , 'email' , 'password'
];
protected $hidden = [
'password' ,
];
//Below changes are optional, according to your need
public $timestamps = false;
const CREATED_AT = 'created_time';
const UPDATED_AT = 'updated_time';
//To get your custom id field, in this case username
public function getId()
{
return $this->username;
}
}
5. Create login views according to the user types you required
6. Update the RedirectIfAuthenticated middleware for auth redirections after login
7. Make sure to not use the default laravel Auth routes. This may cause some inconsistancy in workflow
The laravel version which i worked on and the solution is for is Laravel 6.x1 -
Anyone wanna see me code?
https://codesandbox.io/live/8r6zr1t
ya just open the link and watch me code there is a chat avallible to speak in and idk how to run my code , feel free to add stuff or whatever just a live session forever? idk but ya see ya9 -
I hate with a passion Code Review sessions in Pull Requests. It takes days to get approved especially when you have as Lead tech someone with 3 years of experience that rejects PR because doesn't like how I named the variable and also if that person is different time zone.
In my previous company we used to have 30 minutes session if we had PRs and we would go over it.3 -
Slept for 4 hours after 10 hours programming session and I still feel pretty good.
Does that mean that code == rest? Woops!1 -
They asked me to build a small website they will embed in a native application with some web wrapper in Android and iOS.
But also asked me to build a login web service that will return a JWT. Done.
They want to do a native code login form that opens up the web wrapper with my small website already logged in using the login web service.
I have no idea how to proceed in the backend.
At first i tried using postman with a POST request to the sessions/sign_in route and sending a form with the authenticity token and the email and password; but CSRF stopped me. I don't want to turn it off because of reasons.
Now i am wondering how to use this JWT to generate a cookie with a session inside it that they can use in the web wrapper.
Any help would be appreciated :)4 -
Every time I sit to code I just put up The Weeknd 's "Save Your Tears". At least that gives me hope to save my tears for the next code session.
save your tears for another day(of coding)…. -
Please give me code snippet to create windows ec2 instance using boto3 within aws free tier limit.
import boto3
# Create an AWS session and EC2 client
aws_management_console = boto3.session.Session(profile_name='....')
ec2_console = aws_management_console.client(service_name='ec2')
def create_ec2_instance():
try:
print("Creating EC2 instance")
ec2_console.run_instances(
ImageId="....",
MinCount=1,
MaxCount=1,
InstanceType="t3.micro",
KeyName="...",
SecurityGroupIds=['...1'] # Specify your security group ID(s) as a list
)
except Exception as e:
print(e)
# Call the function to create the EC2 instance
create_ec2_instance()
Have i missed anything in this code?
It's running fine not creating any instance.2